To create a 3D plot in MATLAB, you can use the `plot3` function to visualize data points in a three-dimensional space.
Here's a code snippet demonstrating how to create a simple 3D plot:
% Example of a 3D plot in MATLAB
x = 1:10; % X data points
y = rand(1, 10); % Y data points
z = rand(1, 10); % Z data points
plot3(x, y, z, '-o'); % Create 3D plot with line and markers
grid on; % Add grid for better visualization
xlabel('X-axis'); % Label for X-axis
ylabel('Y-axis'); % Label for Y-axis
zlabel('Z-axis'); % Label for Z-axis
title('Simple 3D Plot'); % Title of the plot
Understanding 3D Plots
What is a 3D Plot?
A 3D plot is a graphical representation of data in three dimensions. Unlike 2D plots, which display information on two axes, 3D plots add a depth component, allowing for a more comprehensive visualization of complex datasets. They are essential in fields such as engineering, physics, and data analysis, where understanding the relationships among three variables is crucial.
Types of 3D Plots
Surface Plots are used to depict three-dimensional surfaces and are particularly useful for visualizing gradients and variations in data. They often come with features that allow you to add color coding to represent additional dimensions of information.
Mesh Plots, similar to surface plots, display a grid-like structure but represent the surfaces as lines without filled colors. This style is often used to highlight contours on a surface.
Scatter Plots visualize individual data points within a three-dimensional space, making them ideal for displaying trends and distributions among three distinct variables.
Line Plots allow the visualization of connections between points in a 3D space, often used to demonstrate motion or relationships over time through a series of coordinates.
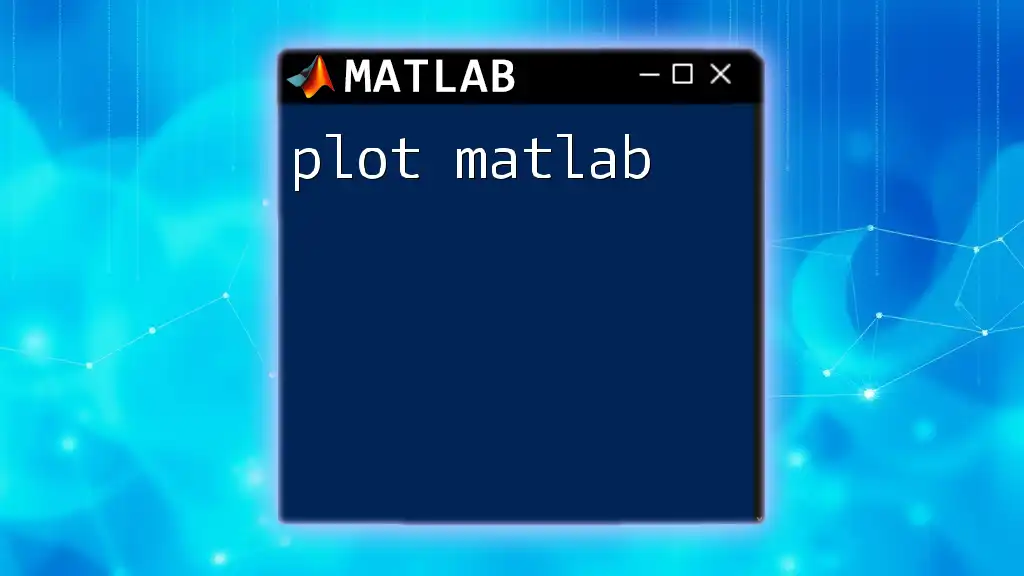
Setting Up MATLAB for 3D Plotting
Installing MATLAB
To start using 3D plotting, you first need to install MATLAB. Download it from the official MathWorks website and follow the installation instructions. Once installed, you can launch the MATLAB interface, where you'll find a rich set of tools for creating various types of plots.
Getting Started with the Workspace
In MATLAB, the workspace is where your data resides. Understanding how to navigate the workspace will significantly enhance your plotting experience. You can save your data in variables, which can then be used directly in your 3D plotting commands.
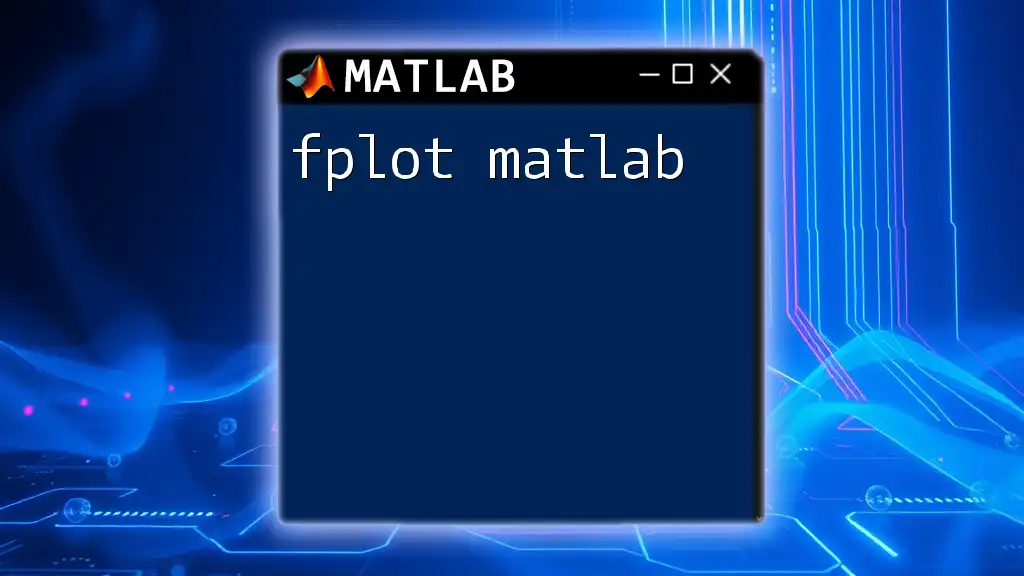
Basic 3D Plotting Commands
Using `plot3()`
The `plot3()` command is one of the most fundamental tools for creating 3D line plots.
Syntax:
plot3(X, Y, Z)
Where `X`, `Y`, and `Z` are vectors of equal length representing the coordinates of points in 3D space.
Example:
x = 0:0.1:10;
y = sin(x);
z = cos(x);
plot3(x, y, z);
grid on;
In this example, `x` represents a range of values, while `y` and `z` are calculated as the sine and cosine of `x`, respectively. The `grid on;` command adds a grid to visualize the 3D space better.
Using `mesh()`
The `mesh()` command creates 3D surface plots with a wireframe representation.
Syntax:
mesh(X, Y, Z)
Where `X`, `Y`, and `Z` are matrices that define the coordinates of the surface.
Example:
[X, Y] = meshgrid(-5:1:5, -5:1:5);
Z = X.^2 + Y.^2;
mesh(X, Y, Z);
Here, `meshgrid` generates a grid of `X` and `Y` values, and `Z` calculates the height based on the xy-plane. This creates a 3D plot showing the paraboloid surface.
Using `surf()`
The `surf()` command builds upon the `mesh` function by creating a filled surface plot.
Syntax:
surf(X, Y, Z)
Similar to `mesh`, this requires the same input but provides additional visual details.
Example:
surf(X, Y, Z);
With `surf`, you can visually enhance the representations with color gradations and shading effects, which help in interpreting the adaptability of surfaces.
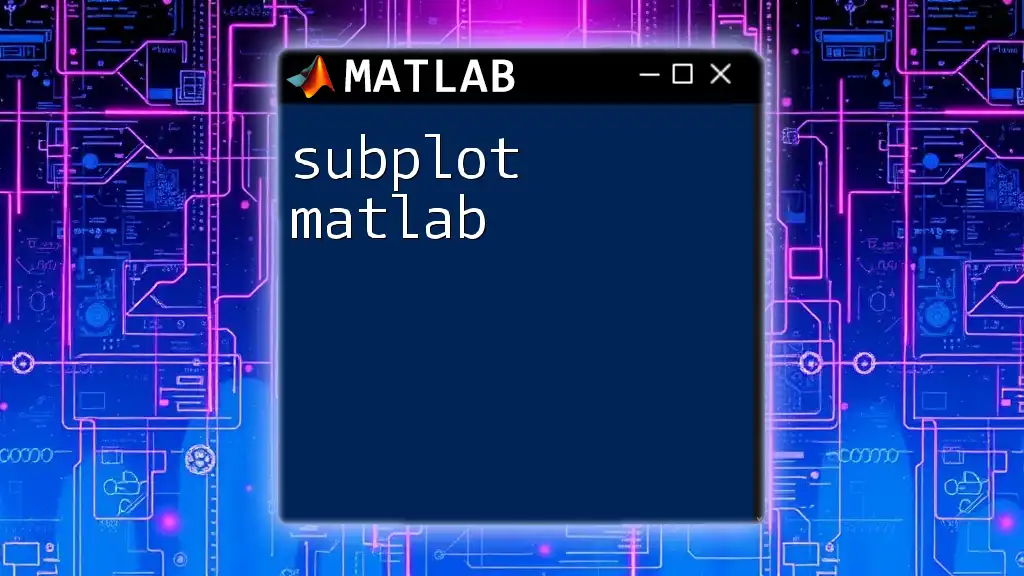
Customizing Your 3D Plots
Adding Labels and Titles
Adding appropriate labels and titles is critical for any plot. It aids in making your visualizations self-explanatory.
Example:
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
title('3D Surface Plot Example');
This code snippet sets the labels for each axis and adds a title to the plot, ensuring your audience understands the subject matter easily.
Changing Viewing Angles
Once your 3D plot is rendered, you might want to adjust the viewing angle to better present your data. The `view()` function allows you to specify the azimuth and elevation angles.
Example:
view(45, 30); % Changes the viewing angle
Playing around with the angles enhances the clarity of the plot, sometimes revealing features that are not evident from other perspectives.
Enhancing Visual Appearance
To make your 3D plots more visually appealing, you can customize the colors, line styles, and markers.
The `colormap()` function provides options for coloring aspects of your plot based on values.
Example:
colormap(jet);
This can manipulate how your plot looks and convey additional information through visual cues.
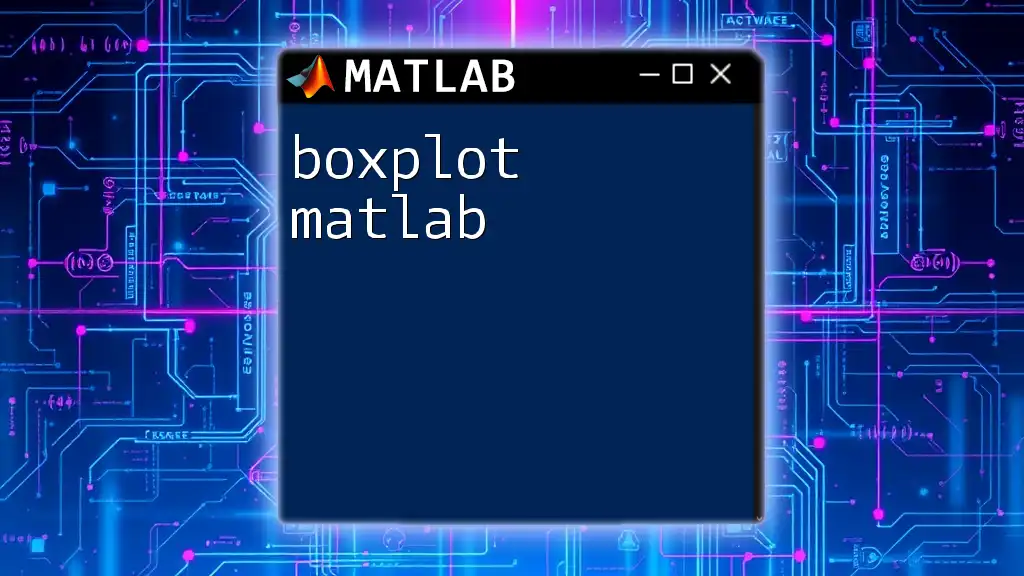
Advanced 3D Plotting Techniques
Animating 3D Plots
Animation can add an engaging dynamic element to your plots, allowing for an illustrative representation of phenomena over time or changes in parameters.
Code Snippet:
for t = 0:0.1:10
plot3(sin(t), cos(t), t);
pause(0.1);
end
The loop creates a simple animation where the point traces a helix in 3D space.
Creating 3D Contour Plots
Contour plots can illustrate the levels of a function in 3D by showing contours, allowing for the visualization of gradients and changes over a surface.
Example:
contour3(X, Y, Z, 50);
This plots the contours of the surface defined by the matrices `X`, `Y`, and `Z`, revealing levels of the function in a 3D format.
Using Additional Toolboxes
MATLAB includes numerous toolboxes such as the Simulink and Mapping Toolbox, which enhance your 3D plotting capabilities significantly. Each toolbox provides specialized functions tailored for unique applications, like modeling and simulation of systems or geographic data visualization.
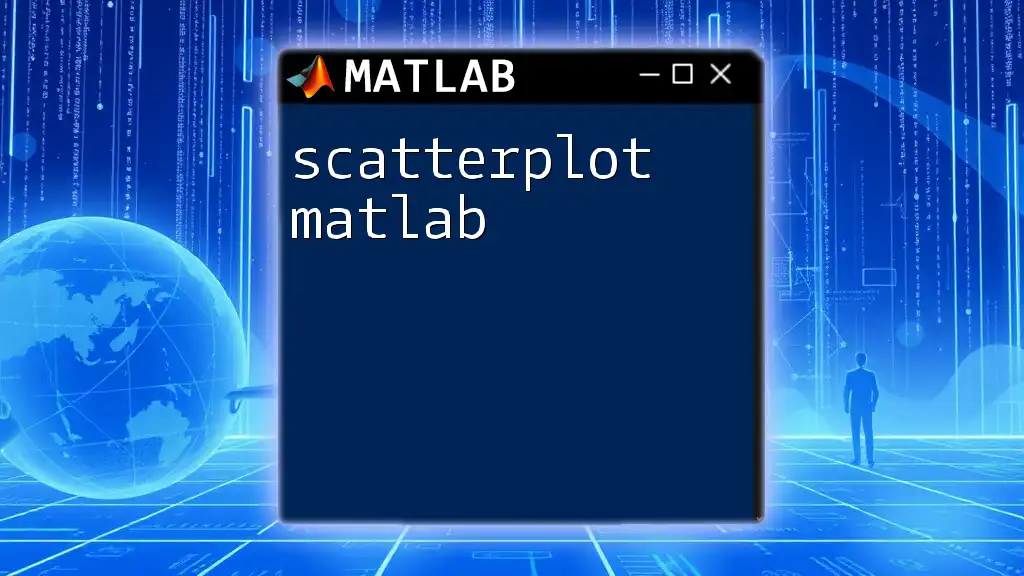
Common Errors and Troubleshooting
Error Messages
While working with 3D plots, you may encounter common errors like mismatched vector sizes. Ensure that the vectors you provide to the plotting functions are of equal length; otherwise, you will see an error message in MATLAB indicating this issue.
Performance Optimization
For large datasets or complex plots, rendering can be slow. To improve performance, consider simplifying the dataset, reducing the number of points plotted, or leveraging built-in optimization techniques provided by MATLAB.
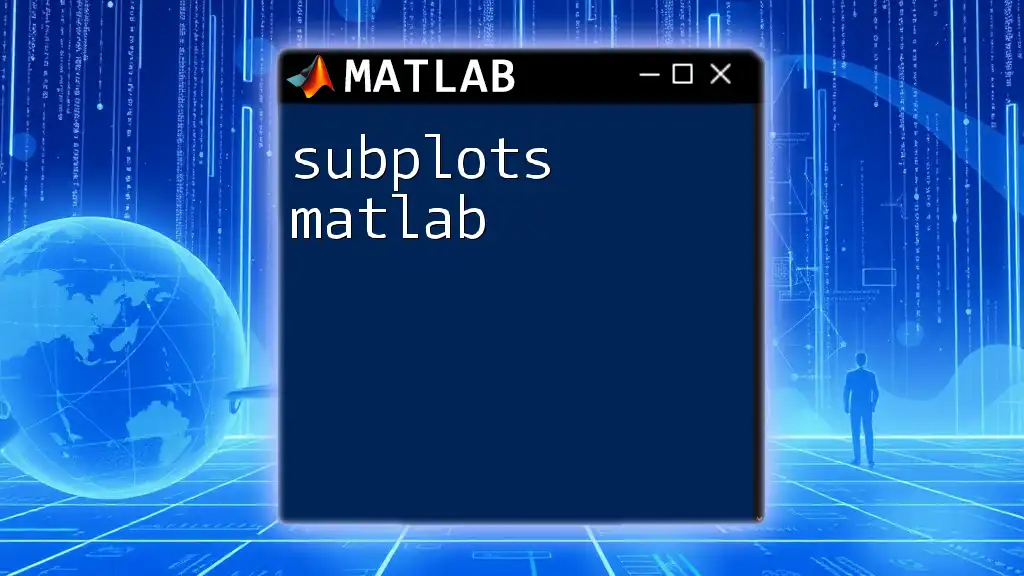
Conclusion
In this guide, we covered how to effectively plot in MATLAB 3D by exploring various commands and techniques. From basic plots like `plot3`, to more advanced techniques such as animating 3D plots, understanding these concepts opens up new avenues for data visualization and analysis.
As you practice these techniques, you’ll be better equipped to represent the complexity of your data and communicate insights effectively. Embrace the versatility of MATLAB’s plotting capabilities and experiment with your own 3D creations!
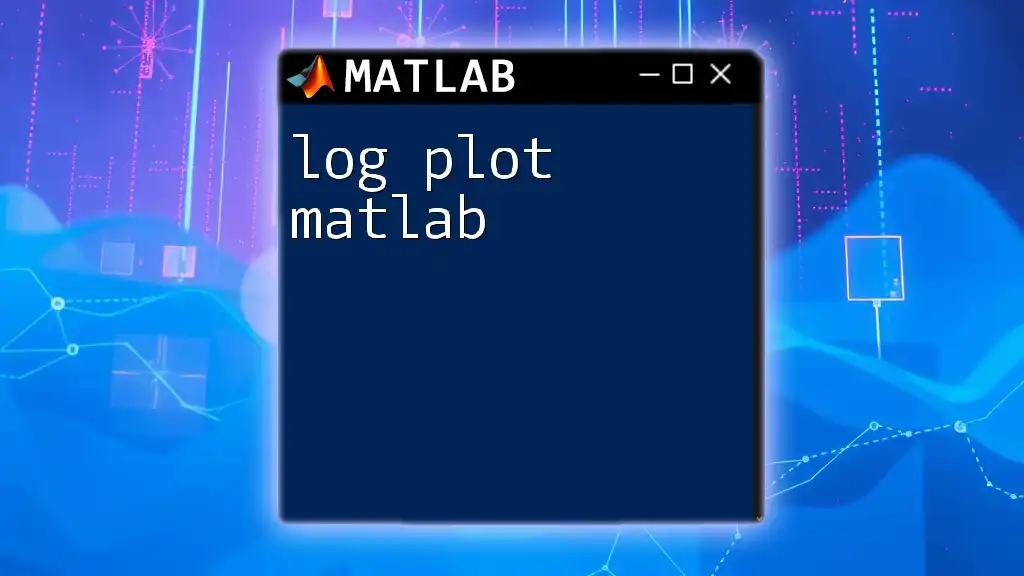
Additional Resources
To continue your learning journey, the official MATLAB documentation offers extensive coverage of commands and features. Engaging with the MATLAB community through forums and online resources can also enhance your skills and provide valuable insights into advanced techniques and best practices.