A unit step signal in MATLAB can be created using the `heaviside` function, which generates a step function that transitions from 0 to 1 at a specified point, typically at time \( t=0 \).
Here’s a simple example to create and plot a unit step signal:
t = -1:0.01:1; % Time vector
u = heaviside(t); % Unit step signal
plot(t, u); % Plot the signal
xlabel('Time (s)');
ylabel('Amplitude');
title('Unit Step Signal');
grid on;
Understanding the Unit Step Signal
What is a Unit Step Signal?
A unit step signal is a fundamental concept in signal processing and control systems. It is often represented mathematically as \( u(t) \) and is defined as:
\[ u(t) = \begin{cases} 0 & \text{if } t < 0 \\ 1 & \text{if } t \geq 0 \end{cases} \]
This piecewise function essentially indicates the transition of a signal from 0 to 1 at \( t = 0 \). The unit step signal is crucial for the analysis of systems' responses, particularly in examining how systems react to changes over time.
Visual Representation
Visualizing the unit step signal helps solidify your understanding of its characteristics. The signal graphically transitions from 0 to 1 at \( t = 0 \). This step change is primarily used in simulations to introduce problems involving transient response in systems.
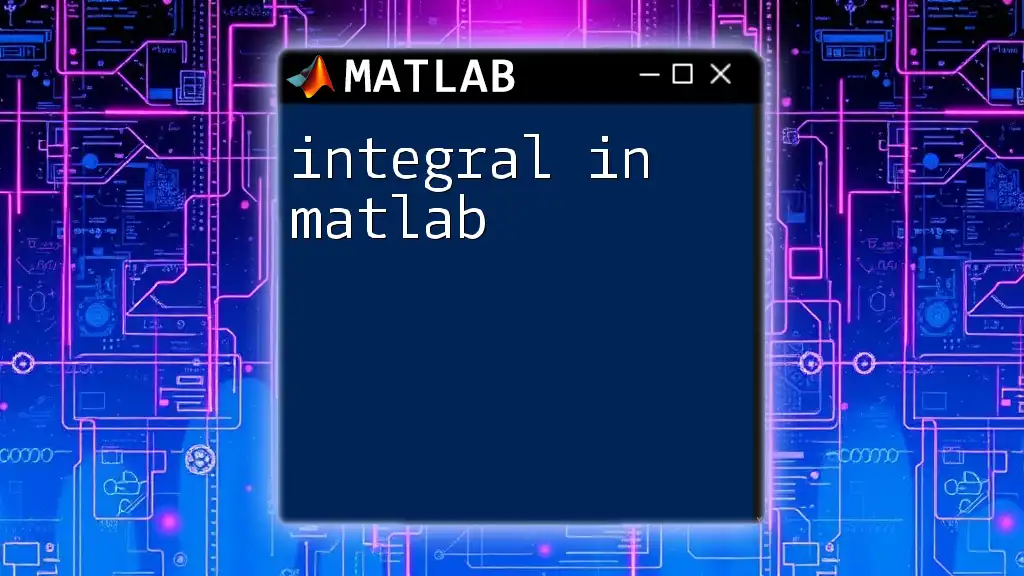
Implementing Unit Step Signal in MATLAB
Setting Up MATLAB Environment
Before diving into coding, ensure your MATLAB environment is ready. Open MATLAB and create a new script file where you can input your commands and visualize the results.
Basic MATLAB Command for Unit Step Signal
Using the `heaviside` Function
MATLAB provides a built-in function called `heaviside`, which represents the unit step function effectively. The syntax for this function is simple, making it easy to generate unit step signals.
Here's how you can implement it:
t = -5:0.1:5; % Create a time vector ranging from -5 to 5
u = heaviside(t); % Generate the unit step signal using the heaviside function
plot(t, u); % Plot the signal
xlabel('Time'); % Label for the x-axis
ylabel('Amplitude'); % Label for the y-axis
title('Unit Step Signal Using Heaviside Function'); % Title of the plot
grid on; % Enable grid for better visualization
In this example, `t` is defined as a vector that ranges from -5 to 5, with increments of 0.1. The `heaviside(t)` command generates the unit step signal, which is then plotted for visualization.
Alternative Method for Unit Step Signal
Custom Implementation
While the `heaviside` function is convenient, you might want to create a custom implementation of the unit step function for more flexibility or educational purposes. Below is the logic and code to accomplish this:
function u = unit_step(t)
u = zeros(size(t)); % Initialize the output vector to zeros
u(t >= 0) = 1; % Set the values to 1 for all t values greater than or equal to 0
end
% Example usage:
t = -5:0.1:5; % Create a time vector
u = unit_step(t); % Call the custom function to generate the unit step signal
plot(t, u); % Plot the generated unit step signal
xlabel('Time');
ylabel('Amplitude');
title('Custom Unit Step Signal');
grid on;
This function, `unit_step`, initializes an output vector of zeros and sets its values to 1 where the input `t` is greater than or equal to 0. This straightforward method reinforces the concept of time-based signal generation.
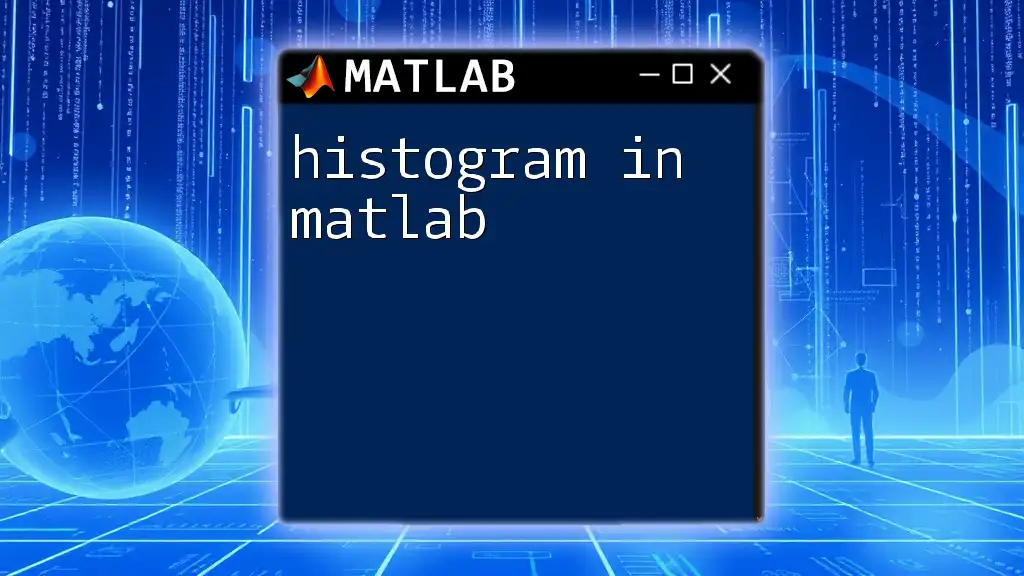
Advanced Features and Manipulation
Discrete vs Continuous Unit Step Signals
Understanding the difference between discrete and continuous unit step signals can help you in various applications. Continuous signals represent the unit step function over a continuous range of time, while discrete signals consist of distinct time intervals, typically used in digital signal processing.
To create a discrete unit step signal, you might sample the continuous signal and work with it in a specific time frame.
Modifying the Unit Step Signal
Scaling and Shifting
Unit step signals can be manipulated through scaling (changing amplitude) and shifting (changing the point in time where the step occurs). For instance, you can shift the unit step left or right by modifying the input time variable.
Here’s how to shift the unit step signal to the right by 1 unit:
tShifted = t - 1; % Shift the time vector by 1 unit
uShifted = heaviside(tShifted); % Generate the shifted unit step signal
plot(t, uShifted); % Plot the shifted signal
xlabel('Time');
ylabel('Amplitude');
title('Shifted Unit Step Signal');
grid on;
In this case, the unit step occurs at \( t = 1 \), illustrating how easy it is to manipulate signals using MATLAB commands.
Using Unit Step in System Simulation
Step Response of a System
The unit step signal is commonly used to simulate the step response of dynamic systems. This response demonstrates how systems react to sudden changes, making the unit step a valuable tool in control systems.
To simulate a first-order system's step response, you can use the following code:
sys = tf([1], [1, 1]); % Define the transfer function of a first-order system
step(sys); % Plot the step response of the system
title('Step Response of a First Order System'); % Title of the plot
grid on; % Enable grid for clarity
In this example, `tf` creates a transfer function model, and `step(sys)` computes and displays the step response. Such simulations are essential in engineering fields, particularly when designing controllers and analyzing system behavior.
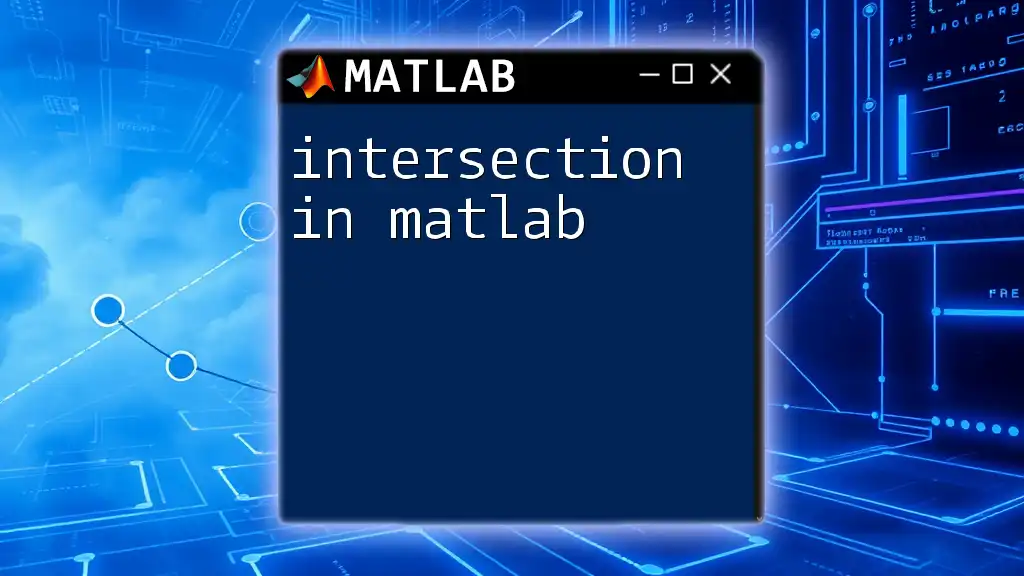
Common Errors and Troubleshooting
Typical Issues When Using MATLAB for Unit Step Signals
When implementing unit step signals in MATLAB, you might encounter common errors such as mislabeling your axes, not appropriately defining your time vector, or using the wrong function syntax. Solutions to these problems typically involve checking code syntax, ensuring all functions are properly called, and verifying that vectors match in dimensions for operations.
Tips for Debugging Code:
- Always initialize vectors before use.
- Use `disp()` or `fprintf()` statements to print intermediate results for verification.
- Check the MATLAB documentation for syntax errors or function usage if uncertain.
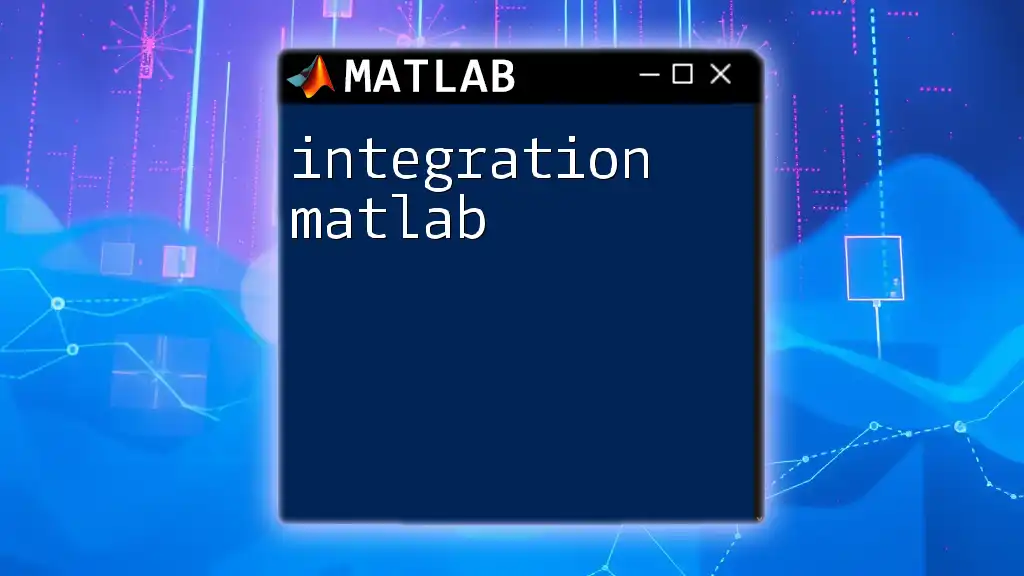
Conclusion
In this comprehensive guide, we've explored the concept of the unit step signal in MATLAB, its mathematical foundation, implementation techniques using built-in functions and custom code, and advanced manipulations like scaling and shifting. We also examined its application in system simulation, highlighting the significance of the unit step in analyzing system responses.
When practicing these concepts, remember that hands-on experience is vital. Experiment with different parameters, observe their effects, and reinforce your understanding of MATLAB commands through practical applications.
Don’t hesitate to delve deeper into MATLAB’s capabilities or reach out to communities and tutorials that foster knowledge in signal processing and control systems.