A row vector in MATLAB is a one-dimensional array where all the elements are arranged in a single row, typically defined using square brackets and spaces or commas to separate the elements.
Here’s an example of how to create a row vector in MATLAB:
rowVector = [1, 2, 3, 4, 5];
Understanding Vectors in MATLAB
Definition of Vectors
In MATLAB, a vector is a one-dimensional array of numbers. It can either be a row vector or a column vector. A row vector has a single row and multiple columns, while a column vector has a single column and multiple rows. Row vectors can be particularly useful for storing multiple data points or coefficients compactly.
Types of Vectors
When dealing with vectors in MATLAB, it’s crucial to understand the types of vectors available. You’ll primarily encounter:
- Row Vectors: Defined with square brackets and containing multiple elements in a single row.
- Column Vectors: Defined with elements in a single column, which can impact how you perform operations on them.
- Multidimensional Arrays: An extension of vectors, used for more complex data representation, essential in various applications.
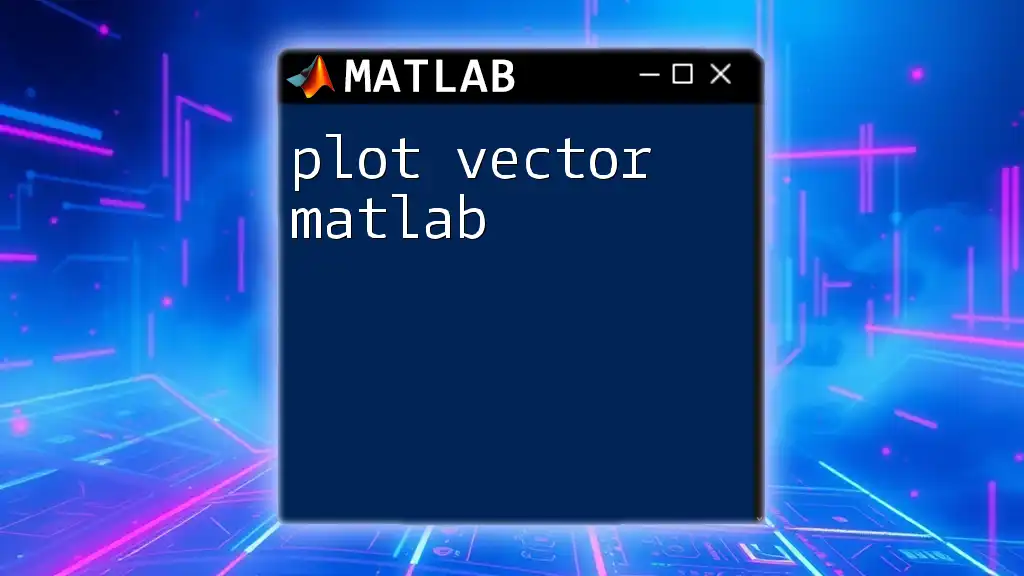
Creating Row Vectors
Basic Creation of Row Vectors
Creating a row vector is straightforward. You can simply use square brackets to define your vector. For example, to create a row vector containing the first five positive integers, use:
row_vector = [1, 2, 3, 4, 5]; % Using square brackets
In this syntax, elements are separated by commas or spaces, and MATLAB treats them as a single row.
Creating Row Vectors Using Built-in Functions
MATLAB offers several convenient functions for generating row vectors.
- Using the `linspace` function: This function generates linearly spaced vectors. For example, if you want to create a row vector with five evenly spaced values between 1 and 10, you can do so as follows:
row_vector = linspace(1, 10, 5); % Creates a row vector with 5 evenly spaced points
- Using the colon operator: This method allows you to create simple sequences efficiently. For instance, to generate a row vector from 1 to 5, you would write:
row_vector = 1:5; % Creates a row vector from 1 to 5
Both methods add flexibility in generating specific collections of data quickly, offering ease of access based on your needs.
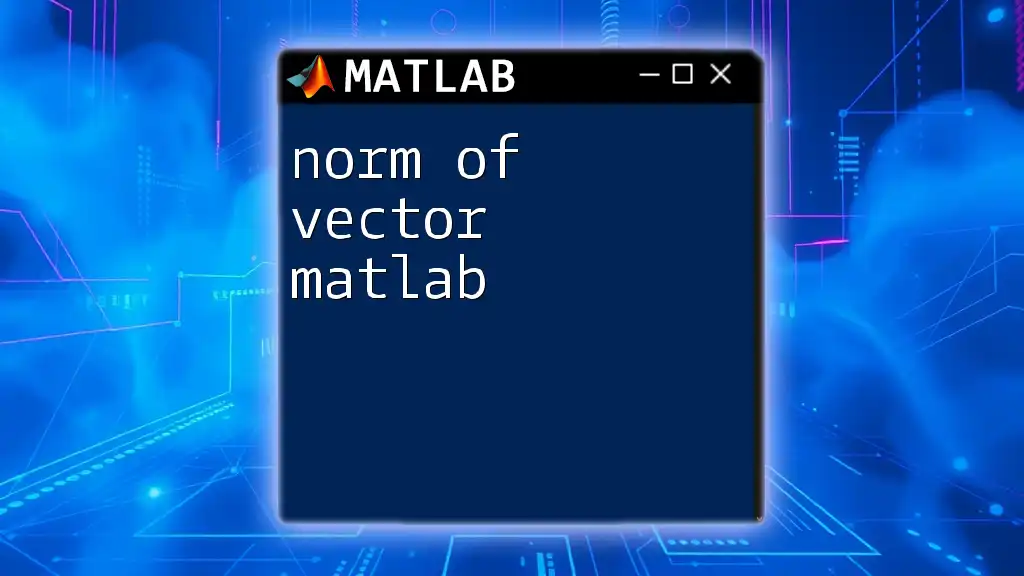
Accessing and Modifying Row Vectors
Accessing Elements of a Row Vector
Once you have a row vector, you can easily access its elements using indexing. MATLAB uses 1-based indexing, meaning the first element is accessed with index 1. For example, to get the second element of the row vector:
element = row_vector(2); % Accessing the second element
You can also access multiple elements using slicing techniques.
Modifying Elements of a Row Vector
Modifying a row vector is just as simple as accessing its elements. You can change the value of a specific element by referencing its index. For example, if you want to change the third element to 10, you would write:
row_vector(3) = 10; % Changing the third element to 10
To add new elements to your row vector, you can access the end of the vector with the keyword `end`. For example:
row_vector(end + 1) = 6; % Appending a new element at the end
This flexibility enables you to dynamically change and grow your data as needed.
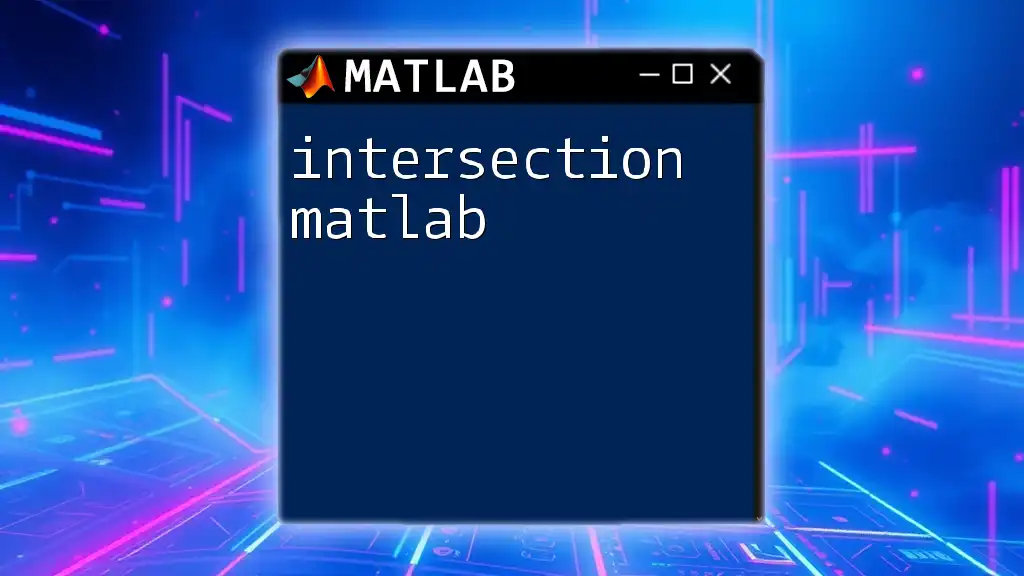
Performing Operations on Row Vectors
Basic Mathematical Operations
MATLAB allows various mathematical operations on vectors, which are essential for data analysis and manipulation. Basic operations like addition, subtraction, multiplication, and division can be performed directly.
For example, consider two row vectors:
vector_a = [1, 2, 3];
vector_b = [4, 5, 6];
result = vector_a + vector_b; % Element-wise addition
The result will be a new vector containing the sum of corresponding elements. Understanding how to operate on row vectors efficiently is key to successful numerical computations.
Concatenating Row Vectors
When you need to merge two row vectors, you can concatenate them. This operation can be very handy for data merging.
For example, to concatenate two row vectors:
vector_a = [1, 2, 3];
vector_b = [4, 5, 6];
combined_vector = [vector_a, vector_b]; % Concatenating two row vectors
This produces a single row vector combining all elements from both vectors, useful for creating datasets or combining results.
Applying Functions to Row Vectors
MATLAB provides numerous built-in functions that can be applied to row vectors, allowing you to perform analysis efficiently. Some common functions include:
- Sum of elements:
total_sum = sum(row_vector); % Calculating the sum of elements
- Mean of the elements:
average = mean(row_vector); % Calculating the mean
These functions are powerful tools you can leverage while analyzing large datasets, automatically providing critical insights in your computations.
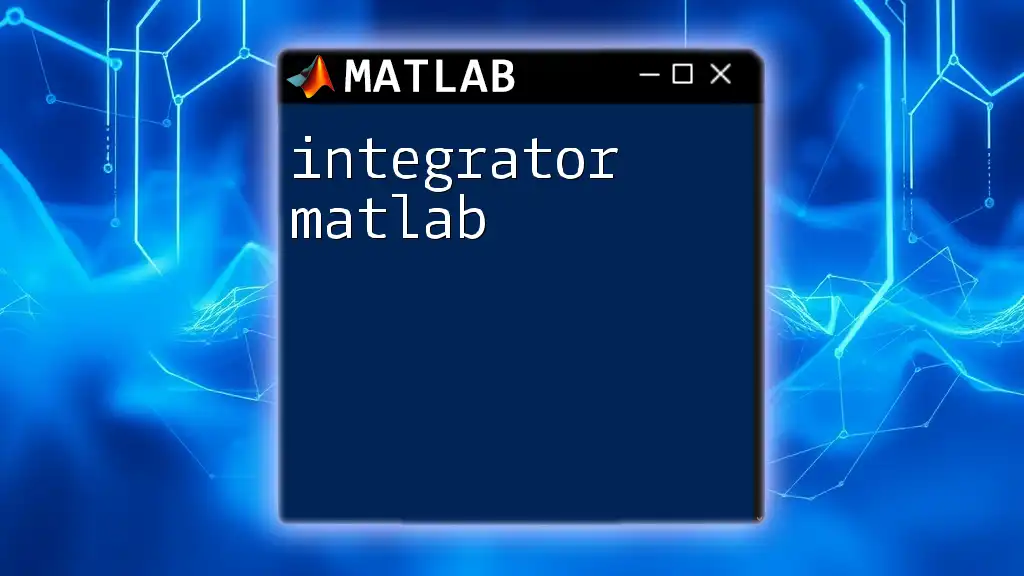
Row Vectors in MATLAB Applications
Using Row Vectors in Data Analysis
Row vectors are often utilized in data analysis to store sample data points or coefficients. For instance, if you have measurements from multiple experiments, you can store them in row vectors for easy access and manipulation. MATLAB’s flexibility with row vectors significantly simplifies tasks such as statistical analysis and multidimensional data handling.
Visualizing Row Vectors
Visualizing data represented by row vectors aids in understanding trends and relationships. MATLAB’s robust plotting capabilities allow you to easily represent row vectors graphically. For example, to plot a relationship between two variables stored in row vectors `x` and `y`, you might use:
x = 1:5;
y = [2, 4, 6, 8, 10];
plot(x, y); % Basic 2D plot
title('Visualization of Row Vector');
xlabel('X-axis');
ylabel('Y-axis');
This visualization can help in grasping the data structure and identifying patterns in your analyses.
Row Vectors in Machine Learning
In the context of machine learning, row vectors frequently represent samples or features. Each row can stand for a single data point, while the columns encode features of that data. Understanding how to manipulate row vectors effectively is crucial for preprocessing datasets and feeding them into algorithms.
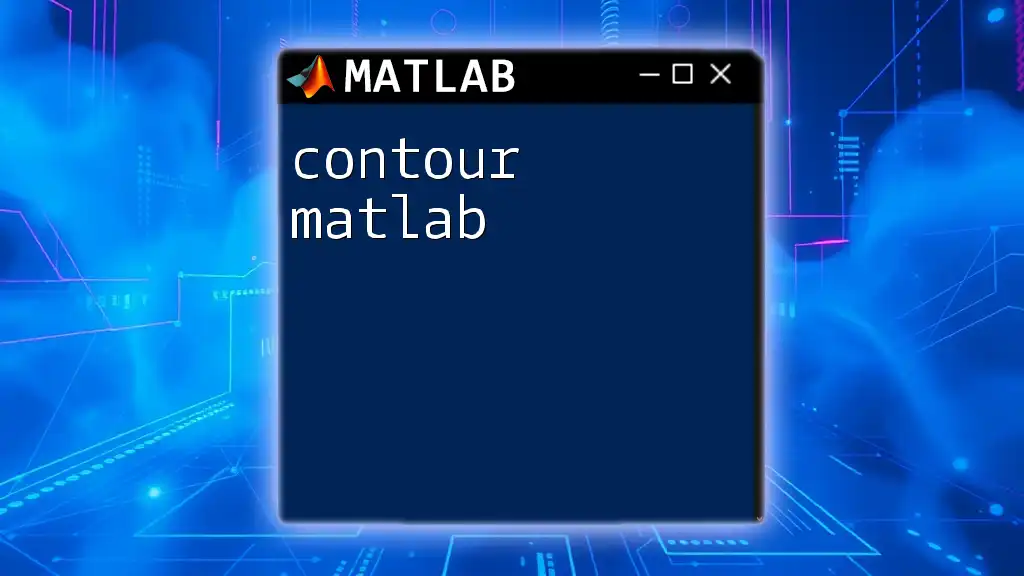
Best Practices and Tips for Working with Row Vectors
Efficient Memory Management
When working with large data sets, be mindful of memory management. MATLAB creates a copy of vectors when you modify them. To optimize, consider using in-place modifications where feasible to prevent unnecessary memory allocation.
Debugging Common Vector Issues
While working with row vectors, you may encounter common pitfalls such as indexing errors or attempts to operate on different vector sizes. Always ensure your operations are dimensionally compatible. Debugging tools in MATLAB can help highlight such issues, allowing for quick resolutions.
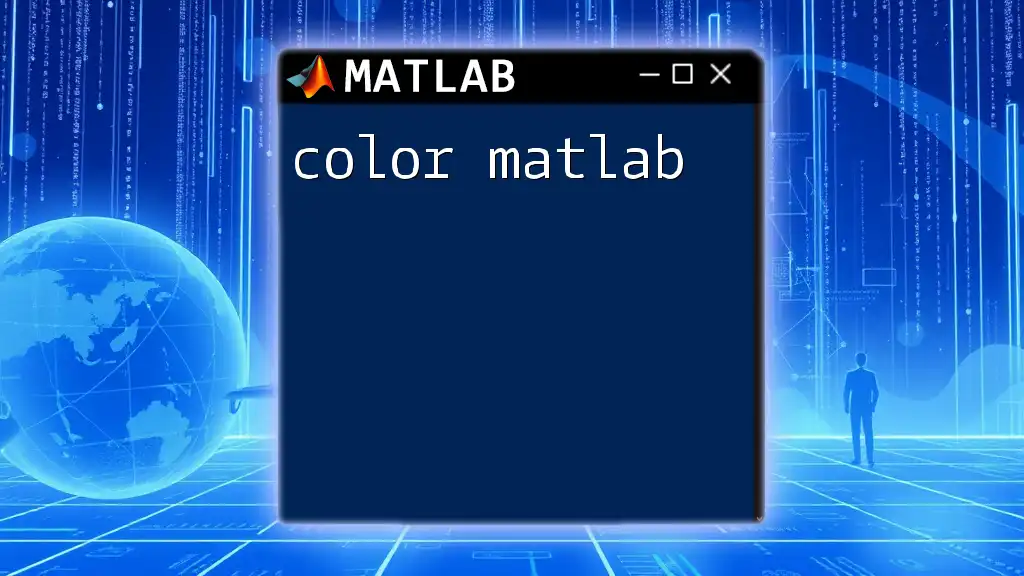
Conclusion
Mastering row vectors in MATLAB is fundamental for anyone looking to navigate data analysis, visualization, or algorithm development effectively. By understanding how to create, manipulate, and analyze these vectors, you can elevate your MATLAB proficiency and enhance your capabilities across numerous applications. Engage with the material, practice consistently, and you'll find that making effective use of row vectors can substantially improve your programming experience in MATLAB.
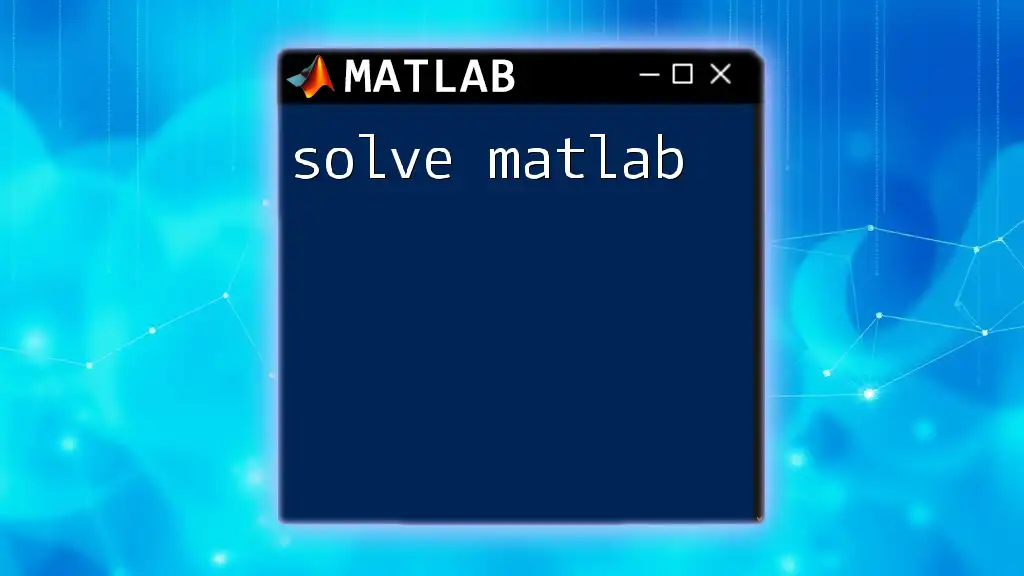
Additional Resources
For further learning, consider exploring the official MATLAB documentation, or recommended books on MATLAB programming and data analysis to solidify your knowledge and skills in mastering row vectors.