Anonymous functions in MATLAB are a concise way to create functions without having to define them in a separate file, allowing you to define simple one-line expressions using the `@` symbol.
Here's a code snippet demonstrating the creation and use of an anonymous function in MATLAB:
square = @(x) x.^2; % Define anonymous function to square a number
result = square(5); % Call the anonymous function with input 5
disp(result); % Display the result
What are Anonymous Functions?
In MATLAB, anonymous functions are a powerful feature that allows you to define a function in a single line without the need to create an entire function file. They are particularly useful for short, simple functions that you may only use once or twice within a script or file. Instead of cluttering your code with lengthy function definitions, anonymous functions provide a concise and efficient alternative.
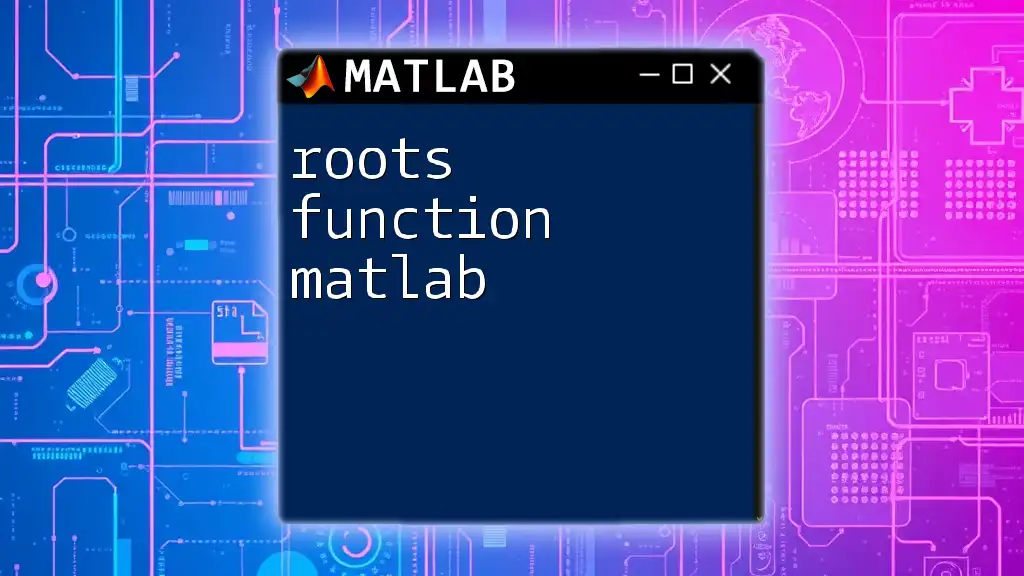
Benefits of Using Anonymous Functions
Using anonymous functions in MATLAB comes with several advantages:
-
Conciseness: They eliminate the boilerplate of function files, letting you define a function inline with minimal syntax.
-
Ease of Use: They allow you to quickly define functions that can be used directly in calls to other functions, such as `arrayfun`, `cellfun`, etc.
-
Efficiency: They are optimized for simple operations, making them ideal for computations that require minimal logic or complexity.
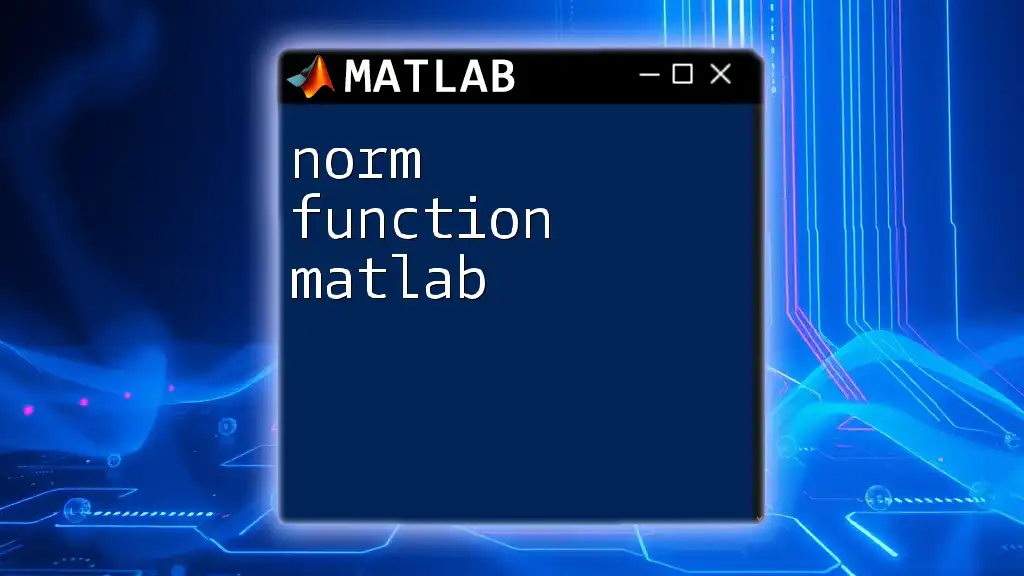
Syntax of Anonymous Functions
Basic Syntax
The fundamental syntax for creating an anonymous function in MATLAB is:
f = @(arglist) expression
- `f` is the function handle/name.
- `arglist` indicates the arguments the function can accept.
- `expression` is the operation performed on the input.
For example, if you want to create a function that calculates the square of a number, you would write:
square = @(x) x^2;
Components Breakdown
- The `@` symbol signifies the start of the anonymous function.
- The argument list can include multiple variables. For example, to create a function that sums two numbers, you would write:
add = @(x, y) x + y;
Here, `x` and `y` are the input variables, and `x + y` is the expression that evaluates when the function is called.
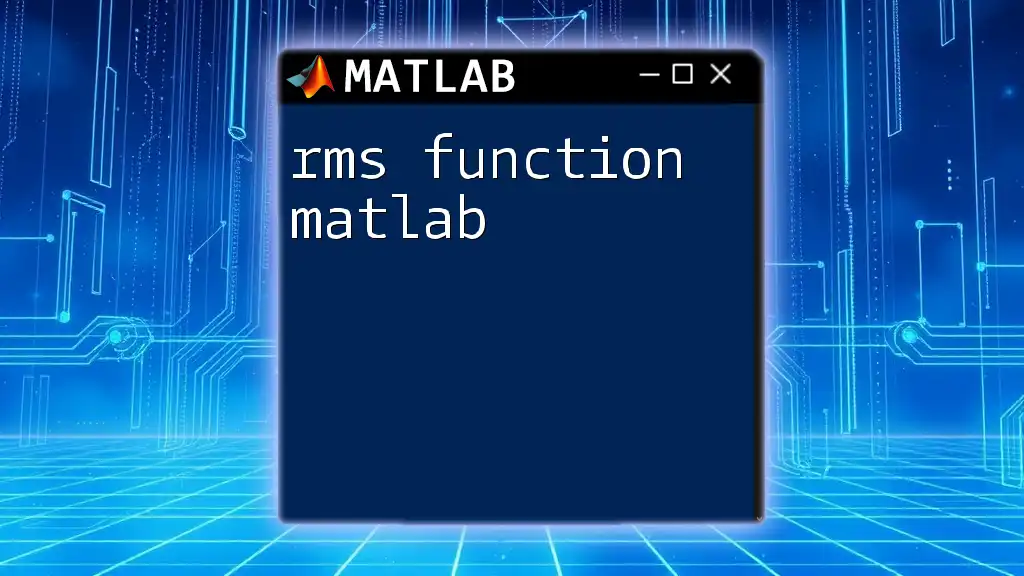
Creating Simple Anonymous Functions
Single Argument Functions
An anonymous function can handle single arguments easily. For instance, you might want to create a function that computes the cube of a number:
cube = @(x) x^3;
To execute this function, simply call it with a value:
result = cube(3); % result will be 27
Multiple Argument Functions
Anonymous functions can also accept multiple arguments. For example, to compute the sum of two numbers, you can define it as follows:
add = @(x, y) x + y;
You can call it like this:
total = add(5, 7); % total will be 12
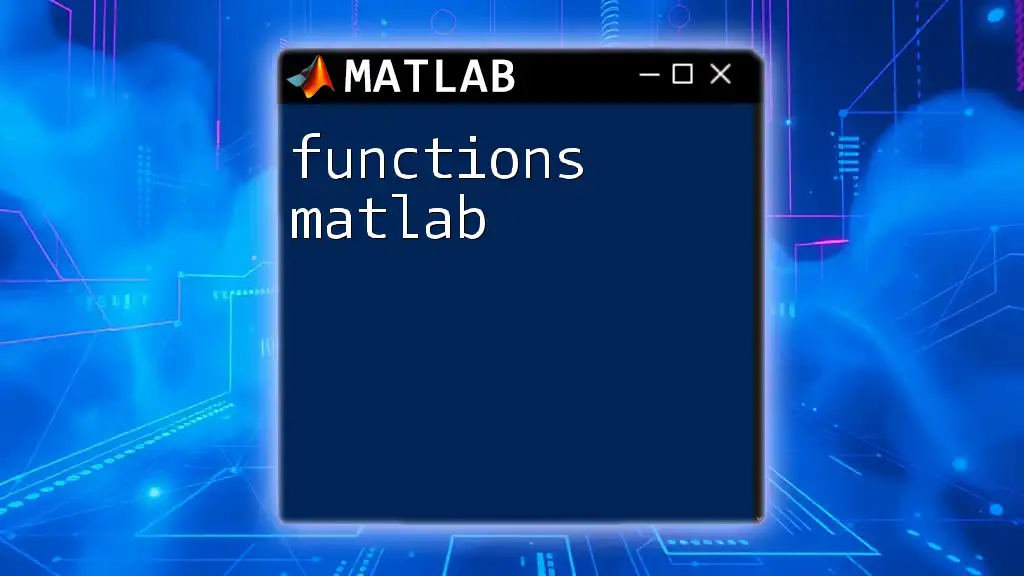
Practical Applications of Anonymous Functions
Using with Built-in Functions
Anonymous functions shine when used with MATLAB's built-in functions such as `arrayfun`, `cellfun`, and `structfun`. For example, suppose you want to apply a square function to an array:
arr = [1, 2, 3, 4];
squared_array = arrayfun(@(x) x^2, arr);
In this case, `arrayfun` applies the anonymous function to each element of the array `arr`, returning an array of squared values.
Combining with Other Data Types
You can also use anonymous functions effectively with cell arrays and structures. For instance, if you have a cell array containing numbers and you wish to compute their squares, you can employ an anonymous function along with `cellfun`:
cellArr = {1, 2, 3, 4};
squaredCellArr = cellfun(@(x) x^2, cellArr);
This transforms each cell's content into its square.
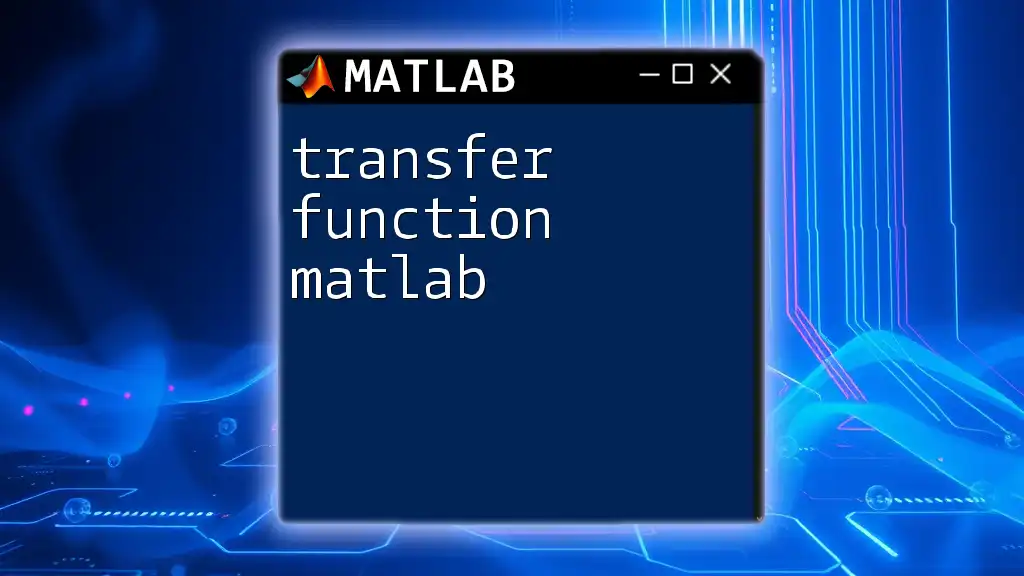
Advanced Concepts
Nested Anonymous Functions
Anonymous functions can be defined within other functions, allowing for interesting use cases. Here’s an example of an anonymous function returning another anonymous function:
multiplier = @(m) @(x) m * x;
double = multiplier(2);
When you call `double(5)`, it returns `10`, demonstrating how one function can dynamically generate another.
Varargin and Named Arguments
If you need flexibility in your function arguments, MATLAB's `varargin` allows you to define anonymous functions with variable-length input arguments. Consider the example:
add_varargin = @(varargin) sum(cell2mat(varargin));
This function will sum any number of inputs passed as arguments, illustrating the flexibility possible with anonymous functions.
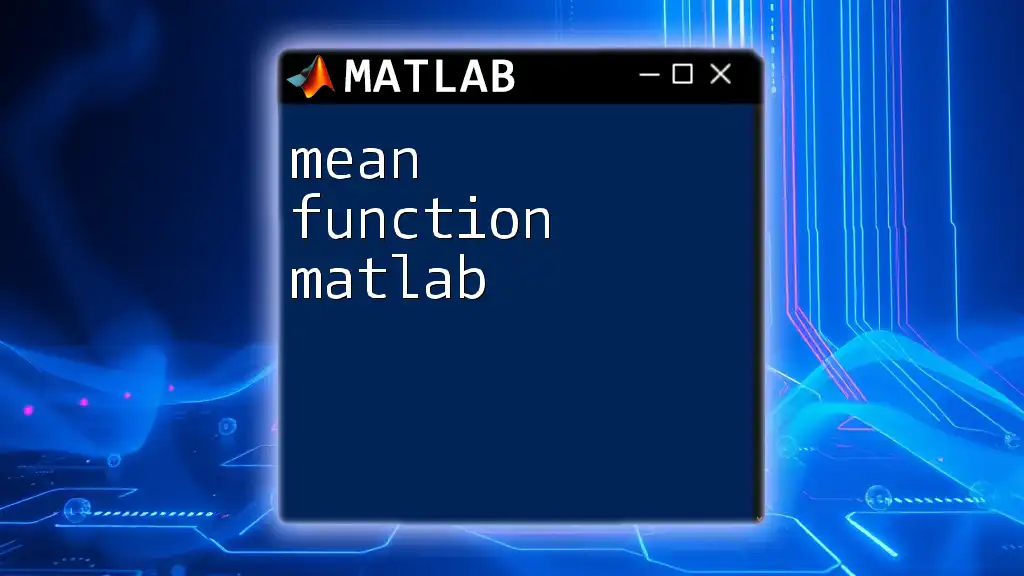
Comparing Anonymous Functions with Regular Functions
Understanding when to use anonymous functions over regular functions is essential. Regular functions are defined in M-files and become useful for more complex operations where clarity and reusability are priorities. In contrast, anonymous functions are best for short, simple computations required only a few times within a larger script.
Performance Analysis
It's worth noting that performance might vary. While anonymous functions can be more efficient for small, one-time operations, they can introduce overhead when used extensively in large computations. You can measure performance with `tic` and `toc` commands to decide which is best for your specific case.
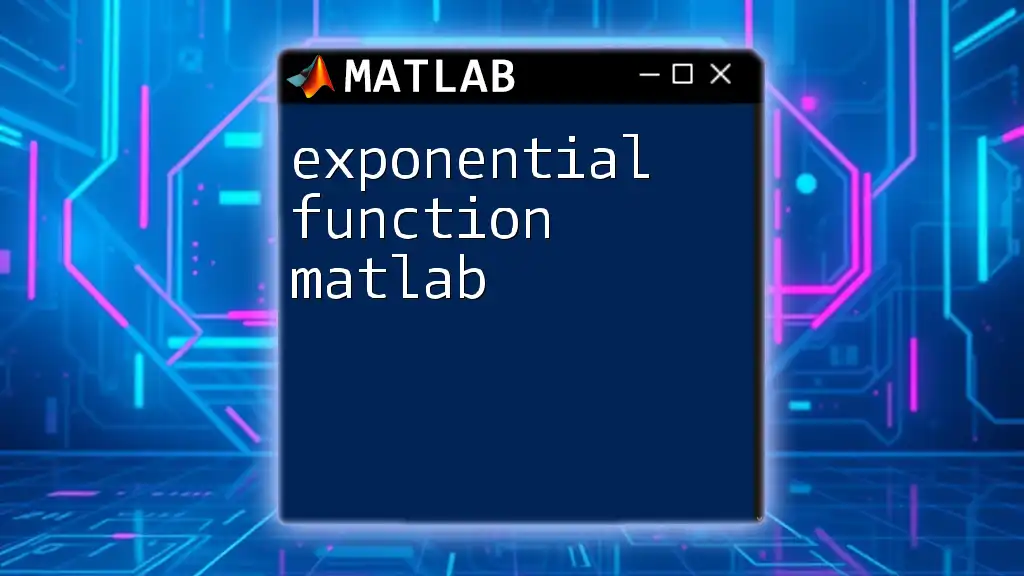
Common Pitfalls and Troubleshooting
When using anonymous functions, several common mistakes can occur:
-
Forgetting to use element-wise operations can result in unexpected behavior, especially in mathematical expressions.
-
Scope issues may arise regarding variables defined outside the anonymous function, leading to confusion.
Debugging Tips
If you encounter errors or unexpected outputs, use MATLAB’s debugging tools like breakpoints and the disp function to check variable states inside the anonymous function. This can help track down issues quickly.
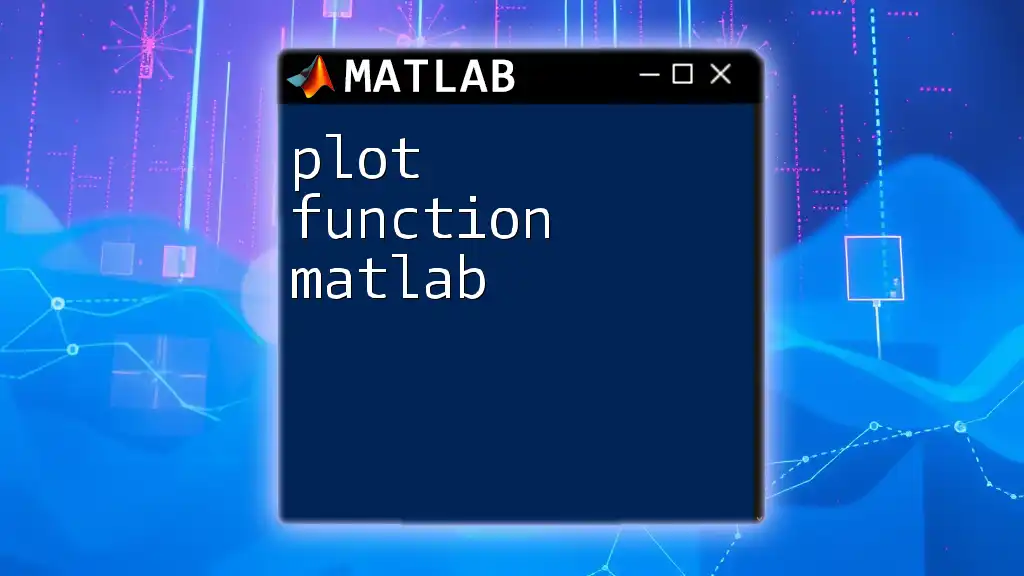
Conclusion
In summary, anonymous functions in MATLAB offer a compact and efficient way to create quick functions for immediate use, enhancing productivity and making code cleaner. By understanding their syntax, advantages, and applications, you can leverage them effectively in your MATLAB projects.
To get hands-on experience, practice creating your own anonymous functions and experiment with built-in MATLAB functions. For deeper learning, consider enrolling in specialized MATLAB courses or browsing additional resources that dive into more complex topics.