The `dsolve` function in MATLAB is used to symbolically solve ordinary differential equations (ODEs) in a straightforward manner.
Here’s a code snippet demonstrating its usage:
syms y(t)
Dy = diff(y, t);
ode = Dy + 2*y == 0; % Example ODE: dy/dt + 2y = 0
sol = dsolve(ode, y(0) == 1); % Solve with initial condition y(0) = 1
disp(sol)
Introduction to dsolve
dsolve is a powerful function in MATLAB that allows users to solve differential equations symbolically. This is particularly beneficial in various fields such as engineering, physics, and applied mathematics, where understanding and interpreting the behavior of dynamic systems is crucial.
Understanding Differential Equations
Differential equations are mathematical equations that involve functions and their derivatives. They can be categorized into two primary types: Ordinary Differential Equations (ODEs), which contain functions of one variable and their derivatives, and Partial Differential Equations (PDEs), which contain multivariable functions and the respective partial derivatives.
Real-world applications of differential equations span a vast array of disciplines, including population modeling, heat conduction, and electrical circuits. Successfully solving these equations provides insights into complex systems' behavior over time.
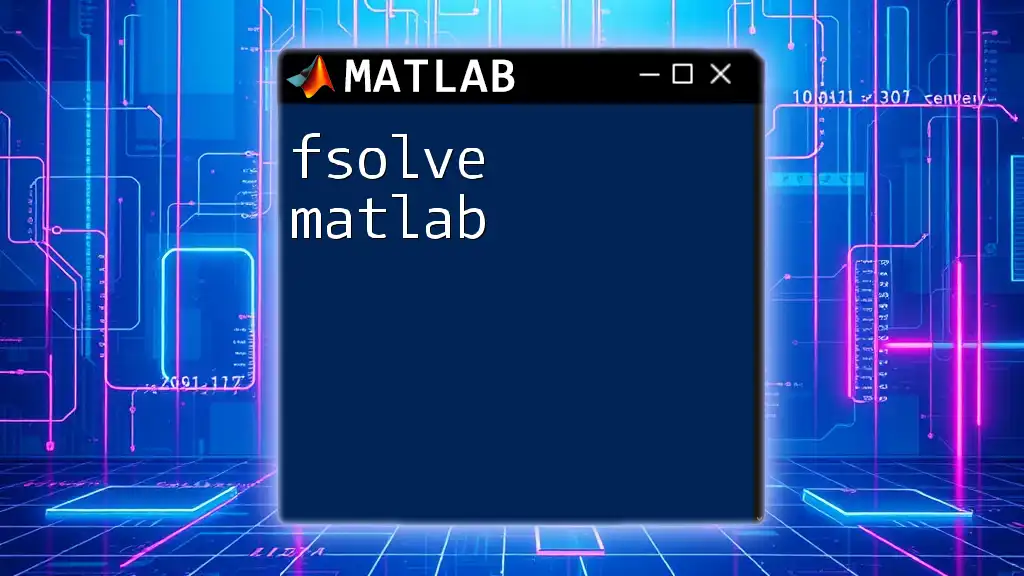
Getting Started with dsolve
Setting Up MATLAB
To get started with `dsolve`, you need to have MATLAB installed, along with the Symbolic Math Toolbox. If you're a new user, you can find installation instructions on the official MATLAB website. Once installed, you can access the toolbox through the MATLAB command window.
Basic Syntax of dsolve
The general syntax of the `dsolve` command is structured as follows:
sol = dsolve(equation, conditions)
Here, `equation` denotes the differential equation or system of equations you want to solve, while `conditions` are optional parameters like initial or boundary conditions that can refine your solution.
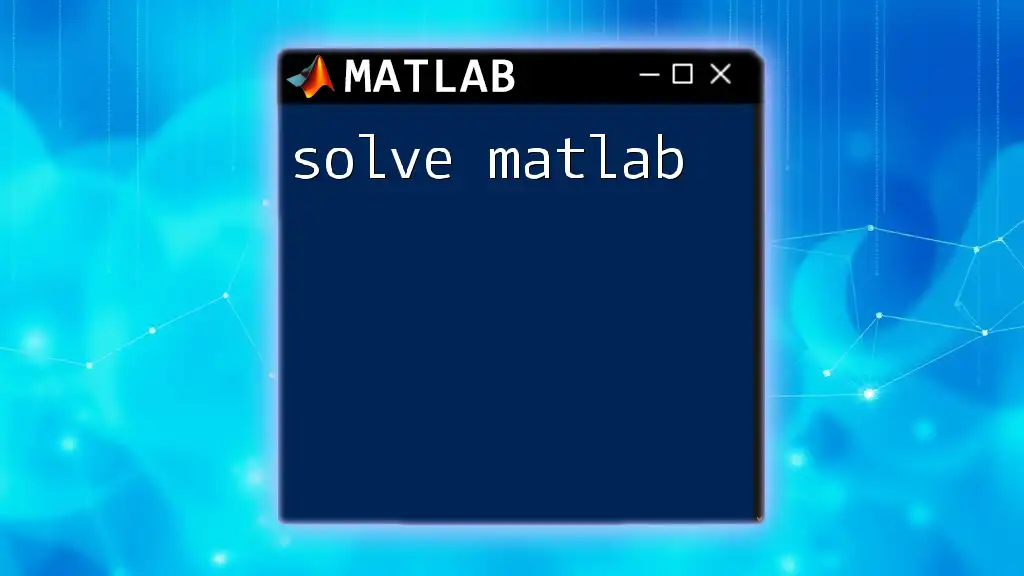
Solving Ordinary Differential Equations (ODEs)
First-Order ODEs
First-order ODEs are equations that involve only the first derivative of a function. The general form can be described as:
\[ \frac{dy}{dx} = f(y, x) \]
Example 1: Simple First-Order Equation
Consider the equation \( \frac{dy}{dx} = y \). To solve it using `dsolve`, you would use the following code:
syms y(x)
ode = diff(y, x) == y;
sol = dsolve(ode);
This command assigns the symbolic variable `y` as a function of `x`, defines the first-order ODE, and then applies `dsolve` to find a solution. The output is \( y(x) = C_1 e^x \), where \( C_1 \) is a constant determined by initial or boundary conditions.
Second-Order ODEs
Second-order ODEs involve the second derivative of a function, typically expressed as:
\[ \frac{d^2y}{dx^2} + p \frac{dy}{dx} + qy = 0 \]
Example 2: Homogeneous Second-Order Equation
Take the equation \( y'' + 3y' + 2y = 0 \). The MATLAB command looks like this:
syms y(x)
ode = diff(y, x, 2) + 3*diff(y, x) + 2*y == 0;
sol = dsolve(ode);
Upon executing this code, you will receive a solution in the form \( y(x) = C_1 e^{-2x} + C_2 e^{-x} \), where \( C_1 \) and \( C_2 \) are constants.
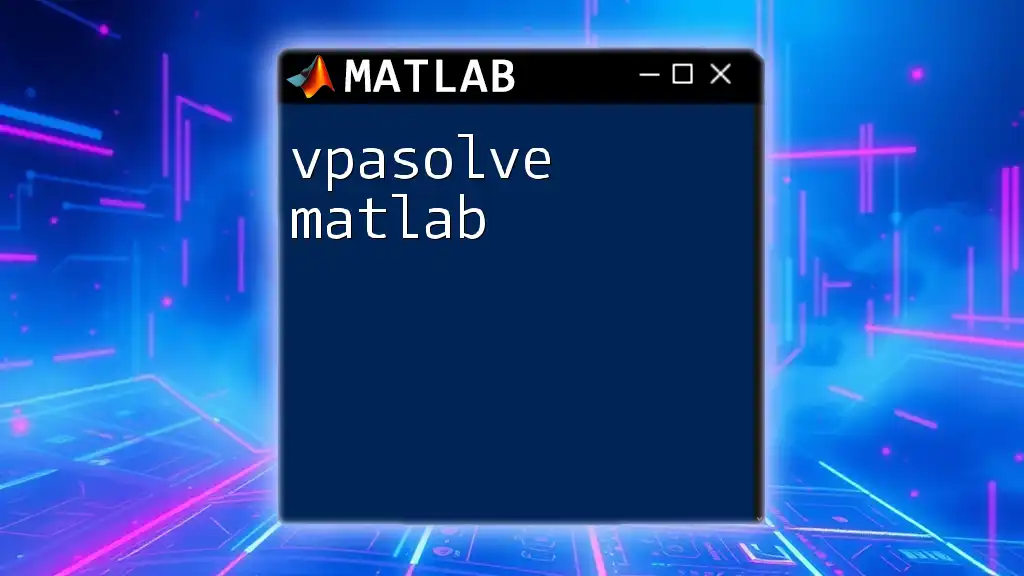
Solving Non-Homogeneous ODEs
Understanding Non-Homogeneous ODEs
Non-homogeneous ODEs contain a non-zero function on one side of the equation, which makes them more complex.
Example 3: Simple Non-Homogeneous Equation
For the equation \( y'' + y = \sin(x) \), utilize the following code snippet:
syms y(x)
ode = diff(y, x, 2) + y == sin(x);
sol = dsolve(ode);
This command solves the non-homogeneous equation and provides a general solution that encapsulates both the complementary function and a particular solution.
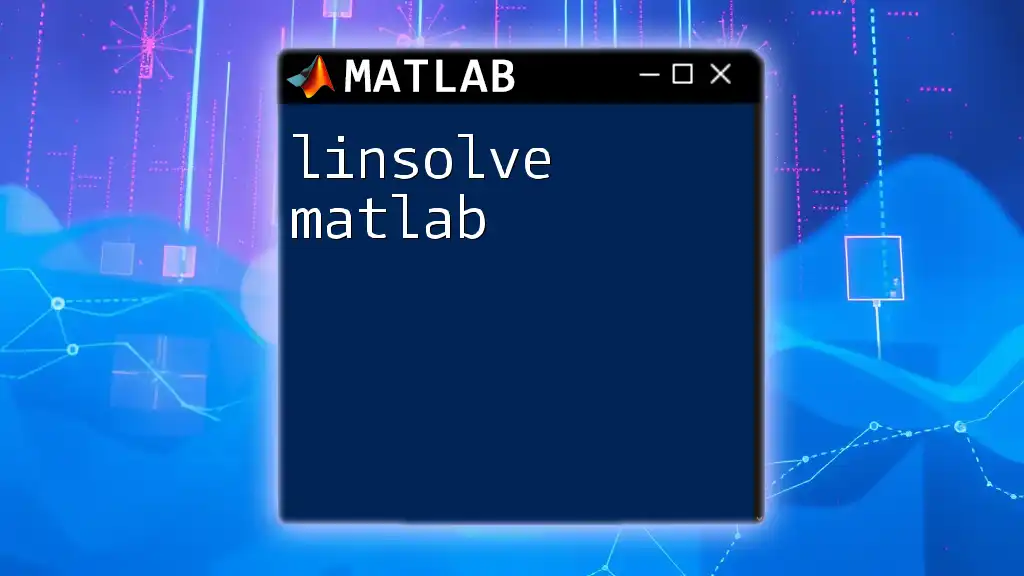
Solving Systems of ODEs
Introduction to Systems of ODEs
Systems of ODEs involve multiple interdependent equations. These often arise in the modeling of chemical reactions, mechanical systems, and other scenarios where two or more variables are linked together.
Example 4: A System of Two Equations
Let's consider the system:
\[ \frac{dy_1}{dt} = y_2, \quad \frac{dy_2}{dt} = -y_1 \]
This can be solved using the following MATLAB commands:
syms y1(t) y2(t)
ode1 = diff(y1, t) == y2;
ode2 = diff(y2, t) == -y1;
sol = dsolve([ode1, ode2]);
The output provides a solution to the coupled system, parameterized by constants derived from your initial conditions.
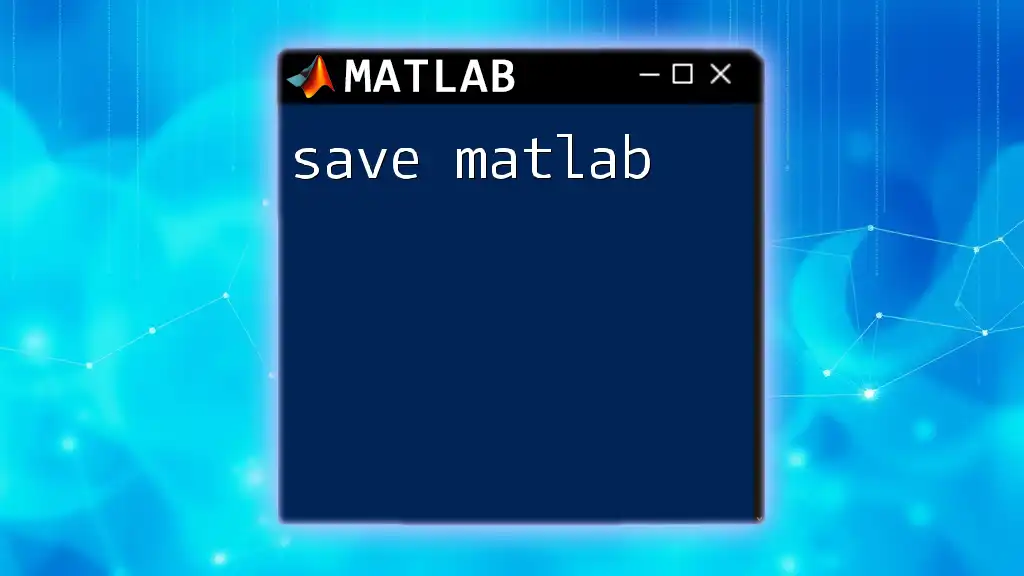
Using Initial Conditions
Importance of Initial Conditions
Initial conditions play a crucial role in obtaining unique solutions for differential equations. They specify values at a particular point, deeply influencing the behavior of the solution curve.
Example 5: Applying Initial Conditions
For the equation \( \frac{dy}{dx} = -2y \) with initial condition \( y(0) = 3 \), the MATLAB code is:
syms y(x)
ode = diff(y, x) == -2*y;
cond = y(0) == 3;
sol = dsolve(ode, cond);
Here, `dsolve` computes the unique solution that satisfies both the ODE and the initial condition, demonstrating how specific numerical values shape the solution's form.
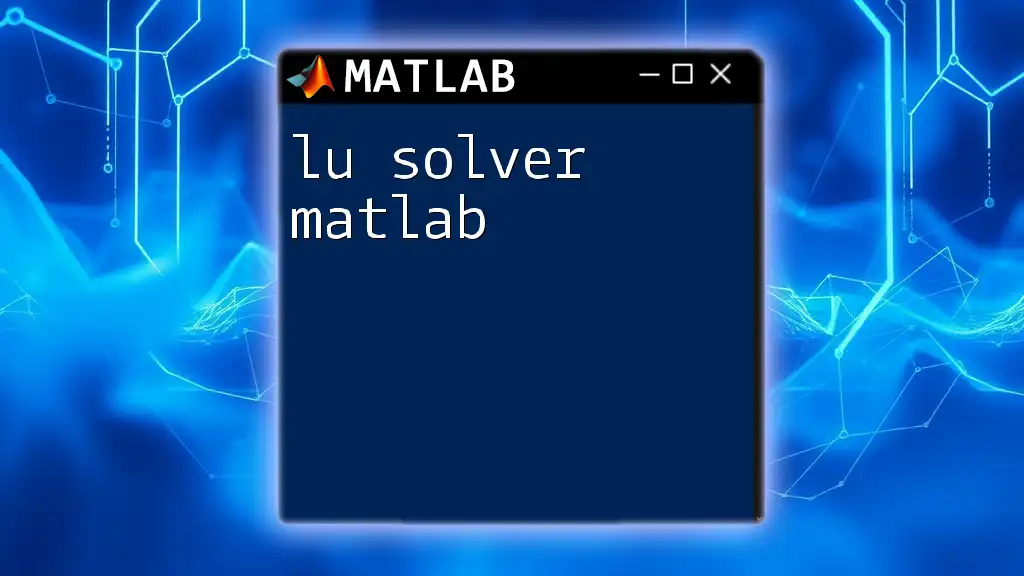
Plotting Solutions
Visualizing the Solution
Visualizing solutions allows for a clearer understanding of the dynamics described by the differential equations.
Example 6: Plotting the Solution
Using the solution from Example 1, you can visualize it with:
fplot(sol, [0, 10]);
title('Solution of dy/dx = y');
xlabel('x-axis');
ylabel('y-axis');
grid on;
This code produces a graph illustrating the exponential growth modeled by the differential equation, enhancing comprehension through visual representation.
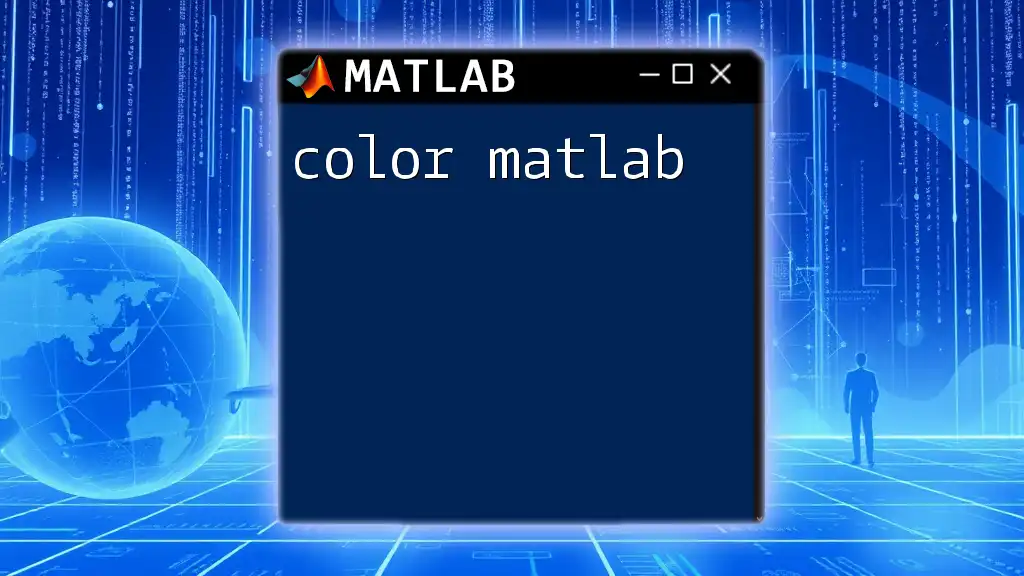
Advanced Features of dsolve
Partial Differential Equations (PDEs)
While `dsolve` is often used for ODEs, it also has capabilities for solving basic PDEs. The syntax remains similar but may require different forms and terms to characterize partial derivatives.
Options and Parameters
`dsolve` offers various options and parameters that allow for more sophisticated problem-solving. These include specifying assumptions on functions or derivatives, which can streamline the process and improve handling complex problems.
Multiple Unknown Functions
In certain scenarios, you may deal with multiple functions. MATLAB's `dsolve` can handle such cases as long as you define them properly, showcasing its versatility in more intricate systems.
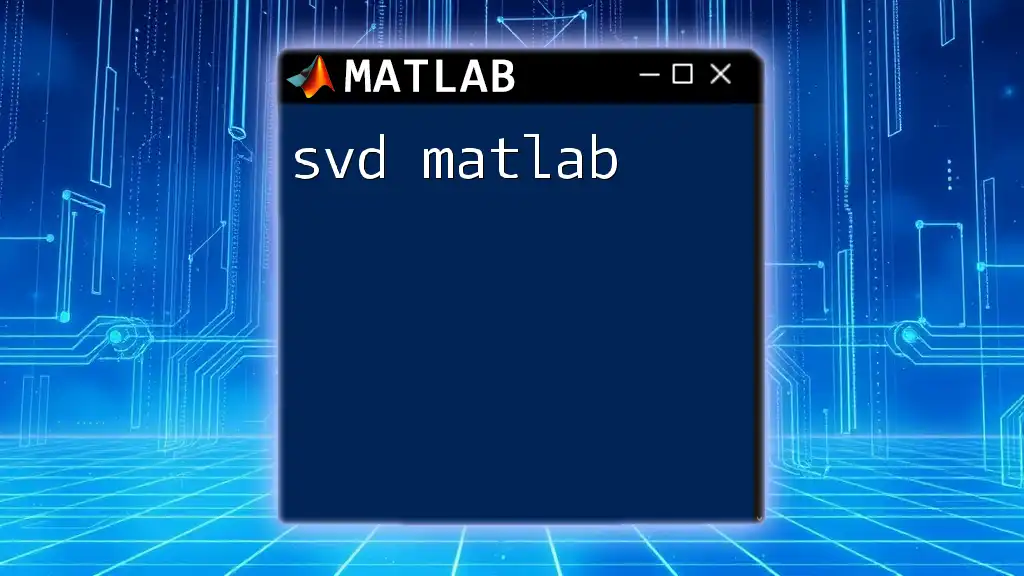
Common Errors and Troubleshooting
Typical Issues Encountered with dsolve
Common pitfalls include syntax errors and mistakes in defining equations or conditions. Ensure that you're using correct MATLAB syntax and the appropriate equation format.
Debugging Tips
When encountering errors, carefully check your code for common mistakes. Use `disp` to print intermediate results or specify initial conditions to better understand the behavior of the solutions.
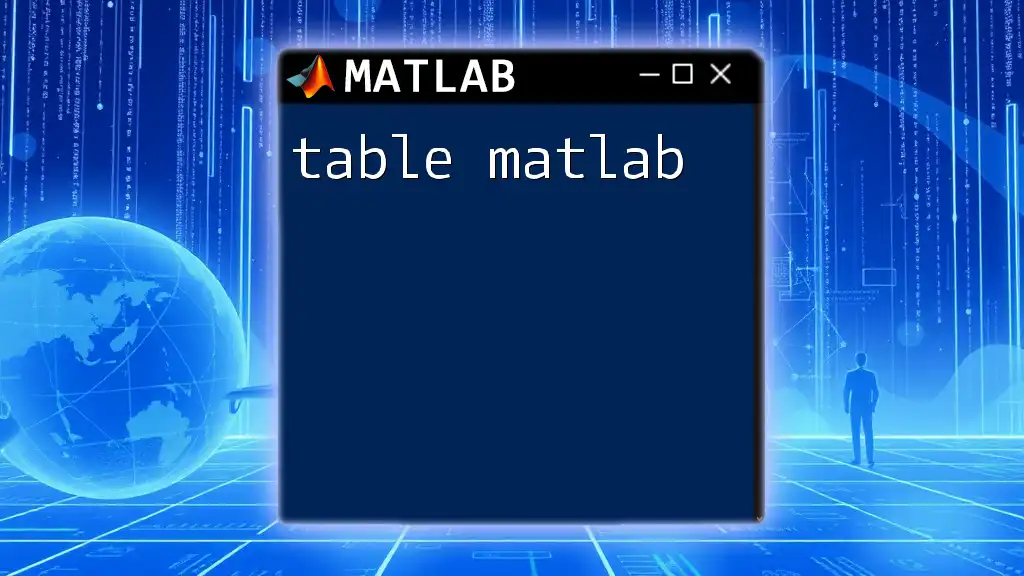
Conclusion
In summary, this comprehensive guide on `dsolve matlab` not only walks through fundamental and advanced topics in solving differential equations with MATLAB but also emphasizes the significance of initial conditions, visualization, and troubleshooting. The flexibility of `dsolve` empowers users to tackle various practical problems in their respective fields.
Encouraging readers to experiment with their own differential equations in MATLAB could lead to deeper understanding and new discoveries. For continued learning, explore additional resources such as documentation, online forums, and MATLAB's vast tutorials on symbolic computation.