A pie plot in MATLAB is a circular statistical graphic that divides a circle into slices to illustrate numerical proportions. Here’s a simple example of how to create a pie chart using MATLAB:
data = [1, 2, 3, 4]; % Data for the pie chart
labels = {'A', 'B', 'C', 'D'}; % Labels for each slice
pie(data, labels); % Create the pie chart
title('Sample Pie Chart'); % Add a title
Introduction to Pie Plots
What is a Pie Plot?
A pie plot, also known as a pie chart, is a circular statistical graphic divided into slices to illustrate numerical proportions. Each slice of the pie represents a category's contribution to the whole, making it an effective visualization tool when you want to show relative sizes.
When to Use a Pie Plot?
Pie charts are particularly useful when displaying data that are part of a whole. This includes scenarios such as:
- Market share distribution among different companies.
- Survey results showing the proportion of responses in different categories.
- Any categorical data where you want to compare parts of a total.
Overview of MATLAB's Pie Plot Functions
In MATLAB, the primary function used for creating pie charts is the `pie` function. This function allows users to not only create basic pie charts but also to customize them extensively.
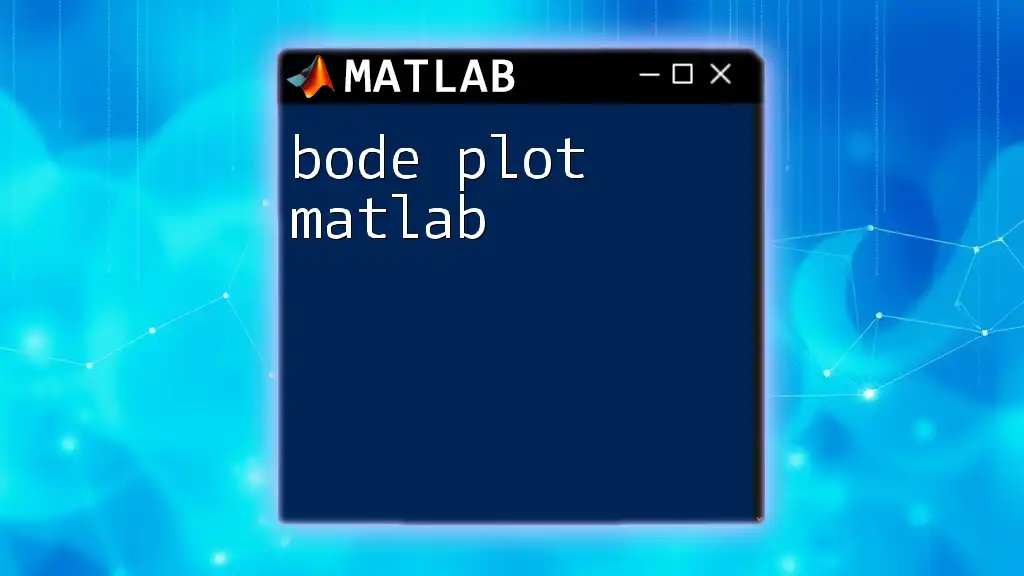
Getting Started with MATLAB
Quick Installation Guide
To begin using pie plots in MATLAB, ensure that MATLAB is installed on your system. You can obtain it from the MathWorks website, where you’ll find detailed instructions for installation and licensing.
MATLAB Interface Overview
Upon opening MATLAB, you'll encounter a user-friendly interface that includes:
- Workspace: Displays all variables in the current session.
- Command Window: Where you can enter commands directly.
- Script Editor: Ideal for writing and saving scripts for later use.
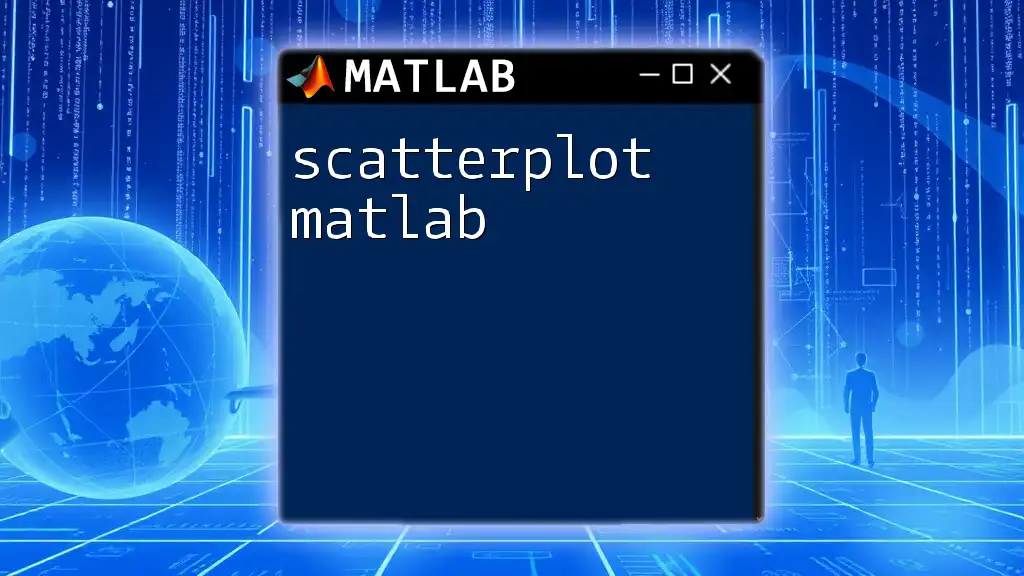
Creating a Basic Pie Plot
Syntax of the Pie Function
The basic syntax for creating a pie plot in MATLAB is straightforward:
pie(data)
The `data` parameter is a vector containing the values that you wish to visualize.
Basic Example: Creating Your First Pie Plot
Here’s how you can create your very first pie plot with MATLAB:
data = [1, 2, 3, 4];
pie(data);
When you execute this code, MATLAB generates a pie chart with four slices, each corresponding to elements in the `data` array. The sizes of the slices will be proportional to the values provided.
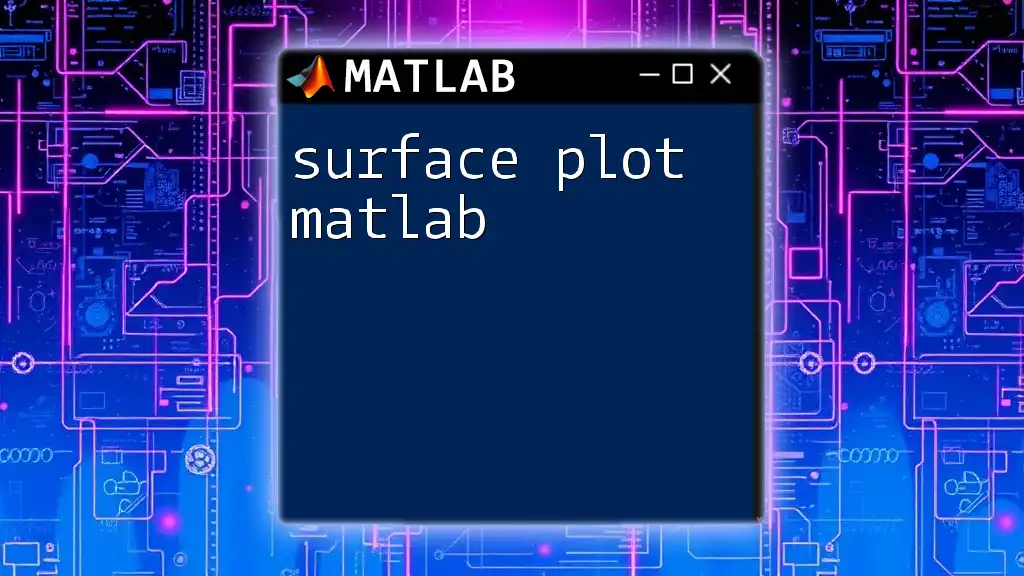
Customizing Pie Plots
Adding Labels to Pie Slices
To enhance the interpretability of your pie chart, you can add labels that indicate what each slice represents. You can achieve this by passing a cell array of strings as a second argument:
labels = {'Category A', 'Category B', 'Category C', 'Category D'};
pie(data, labels);
In this case, each label corresponds to a slice of the pie, providing immediate context to the viewer.
Changing Colors of Pie Slices
Colors can significantly impact the readability and aesthetics of your pie chart. To change the defaults, you can specify a color matrix:
colors = [0.5 0.3 0.1; 0.2 0.4 0.6; 0.1 0.5 0.8; 0.9 0.1 0.3];
pie(data, colors);
This array defines the RGB values for each slice, allowing for a customized appearance.
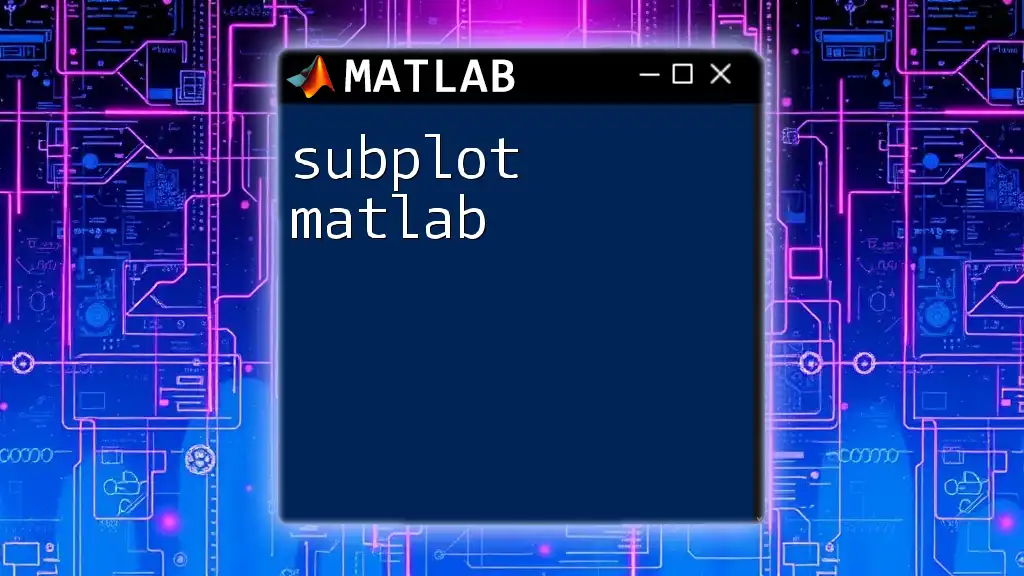
Advanced Customizations
Exploding Slices for Emphasis
Sometimes highlighting a particular slice can draw attention to significant data. This can be achieved using the explode parameter:
explode = [1, 0, 0, 0]; % Only explode the first slice
pie(data, explode, labels);
In this example, the first slice will be 'exploded' or pulled out from the rest of the pie chart, making it stand out.
Adding Legends to Your Pie Chart
Including legends can enhance understanding further. You can easily add a legend corresponding to your labels:
legend(labels);
With this addition, viewers can relate the slices to their respective categories more intuitively.
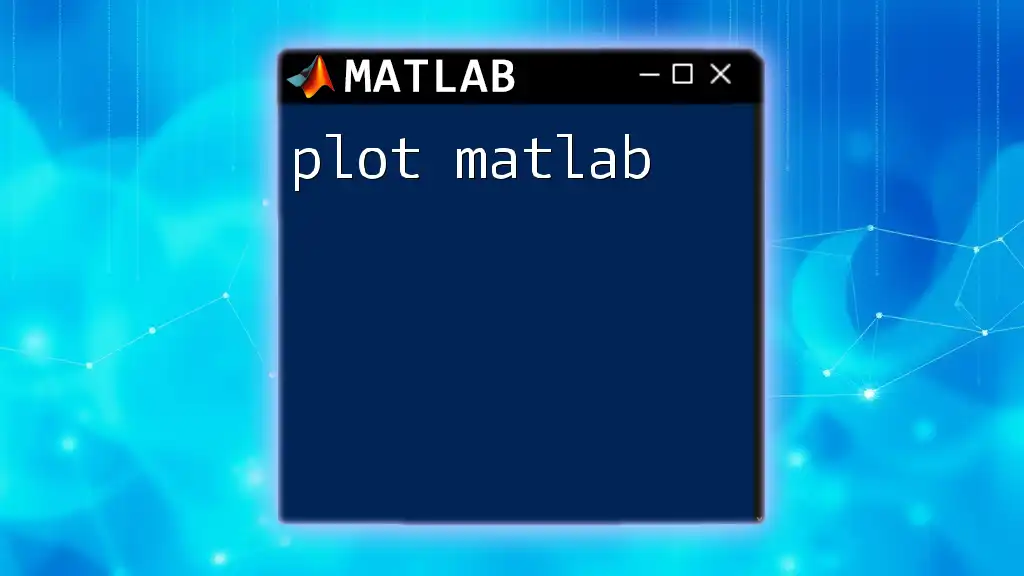
Troubleshooting Common Issues
Common Errors in Creating Pie Plots
Despite its simplicity, you may encounter errors if the input vector's format is incorrect. Always ensure that your data is a numeric vector. If you receive an error, check the type and dimensions of your `data`.
Performance Tips for Large Data Sets
When plotting larger datasets, pie charts can become cluttered and less effective. In such cases, consider using a bar chart or other visualizations better suited to handle vast amounts of data. If you stick with pie charts for smaller segments, group small slices into an "Other" category to maintain clarity.
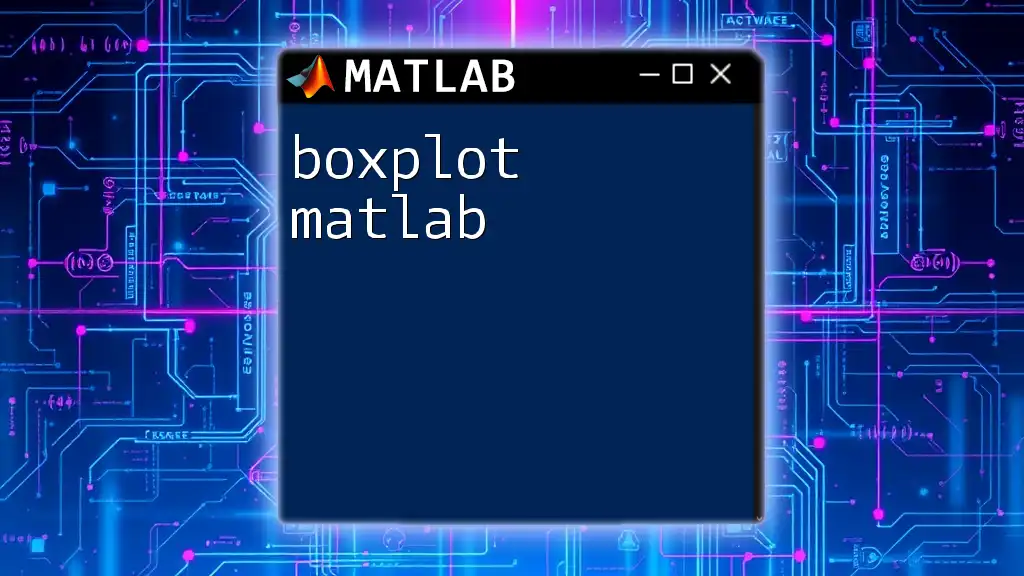
Enhancing Your Pie Plots with MATLAB Features
Using 3D Pie Charts
For those looking for a more visually dynamic representation, MATLAB supports 3D pie charts, although less commonly used than their 2D counterparts. The potential drawbacks include complex interpretations and increased difficulty in accurately gauging proportions. Still, they can be created with the `pie3` function:
data = [1, 1, 1, 1];
pie3(data);
This creates a three-dimensional version of a pie chart, offering a unique perspective.
Exporting Pie Plots
Once you've finalized your pie chart, exporting it is an essential step for sharing or publication. You can save your pie chart to various file formats using:
saveas(gcf, 'myPieChart.png');
This command saves the current figure as a PNG image, which can then be included in reports or presentations.
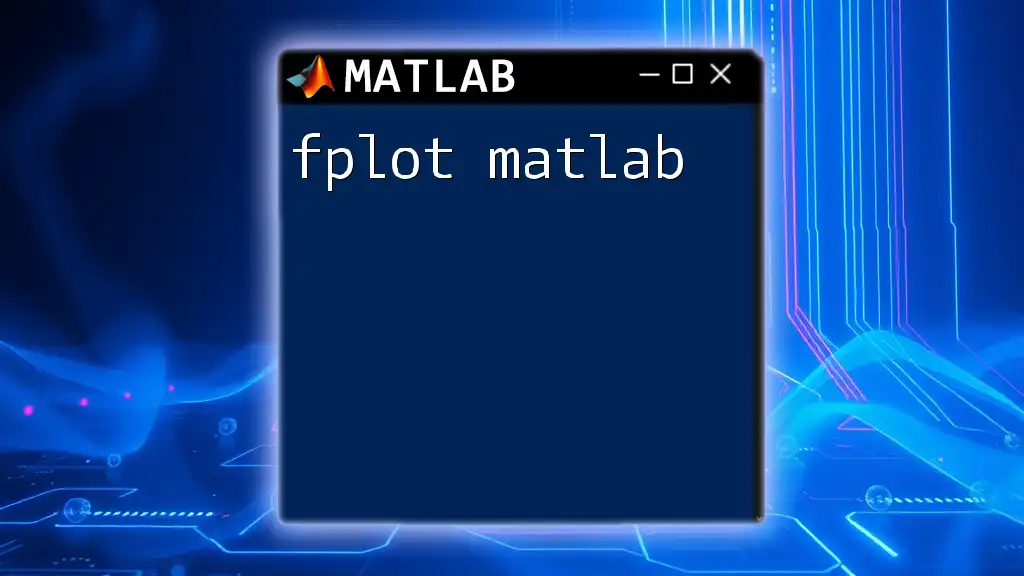
Real-World Applications of Pie Plots
Case Studies and Examples
Pie charts are prevalent in various fields. For instance, businesses often use them to illustrate market share distributions during presentations, effectively conveying data to stakeholders. In educational environments, pie charts can summarize student survey results on topics such as satisfaction or preferred learning methods. Health professionals might visualize patient demographics to identify areas for improvement or focus.
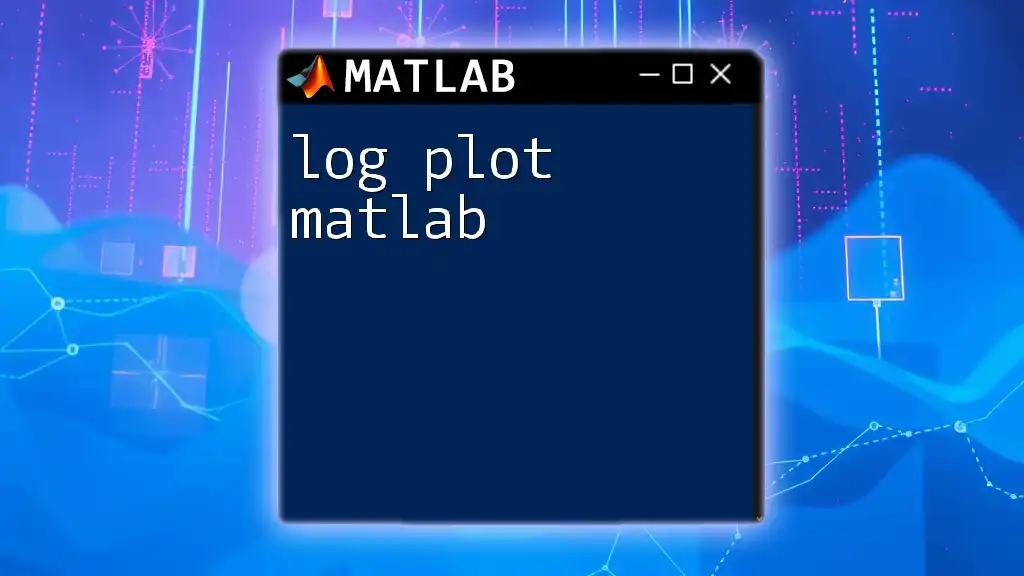
Conclusion
In this guide, we’ve explored the essentials of creating pie plots in MATLAB. Starting from the fundamental steps to advanced customizations, including exploding slices and legends, the tools provided by MATLAB make it easy to visualize categorical data effectively. Remember to experiment with different datasets and customizations to make your pie charts not only informative but also appealing.
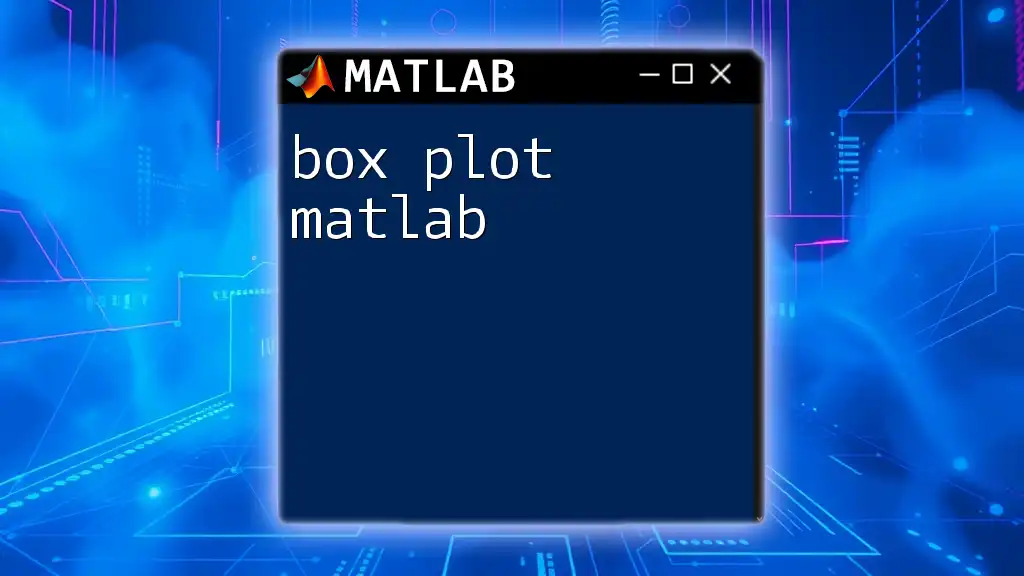
Additional Resources
For further exploration, refer to the [MATLAB documentation](https://www.mathworks.com/help/matlab/) for detailed insights into the `pie` function and its options. Engaging with MATLAB user communities can also yield valuable tips and collaborative learning opportunities.