In MATLAB, axis labels enhance the clarity of your graphs by providing context to the data presented on the x-axis and y-axis. Here's how to set axis labels in your plot:
xlabel('X-Axis Label');
ylabel('Y-Axis Label');
Understanding Axis Labels in MATLAB
Axis labels serve a critical function in data visualization—they provide essential context for the data being presented. Without clear and informative labels, even the most sophisticated plots can be difficult to interpret.
What are Axis Labels?
Axis labels are textual annotations that indicate what each axis of a plot represents. They help the viewer to quickly understand the variables being plotted and their respective scales. Every time you create a plot, they are fundamental for conveying the data's meaning clearly.
Types of Plots that Require Axis Labels
Most types of plots, from simple 2D line graphs to more complex 3D distributions and scatter plots, require properly labeled axes. When each axis is clearly defined, viewers can more easily grasp the relationship between the plotted data points.
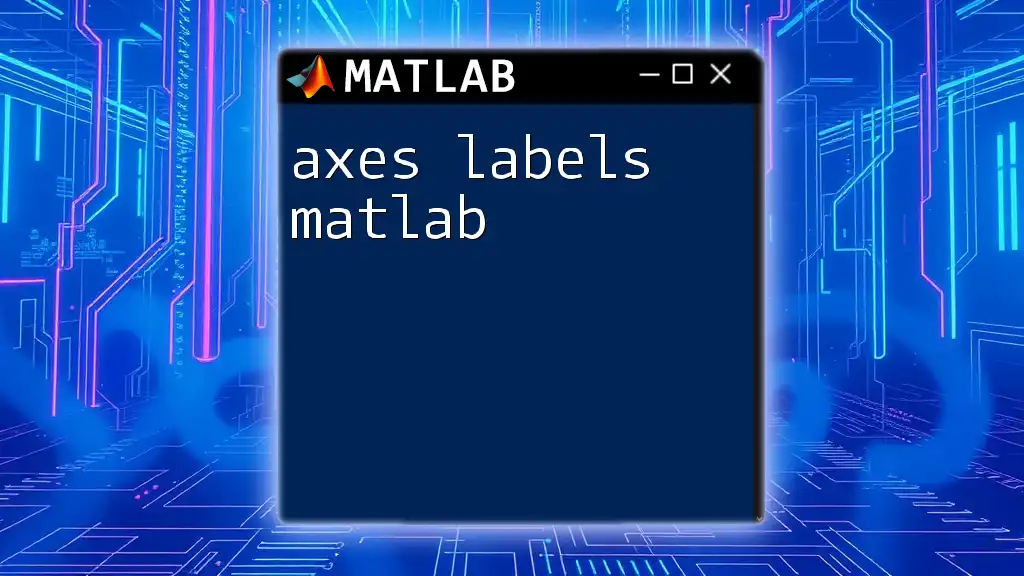
Setting Axis Labels in MATLAB
To create effective visualizations in MATLAB, you need to master the basic commands for adding axis labels. The primary functions you will use are `xlabel` and `ylabel`.
Basic Command for Axis Labels
Using `xlabel` and `ylabel` is straightforward. Below is a simple example demonstrating how to apply these functions:
% Basic example of using xlabel and ylabel
x = 1:10;
y = rand(1, 10);
plot(x, y);
xlabel('X Axis Label');
ylabel('Y Axis Label');
In this example, the x-axis is labeled "X Axis Label," and the y-axis is labeled "Y Axis Label." This is a fundamental step toward enhancing the interpretability of the plot.
Customizing Axis Labels
Once you have established basic labels, it’s time to customize them for better presentation.
Font Size and Style
You can change the font size and style to draw attention to your axis labels. Here's how you can modify these properties:
xlabel('X Axis Label', 'FontSize', 14, 'FontWeight', 'bold');
ylabel('Y Axis Label', 'FontSize', 14, 'FontWeight', 'italic');
In this code snippet, the x-axis label is bold and larger in size, while the y-axis label is italicized. Customizing font attributes can improve readability, particularly in presentations.
Color and Alignment
Color and alignment play a significant role in enhancing the visual appeal of your plots. You can change the color of your axis labels and align them as follows:
xlabel('X Axis', 'Color', 'blue', 'HorizontalAlignment', 'center');
This example sets the x-axis label to blue and centers it beneath the axis. Using colors that contrast nicely with the plot background can help ensure that your labels stand out.
Adding Titles and Legends
Using the `title` Function
A plot is often incomplete without a descriptive title. The `title` function allows you to add context to your visualization. This is how you do it:
title('Sample Plot Title', 'FontSize', 16);
Making the title larger not only enhances visibility but also asserts the significance of the plot being displayed.
Creating Legends
Legends are essential when you have multiple datasets to distinguish. The `legend` function works in conjunction with axis labels as shown below:
plot(x, y, 'DisplayName', 'Random Data');
legend show;
In this instance, the `DisplayName` property assigns a name to the plotted data, which will appear in the legend.
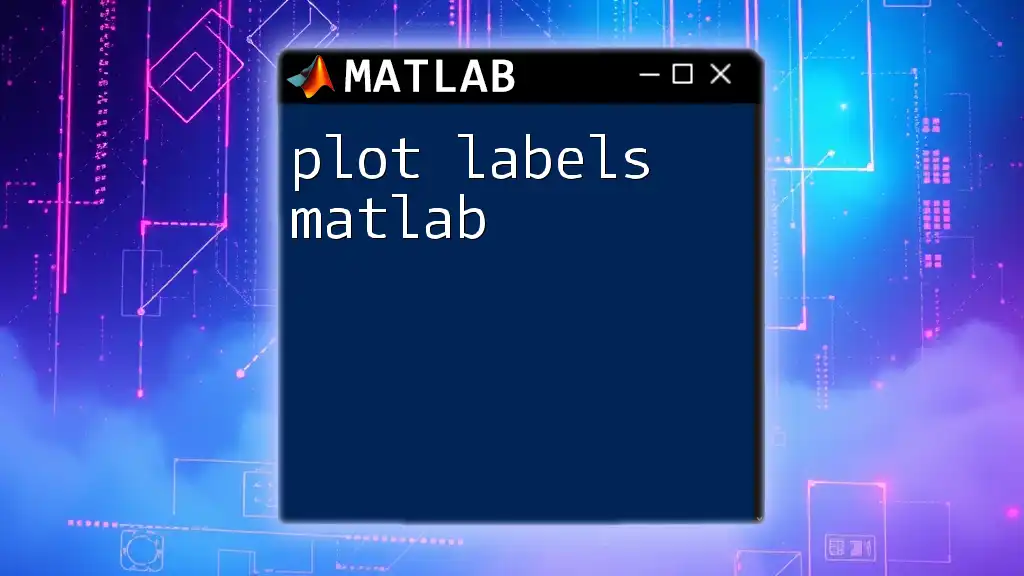
Advanced Customization of Axis Labels
Using LaTeX for Professional Formatting
For more advanced users, incorporating LaTeX into your axis labels adds a level of professionalism, particularly in scientific and academic contexts. Here's how you can format a label with LaTeX:
xlabel('$\theta$ (degrees)', 'Interpreter', 'latex', 'FontSize', 12);
Using LaTeX allows you to include complex mathematical expressions that standard text cannot convey.
Multi-Axis Labeling
When dealing with data requiring more than one response variable, MATLAB offers functionalities like `yyaxis` for dual-axis plots.
yyaxis left;
plot(x, y);
ylabel('Left Y Axis Label');
yyaxis right;
plot(x, rand(1, 10));
ylabel('Right Y Axis Label');
In this example, two different y-axes are created, enabling you to compare two datasets on the same x-axis without confusion.
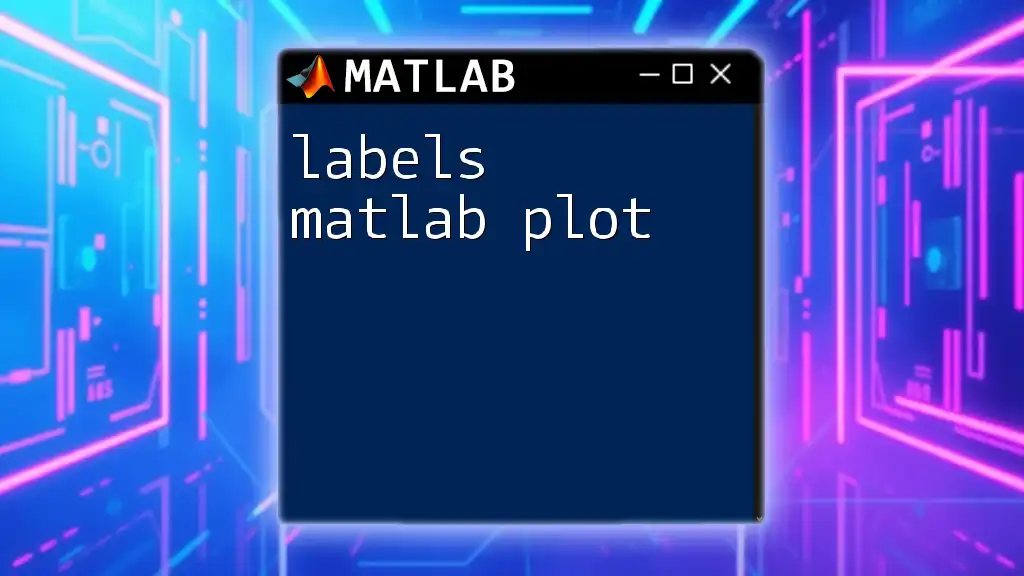
Best Practices for Axis Labels
Consistency in Labels
Maintaining consistency across multiple plots is crucial for clarity. Stick to similar terminologies, font sizes, and styles throughout your visualizations to build familiarity and understanding.
Clarity and Readability
Always aim for clarity in your axis labels. Use terms that accurately reflect the data represented. Avoid jargon that might confuse the audience.
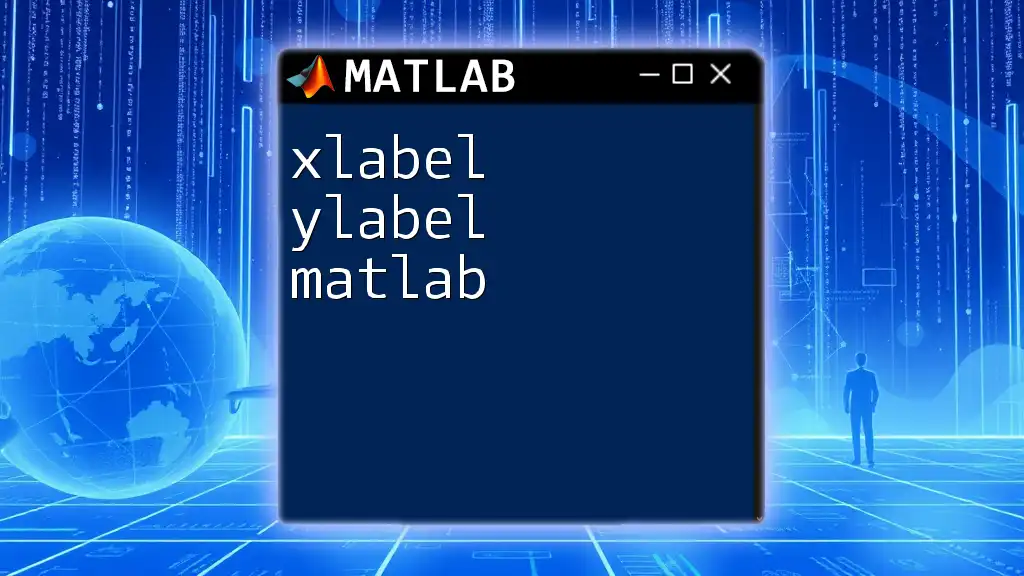
Common Issues with Axis Labels
Labels Overlapping with Data
One challenge users often face is overlapping labels and data points. Fortunately, there are strategies to avoid this issue, such as rotating the axis labels:
xtickangle(45);
This code snippet changes the angle of the x-axis ticks, improving clarity and avoiding overlap.
Labels Not Displaying Correctly
Sometimes labels might not appear correctly due to visibility issues related to axis limits. Always check to ensure that your labels fall within the defined plot boundaries to ensure they display as intended.
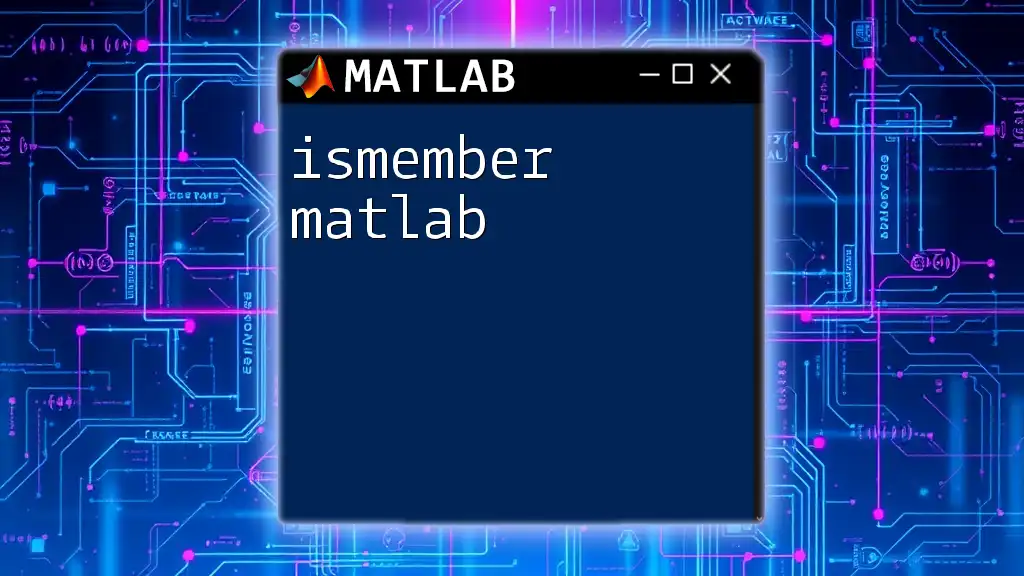
Conclusion
In summary, axis labels in MATLAB are indispensable for creating informative plots. They enhance clarity and allow viewers to quickly interpret the data being presented.
To develop and refine your MATLAB plotting skills, practice implementing the commands and concepts discussed. As always, don’t hesitate to share your experiences with axis labels in your own MATLAB projects; engaging with the community can lead to new insights and techniques.
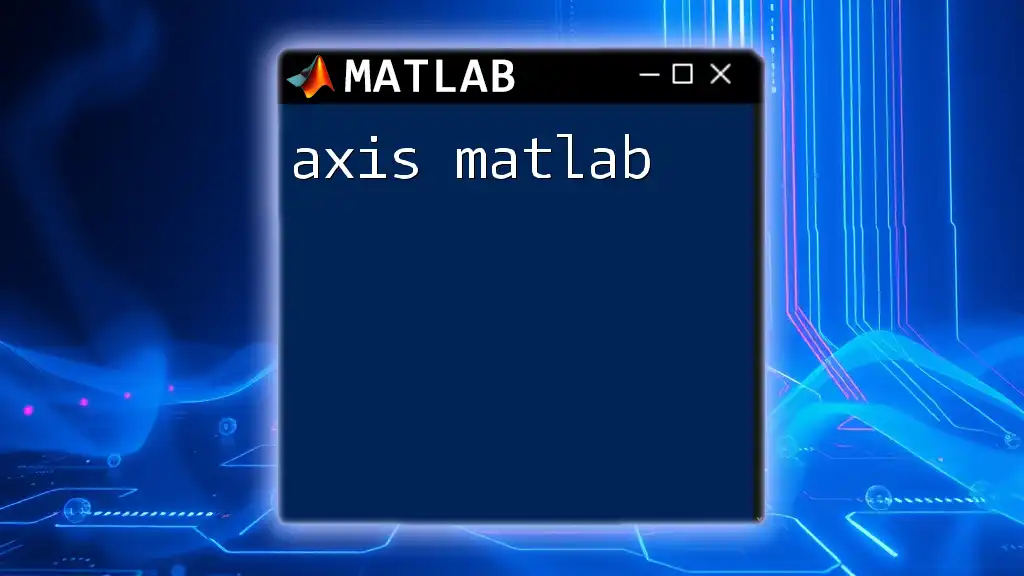
Additional Resources
For further reading, you can explore the official MATLAB documentation focusing on axis properties and customization options. Engaging with online MATLAB communities can also provide valuable support and additional insights as you continue your learning journey.