The `rng` command in MATLAB is used to control the random number generator, allowing you to set a specific seed for reproducibility in random number generation.
rng(123); % Set the random seed to 123 for reproducibility
Understanding the `rng` Function
What is `rng`?
The `rng` function in MATLAB plays a crucial role in controlling the pseudorandom number generator. This function allows users to set the seed for random number generation, which is essential for maintaining reproducibility in simulations and stochastic processes. When the seed is set to a specific value, it guarantees that the sequence of random numbers generated will be the same each time you run the code, making it easier to debug and verify results.
Syntax of `rng`
The basic syntax of the `rng` function is structured as follows:
rng(seed, generatorType)
Parameters Explained
-
seed: The seed value initializes the random number generator's sequence. Different seed values will produce different sequences of random numbers. For example, using `rng(1)` will yield a different sequence than `rng(2)`.
-
generatorType: This parameter allows you to select different algorithms for random number generation. Some common options include:
- 'twister': This is the default generator in MATLAB, known for its high performance and large period.
- 'philox': Designed for better parallel computing with SIMD instructions.
- 'mt19937ar': Another variant of the Mersenne Twister algorithm.
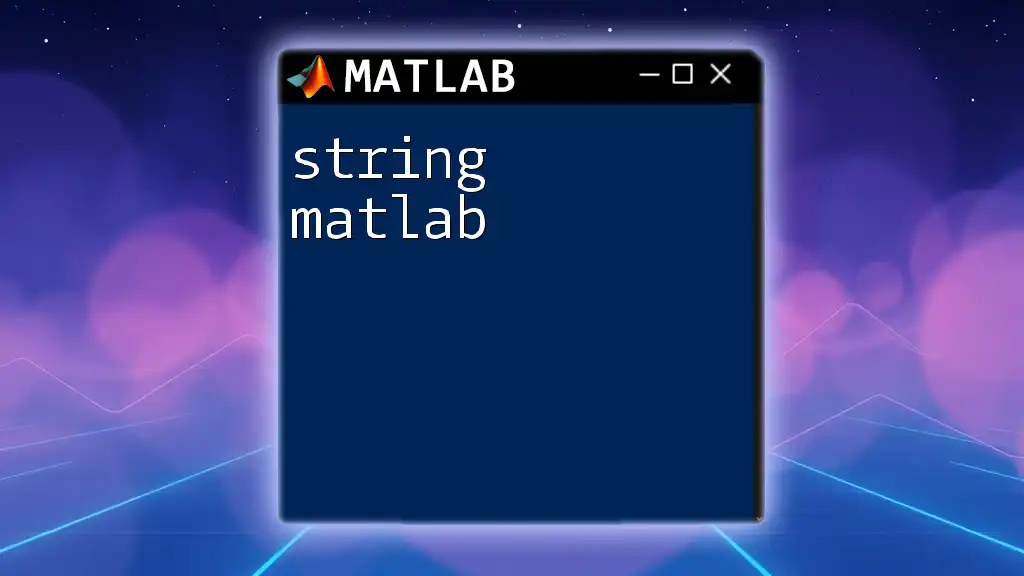
Setting Up Your Random Number Generator
Setting the Seed
Setting the seed is a fundamental step in random number generation when using `rng`. By doing so, you can recreate your random number sequences.
For example, you can set the seed as follows:
rng(1); % Sets the seed to 1
randomNumbers = rand(1, 5); % Generates a 1x5 array of random numbers
Every time you run this code, you'll get the same sequence of random numbers when the seed is set to 1.
Choosing a Generator Type
Choosing the right generator type can impact performance and the statistical properties of the random numbers generated. You might want to experiment with different types, such as:
rng(1, 'twister'); % Using the default generator
rng(2, 'philox'); % Switching to the Philox generator
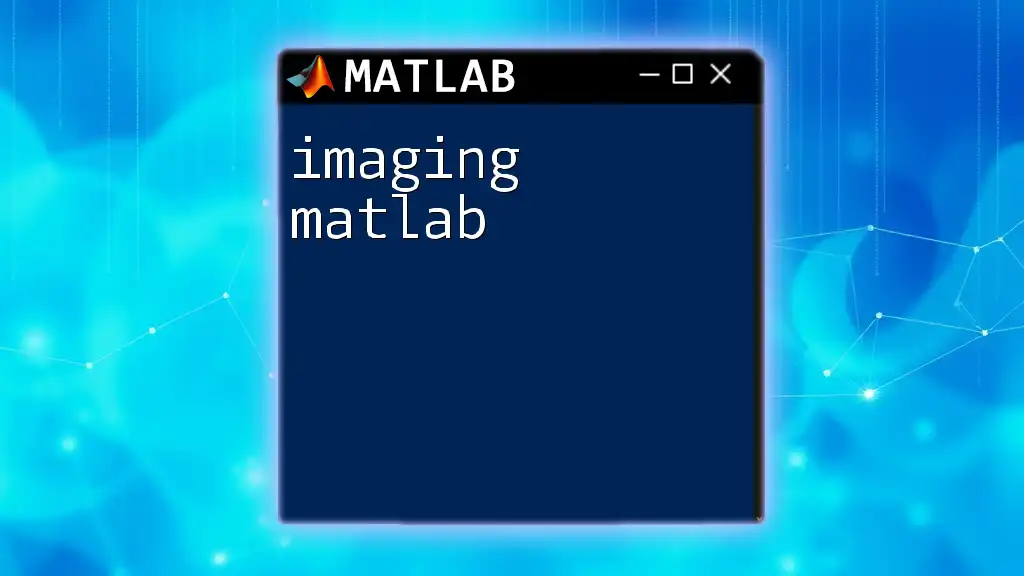
Using `rng` for Reproducibility
Importance of Reproducibility
In research and application development, consistent results are paramount. The `rng` function ensures that each time you run your simulations with the same seed, you produce the same outcomes. This aspect is particularly crucial when you're sharing your findings or working collaboratively.
Example of Reproducibility
To demonstrate the importance of `rng`, consider the following example, which illustrates how using the same seed can yield identical results:
rng(5); % Sets the seed
data1 = rand(1, 10);
rng(5); % Setting the same seed again
data2 = rand(1, 10);
disp(all(data1 == data2)); % Should display true
In this example, `data1` and `data2` will contain the same random numbers because the seed has been set consistently.
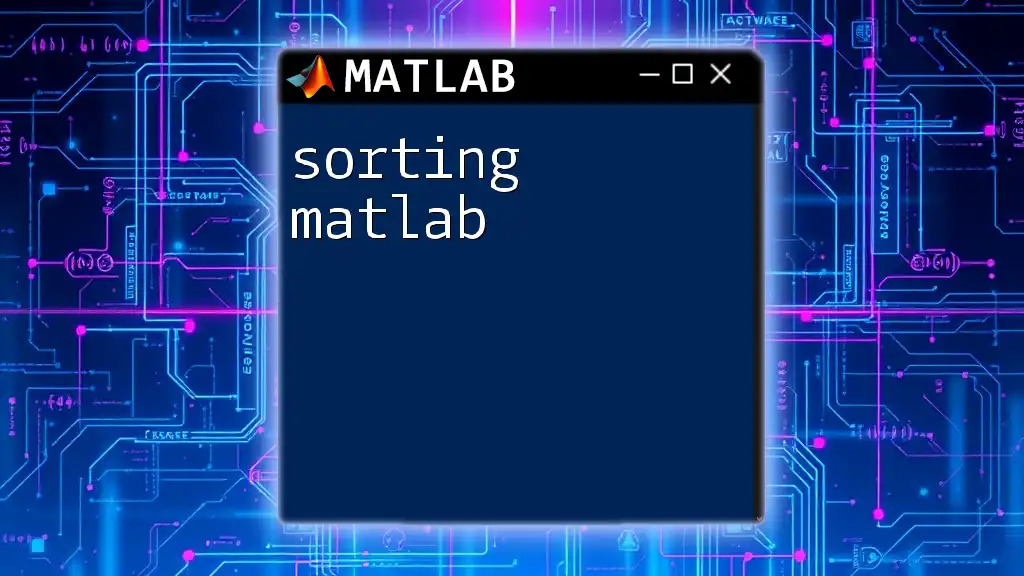
Common Use Cases for `rng`
Simulations
In the realm of simulations, especially in Monte Carlo methods, `rng` is indispensable. For instance, if you want to simulate a million random variables, you can set it up as follows:
rng(42); % Setting a specific seed
N = 1e6; % Number of simulations
results = rand(1, N); % Simulates N random variables
With this setup, every time you run your simulation with the seed of 42, the generated random numbers remain the same, ensuring consistency in your results.
Statistical Sampling
Another common use case is statistical sampling. When running analyses that require drawing random samples from a dataset, reproducing the same samples can be necessary for validation purposes.
For example, consider this code snippet for random sampling:
data = [1, 2, 3, 4, 5, 6];
rng(3); % Ensure reproducibility
sampledData = datasample(data, 3); % Randomly sample 3 elements
By setting the seed to 3, the same elements will be selected from `data`, providing reliable and reproducible outcomes.
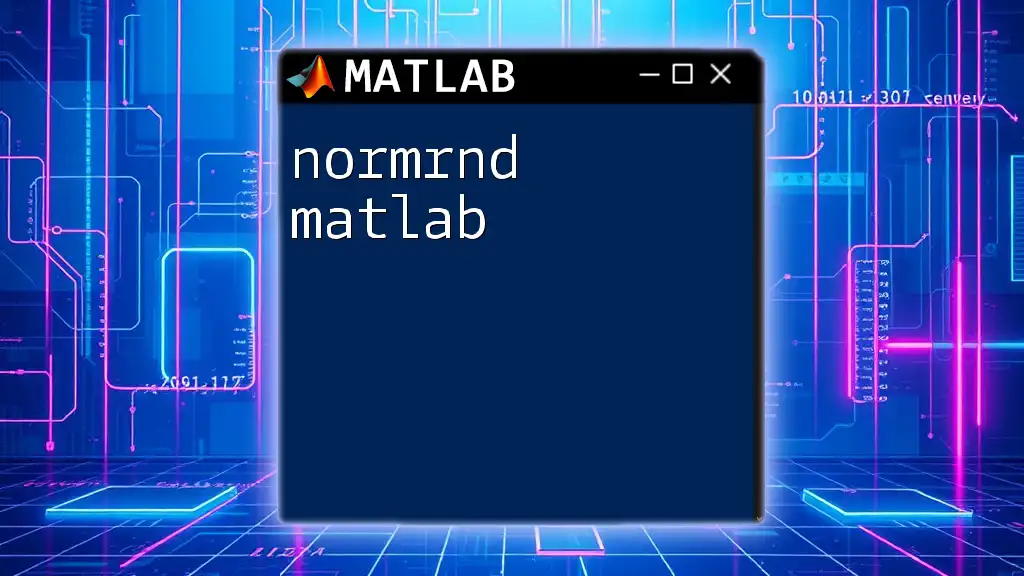
Exploring Variations with `rng`
Re-initializing the Random Generator
At times, you may wish to return to MATLAB's default state for random number generation. The following command reinitializes the generator:
rng('default'); % Resets to default settings
This can be useful in scenarios where you want to start fresh, especially when you're experimenting with different seeds and generator types.
Advanced Techniques
Utilizing Different Algorithms
Exploring various algorithms can yield different random characteristics tailored to specific applications. For example, by implementing the `philox` generator, you might enhance performance in parallel computations:
rng(1, 'philox'); % Using the Philox algorithm for random numbers
Combining `rng` with Other Functions
The `rng` function can be effectively combined with MATLAB's built-in functions to yield various random variables from different distributions. For instance, generating random numbers from a normal distribution could look like this:
rng(1); % Setting the seed
randomValues = normrnd(0, 1, [100, 1]); % Generating random numbers from a normal distribution
Here, `normrnd` generates 100 random numbers from the standard normal distribution, and the reproducibility of results is preserved by the seed setting.
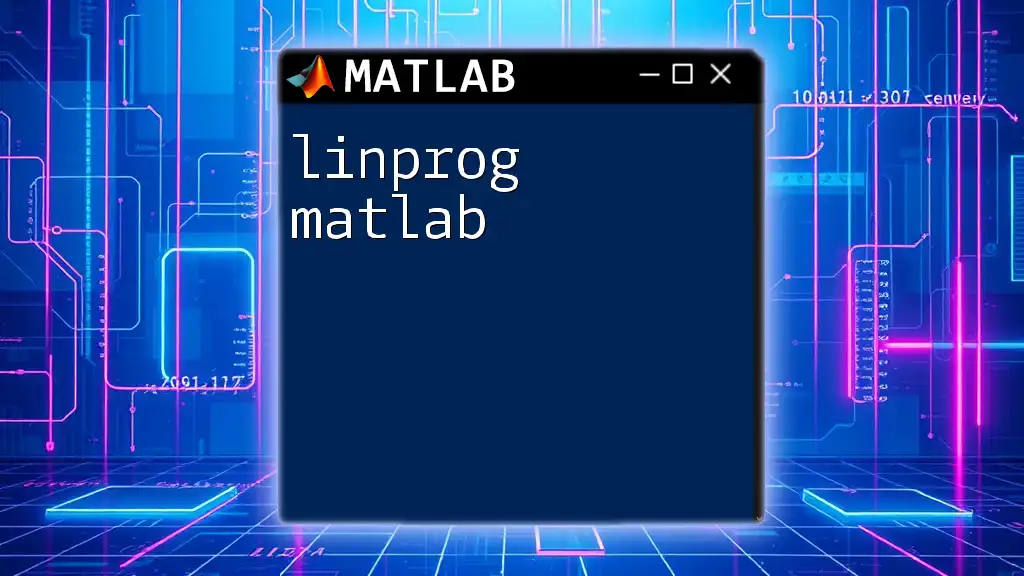
Conclusion
The `rng` function in MATLAB is an essential tool for managing the random number generation process. By utilizing `rng`, you can create reproducible results that are essential for simulations, statistical analysis, and scientific research. Remember to experiment with different seeds and generator types to fully harness the capabilities of this powerful function.
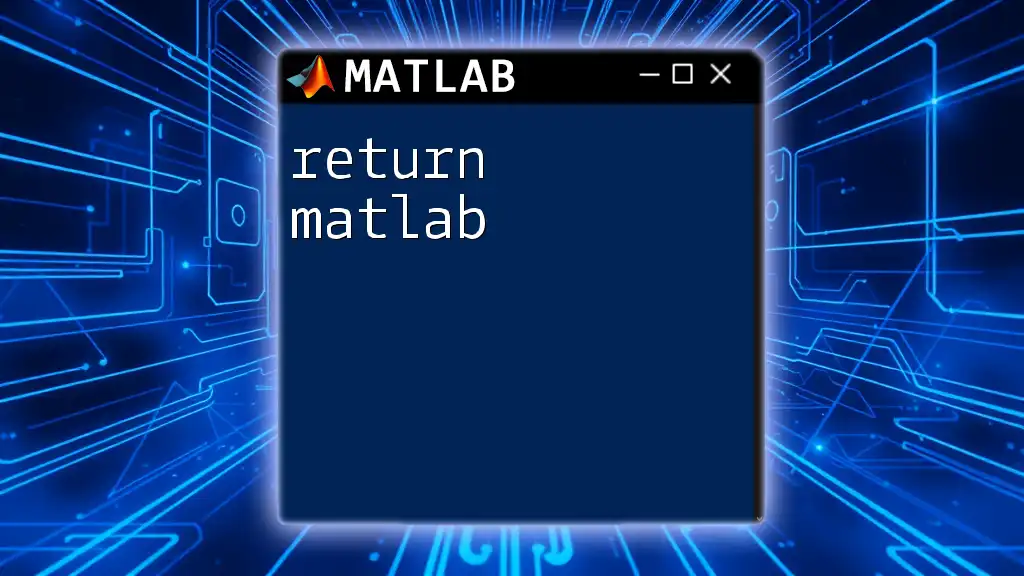
Frequently Asked Questions (FAQs)
What happens if I don’t set a seed before generating random numbers?
If you don't set a seed, MATLAB will automatically choose one based on the current time, leading to different results each time you run your code.
How can I restore the random state to my desired configuration?
You can achieve this by calling `rng()` with your desired seed or by using `rng('default')` to reset to the default configuration.
Can you explain the differences between the various generators?
Different generators have varying statistical properties and performance characteristics. The choice depends on the specific requirements of your application, such as speed or randomness quality.
Utilizing these practices will ensure you effectively leverage the `rng` function in your MATLAB projects, driving consistency and reliability in your results.