The `ttest2` function in MATLAB performs a two-sample t-test to determine if the means of two independent groups are significantly different from each other.
Here’s a code snippet to illustrate its usage:
% Sample data for two groups
group1 = [23, 21, 18, 25, 22];
group2 = [30, 29, 31, 34, 32];
% Perform a two-sample t-test
[h, p] = ttest2(group1, group2);
% Display the results
fprintf('Hypothesis test result (h): %d\n', h);
fprintf('p-value: %.4f\n', p);
Understanding `ttest2`
What is `ttest2`?
The `ttest2` function in MATLAB is a powerful tool for conducting a two-sample t-test. This statistical method compares the means of two independent groups to determine whether there is evidence to reject the null hypothesis, which states that the means of two populations are equal.
When to Use `ttest2`
Using `ttest2` is appropriate in several scenarios:
- Independent Samples: When you have two separate groups and want to compare their means.
- Assumption Checks: Before conducting a t-test, ensure that:
- The samples are independent of each other.
- The data follows a normal distribution.
- The variances of the two groups are either equal or unequal.
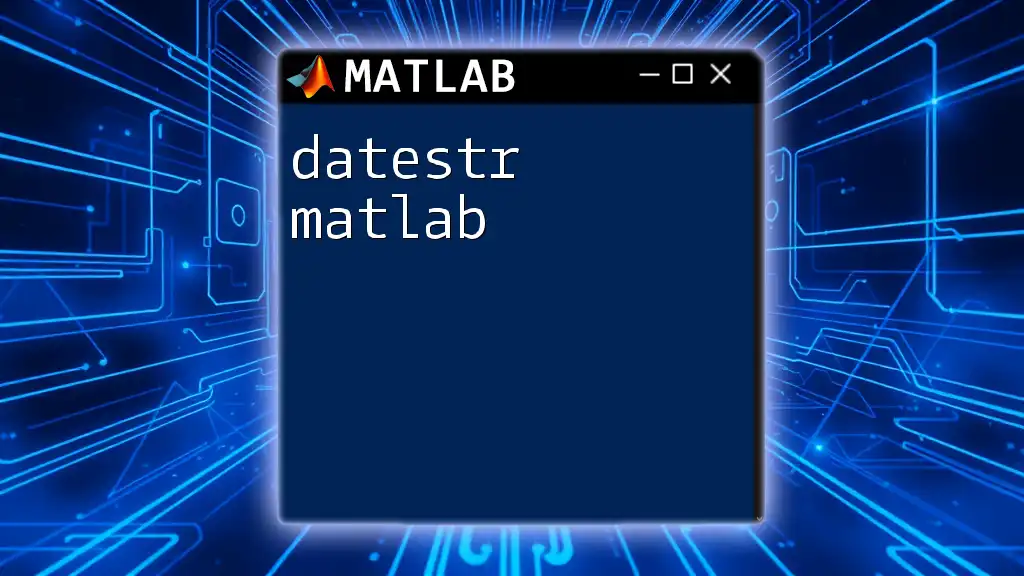
Syntax and Usage of `ttest2`
Basic Syntax
The basic syntax for `ttest2` is straightforward:
[h, p] = ttest2(x, y)
Here, `x` and `y` are the two data sets, and the function will return:
- h: A hypothesis test result (0 or 1).
- p: The p-value associated with the test.
Parameters and Options
Input Arguments
In `ttest2`, both `x` and `y` can be vectors or matrices. It's essential to ensure that the lengths of the input arguments are appropriate, especially if you're conducting tests for samples of different sizes.
If either data set contains missing values, `ttest2` will automatically ignore those values, ensuring that the test remains valid.
Additional Name-Value Pair Arguments
`ttest2` allows for several optional arguments to customize your testing approach. Two common parameters include:
- 'Alpha': Set your significance level (default is 0.05).
- 'Tail': Determines whether your test is one-tailed or two-tailed (default is 'both').
- 'Vartype': Choose between 'equal' or 'unequal' variances.
Example of customizing parameters:
[h, p] = ttest2(x, y, 'Alpha', 0.05, 'Tail', 'both', 'Vartype', 'unequal');
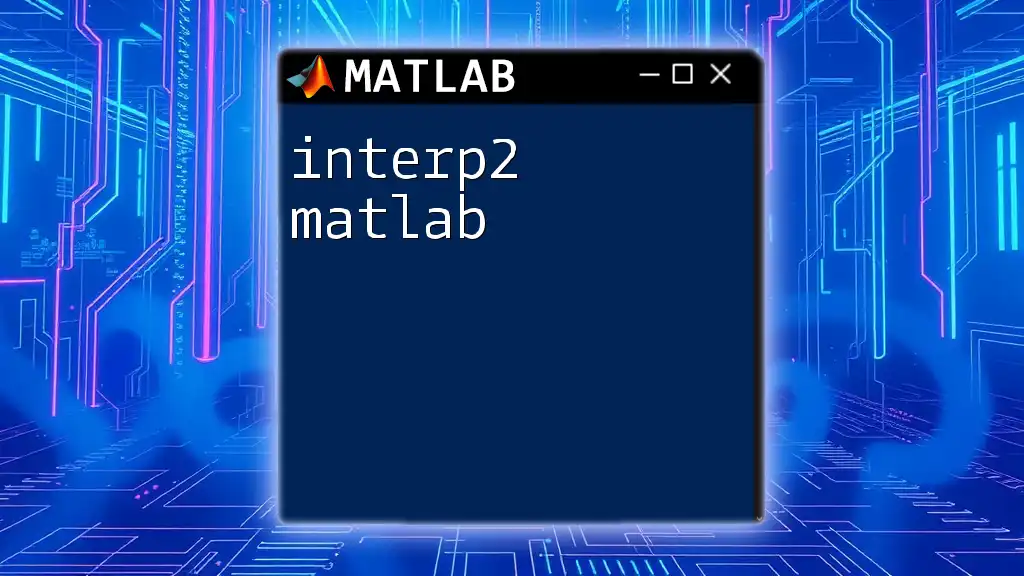
Practical Examples of Using `ttest2`
Example 1: Basic Two-Sample t-Test
Problem Statement: Suppose you are studying the effects of two different training programs on weight loss. You collect data from two independent groups.
Sample Data Creation:
group1 = randn(50, 1) + 1; % Group undergoing Training A
group2 = randn(50, 1); % Group undergoing Training B
Executing `ttest2`:
[h, p] = ttest2(group1, group2);
Interpreting Results: A result of `h = 1` indicates that you reject the null hypothesis, suggesting a significant difference in the means of the two groups, while a `h = 0` means no significant difference was found. The value of `p` indicates the strength of evidence against the null hypothesis.
Example 2: One-Tailed t-Test
Introduction to One-Tailed Testing: When you have a specific direction in mind (e.g., Group A's mean is greater than Group B's), you can conduct a one-tailed test.
Adjusting the Syntax:
[h, p] = ttest2(group1, group2, 'Tail', 'right');
Conclusion from Results: If `h = 1`, this confirms that Group A has a significantly higher mean than Group B based on the specified level of significance.
Example 3: Handling Unequal Variances
Explanation of Variance Assumptions: It’s crucial to check if your data sets have equal variances. In cases where they do not, you can adjust the `ttest2` function accordingly.
Example with Real-World Data:
group3 = randn(50, 1) + 5; % Group with a higher mean
group4 = randn(50, 1); % Group with a lower mean
Running the t-Test with Unequal Variances:
[h, p] = ttest2(group3, group4, 'Vartype', 'unequal');
By specifying `Vartype`, MATLAB acknowledges the unequal variances when calculating the test statistics.
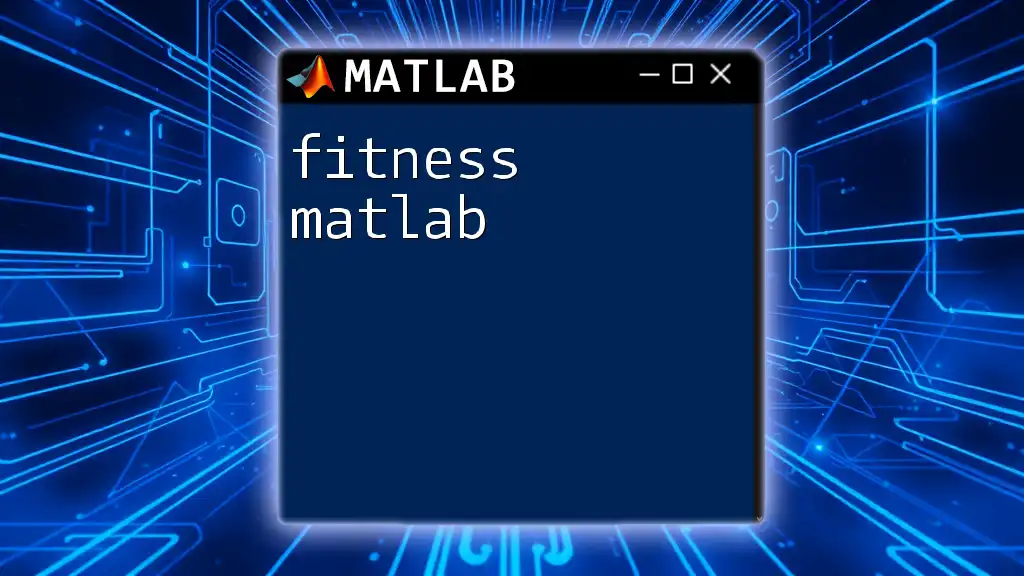
Interpreting the Results
Understanding p-values
The p-value derived from `ttest2` indicates the probability of observing the data, or something more extreme, given that the null hypothesis is true. A common threshold is `p < 0.05`, which provides significant evidence against the null hypothesis. Conversely, a p-value greater than this threshold suggests insufficient evidence to reject the null hypothesis.
Reporting Results
When reporting findings from `ttest2`, be meticulous. Include both the test statistic and the p-value. Also, consider presenting confidence intervals for better interpretation of the mean differences. The MATLAB code for calculating confidence intervals might look like this:
ci = tinv([0.025 0.975], df) * std_err + mean;
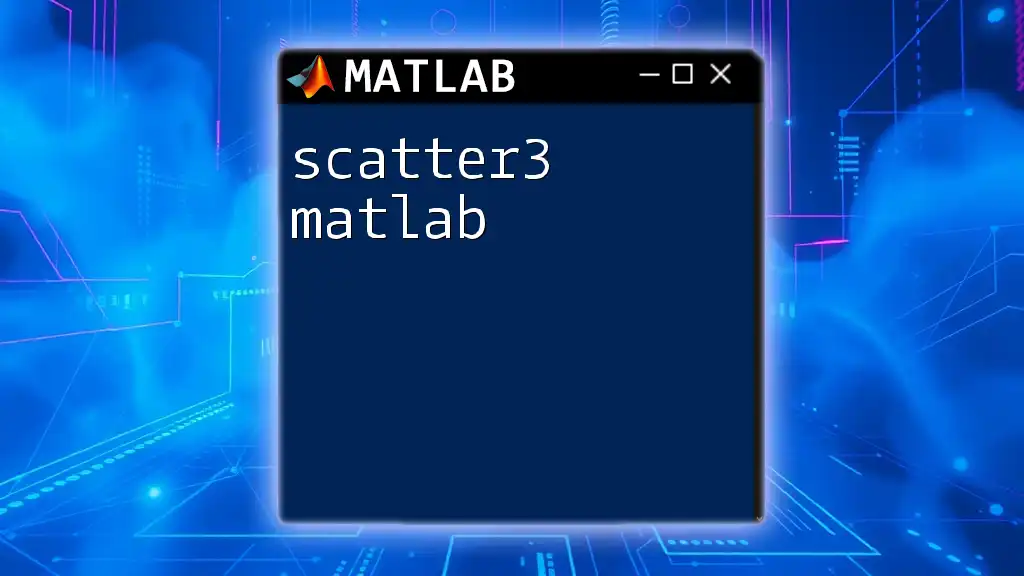
Common Pitfalls and Troubleshooting
Checking Assumptions
Before performing the t-test, check the following assumptions:
- Normality: Use the Shapiro-Wilk test or Q-Q plots to assess normality.
- Homogeneity of Variances: Levene’s test can help verify if the variances between groups are equal.
Debugging Common Errors
MATLAB might return various error messages when using `ttest2`. Common issues include mismatched dimensions or incorrect data types. Always validate your inputs, ensuring that both `x` and `y` are appropriately formatted and contain relevant data.
Identifying when to consult more advanced statistical methods may also prevent potential misunderstandings.
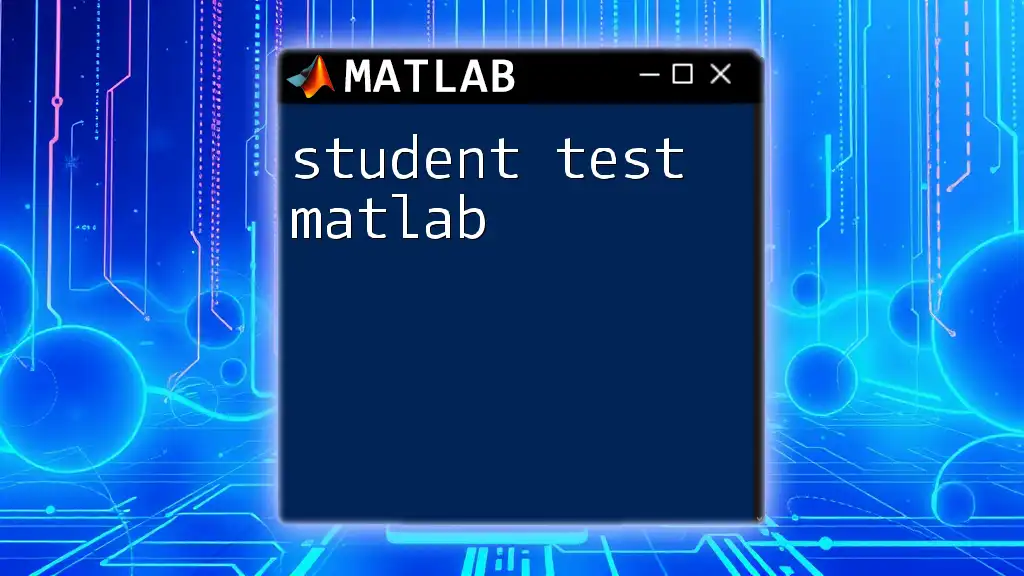
Conclusion
The `ttest2` function in MATLAB provides a robust mechanism for comparing the means of two independent samples. By understanding its syntax, application scenarios, and result interpretation, users can effectively leverage this function to make informed statistical conclusions. Continued exploration and practice with MATLAB statistics will enhance your analytical skills and broaden your data analysis capabilities.