The `switch` statement in MATLAB allows you to execute different blocks of code based on the value of a variable, enabling cleaner and more manageable conditional logic compared to multiple `if` statements.
Here's a code snippet demonstrating the use of a `switch` case in MATLAB:
value = 'B';
switch value
case 'A'
disp('You selected A');
case 'B'
disp('You selected B');
case 'C'
disp('You selected C');
otherwise
disp('Input not recognized');
end
Understanding the Syntax of Switch Case in MATLAB
Basic Structure of the Switch Case Statement
A switch case statement in MATLAB serves as a decision-making construct. It allows the evaluation of an expression against a series of potential matching values. This structure is particularly useful for handling multiple potential outcomes based on a single variable or expression, thereby improving code readability and maintainability.
The primary components of a switch case statement include:
- The `switch` keyword, which initiates the statement and is followed by the expression to be evaluated.
- Several `case` blocks, each defining different values that the expression can take.
- An optional `otherwise` block, which executes if none of the specified cases match.
General Syntax
The general syntax for a MATLAB switch case statement is as follows:
switch expression
case value1
% code to execute if expression == value1
case value2
% code to execute if expression == value2
otherwise
% code to execute if none of the cases match
end
This structure allows for the expression to be compared to multiple values effortlessly, enhancing the decision-making process in your code.
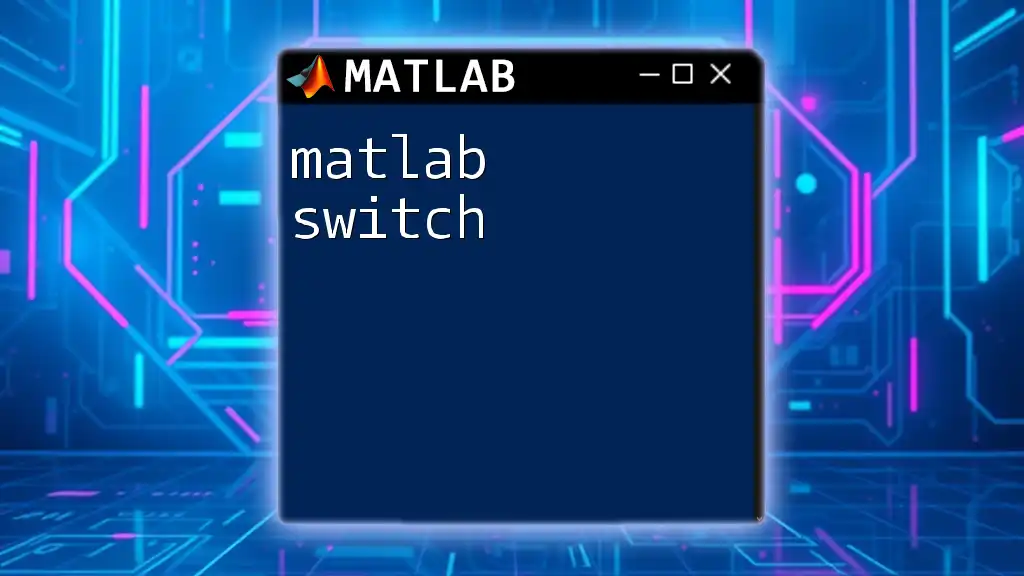
How to Use Switch Case in MATLAB
Declaring a Variable and Using It in Switch Case
To illustrate how to use the MATLAB switch case construct, let's declare a variable representing the day of the week. Below is an example that demonstrates how the switch statement evaluates the variable against various case values:
day = 'Monday';
switch day
case 'Monday'
disp('Start of the week');
case 'Friday'
disp('End of the week');
otherwise
disp('Midweek days');
end
In this example, the output will be "Start of the week" since the variable day is set to 'Monday'. The switch case allows for a clear, straightforward assignment of actions based on the variable's value.
Multiple Case Values
MATLAB also allows you to group multiple case values within a single case block. This feature can greatly reduce redundancy in your code. Here’s an example:
color = 'Red';
switch color
case {'Red', 'Green'}
disp('Stop or Go');
case 'Blue'
disp('Sky Color');
otherwise
disp('Unknown Color');
end
In this instance, if the value of color is either 'Red' or 'Green', the output will be "Stop or Go". This capability of handling multiple cases reinforces the flexibility and efficiency of the MATLAB switch case structure.
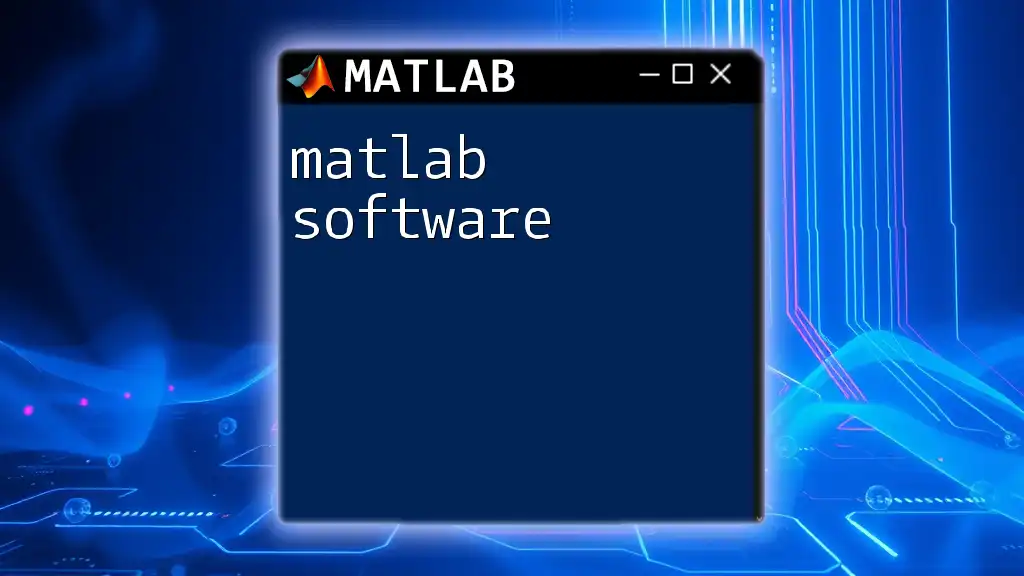
Advantages of Using Switch Case
Cleaner Code and Improved Readability
One of the main advantages of using a switch case statement is that it results in cleaner code. When faced with multiple conditions, a switch case can eliminate the need for nested if-else statements, leading to greater readability. For example, a long chain of if-else statements can become cumbersome, while a switch case presents a straightforward and organized structure.
Performance Benefits
From a performance standpoint, switch cases can be more efficient than long chains of if-else statements. MATLAB optimizes switch case constructs, leading to potentially faster execution, especially when dealing with many conditions. The logical branching is handled in an effective manner, minimizing the computational overhead.
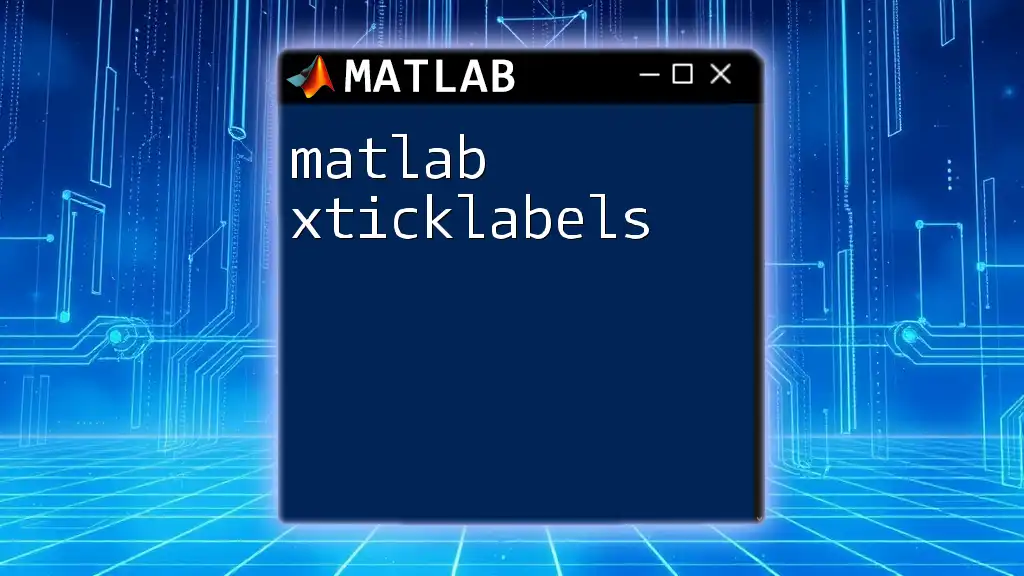
Best Practices for Using Switch Case
Keep Cases Focused and Simple
It's essential to ensure that each case is uncomplicated and retains a single focus. A clear, structured approach can aid in maintainability. For instance, each case should contain operations directly related to that case's output. Avoid cramming excessive logic into a single case block, as this can lead to confusion and redundancy.
Avoid Overusing Switch Case
While the switch case is powerful, it's vital to recognize when to use it appropriately. In scenarios where you have complex conditions or require boolean logic, other control structures like if-else statements may be more suitable. Balancing readability and complexity will ultimately enhance the quality of your code.
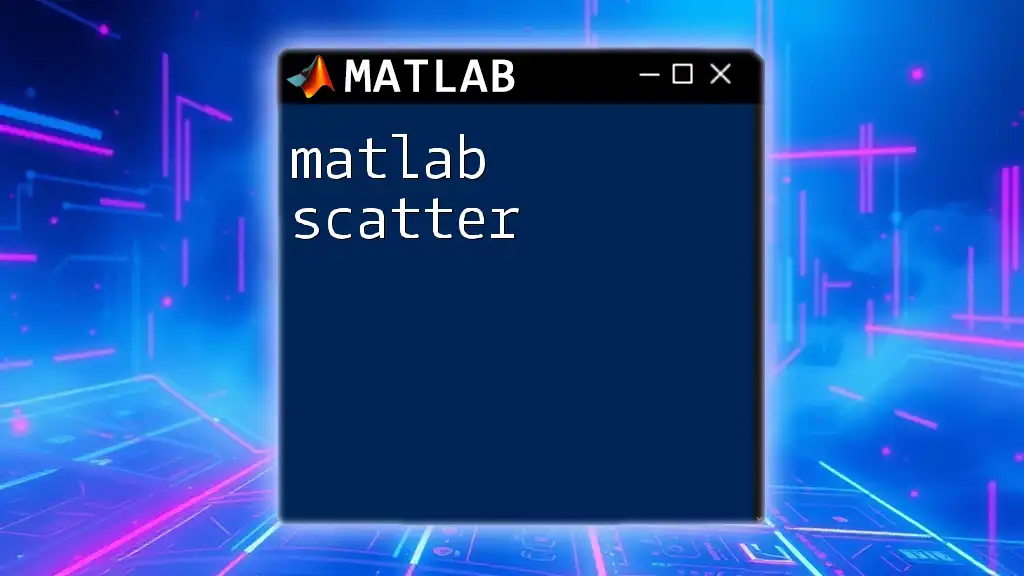
Common Mistakes When Using Switch Case
Forgetting the `otherwise` Block
A common mistake is neglecting to include an otherwise block. The consequences of this oversight can lead to unexpected behavior if none of the cases match. If you don’t provide a default action, your code might not handle all scenarios properly, resulting in subtle bugs. In practice, including an otherwise block is always recommended to maintain robustness in your code.
Case Insensitivity
Another frequent error arises from misunderstanding MATLAB's case sensitivity. For example, consider the following snippet:
input = 'Yes';
switch input
case 'yes' % This does not match
disp('Input is Yes');
otherwise
disp('Input is not matching');
end
In this case, the input does not match the case due to varying letter cases. MATLAB treats 'Yes' and 'yes' as distinct values, which may lead to confusion. Always ensure that your comparisons account for case sensitivity when coding.
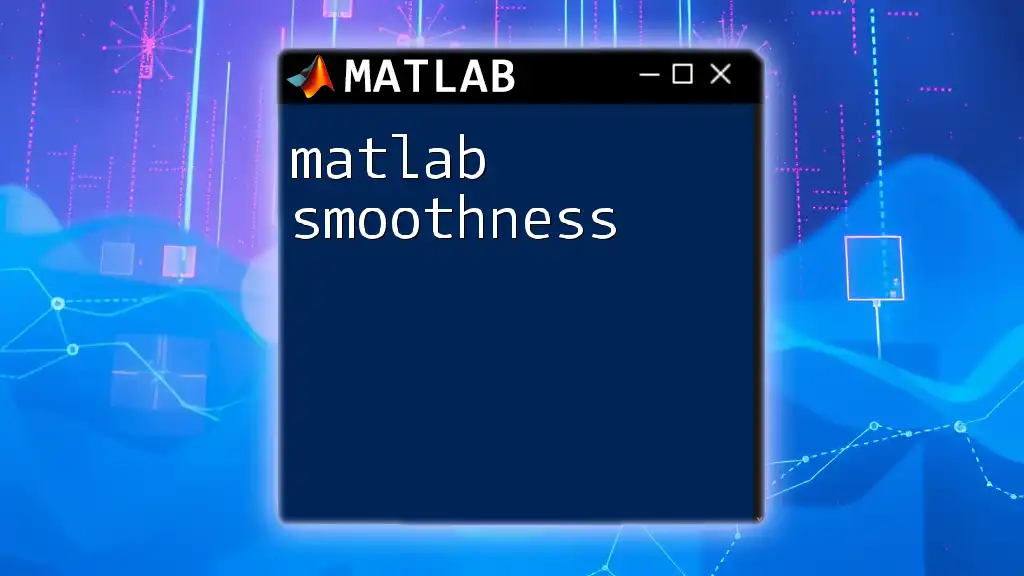
Conclusion
The MATLAB switch case statement is a crucial tool for efficient decision-making within your code. By streamlining the evaluation of multiple conditions, it enhances both code clarity and performance. As you continue to explore and utilize this structure, consider practicing its implementation in various scenarios to solidify your understanding.
Feel free to share any insights or questions in the comments section, as engaging with the community can further enrich your learning experience.
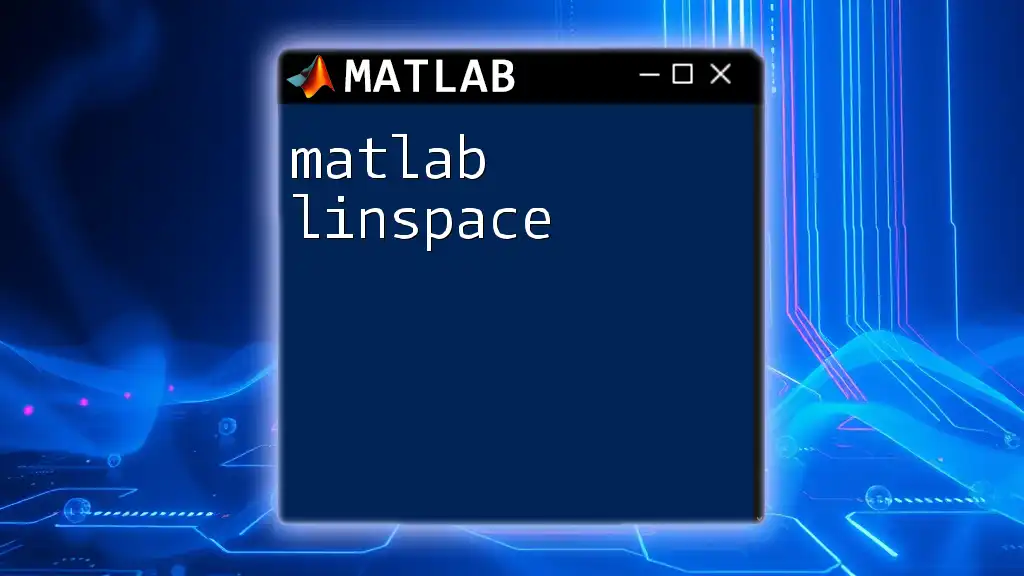
Additional Resources
To expand your knowledge on the MATLAB switch case statement, consider exploring the official MATLAB documentation, which provides comprehensive insights. Additionally, numerous tutorials and online courses can further enhance your proficiency in MATLAB programming.