The MATLAB symmetric sign component decomposition for a binary matrix enables the breakdown of the matrix into its symmetric and antisymmetric parts, helping to analyze its structural properties efficiently.
Here’s a code snippet demonstrating how to perform this decomposition:
% Define a binary matrix
A = [1 0 1; 0 1 0; 1 0 1];
% Compute symmetric and antisymmetric components
S = 0.5 * (A + A'); % Symmetric part
T = 0.5 * (A - A'); % Antisymmetric part
% Display results
disp('Symmetric Component:');
disp(S);
disp('Antisymmetric Component:');
disp(T);
Understanding Symmetric Sign Component Decomposition
Defining the Binary Matrix
A binary matrix is a matrix in which every element is either 0 or 1. These matrices are essential in various fields, primarily because they simplify data representation and processing in algorithms. The significance of binary representation is profound in the context of data science and machine learning, where, for instance, binary matrices are often used to represent datasets with categorical features.
Concept of Component Decomposition
Matrix decomposition refers to breaking down a matrix into simpler, constituent parts. These parts can reveal underlying structures and patterns within the data. Among the various methods of decomposition, symmetric sign component decomposition holds a special place, particularly for binary matrices. This decomposition aims to split the matrix into a symmetric part (which captures redundancies) and a sign component (which emphasizes the structure of binary data).

The Symmetric Sign Component Decomposition Process
Mathematical Foundation
To effectively implement symmetric sign component decomposition, understanding the mathematical principles is essential. The central idea is that any binary matrix can be structured into a pair of matrices: one that is symmetric and captures the relationships of similarities among the binary attributes, and another that retains the sign components for the unique identifications.
A typical equation representing the decomposition might look like:
\[ A = D + L \]
Where \( A \) is the original binary matrix, \( D \) is the symmetric part, and \( L \) is the sign component. The properties of symmetry and consistency are vital in ensuring that this decomposition remains effective across different binary matrices.
Implementation Flow
The implementation of symmetric sign component decomposition in MATLAB typically includes several steps. First, you initiate with the original binary matrix, follow through with mathematical transformations, and finally, extract the symmetric and sign components.
The key considerations during this implementation include ensuring the matrix is appropriately defined and understanding the limitations associated with dimension compatibility, as well as any data type constraints pertaining to binary matrices.
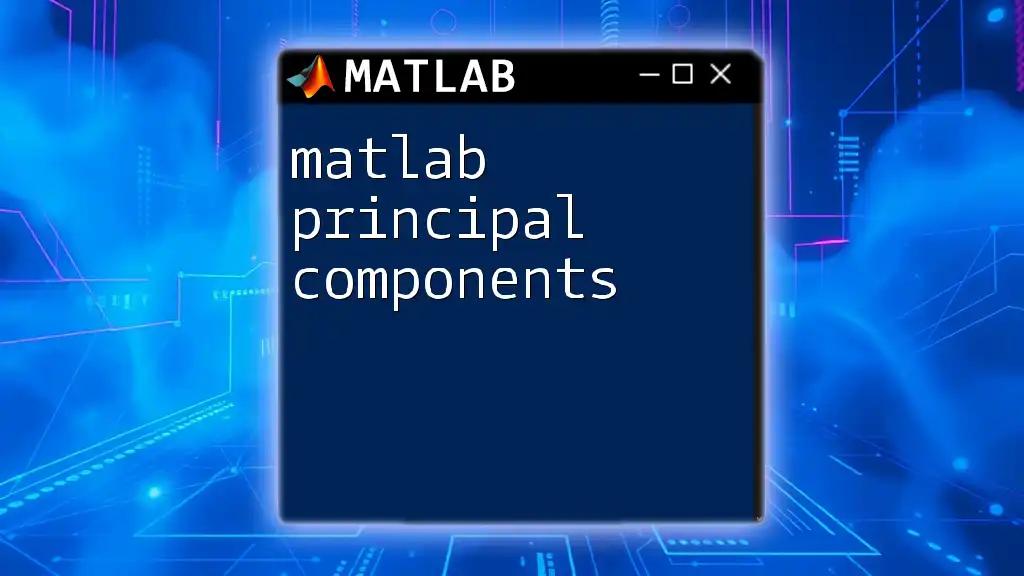
MATLAB Basics for Binary Matrices
Intro to MATLAB for Beginners
For those new to MATLAB, navigating the environment may seem daunting. However, familiarizing oneself with common commands enables easier handling of binary matrices. A few essential commands include `zeros()`, `ones()`, and `logical()`, as they allow quick matrix creation and manipulation.
Creating a Binary Matrix in MATLAB
Creating a binary matrix can be done efficiently using MATLAB's built-in functions. Here’s a simple example:
% Example: Creating a binary matrix
binaryMatrix = [1 0 0; 0 1 0; 0 0 1];
disp(binaryMatrix);
This snippet initializes a 3x3 binary matrix. The representation is clear and concise, allowing for immediate manipulation.
Basic Operations on Binary Matrices
Basic operations like addition, subtraction, and multiplication are often adapted in the context of binary matrices:
- Addition and Subtraction: These operations can be performed element-wise, with values exceeding 1 set back to 1, reflecting binary constraints.
- Logical Operations: Logical operations such as AND, OR, and NOT can be invaluable for binary matrix manipulation and yield insightful results based on the underlying data.
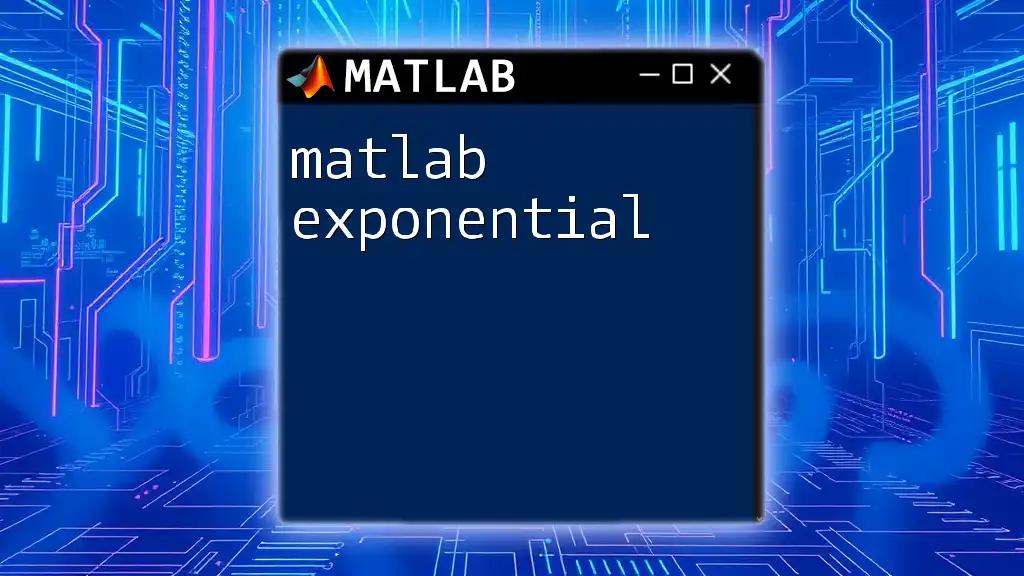
Conducting Symmetric Sign Component Decomposition in MATLAB
Building the Function
Creating a function to perform symmetric sign component decomposition requires a structured approach. Below is a template function to illustrate how the function might be structured:
function [D, L] = symmetricSignDecomposition(A)
% Function to decompose binary matrix A
% D - Decomposed symmetric matrix
% L - Sign component matrix
% Initialize matrices
D = zeros(size(A));
L = zeros(size(A));
% Algorithm to populate D and L goes here
% Return the results
end
Step-by-Step Implementation
Implementing the decomposition process involves several layers of computation. Begin by loading or creating your binary matrix, then execute the function created. Here’s how this could look in practice:
% Example implementation
A = [1 0 1; 0 1 0; 1 0 1];
[D, L] = symmetricSignDecomposition(A);
After running the above code, you will have \( D \) and \( L \) as outputs, providing you with the symmetric and sign component matrices respectively.
Interpreting Results
After obtaining the decomposition, it is crucial to understand what each output matrix signifies. The matrix \( D \) will typically encapsulate redundancies and relationships, while \( L \) will clarify the distinct binary structures. Visualization tools in MATLAB can help display these matrices more effectively, enhancing interpretability.
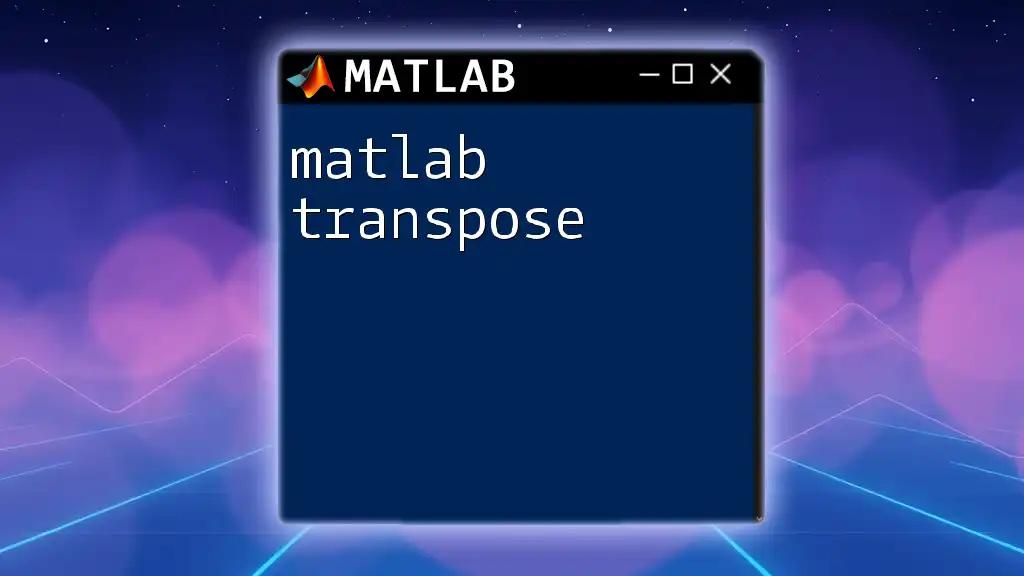
Example Use-Cases
Data Classification
Symmetric sign component decomposition can significantly enhance data classification tasks. By identifying patterns in binary attributes, machine learning models can be trained for greater accuracy.
Image Processing
In image processing, this decomposition can aid in enhancing binary images. For instance, it can help separate background and foreground elements, enabling clearer image segmentation.
Network Analysis
In contexts like network analysis, symmetric sign component decomposition can be used to identify connected components within binary matrix representations of graphs, facilitating a deeper understanding of network structures.

Debugging and Optimization
Common Errors and Solutions
When implementing the symmetric sign component decomposition, you might encounter common issues such as matrix dimension mismatches or incorrect logical operations. A careful review of matrix sizes and logical conditions can often resolve these errors swiftly.
Performance Optimization Techniques
Optimizing the performance of the decomposition function may involve refining algorithms to ensure they're efficient, especially with large matrices. For example, preallocating matrices instead of dynamically resizing them can lead to substantial performance improvements.
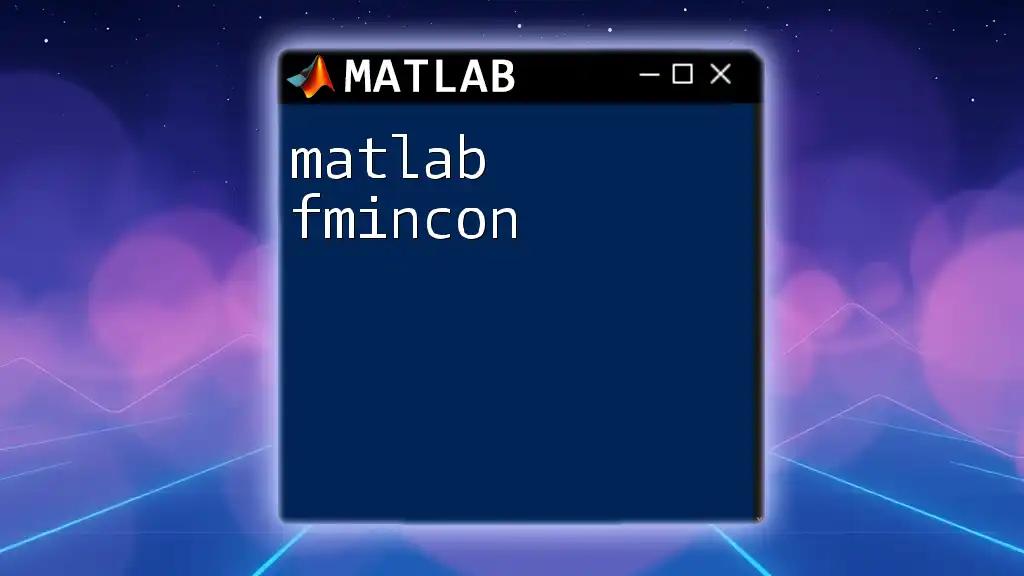
Conclusion
In summary, symmetric sign component decomposition for binary matrices is a powerful technique that highlights underlying patterns within binary datasets. By mastering this decomposition process in MATLAB, one can unlock enhanced analytical capabilities in various applications, from machine learning to image processing.
I encourage you to experiment with your own binary matrices and apply this technique. With practice, you'll discover the full potential of symmetric sign component decomposition.
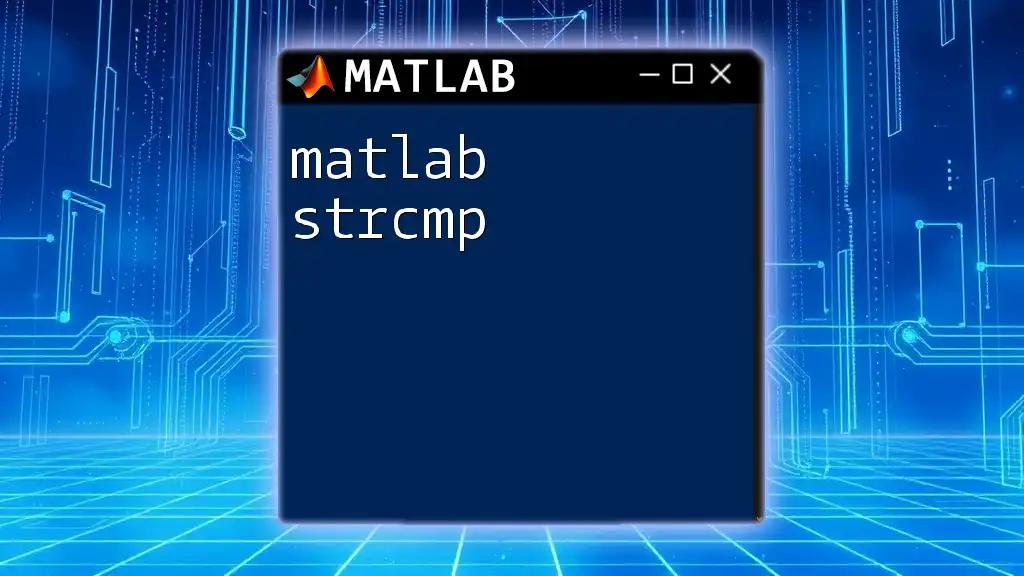
Call to Action
If you're eager to dive deeper into MATLAB and enhance your skill set, consider joining our courses designed to accelerate your learning journey! Engage with our community and share your experiences – your insights could inspire others on a similar path.
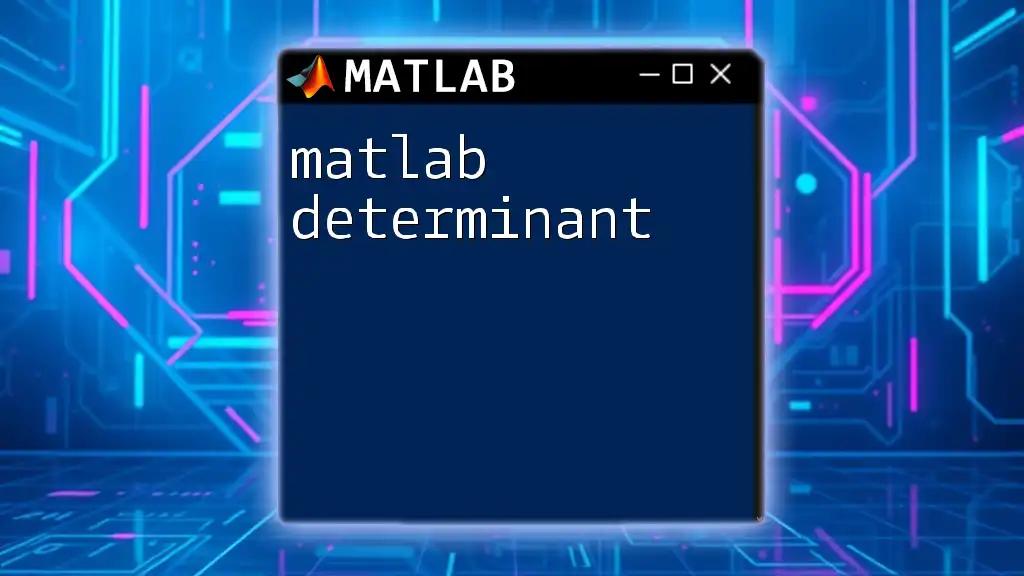
References
For further reading, look into academic texts on matrix algebra and advanced MATLAB documentation to explore more about matrix manipulation techniques and their applications in data analysis.