In MATLAB, squaring a number can be done simply by using the exponentiation operator (`.^`), allowing for both scalar and array operations.
Here’s a code snippet demonstrating how to square a number and an array:
% Squaring a number
num = 5;
squaredNum = num^2;
% Squaring an array
arr = [1, 2, 3, 4];
squaredArr = arr.^2;
Understanding Squaring in MATLAB
What Does Squaring Mean?
Squaring a number refers to the operation of multiplying that number by itself, mathematically represented as \( x^2 \). This operation plays a critical role in various mathematical calculations, including those encountered in engineering and scientific research.
Why Use MATLAB for Squaring?
MATLAB stands out as a powerful tool for mathematical computations due to its built-in capabilities, extensive functions, and user-friendly environment. The benefits of using MATLAB for squaring operations include speed and efficiency, especially when handling large datasets or performing complex calculations. Furthermore, the ability to visualize results makes MATLAB an ideal choice for students and professionals alike.
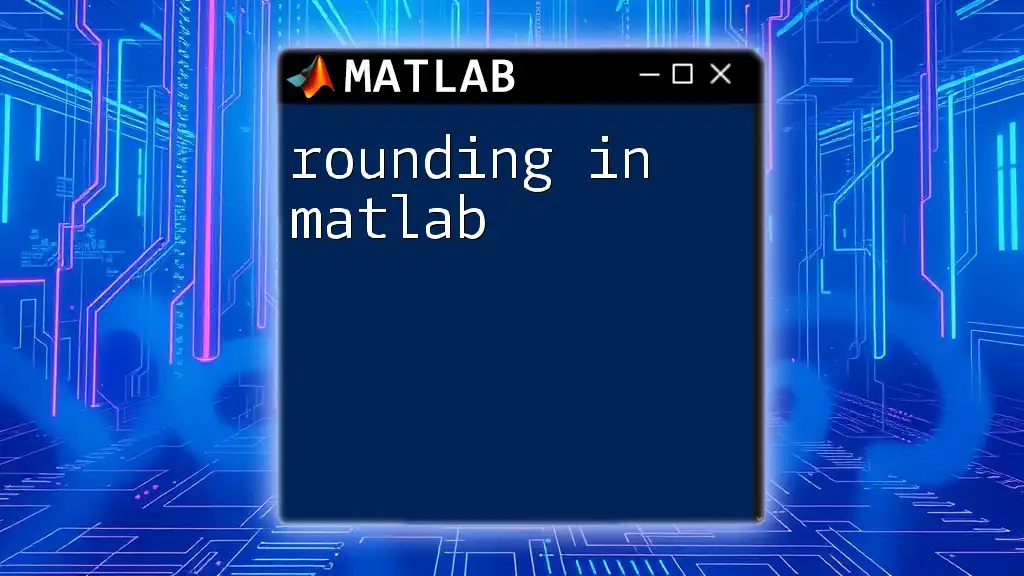
Basic Syntax for Squaring in MATLAB
Using the Power Operator
In MATLAB, the most straightforward way to square a number is by using the power operator. The syntax is simple:
result = x^2
This command calculates the square of the variable `x`. Here's an example to illustrate its usage:
x = 5;
result = x^2;
disp(result); % Output: 25
This snippet assigns the value 5 to `x`, squares it, and outputs the result (which is 25).
Using the `power` Function
Another method for squaring a number in MATLAB is by utilizing the built-in `power` function. This method is particularly useful when you want to clarify that you are performing a power operation. The syntax for this function is:
result = power(x, 2)
Here’s an example:
x = 4;
result = power(x, 2);
disp(result); % Output: 16
In this case, the `power` function explicitly states that you are raising `x` to the second power, which results in 16.
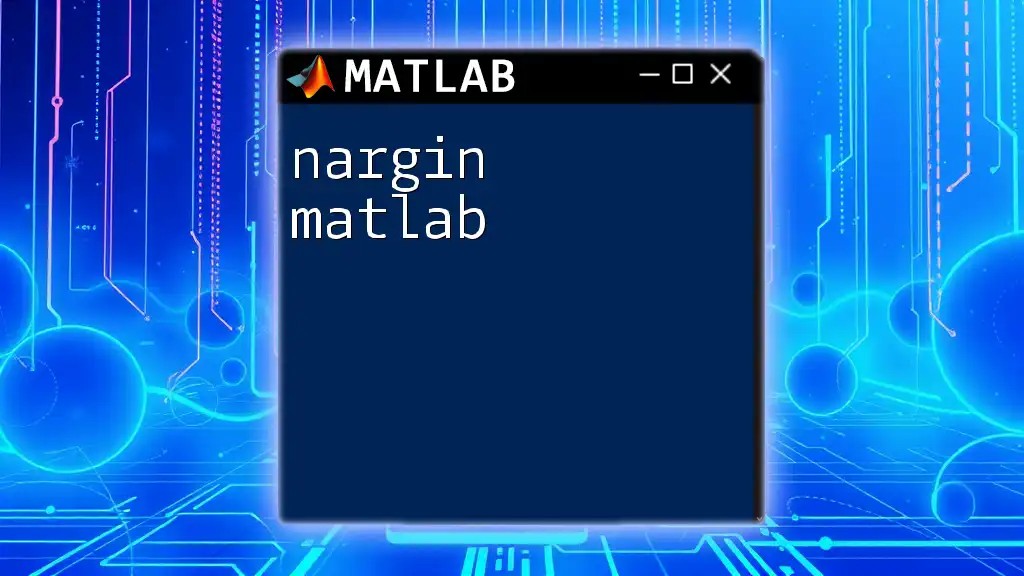
Squaring Arrays and Matrices
Element-wise Squaring
MATLAB also allows squaring of arrays element-wise, which means that each individual element in the array is squared independently. For this operation, you will need to use the element-wise operator `.^`. The syntax is:
result = x.^2
Here’s an example of how to perform element-wise squaring:
x = [1, 2, 3];
result = x.^2;
disp(result); % Output: 1 4 9
In this example, each element of the array `x` is squared, yielding the result `[1, 4, 9]`.
Squaring a Matrix
When it comes to squaring a matrix, you have to approach it differently. Squaring a matrix refers to matrix multiplication of the matrix with itself. The syntax is as follows:
result = x * x
Here's how this works in practice:
A = [1, 2; 3, 4];
result = A * A;
disp(result);
% Output:
% 7 10
% 15 22
This code snippet demonstrates how the matrix `A` is multiplied by itself, which gives a new matrix as the output. The resulting matrix contains the sums of the products of the rows and columns of the original matrix.
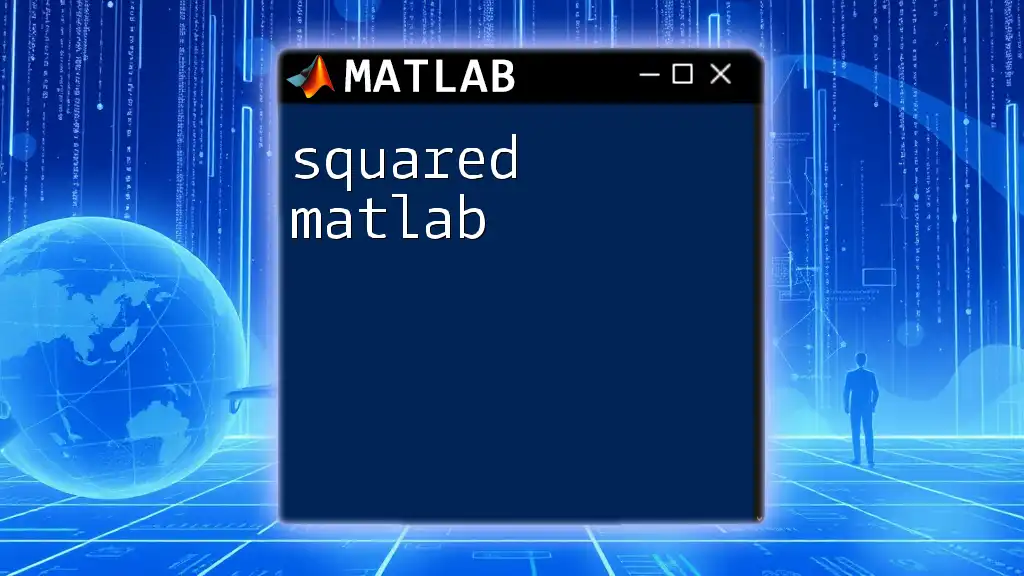
Advanced Squaring Techniques
Using Anonymous Functions
For more advanced applications, you may create anonymous functions to perform squaring operations. This allows for more flexibility in your computations. The syntax to create an anonymous function for squaring is:
square = @(x) x.^2
Here is an example of using this function:
square = @(x) x.^2;
result = square(6);
disp(result); % Output: 36
In this snippet, we define an anonymous function `square` that squares any input, and then we apply it to the value 6.
Handling Complex Numbers
MATLAB also effectively manages complex numbers. Squaring a complex number involves using the same power operator or function, but the result will be a complex value. For instance:
z = 3 + 4i; % 4i represents the imaginary unit
result = z^2;
disp(result); % Output: -7 + 24i
This way, the syntax remains consistent, yet it requires an understanding of complex number rules, which makes MATLAB extremely versatile for mathematical computations.
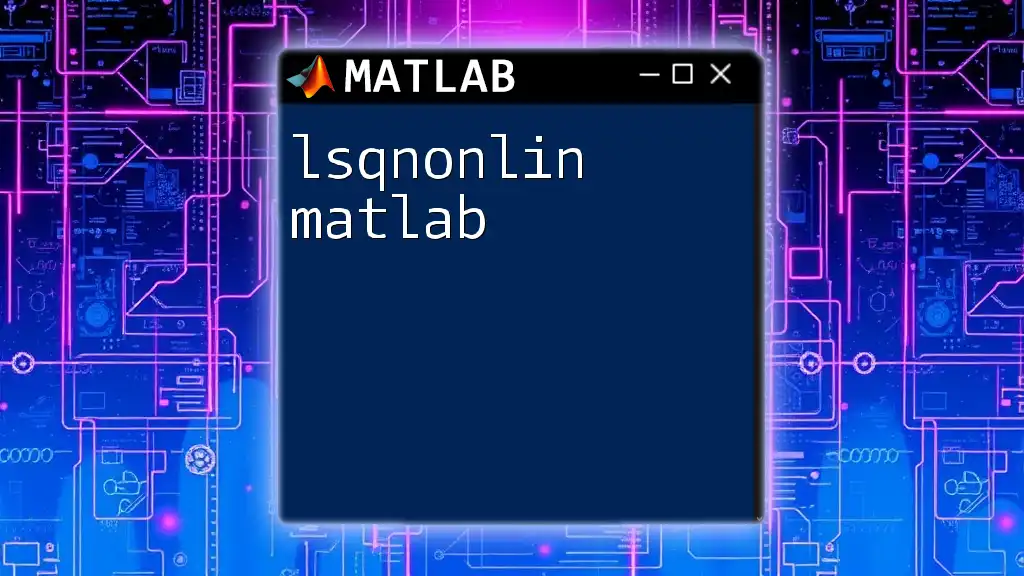
Common Mistakes and Troubleshooting
Frequent Errors When Squaring
When working with squaring in MATLAB, novice users often encounter mistakes, primarily stemming from misunderstanding operator precedence.
One common error is confusing the element-wise power operator (`.^`) with the matrix power operator (`^`). Remember, the element-wise operator should be used when squaring arrays, while the matrix operator is used only for squaring square matrices.
Debugging Squaring Operations
To debug squaring operations effectively, the display functions like `disp()` and `fprintf()` can be quite helpful. They allow you to check values at various points in your code, ensuring that variables contain the expected results before proceeding.
Utilizing breakpoints inside your code can also enable step-by-step execution, allowing you to observe how variables change at each step and identify where an error may have occurred.
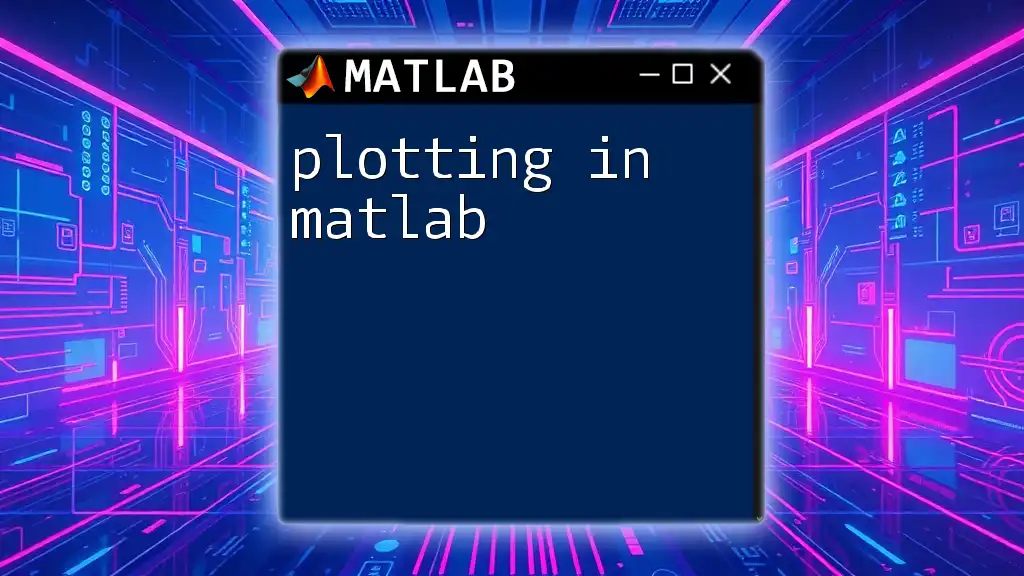
Conclusion
In conclusion, squaring in MATLAB encompasses various methods and techniques, from basic power operations and functions to more advanced applications like element-wise squaring and handling complex numbers. Mastering these squaring operations will significantly enhance your MATLAB skills and your overall mathematical capabilities in programming and computation.
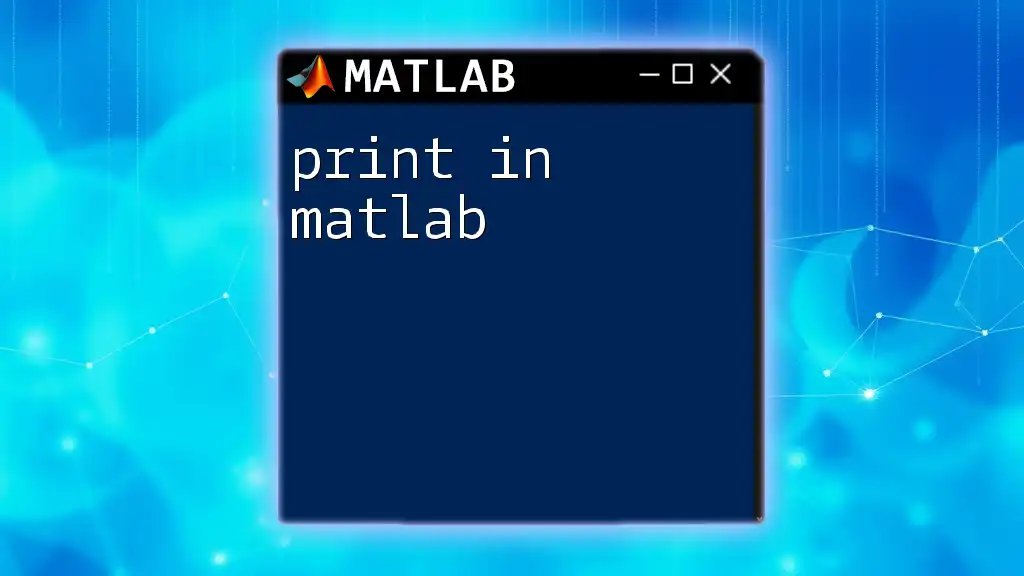
Additional Resources
For those looking to deepen their understanding of squaring and other mathematical operations in MATLAB, consider exploring the official MATLAB documentation for extensive examples and explanations. Additionally, numerous online resources, forums, and communities can provide further insights into advanced MATLAB functionalities.
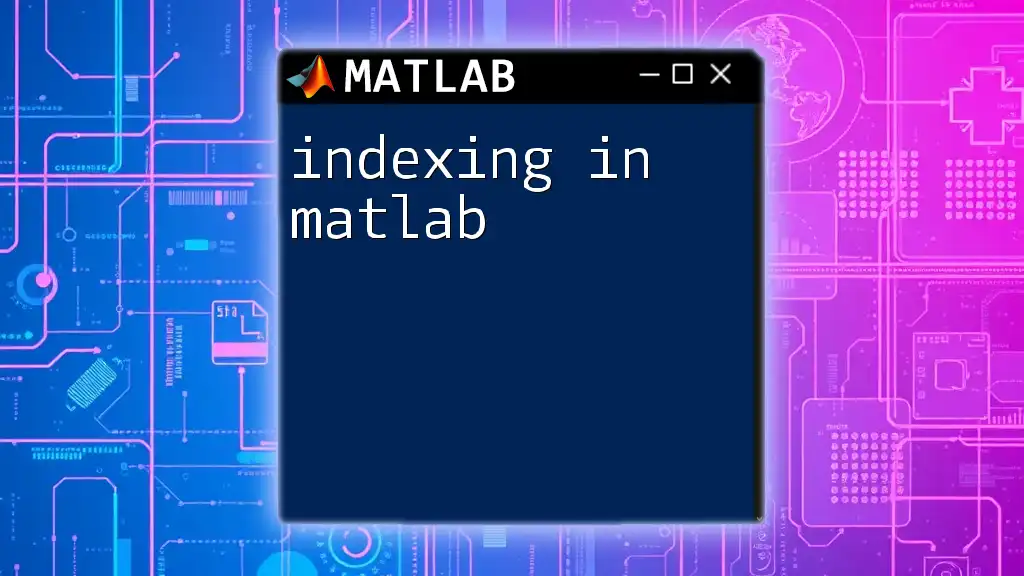
Call to Action
If you're eager to enhance your MATLAB skills and learn hands-on with practical examples, sign up for our comprehensive courses that provide in-depth lessons on squaring and other essential MATLAB commands. Join us today to take your MATLAB expertise to the next level!