The vector product (also known as the cross product) in MATLAB can be calculated using the `cross` function, which returns a vector that is perpendicular to the plane formed by the two input vectors.
A = [1, 2, 3]; % First vector
B = [4, 5, 6]; % Second vector
C = cross(A, B); % Vector product of A and B
Understanding Vectors in MATLAB
Definition of Vectors
A vector is a mathematical object that has both a magnitude and a direction. In MATLAB, vectors can be represented as arrays, allowing for various mathematical operations to be performed efficiently. In programming, understanding how to manipulate vectors is crucial for tasks ranging from data analysis to solving complex mathematical problems.
Types of Vectors
Vectors in MATLAB can be categorized as row vectors and column vectors.
-
Row Vectors: These are one-dimensional arrays with elements arranged in a single row. For example,
A = [1, 2, 3]; % This is a row vector
-
Column Vectors: These are also one-dimensional but have elements arranged vertically in a single column. For example,
B = [1; 2; 3]; % This is a column vector
You can create vectors in MATLAB using several methods, such as the concatenation operator `[]` or the `linspace` function.
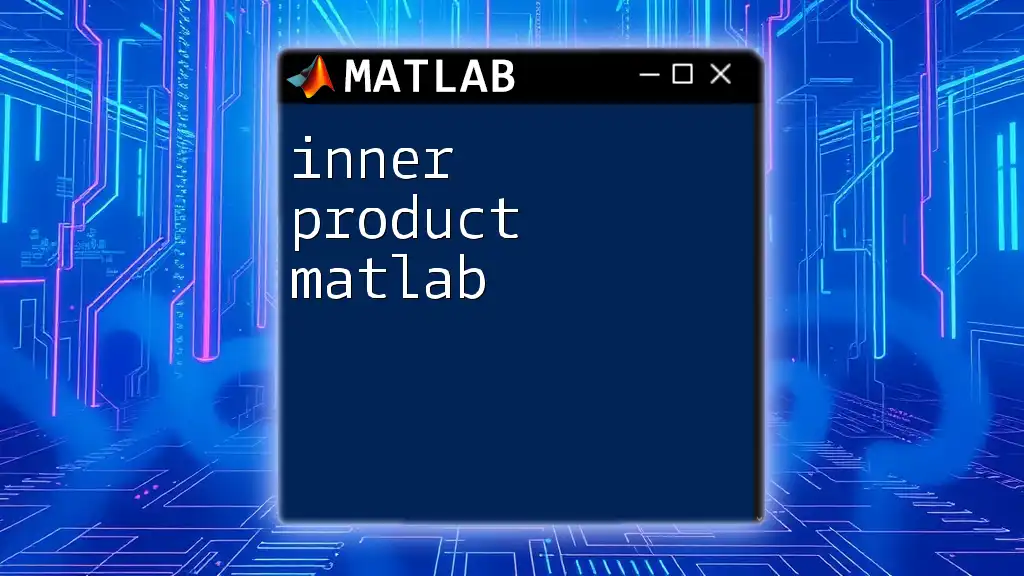
What is a Vector Product?
Definition of Vector Product
The vector product refers to the mathematical operations that involve vectors, resulting in another vector or a scalar depending on the type of operation. The two primary types of vector products are the dot product and the cross product.
Types of Vector Products
Dot Product
The dot product (also known as the scalar product) of two vectors results in a scalar value. It mathematically represents the cosine of the angle between the two vectors multiplied by their magnitudes. This operation is widely used in physics and engineering, for example, in calculating work done when a force is applied in the direction of displacement.
Cross Product
The cross product of two vectors results in another vector. This new vector is orthogonal to the plane created by the two vectors and its magnitude is proportional to the area of the parallelogram formed by them. This operation is particularly useful in fields such as physics and computer graphics, where it can describe rotational movements or normals to surfaces.
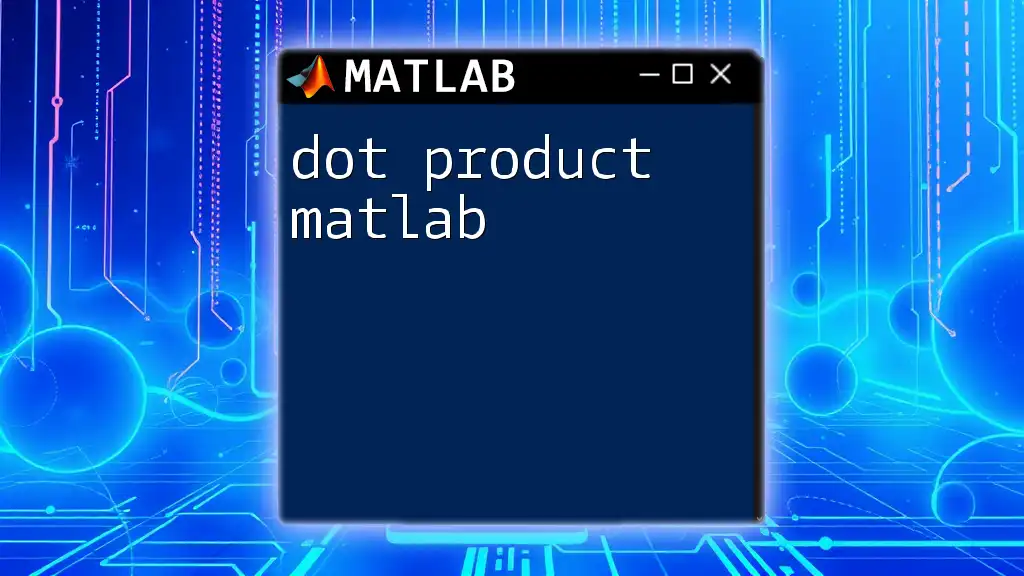
Performing Vector Products in MATLAB
Dot Product in MATLAB
Using the `dot` Function
In MATLAB, the dot product can be calculated easily using the `dot` function. The syntax is as follows:
result = dot(A, B)
For example, consider two vectors:
A = [1, 2, 3];
B = [4, 5, 6];
result = dot(A, B); % Output: 32
Here, the dot product of vectors A and B results in the scalar `32`, which indicates both the magnitude and direction of the interaction between the two vectors.
Manual Calculation of Dot Product
Alternatively, you can compute the dot product manually by using basic array operations. The formula involves multiplying corresponding elements of the vectors and then summing those products:
A = [1, 2, 3];
B = [4, 5, 6];
result = sum(A .* B); % Output: 32
This approach provides a deeper understanding of the underlying calculation.
Cross Product in MATLAB
Using the `cross` Function
The cross product can be computed using the `cross` function in MATLAB. The syntax is as follows:
result = cross(A, B)
For instance:
A = [1, 2, 3];
B = [4, 5, 6];
result = cross(A, B); % Output: [-3, 6, -3]
This result indicates that the cross product of vectors A and B is `[-3, 6, -3]`, which is orthogonal to both input vectors.
Manual Calculation of Cross Product
You can also compute the cross product manually by using the formula involving the determinant of a matrix. The steps are as follows:
A = [1; 2; 3];
B = [4; 5; 6];
result = [A(2)*B(3) - A(3)*B(2), A(3)*B(1) - A(1)*B(3), A(1)*B(2) - A(2)*B(1)]; % Output: [-3, 6, -3]
This calculation reinforces the geometric interpretation of the cross product.
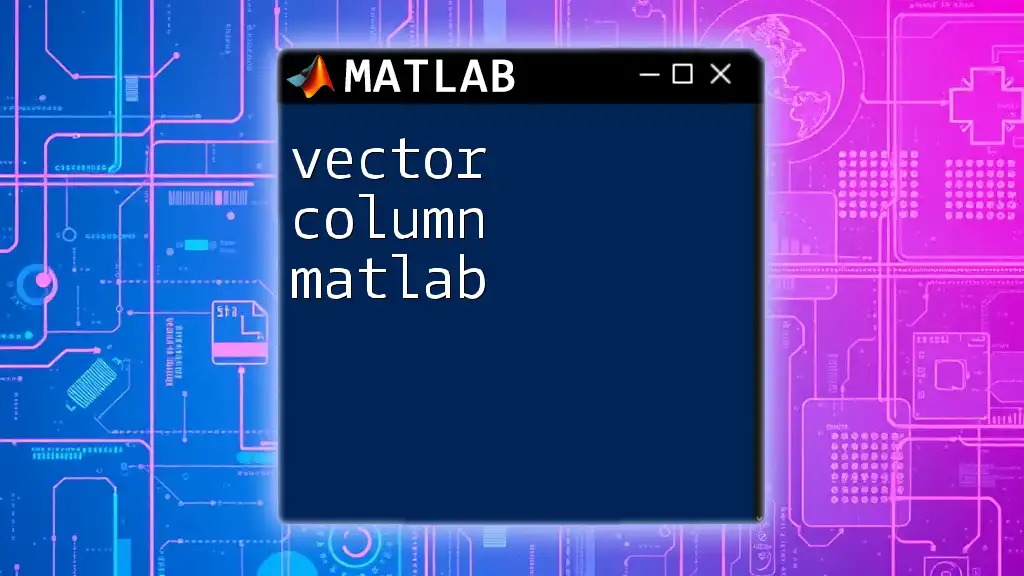
Common Applications of Vector Products
Physics Applications
In physics, vector products play a crucial role in understanding concepts such as torque and angular momentum. The cross product helps calculate torque, which is the rotational effect produced by a force applied at some distance from a pivot point. This allows physicists to analyze rotational motion effectively.
Computer Graphics Applications
In the realm of computer graphics, vector products are extensively used for creating 3D models. The cross product is often employed to calculate normal vectors, which are vital for lighting calculations and shading techniques. It helps algorithms determine how surfaces interact with light, producing realistic visual effects.
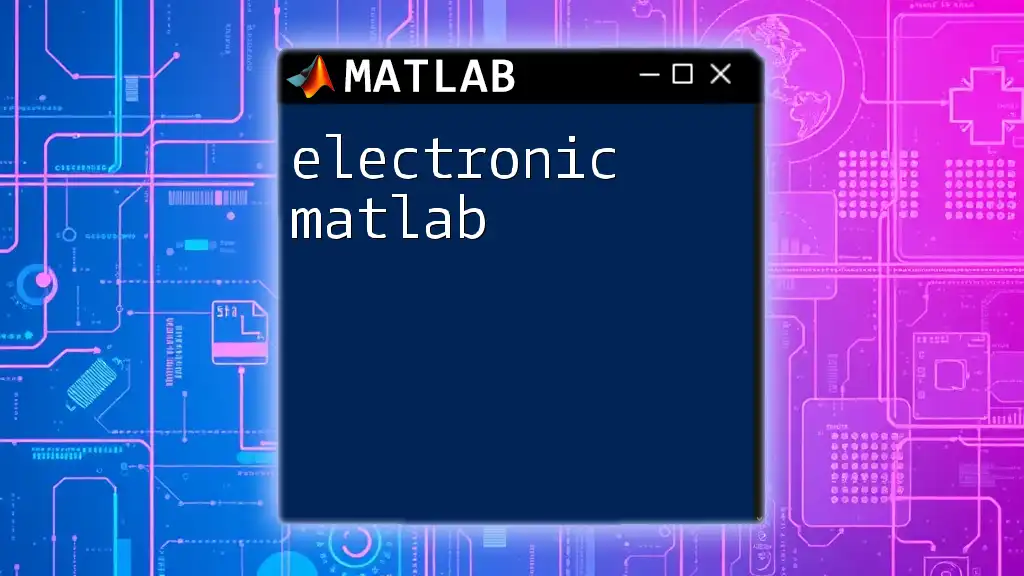
Best Practices and Tips for Working with Vector Products in MATLAB
Code Optimization
One of the best practices for working with vectors in MATLAB is to focus on vectorization. Vectorized operations are generally much faster than using loops, as MATLAB is optimized for matrix and vector operations. Always aim to minimize loops for better performance.
Debugging Common Errors
When working with vector products, a common error arises from dimension mismatch. Ensure that the vectors involved in dot or cross product operations adhere to the correct dimensions. For example, two vectors must have the same number of elements for the dot product, while for the cross product, they should each be 3-dimensional.
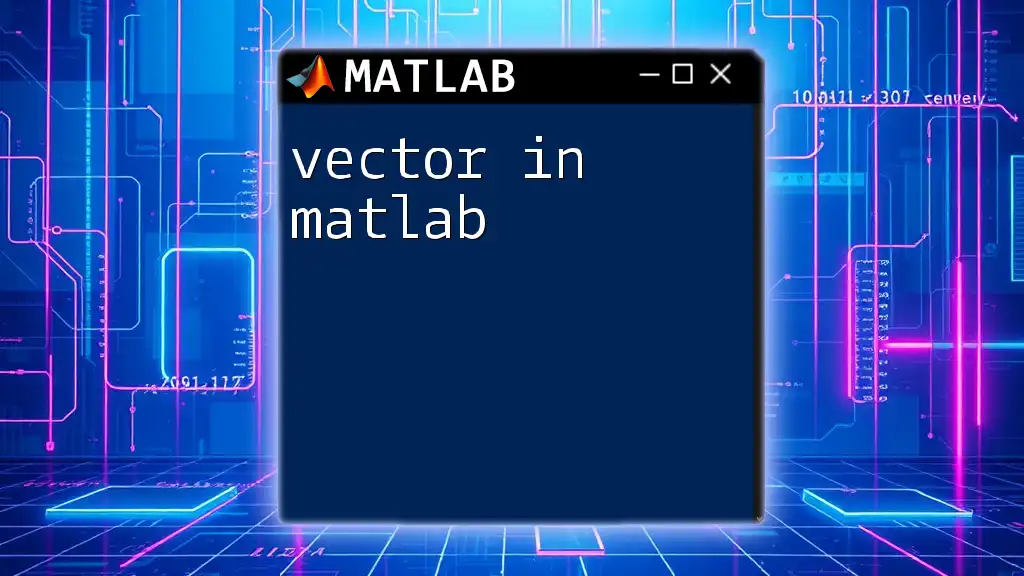
Conclusion
Recap of Vector Products
In summary, the discussion around vector product in MATLAB highlights the importance of understanding both the dot and cross products. Mastering these operations enables effective handling of vectors in various fields.
Encouragement to Practice
For those looking to deepen their understanding, practicing vector operations in MATLAB will solidify your skills and preparation for tackling more advanced topics. Engaging with the material will promote confidence and proficiency.
Additional Resources
To further your learning, consider exploring MATLAB's documentation, online tutorials, and recommended textbooks. These resources will help you advance your skills and apply vector product operations effectively in your projects.