The `pinv` function in MATLAB computes the pseudoinverse of a matrix, which is particularly useful for solving linear systems that may not have a unique solution.
Here's an example of using `pinv`:
A = [1, 2; 3, 4]; % Define a 2x2 matrix
B = pinv(A); % Compute the pseudoinverse of matrix A
What is MATLAB `pinv`?
The `pinv` function in MATLAB computes the pseudo-inverse of a matrix. The pseudo-inverse is a generalized inverse that can be applied to non-square or singular matrices, making it a vital tool in various mathematical and engineering applications. It allows solving linear equations, particularly in least squares problems, where traditional inversion techniques may falter due to non-invertibility.
Why Use `pinv`?
Using `pinv` becomes essential in situations where a matrix either does not have an inverse or is not square. Its benefits include:
- Solving overdetermined systems: When there are more equations than unknowns.
- Finding least squares solutions: Giving the "best fit" to a set of data points, even when the data is not perfectly linear.
- Handling singular or ill-conditioned matrices: The pseudo-inverse provides a stable solution where direct inversion fails.
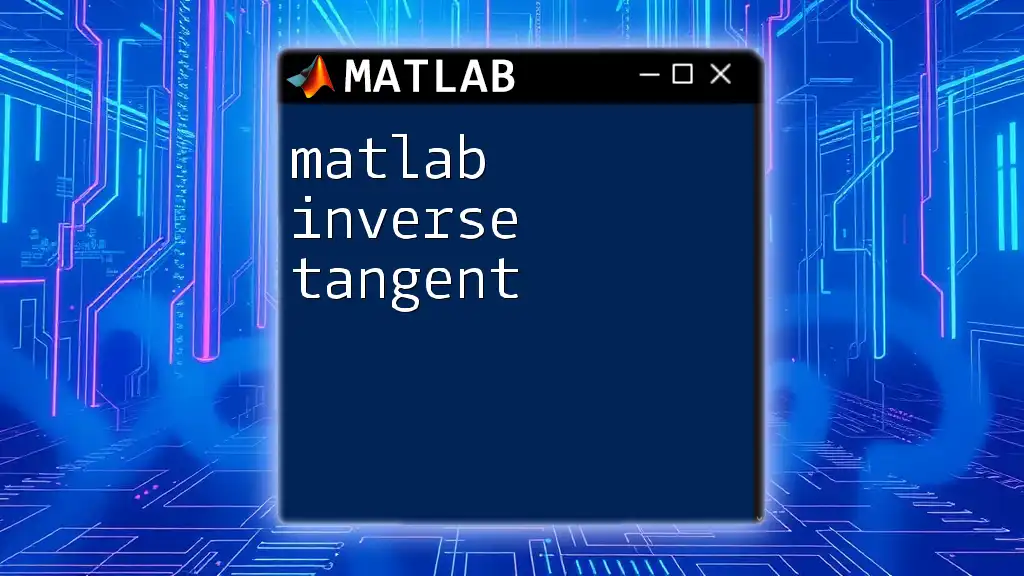
Understanding Matrices and Inverses
What is a Matrix?
In linear algebra, a matrix is a rectangular array of numbers arranged in rows and columns. Matrices come in various types, such as:
- Square Matrices: Equal number of rows and columns.
- Rectangular Matrices: Different number of rows and columns.
Regular Inverse vs. Pseudo-Inverse
Regular Inverse
A regular inverse exists only for square matrices that are non-singular, meaning they have full rank. If a square matrix \( A \) has an inverse \( A^{-1} \), the following holds:
\[ AA^{-1} = A^{-1}A = I \]
where \( I \) is the identity matrix. For example, the matrix
A = [1 2; 3 4]
has an inverse.
On the other hand, a matrix is considered non-invertible or singular if its determinant is zero. This can occur in various applications, making the regular inverse inadequate.
Pseudo-Inverse
The pseudo-inverse \( A^+ \) of a matrix \( A \) extends the concept of matrix inversion. It can be calculated even if \( A \) is not square or is singular. Mathematically, the pseudo-inverse satisfies the following conditions:
-
For a matrix \( A \) of size \( m \times n \), \[ AA^+A = A \]
-
It minimizes the residual sum of squares, providing the best-fit solution for systems of linear equations.
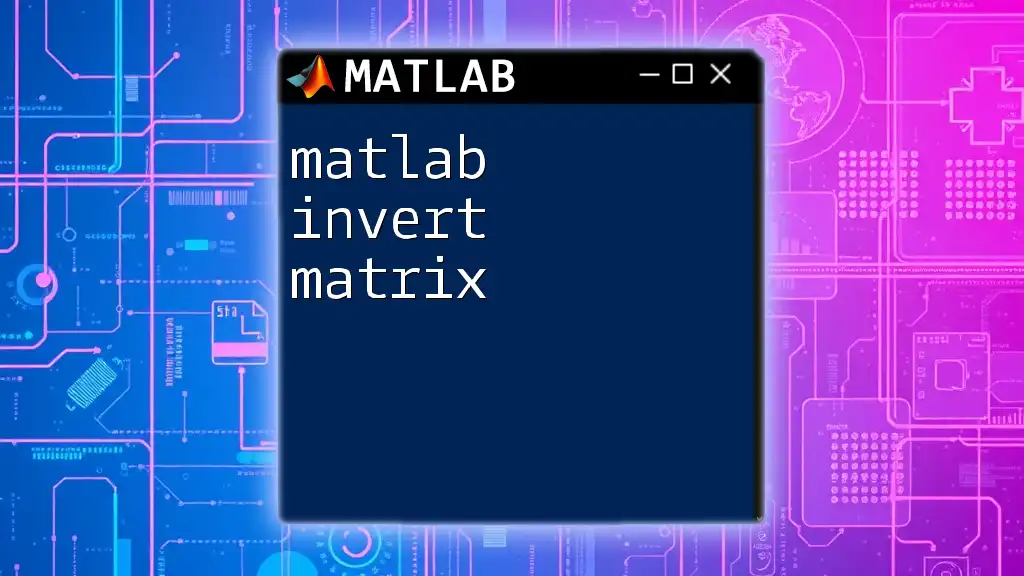
How to Use `pinv` in MATLAB
Syntax of `pinv`
The syntax for using `pinv` in MATLAB is straightforward:
B = pinv(A)
Here, `A` is the input matrix, and `B` is its pseudo-inverse.
Example Usage
Basic Example
For a simple case, consider a 2x2 matrix:
A = [1 2; 3 4];
B = pinv(A);
disp(B);
When you run this code, you get the pseudo-inverse of matrix `A`. The output will be:
-2.0000 1.0000
1.5000 -0.5000
This result shows how `pinv` finds a matrix `B` that satisfies the condition \( AB = I \) as closely as possible.
Larger Matrices Example
Another example with a 3x2 matrix demonstrates `pinv` effectively:
A = [1 2; 3 4; 5 6];
B = pinv(A);
disp(B);
This code outputs the pseudo-inverse of a matrix with more rows than columns, which is a common scenario in data fitting tasks.
Applications of `pinv`
Solving Linear Systems
One of the powerful applications of MATLAB `pinv` is solving the linear system \( Ax = b \). If we have:
A = [1 2; 3 4];
b = [5; 6];
We can solve for \( x \) as follows:
x = pinv(A) * b;
disp(x);
In this case, `x` will provide the least squares solution, which would minimize the error in the equation, especially useful when the system is overdetermined (more equations than unknowns).
Data Fitting and Regression Analysis
The `pinv` function is frequently used in regression analysis to fit a model to empirical data. For instance, if you have a set of data points and want to find the best-fitting line \( y = mx + c \), you can set up a design matrix and use `pinv` to yield coefficients.
X = [1 1; 1 2; 1 3]; % Design matrix
y = [1; 2; 3]; % Observations
beta = pinv(X) * y;
disp(beta);
This will provide the coefficients for the model, allowing you to draw meaningful conclusions from the data.
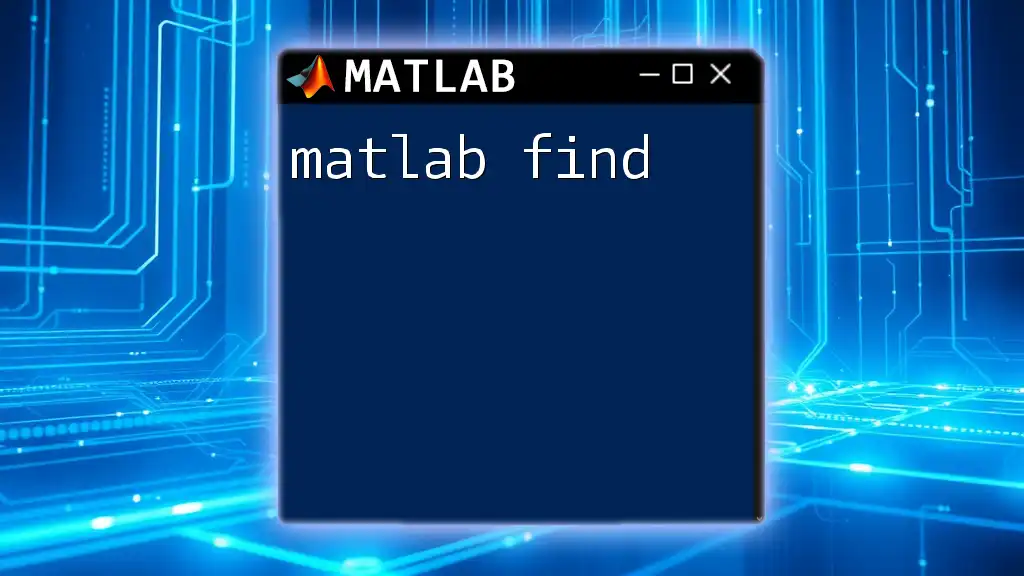
Advanced Topics
Numerical Stability and Performance
While `pinv` is robust, it can run into numerical stability issues in specific scenarios, particularly with large or ill-conditioned matrices. In such cases, alternative methods like QR decomposition may provide improved results. For poor-quality data or matrices with very small singular values, using `pinv` requires careful consideration of the input matrix properties.
Using `pinv` with Complex Numbers
The `pinv` function can also handle complex numbers in matrices. For example:
A = [1+2i 3; 4 5+6i];
B = pinv(A);
disp(B);
This reflects how `pinv` operates consistently, providing pseudo-inverses even with complex entries, extending its utility in engineering and physics applications.
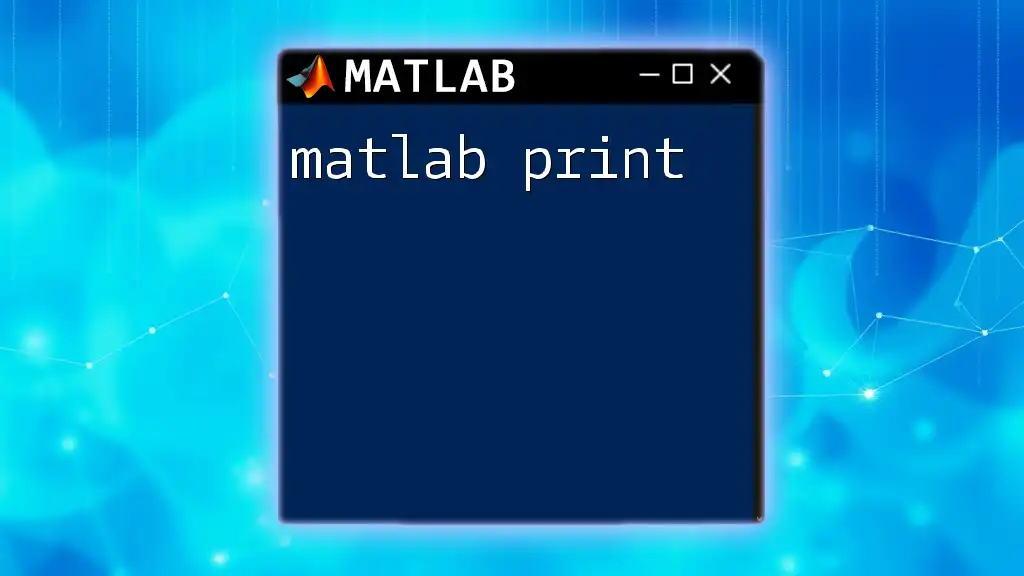
Common Errors and Troubleshooting
Common Issues
Users may encounter issues such as the following:
- Inconsistent solutions when using `pinv` on poorly conditioned matrices.
- Unexpected outputs when applying `pinv` on large datasets with extreme values.
Solutions
To mitigate these issues, check:
- The rank of your matrix using the `rank` function to ensure you have full rank before applying related methods.
- The residuals after solving to validate the solution's accuracy.
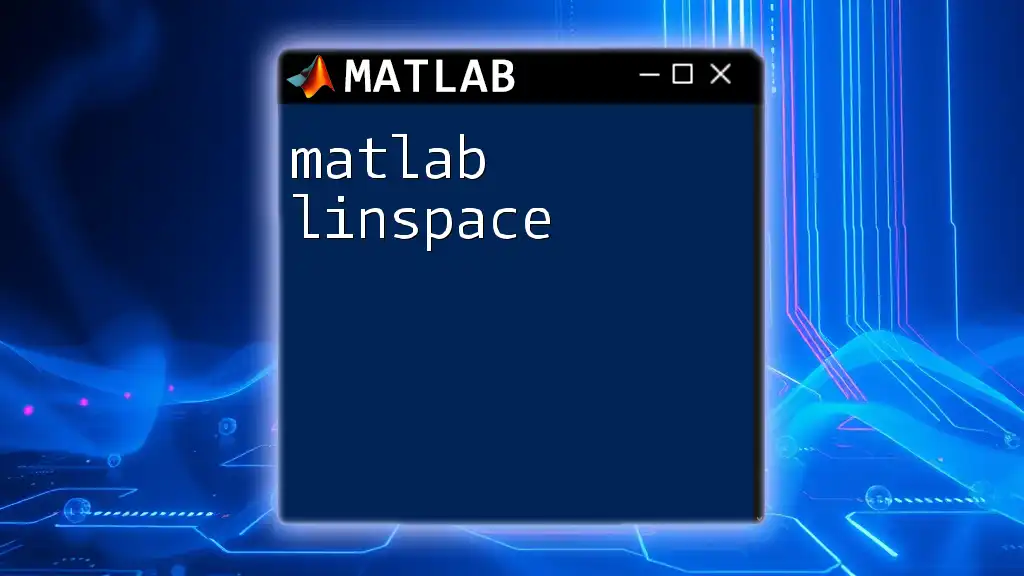
Conclusion
In summary, MATLAB `pinv` is a powerful function for computing the pseudo-inverse of matrices, helping to solve linear systems and perform regression analysis effectively. Understanding its functionality can greatly enhance your numerical computations, especially in cases involving non-square or singular matrices.
Further Resources
Consider exploring additional MATLAB documentation and resources related to matrix operations, numerical linear algebra, and regression modeling for a more in-depth understanding.
Call to Action
Try implementing `pinv` in your MATLAB environment with your matrices to see firsthand how it can aid in problem-solving. Engage with the material, practice, and soon you’ll become proficient in leveraging this essential command.
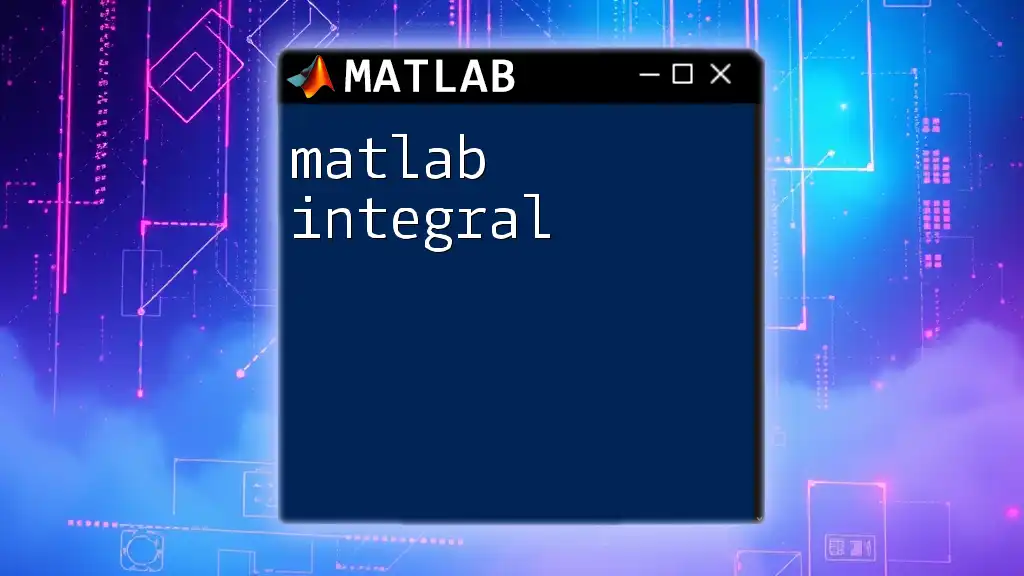
Frequently Asked Questions (FAQs)
What is the difference between `inv` and `pinv` in MATLAB?
`inv` is applicable only to square, non-singular matrices while `pinv` can be used for any matrix, regardless of its shape or rank.
Can `pinv` be used for non-square matrices?
Yes, `pinv` is particularly designed to handle non-square matrices, providing a best-fit solution even when a matrix cannot be inverted directly.
How does `pinv` handle singular matrices?
When a matrix is singular, `pinv` computes a pseudo-inverse that provides a least squares solution, typically minimizing the error for linear equations related to the singular matrix.