The Symbolic Toolbox in MATLAB allows users to perform symbolic computation, enabling them to define and manipulate mathematical expressions analytically rather than numerically.
Here’s a simple code snippet demonstrating the use of symbolic variables and differentiation:
syms x
f = x^2 + 3*x + 5;
df = diff(f, x);
disp(df)
Getting Started with the Symbolic Toolbox
Installing the Toolbox
To make use of the symbolic toolbox in MATLAB, it’s essential first to ensure you have the right setup. Check the system requirements and verify that your MATLAB version supports the symbolic toolbox.
If you need to install the toolbox, follow these steps:
- Launch MATLAB and navigate to the Add-Ons menu.
- Search for "Symbolic Math Toolbox."
- Follow the prompts to install it directly from this interface.
Setting Up the Environment
To get started, you must load the toolbox into your workspace. This is done with the `syms` command, which allows you to declare symbolic variables.
syms x y z
This command prepares `x`, `y`, and `z` as symbolic variables, which you can use in various mathematical operations.
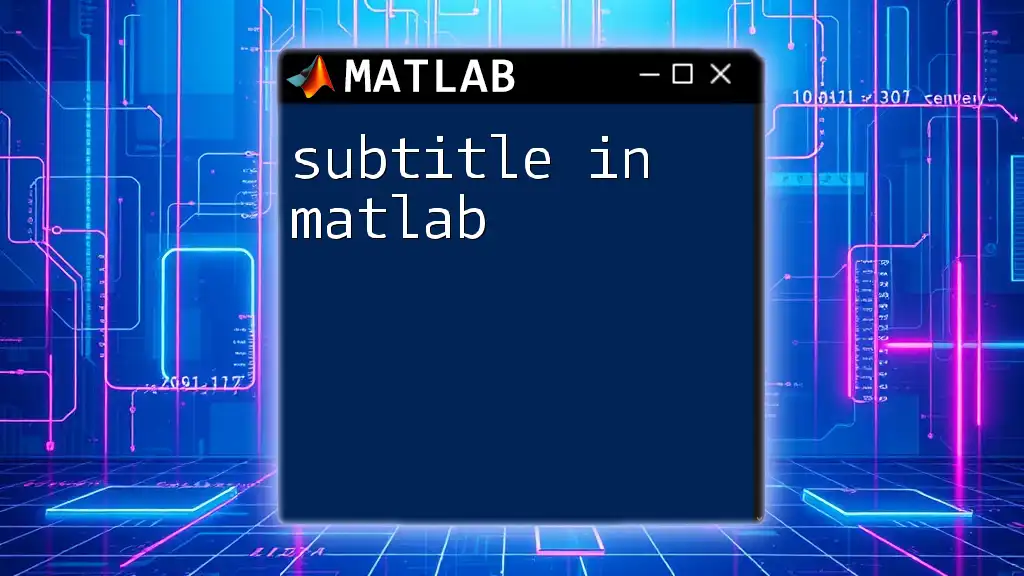
Core Functions and Features
Defining Symbolic Variables
Creating symbolic variables in MATLAB is straightforward with the `syms` command. You can declare single or multiple variables, allowing for flexible computation.
syms a b c
This single line defines three symbolic variables—`a`, `b`, and `c`. You can also define them as a vector:
syms x1 x2 x3
This is particularly useful when you're working with equations that require several variables at once.
Performing Algebraic Operations
The symbolic toolbox allows you to perform various algebraic operations seamlessly.
Basic Arithmetic: You can carry out basic arithmetic operations, such as addition, subtraction, multiplication, and division directly on symbolic variables. It makes mathematical expressions more intuitive:
f = x^2 + 3*x + 2;
This defines a quadratic expression symbolically, which can be manipulated further as needed.
Expanding and Factoring Expressions: The toolbox includes built-in functions to expand and factor expressions.
To expand an expression, you can use:
expand((x + 1)^2)
To factor an expression, use:
factor(x^2 - 4)
By using these functions, you can convert expressions into their required forms quickly.
Solving Equations Symbolically
One of the standout features of the symbolic toolbox in MATLAB is the ability to solve equations.
Solving Algebraic Equations: You can use the `solve` function to find the roots of equations symbolically.
For instance, solving a simple quadratic equation can be done as follows:
sol = solve(x^2 - 4 == 0, x)
The result returns the roots, which in this instance would be `x = -2` and `x = 2`.
Systems of Equations: If you need to solve multiple equations, the toolbox can handle that too. For example:
eq1 = x + y == 10;
eq2 = x - y == 2;
sol = solve([eq1, eq2], [x, y])
This finds a solution for both `x` and `y` simultaneously, making it invaluable for complex problems.
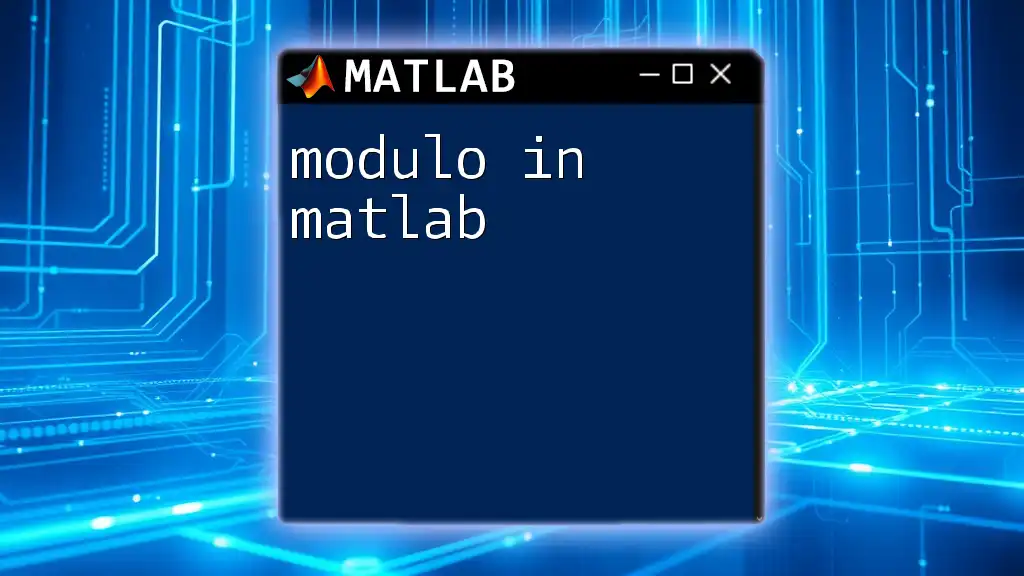
Advanced Features of the Symbolic Toolbox
Differentiation and Integration
A significant advantage of the symbolic toolbox in MATLAB is its ability to perform calculus operations.
Symbolic Differentiation: You can differentiate expressions effortlessly. For example:
diff(f, x)
This computes the derivative of `f` with respect to `x`, providing a symbolic representation of the derivative.
Symbolic Integration: Integration is also intuitive with the `int` function. You can calculate both indefinite and definite integrals easily:
int(f, x) % Indefinite
int(f, x, 0, 1) % Definite
These commands help you interpret complex functions in terms of area under curves and more.
Performing Limits
You can also calculate limits using the `limit` function, which is crucial in analysis when studying the behavior of functions.
For example, if you wish to compute the limit as `x` approaches zero for the sine function divided by `x`, you can use:
limit((sin(x)/x), x, 0)
This will return `1`, demonstrating the power of symbolic computation in seamlessly handling limits.
Series Expansion
Another useful feature is the ability to compute Taylor series using the `taylor` function, which allows you to approximate functions near a certain point effectively:
taylor(exp(x), x)
This will return the Taylor series expansion of the exponential function, facilitating various applications in engineering and physics.
Matrix Operations
The symbolic toolbox can also handle symbolic matrices, enabling users to perform operations on multidimensional arrays of symbolic elements.
To create a symbolic matrix, you can do the following:
A = [syms a b; syms c d];
With this matrix, you can perform operations such as multiplication or inversion:
C = A * A'
This capability is particularly important for engineers and scientists working with linear systems.
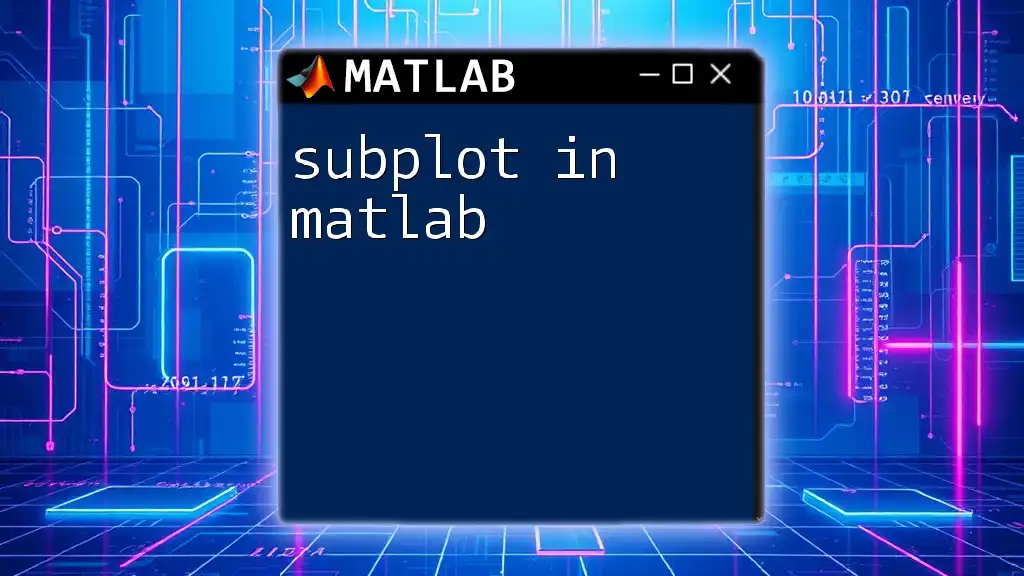
Practical Applications of the Symbolic Toolbox
Engineering Applications
One of the prominent uses of the symbolic toolbox in MATLAB is solving differential equations. For example, you can solve simple ordinary differential equations (ODEs) with:
dsolve('Dx = -x', 'x(0) = 1')
This results in the symbolic solution of the ODE, showing the power of symbolic computations in engineering contexts.
Physics Applications
In physics, accurate symbolic representations are vital for understanding complex relationships. The toolbox allows you to express equations symbolically and solve them analytically, which is beneficial for a wide range of applications in the physical sciences.
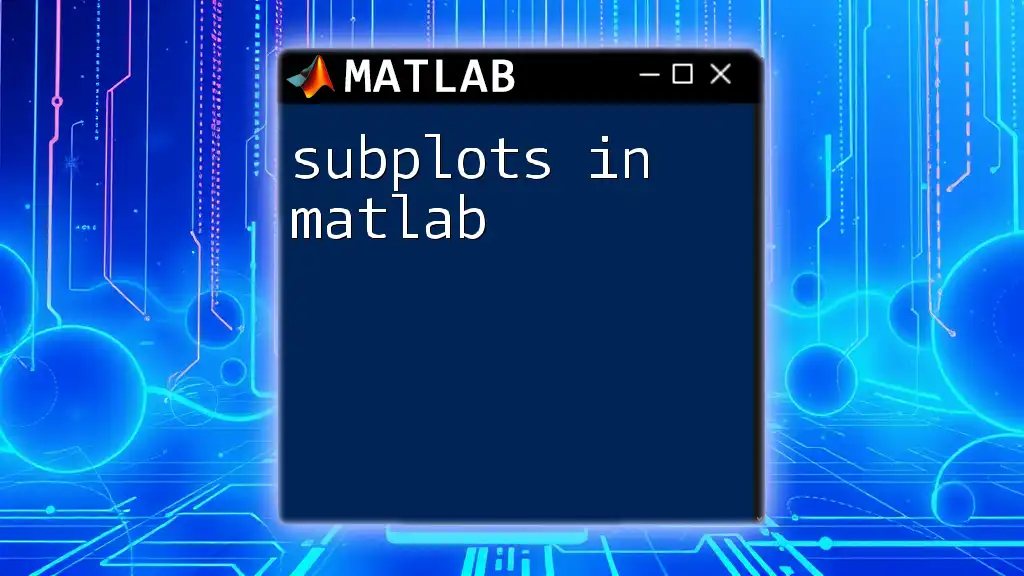
Troubleshooting Common Issues
Common Errors and Solutions
While working with the symbolic toolbox in MATLAB, you may encounter various errors related to syntax or variable definitions. One common issue occurs when trying to execute operations on non-symbolic types. Always ensure variables are defined with `syms` before operations.
Performance Tips
To improve performance while performing symbolic computations, consider following these best practices:
- Simplify expressions before operation to minimize complexity.
- Clear variables not in use, freeing up system memory.
Being mindful of your operations enhances the efficiency of your computations significantly.
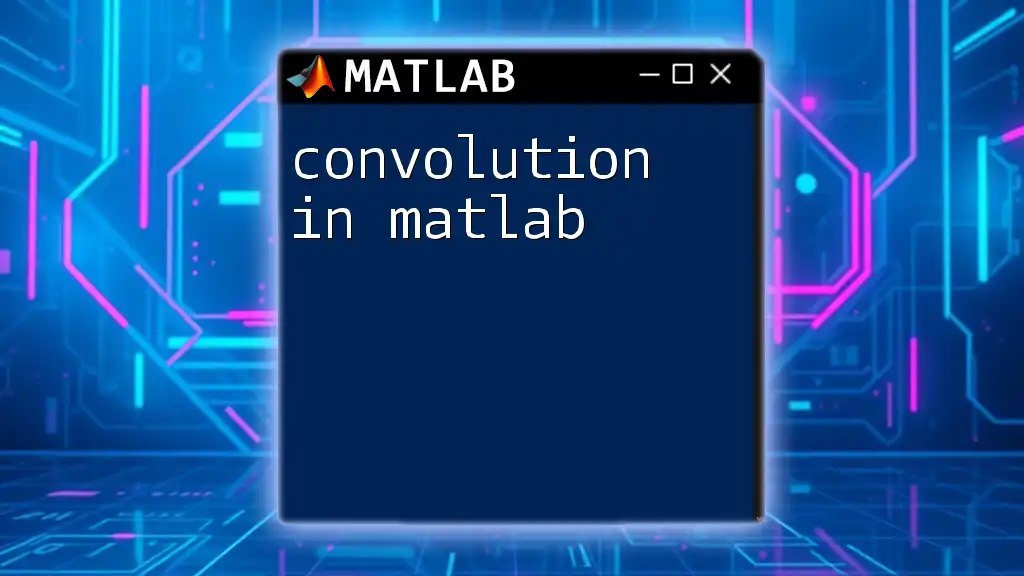
Conclusion
The symbolic toolbox in MATLAB is a powerful tool that greatly enhances computational capabilities in various fields such as engineering, physics, and mathematics. From defining symbolic variables to solving complex equations symbolically, this toolbox offers functionalities that streamline calculations and modeling. As you explore these features, you gain the ability to manipulate mathematical expressions in a way that is both intuitive and accessible, paving the way for deeper analysis and understanding.
Encouraging experimentation with more complex symbolic computations can lead to innovative solutions to real-world problems. The journey into symbolic mathematics begins here—dive in!
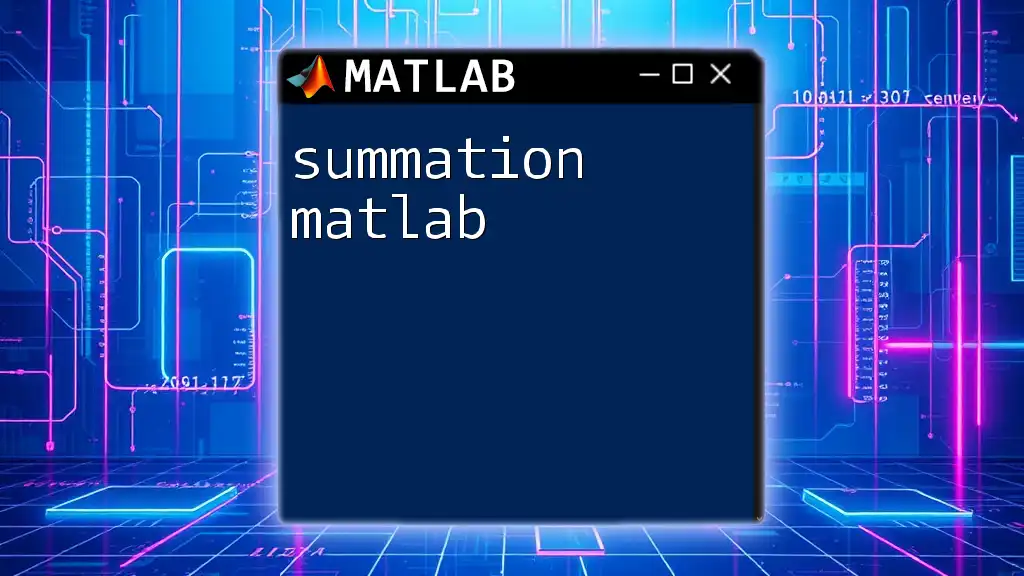
References and Further Reading
For those interested in expanding their knowledge and skills with the symbolic toolbox, consider the following resources:
- Books: Look for comprehensive texts on MATLAB Symbolic Math Toolbox to deepen your understanding.
- Online Tutorials: Websites like MATLAB’s official documentation and forums offer valuable insights and user experiences.
- Charts: Utilize visual aids that summarize key functions and operations for quick reference.