A moving average filter in MATLAB is used to smooth data by averaging a set number of samples, significantly reducing noise and fluctuations.
Here's a simple code snippet to implement a moving average filter:
% Example of a Moving Average Filter in MATLAB
data = [5, 6, 7, 8, 6, 5, 4, 3, 2, 1]; % Sample data
windowSize = 3; % Specify the window size
movAvg = filter(ones(1, windowSize) / windowSize, 1, data); % Apply moving average filter
disp(movAvg); % Display the result
What is a Moving Average Filter?
Definition
A moving average filter is a statistical tool used to smooth data by creating averages over a specific number of points from a dataset. It helps in reducing noise and enhancing signal quality in various applications. There are different types of moving averages: simple, weighted, and exponential, each serving unique purposes based on the requirement.
Applications
Moving average filters find their relevance in several fields, including:
- Signal Processing: Smoothing out rapid fluctuations in signal data.
- Time Series Analysis: Identifying trends and cyclical patterns over time.
- Image Processing: Reducing noise and blurring images to improve visuals.
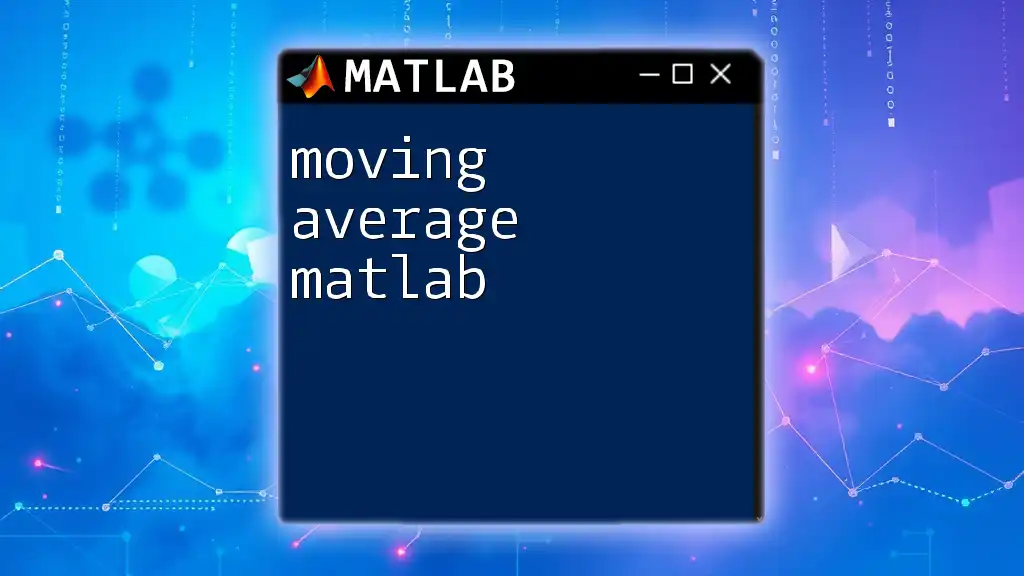
Understanding the Theory Behind the Moving Average Filter
Mathematical Concept
At its core, the moving average filter computes the average of a dataset over a specified window or number of preceding points, iteratively recalculating as it moves through the data. This operation is powerful in minimizing random variations and highlighting actual trends.
Types of Moving Average Filters
Simple Moving Average (SMA)
A Simple Moving Average is calculated by taking the unweighted mean of the most recent data points. SMA is often preferred when the data has no inherent weighting, and it offers straightforward computation. However, it may not react quickly to changes in the dataset due to its averaging nature.
Weighted Moving Average (WMA)
A Weighted Moving Average assigns different weights to the data points, emphasizing the more recent values. This filtering method is beneficial when you believe later observations are more likely to be representative of the underlying trend. The formula adjusts based on the assigned weights, providing more nuanced results than SMA.
Exponential Moving Average (EMA)
The Exponential Moving Average applies a bias that favors recent data points but does so exponentially. Unlike SMA, in which all observations in the window are treated equally, EMA reacts more significantly to newer data. It is particularly useful in trading and forecasting scenarios where recent trends are pivotal.
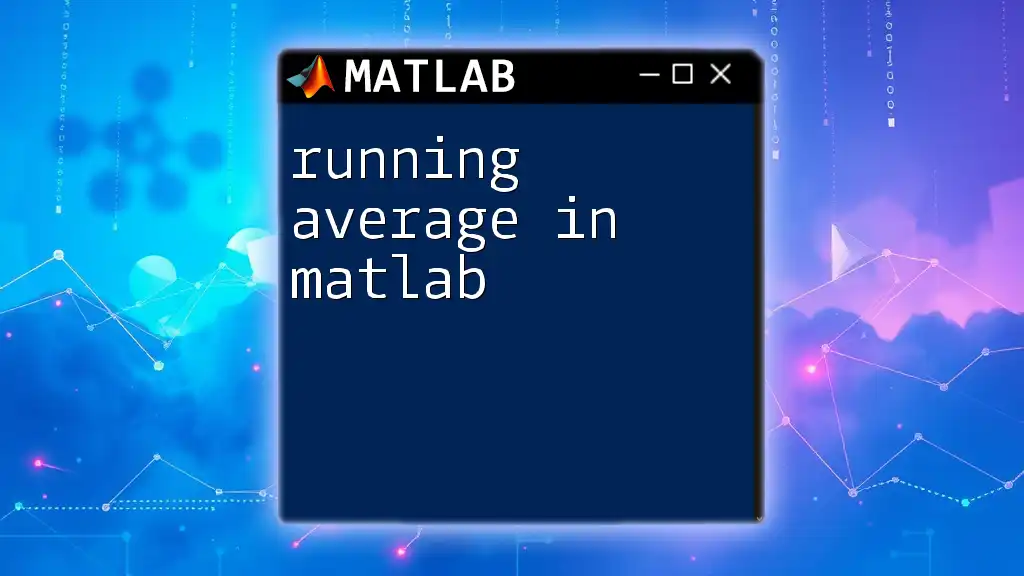
Implementing Moving Average Filter in MATLAB
Setting Up Your Environment
To get started, ensure you have MATLAB installed on your system. Open MATLAB and create a new script or function file where you can write your code snippets for implementing the moving average filter.
Basic Syntax for Moving Average Filter
Simple Moving Average Implementation
The simplest implementation of a moving average filter in MATLAB can be through the `movmean` function. Here is an example:
% Example of Simple Moving Average
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
windowSize = 3;
sma = movmean(data, windowSize);
This code computes the simple moving average of the `data` array with a defined window size of 3.
Weighted Moving Average Implementation
For a weighted approach, we can manually apply weights to the moving average as follows:
% Example of Weighted Moving Average
weights = [0.1, 0.3, 0.6]; % Assigning weights
wma = filter(weights, sum(weights), data);
This implementation emphasizes more recent values due to the weights assigned, leading to a more responsive moving average.
Exponential Moving Average Implementation
To compute the Exponential Moving Average, you can use a straightforward loop or vectorized method:
% Example of Exponential Moving Average
alpha = 0.1; % Smoothing factor
ema = zeros(size(data));
ema(1) = data(1);
for i = 2:length(data)
ema(i) = alpha * data(i) + (1 - alpha) * ema(i-1);
end
This loop initializes the first value and then recursively calculates the EMA for the entire dataset.
Visualizing the Results
Once you have computed the moving averages, it’s essential to visualize the results to understand the smoothing effect better. You can use MATLAB’s plotting functions as follows:
plot(data, 'b--', 'DisplayName', 'Original Data');
hold on;
plot(sma, 'r', 'DisplayName', 'Simple Moving Average');
plot(wma, 'g', 'DisplayName', 'Weighted Moving Average');
plot(ema, 'k', 'DisplayName', 'Exponential Moving Average');
legend show;
hold off;
This code snippet generates a plot with the original data and all three moving averages for comparison.
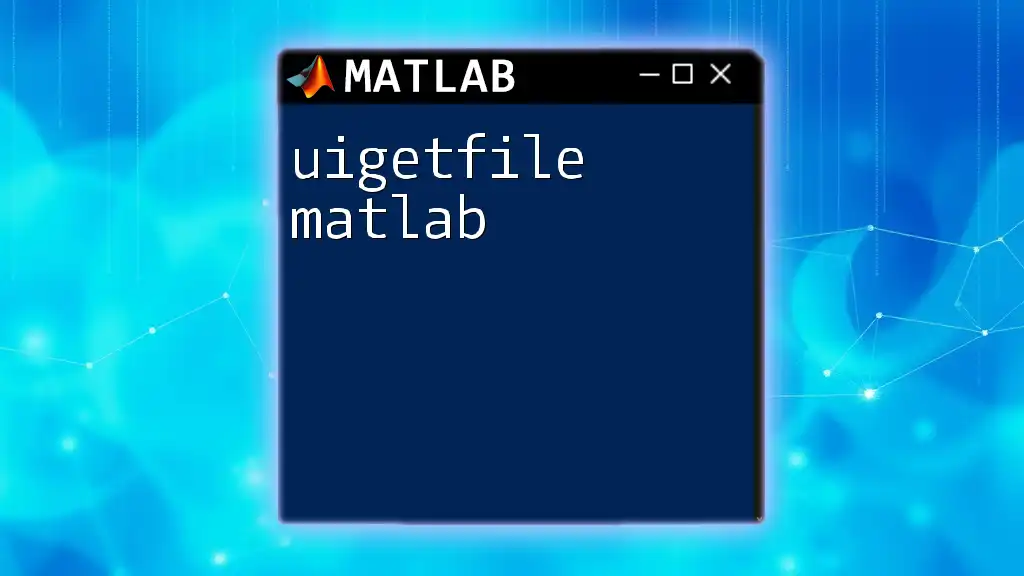
Applications of Moving Average Filters in MATLAB
Signal Processing Applications
In signal processing, moving average filters are instrumental in denoising signals, thereby extracting valuable information. For instance, if you have a signal with high-frequency noise, you can apply a moving average filter to achieve a clearer output.
Time Series Analysis
In financial markets, moving averages are extremely useful for trend analysis. They help identify the direction of a trend and assist with decisions regarding buying or selling assets. Implementing SMA or EMA in MATLAB can provide insights through historical price data analysis.
Image Processing
Moving averages can also be utilized in image processing to smooth images, thereby mitigating noise. By applying a 2D moving average filter on an image matrix, you can enhance its quality, making it visually appealing. For example, you might represent an image as a 2D array and use MATLAB’s `conv2` function to apply the moving average.
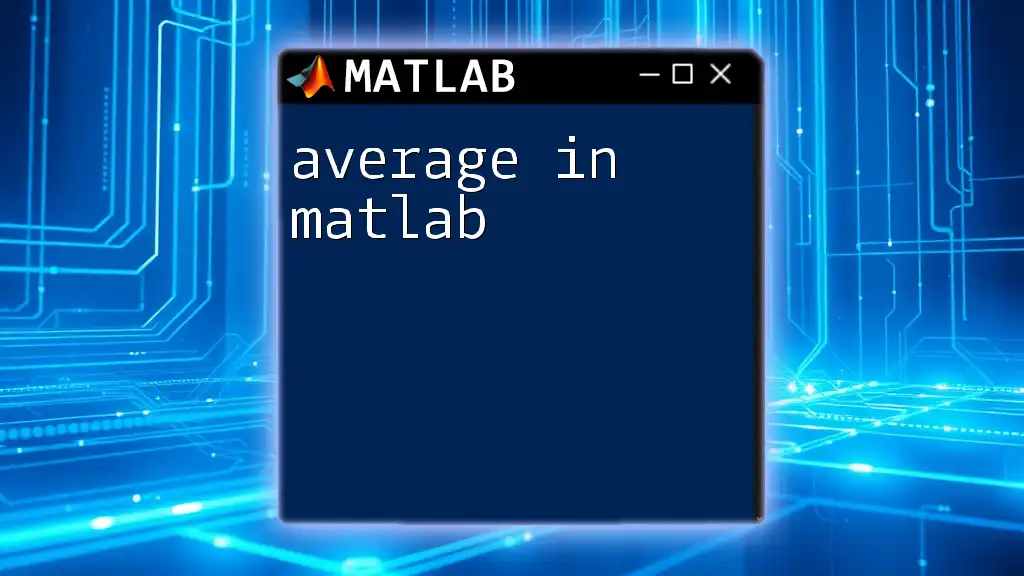
Best Practices & Tips
Choosing the Right Window Size
Selecting an appropriate window size is crucial, as it directly impacts the smoothing effect. A larger window size may over-smooth data, eliminating meaningful variations, while a smaller window size may not effectively reduce noise. Experimentation is often required to strike the right balance.
Performance Considerations
Ensure that your implementation is efficient, especially for larger datasets. Use built-in MATLAB functions such as `movmean` and `filter`, as they are optimized for performance. Avoid explicit loops when possible – vectorized operations are preferred for quicker execution.
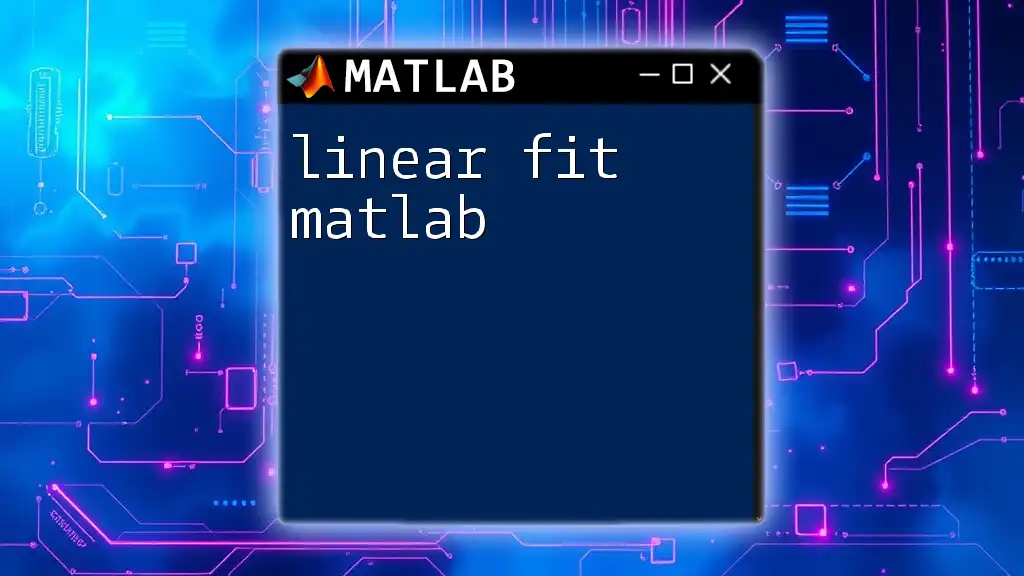
Troubleshooting Common Issues
Common Errors and Solutions
When implementing a moving average filter, you might encounter errors regarding matrix dimensions or indexing issues. Always ensure that your data is adequately formatted and that the dimensions are compatible with the operations you are performing. If you run into any issues, MATLAB’s error messages can provide insights for troubleshooting.
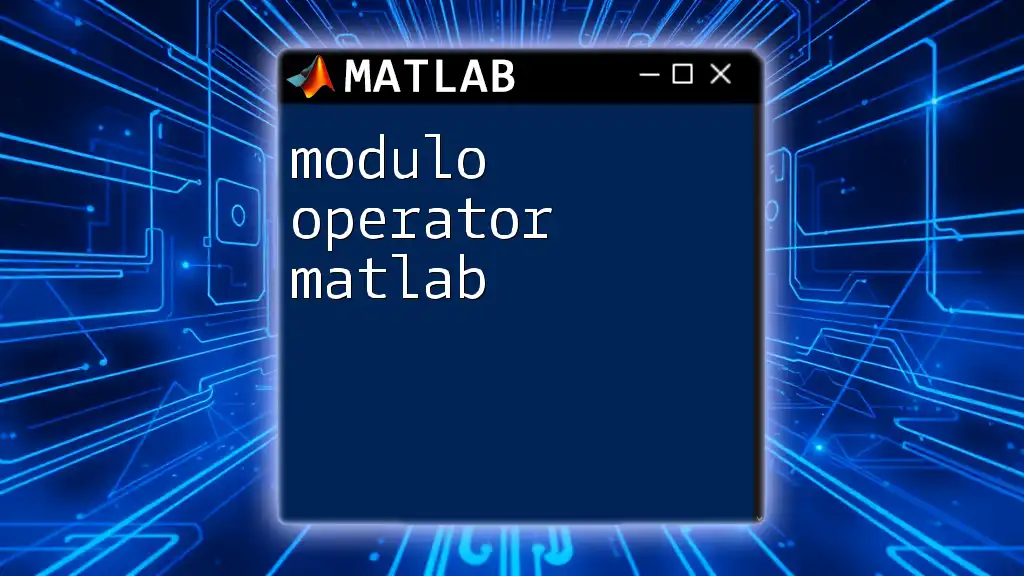
Conclusion
Moving average filters in MATLAB offer a powerful way to smooth data, reduce noise, and enhance the quality of signals, series, and images. By exploring simple, weighted, and exponential moving averages, you can develop a strong foundation for analyzing trends and optimizing data. Experiment with the examples provided and discover the potential of moving average filters in your projects.
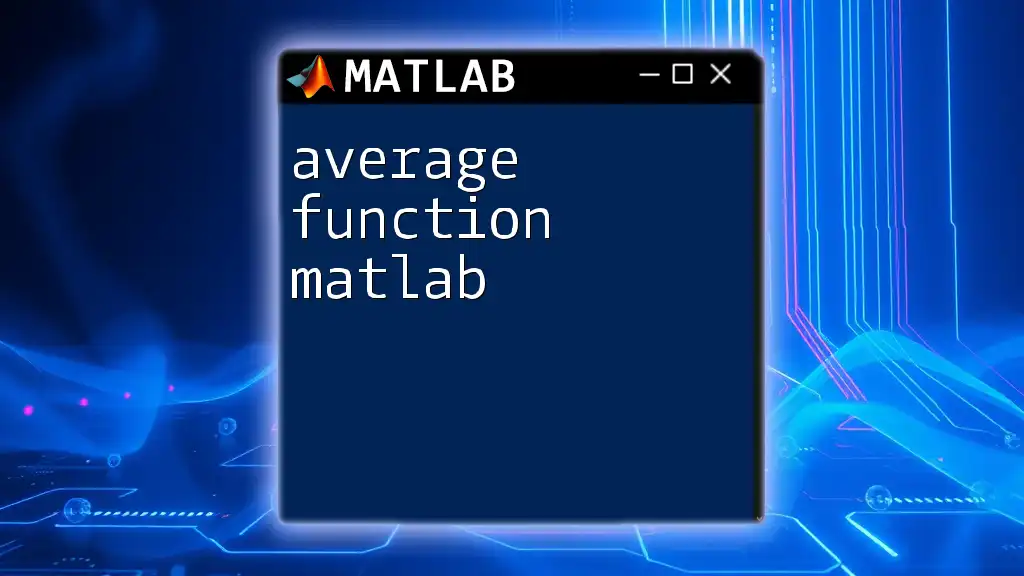
Further Reading & Resources
For a deeper understanding, consider consulting MATLAB's official documentation for `movmean`, `filter`, and `conv2`. Additionally, articles and resources on signal processing and time series analysis will further enrich your learning experience.
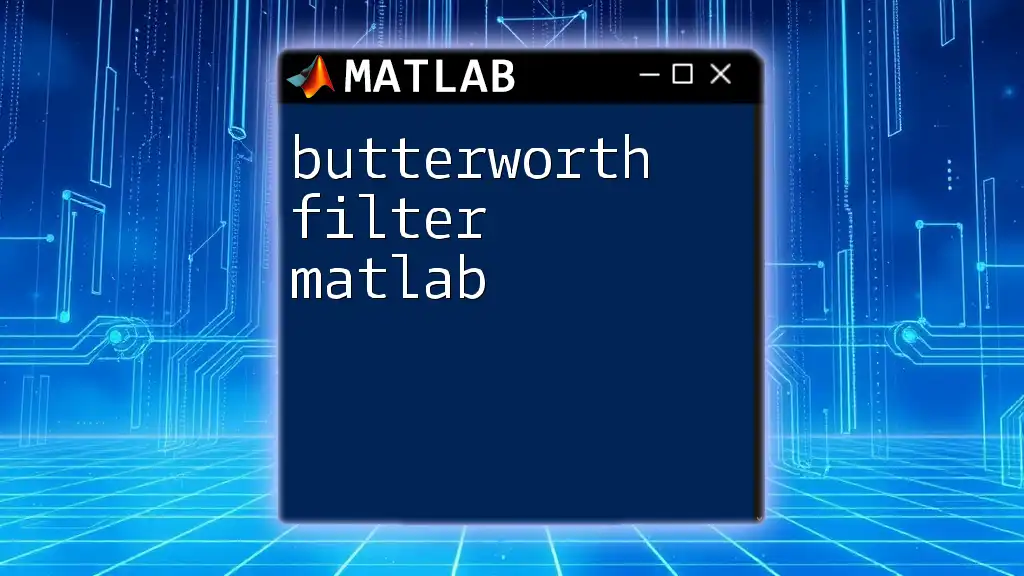
Call to Action
Stay updated with our posts to enhance your MATLAB skills! If you have any questions or need clarifications regarding moving average filters, feel free to share your thoughts in the comments section.