The MATLAB `snr` function calculates the signal-to-noise ratio (SNR) of a signal, indicating how much a signal has been corrupted by noise, and can be used as follows:
% Example of calculating SNR in MATLAB
signal = [1, 2, 3, 4, 5]; % Example signal
noise = [0.5, 0.5, 0.5, 0.5, 0.5]; % Example noise
snr_value = snr(signal, noise); % Calculate SNR
disp(snr_value); % Display SNR value
Understanding Signal-to-Noise Ratio (SNR)
What is Signal-to-Noise Ratio?
Signal-to-Noise Ratio (SNR) is a crucial concept in signal processing that quantifies the level of a desired signal to the level of background noise. A higher SNR indicates a cleaner, more reliable signal. It's widely measured in decibels (dB), with the formula typically represented as SNR = 10 * log10(P_signal / P_noise), where:
- P_signal is the power of the signal.
- P_noise is the power of the noise.
Understanding SNR is essential for applications ranging from audio signal processing to telecommunications, as it helps determine the quality of signals, especially in noisy environments.
Types of SNR
There are several variants of SNR that are important to consider:
- Peak SNR vs. Average SNR: Peak SNR focuses on the highest power levels, while Average SNR looks at the signal power averaged over time.
- Spatial SNR: Involves SNR measurements across different spatial locations, relevant for applications like wireless communications.
- Temporal SNR: Considers how SNR varies over time, crucial for signals that change dynamically.
- Frequency-domain SNR: Deals with SNR in the frequency spectrum, helpful in analyzing signals through Fourier Transform techniques.

MATLAB SNR Function
Overview of the SNR function in MATLAB
MATLAB provides built-in tools for calculating SNR, making it a powerful ally in signal processing. The snr function is central to these calculations, allowing users to assess the quality of signals with ease.
Syntax of the SNR Function
The syntax of the SNR function in MATLAB is straightforward:
snr(signal, noise)
Here, signal refers to the original clean signal, while noise refers to the noise that has been added to it. You can also incorporate optional parameters depending on your specific use case, such as specifying the background and excess noise types.
Example of Basic SNR Calculation
To illustrate basic SNR calculation in MATLAB, let's consider an example involving random signals:
% Generate a clean signal
fs = 1000; % Sampling frequency
t = 0:1/fs:1;
signal = sin(2*pi*50*t);
% Generate noise
noise = randn(size(t));
% Add noise to the signal
noisy_signal = signal + noise;
% Calculate SNR
snr_value = snr(signal, noise);
disp(['SNR Value: ', num2str(snr_value), ' dB']);
In this example:
- We create a simple sine wave as a clean signal.
- Random noise is generated and then added to this signal.
- Finally, we calculate the SNR using the MATLAB `snr` function.
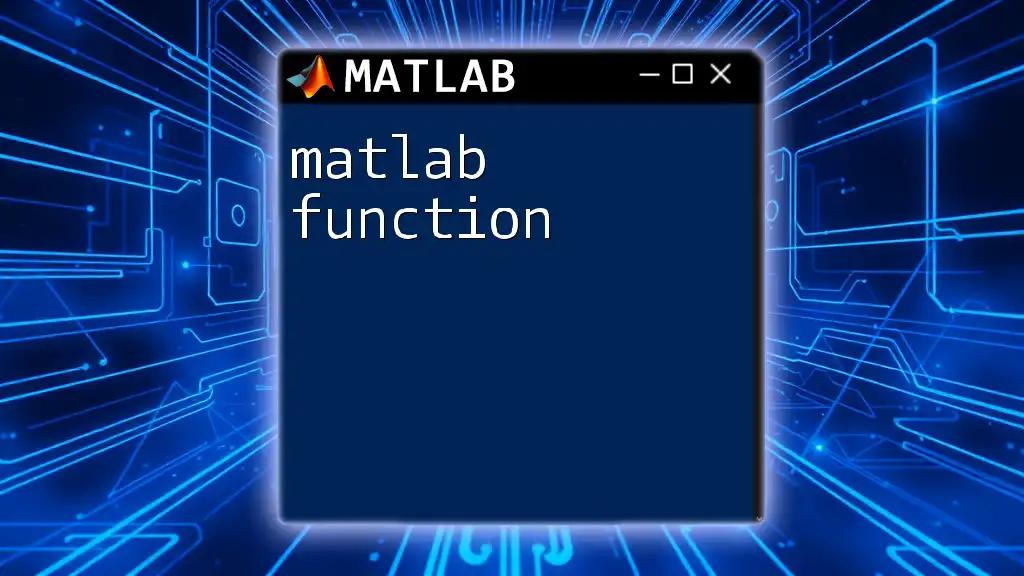
Practical Applications of SNR in MATLAB
Analyzing SNR in Real-World Signals
SNR plays a pivotal role in various applications such as audio processing and wireless communication. For instance, in audio applications, a high SNR signifies better sound quality, making it essential for music production and broadcasting. In telecommunications, SNR determines how well information can be transmitted over channels susceptible to disturbance.
Visualizing SNR with MATLAB
One powerful aspect of MATLAB is its ability to visualize data effectively. By plotting both the clean and noisy signals, we can gain insight into how noise is impacting the signal. Here’s how you can visualize the signals:
% Plotting the signals
figure;
subplot(2,1,1), plot(t, signal), title('Clean Signal');
subplot(2,1,2), plot(t, noisy_signal), title('Noisy Signal');
This snippet creates a two-panel plot where the top shows the clean signal and the bottom displays how noise alters the original signal. By examining these plots, you can intuitively assess the impact of noise, thereby understanding SNR better.

Advanced SNR Calculations in MATLAB
Using Additional Functions with SNR
To elevate your analysis, you can combine the SNR function with FFT (Fast Fourier Transform). FFT allows for the evaluation of SNR in the frequency domain, revealing critical insights into signal behavior.
Example Using FFT to Analyze SNR
% FFT of the signals
Y = fft(noisy_signal);
f = (0:length(Y)-1)*fs/length(Y);
% Plotting the FFT
figure;
plot(f, abs(Y));
title('FFT of Noisy Signal');
xlim([0 fs/2]);
xlabel('Frequency (Hz)');
ylabel('Magnitude');
In this illustration, the FFT of the noisy signal is calculated and plotted. This can help analyze how much noise exists at different frequencies, offering further insight into the SNR in the frequency domain.
Custom SNR Calculations
For a deeper understanding, you may want to calculate SNR manually. The fundamental formula is:
SNR (dB) = 10 * log10(P_signal / P_noise)
To manually calculate SNR, you can implement the following example:
signal_power = sum(abs(signal).^2) / length(signal);
noise_power = sum(abs(noise).^2) / length(noise);
manual_snr = 10 * log10(signal_power / noise_power);
disp(['Manual SNR Value: ', num2str(manual_snr), ' dB']);
Here, you calculate the power of both the signal and noise before applying the SNR formula. This method reinforces your understanding of the SNR calculation process.

Troubleshooting Common SNR Issues in MATLAB
Common Errors and How to Resolve Them
When dealing with SNR calculations, you may encounter common errors, such as misinterpreting the length of signals or incorrectly configuring noise characteristics. Make sure to validate the inputs to avoid discrepancies in your SNR results.
Optimizing Signal Quality
Improving SNR often involves filtering techniques to minimize noise. Consider applying low-pass filters or adaptive filtering, depending on your signal characteristics. Understanding the noise characteristics will help you choose the best filtering techniques to enhance your results.
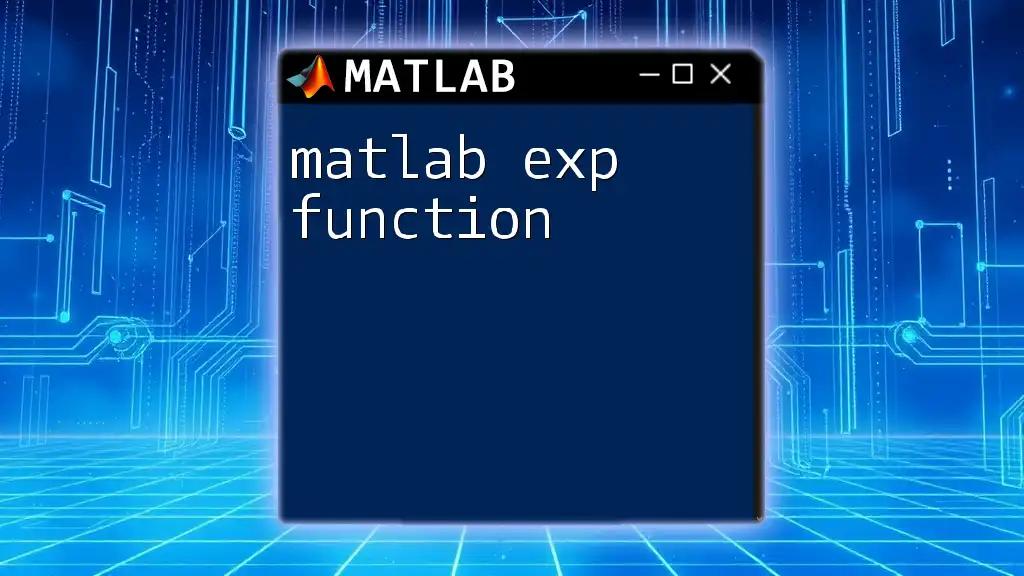
Conclusion
In this comprehensive guide, we explored the MATLAB SNR function formula, offered practical examples, and provided insight into real-world applications. Understanding how to calculate and analyze SNR is crucial for anyone working in signal processing. With the practical tips shared, you can enhance your MATLAB skills and effectively apply knowledge of SNR to various projects and applications.