The `normpdf` function in MATLAB computes the probability density function of the normal distribution for given values, allowing users to evaluate how likely a given value is under a specified mean and standard deviation.
Here's a code snippet illustrating its use:
x = -5:0.1:5; % Range of x values
mu = 0; % Mean
sigma = 1; % Standard deviation
y = normpdf(x, mu, sigma); % Compute the normal probability density
plot(x, y); % Plot the result
title('Normal Distribution');
xlabel('X values');
ylabel('Probability Density');
What is `normpdf`?
The `normpdf` function in MATLAB is a critical tool in statistics and data analysis. It is designed to compute the normal probability density function (PDF) for a given set of data points. Understanding `normpdf` is crucial for anyone working with data that assumes a normal distribution, which is common in many fields, including finance, engineering, and social sciences.
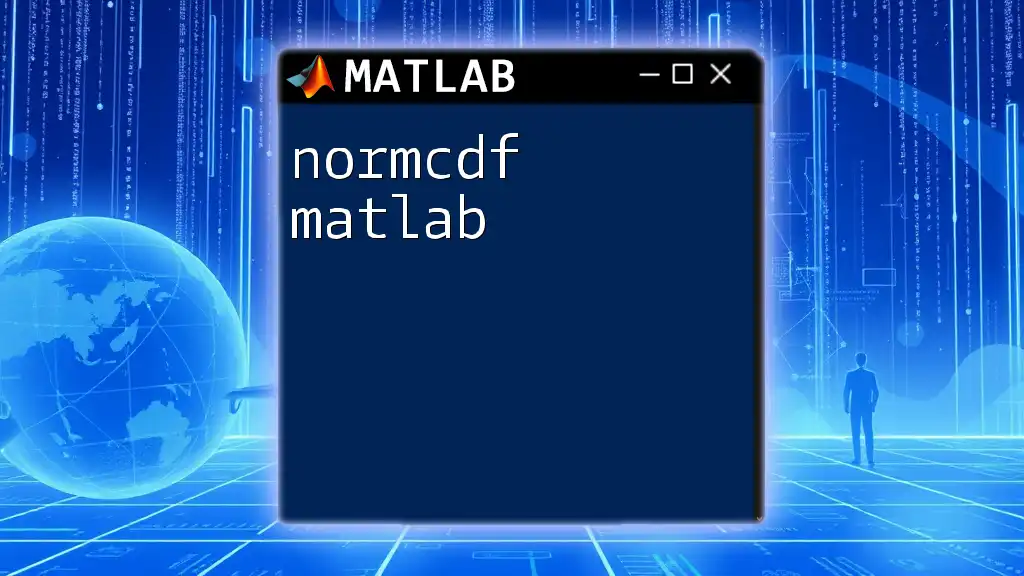
Syntax of `normpdf`
The basic syntax of the `normpdf` function is as follows:
y = normpdf(x, mu, sigma)
Parameters:
-
`x`: This represents the input values—these can be scalars, vectors, or matrices. It’s important to remember that `normpdf` evaluates the PDF at each value of `x`.
-
`mu`: This parameter specifies the mean of the normal distribution. The shape of the distribution is significantly influenced by this value, determining where the peak of the graph will lie.
-
`sigma`: This represents the standard deviation of the distribution. It controls the dispersion or spread of the distribution; a larger value for `sigma` means a wider spread.
Return Value
The function returns `y`, which contains the computed probability densities corresponding to each value in `x`. The values of `y` can be interpreted as the height of the normal distribution curve at the specified points in `x`.
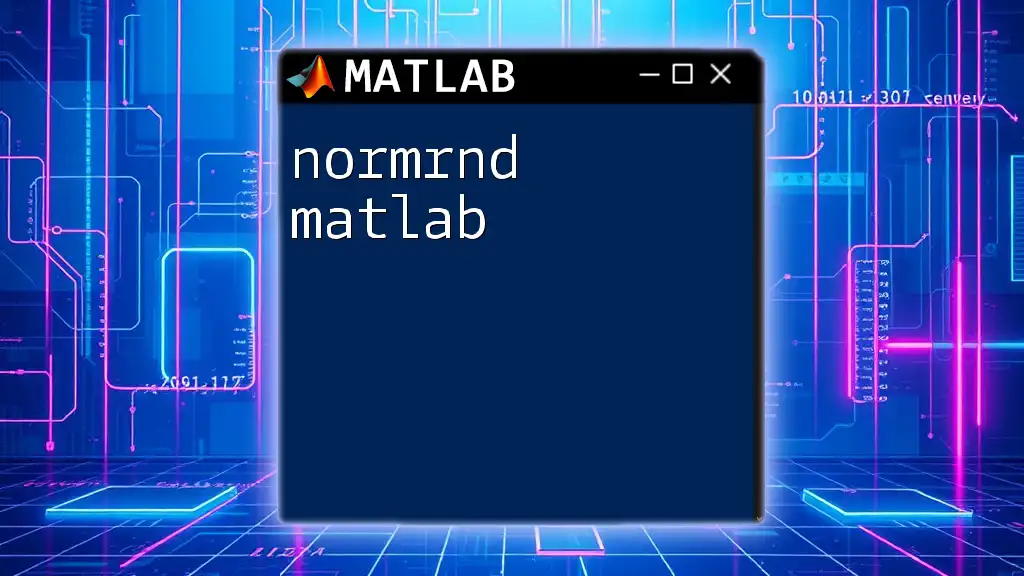
Examples of `normpdf`
Simple Example
To understand how `normpdf` works, consider a simple example where we create a standard normal distribution, which has a mean of 0 and a standard deviation of 1. Below is the MATLAB code snippet that illustrates this:
x = -3:0.1:3; % Range of x values
mu = 0; % Mean
sigma = 1; % Standard deviation
y = normpdf(x, mu, sigma);
plot(x, y);
title('Normal Distribution (mu = 0, sigma = 1)');
xlabel('x');
ylabel('Probability Density');
In this example, we defined a range of `x` values from -3 to 3. Using `normpdf`, we computed the probability density for these values with the specified mean and standard deviation. The resulting plot visually represents the normal distribution, highlighting how the PDF forms a bell-shaped curve, peaking at the mean value.
Multiple Points in a Variable
The versatility of `normpdf` also allows us to calculate PDFs for different means and standard deviations in one go. Here’s how to visualize multiple distributions:
mu_values = [-1, 0, 1]; % Different means
sigma_values = [0.5, 1, 1.5]; % Different standard deviations
x = -3:0.1:3;
hold on;
for i = 1:length(mu_values)
y = normpdf(x, mu_values(i), sigma_values(i));
plot(x, y, 'DisplayName', ['mu = ' num2str(mu_values(i)) ', sigma = ' num2str(sigma_values(i))]);
end
hold off;
legend show;
title('Normal Distributions with Different Parameters');
xlabel('x');
ylabel('Probability Density');
In this code, we utilized a loop to iterate through various means and standard deviations, plotting each normal distribution. By observing the different curves generated, you can see how altering `mu` and `sigma` affects the overall shape of the PDF. Distributions with higher means shift to the right, while varying standard deviations affect the width of the bell-shaped curve.
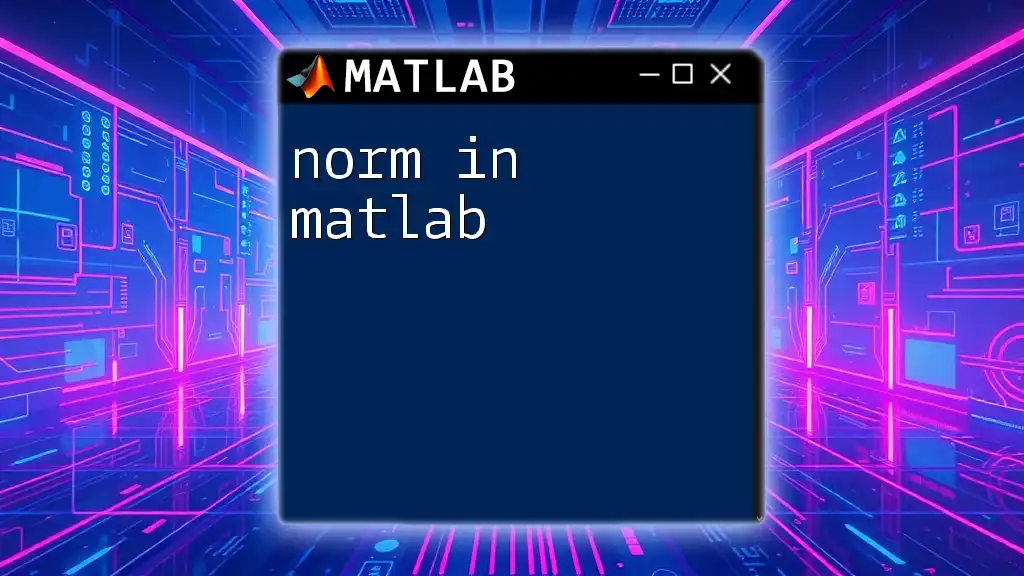
Advanced Usage of `normpdf`
Input as Vectors and Matrices
One of the powerful features of `normpdf` is its ability to accept vector and matrix inputs for `x`. When supplied with matrices, MATLAB applies the operation element-wise, returning an array of the same size with evaluated densities.
Combining with Other MATLAB Functions
Integrating `normpdf` with other MATLAB functions can yield invaluable insights. For instance, you can use it alongside the `histogram` function to visualize how a dataset fits a normal distribution. Here’s an example code snippet:
data = randn(1000,1); % Generating random data
histfit(data); % Histogram with fitted normal distribution
In this case, `histfit` will plot a histogram of the randomly generated data and overlay a normal distribution determined using `normpdf`. This visual representation allows you to quickly assess how well your data aligns with the theoretical normal distribution.
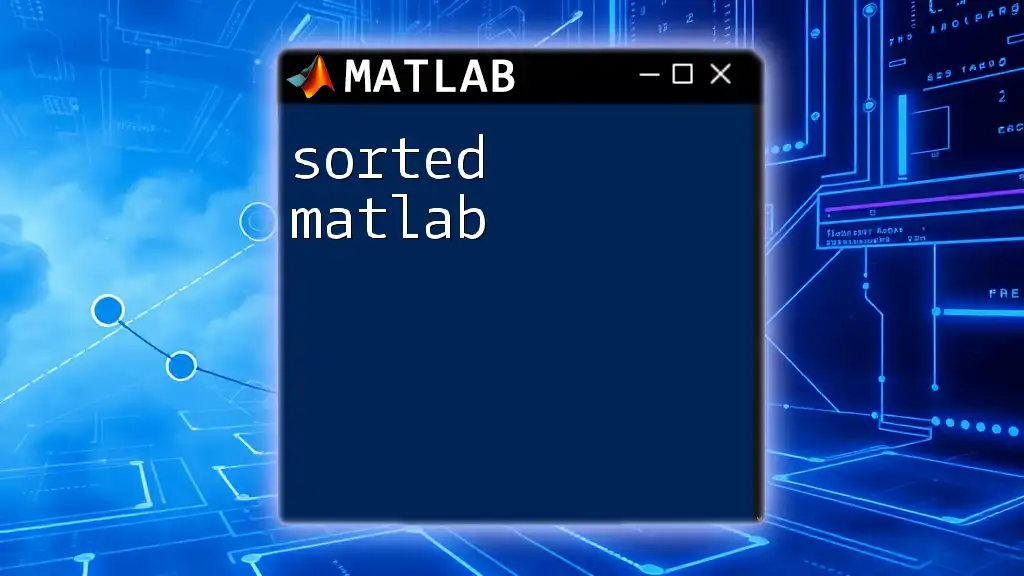
Common Errors and Troubleshooting
When using `normpdf`, you may encounter some common pitfalls. Input-related errors often arise when `mu` or `sigma` are not correctly specified as scalar values, leading to dimension mismatch or other unexpected errors. It is crucial to ensure that the dimensions of `x`, `mu`, and `sigma` are consistent with each other.
If the output from `normpdf` seems illogical—perhaps a value is unexpectedly high or low—it’s essential to double-check that inputs are correctly defined and reflect a plausible normal distribution. Remember to validate your `mu` and `sigma` values, especially making sure that `sigma` is positive, as a non-positive standard deviation does not make sense in the context of probability density functions.
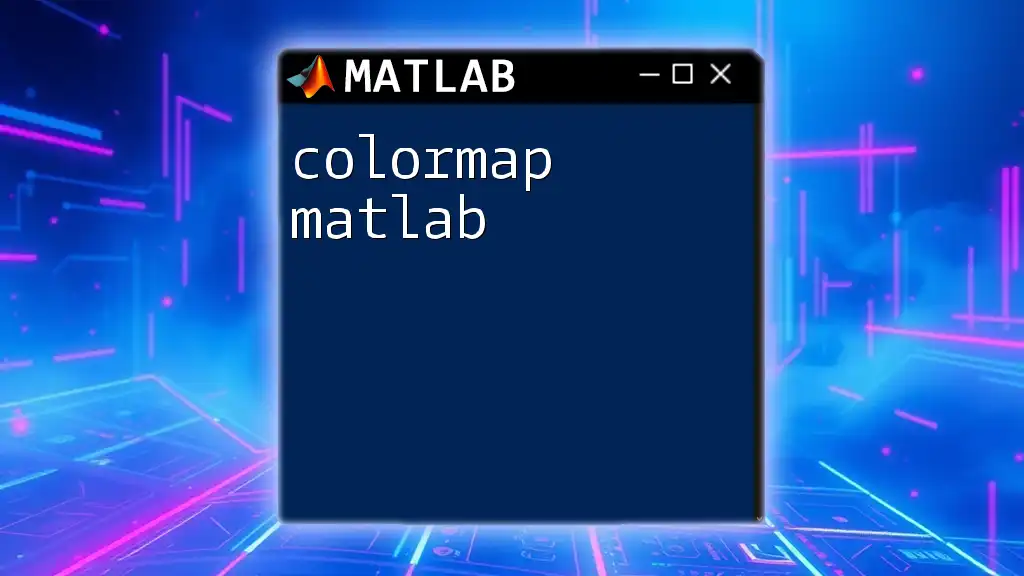
Practical Applications of `normpdf`
In Statistics
`normpdf` plays a vital role in statistics, enabling practitioners to calculate the probability density for various continuous random variables. It is often foundational in performing hypothesis testing, confidence interval estimation, and any statistical analysis that relies on the normal distribution.
In Finance
In finance, `normpdf` finds applications in modeling risks and predicting stock returns. A normal distribution is sometimes assumed for the return on investments, making `normpdf` an essential tool for risk assessment, portfolio optimization, and pricing derivative instruments.
In Engineering
Engineers frequently apply `normpdf` in quality control, where it aids in understanding variations in product measurements. Statistical process control often employs normal distribution techniques, making `normpdf` invaluable in settings that require ensuring product reliability and conformance to specifications.
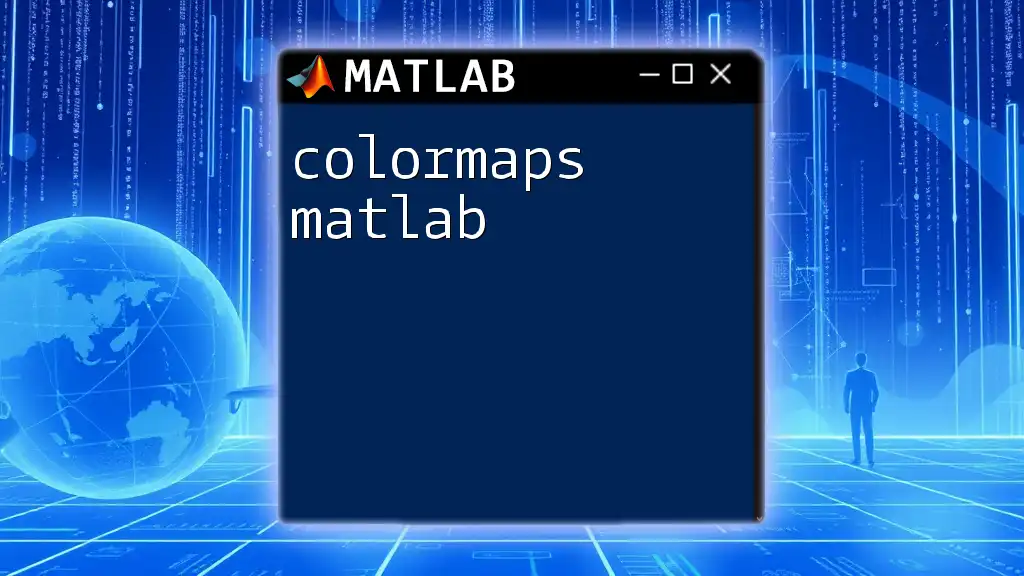
Summary of Key Points
To conclude, `normpdf` in MATLAB is an essential function for computing normal probability densities across a range of applications in various fields. By mastering its syntax, understanding its parameters, and learning to troubleshoot it effectively, you’ll be equipped to leverage the power of the normal distribution in your analytical pursuits.
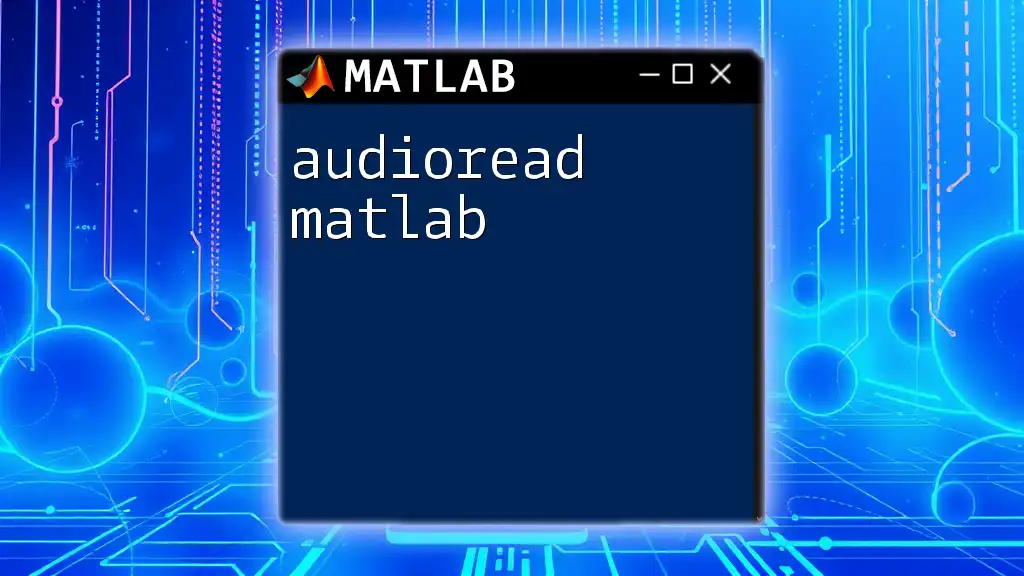
Further Reading and Resources
For those looking to deepen their understanding, exploring MATLAB’s official documentation around probability distributions and statistics can provide further insights. Additionally, various online tutorials and guides will support the practical application of `normpdf` in diverse contexts.
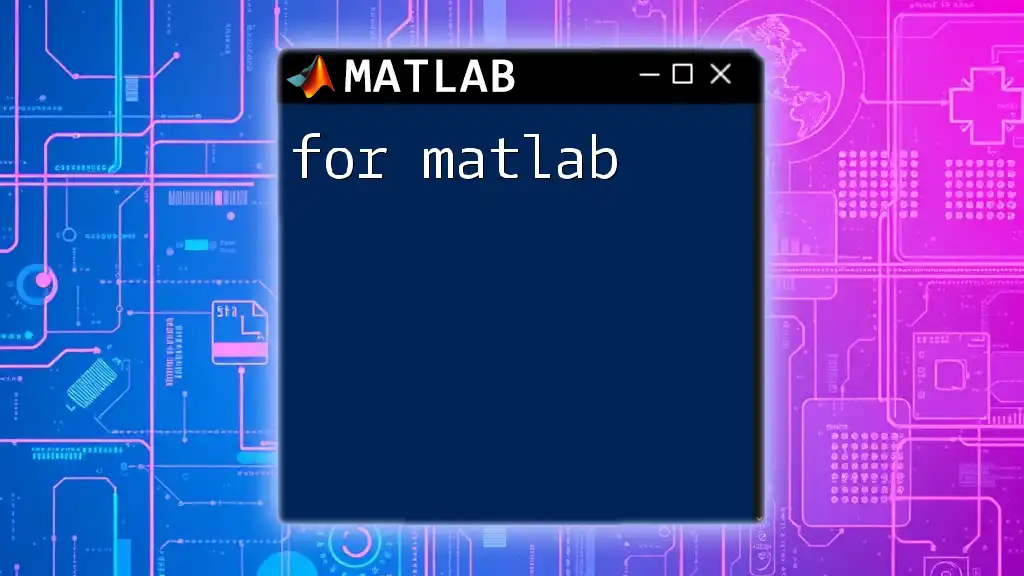
Call to Action
Now that you have a comprehensive understanding of `normpdf` in MATLAB, it’s time to dive in and practice implementing this function in your projects. If you have any questions or comments about using `normpdf`, feel free to leave a message. Happy coding!