"Getting started with MATLAB from MathWorks involves familiarizing yourself with its interactive environment and basic syntax, allowing you to execute powerful mathematical computations efficiently."
Here's a simple example of how to create and manipulate a matrix in MATLAB:
% Create a 2x2 matrix
A = [1, 2; 3, 4];
% Calculate the transpose of the matrix
B = A';
Understanding the MATLAB Environment
MATLAB Interface
The MATLAB desktop environment is user-friendly and designed to enhance productivity by providing easy access to all of MATLAB's features. In this environment, you'll encounter several key components:
- Command Window: This is where you can enter commands and view output directly. It's the primary interface for interaction.
- Workspace: This area displays all the variables that you create during your session. You can see their values and types, making it easy to manage your data.
- Editor: Here, you can write scripts and functions. The Editor provides features like syntax highlighting, debugging tools, and code formatting.
Familiarizing yourself with these tools is crucial for efficient use of MATLAB as a programming environment.
Toolboxes and Add-ons
MATLAB's power lies in its toolboxes, which are collections of specialized functions for specific applications, such as signal processing, image processing, and control systems. Add-ons can be installed to expand your capabilities even further. Understanding the available toolboxes relevant to your field will save you time and effort as you can leverage pre-built functions tailored to your needs.
Getting Help and Documentation
Learning to use the help function within MATLAB can significantly enhance your learning experience. By typing:
help function_name
you can access quick documentation on any built-in function directly in the Command Window. This is a great way to learn about syntax, parameters, and example usage without needing to leave the MATLAB environment.
The official MathWorks documentation is an invaluable resource, providing comprehensive guides, tutorials, and example scripts. Regularly consult this documentation as you familiarize yourself with MATLAB; it is essential for developing a deeper understanding.

Basic MATLAB Commands
Variables and Data Types
Declaring variables in MATLAB is straightforward. You can easily create variables by assigning values like so:
x = 10; % Integer
y = 3.14; % Float
z = 'Hello'; % String
In MATLAB, the most common data type is the double – a floating-point number. This simplicity allows you to focus on your calculations rather than data type management.
It's also important to explore other data types such as cell arrays and structures, which come in handy for organizing complex data sets. For example, you can create a 1D cell array like this:
myCellArray = {10, 'Text', [1, 2, 3]};
Operations and Functions
MATLAB excels at performing mathematical operations. The basic operations include addition, subtraction, multiplication, and division:
result = x + y; % Addition
Make sure to become familiar with operator precedence to ensure your expressions evaluate as expected. Learn how to use parentheses effectively — they influence the order of operations in your calculations.
Built-in functions such as `sum`, `mean`, and `max` are essential to MATLAB programming. For instance, to sum a vector of numbers:
total = sum([1, 2, 3, 4, 5]);
These functions save you from writing complex loops for common tasks and enhance code readability.

Control Structures
Conditional Statements
Conditional statements allow you to implement logic in your code. The if-else statement is crucial for decision-making processes:
if x > 5
disp('x is greater than 5');
else
disp('x is less than or equal to 5');
end
With this structure, you can guide the flow of your program based on variable values, which is especially useful in simulations and data analysis.
Loops
Loops enable you to run repetitive tasks efficiently. The for loop is particularly beneficial:
for i = 1:5
disp(i);
end
This loop will display numbers from 1 to 5, showcasing how easy it is to execute repetitive tasks with concise syntax.
In addition to the for loop, the while loop is vital for situations where the number of iterations isn't known beforehand:
j = 1;
while j <= 5
disp(j);
j = j + 1;
end
Each loop constructs a different approach to managing iterations based on your needs.

Plotting Data in MATLAB
Basic Plotting Functions
One of the most powerful features of MATLAB is its data visualization capabilities. You can create simple graphs using the `plot` function:
x = 0:0.1:10; % X data
y = sin(x); % Y data
plot(x, y); % Create plot
This line of code generates a basic sine wave plot. Visualization is crucial for interpreting data trends and patterns.
Customizing Plots
MATLAB allows you to enhance your plots with details. Adding titles and labels is a great way to make your visuals clearer and more informative:
title('Sine Wave');
xlabel('X-Axis');
ylabel('Y-Axis');
Customizing your plots is essential for presenting data effectively, particularly in academic or professional settings where clarity is paramount.
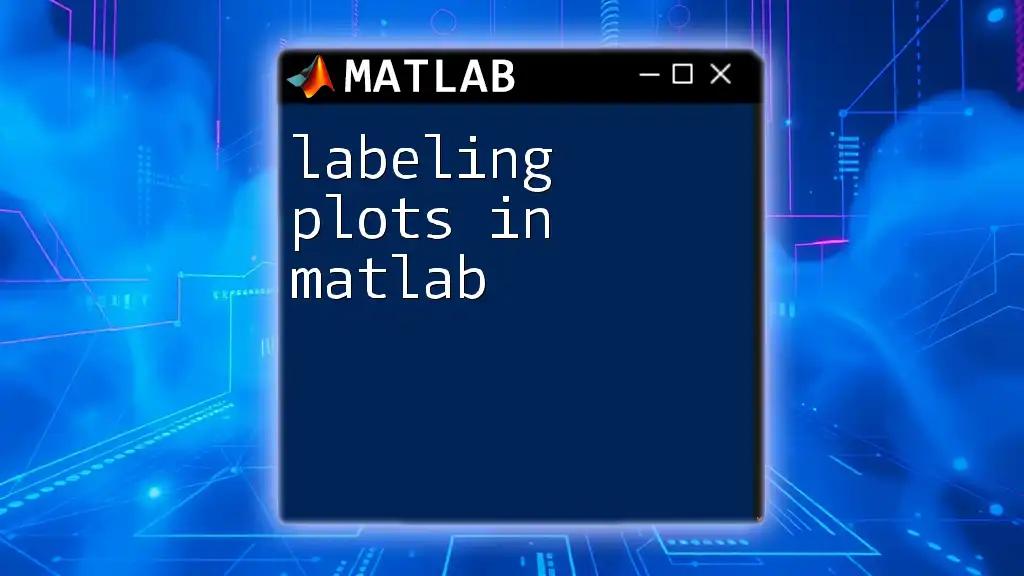
Working with Matrices
Matrix Creation
MATLAB’s name comes from “matrix laboratory,” emphasizing its strength in matrix operations. You can define matrices as follows:
A = [1, 2, 3; 4, 5, 6]; % 2x3 matrix
Matrices allow you to organize data compactly and are fundamental for many mathematical operations.
Matrix Operations
MATLAB simplifies performing mathematical operations on matrices. You can add, subtract, or multiply matrices straightforwardly:
B = [1, 0; 0, 1]; % Identity matrix
C = A * B; % Matrix multiplication
Matrix manipulation is a cornerstone of many algorithms in engineering and data analysis, making this knowledge vital.
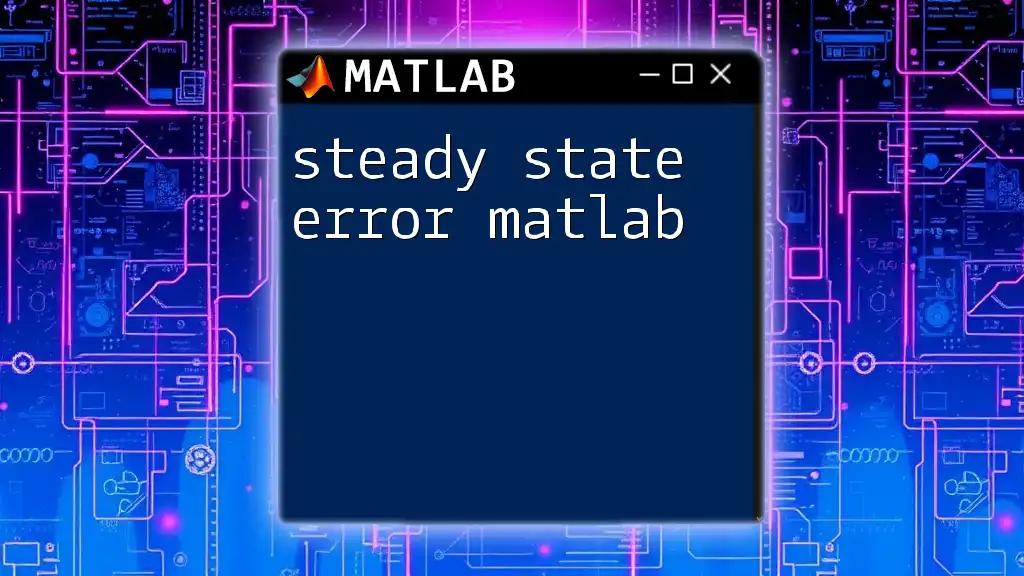
Conclusion
In this comprehensive guide to getting started with MATLAB MathWorks, we covered essential concepts such as the MATLAB environment, basic commands, control structures, plotting data, and matrix operations. Understanding these foundational aspects equips you to tackle more complex problems and projects.
As you continue your journey with MATLAB, consider exploring online courses, tutorials, and further reading materials available through MathWorks and the broader community. MATLAB is a powerful tool that can unlock your potential in engineering, data science, and beyond. Embrace the learning experience, and don't hesitate to leverage the abundant resources at your disposal!