MATLAB syntax refers to the set of rules and conventions that dictate how commands and functions are structured in the MATLAB programming environment, allowing users to perform a variety of mathematical and engineering tasks efficiently.
Here's a simple example of MATLAB syntax to create and plot a sine wave:
t = 0:0.01:2*pi; % Define time vector
y = sin(t); % Compute sine of t
plot(t, y); % Plot the sine wave
xlabel('Time (s)'); % Label x-axis
ylabel('Amplitude'); % Label y-axis
title('Sine Wave'); % Title of the plot
grid on; % Add grid lines
What is MATLAB Syntax?
MATLAB syntax refers to the set of rules that defines how MATLAB commands are structured. Proper syntax is crucial because even small typographical errors can lead to significant consequences, including unexpected behavior or program crashes. Understanding MATLAB syntax is essential for anyone who aims to write efficient and error-free code.
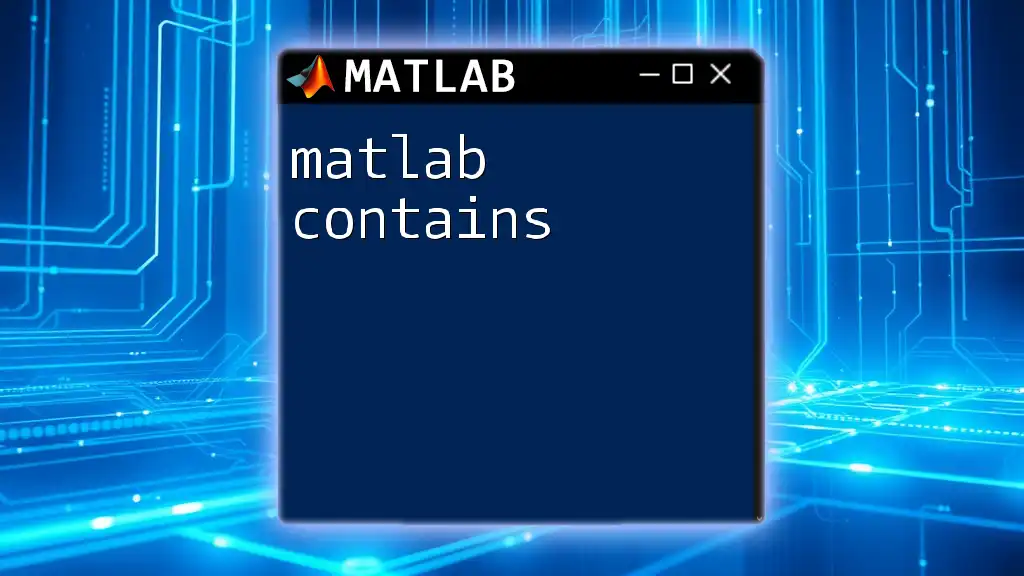
Basic Structure of MATLAB Commands
Command Format
Every MATLAB command follows a basic structure. Typically, it involves a function or operation followed by the required parameters. For instance, arithmetic operations in MATLAB are straightforward.
a = 5;
b = 10;
c = a + b; % c equals 15
In this example, `a` and `b` are assigned values, and `c` is calculated by adding `a` and `b`. It’s simple, yet effective in illustrating the command format.
Case Sensitivity
In MATLAB, variable names are case-sensitive, meaning `A` and `a` would be treated as two distinct variables. This feature emphasizes the importance of consistent naming conventions throughout your code, as it can be easy to overlook a subtle variation.
A = 10;
a = 5; % A and a are different variables
In the example, one can observe how different cases lead to the creation of separate variables `A` and `a`.
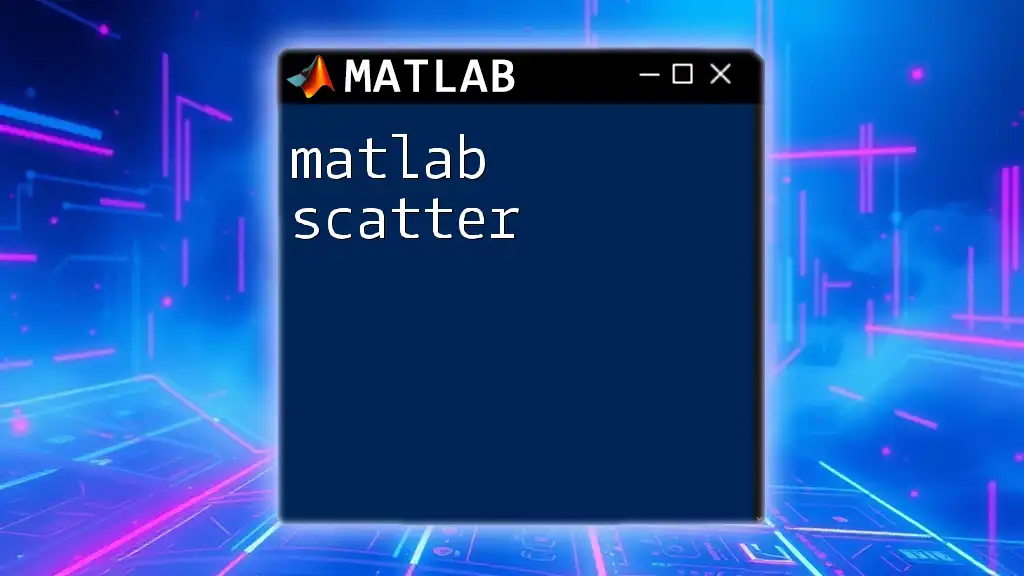
Data Types and Variable Assignment
Common Data Types
MATLAB primarily works with several fundamental data types, including scalars, vectors, matrices, and strings. Understanding these types is pivotal as they form the backbone of numerical computations in MATLAB.
-
Scalar: A single numeric value.
x = 5;
-
Vector: A one-dimensional array of numbers.
v = [1, 2, 3];
-
Matrix: A two-dimensional array of numbers.
A = [1, 2, 3; 4, 5, 6];
-
String: A sequence of characters.
str = 'Hello, MATLAB!';
Variable Assignment
Assigning variables in MATLAB is straightforward. You simply use the equals sign (=) to assign a value to a variable. It's essential to choose meaningful variable names to enhance code readability. Using descriptive names helps others (and your future self) understand the purpose of the variable at a glance.
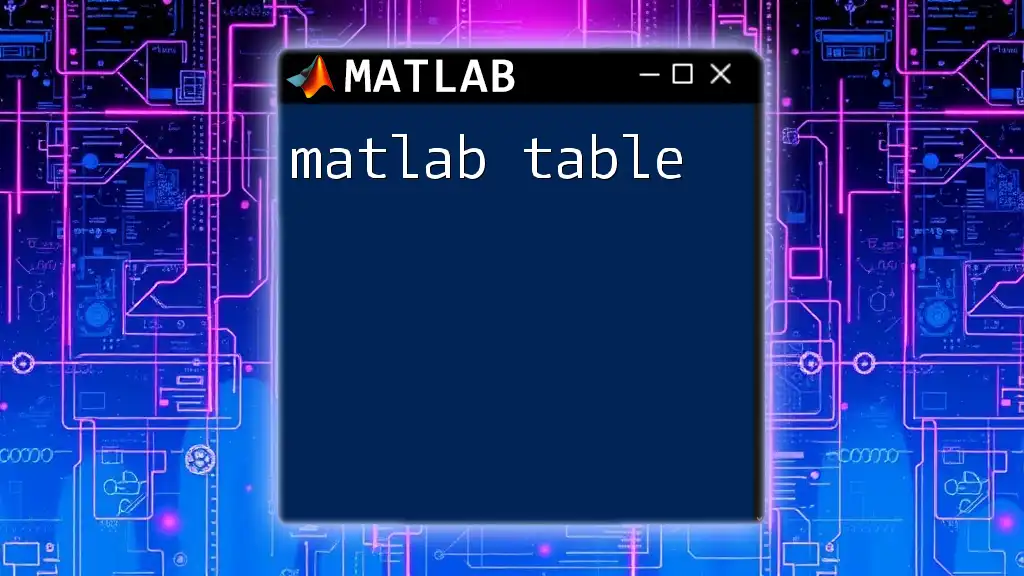
Control Structures
Conditional Statements
Conditional statements in MATLAB allow you to execute specific code blocks based on certain conditions. The most commonly used statements are `if`, `elseif`, and `else`.
x = 10;
if x < 10
disp('Less than 10');
elseif x == 10
disp('Equal to 10');
else
disp('Greater than 10');
end
In the example above, MATLAB evaluates the condition and executes the corresponding block of code, showcasing the flow of control based on the variable `x`.
Loops
Loops enable you to repeat a block of code multiple times, which is essential for tasks that require iterative calculations or operations. The two main types of loops in MATLAB are `for` and `while`.
Here’s a simple `for` loop example:
for i = 1:5
disp(i); % Displays numbers from 1 to 5
end
This loop iterates from 1 to 5 and displays each number, demonstrating an efficient way to perform repetitive tasks in a clear manner.
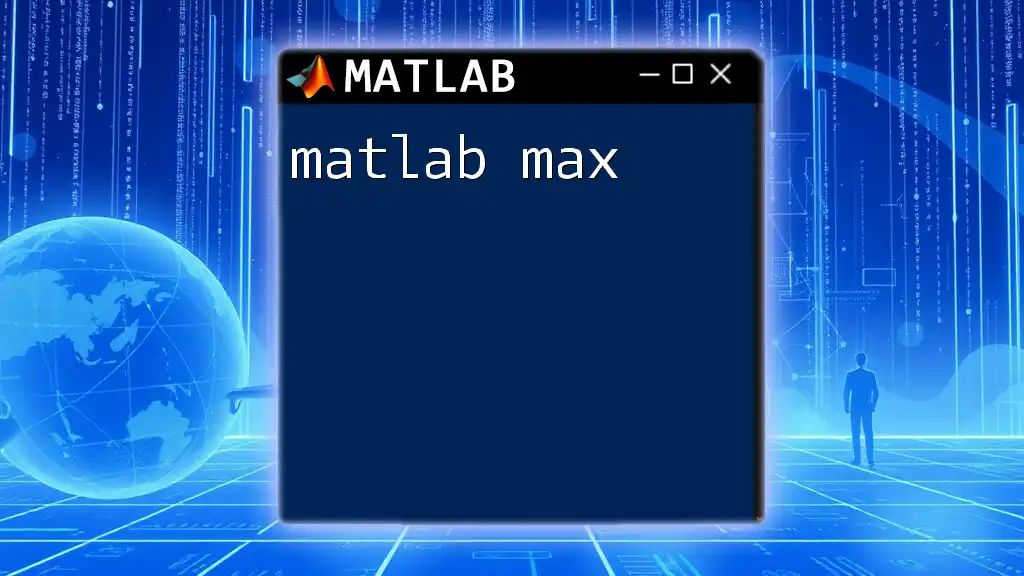
Functions and Scripts
Defining Functions
Functions are reusable pieces of code that perform specific tasks. Defining functions in MATLAB is convenient, allowing you to create modular and organized code.
function result = addNumbers(a, b)
result = a + b;
end
In this example, a function named `addNumbers` is created that takes two parameters and returns their sum.
Scripts vs. Functions
While both scripts and functions can execute code, they differ in terms of scope and usage. Scripts are collections of commands that execute in the base workspace, making them less modular. Functions, on the other hand, have their own workspace, allowing for better encapsulation of logic and reduction of errors.
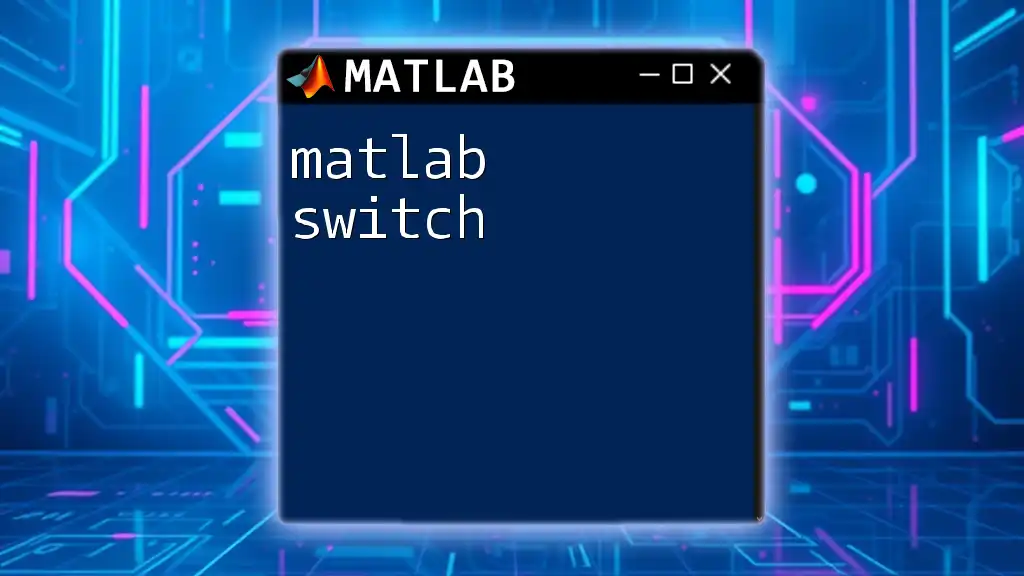
Built-in Functions and Operators
Common Built-in Functions
MATLAB provides a myriad of built-in functions to perform common mathematical operations. These functions save time and reduce coding effort.
For instance, the `sum()` function calculates the sum of elements in an array:
A = [1, 2, 3, 4];
total = sum(A); % total equals 10
This showcases how built-in functions can be leveraged for efficient computation without the need to write custom code for every operation.
Operators in MATLAB
MATLAB includes various types of operators, including:
Arithmetic Operators
These operators perform basic mathematical operations, such as addition, subtraction, multiplication, and division.
result = (2 * 3) + (4 / 2); % result equals 7
This line of code illustrates how to combine arithmetic operations in a single expression.
Relational and Logical Operators
In MATLAB, relational operators (e.g., `<`, `>`, `==`) allow for comparisons, while logical operators (e.g., `&`, `|`, `~`) facilitate the evaluation of Boolean expressions.
% Logical expression
if (x > 5) && (y < 10)
disp('Condition met');
end
This showcases the flexibility in evaluating conditions using logical statements within an if-condition.
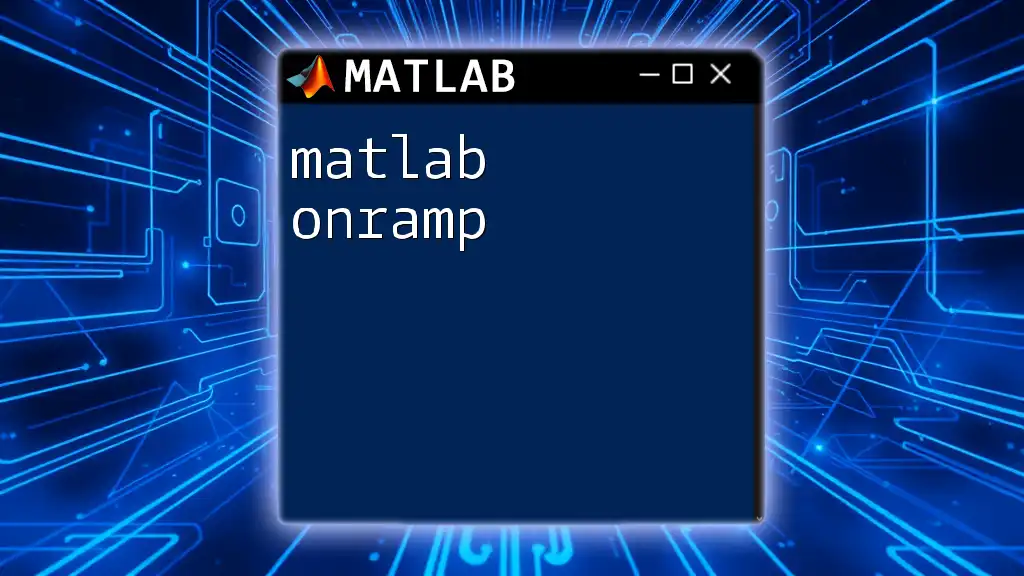
Comments and Documentation
Writing Comments
Comments are vital for explaining the purpose and functionality of your code. They help others (and future you!) understand what the code does.
In MATLAB, comments can be created using `%` for single-line comments, and `%{ %}` for block comments.
% This is a single-line comment
%{
This is a
block comment
%}
Using comments effectively increases code readability and maintainability.
Documenting Functions
Proper documentation is crucial for any function to explain what it does, the parameters it takes, and the return values. Including help text in function definitions can aid other users in understanding how to utilize your code effectively.
function result = addNumbers(a, b)
% addNumbers Adds two numbers
% result = addNumbers(a, b) returns the sum of a and b.
result = a + b;
end
In this example, the help text clarifies the function's purpose and guides users on its use.
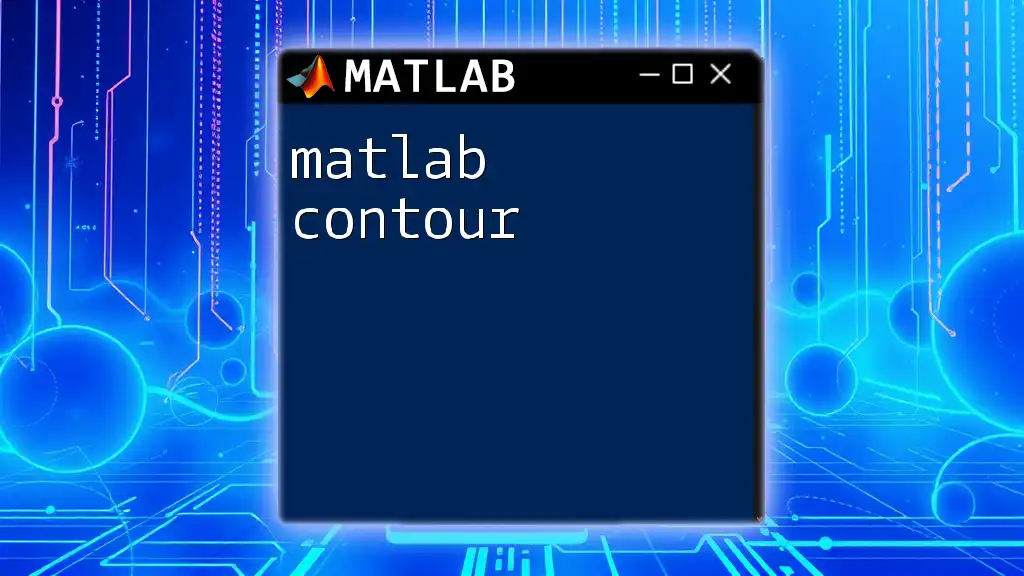
Error Handling in MATLAB
Common Errors
When working with MATLAB syntax, it's common to encounter errors, such as syntax errors, runtime errors, and logical errors. Being aware of these can help you easily identify and resolve issues in your code.
Using `try-catch` Statements
To handle errors gracefully, MATLAB provides the `try-catch` blocks, which allow you to catch errors and continue execution without crashing your program.
try
x = 1 / 0; % This will cause an error
catch
disp('An error occurred!');
end
In this example, if the operation inside the `try` block causes an error, the code execution will jump to the `catch` block, allowing for error management and troubleshooting.
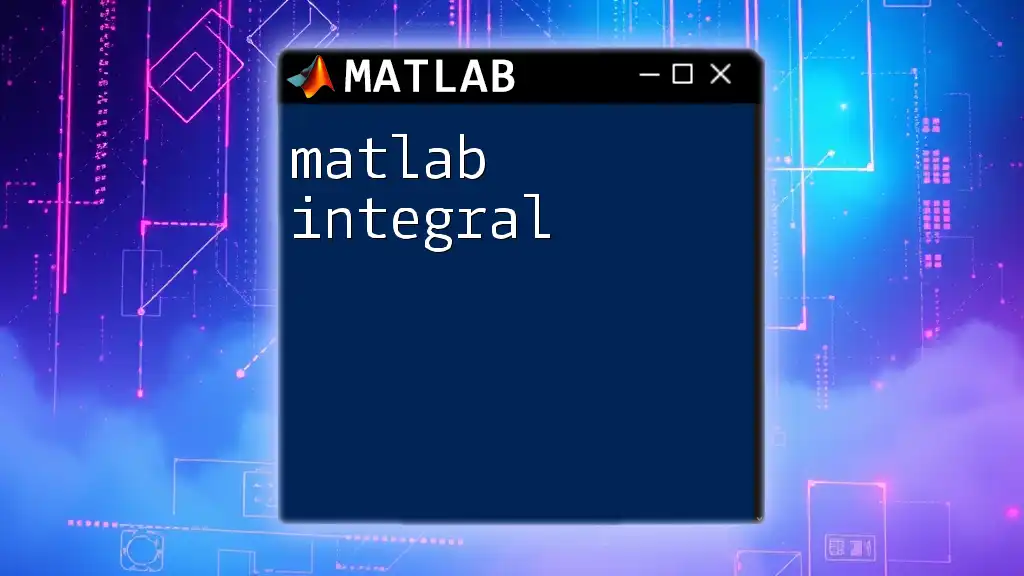
Conclusion
Mastering MATLAB syntax is crucial for writing efficient, error-free programs. From understanding the basic structure of commands to utilizing built-in functions and handling errors, each aspect plays a vital role in the programming process. By adhering to good practices like documenting your code and using meaningful variable names, you can make your programming experience in MATLAB smooth and productive.
Continue practicing, experimenting, and building your skills in MATLAB to become a more proficient user and programmer!
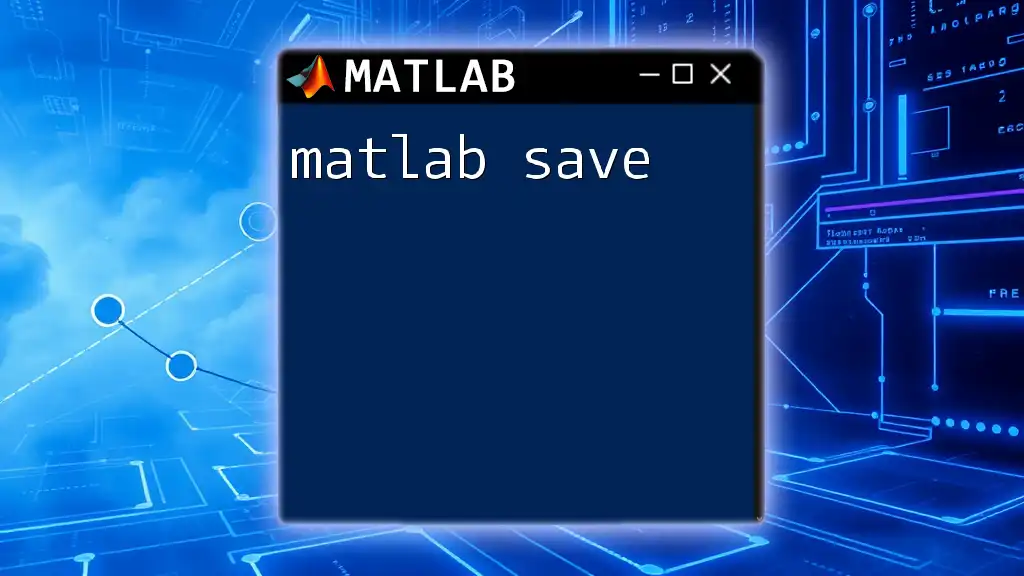
Additional Resources
- To further enhance your MATLAB knowledge, consider visiting [MATLAB Documentation](https://www.mathworks.com/help/matlab/) for in-depth materials and guides.
- Exploring online courses or textbooks can provide structured learning opportunities for mastering MATLAB syntax and its applications.