The `unwrap` function in MATLAB is used to remove discontinuities in phase data by adding multiples of \(2\pi\) to the phase angles, ensuring smooth transitions.
Here is a code snippet demonstrating its usage:
% Example of MATLAB unwrap function
phaseData = [0, pi/2, -pi, -3*pi/2, pi]; % Example phase data
unwrappedData = unwrap(phaseData); % Unwrap the phase data
disp(unwrappedData); % Display the unwrapped phase data
What is Phase Unwrapping?
Definition
Phase unwrapping refers to the process of converting a wrapped phase representation into a continuous phase representation. In many applications, especially in signal processing, signals can undergo transformations that result in phase angles being wrapped into a limited range, typically from \(-\pi\) to \(\pi\). This wrapping can obscure the true phase information, leading to misinterpretations when analyzing signals.
Applications
Unwrapping the phase is particularly vital in a variety of fields including:
- Communication Systems: Accurate phase information is crucial for demodulating signals, especially in complex modulation schemes.
- Radar Signal Processing: In radar applications, phase unwrapping helps in determining the target motion accurately.
- Audio Signal Analysis: Unwrapping enhances the clarity of the phase information, helping in effects such as phase vocoding or sound synthesis.
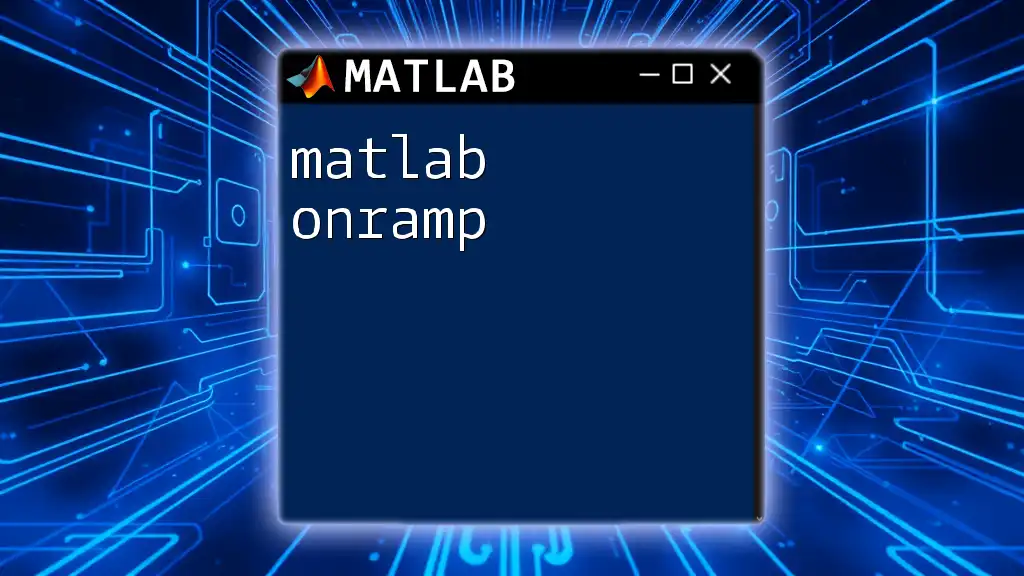
Understanding MATLAB’s `unwrap` Function
Syntax
The basic syntax of the `unwrap` function in MATLAB is straightforward:
Y = unwrap(P)
In this syntax:
- `P`: Input phase angles, which can be a vector or a matrix.
- `Y`: The output unwrapped phase angles, maintaining the same size as the input.
Input Parameters
The `unwrap` function in MATLAB can handle various input types:
- It accepts both 1-D and multi-dimensional arrays.
- When dealing with multi-dimensional arrays, `unwrap` processes input column-wise by default.
Output
The output of the `unwrap` function is an array of the same size as the input, where the phase angles are "unwrapped," meaning they are adjusted such that the differences between adjacent elements do not exceed \(\pi\).
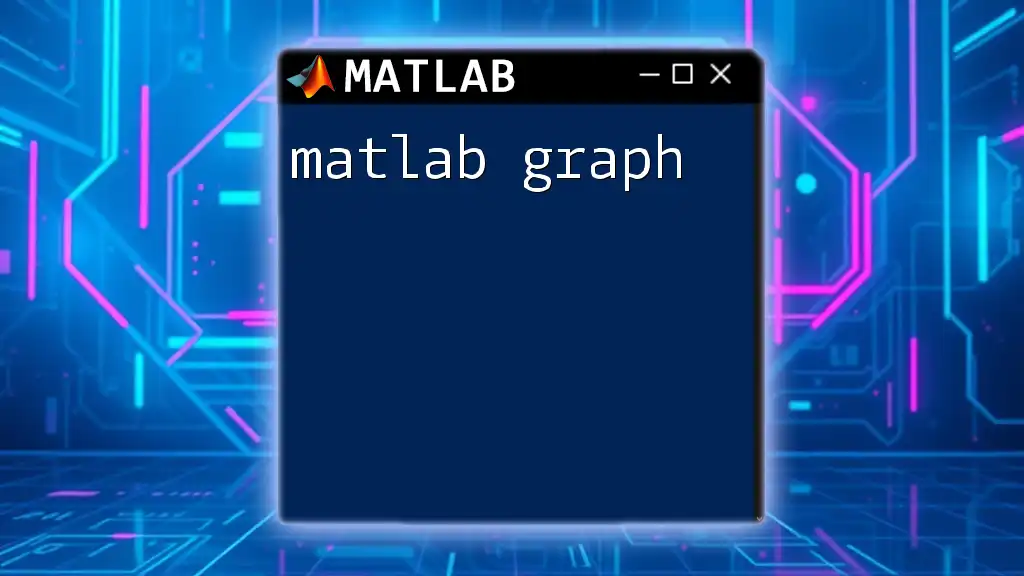
How `unwrap` Works
The Algorithm
The underlying algorithm of `unwrap` is based on adding or subtracting multiples of \(2\pi\) to neighboring angles to ensure that transitions between values do not jump across the wrapped boundary. For example, if the current angle is near \(\pi\) and the next one is near \(-\pi\), then it unpacks the next angle by adding \(2\pi\) to create a smoother transition.
Example of Unwrapping
Consider an array of phase angles where discontinuities might exist:
phase = [0, pi/2, -pi, 3*pi/2, -3*pi/2];
unwrapped_phase = unwrap(phase);
In this example:
- The original wrapped phase angles contain a jump from \(-\pi\) to \(3\pi/2\).
- The `unwrap` function resolves this by adding \(2\pi\) to ensure a continuous transition, resulting in a more interpretable phase representation.
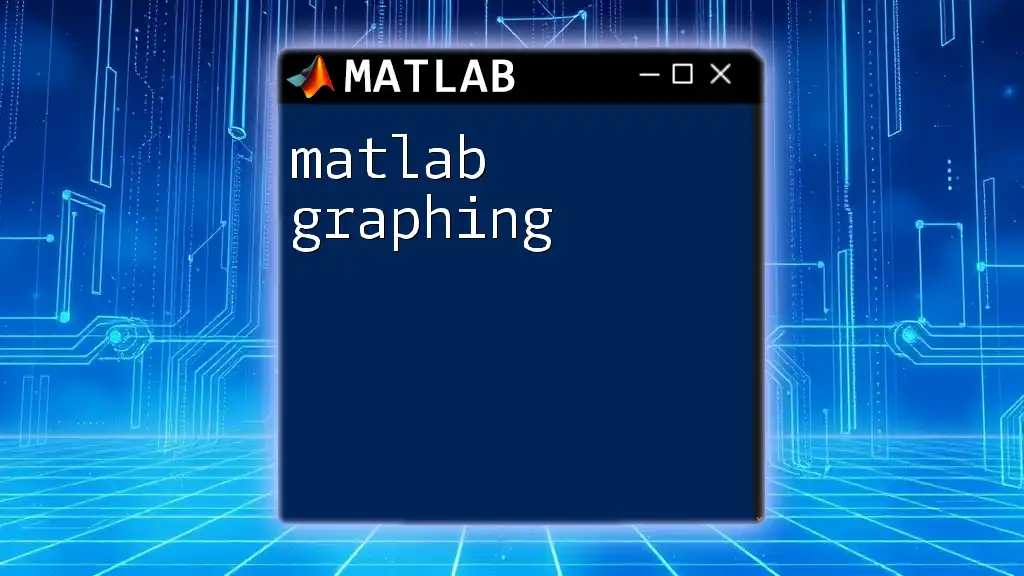
Practical Use Cases
Use Case 1: Signal Processing
In modern signal processing, the unwrapped phase is vital for accurate interpretation. Here’s how you might use `unwrap` in practice:
t = 0:0.01:1;
sampled_signal = sin(2*pi*10*t) + 0.1*randn(size(t)); % noisy signal
phase_signal = angle(hilbert(sampled_signal)); % Hilbert transform
unwrapped_phase_signal = unwrap(phase_signal);
In this case:
- The signal undergoes a Hilbert transform to obtain the phase.
- By applying `unwrap`, the code produces a representation of the phase that eliminates discontinuities, allowing for better analysis of the signal's dynamics.
Use Case 2: 2D Phase Data
Introduction to 2D Data
When dealing with two-dimensional matrices, phase unwrapping can be crucial, particularly in imaging applications where phase information is derived from pixels.
Example Code for 2D Unwrapping
[X,Y] = meshgrid(-2*pi:0.1:2*pi, -2*pi:0.1:2*pi);
Z = sin(X + Y);
wrapped_phase = atan2(Z, Z);
unwrapped_phase_2D = unwrap(wrapped_phase);
This example illustrates:
- How to create a grid and calculate a phase representation using `atan2`.
- The `unwrap` function is applied on the 2D matrix to resolve any wrapped phases, resulting in a clear mapping of phase information over the area defined by `X` and `Y`.
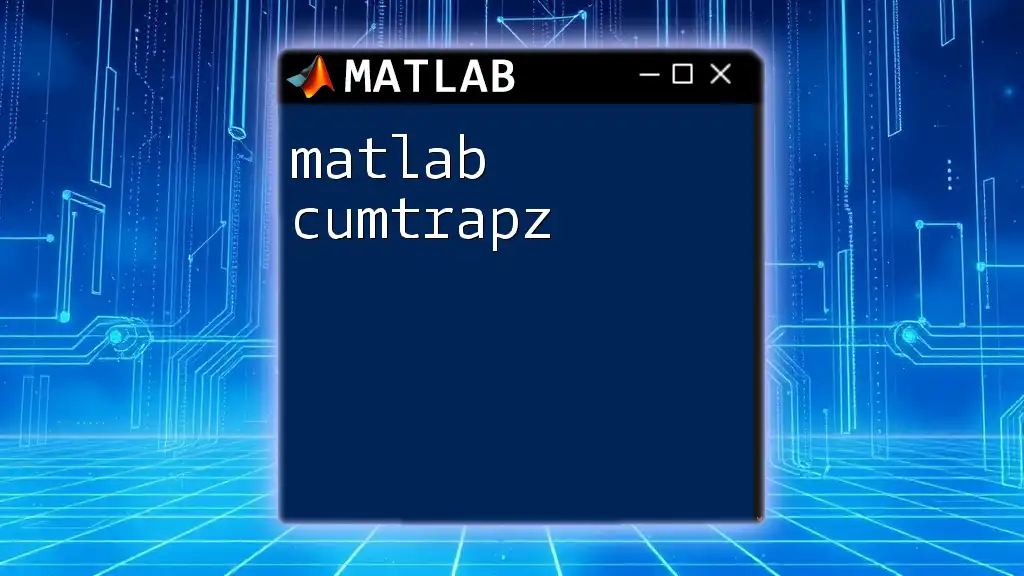
Common Pitfalls and Troubleshooting
Common Issues
When using `unwrap`, it is essential to consider how phase wrapping might be misinterpreted. Some common mistakes include:
- Misreading the values due to abrupt jumps in the phase information.
- Failing to recognize that unwrapping may lead to unexpected results if the phase data is not appropriately acquired.
Solutions
To ensure optimal use of `unwrap`, consider:
- Always visualizing the results of the unwrapped phase to verify continuity.
- Testing the function with controlled inputs to see how `unwrap` behaves.
- Engaging in debugging using simpler scenarios before tackling complex datasets.
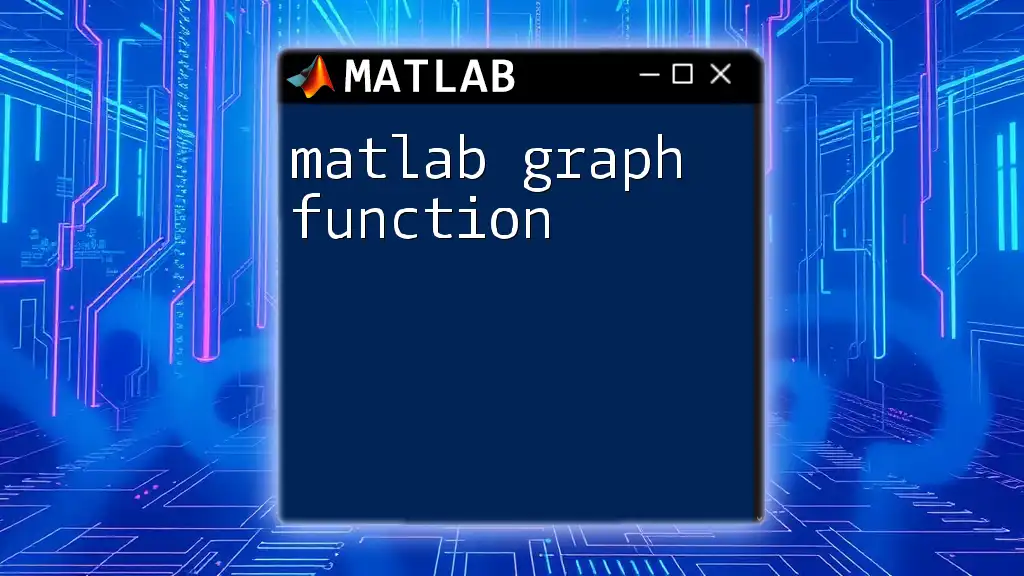
Performance Considerations
Speed and Efficiency
The computational efficiency of `unwrap` can be influenced by the size of the input data. Large datasets may incur performance penalties; thus, consider:
- Preprocessing data to reduce dimensionality.
- Using logical indexing to limit the scope of the `unwrap` operations.
Alternatives to `unwrap`
Although `unwrap` is effective, alternative MATLAB functions can also handle phase data, such as:
- `angle`: For directly retrieving the angle of complex numbers.
- `imag`: For working with imaginary components.
Choosing the right approach depends on the specific application needs. If phases are continuously increasing or decreasing, `unwrap` is generally the best choice.
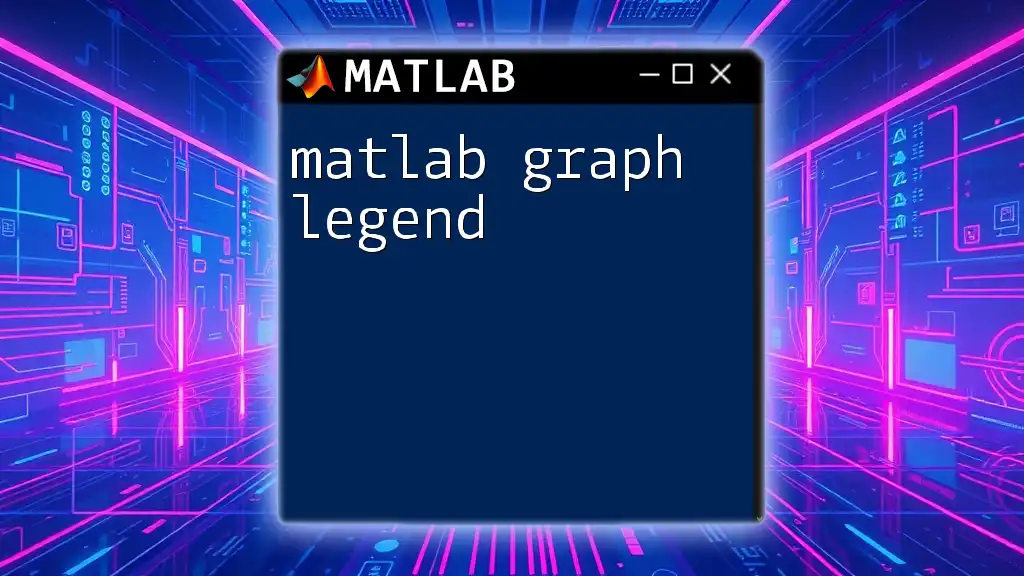
Conclusion
The `unwrap` function in MATLAB is a powerful tool for dealing with phase information in various applications, particularly in signal processing and imaging. By mastering this function, users can better interpret and manipulate phase data, ultimately leading to more accurate analyses and improved outcomes in their projects.
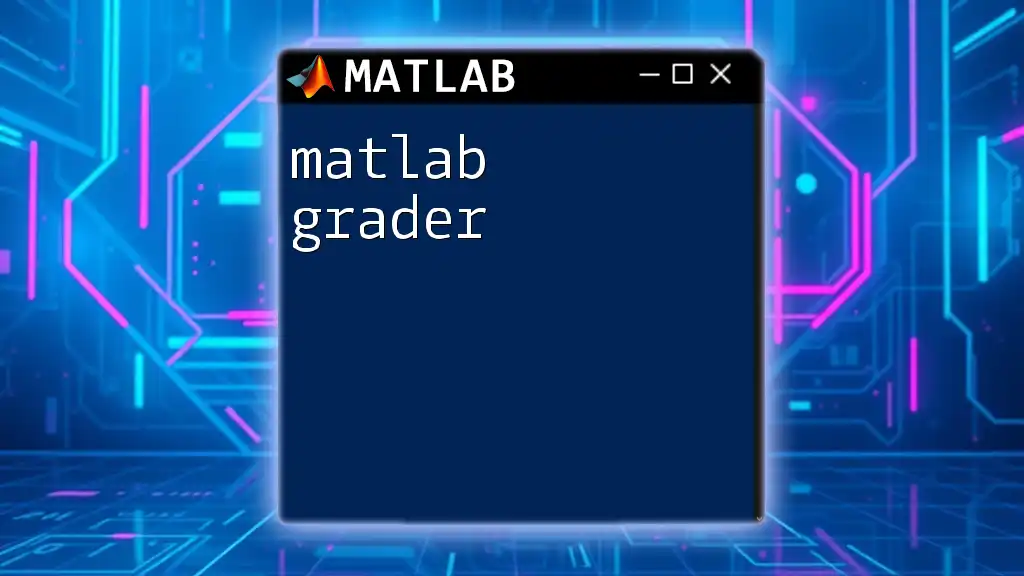
Additional Resources
Further Reading
To expand your understanding, refer to the official MATLAB documentation on `unwrap`, as well as relevant textbooks that cover signal processing principles in depth.
Community and Support
Joining forums, GitHub repositories, or MATLAB user groups can provide additional insights and assistance, allowing users to engage with experienced professionals and learners sharing similar interests.
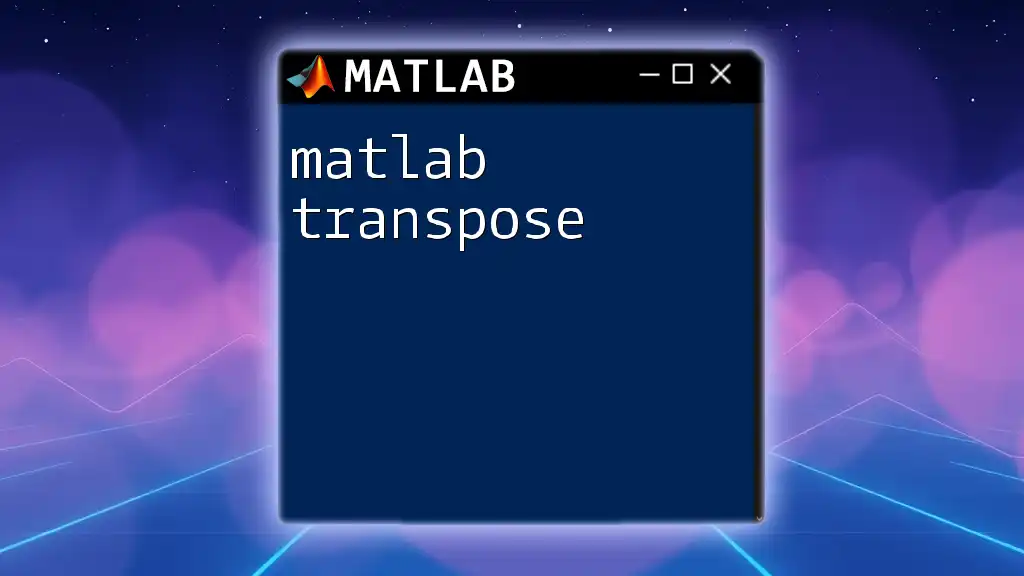
Call to Action
Consider signing up for specialized courses on MATLAB commands to enhance your skills in phase analysis and many other MATLAB features. If you have questions about the `unwrap` function or want to share your experiences, feel free to engage with the community by leaving comments or inquiries!