`fmincon` is a MATLAB function used for solving constrained nonlinear optimization problems.
Here's a simple example of its usage:
% Define the objective function
objective = @(x) (x(1)-3)^2 + (x(2)-2)^2;
% Define the constraints
A = []; b = []; Aeq = []; beq = [];
lb = [0, 0]; ub = [5, 5]; % Lower and upper bounds
% Initial guess
x0 = [0, 0];
% Call fmincon
[x, fval] = fmincon(objective, x0, A, b, Aeq, beq, lb, ub);
What is `fmincon`?
`fmincon` is a powerful MATLAB function used for solving constrained nonlinear optimization problems. This function is invaluable in various fields, including engineering design, finance, and machine learning, as it allows users to minimize a scalar objective function subject to constraints.
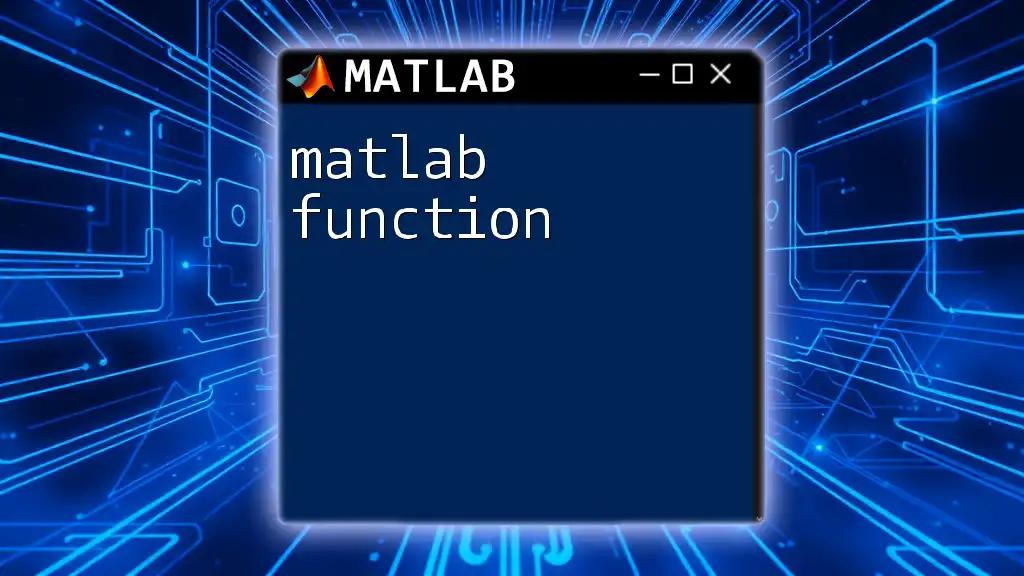
When to Use `fmincon`?
You might consider using `fmincon` specifically when dealing with optimization problems that have constraints—those that require not just finding a minimum value, but also ensuring that specific conditions are met. For example, in engineering, you might need to minimize the weight of a structure while keeping it strong enough to support a specific load.
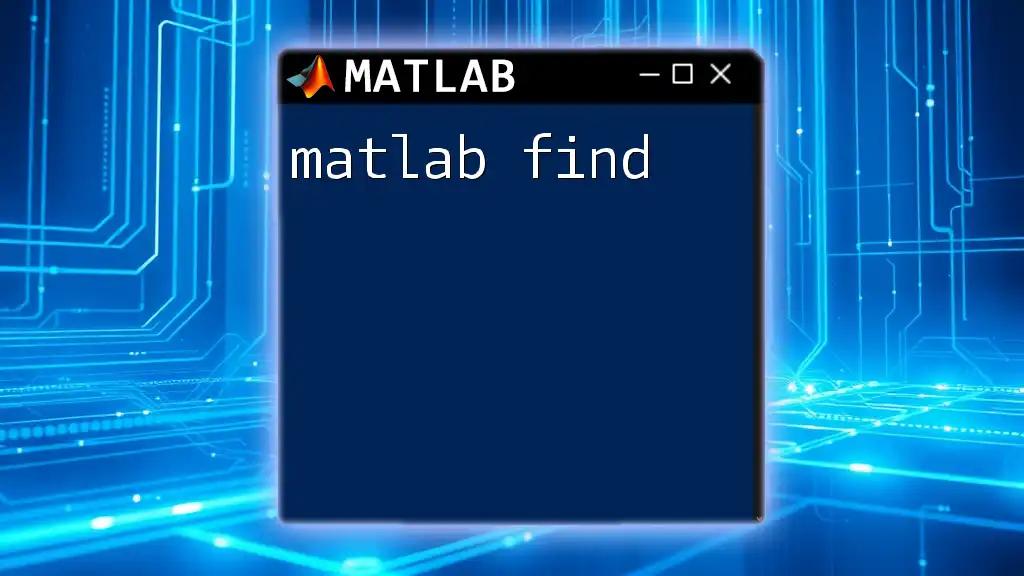
Understanding Optimization Problems
Overview of Optimization
Optimization refers to the process of making something as effective or functional as possible. In computational terms, it often means minimizing or maximizing a function by changing its variable inputs. It's a fundamental aspect of many disciplines, from economics to operations research.
Types of Optimization Problems
Optimization problems can broadly fall into two categories:
- Unconstrained Optimization: Problems that do not require satisfying any constraints. For instance, minimizing a simple quadratic function.
- Constrained Optimization: This includes equality or inequality constraints. For example, minimizing costs while adhering to budgetary limits.
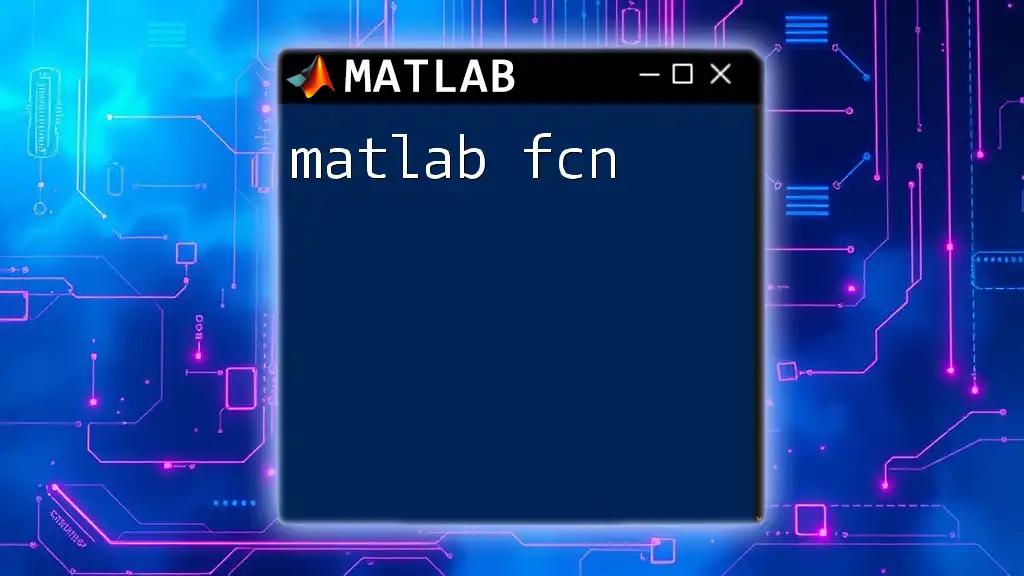
Setting Up the Optimization Problem
Defining the Objective Function
Before using `fmincon`, you must define an objective function—the function you want to minimize. The objective function is crucial; it characterizes what you're aiming to achieve through optimization.
Here's a simple code snippet to create a quadratic objective function:
function cost = objectiveFunction(x)
cost = x(1)^2 + x(2)^2;
end
Identifying Constraints
Constraints can be classified into:
- Equality Constraints: Conditions like \( h(x) = 0 \), which must be exactly satisfied.
- Inequality Constraints: Conditions such as \( g(x) \leq 0 \) or \( g(x) \geq 0 \), which must hold true.
MATLAB provides functions to encapsulate these constraints effectively.
Building Initial Guesses
Setting a good initial guess is vital for the `fmincon` algorithm to converge effectively because optimization results can be highly dependent on where the search begins. An initial guess might look like this:
x0 = [0, 0]; % Initial guess
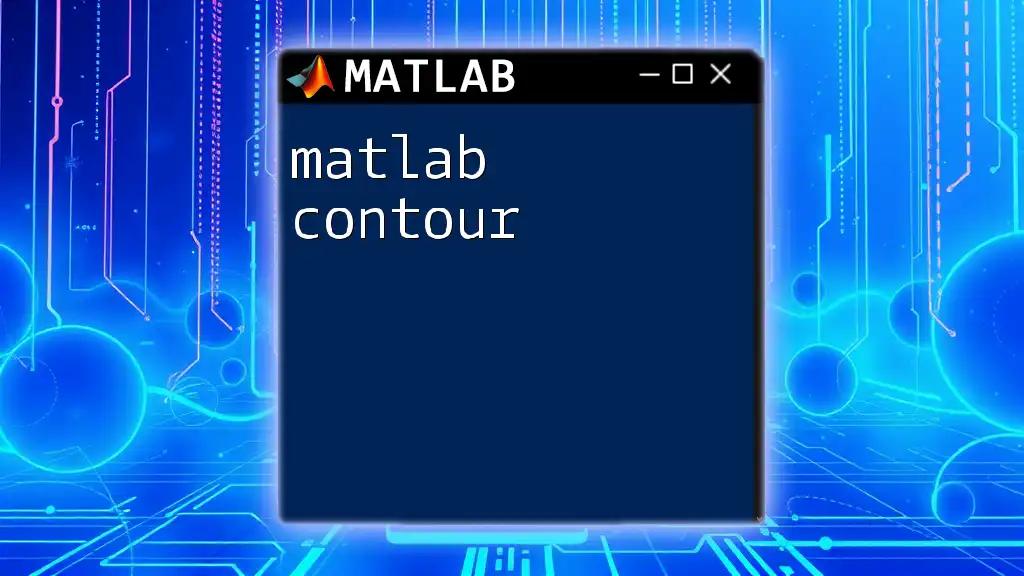
Using `fmincon` in MATLAB
Basic Syntax of `fmincon`
The basic syntax for the `fmincon` function involves specifying the objective function, initial guess, and constraints. Here’s a simplified overview of the typical command format:
[xOpt, fOpt] = fmincon(fun, x0, A, b, Aeq, beq, lb, ub, nonlcon, options)
Step-by-Step Implementation
Step 1: Define the problem. This involves setting your objective function, constraints, and initial guess.
% Objective function
objective = @(x) objectiveFunction(x);
% Linear constraints
A = []; b = [];
Aeq = []; beq = [];
% Bounds
lb = []; ub = [];
Step 2: Call `fmincon`. Utilize options to control iterations and display outputs, ensuring you get a clear picture of the algorithm's progress.
options = optimoptions('fmincon', 'Display', 'iter');
[xOpt, fOpt] = fmincon(objective, x0, A, b, Aeq, beq, lb, ub, [], options);
Step 3: Analyze the output. The `xOpt` variable will contain the optimal values of your variables, while `fOpt` gives you the minimum value of the objective function.
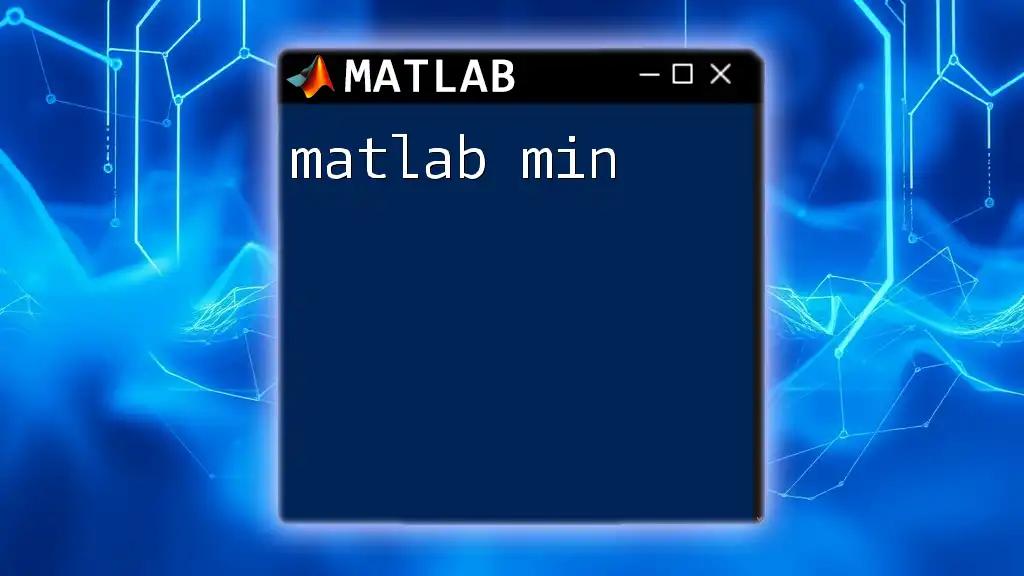
Advanced Features of `fmincon`
Setting Options
Customizing your optimization options can significantly impact performance. For instance, you might choose to increase the maximum number of iterations or adjust tolerances for convergence:
options = optimoptions('fmincon', 'MaxIterations', 1000, 'StepTolerance', 1e-6);
Handling Different Solver Algorithms
`fmincon` offers various algorithms, including:
- Interior-point: Suitable for large-scale problems.
- Active-set: Useful for smaller problems with a mix of linear and nonlinear constraints.
- Sequential Quadratic Programming (SQP): Good for problems with smooth nonlinear functions.
Choosing the right algorithm can improve speed and solution accuracy.
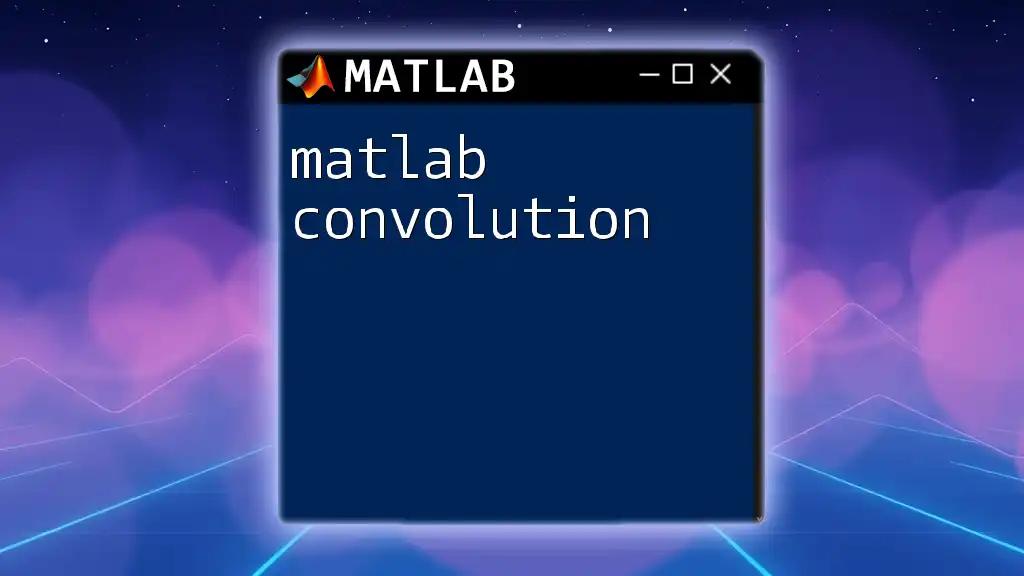
Practical Examples of `fmincon` Usage
Example 1: Simple Constrained Optimization Problem
Consider a scenario where you want to minimize a cost function subject to constraints on the variables:
% Objective function
function cost = objectiveFunction(x)
cost = (x(1) - 1)^2 + (x(2) - 2.5)^2;
end
% Constraints
function [c, ceq] = constraints(x)
c = [x(1)^2 + x(2)^2 - 2]; % inequality constraint
ceq = []; % no equality constraints
end
x0 = [0, 0]; % Initial guess
[xOpt, fOpt] = fmincon(@objectiveFunction, x0, [], [], [], [], [], [], @constraints);
Example 2: Multi-variable Optimization with Constraints
In a more complex case, you might find it necessary to optimize multiple variables with both equality and inequality constraints. Here’s a breakdown of how to do that:
% Objective function
objective = @(x) x(1)^2 + x(2)^2 + x(3)^2;
% Constraints
function [c, ceq] = multiConstraints(x)
c = [x(1) + x(2) + x(3) - 5]; % Linear inequality
ceq = [x(1)^2 + x(2)^2 - 1]; % Nonlinear equality
end
x0 = [0, 0, 0];
[xOpt, fOpt] = fmincon(objective, x0, [], [], [], [], [], [], @multiConstraints);
Example 3: Real-world Application Case Study
You might consider using `fmincon` in financial modeling, for instance, to optimize a portfolio's returns subject to risk constraints. By implementing similar steps as outlined above, one can define the return function, the risk measure as constraints, and find an optimal asset allocation strategy.
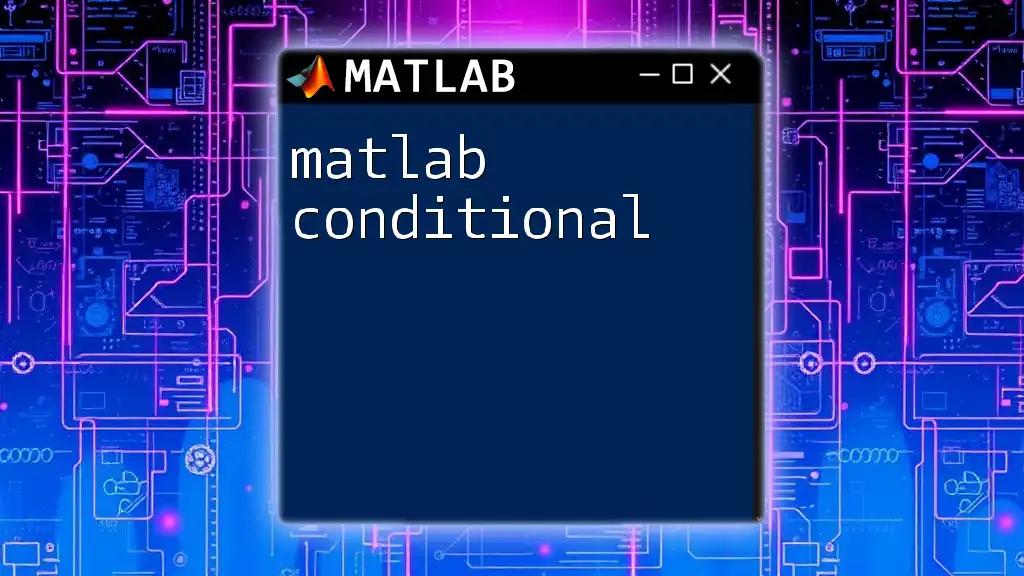
Common Issues and Troubleshooting
Error Handling Tips
When using `fmincon`, errors can often arise from improperly formatted inputs. Always ensure that your constraints are in the correct form and that the dimensions of your vectors match.
Improving Performance
If you encounter slow convergence or excessive iterations, consider checking the scale of your problem. Normalizing the data can often lead to quicker convergence. Adjusting the `MaxIterations` and tolerance levels can also facilitate performance improvements.
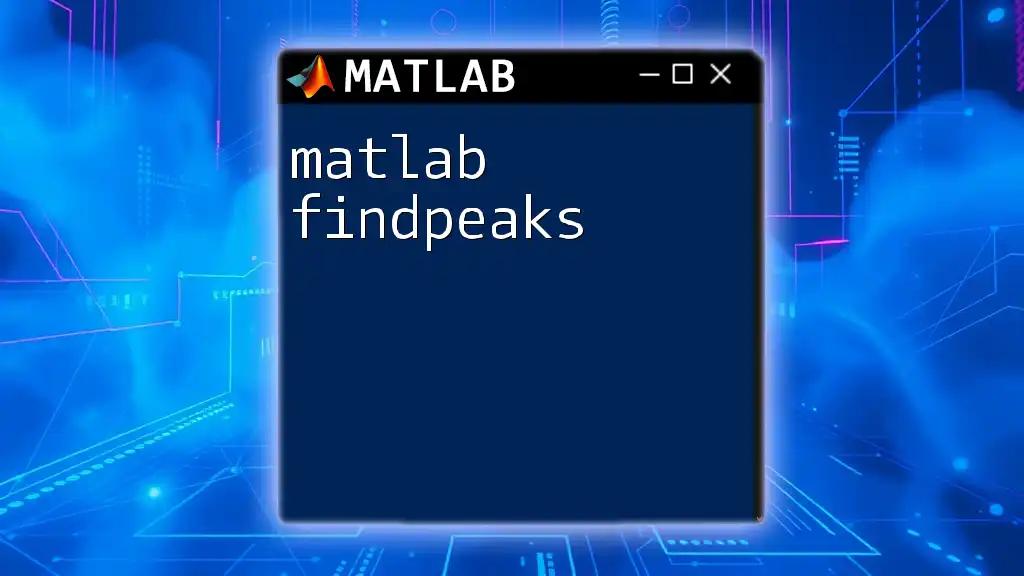
Conclusion
In summary, `fmincon` is an indispensable tool in MATLAB for solving constrained optimization problems. Through careful definition of your objective function, constraints, and proper implementation of options, you can effectively navigate complex optimization scenarios.
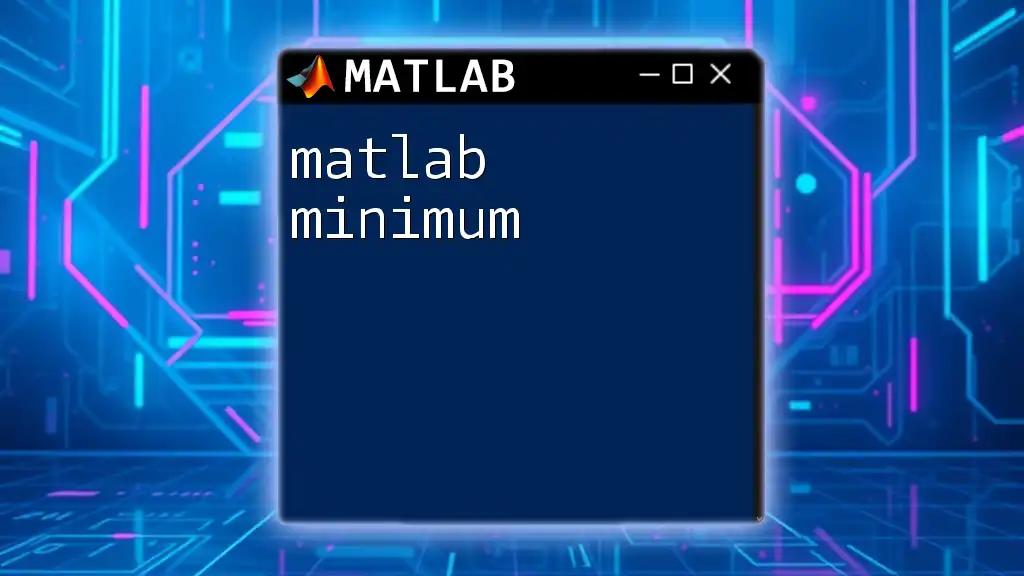
References and Further Reading
For those looking to dive deeper into `fmincon` and optimization in MATLAB, check the official MATLAB documentation and consider supplementary resources like textbooks and online courses that focus on optimization techniques.
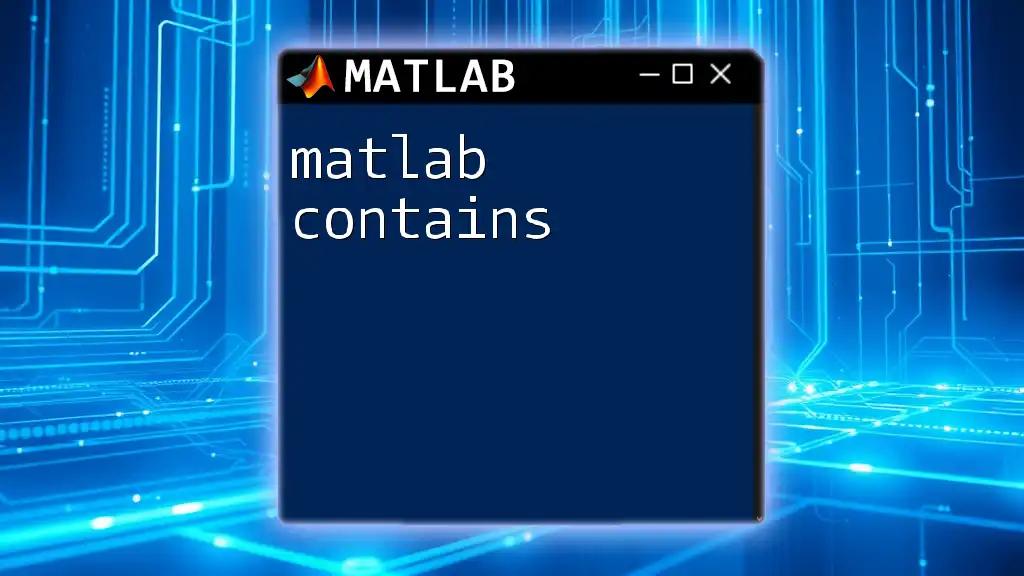
Call to Action
Join our learning community to stay updated with the latest tutorials and insights on MATLAB. Share your experiences and challenges with `fmincon` in the comments section below—let's learn together!