A Bode plot in MATLAB is a graphical representation of a linear system's frequency response, showing both magnitude and phase across a range of frequencies.
% Example code to create a Bode plot for a transfer function
num = [1]; % Numerator coefficients
den = [1, 2, 1]; % Denominator coefficients
sys = tf(num, den); % Create transfer function
bode(sys); % Generate the Bode plot
grid on; % Add grid for better visualization
Understanding Bode Plots
What is a Bode Plot?
A Bode Plot is a graphical representation of a linear, time-invariant system's frequency response. It consists of two plots: one for magnitude and another for phase. The magnitude plot shows how much the output of a system changes relative to the input across various frequencies. The phase plot illustrates how the phase of the output changes with frequency.
The Significance of Bode Plots
Bode plots are essential tools in control systems and signal processing. They allow engineers to evaluate the stability and performance of systems. By examining the magnitude plot, you can determine how gains vary with frequency. Meanwhile, the phase plot helps assess phase shifts, crucial for understanding the behavior of feedback systems. The ability to anticipate system behavior via Bode plots allows for better design and modifications of systems in various engineering fields.
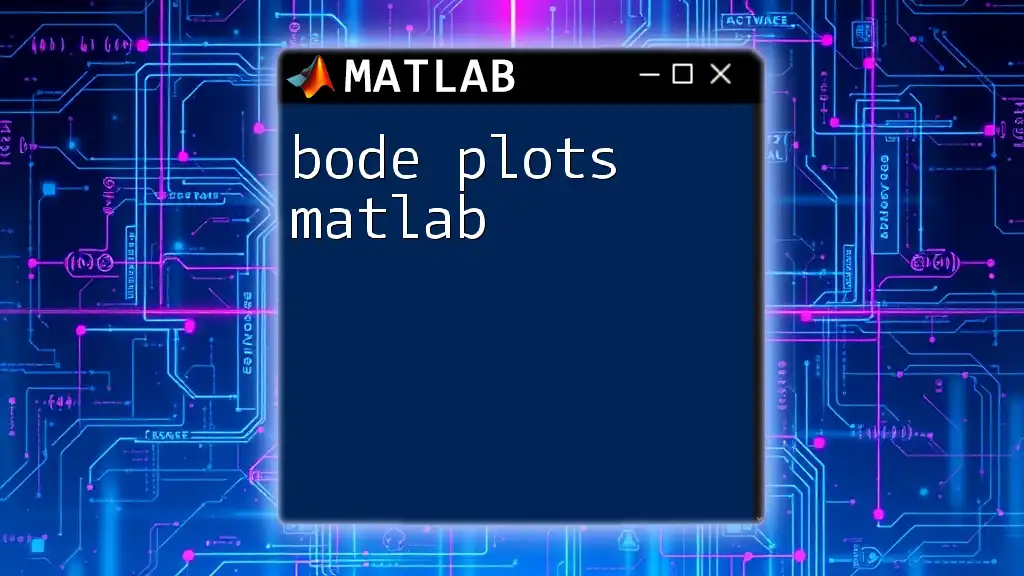
Getting Started with MATLAB
Setting Up the Environment
Before creating Bode plots in MATLAB, ensure that you have MATLAB installed along with the necessary toolboxes, particularly the Control System Toolbox. Familiarizing yourself with the MATLAB interface is invaluable; key components include the Command Window, Workspace, and Editor for running scripts.
Loading Necessary Functions
The primary functions for creating Bode plots in MATLAB revolve around system representations such as transfer functions, state-space, and zero-pole-gain models. Knowing how to define these systems is the first step to effectively utilizing Bode plot functions.
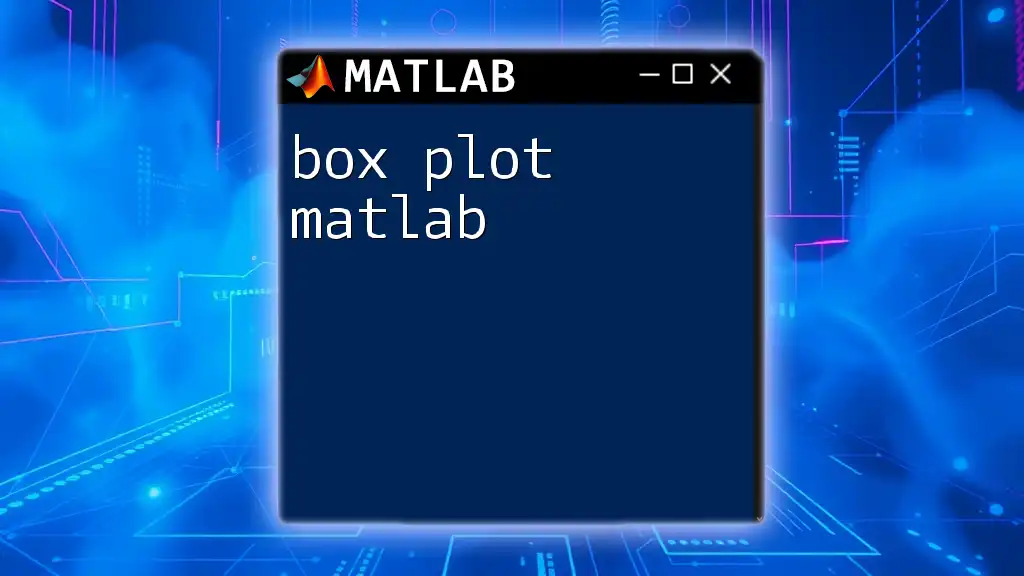
Creating Bode Plots in MATLAB
Using Transfer Functions
Syntax Explanation
To create a Bode plot in MATLAB, the most straightforward syntax involves using the `bode` function. For example:
bode(tf)
Here, `tf` is the transfer function of your system.
Example: Simple Transfer Function
Let’s consider a simple first-order transfer function:
s = tf('s'); % Define the variable s
H = 1 / (s + 1); % Define the transfer function
bode(H); % Create Bode Plot
grid on; % Enable grid for better visualization
In this example, we define `s` as a complex variable and create a first-order system described by the transfer function \(H(s) = \frac{1}{s + 1}\). The `bode` command generates the plot, while `grid on` enhances visibility by adding a grid to the graph.
Using State-Space and Zero-Pole-Gain Models
State-Space Representation
An alternative method for plotting Bode plots is using state-space models. To do this, you first need to define the matrices corresponding to your state-space system. The syntax is as follows:
bode(ss(A,B,C,D))
Example: State-Space Bode Plot
You can create a Bode plot from a state-space representation by defining the system matrices \(A\), \(B\), \(C\), and \(D\):
A = [0 1; -1 -1];
B = [0; 1];
C = [1 0];
D = 0;
sys = ss(A,B,C,D);
bode(sys);
This example will generate a Bode plot for a second-order system defined by the given state-space representation.
Zero-Pole-Gain Representation
You might prefer to use the zero-pole-gain (ZPK) representation for certain systems. The syntax is:
bode(zpk(z,p,k))
Where `z` denotes zeros, `p` denotes poles, and `k` denotes the system gain.
Example: ZPK Bode Plot
Here’s how to create a Bode plot using a zero-pole-gain model:
z = [-1]; % Define zero
p = [-2 -3]; % Define poles
k = 1; % Define gain
H = zpk(z,p,k);
bode(H);
This example will show how ZPK models can also effectively visualize frequency response.
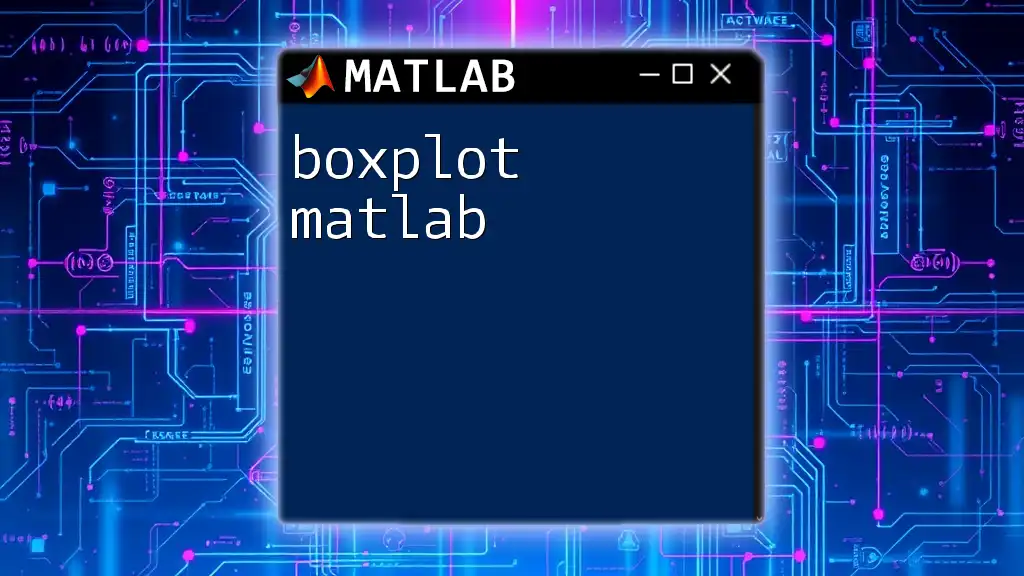
Customizing Bode Plots
Plotting Multiple Systems
One of the strengths of Bode plots is their ability to allow the comparison of multiple systems. To overlay Bode plots, use:
bode(H1, H2);
hold on;
This example compares two different systems \(H1\) and \(H2\).
Customizing Axes and Styles
Adjusting Frequency Range
You can restrict the frequency range shown in the Bode plot by modifying the command:
bode(H, {0.1, 100});
This command restricts the frequency display from 0.1 to 100 rad/s.
Changing Line Styles and Colors
To enhance the appearance of your plots with specific colors or styles, you can utilize the `bodeplot` function:
bodeplot(H, 'r--');
This line specifies that the Bode plot should be red and dashed.
Adding Titles and Labels
For clarity, adding titles and labels is vital when interpreting Bode plots:
title('Bode Plot of a First-Order System');
xlabel('Frequency (rad/s)');
ylabel('Magnitude (dB)');
Effective labeling helps convey the necessary information to the viewer.
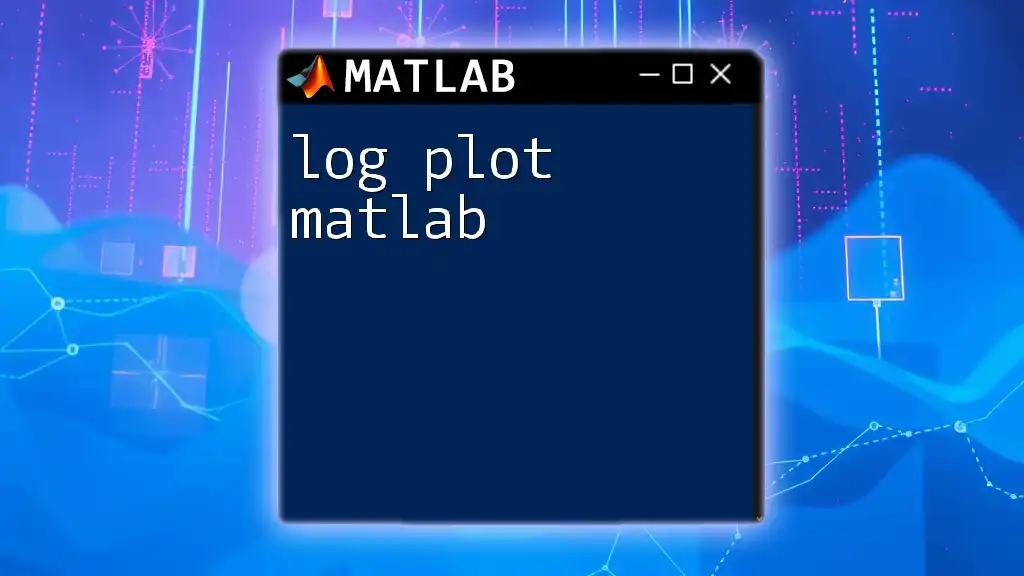
Analyzing Bode Plots
Interpreting Magnitude and Phase
Interpreting Bode plots involves recognizing key features. The magnitude plot is usually in decibels (dB), while the phase plot is in degrees. The cutoff frequency, where output power drops 3 dB from its maximum, indicates a critical point for system response.
Using Bode Plot Data
You can extract data from Bode plots for additional analysis, allowing you to utilize it in further calculations:
[mag, phase, w] = bode(H);
This command retrieves the magnitude `mag`, phase `phase`, and frequency vector `w`, enabling further manipulation.
Stability Criteria from Bode Plots
One of the most significant applications of Bode plots is in stability analysis. Gain and phase margins can be extracted from these plots, offering insights into system stability. A positive gain margin and a phase margin greater than 0° indicate system stability.
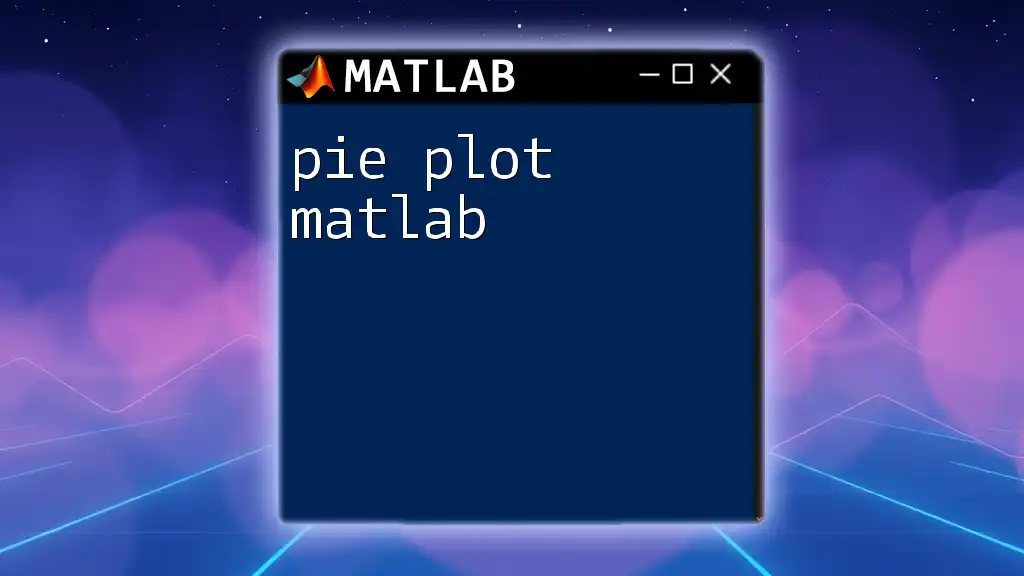
Practical Applications of Bode Plots
Control System Design
Bode plots are integral to control system design, allowing engineers to tune systems for desired performance metrics, such as speed and stability.
Filter Design and Analysis
Bode plots facilitate the design of filters by visualizing how different frequencies are attenuated or amplified, critical in communications and signal processing fields.
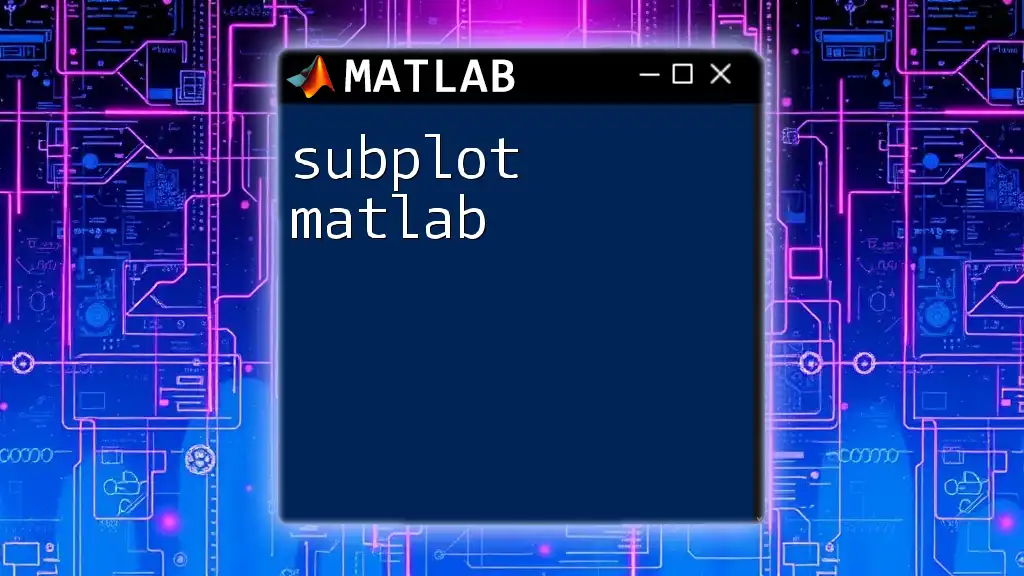
Conclusion
Bode plots in MATLAB are powerful tools for analyzing and designing control systems. By utilizing transfer functions, state-space, and zero-pole-gain representations, you can effectively construct these plots and extract valuable system insights. With practice, you can harness the full capabilities of Bode plots to support your engineering projects and enhance your understanding of system dynamics. Exploring further into MATLAB's control system functionalities will deepen your skills and application in various real-world scenarios.