The Newton-Raphson method in MATLAB is an iterative numerical technique used to find the roots of real-valued functions, and can be implemented succinctly as follows:
function root = newtonRaphson(func, dFunc, x0, tol, maxIter)
for i = 1:maxIter
x1 = x0 - func(x0) / dFunc(x0);
if abs(x1 - x0) < tol
root = x1;
return;
end
x0 = x1;
end
error('Maximum iterations reached without convergence');
end
Understanding the Newton-Raphson Method
What is the Newton-Raphson Method?
The Newton-Raphson method is a widely used algorithm for estimating the roots of a real-valued function. It is particularly powerful due to its rapid convergence properties, especially when the initial guess is close to the true root. Historically, the method has been attributed to Sir Isaac Newton and later refined by Joseph Raphson, hence the name.
Key Concepts
To fully grasp the Newton-Raphson method, it is crucial to understand a few fundamental concepts:
-
Roots: A root of a function \( f(x) \) is a value \( x = r \) such that \( f(r) = 0 \). Finding these roots is essential for many mathematical and engineering applications.
-
Iterative Formula: The essence of the Newton-Raphson method lies in its iterative formula, defined as:
\[ x_{n+1} = x_n - \frac{f(x_n)}{f'(x_n)} \]
This formula iteratively updates the current approximation \( x_n \) based on the function evaluation and its derivative.
-
Convergence: The speed at which the method converges to a root is remarkable, particularly if the initial guess is sufficiently close to the actual root. However, if the initial guess is too far, or if the function behaves poorly (e.g., horizontal tangents), convergence can be slow or may even diverge.
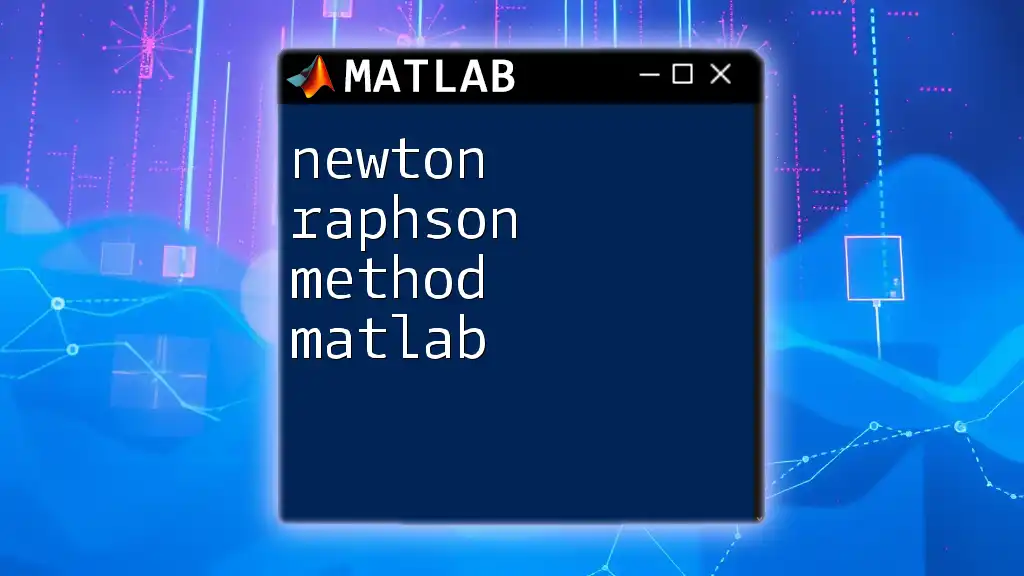
Setting Up MATLAB for Newton-Raphson
Installing and Preparing MATLAB
Before diving into the implementation, ensure that you have MATLAB installed on your computer. If you haven't already done so, you can find the installation guide on the official MATLAB website. Once installed, make sure your environment is ready for coding.
Opening MATLAB Environment
Upon launching MATLAB, you will be greeted with the interface containing several panels. The Command Window allows you to execute commands directly, while the Script Editor provides a space for writing and saving longer scripts. Familiarizing yourself with these tools is essential for efficient coding.
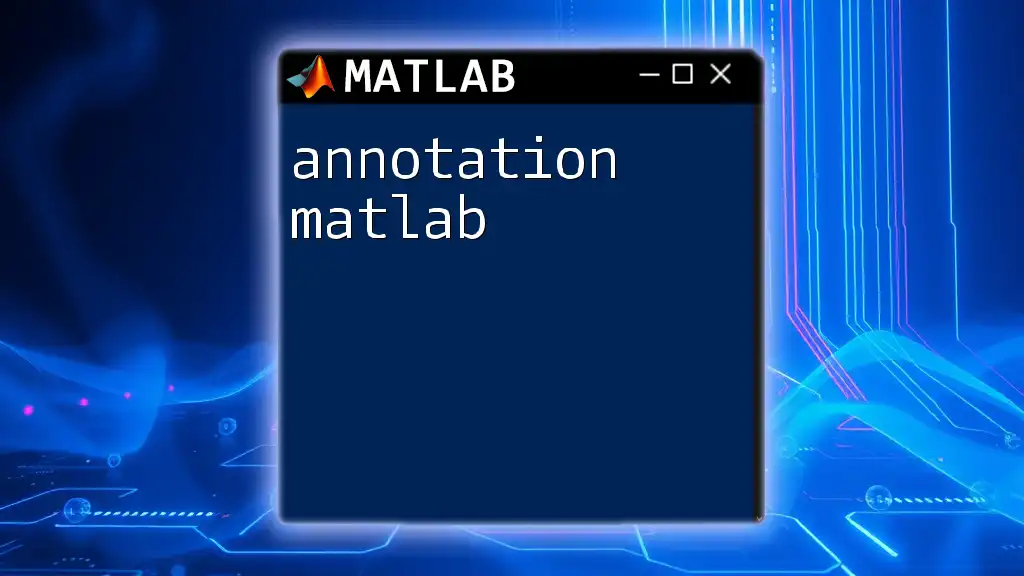
Implementing the Newton-Raphson Method in MATLAB
Step-by-Step Implementation
The implementation of the Newton-Raphson method in MATLAB requires defining both the function for which you want to find the root and its derivative.
- Defining a Function: First, let’s define a simple function \( f(x) = x^2 - 4 \). This function has roots at \( x = -2 \) and \( x = 2 \). The MATLAB code for this function is as follows:
function y = f(x)
y = x^2 - 4;
end
- Calculating the Derivative: The next step involves defining the derivative \( f'(x) \). For our function, the derivative is \( f'(x) = 2x \):
function dy = df(x)
dy = 2 * x;
end
Writing the Newton-Raphson Function
Now that we have both the function and its derivative defined, we can write the main function to execute the Newton-Raphson algorithm. The function will take an initial guess, a tolerance level for convergence, and a maximum number of iterations as input parameters.
Here is the code for the Newton-Raphson function:
function root = newtonRaphson(x0, tol, maxIter)
for iter = 1:maxIter
f_val = f(x0);
df_val = df(x0);
if df_val == 0
error('Zero derivative. No solution found.');
end
x1 = x0 - f_val / df_val; % Update root
if abs(x1 - x0) < tol
root = x1;
return;
end
x0 = x1; % Update for the next iteration
end
error('Maximum iterations reached without convergence.');
end
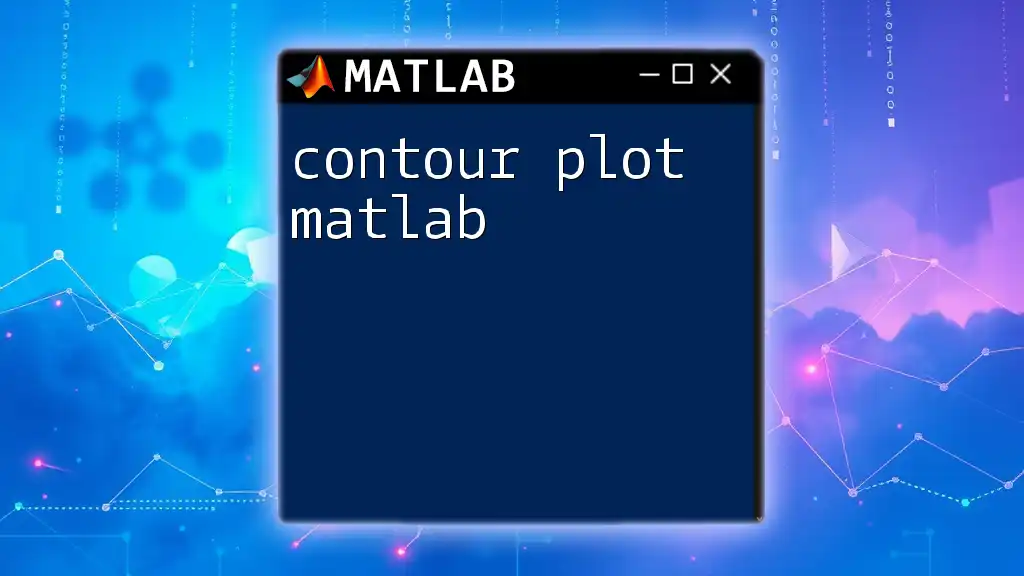
Example of Finding a Root
Defining the Problem
Let's use our defined functions to find the root of \( f(x) = x^2 - 4 \). The goal is to start the iteration with an initial guess, say \( x_0 = 3 \).
Running the Code in MATLAB
To run the Newton-Raphson method, we can execute the following code snippet in MATLAB:
x0 = 3; % Initial guess
tol = 1e-6; % Tolerance
maxIter = 100; % Maximum iterations
root = newtonRaphson(x0, tol, maxIter);
fprintf('The root found is: %.6f\n', root);
By executing this code, MATLAB will iterate to find the root of the function, and you should see output indicating the root found.
Analyzing the Output
The expected output will be:
The root found is: 2.000000
This confirms that the method successfully identified the root \( x = 2 \). If the implementation does not converge, it may be necessary to adjust the initial guess or check the function's behavior and derivatives at that point.
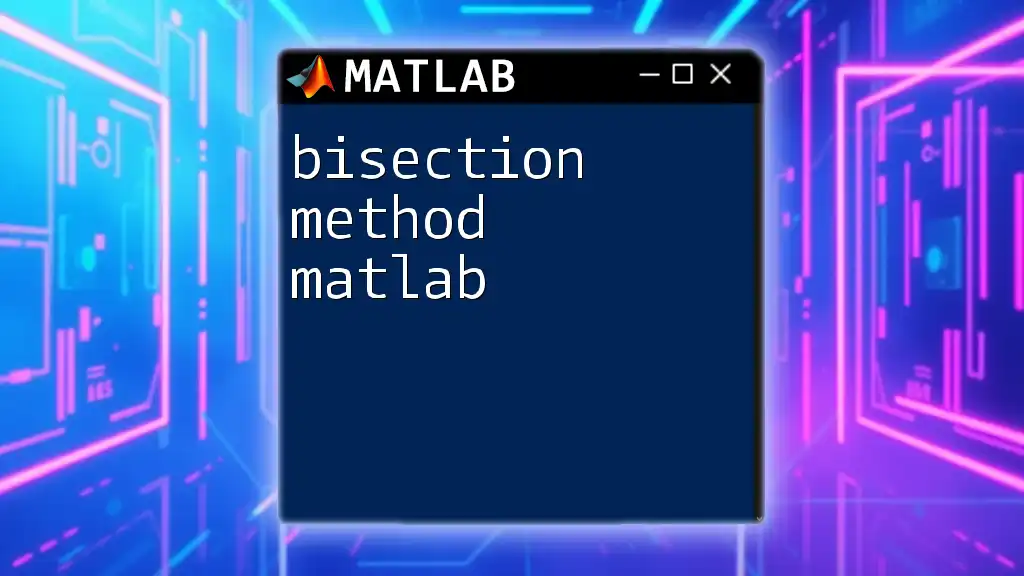
Integrating the Newton-Raphson Method with Visualization
Plotting the Function
Visual representation of the function and its roots can greatly aid understanding. You can visualize the function along with the identified root using the following MATLAB code:
x = linspace(-5, 5, 100);
plot(x, f(x));
hold on;
plot(root, f(root), 'ro'); % Root indicated in red
title('Function Plot with Root Indicated');
xlabel('x');
ylabel('f(x)');
grid on;
hold off;
This plot illustrates the function \( f(x) \) graphically, showcasing where it crosses the x-axis, which correlates with the root.
Modifying the Code for Multiple Roots
For functions that may have multiple roots, nuanced changes in the approach are necessary. For example, consider the cubic function \( f(x) = x^3 - 6x^2 + 11x - 6 \). You can use the same methodology while changing the functions appropriately. Care must be taken with initial guesses to ensure the specific root of interest is approached.
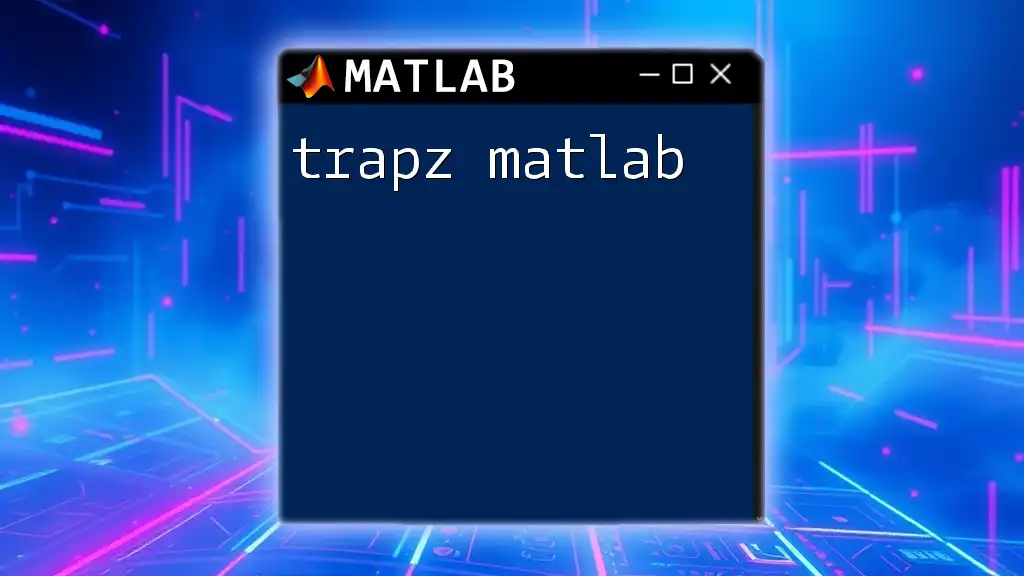
Tips for Efficient Use of Newton-Raphson in MATLAB
Choosing the Initial Guess
The choice of the initial guess is critical to the success of the Newton-Raphson method. A guess close to the actual root will significantly enhance the algorithm's speed and efficiency.
Maximizing Convergence Speed
To maximize the convergence speed, it is beneficial to understand the behavior of the function around the suspected root. Functions that are smooth and increase (or decrease) steadily near their roots tend to yield quicker convergence.
Common Pitfalls
There are some common pitfalls to watch out for when implementing the Newton-Raphson method:
-
Zero Derivative: Ensure that the derivative is not zero at your guess. A zero derivative can lead to division by zero errors, which will stymie the entire computation.
-
Local Minima/Maxima: Be cautious if your guess lies close to local minima or maxima, as the method may fail to converge in such cases.
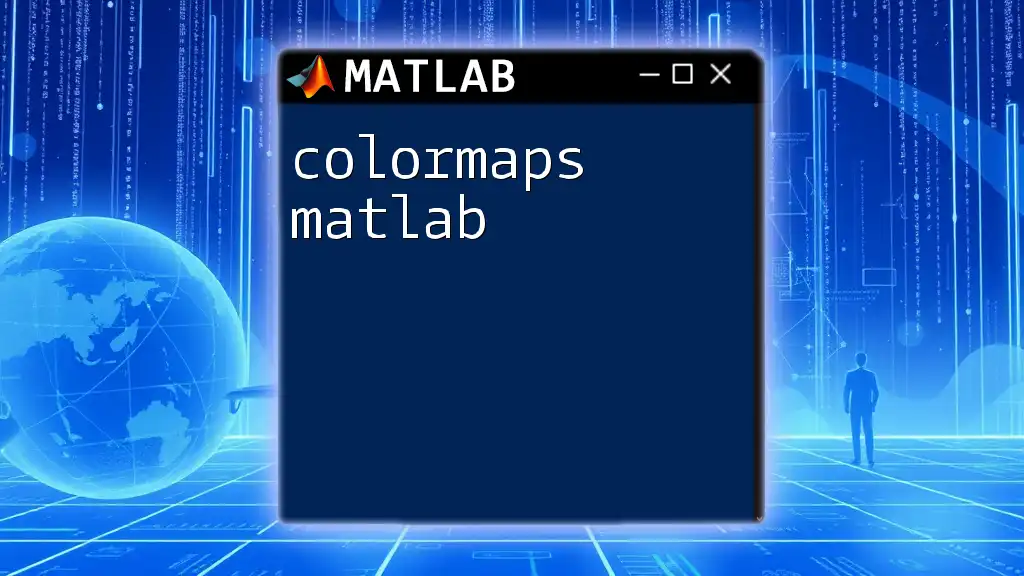
Conclusion
The Newton-Raphson method is a powerful numerical method for finding roots of functions, and its implementation in MATLAB is straightforward and efficient. By following the outlined steps for defining functions, writing the iterative algorithm, and visualizing results, you can adeptly apply this algorithm to various mathematical problems. Practice and experiment with different functions to deepen your understanding and expand your numerical analysis toolkit.
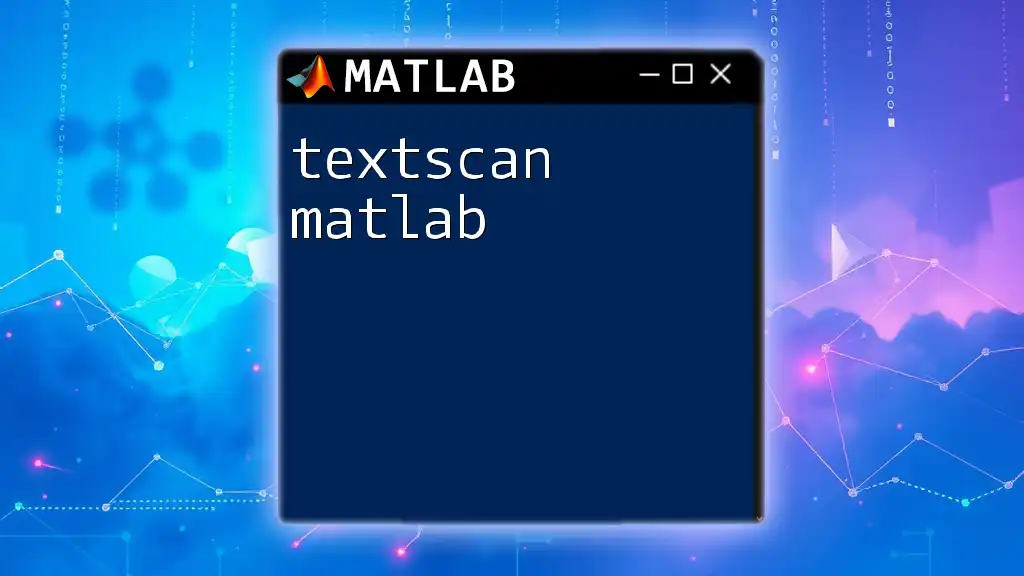
Additional Resources
For further learning, consider exploring the official MATLAB documentation and consulting numerical analysis textbooks. Online forums and communities can provide additional help and insights.
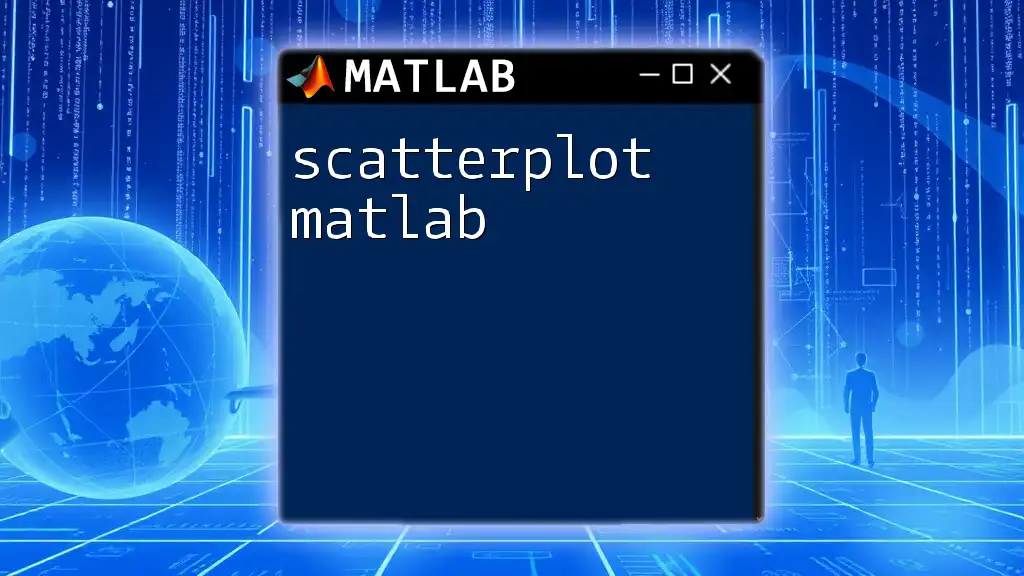
FAQs
What if my function has multiple roots?
The Newton-Raphson method can still find multiple roots, but you may need to adjust your initial guess for each root if they are close together.
Why does my implementation converge slowly?
Slow convergence can occur due to a poor initial guess or function characteristics that hinder the method's efficiency. Consider closely analyzing the function's behavior.
Can I use the method for complex functions?
The Newton-Raphson method can be modified for complex functions, but additional considerations and modifications may be required to handle complex numbers effectively.