A surface plot in MATLAB visualizes three-dimensional data using a grid of points to represent the relationship between variables, allowing for a clear display of surfaces in a 3D space.
Here’s a simple example of how to create a surface plot in MATLAB:
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = sin(sqrt(X.^2 + Y.^2));
surf(X, Y, Z);
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
title('3D Surface Plot');
What is a Surface Plot?
A surface plot is a three-dimensional graphical representation of data points in a coordinate system, where values are plotted on the X, Y, and Z axes. Surface plots visualize relationships between three variables, providing insights into the data that might be lost in two-dimensional representations. They are particularly useful for analyzing surfaces, such as elevation data in geographic information systems or response surfaces in optimization problems. Surface plots are widely used in fields like engineering, physics, and data science to illustrate complex datasets and trends.
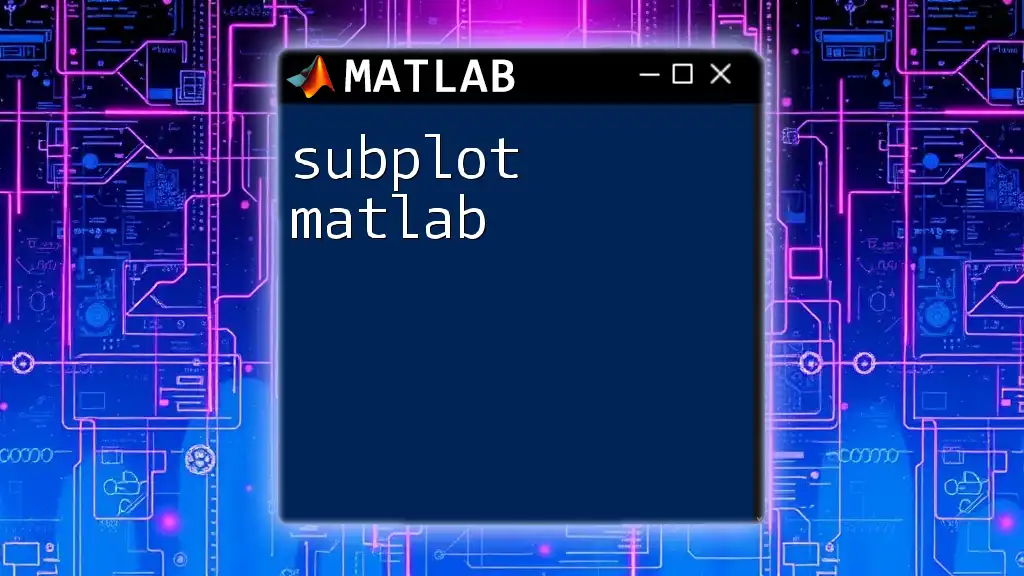
Getting Started with MATLAB
Before diving into surface plots, ensure that you have MATLAB installed on your computer. Visit the official MathWorks website to download and install MATLAB if you haven't done so already. Once installed, open MATLAB and familiarize yourself with the environment, including the Command Window, Workspace, and Editor.
Preparing Your Data
To create a surface plot in MATLAB, you need to prepare your data in matrices. Specifically, you must provide three matrices: `X`, `Y`, and `Z`, where each entry corresponds to a point in three-dimensional space.
Data Requirements
- The `X` and `Y` matrices define the grid over which the surface will be created.
- The `Z` matrix contains the values at each grid point, which will be plotted as the height of the surface.
It is essential to ensure that your `X` and `Y` matrices are consistent with one another and properly gridded, often created using the `meshgrid` function.
Creating Basic Surface Plots
To create a basic surface plot, you can use MATLAB's built-in `surf` function, which takes the `X`, `Y`, and `Z` matrices as inputs.
Code Snippet
[X, Y] = meshgrid(-5:0.1:5, -5:0.1:5);
Z = sin(sqrt(X.^2 + Y.^2));
surf(X, Y, Z);
In this code:
- The `meshgrid` function generates the grid for `X` and `Y`.
- The Z-values are calculated using the sine function, creating a wavy surface.
- The `surf` function then visualizes this data as a three-dimensional surface plot.
The resulting visualization will reveal a fluid, wave-like surface that represents the function defined over the grid.
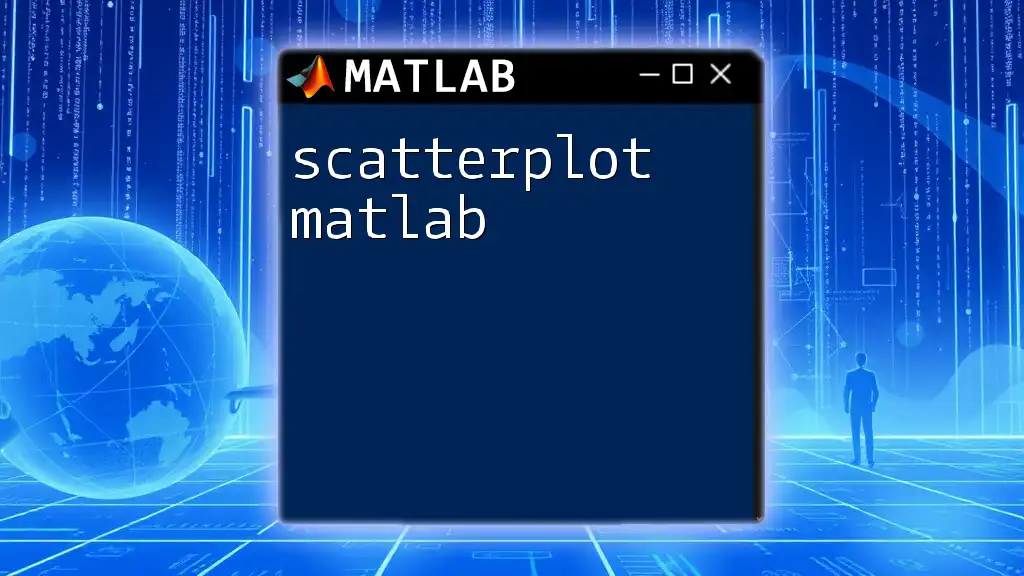
Customizing Surface Plots
Color Maps
Color maps enhance the visual appeal and communicative power of surface plots. MATLAB provides several built-in color maps, allowing you to choose one that best represents your data.
You can apply a color map using the `colormap` function. For example:
Code Snippet
colormap(jet);
This command applies the "jet" color map, transitioning from blue to red, indicating low to high values. Choosing the right color map can help convey meaning and draw attention to areas of interest in your plot.
Modifying Axes
Clear labeling of axes is crucial for interpretation. You can add labels and titles to your surface plot using the `xlabel`, `ylabel`, `zlabel`, and `title` functions.
Code Snippet
xlabel('X-axis Label');
ylabel('Y-axis Label');
zlabel('Z-axis Label');
title('My Surface Plot');
This practice helps your audience understand what the axes represent and gives context to the data presented.
Surface Properties
Lighting and Shading
Adding lighting to a surface plot can significantly improve its visual quality and depth perception. MATLAB allows you to adjust the lighting with the `camlight` and `lighting` functions.
Code Snippet
lighting gouraud; % Sets the shading method
camlight left; % Adjusts the camera light position
These commands enhance the surface's illumination, making peaks and valleys more pronounced, which is vital for visual analysis of the data.
Transparency and Edge Color
You can control the transparency of the surface and modify its edge colors to enhance visual clarity. Adjusting surface transparency is essential when overlaying multiple surfaces.
Code Snippet
surf(X, Y, Z, 'FaceAlpha', 0.5, 'EdgeColor', 'none');
In this snippet:
- `'FaceAlpha'` adjusts the surface's transparency, allowing for layered visualizations.
- `'EdgeColor'` set to 'none' eliminates edge coloring, making the plot look cleaner and more aesthetically pleasing.
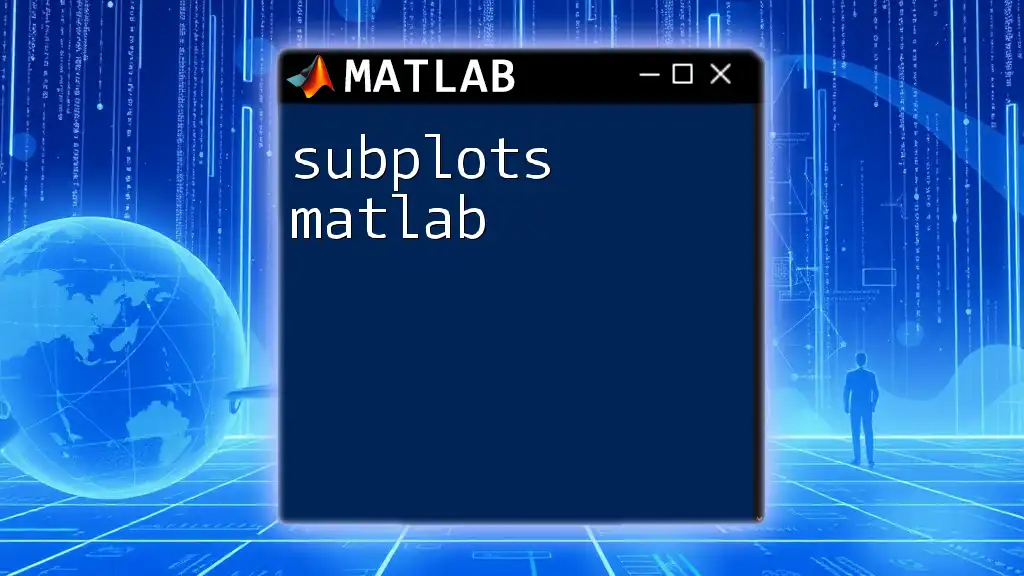
Advanced Surface Plot Techniques
Overlaying Multiple Surface Plots
When analyzing multiple datasets simultaneously, overlaying surface plots can provide valuable insights. You can use the `hold on;` command to plot multiple surfaces within the same figure.
Code Snippet
hold on;
Z2 = cos(sqrt(X.^2 + Y.^2)); % Another function for comparison
surf(X, Y, Z2, 'FaceAlpha', 0.3); % Overlay with transparency
hold off;
In this example, the cosine function’s surface is added to the existing plot, providing a comparative visualization that allows you to see the interaction between the two datasets.
Interactive Surface Plots
MATLAB also supports interactive surface plots that allow users to explore data more dynamically. Using the `rotate3d` feature, you can easily manipulate the view angle of the surface.
Simply type the following command in the command window:
rotate3d on;
With interactive capabilities, users can zoom in, rotate, and examine surfaces from various perspectives, leading to deeper insights.
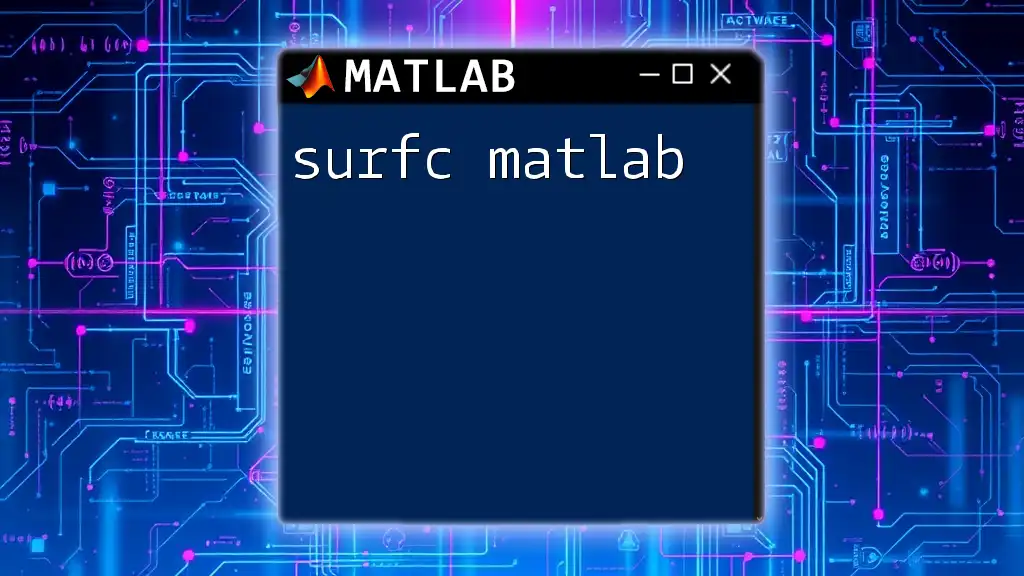
Exporting Surface Plots
Once you have created your surface plot and customized it to your preference, you may want to save it for publications or presentations. MATLAB supports various formats for saving plots, including PNG, JPEG, and EPS.
To export your plot to a file format of your choice, use the `saveas` command. For example:
Code Snippet
saveas(gcf, 'my_surface_plot.png');
This command saves the current figure as a PNG file, which can then be easily integrated into reports or shared with colleagues.
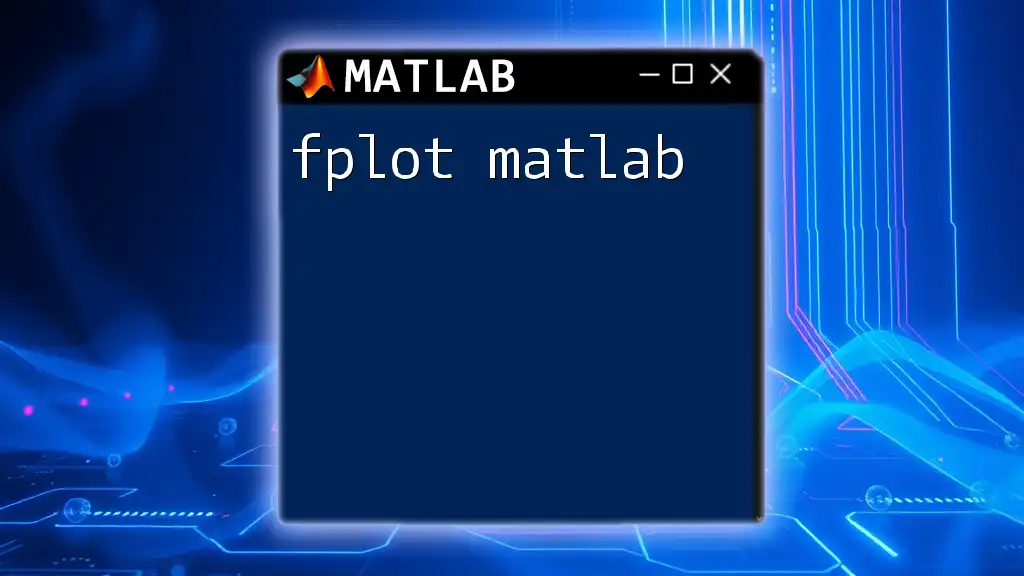
Common Issues and Troubleshooting
Creating surface plots can sometimes lead to common issues, such as:
- Inputs having mismatched dimensions.
- Poor visualization due to inappropriate scaling.
- Inability to visualize a clear surface due to insufficient data points.
To troubleshoot these problems, always ensure that your input matrices are well-defined and match in size. Additionally, adjust the viewing angle and scaling to prevent distortion.
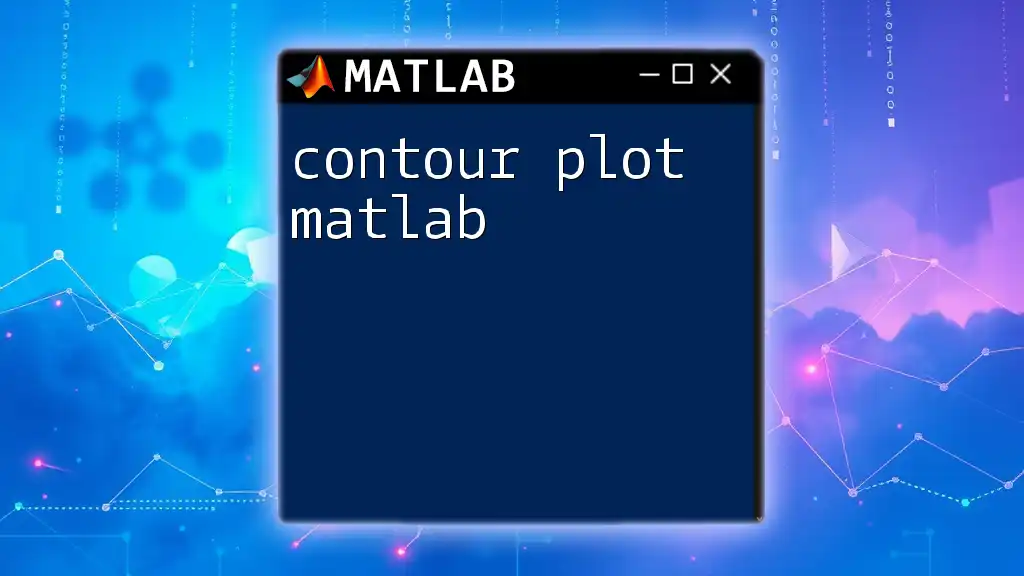
Conclusion
Surface plots serve as powerful tools for visualizing three-dimensional data in MATLAB. By utilizing the various features and customizations discussed, you can create informative and engaging visualizations that can effectively communicate complex data relationships. Embrace the practice of creating and refining surface plots to enhance your data analysis capabilities, and explore the vast potential of this versatile plotting technique in MATLAB.