MATLAB Symmetric Sign Component Decomposition refers to the process of breaking down a signal into its symmetric and anti-symmetric components using the sign function for analysis or processing.
Here's a code snippet demonstrating how to perform this decomposition:
% Example MATLAB code for Symmetric Sign Component Decomposition
signal = [1, -2, 3, -4, 5]; % Example signal
symmetric = (signal + fliplr(signal)) / 2; % Symmetric component
antisymmetric = (signal - fliplr(signal)) / 2; % Anti-symmetric component
What is Symmetric Sign Component Decomposition?
Symmetric Sign Component Decomposition (SSCD) is a powerful technique used primarily in signal processing to break down complex signals into their constituent components based on symmetry. This method not only simplifies the analysis of signals but also enhances the interpretability of various phenomena in a diverse range of fields, including engineering, physics, and finance. By isolating symmetric and asymmetric components, one can gain insights into the underlying structures of the signals being analyzed.

Understanding the Basics of MATLAB
What is MATLAB?
MATLAB (Matrix Laboratory) is a high-performance programming language and interactive environment designed for numerical computation, visualization, and programming. It is widely used by engineers and scientists for modeling and analyzing data. The flexibility and extensive built-in functions make MATLAB an indispensable tool in various domains, particularly in signal processing tasks such as SSCD.
MATLAB Syntax Overview
In MATLAB, understanding the syntax is crucial for effective programming. Key commands and structures include:
- Scripts: A sequence of MATLAB commands saved in a file.
- Functions: Customizable blocks of code that accept input arguments and return output.
Grasping the basic syntax will empower you to efficiently implement matlab symmetric sign component decomposition in your projects.
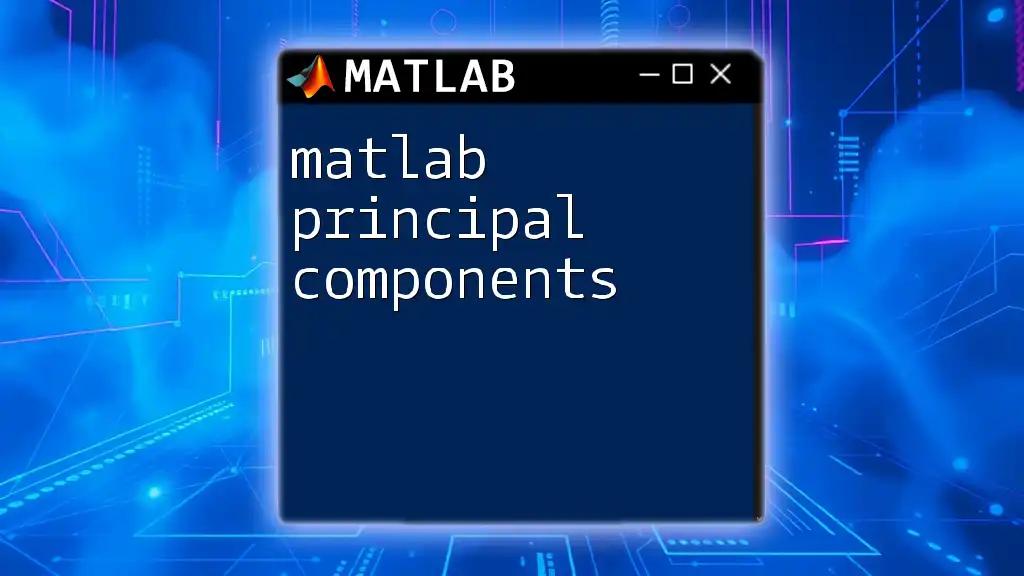
Theoretical Background
Concept of Symmetric Sign Component Decomposition
The fundamental principle of symmetric sign component decomposition lies in the concept of separating a signal into parts that exhibit symmetry. This is particularly useful for analyzing periodic signals, where the characteristics of symmetry can reveal significant information about the waveforms.
Mathematical Formulation
The mathematical formulation for SSCD revolves around identifying symmetric and asymmetric components of the signal. The symmetric component can be represented by:
\[ S(t) = \frac{1}{2}[x(t) + x(-t)] \]
where \( x(t) \) is the original signal, and \( x(-t) \) is the time-reversed version. This equation shows how the symmetric components can be computed, revealing structure within the original data.

Implementation in MATLAB
Setting Up Your Environment
To successfully implement matlab symmetric sign component decomposition, make sure your environment is set up correctly:
- Install MATLAB: Make sure you have the latest version of MATLAB installed.
- Load Required Toolboxes: Some functions used may require additional toolboxes such as Signal Processing Toolbox.
Basic Commands for Signal Processing
Familiarizing yourself with the core MATLAB commands is essential. Among these, key functions include:
- `fft`: Computes the Fast Fourier Transform.
- `ifft`: Computes the Inverse Fast Fourier Transform.
- `filter`: Designs a digital filter to process signals.
These commands are integral for manipulating and analyzing signals effectively.
Step-by-Step Guide to Symmetric Sign Component Decomposition
Importing Your Data
To begin, you need to import the signal data you wish to analyze. This can be achieved using the following code snippet:
data = load('your_data_file.mat');
Make sure your data file is in the MATLAB workspace, and it should ideally contain a variable representing the signal.
Initial Signal Preparation
Before applying SSCD, the signal often requires preprocessing. This step could involve filtering to remove noise. Here’s an example of filtering a signal:
filtered_signal = lowpass(data.signal, cutoff_frequency);
This command applies a low-pass filter to the signal stored in `data.signal`, allowing only frequencies below the specified cutoff frequency to pass through, thus preparing your data.
Performing Symmetric Sign Component Decomposition
The next step is to apply the symmetric sign component decomposition algorithm. While MATLAB doesn’t have a built-in function for SSCD, you can brainstorm a simple custom implementation. Here’s an example:
function [symmetric_component, asymmetric_component] = ss_decompose(signal)
% Compute the symmetric and asymmetric components
symmetric_component = 0.5 * (signal + flipud(signal));
asymmetric_component = 0.5 * (signal - flipud(signal));
end
In this function, `flipud` reverses the signal vertically (time-reversed), separating out the symmetric and asymmetric components effectively.
Analyzing the Results
Visualizing Components
Visual representation is key to understanding your findings. After the decomposition, you may want to visualize the original and the decomposed components:
time = 0:0.01:length(filtered_signal)-1; % create a time vector
plot(time, filtered_signal, 'r'); % plot filtered signal in red
hold on;
plot(time, symmetric_component, 'g'); % plot symmetric in green
hold off;
legend('Filtered Signal', 'Symmetric Component');
title('Signal Decomposition Visualization');
This code will produce a plot showing the relationship between the original filtered signal and its symmetric component.
Interpreting the Output
Interpreting the outputs involves assessing the characteristics of both components. Symmetric components often represent periodic behaviors, while asymmetric components might indicate deviations or noise. This interpretation plays a crucial role in the subsequent analysis and decision-making based on the data.
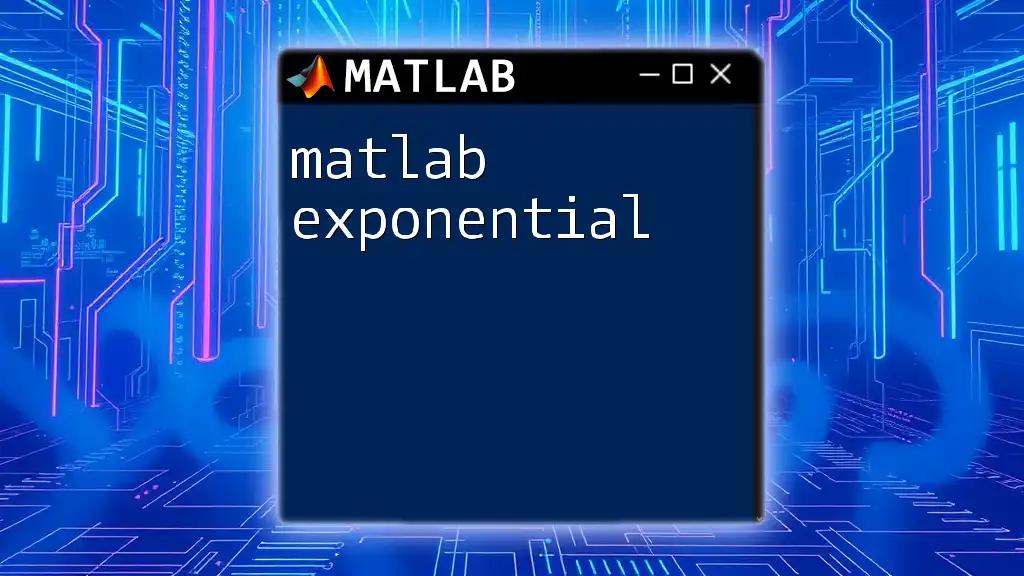
Practical Applications
Case Studies Using Symmetric Sign Component Decomposition
Numerous real-world applications utilize SSCD. For instance, in financial signal processing, detecting trends in stock prices often involves separating signals from noise, a prime application of SSCD. In engineering, analyzing signals in communication systems can help in identifying and correcting transmission errors efficiently.
Common Pitfalls and Troubleshooting
When implementing matlab symmetric sign component decomposition, it's important to be aware of potential challenges:
- Incorrect Data Input: Ensure your data is formatted correctly.
- Filtering Issues: Poorly chosen filter parameters can significantly distort results.
- Interpreting Results: Misinterpreting the symmetric and asymmetric components might lead to incorrect conclusions.
To troubleshoot these problems, always verify the steps of your implementation and validate the output through visualization and statistical checks.
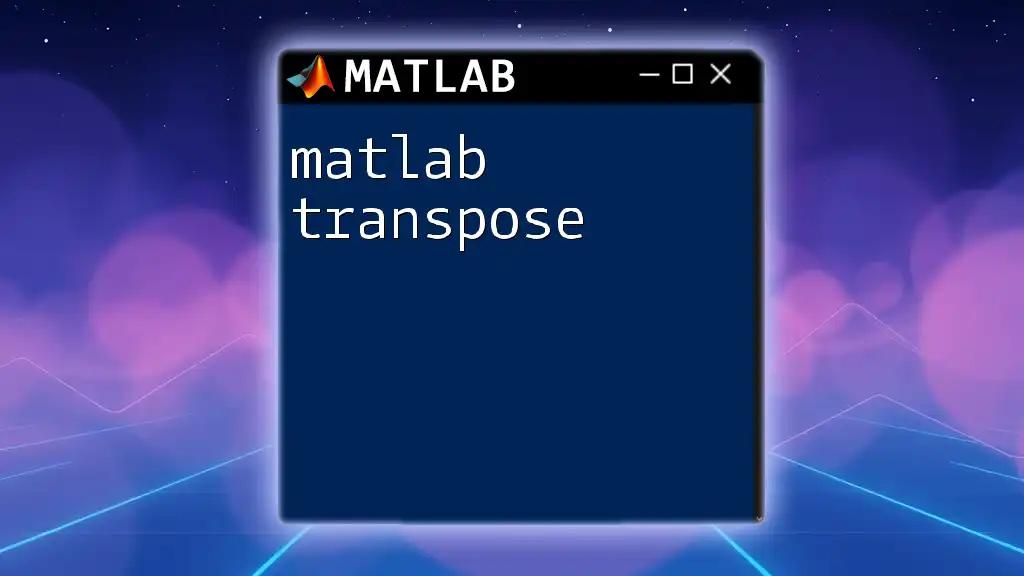
Conclusion
In summary, symmetric sign component decomposition is a substantial method for analyzing and interpreting signals in MATLAB. Through this comprehensive guide, you have grasped how to set up your environment, process data, perform the decomposition, and analyze the results effectively. Mastering these techniques opens up a wealth of possibilities in various scientific and engineering fields.
Next Steps and Additional Resources
For continuous improvement, consider delving deeper into MATLAB with more advanced tutorials and resources specific to signal processing. Joining online communities or forums can also enhance your knowledge and provide assistance from peers.

Frequently Asked Questions
What is the time complexity of symmetric sign component decomposition? The time complexity primarily depends on the size of the input signal and the operations involved in signal processing, which typically range from O(n) to O(n log n) for FFT-based approaches.
Can this method be applied to non-linear signals? Yes, while SSCD is primarily suited for linear and periodic signals, adjustments and modifications can allow for its application in non-linear scenarios.
How does this decomposition compare with other decomposition methods? SSCD focuses specifically on symmetry, while other methods like wavelet transforms or envelope detection have different objectives, such as localization or frequency analysis.
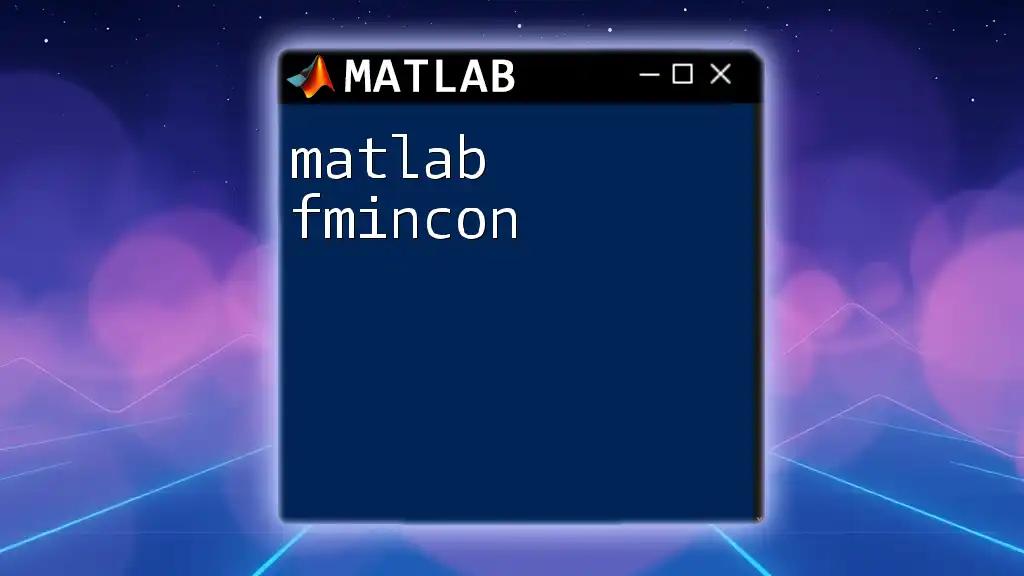
Call to Action
Ready to enhance your MATLAB skills further? Join our MATLAB community for workshops, discussions, and learning opportunities specifically tailored to signal processing. Together, explore the vast realms of matlab symmetric sign component decomposition and beyond!