In MATLAB, you can compute the running average (or moving average) of a numerical array using the `movmean` function, which simplifies the process by applying a specified window size to the input data.
Here's an example code snippet:
data = [1 2 3 4 5 6 7 8 9 10]; % Sample data
windowSize = 3; % Size of the moving window
runningAverage = movmean(data, windowSize); % Calculate running average
disp(runningAverage); % Display the result
What is a Running Average?
A running average, also known as a moving average, is a statistical method used to analyze data by creating averages of different subsets of a complete dataset. This technique is particularly useful for smoothing out short-term fluctuations and highlighting longer-term trends. In MATLAB, the concept of a running average is primarily applied in data analysis and signal processing, where it helps to reduce noise and make patterns in the data clearer.
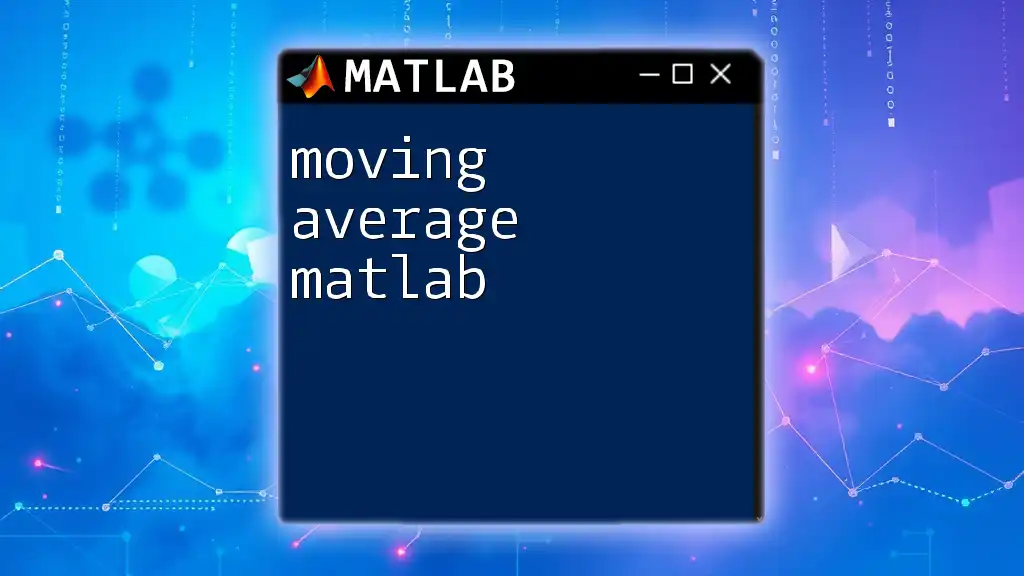
Applications of Running Averages
Running averages find their applications in various domains, including:
- Finance: Analyzing stock prices to clarify trends over time.
- Signal Processing: Smoothing signals to eliminate noise.
- Quality Control: Monitoring variations in production processes.
- Climate Data: Observing trends in temperature or precipitation over time. By applying a running average, researchers and analysts can gain insights that are often obscured by data variability.
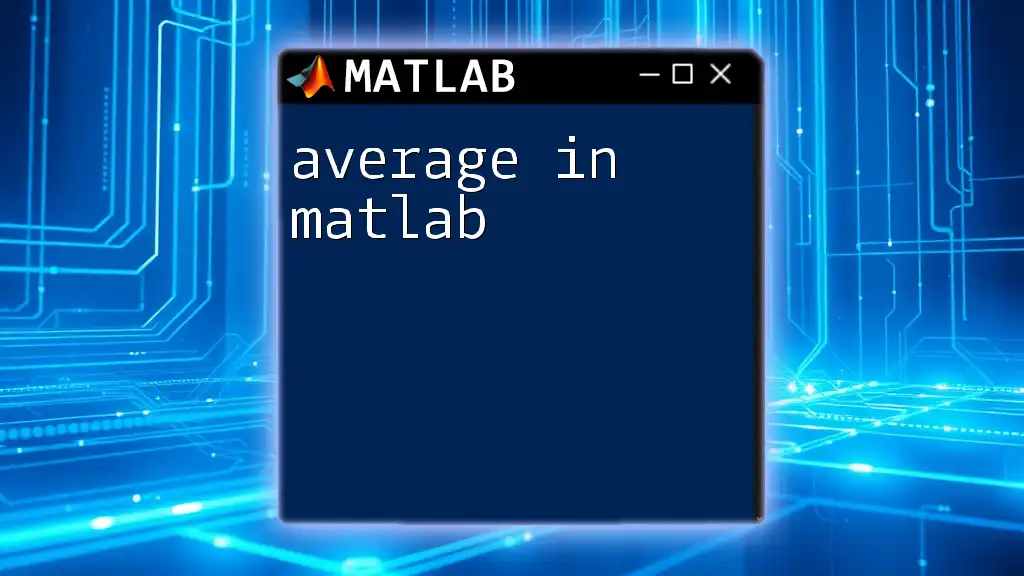
Understanding MATLAB Basics
MATLAB is a powerful environment designed for mathematical computations, making it ideal for working with statistical methods like running averages. Familiarizing yourself with key MATLAB commands is essential for implementing running averages effectively.
Key MATLAB Commands Relevant to Running Averages
Some basic commands that will be relevant when calculating a running average include:
- `mean`: Computes the average of an array.
- `filter`: Applies a digital filter to the data.
- `conv`: Performs mathematical convolution.
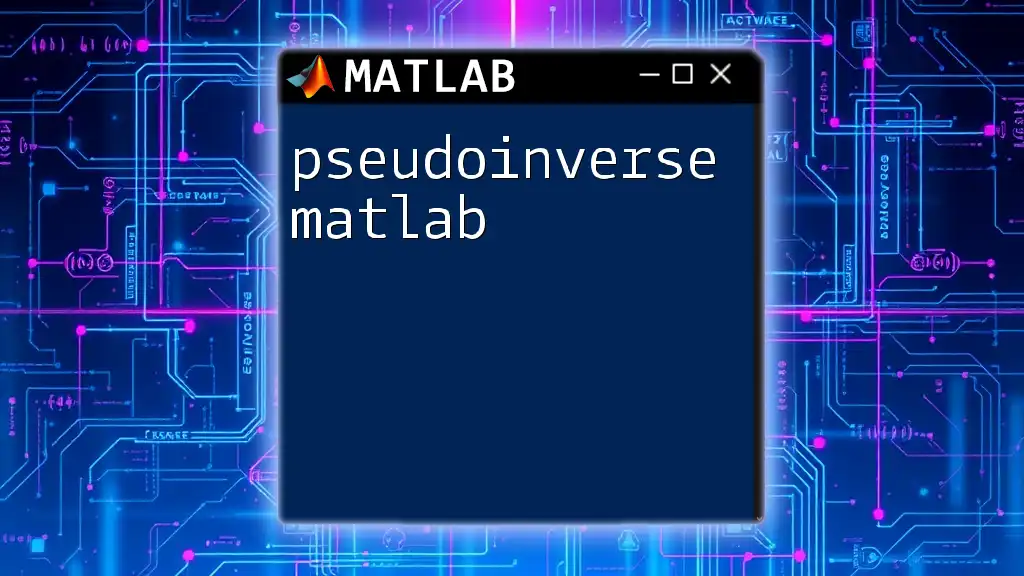
Methods to Calculate a Running Average in MATLAB
Using the `movmean` Function
Introduction to `movmean`
The `movmean` function is designed specifically for calculating the moving average of a dataset. It simplifies the process of obtaining a running average with just a few parameters.
Syntax and Parameters
The syntax for `movmean` is as follows:
Y = movmean(X, K)
Here, `X` represents the input data array, `K` is the window size (the number of elements over which the average is computed), and there are optional parameters that can specify the method of calculation (e.g., `Endpoints`).
Example Code Snippet
To calculate a simple running average using `movmean`, you can use the following example:
data = [1, 2, 3, 4, 5, 6];
running_avg = movmean(data, 3); % Running average with a window of 3
disp(running_avg);
This will yield the running average of the input data using the specified window size.
Visualization of Results
Visualizing the results can help compare the original data with its running average. Typically, you would use MATLAB’s plotting capabilities to illustrate how the running average smooths out the original data.
Using the `filter` Function
Introduction to Filtering
The `filter` function provides a powerful way to apply linear filters to your data, making it suitable for computing running averages.
Syntax and Parameters
The standard syntax for the `filter` function is:
Y = filter(B, A, X)
In the context of a running average, the coefficients `B` and `A` are defined as follows:
- `B` is set to the averaging kernel (e.g., `(1/N)`), where `N` is the window size.
- `A` is typically set to 1 for a simple average calculation.
Example Code Snippet
Here’s how to compute a running average using the `filter` function:
data = [1, 2, 3, 4, 5, 6];
N = 3; % Window size
b = (1/N) * ones(1, N); % Coefficient for averaging
a = 1; % No delay
running_avg = filter(b, a, data);
disp(running_avg);
This code will compute the running average by applying the defined filter to the given data.
Practical Considerations
When using the `filter` function, it’s essential to be aware of potential boundary effects. The output at the beginning of the dataset may not accurately reflect the running average. Various techniques can help mitigate this issue, such as padding the data or using a different edge-handling strategy.
Using Convolution for Running Averages
Introduction to Convolution
Convolution is a mathematical operation that can be used to implement a running average via linear combinations of successive elements.
Syntax and Implementation
In MATLAB, the `conv` function is utilized for convolution:
Y = conv(X, K)
In this context, the kernel `K` is an averaging vector defined as:
K = (1/N) * ones(1, N)
where `N` represents the desired window size for the average.
Example Code Snippet
Here’s an example of using convolution to calculate a running average:
data = [1, 2, 3, 4, 5, 6];
N = 3;
kernel = (1/N) * ones(1, N); % Define the averaging kernel
running_avg = conv(data, kernel, 'same'); % 'same' retains the original size
disp(running_avg);
This approach averages elements based on their neighborhood values.
Visualizing the Convolution Result
Using visualization tools in MATLAB, you can compare the convolved data with the original dataset to see how effective the convolution method is in producing a running average.
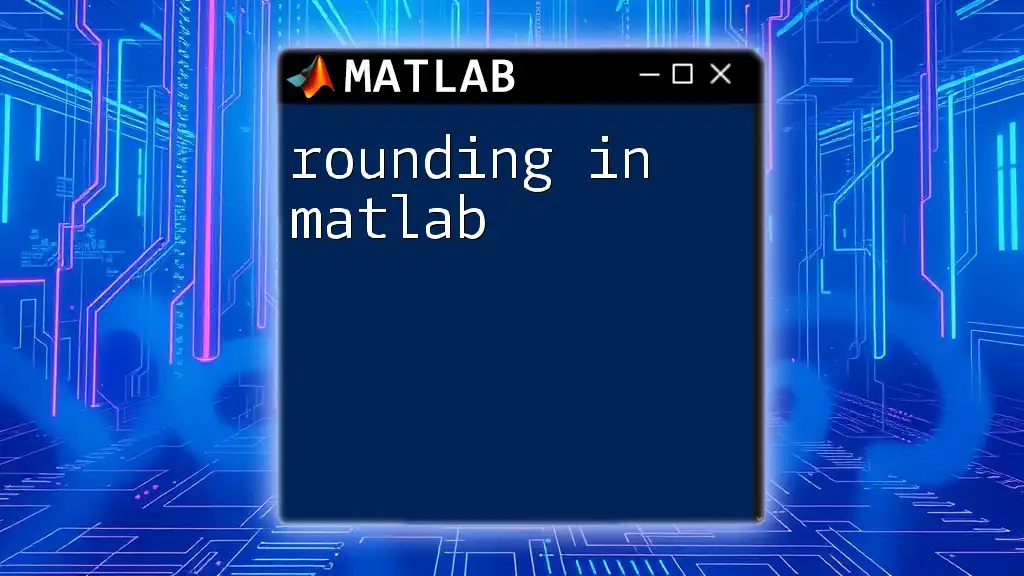
Comparing Different Methods
When implementing a running average in MATLAB, it's important to consider the pros and cons of each method:
- `movmean`: Easy to use and efficient for large datasets, but may not be as flexible as filtering methods.
- `filter`: Offers more control over custom filters but requires a greater understanding of filtering concepts.
- `conv`: Provides a straightforward mathematical approach but can have edge effects that require careful handling.
Choosing the Right Method for Your Data
Selecting the appropriate method depends on factors such as the size of the dataset, the desired smoothness, and the specific requirements of the analysis. For instance, if you need a simple average with minimal setup, `movmean` is likely your best bet. If you need custom filtering capabilities, then `filter` may be the way to go.
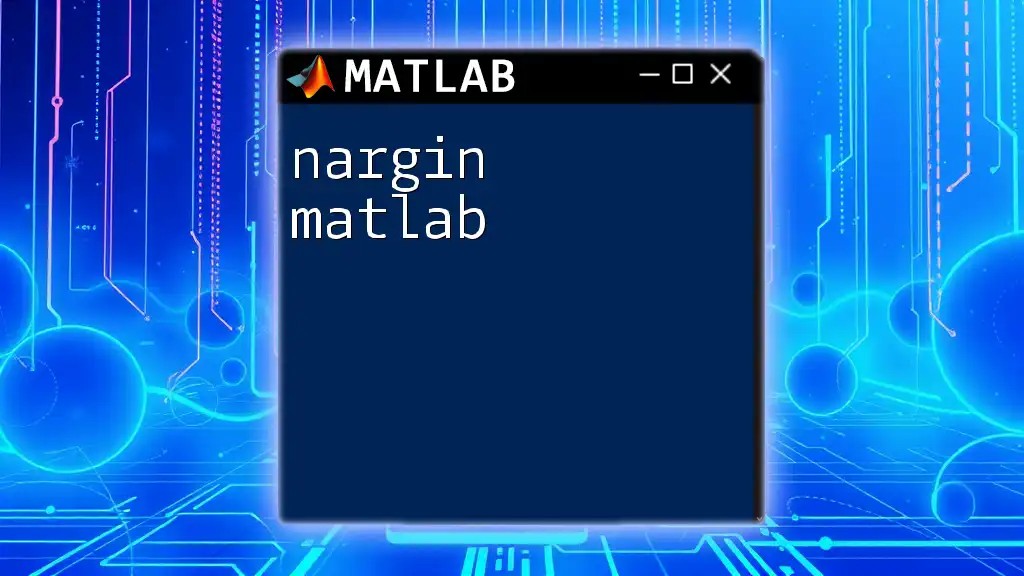
Conclusion
In this guide, we covered the essentials of calculating a running average in MATLAB using different methods: `movmean`, `filter`, and convolution. Understanding these techniques not only helps in smoothing out your data but also enhances your ability to extract meaningful insights from datasets.
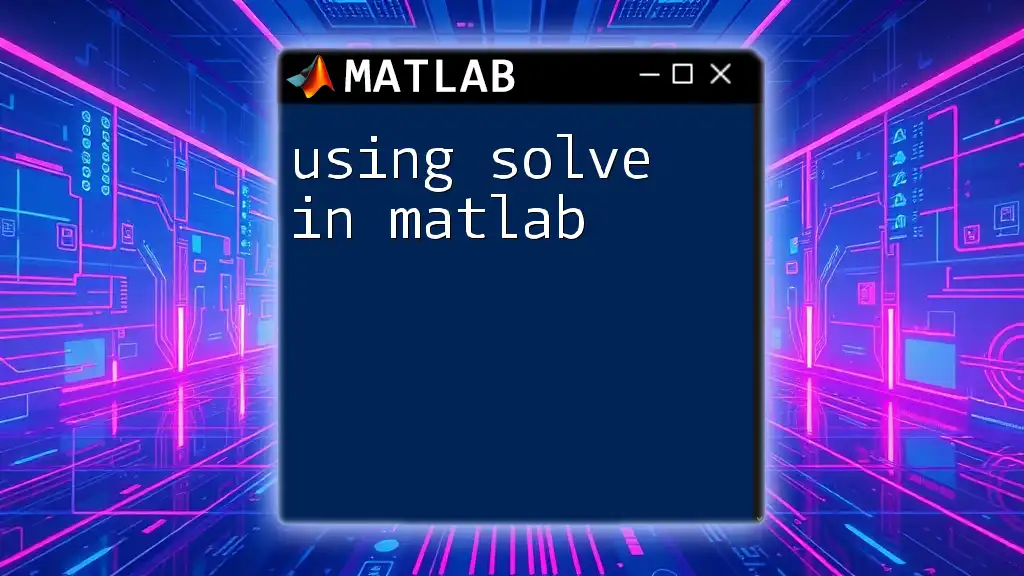
Additional Resources
To further enrich your learning experience, explore the official MATLAB documentation for the functions discussed, as well as books and online courses that can enhance your understanding of MATLAB and data analysis techniques.
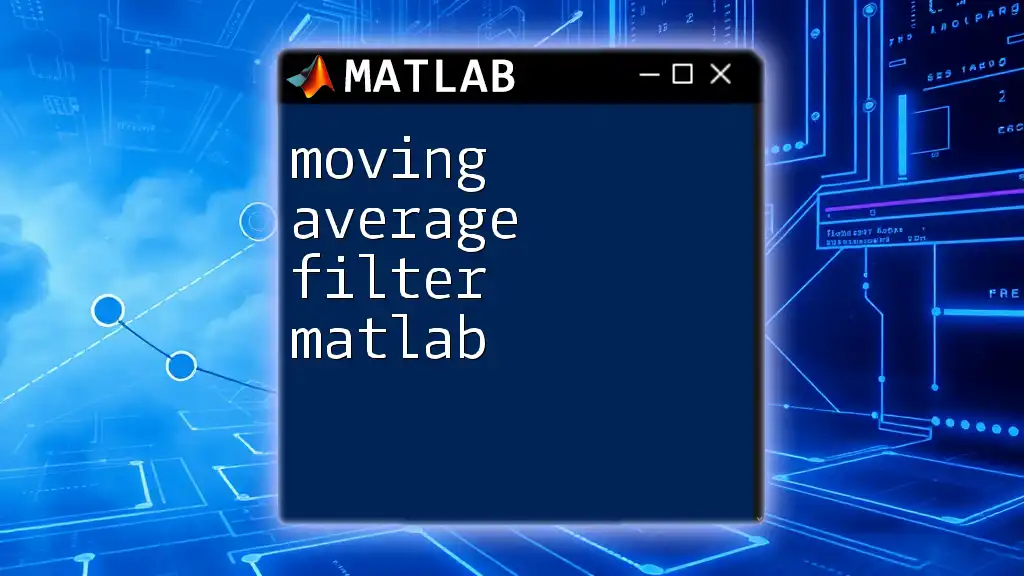
FAQs
Addressing common questions surrounding running averages in MATLAB can help clarify misunderstandings and ease the learning curve for newcomers. Providing thoughtful answers will serve as a valuable resource for those engaging with statistical analysis in MATLAB.