In MATLAB, the constant `pi` represents the mathematical constant π (pi), which is the ratio of a circle's circumference to its diameter and can be used directly in calculations involving circles or angles.
Here's a code snippet demonstrating how to use `pi` in MATLAB:
% Calculate the circumference of a circle with a radius of 5
radius = 5;
circumference = 2 * pi * radius;
disp(['Circumference of the circle: ' num2str(circumference)]);
Understanding pi in MATLAB
What is Pi?
Pi (π) is a mathematical constant that represents the ratio of a circle's circumference to its diameter. Its importance transcends mere geometry, appearing frequently in various areas of mathematics, physics, engineering, and beyond. When working with MATLAB, it is essential to utilize the constant π accurately to ensure precision in computations.
MATLAB's Representation of Pi
In MATLAB, pi is represented as a built-in constant. You can access the value through the identifier `pi`, which provides a high level of precision, avoiding the pitfalls of floating-point arithmetic that come from manually entering an approximation, such as 3.14 or 22/7. Utilizing the built-in constant is beneficial as it ensures that your results are mathematically valid and as accurate as possible.
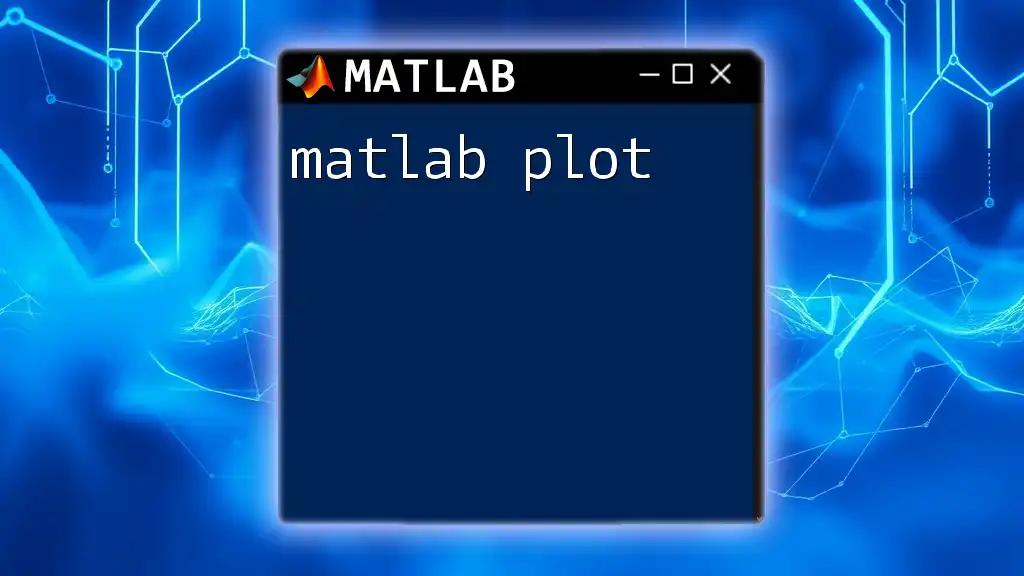
Using pi in MATLAB
Basic Mathematical Operations with pi
MATLAB allows you to perform a variety of operations using the pi constant, making it easier to incorporate essential mathematics directly into your calculations.
- Addition and Subtraction: Here’s how to add or subtract from pi:
result1 = pi + 1; % Adding 1 to pi
- Multiplication and Division: You can also multiply and divide by pi easily:
result2 = 2 * pi; % Doubling pi
result3 = pi / 2; % Half of pi
After performing operations, you can display the results using:
disp(result1);
disp(result2);
disp(result3);
Pi in Trigonometric Functions
Trigonometric functions are particularly important when working with angles in radians, where pi plays a crucial role.
- Using pi with sin, cos, tan Functions: To illustrate how to work with trigonometric functions, consider the angle theta defined as 45 degrees (which is π/4 radians):
theta = pi/4; % 45 degrees
sin_value = sin(theta);
cos_value = cos(theta);
tan_value = tan(theta);
- Visualizing Trigonometric Functions Involving pi: You can also visualize how these functions behave with respect to pi by plotting them:
x = 0:0.1:2*pi;
y1 = sin(x);
y2 = cos(x);
plot(x, y1, x, y2);
legend('sin(x)', 'cos(x)');
title('Sine and Cosine Functions');
xlabel('x (radians)');
ylabel('Function values');
This plot helps you appreciate the periodic nature of these functions, highlighting their relationship with π effectively.
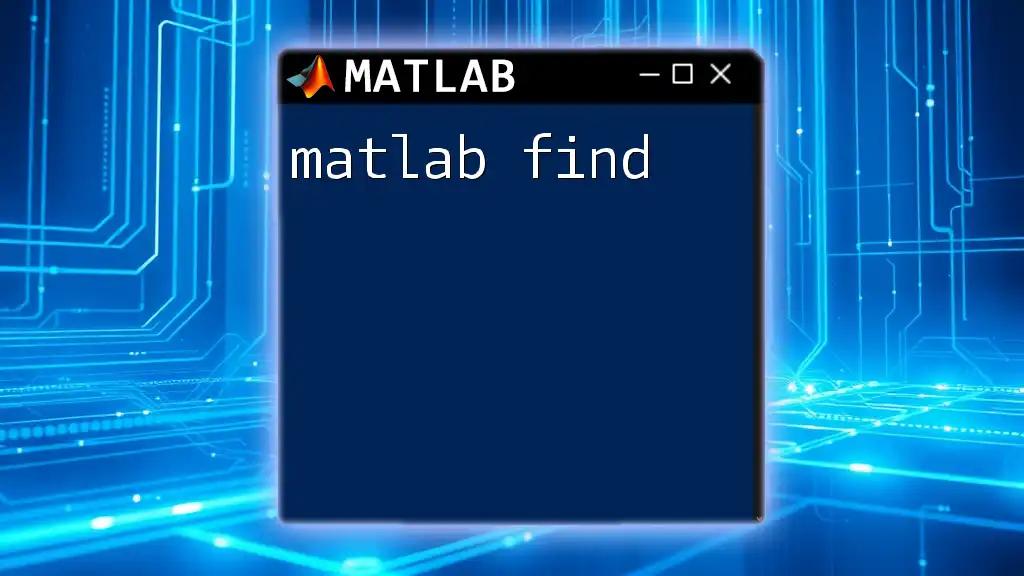
Applications of Pi in MATLAB
Geometry and Area Calculations
Pi is vital in various geometric calculations, especially when dealing with circles.
- Circle Area Calculation: The formula for the area of a circle is given by A = πr². Utilizing MATLAB, you can compute this effortlessly:
radius = 5;
area = pi * radius^2;
- Example with Different Radii: To enhance this further, you can create a function that calculates the area for any given radius:
function area = circle_area(radius)
area = pi * radius^2;
end
This demonstrates the flexibility of using pi in practical applications.
Fourier Transformations
Fourier transforms are powerful tools for analyzing frequency components in signals, and they often involve pi in their computations.
For instance, to analyze a simple sine wave using Fourier transformation:
t = 0:0.001:1; % Time vector
x = sin(2*pi*50*t); % 50 Hz sine wave
Y = fft(x); % Fourier Transform
In this example, pi plays a crucial role in defining the frequency of the sine wave effectively.
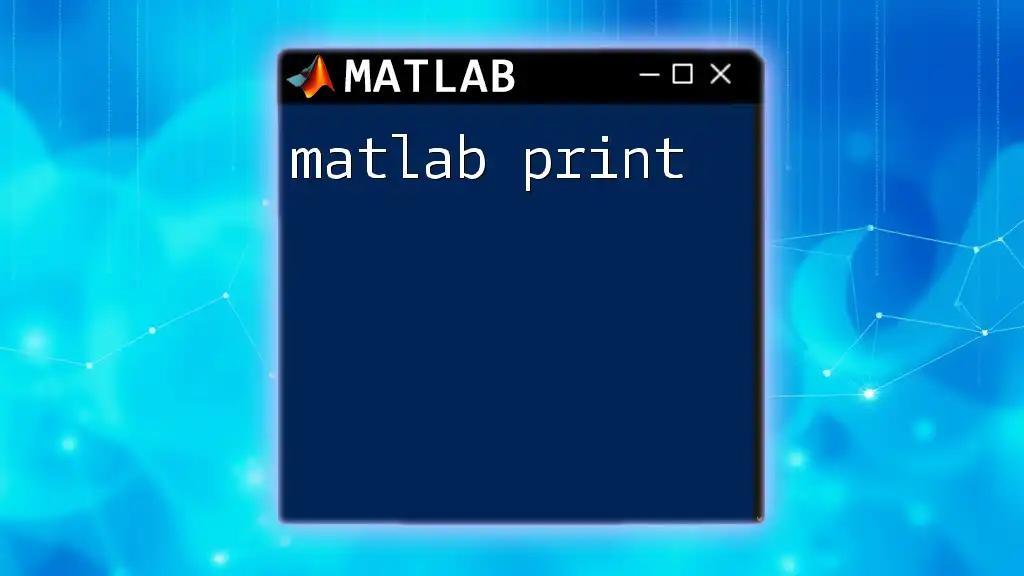
Best Practices When Using Pi in MATLAB
Importance of Precision
Using MATLAB's built-in `pi` is critically important. By relying on this constant, you avoid the risks associated with numerical approximations that can lead to significant errors in calculations. By leveraging precision, you ensure that the outcomes of your computations are both reliable and accurate.
Performance Optimization Tips
To optimize computational performance, consider storing results involving pi in variables rather than recalculating it multiple times. For example:
myPi = pi; % Store pi once for better performance
result = myPi * radius^2;
This small change can enhance performance significantly, especially in larger computations.
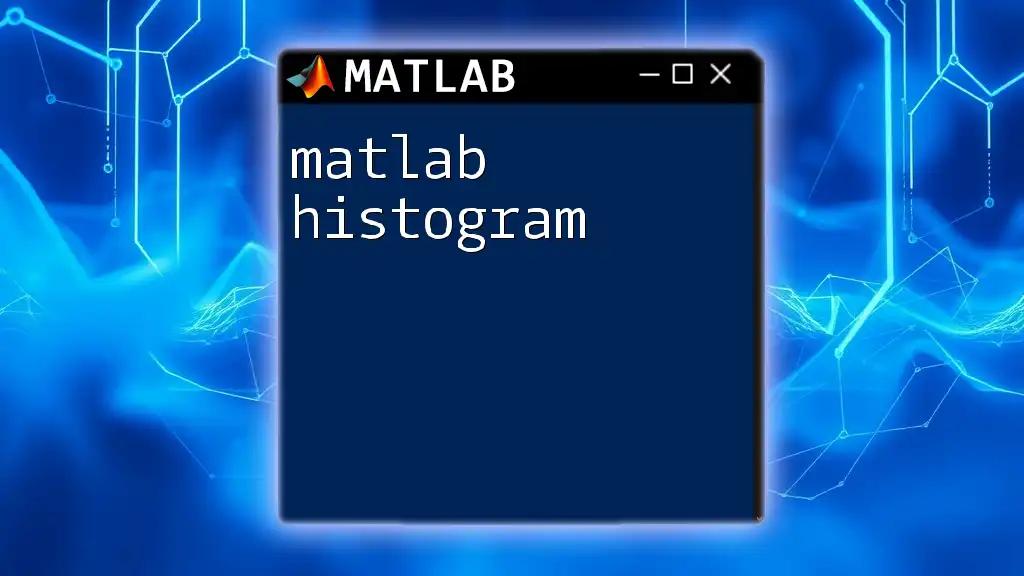
Conclusion
In summary, understanding and utilizing MATLAB pi is vital for accurate mathematical modeling and computations. Whether you're dealing with simple arithmetic or complex transformations, using the built-in constant for pi can dramatically enhance the precision and reliability of your results. Practice with the provided examples to master your skills and explore the many applications of pi in engineering and science.

Additional Resources
For continued learning, refer to MATLAB’s official documentation and explore recommended books and online courses that provide deeper insights into MATLAB programming.
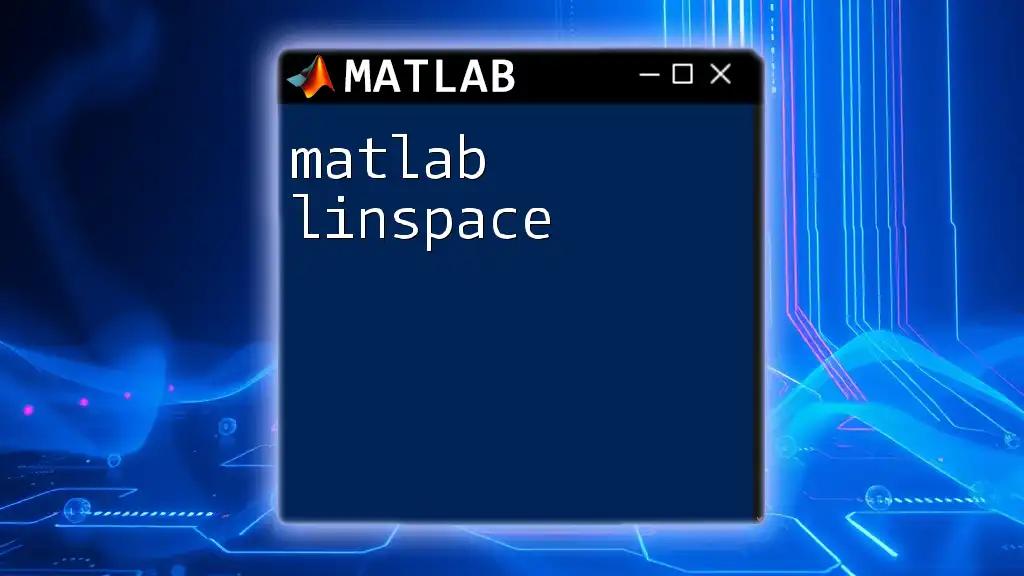
Call to Action
Ready to take your MATLAB skills to the next level? Sign up to receive additional tutorials and updates tailored to enhance your learning experience. Don’t forget to share this guide with classmates or colleagues who are interested in improving their MATLAB skills!