In MATLAB, you can calculate the natural logarithm of a number using the `log` function, which returns the logarithm base \( e \) of the input value.
% Calculate the natural logarithm of a number
x = 10;
natural_log = log(x);
disp(natural_log);
Understanding Natural Logarithms
What is a Natural Logarithm?
The natural logarithm, denoted as ln, is the logarithm to the base e, where e is approximately equal to 2.71828. The natural log function is fundamental in various branches of mathematics, particularly in calculus and complex analysis.
The properties of the natural logarithm are crucial to understand:
- ln(e) = 1: The natural logarithm of e is 1, which forms a foundational point in understanding logarithmic relationships.
- ln(1) = 0: The natural logarithm of 1 is always 0, implying that any number raised to the power of 0 equals 1.
- Logarithmic identities: For instance, the identity ln(ab) = ln(a) + ln(b) reveals how multiplication in the argument converts to addition in the logarithm.
The Mathematical Foundation
At its core, the natural logarithm is the inverse function of the exponential function. This means if \( y = ln(x) \), then \( x = e^y \). A graphical representation of this relationship often showcases the natural log function's growth, which approaches infinity but never touches the x-axis (as it is undefined for values less than or equal to zero).
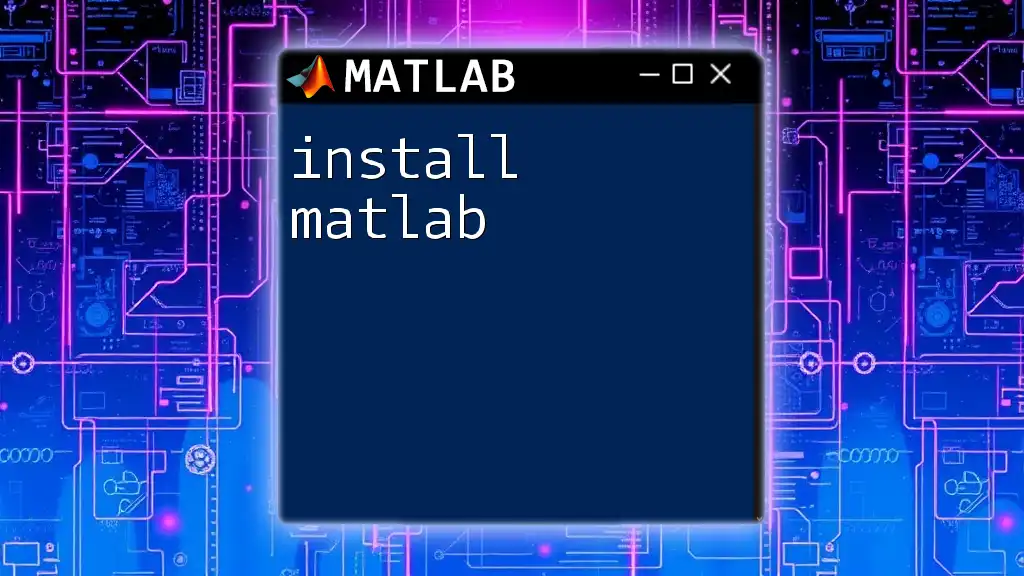
How to Calculate Natural Logarithms in MATLAB
The `log` Function
In MATLAB, the natural logarithm can be calculated using the `log` function. Its syntax is straightforward:
result = log(x)
Here, x must be greater than zero. If you pass zero or a negative number, MATLAB will return NaN (Not a Number).
Example of Using the `log` Function
Consider an array of numbers for which we want to calculate the natural logarithm:
% Example: Calculate natural logarithm of numbers
values = [1, exp(1), exp(2)];
naturalLogs = log(values);
disp(naturalLogs);
When executed, this code will yield: `0, 1, 2`, matching the expectations given the properties of logarithms. The results confirm that the calculator indeed processes the natural logarithm accurately for the specified values.
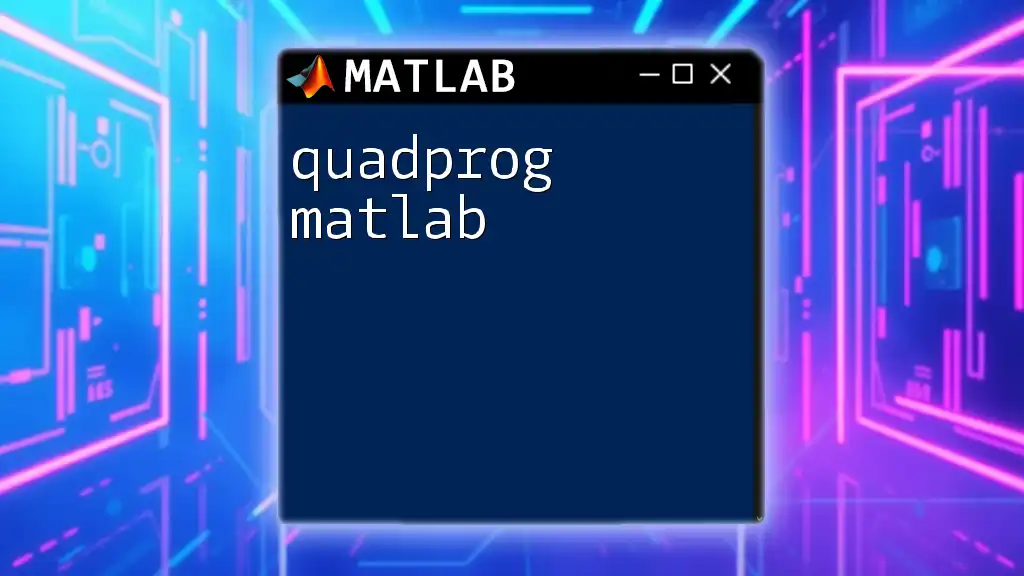
Dealing with Complex Numbers
Complex Logarithms in MATLAB
In MATLAB, complex logarithms can also be computed. When you are dealing with complex numbers, the `log` function still applies, but it's important to understand how it works:
result = log(z)
In this case, z can be any complex number.
Example of Complex Logarithms
Let's say we want to find the natural logarithm of a complex number such as \( 1 + 2i \):
% Example: Calculate natural logarithm of a complex number
complexValue = 1 + 2i;
naturalLogComplex = log(complexValue);
disp(naturalLogComplex);
The output will provide a complex result indicating the logarithm's behavior when applied to numbers with real and imaginary components. This is a vital tool for those engaging with complex analysis.
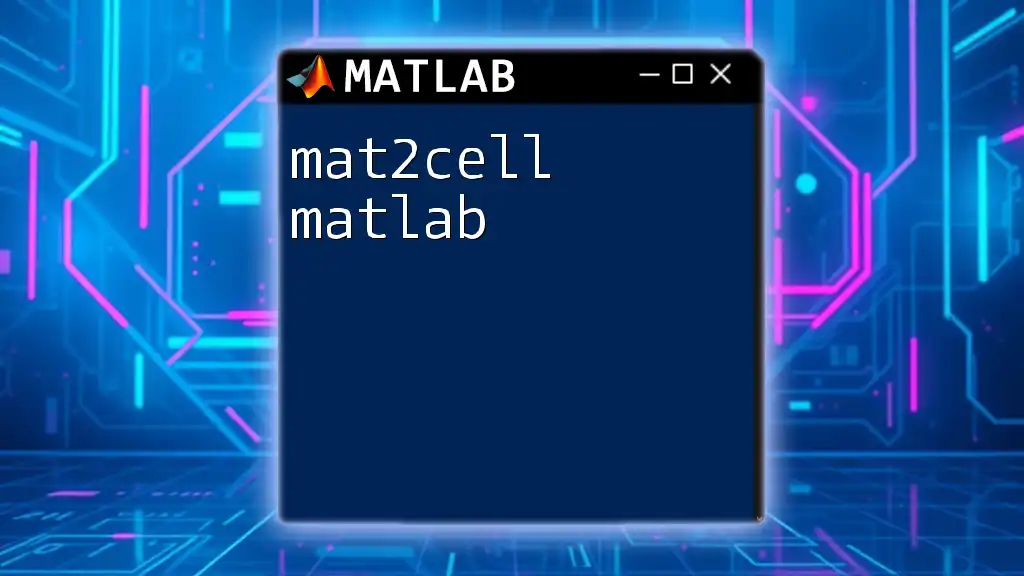
Properties of the `log` Function
Key Properties and Relationships
One of the benefits of logarithmic functions is that they adhere to certain properties that transcend their base. For example, you can leverage these identities in MATLAB:
- The identity ln(a*b) converts to ln(a) + ln(b).
- Similarly, ln(a/b) can be computed as ln(a) - ln(b).
Example Demonstration of Properties
To verify these logarithmic properties in MATLAB, consider the following example:
% Example: Verifying the property ln(a*b) = ln(a) + ln(b)
a = 2;
b = 3;
ln_product = log(a * b);
ln_sum = log(a) + log(b);
disp(['ln(a*b) = ', num2str(ln_product), ' and ln(a) + ln(b) = ', num2str(ln_sum)]);
When you run this code, you'll find that both results match, demonstrating the reliability of logarithmic properties within MATLAB.
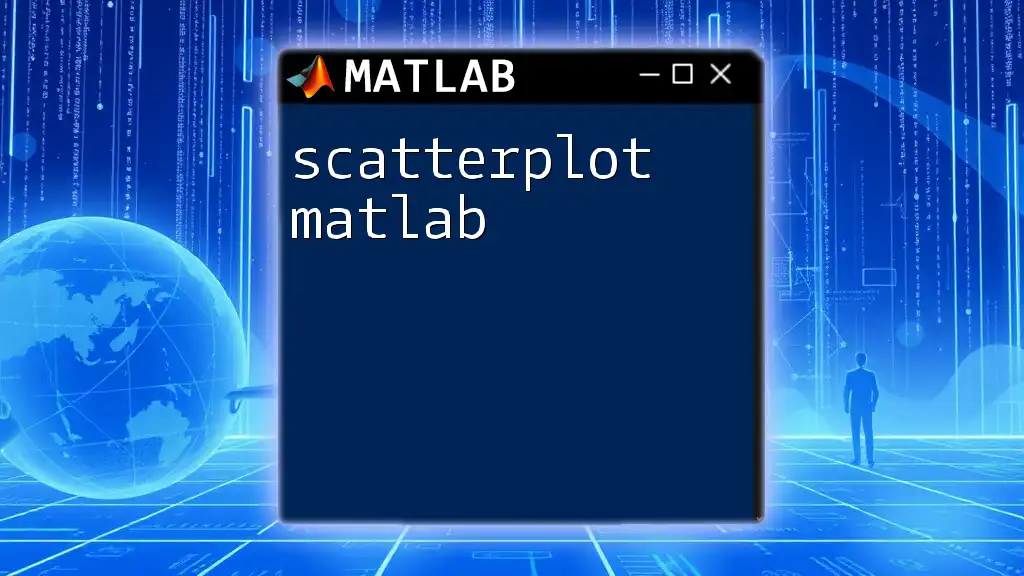
Common Applications of Natural Logarithms in MATLAB
Data Transformation
Natural logarithms are extensively used in data analysis for transforming data. This transformation can be particularly helpful in normalizing datasets or stabilizing variance in certain distributions.
Here’s how you might implement a log transformation on a dataset:
% Example: Data transformation using natural logarithm
data = [10, 20, 30, 40, 50];
logTransformedData = log(data);
disp(logTransformedData);
Executing this script will provide log-transformed values, enabling easier comparative analysis across different scales of data.
Statistical Analysis
Natural logarithms are also crucial in statistical modeling. A common application is within the context of fitting a log-normal distribution. This distribution is particularly useful when the data is positively skewed.
To visualize the fit of a log-normal distribution, consider the following code snippet:
% Example: Fitting a log-normal distribution
data = lognrnd(0, 1, 1000, 1); % Generate log-normal data
histogram(data, 'Normalization', 'pdf');
hold on;
x = linspace(0, 10, 100);
pdf = lognpdf(x, 0, 1);
plot(x, pdf, 'r', 'LineWidth', 2);
hold off;
In this example, the histogram will show the distribution of the generated log-normal data, complemented by the theoretical probability density function overlayed in red.
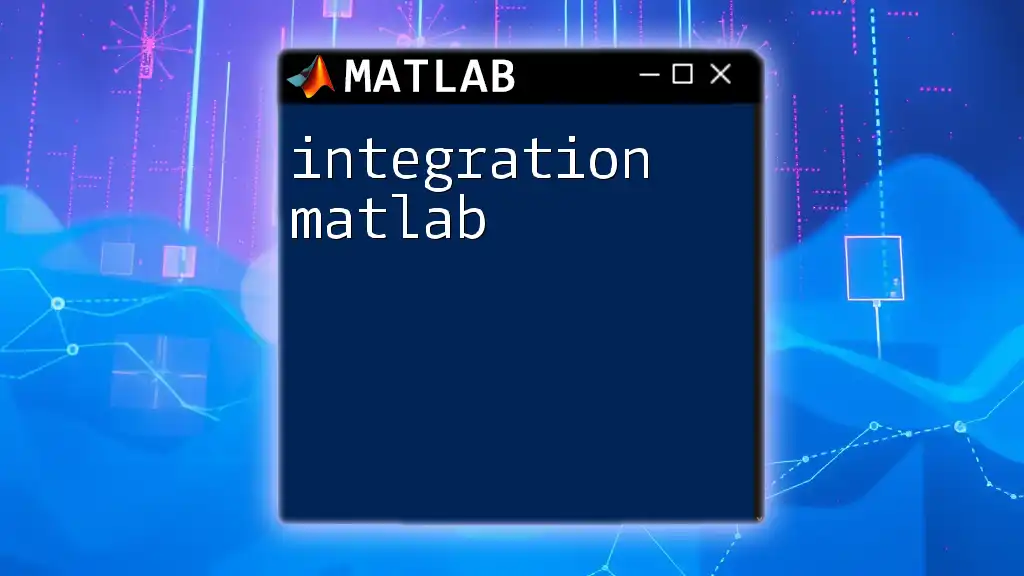
Troubleshooting Common Issues
Common Errors When Using `log`
While using the `log` function in MATLAB is straightforward, users occasionally encounter issues. Common warnings arise when input values are zero or negative, leading to NaN outputs.
How to Fix These Errors
To avoid these pitfalls, always ensure your input values remain above zero. For datasets, it may be beneficial to pre-process the data to remove or adjust invalid values before applying the logarithm function.
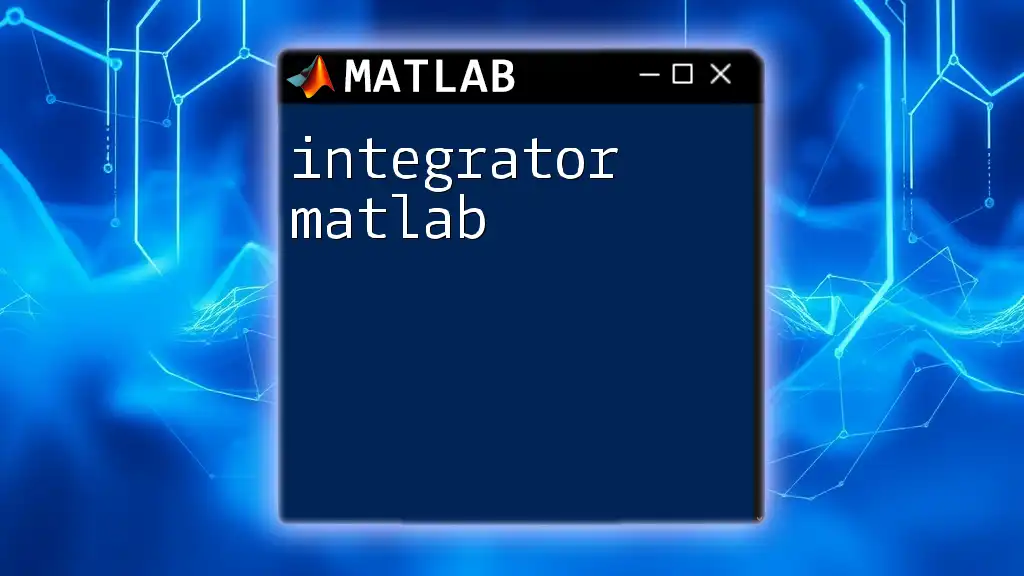
Conclusion
Natural logarithms are an invaluable tool in MATLAB, offering simplicity and power to calculations across various domains. Whether you're analyzing data or modeling distributions, understanding the nature of the natural log function helps expand your capabilities in MATLAB.
In practice, it's essential to experiment and utilize these functions to develop a strong grasp of their potential in real-world applications. With practice, the command will become a second nature, enhancing both your programming skills and analytical prowess.
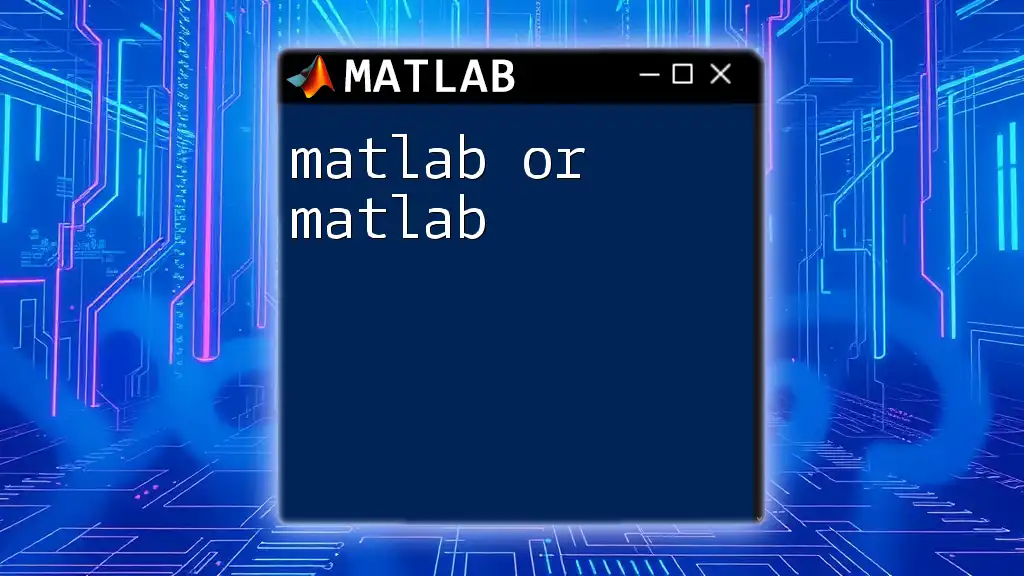
Additional Resources
For more in-depth learning and resources, consider checking the official MATLAB documentation. Books and articles focused on logarithmic functions and MATLAB programming can also provide deeper insights and advanced techniques in mastering the use of natural logarithms.
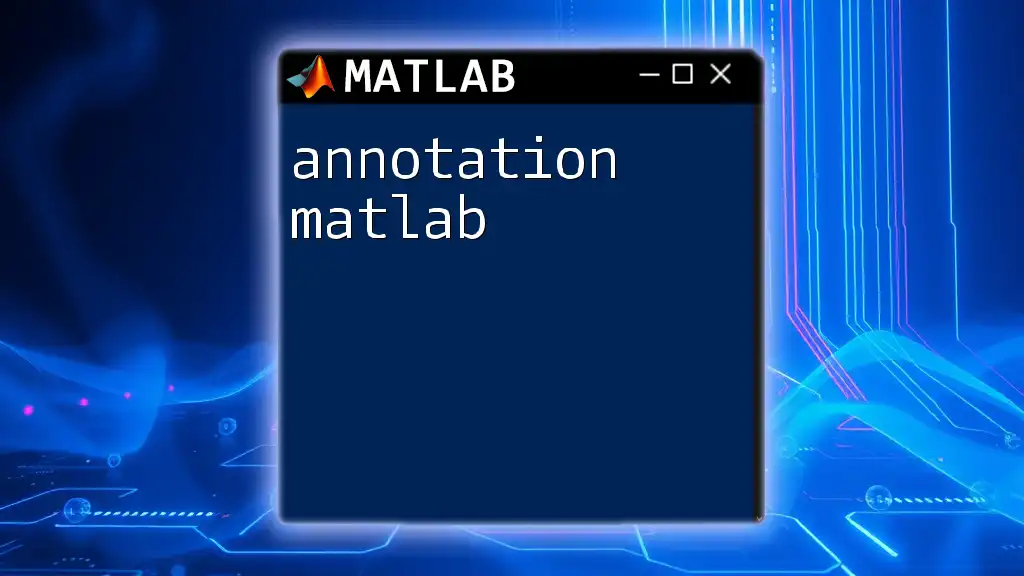
Call to Action
Join our MATLAB tutorial company for extensive training and resources focused on practical applications in MATLAB programming, and start your journey to mastering the natural log in MATLAB today! Share this guide with fellow learners to broaden their understanding as well!