The `syms` command in MATLAB is used to create symbolic variables for use in symbolic computations such as algebraic manipulations and equation solving.
syms x y
f = x^2 + y^2;
Understanding Symbolic Variables in MATLAB
What are Symbolic Variables?
Symbolic variables in MATLAB allow users to perform calculations symbolically rather than numerically. This means that, instead of computing specific numerical results, symbolic computations keep the mathematical expressions intact and manipulate them algebraically. Symbolic variables are particularly useful in fields such as mathematics, physics, and engineering, where algebraic manipulation is needed.
Benefits of Using Symbolic Computation
The advantages of using symbolic computation include the ability to:
- Perform algebraic simplifications, differentiation, and integration with high precision.
- Solve equations symbolically, allowing for exact solutions rather than approximate numerical ones.
- Model complex mathematical problems that are not readily solvable with numerical methods.
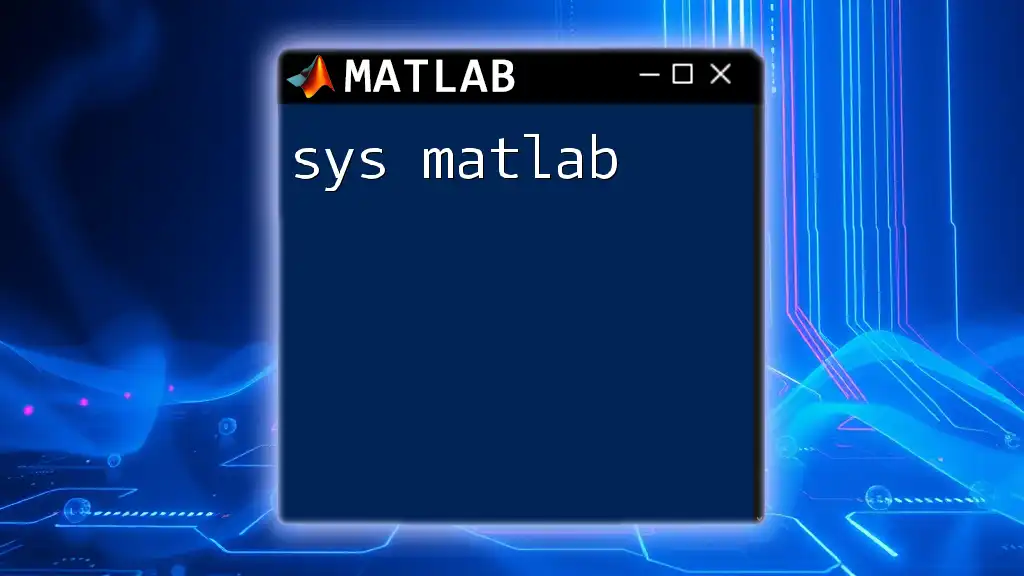
Getting Started with `syms`
Syntax of the `syms` Command
The `syms` command is essential for defining symbolic variables in MATLAB. The basic syntax is straightforward:
syms x
Here, `x` becomes a symbolic variable. You can also create multiple symbolic variables in a single command:
syms x y z
This command initializes `x`, `y`, and `z` as symbolic variables, allowing you to use them in various mathematical expressions.
Creating Different Types of Symbolic Variables
Single Symbolic Variable
To define a single symbolic variable, simply use:
syms x
Now, `x` can be used in computations, such as forming equations or expressions that require symbolic manipulation.
Multiple Symbolic Variables
Creating multiple symbolic variables simultaneously can streamline your code. For example:
syms x y z
This initializes `x`, `y`, and `z` in one line, enabling you to work with all three variables at once.
Symbolic Arrays
You can create symbolic arrays, which are particularly useful when dealing with systems of equations or matrices. For instance:
syms x [1 3] % Creates a 1x3 symbolic array
This code generates a one-dimensional symbolic array containing three symbolic variables designated as `x(1)`, `x(2)`, and `x(3)`.
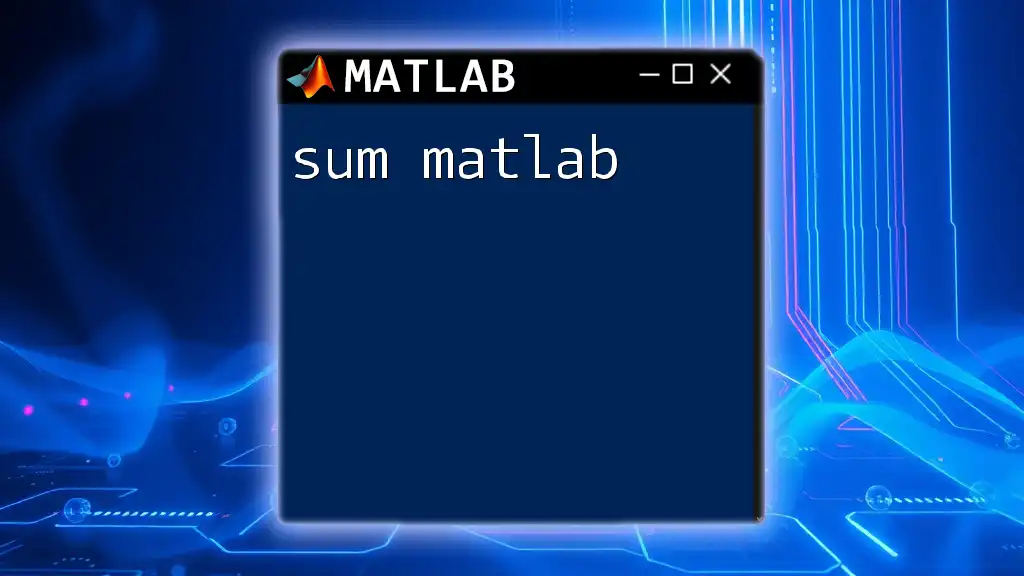
Operations with Symbolic Variables
Basic Arithmetic Operations
Symbolic variables can undergo standard arithmetic operations just like numerical variables. Consider the following expression:
syms x y
z = x + y;
Here, `z` is the symbolic representation of the sum of `x` and `y`.
Algebraic Operations
Simplification
One of the powerful features of symbolic computation is the ability to simplify complex expressions using the `simplify()` function. For example:
expr = (x^2 - 4)/(x - 2);
simplified_expr = simplify(expr);
This simplifies the rational expression to `x + 2`, showcasing how symbolic manipulation can lead to clearer and more manageable forms of expressions.
Factorization and Expansion
Similarly, symbolic variables allow you to factor or expand expressions. Using the `factor()` and `expand()` functions, you can manipulate expressions easily:
expanded_expr = expand((x + 2)*(x - 3));
factored_expr = factor(x^2 - 9);
The first line expands the product, while the second line factors a polynomial, illustrating how symbolic variables support intricate algebraic manipulation.
Solving Equations Symbolically
Solving Linear Equations
Symbolic variables are also adept at solving equations algebraically. For example, to solve a simple linear equation:
syms x
eqn = 2*x + 3 == 7;
solution = solve(eqn, x);
Executing this code yields the exact solution for `x`, which is `2`.
Solving Nonlinear Equations
Similarly, symbolic variables can solve nonlinear equations. Here’s an example:
eqn = x^2 - 4 == 0;
solutions = solve(eqn, x);
This will return `-2` and `2`, demonstrating how symbolic computations can yield precise solutions for complex equations.
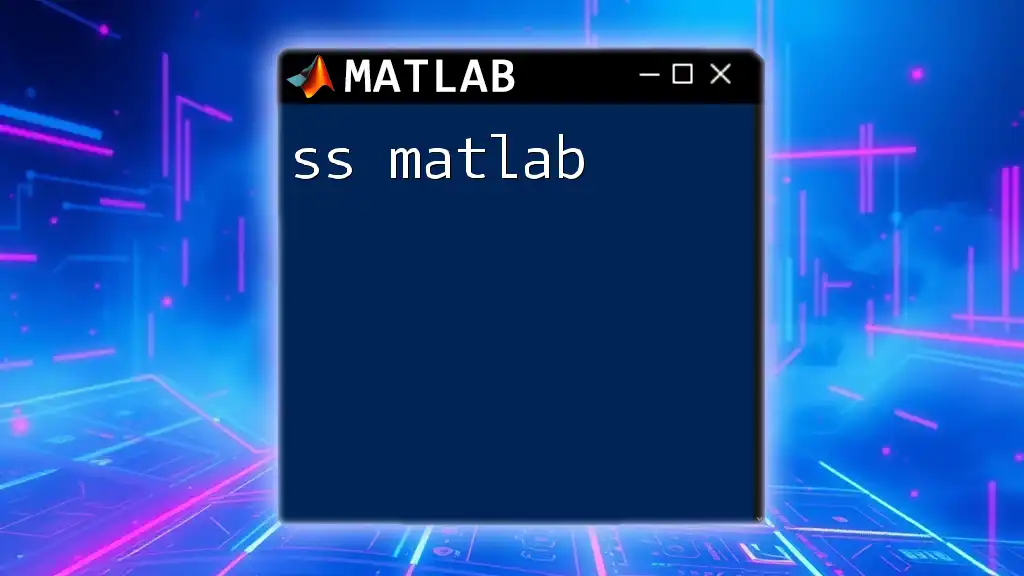
Advanced Usage of `syms`
Defining Functions with Symbolic Variables
You can create symbolic functions that can be manipulated within mathematical computations. For instance:
syms x
f = x^2 + 2*x + 1; % f is now a symbolic function
This function can then undergo differentiation, integration, and other analyses.
Using `syms` with Calculus
Differentiation
Symbolic differentiation is straightforward in MATLAB. For instance:
syms x
f = x^3 + 3*x^2 + 2*x + 1;
df = diff(f, x); % Derivative of f with respect to x
This quick operation yields the derivative of the function `f`.
Integration
Just as intuitive is symbolic integration:
integral_f = int(f, x); % Integral of f with respect to x
This code computes the indefinite integral of the function, illustrating the ease of symbolic calculus in MATLAB.
Plotting Symbolic Expressions
Visualizing symbolic expressions can lead to deeper insights into their behavior. Using the `fplot` function:
f = x^2 - 4;
fplot(f, [-5 5]); % Plots the function between -5 and 5
This command generates a plot for the quadratic equation, providing a visual representation of its roots and behavior.
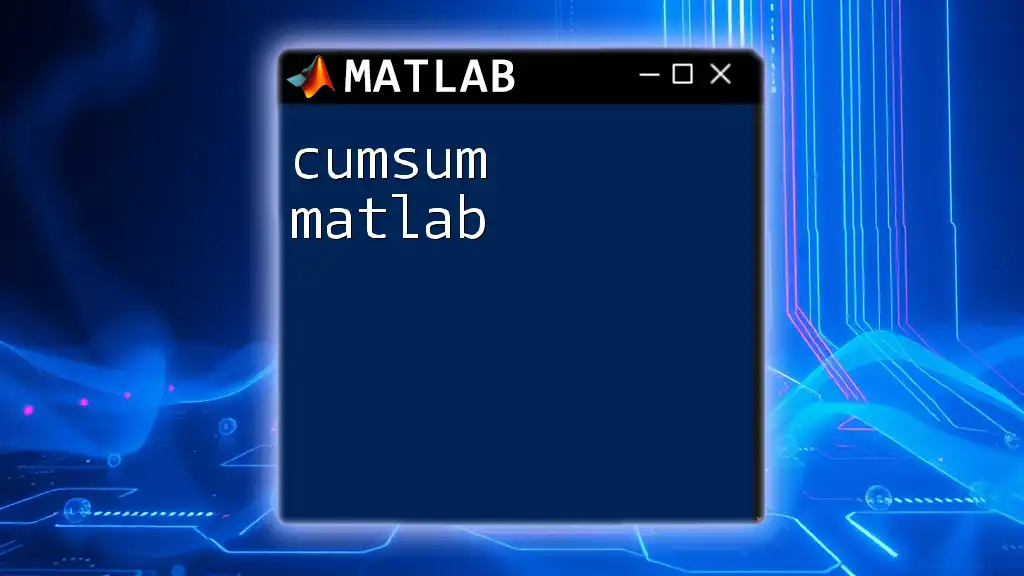
Best Practices for Using Symbolic Variables
Efficient Coding Techniques
When using symbolic variables, it’s essential to minimize redundancy in your expressions. Utilizing symbolic arrays can help streamline computations, leading to clearer and more efficient code.
Common Pitfalls and How to Avoid Them
A frequent challenge arises from confusing symbolic and numerical computations. Remember that symbolic variables retain their algebraic form, while numeric computations yield approximations. Be cautious of memory management when working with large symbolic matrices or complex expressions to prevent performance issues.
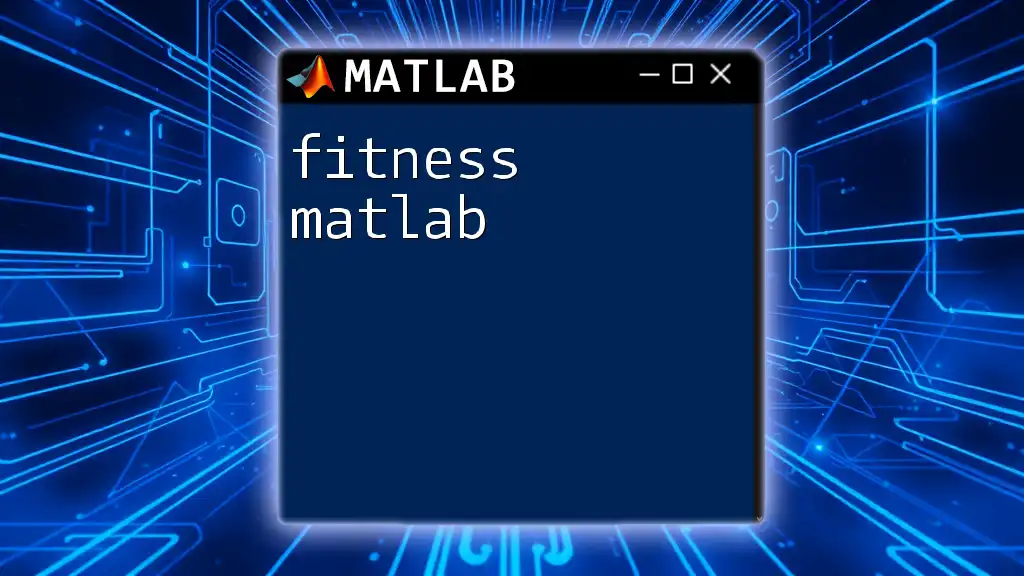
Conclusion
The `syms` command is an invaluable tool in MATLAB for engaging in symbolic computation, enabling users to manipulate and analyze mathematical expressions with precision and clarity. Whether solving equations, performing calculus, or visualizing functions, mastering the `syms` command expands the toolkit available to engineers, mathematicians, and scientists alike.
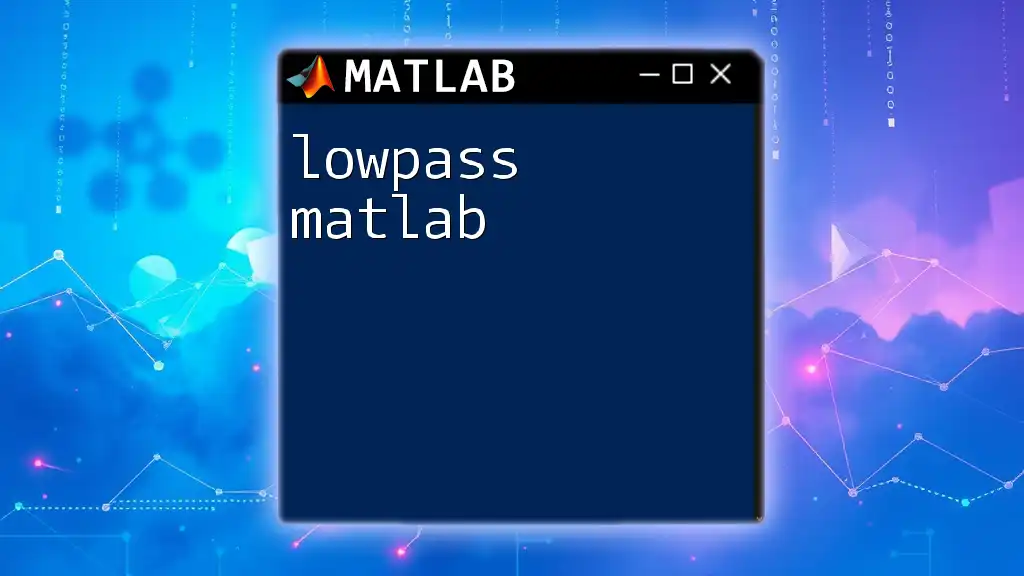
Further Reading and Resources
For those eager to learn more about symbolic computation in MATLAB, referring to the official MATLAB documentation is recommended. Additionally, exploring specialized textbooks and online courses can further enhance your understanding of symbolic math and its application in real-world problems.