In MATLAB, you can plot an xnxn matrix as a graph by using the `imagesc` function, which visualizes matrix data with color coding for quick interpretation.
% Example MATLAB code to plot an xnxn matrix
A = rand(10, 10); % Generate a 10x10 random matrix
imagesc(A); % Create a color-scaled image of the matrix
colorbar; % Display a color bar indicating data values
title('Plot of a 10x10 Random Matrix'); % Add a title to the plot
Understanding the xnxn Matrix
Definition of a Matrix
In mathematics, a matrix is a rectangular array of numbers, symbols, or expressions, arranged in rows and columns. Each element of a matrix can be identified by its row and column indices.
An xnxn matrix is a specific type of square matrix where the number of rows equals the number of columns. This means it has dimensions defined by the same positive integer n. For example, a matrix with dimensions 3x3 has 3 rows and 3 columns, forming a perfect square.
Characteristics of xnxn Matrices
xnxn matrices possess unique properties that are significant in various fields:
-
Symmetry: Many xnxn matrices are symmetric, meaning the matrix is equal to its transpose. This property is fundamental in linear algebra and has applications in optimization problems and physics.
-
Determinants and Eigenvalues: Square matrices have associated determinants and eigenvalues, which are crucial for solving linear systems, performing transformations, and more.
-
Applications: The versatility of xnxn matrices makes them invaluable in statistics, computer graphics, machine learning, and signal processing, among other domains.
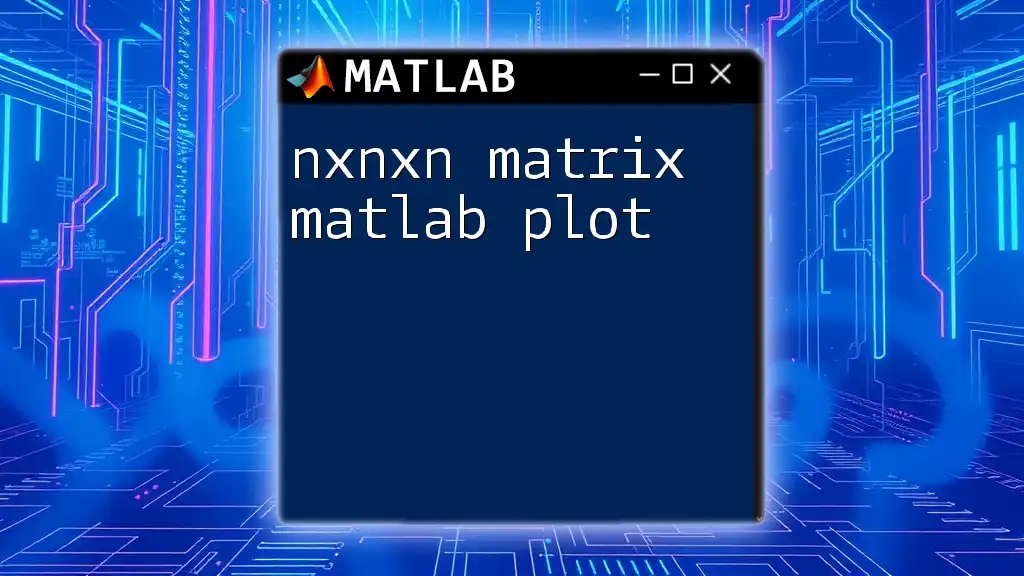
Setting Up MATLAB for Matrix Plotting
Installation and Basic Setup
To get started with plotting xnxn matrices in MATLAB, ensure you have the latest version installed. As you launch MATLAB, familiarize yourself with the interface, which contains the command window, workspace, and various toolbars.
Loading Data into MATLAB
You can create an xnxn matrix directly in the command window. For example, to create a 3x3 matrix, you would use the following command:
A = [1 2 3; 4 5 6; 7 8 9]; % Example of a 3x3 matrix
This command initializes a matrix A with the specified values. From here, you can start visualizing and manipulating the matrix.
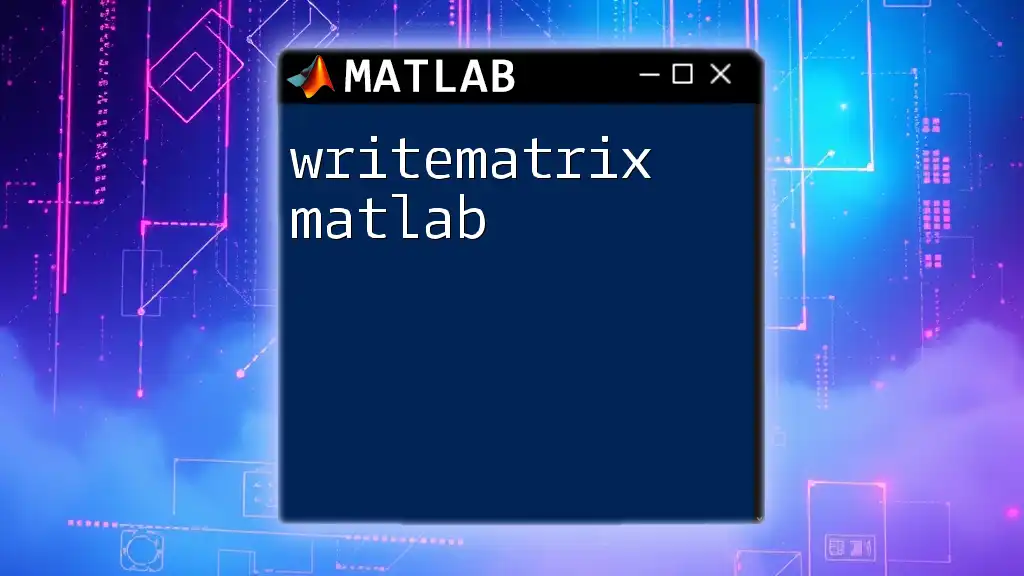
Visualizing the xnxn Matrix in MATLAB
Basic Plotting Functions
Using `imagesc`
The `imagesc` function is a straightforward way to visualize the contents of a matrix as an image. It scales the color data to the range of your data, making it easy to interpret visually.
To use `imagesc` with matrix A:
imagesc(A); % Visualizes the matrix A as an image
colorbar; % Adds a colorbar to indicate value scale
This command displays matrix A as a colored grid where each color corresponds to a different value range. Adding a colorbar provides context for how the colors relate to the values in the matrix.
Using `surf`
For a 3D perspective, the `surf` function creates a surface plot of the matrix, allowing you to see the relationships between the data points in three dimensions.
Here’s how to use it:
surf(A); % Creates a 3D surface plot of matrix A
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
In this example, the xlabel, ylabel, and zlabel define axes labels, making the plot more informative. Surface plots are particularly useful for analyzing peaks and valleys in the data.
Customizing Plots
Changing Color Maps
Visual appeal and clarity can be enhanced by changing the color map. MATLAB offers various color maps, allowing you to choose one that best fits your data visualization needs.
For example, to change the color map to 'jet', you can execute this command:
colormap(jet); % Changes the color map to 'jet'
This command will apply a rainbow spectrum to the matrix plot, enhancing the visual differentiation between different values.
Adding Titles and Labels
Labeling your plots is crucial for conveying information clearly. Adding titles and axes labels helps viewers understand the context of the data:
title('3x3 Matrix Visualization');
xlabel('Column Index');
ylabel('Row Index');
These annotations help clarify what the viewer should focus on, particularly when presenting findings.
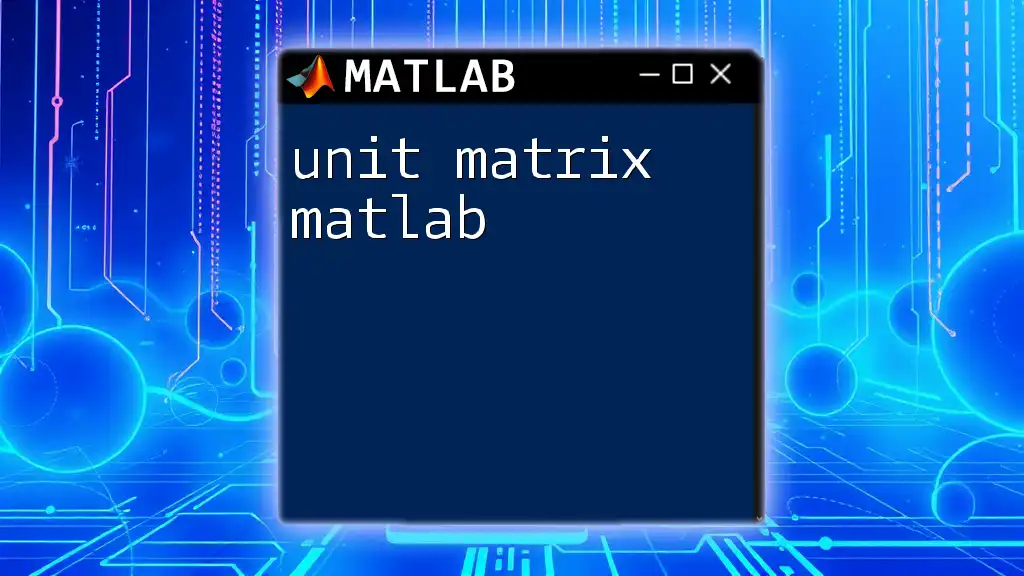
Advanced Matrix Plotting Techniques
Heatmaps
Heatmaps are an efficient way to visualize data density across a matrix. They are particularly effective for larger datasets, providing insightful representations of patterns within the data.
Here's how to create a heatmap for matrix A:
heatmap(A);
The heatmap function automatically assigns colors to the cells based on values, making it simple to identify high and low concentrations of values at a glance. Heatmaps are beneficial when comparing multiple datasets simultaneously.
Subplots for Multiple Matrices
Sometimes, it is useful to compare different matrices side by side. The `subplot` function allows you to arrange multiple plots in a single figure window.
For instance, if you want to plot two different matrices:
B = [10 11 12; 13 14 15; 16 17 18];
subplot(1,2,1);
imagesc(A);
title('Matrix A');
subplot(1,2,2);
imagesc(B);
title('Matrix B');
In this code, `subplot(1,2,1)` and `subplot(1,2,2)` define a 1-row, 2-column grid layout for the plots. Having the visual comparisons readily available allows for a more immediate analysis of the differences and similarities between the two matrices.
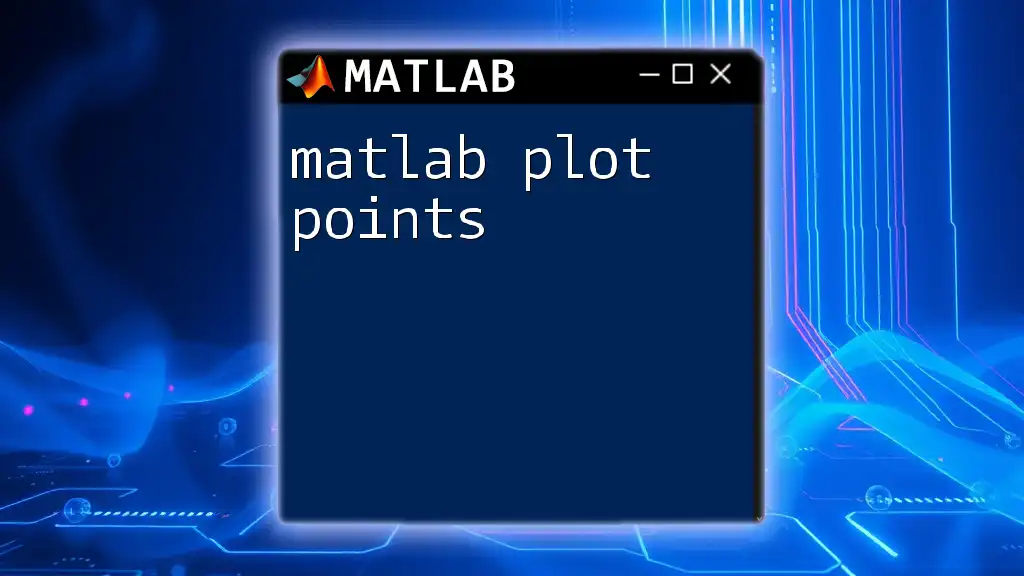
Troubleshooting Common Issues
Common Plotting Errors
While plotting matrices in MATLAB, you might encounter errors. Most common issues include incorrect matrix dimensions or data types. Always ensure your matrix is a numerical array and that its size conforms to the requirements of the plotting function you are using.
If your code throws an error, checking the dimensions can often clarify the issue.
Performance Considerations
Older versions of MATLAB or working with very large xnxn matrices can lead to performance slowdowns when plotting. To enhance performance, consider:
- Reducing the matrix size if possible
- Using functions optimized for larger datasets
- Visualizing only a subset of the data when practical
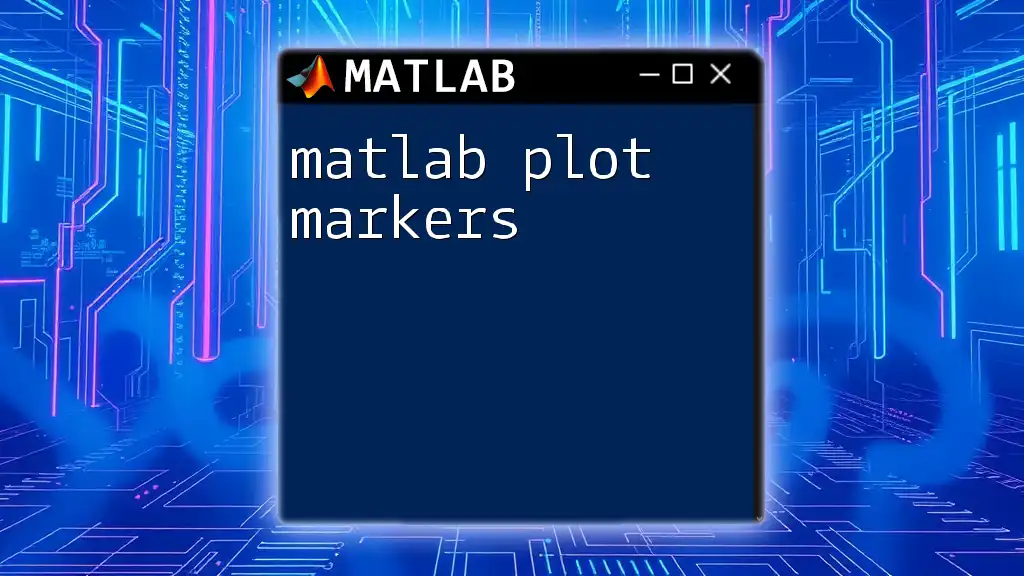
Conclusion
Understanding how to plot and analyze xnxn matrices in MATLAB provides invaluable insights into your data. With the techniques outlined in this guide, you can visualize matrix data effectively, enabling clearer communication of analytical findings. The blend of basic and advanced plotting functions prepares you to tackle various visualization challenges, enhancing your data storytelling capabilities.
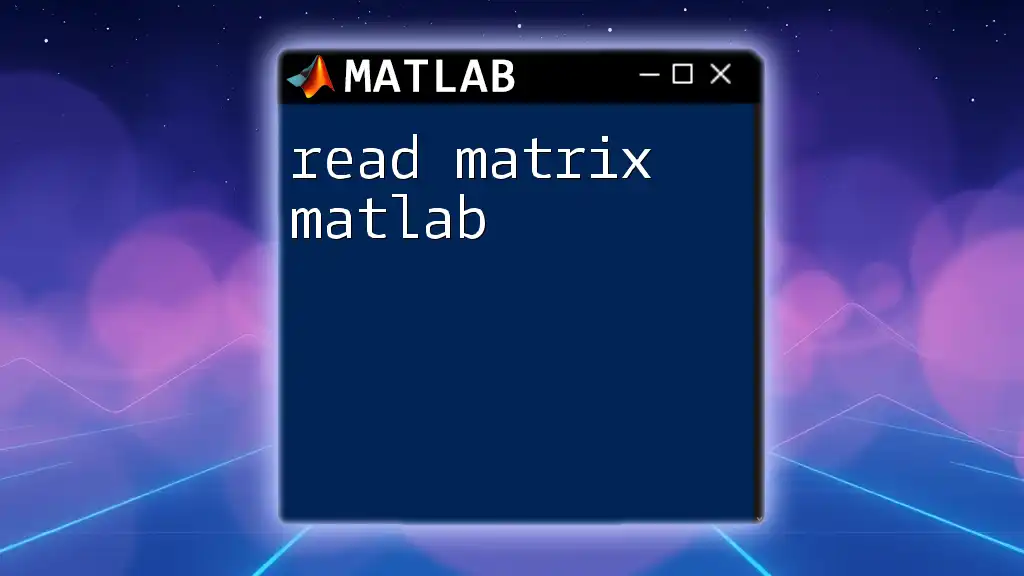
Additional Resources
Books and Online Courses
For those interested in deepening their MATLAB knowledge, numerous resources are available, including books and online courses focused on MATLAB programming and visualization techniques.
MATLAB Documentation
The official MATLAB documentation is an excellent resource for understanding built-in functions and features. Refer to this comprehensive resource to explore specific functions and their applications further.
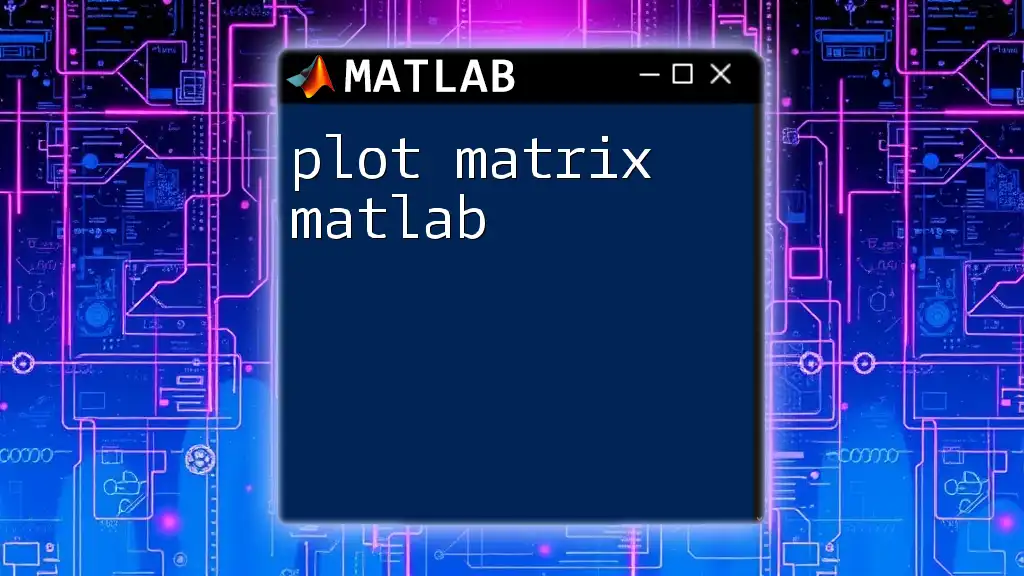
Call to Action
Stay innovative in your approach to learning MATLAB. Subscribe to our company for more cutting-edge tutorials, and don't hesitate to share your own experiences with matrix plotting in the comments. Your community is here to learn and grow with you!