In MATLAB, a `do while` loop allows you to execute a block of code repeatedly as long as a specified condition evaluates to true, ensuring that the code within the loop runs at least once before the condition is tested.
Here's an example code snippet in markdown format:
count = 1;
do
fprintf('Count is: %d\n', count);
count = count + 1;
while count <= 5;
(Note: MATLAB uses `while` loops but does not have a native `do while` structure, so the above represents the logic; instead, use a `while` loop where the initial action occurs before checking the condition.)
The "Do While" Loop: An Overview
What is a Do While Loop?
The do while loop is a control flow statement that allows code to be executed multiple times based on a boolean condition. Importantly, this loop guarantees that the code block will run at least once before the condition is evaluated. This characteristic makes it distinct from a while loop, which evaluates the condition before executing the loop body.
Syntax of the Do While Loop in MATLAB
The basic syntax of a do while loop in MATLAB is as follows:
do
% execute code
while condition;
Here, the code inside the `do` block will execute, and after its completion, the condition will be checked. If the condition evaluates to true, the loop will execute again; if false, the loop will terminate.
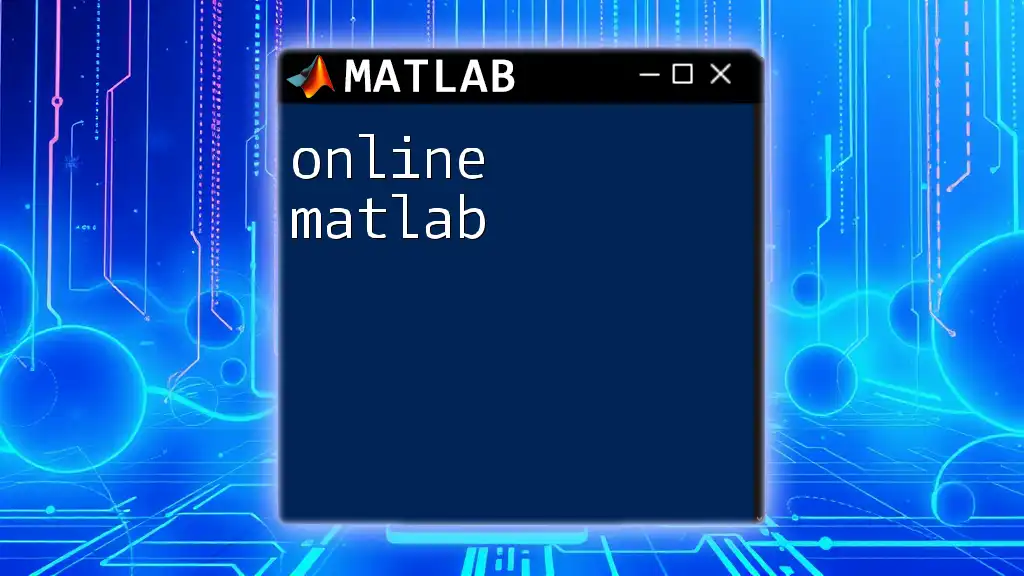
How Does the Do While Loop Work?
Execution Flow
The execution flow of a do while loop is straightforward. The statements inside the loop will execute first, then the condition is checked. If the condition turns out to be true, the loop repeats; if false, execution moves to the next statement following the loop. This mechanism ensures that at least one iteration occurs.
Comparison with Other Loops
-
Do While vs. While Loop: The while loop checks the condition first and may skip execution entirely if the condition is false from the beginning. In contrast, the do while loop guarantees that the body of the loop runs at least once.
-
Do While vs. For Loop: While the for loop typically iterates over a fixed number of times or elements, the do while loop is more flexible for scenarios requiring repeated execution contingent on a condition.
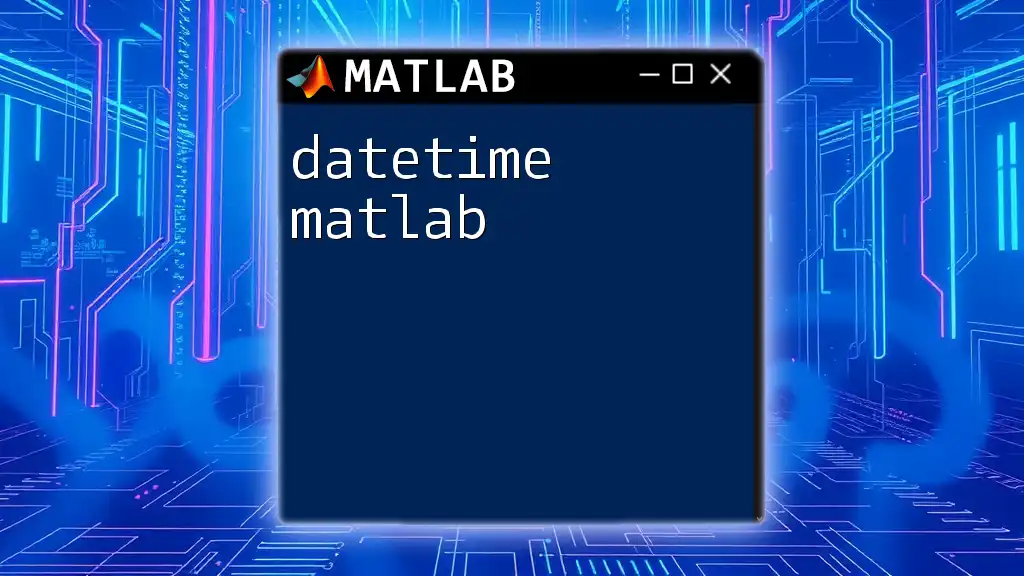
When to Use a Do While Loop
Use Cases
The do while loop shines in situations where the initial execution must take place regardless of the condition—such as prompting user input until a valid response is obtained. This makes it particularly useful for input validation tasks or scenarios where you need to display information before soliciting conditions to continue.
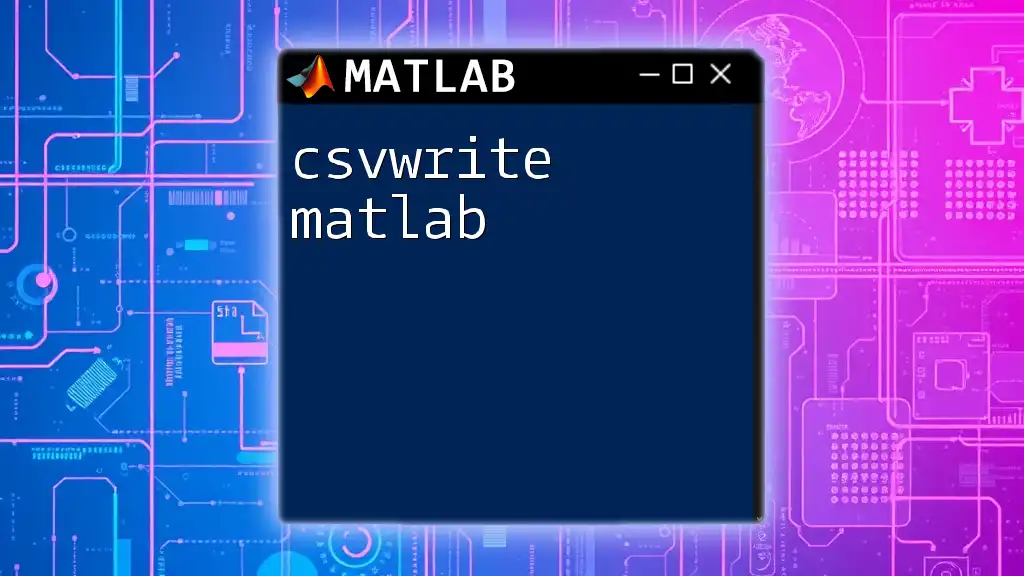
Code Snippets and Examples of Do While Loop in MATLAB
Basic Example
Let’s examine a simple example that demonstrates the behavior of a do while loop:
count = 0;
do
count = count + 1;
disp(count);
while count < 5;
In this snippet, `count` starts at 0. The code inside the `do` block will execute, incrementing `count` by 1 and displaying it. This process continues until `count` reaches 5. The expected output will be:
1
2
3
4
5
Real-World Example: User Input Validation
Another practical application is using a do while loop for user input validation. Here’s a code snippet that continuously prompts the user for a valid number until they provide one:
isValid = false;
do
userInput = input('Enter a number between 1 and 10: ');
isValid = (userInput >= 1 && userInput <= 10);
while ~isValid;
disp(['You entered: ', num2str(userInput)]);
In this example, the user will be prompted to enter a number. The loop will continue until the user inputs a valid number between 1 and 10, ensuring that the program can handle unexpected input safely.
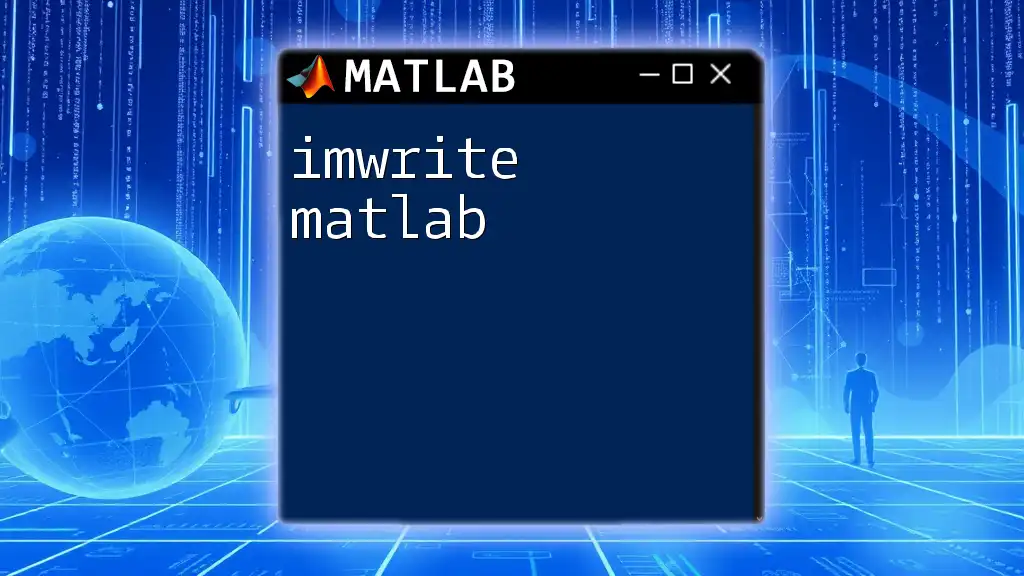
Common Mistakes and Pitfalls
Infinite Loops
One common mistake when using a do while loop is creating an infinite loop. This can occur if the condition is not updated within the loop or if the condition is always true. Developers must ensure their loop logic updates the condition appropriately to avoid being stuck in an indefinite cycle.
Overuse of Do While Loops
Additionally, there can be a tendency to overuse do while loops in scenarios where a while or for loop would be more efficient or readable. Always assess whether the logic truly benefits from the guaranteed execution that a do while loop provides.
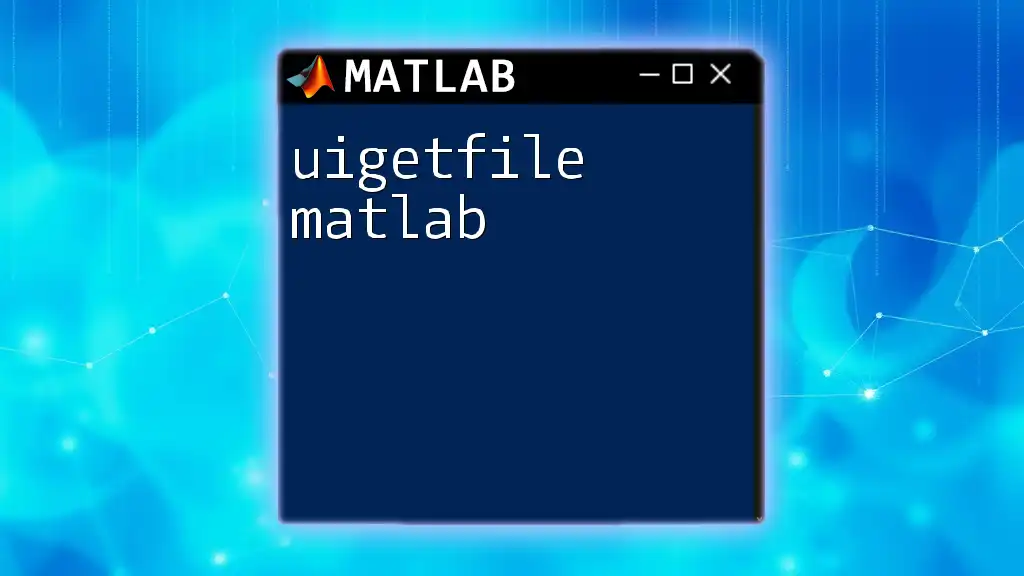
Debugging Do While Loops
Best Practices for Debugging
Debugging do while loops may require careful attention to how the condition is initialized and updated. It is best practice to:
- Begin with a clear understanding of what condition is evaluated.
- Ensure all initialization occurs before the loop starts.
Using MATLAB’s Debugging Tools
MATLAB comes with powerful debugging tools. Make use of breakpoints to pause execution and inspect variable states before and after the do while loop executes. Additionally, the command window allows you to evaluate expressions and monitor variable changes dynamically.
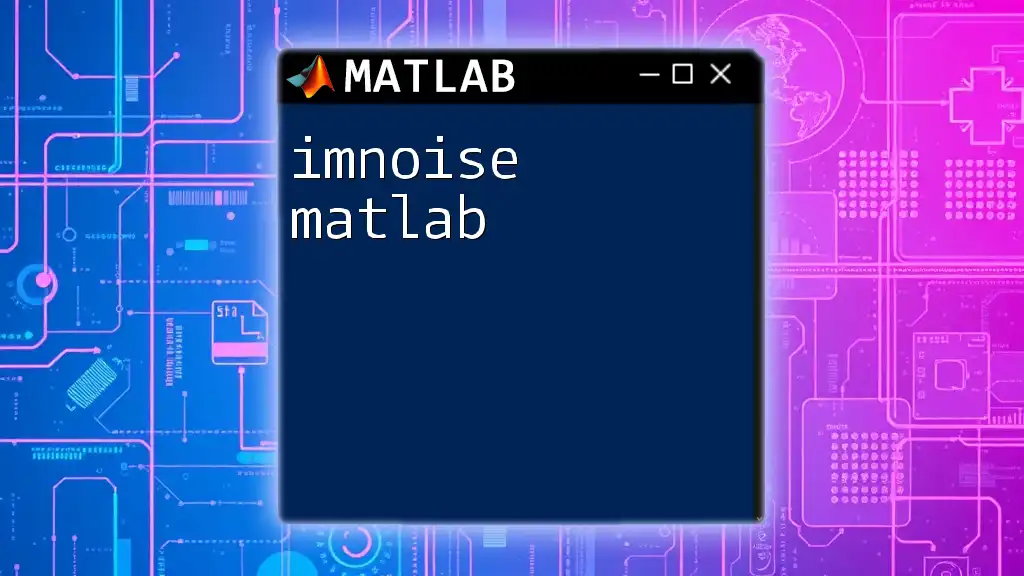
Conclusion
In summary, the do while loop in MATLAB is a valuable programming structure that enables repeated execution of code based on a condition, while ensuring that the loop body runs at least once. It is particularly useful for user input validation and scenarios where an initial execution is crucial. By understanding its syntax, flow, and potential pitfalls, you can effectively implement do while loops in your MATLAB programs.
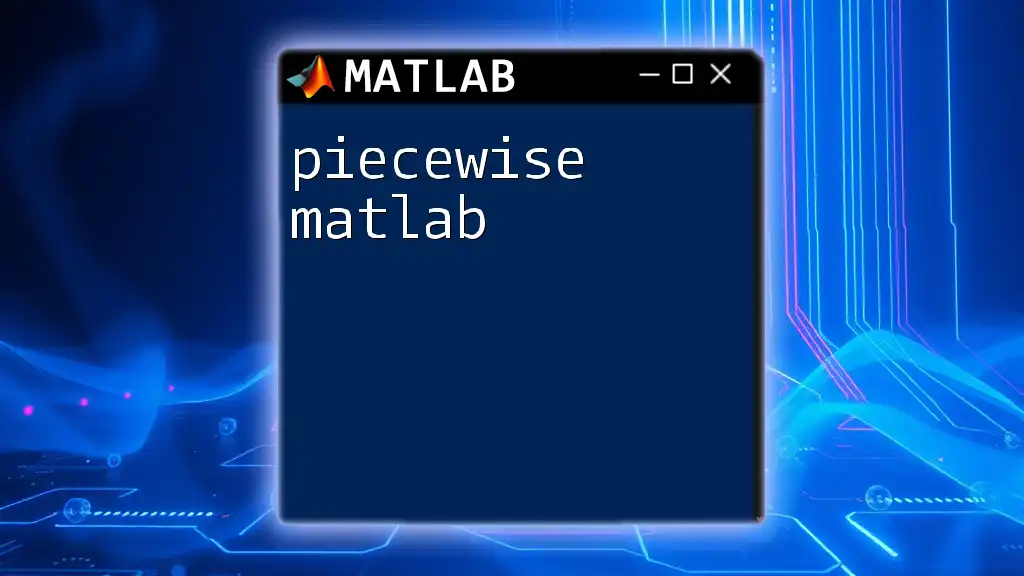
Additional Resources
To further your knowledge, you can explore the official MATLAB documentation for more details on loop structures and syntax. Engaging in online MATLAB community forums can also provide insights and additional support as you refine your programming skills.