The `eval` function in MATLAB executes a string containing MATLAB expressions, allowing for dynamic command evaluation at runtime.
result = eval('3 + 5'); % This will evaluate the expression and assign the result (8) to the variable 'result'
Understanding `eval`
What is `eval`?
`eval` is a powerful function in MATLAB that interprets and executes a string containing a valid MATLAB expression. This allows you to dynamically evaluate code, giving you flexibility in programming. However, it is essential to use this function carefully as it can lead to complexity and performance issues in your code.
Syntax of `eval`
The basic syntax of `eval` is straightforward:
eval(expression)
Here, `expression` is a string that MATLAB interprets as a command. For example, if you wanted to raise a number to a power, you could use:
result = eval('2^3'); % result = 8
By evaluating the string `'2^3'`, the output is calculated and stored in the variable `result`.
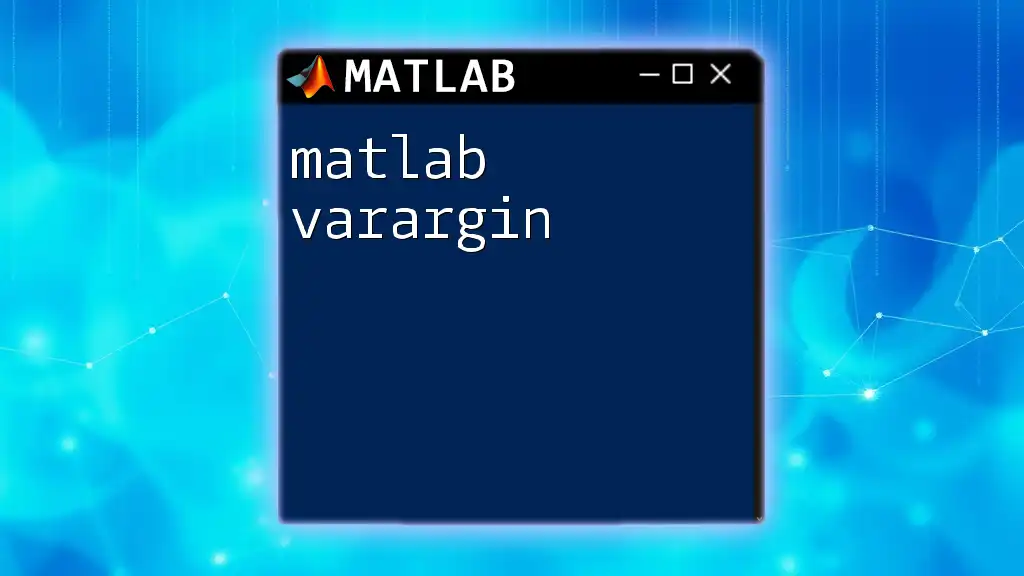
Basic Usage of `eval`
Executing Simple Expressions
`eval` is particularly useful for executing basic mathematical expressions or operations. For instance:
expr = '3 + 5';
result = eval(expr); % result = 8
In this snippet, we evaluated a simple arithmetic expression that sums two numbers. This capability to interpret strings as executable code is what makes `eval` stand out.
Dynamic Variable Creation
One compelling feature of `eval` is the ability to dynamically create variable names. This is achieved by forming a string that represents the variable name and its desired value. For instance:
varName = 'myVar';
eval([varName ' = 10;']); % Creates myVar with a value of 10
Here, `eval` interprets the concatenated string, creating a variable named `myVar` with an integer value of 10. However, this can lead to code that is difficult to read and manage, so caution is advised.
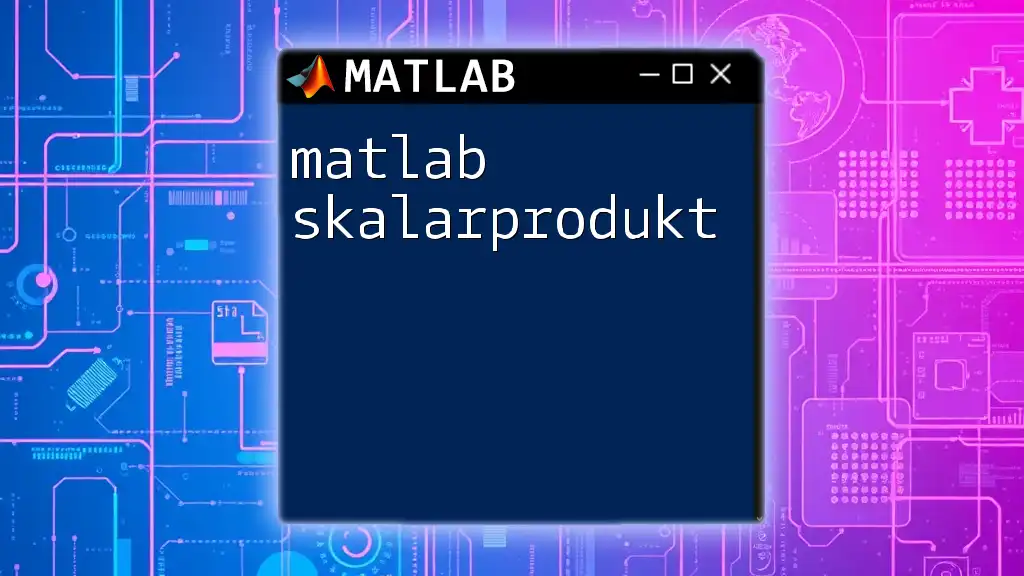
Advanced Usage of `eval`
Working with Expressions
`eval` can also be employed to execute multiple commands in one go by combining them in a single string. For example, you may want to set several variables at once:
expr = 'a = 2; b = 3; result = a + b;';
eval(expr); % The variable 'result' is now 5
This technique proves useful when you have a group of commands that depend on each other.
Using `eval` for Conditional Execution
You can leverage `eval` to dynamically execute commands based on conditions. For example:
condition = true;
if condition
eval('disp(''Condition met'')');
else
eval('disp(''Condition not met'')');
end
In this code block, the `eval` function interprets the command to display a message depending on the condition's truth value.
Managing Multiple Variables
With `eval`, you can manipulate multiple variables easily. This example demonstrates how to create a series of variables in a loop:
for i = 1:3
eval(['x' num2str(i) ' = i;']);
end
% This creates x1 = 1, x2 = 2, x3 = 3
While this shows the power of `eval`, it's crucial to maintain clarity in your code to avoid confusion later.
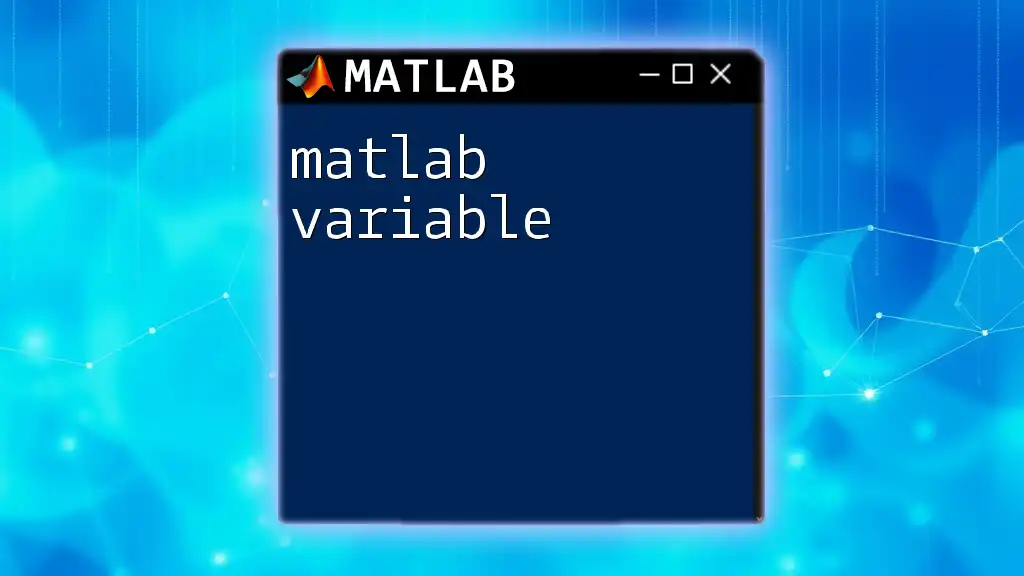
Performance Considerations
When to Avoid Using `eval`
While `eval` offers significant flexibility, it can have performance drawbacks. Whenever possible, you should seek alternatives to minimize runtime overhead. Code executed using `eval` is typically slower than direct function calls or logical operations, as MATLAB must interpret the string each time it runs.
Efficient Alternatives to `eval`
Prefer using functions such as `str2func` for greater efficiency. For example:
myFunction = 'sin';
x = str2func(myFunction)(pi); % Uses str2func to evaluate sin(pi)
This maintains performance efficiency while avoiding the complications that come with using `eval`.
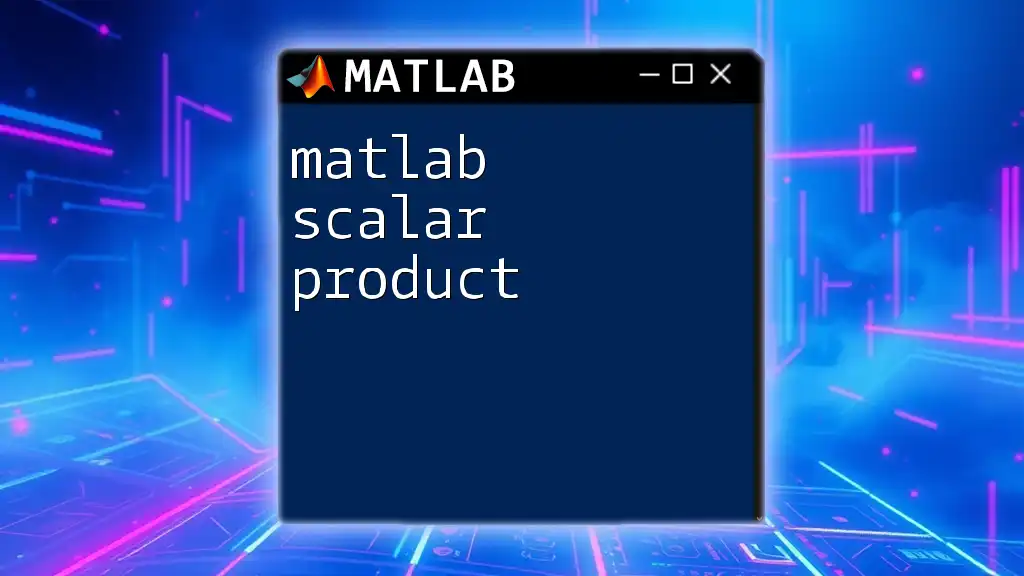
Best Practices with `eval`
Maintaining Code Readability
Using `eval` can complicate the readability of your code. Ensure to comment generously on sections where `eval` is employed to clarify its purpose. Avoid overusing `eval` in favor of more straightforward MATLAB constructs that achieve the same goal.
Debugging and Testing
When debugging code containing `eval`, utilize `try-catch` blocks to manage potential errors gracefully:
try
eval('undefinedVar'); % This will throw an error
catch ME
disp(['Error: ' ME.message]);
end
This approach lets you catch exceptions and take action accordingly, preserving the robustness of your code.
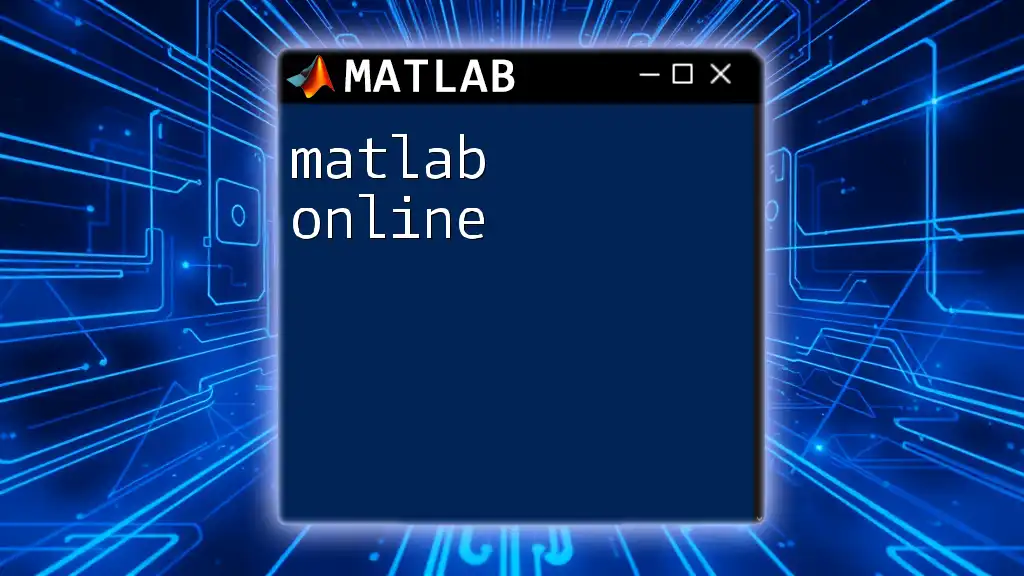
Common Pitfalls of Using `eval`
Variable Name Conflicts
One significant risk of using `eval` is the potential for variable name conflicts. Dynamically created variable names can inadvertently overwrite existing variables, leading to unexpected behavior in your code.
Error Handling in `eval`
Because `eval` does not inherently manage errors, it's essential to implement error handling carefully. Avoiding reliance on `eval` can reduce complexity and improve code safety.
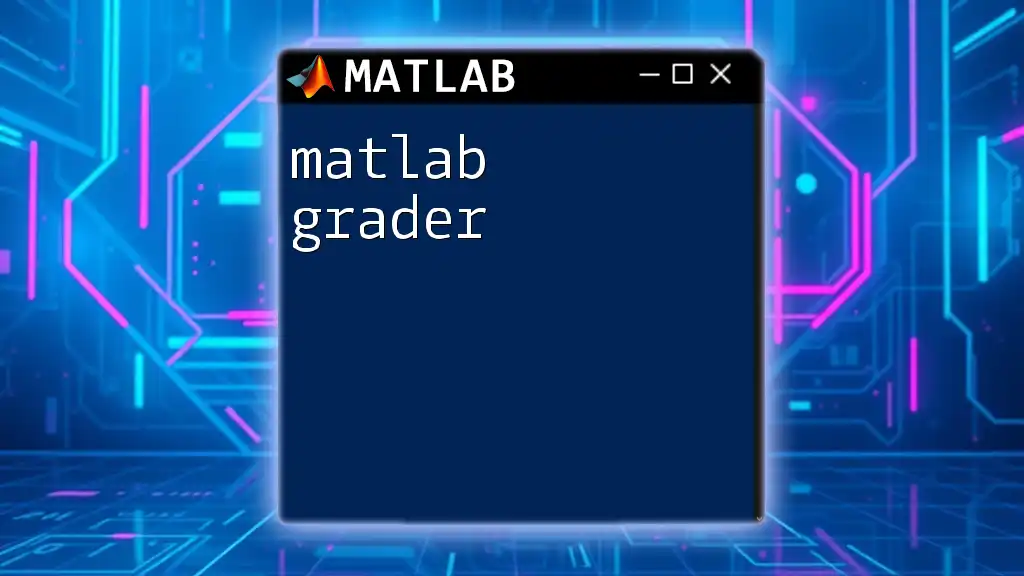
Case Studies
Real-World Applications of `eval`
`eval` finds practical applications across various scenarios, especially when constructing dynamic user interfaces or customizing workflows in engineering applications. For instance, scientists might use `eval` within a loop for data analysis where the expressions to evaluate depend on external inputs or configurations.
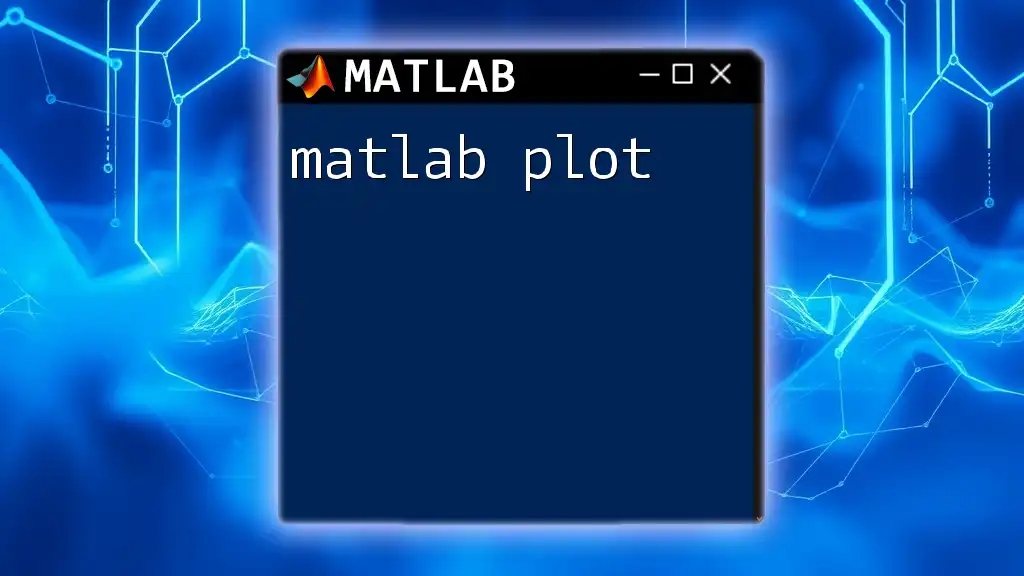
Conclusion
In summary, while the `matlab eval` function provides incredible flexibility and power in programming, it is crucial to use it judiciously. Emphasizing best practices will keep your code clearer, safer, and more efficient. Always weigh the benefits against the potential pitfalls to make informed decisions in your MATLAB programming journey.
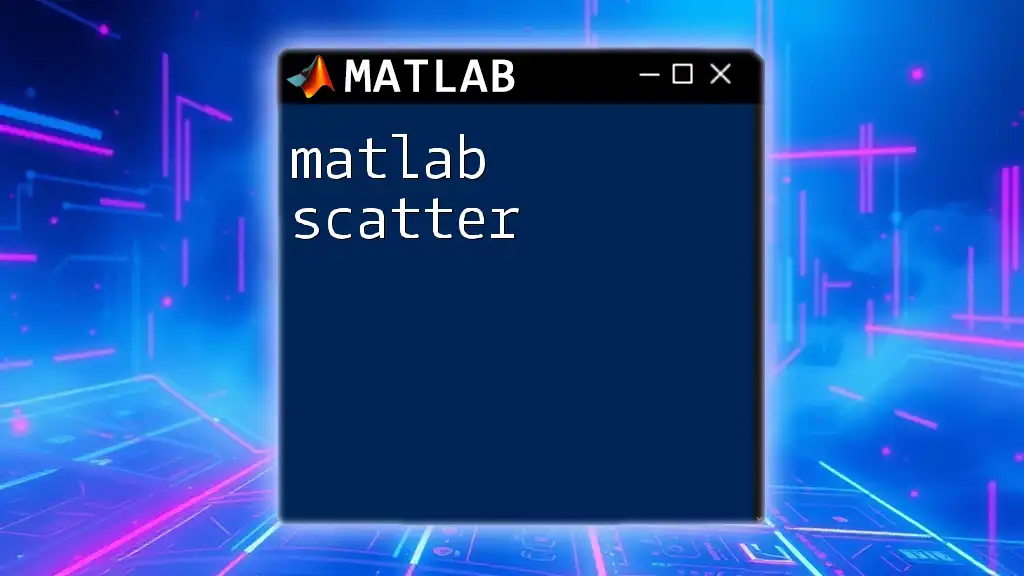
Additional Resources
For further learning, consider exploring the official [MATLAB documentation](https://www.mathworks.com/help/) and various online courses that dive deeper into `eval` and its applications in MATLAB programming.