The MATLAB `sinc` function computes the normalized sinc function, defined as sin(πx)/(πx), which is commonly used in signal processing and interpolation tasks.
x = -10:0.1:10; % Create a vector of x values
y = sinc(x); % Compute the sinc function
plot(x, y); % Plot the sinc function
title('Plot of the sinc function');
xlabel('x');
ylabel('sinc(x)');
Understanding the Sinc Function
What is the Sinc Function?
The sinc function is a mathematical function defined as:
sinc(x) = sin(πx) / (πx) for \( x \neq 0 \)
sinc(0) = 1
This function is significant because it serves as the idealized shape of a low-pass filter in signal processing. In essence, it arises in various contexts, particularly in the Fourier transform, where it represents the amplitude response of a perfect low-pass filter.
The graph of the sinc function exhibits oscillations that diminish gradually, demonstrating its non-local behavior, which is crucial in many analyses involving frequencies.
Applications of the Sinc Function
The sinc function plays an integral role in multiple fields:
- Signal Processing: It is widely used in designing digital filters, especially when reconstructing signals from samples.
- Fourier Transforms: It helps in representing the frequency components of signals.
- Interpolation: The sinc function acts as a kernel in sinc interpolation, allowing for the reconstruction of continuous signals from discrete samples.
Real-world applications of the sinc function include audio processing, image reconstruction, and telecommunications, where precise signal representation is vital for conveying information accurately.
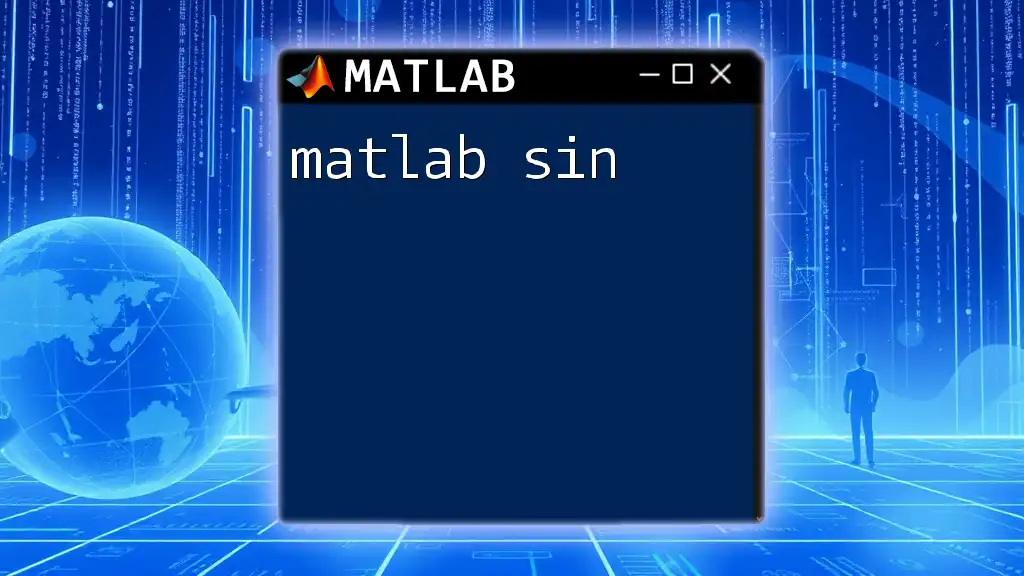
The Sinc Command in MATLAB
Overview of MATLAB Sinc Command
In MATLAB, the sinc function is provided directly with the command sinc(X). The input can be either a vector or a matrix, making it easy to compute sinc values across a wide range.
Basic Usage of the Sinc Command
To generate sinc values, you can use MATLAB’s built-in command as follows:
x = -10:0.1:10; % Define the range
y = sinc(x); % Compute the sinc values
plot(x, y); % Plotting the graph
title('Sinc Function in MATLAB');
xlabel('x');
ylabel('sinc(x)');
grid on;
In this example, we create a vector `x` ranging from -10 to 10 with increments of 0.1. The `sinc` function computes the sinc values for this vector, and we plot the result. The grid enhances readability, allowing for an easier analysis of the function's properties.
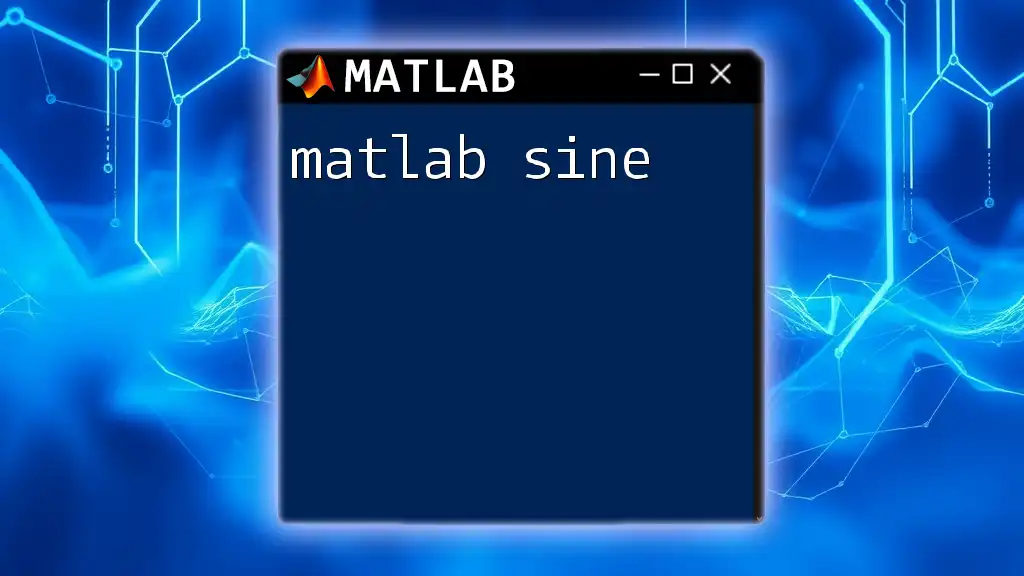
Advanced Usage
Modifying the Sinc Function
Scaling and Shifting
The sinc function can be modified through scaling and shifting, enabling control of its characteristics.
- Scaling alters the width of the function. For instance, to scale the sinc function:
factor = 2; % Scaling factor
y_scaled = sinc(factor * x);
plot(x, y_scaled);
title('Scaled Sinc Function');
In this example, using a scaling factor of 2 compresses the sinc function, making it narrower by increasing its oscillation frequency.
- Shifting enables the relocation of the function along the x-axis. To shift the sinc function, use:
shift = 1; % Shift value
y_shifted = sinc(x - shift);
plot(x, y_shifted);
title('Shifted Sinc Function');
Here, a shift value of 1 moves the graph to the right by 1 unit, allowing for a new anchor point.
Sinc Function in Filtering
The sinc function is known as the ideal low-pass filter. This characteristic can be leveraged to design filters in digital signal processing. A commonly used filter design technique is to create the filter coefficients using the sinc function.
Consider the following code example to create a low-pass filter using the sinc function:
fs = 100; % Sampling frequency
cutoff_freq = 10; % Cut-off frequency
sinc_filter = sinc(cutoff_freq/fs * (x - shift));
Here, we define a sampling frequency and a cut-off frequency, using the sinc function to generate filter coefficients. This implementation facilitates the construction of filters that effectively pass low frequencies while attenuating higher frequencies.
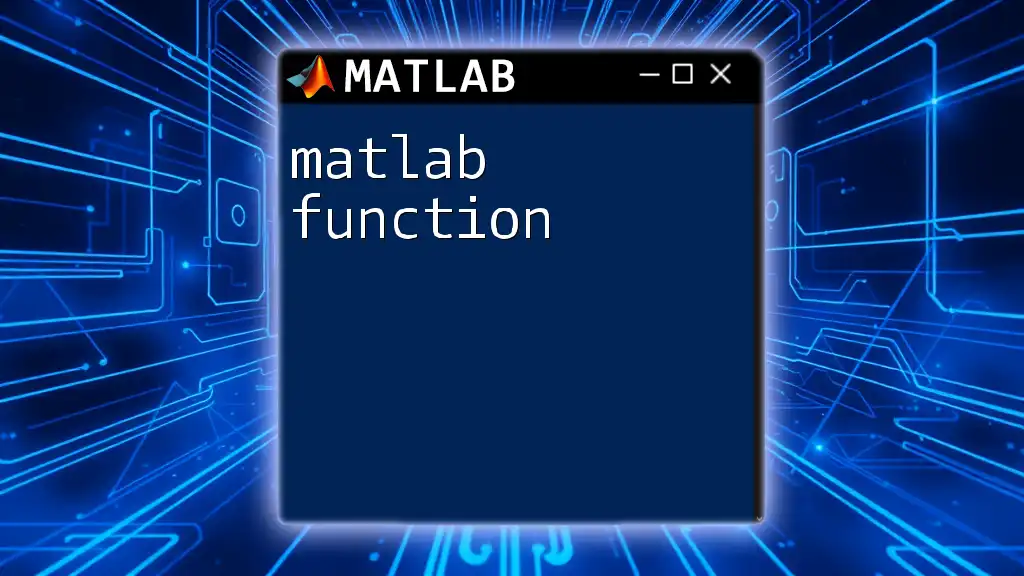
Tips for Optimizing MATLAB Sinc Usage
Best Practices
To maximize efficiency and accuracy when using the `sinc` function in MATLAB, it is crucial to:
-
Avoid Numerical Instabilities: When computing the sinc function for small values of x, careful consideration should be given to avoid division inaccuracies. The built-in `sinc` function effectively manages this.
-
Utilize Vectorization: Whenever possible, use MATLAB’s vector operations instead of loops to increase performance and code readability.
Performance Tips
When working with large datasets or when performance is a priority, consider these techniques:
-
Efficient Computation Techniques: Vectorized operations in MATLAB can significantly speed up calculations. Ensure that you are operating on entire arrays or matrices rather than individual elements.
-
Memory Management: When working with large arrays, preallocate memory for your variables to avoid resizing them during calculations, which can slow down execution.
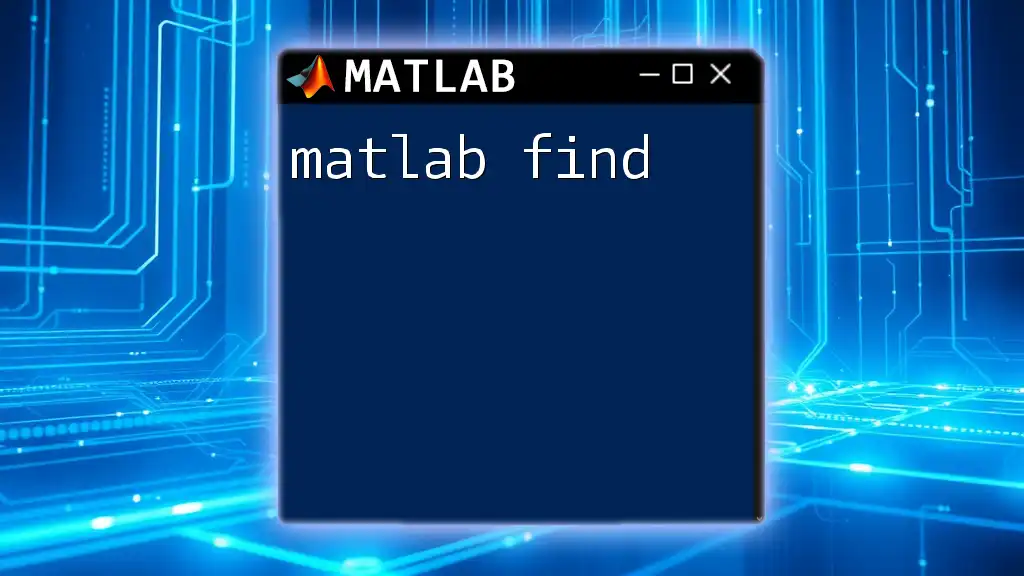
Conclusion
Understanding the MATLAB sinc function opens the door to a wealth of applications in signal processing and mathematics. By mastering the basic commands, advanced modifications, and principles of filter design, you can harness the capabilities of the sinc function effectively.
As you explore the intricacies of using the `sinc` function in MATLAB, don’t hesitate to experiment with different parameters to visualize and comprehend its behavior. The more you engage with these concepts, the more adept you will become in utilizing MATLAB for various computational tasks.
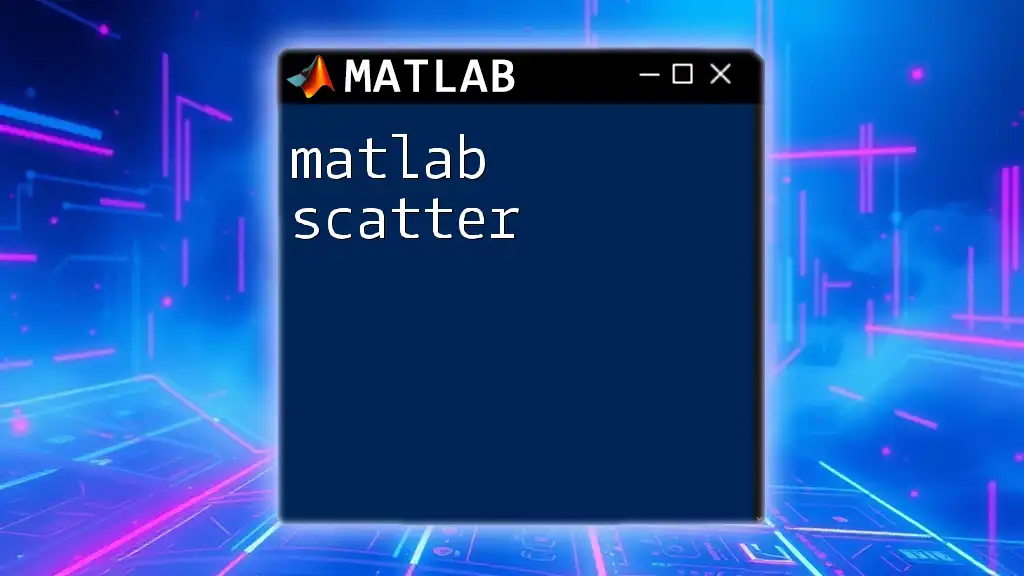
Further Resources
For deeper insights into the sinc function and its applications, consider exploring the official MATLAB documentation or perusing educational materials focused on signal processing. Engaging in online forums can also provide valuable community support as you expand your knowledge base.