The Butterworth filter in MATLAB is designed to have a flat frequency response in the passband, and you can easily create one using the `butter` function for designing filters.
Here's a simple example to create a second-order low-pass Butterworth filter:
% Define the filter specifications
order = 2; % Filter order
cutoff_freq = 0.2; % Normalized cutoff frequency (0 to 1, where 1 corresponds to Nyquist frequency)
% Design the Butterworth filter
[b, a] = butter(order, cutoff_freq);
% Apply the filter to a signal (example signal x)
filtered_signal = filter(b, a, x);
What is a Butterworth Filter?
A Butterworth filter is a type of signal processing filter designed to have a maximally flat frequency response in the passband. This means that within the passband, the filter does not have ripples or variations in gain; thus, it delivers a smooth response. The distinguishing feature of the Butterworth filter is its ability to maintain a level of flatness in the frequency response, ensuring minimal distortion of signals that pass through it.
Why Use a Butterworth Filter?
One of the primary reasons to use a Butterworth filter over other types of filters is its smoothness in the passband, which makes it particularly advantageous for applications where signal integrity is crucial. Common applications include audio processing, communications, and various fields in engineering.
Other filters, such as Chebyshev or Elliptic filters, may have sharper transitions between passband and stopband, but they compromise on smoothness in the passband, making Butterworth a preferred choice in many cases.
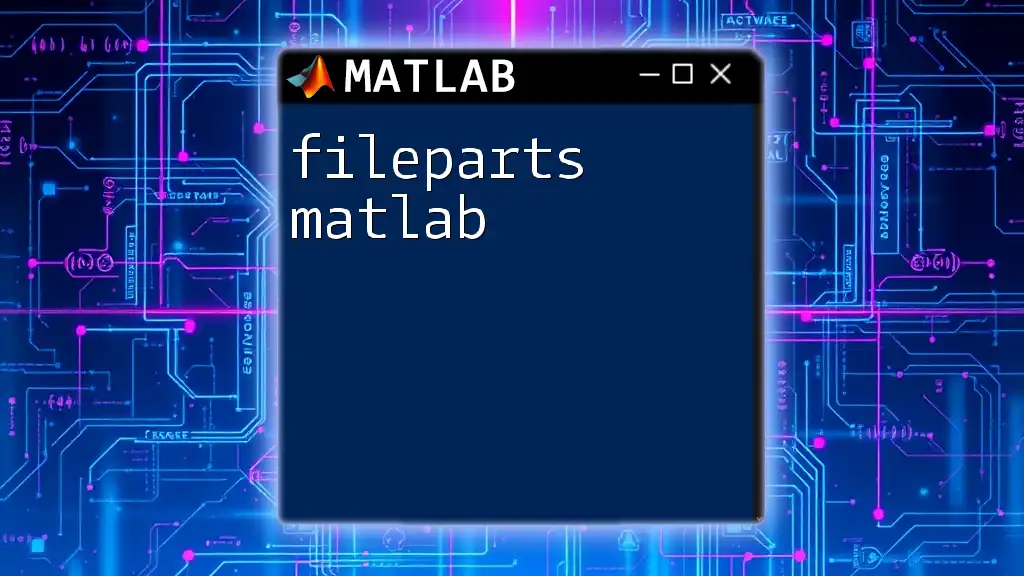
Understanding the Basics of Filter Design
Key Terms in Filter Design
To fully grasp the concept of Butterworth filters, it's essential to understand several key terms in filter design:
- Passband: The range of frequencies that the filter allows to pass with minimal attenuation.
- Stopband: The range of frequencies that are significantly attenuated by the filter.
- Cutoff Frequency: The frequency at which the filter begins to attenuate input signals.
- Filter Order: The degree of the filter, determining how sharply it differentiates between the passband and stopband. A higher order results in a steeper roll-off.
Types of Filters
Filters can be classified into various types, including:
- Low-pass filters: Allow signals below a certain frequency to pass while attenuating higher frequencies.
- High-pass filters: Allow signals above a certain frequency to pass while attenuating lower frequencies.
- Band-pass filters: Allow signals within a certain frequency range to pass while attenuating signals outside this range.
- Band-stop filters: Attenuate signals within a specific frequency range while allowing signals outside that range to pass.
Understanding these types allows for a more informed choice when it comes to filter implementation, particularly when comparing them against the characteristics of a Butterworth filter.
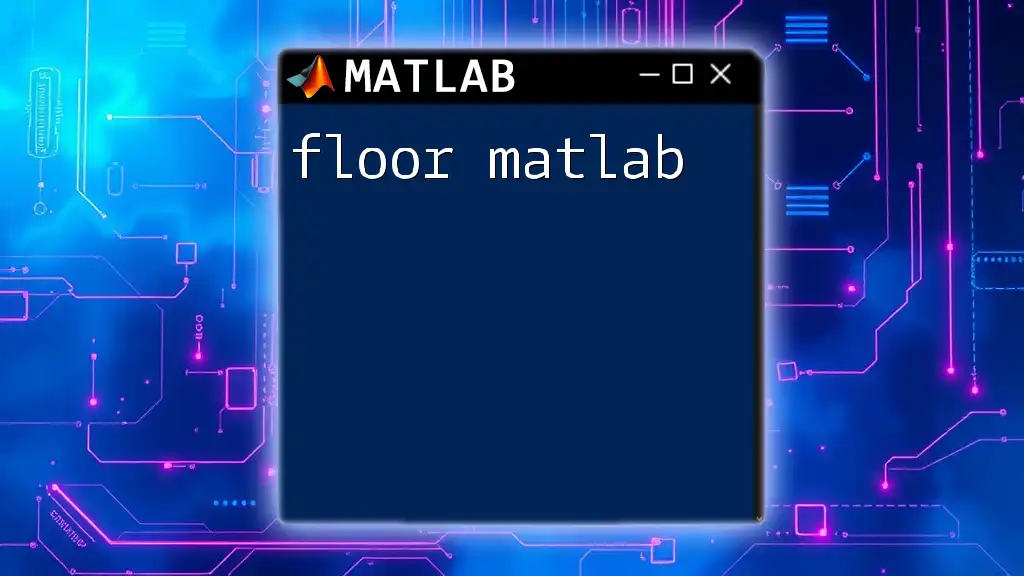
MATLAB and its Role in Filter Design
Introduction to MATLAB
MATLAB (Matrix Laboratory) is a high-performance programming language that is essential for mathematical computations, including those involving signal processing. It provides built-in functions and toolboxes for designing and implementing filters like the Butterworth filter. The extensive libraries and graphical capabilities of MATLAB make it an ideal choice for practically implementing filter designs.
Getting Started with MATLAB
For those new to MATLAB, starting with basic commands is crucial. Familiarize yourself with fundamental operations like matrix manipulation, plotting, and using built-in functions. Getting your MATLAB environment set up, including installing toolboxes related to signal processing, can significantly enhance your filter design experience.
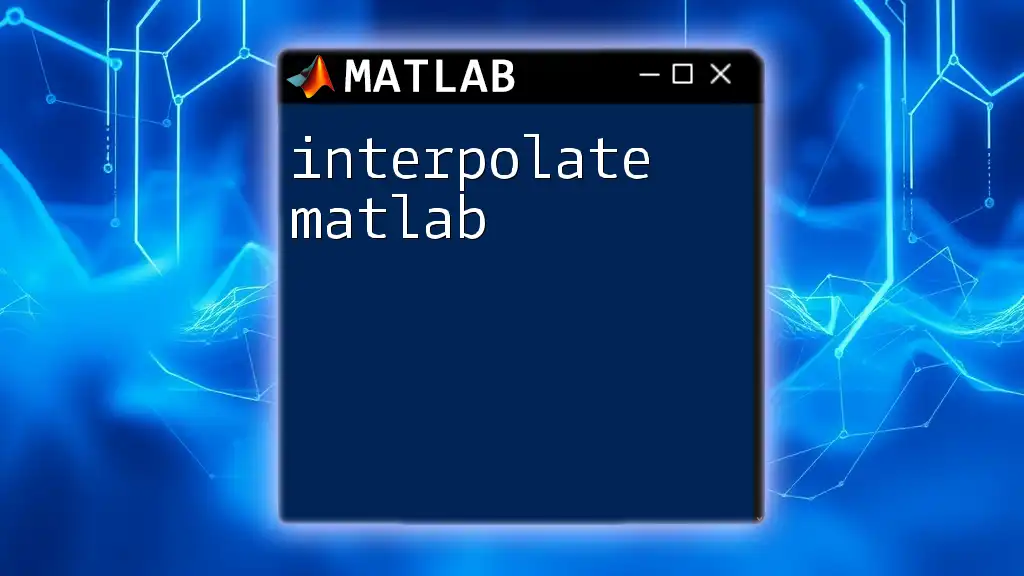
Implementing a Butterworth Filter in MATLAB
Designing a Butterworth Filter
To create a Butterworth filter, follow these steps:
- Specify Filter Parameters: Define the filter order and cutoff frequency based on the requirements of your signal.
- Use the `butter()` Function: MATLAB's built-in `butter()` function is specifically designed for creating Butterworth filters.
Here is how you can implement this in MATLAB:
% Define parameters
fs = 1000; % Sampling frequency in Hz
fc = 100; % Cut-off frequency in Hz
order = 4; % Filter order
% Design the Butterworth filter
[b, a] = butter(order, fc/(fs/2), 'low');
In the above code, `fs` is the sampling frequency, `fc` represents the cutoff frequency, and `order` indicates the filter order. The `butter()` function returns the filter coefficients in vector `b` (numerator) and `a` (denominator).
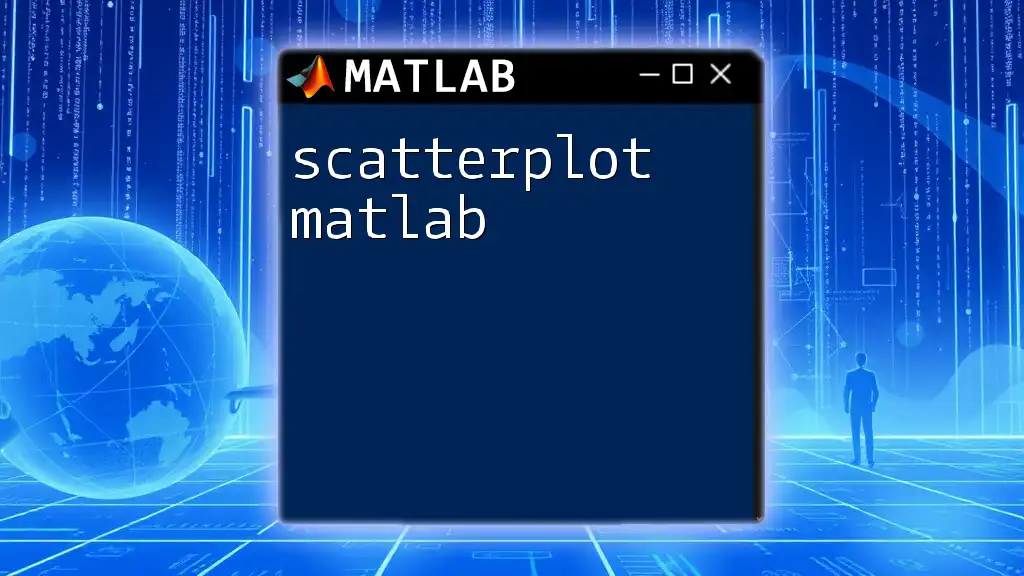
Analyzing Frequency Response
Understanding Frequency Response
Frequency response is crucial for understanding how a filter behaves. It describes how different frequencies are attenuated or amplified by the filter. Users often differentiate between impulse response and frequency response when analyzing filters, as each provides unique insights into filter behavior.
Using MATLAB to Analyze Frequency Response
To examine the frequency response of your Butterworth filter, use the `freqz()` function, which plots the frequency response of a digital filter.
Here's an example of how to visualize the filter response:
% Visualize frequency response
[h, f] = freqz(b, a);
plot(f*fs/(2*pi), abs(h));
title('Frequency Response of the Butterworth Filter');
xlabel('Frequency (Hz)');
ylabel('Magnitude');
In this code, `h` stores the complex frequency response, while `f` contains the corresponding frequencies. The `plot()` function visualizes how the magnitude of the filter response varies with frequency.
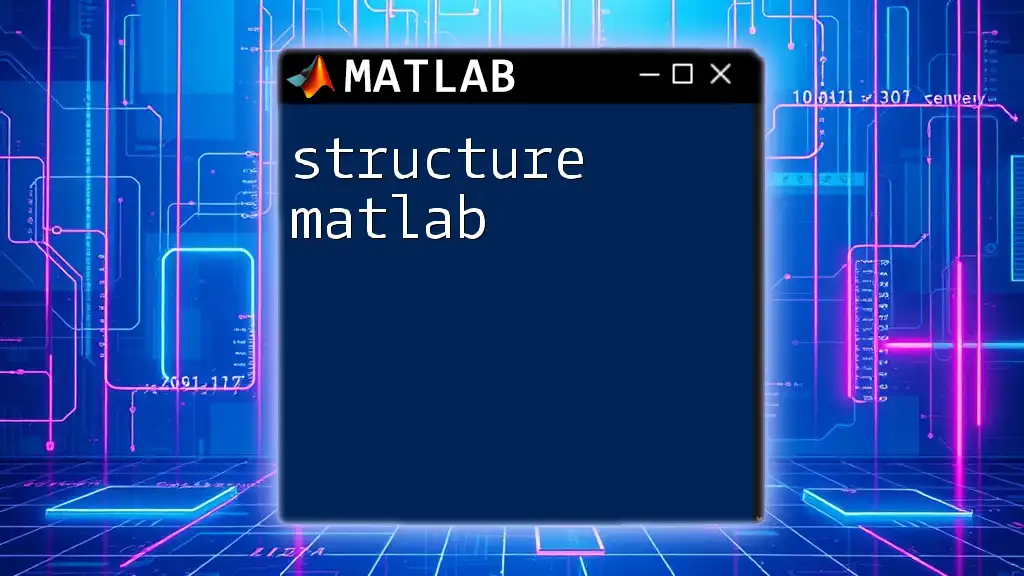
Implementing the Filter on Sample Data
Generating Sample Data
Creating sample signals is an important step to test your Butterworth filter’s effectiveness. You can easily generate a noisy signal using sine waves and random noise.
Applying the Butterworth Filter to the Sample Data
To filter the generated signal, you will utilize the `filter()` function, which applies the filter coefficients to your input signal.
Here’s an example code snippet:
% Generate sample data
t = 0:1/fs:1-1/fs; % Time vector
signal = sin(2*pi*50*t) + 0.5*randn(size(t)); % Signal with noise
% Filter the signal
filtered_signal = filter(b, a, signal);
This code generates a noisy sine wave and then applies the Butterworth filter to extract the underlying signal without noise.
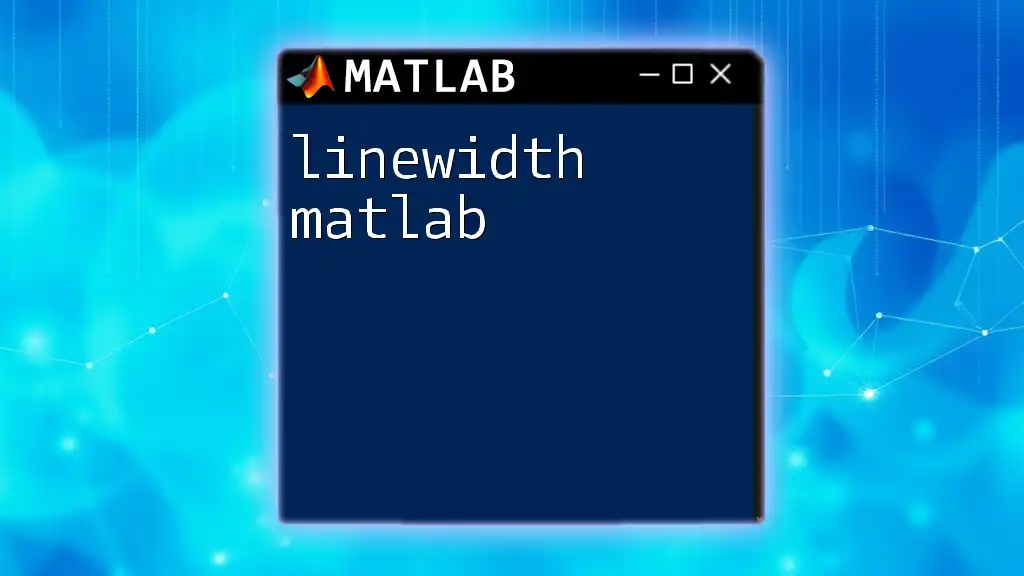
Visualizing the Results
Plotting the Original vs. Filtered Signal
Visualizing your results is essential for evaluating the performance of the filter. You can plot both the original noisy signal and the filtered output to see the impact of the Butterworth filter.
Example code for visualization is shown below:
% Plotting signals
figure;
subplot(2,1,1);
plot(t, signal);
title('Original Signal');
xlabel('Time (seconds)');
ylabel('Amplitude');
grid on;
subplot(2,1,2);
plot(t, filtered_signal);
title('Filtered Signal (Butterworth)');
xlabel('Time (seconds)');
ylabel('Amplitude');
grid on;
The use of `subplot()` allows you to display both the original and filtered signals in a single figure for easy comparison.
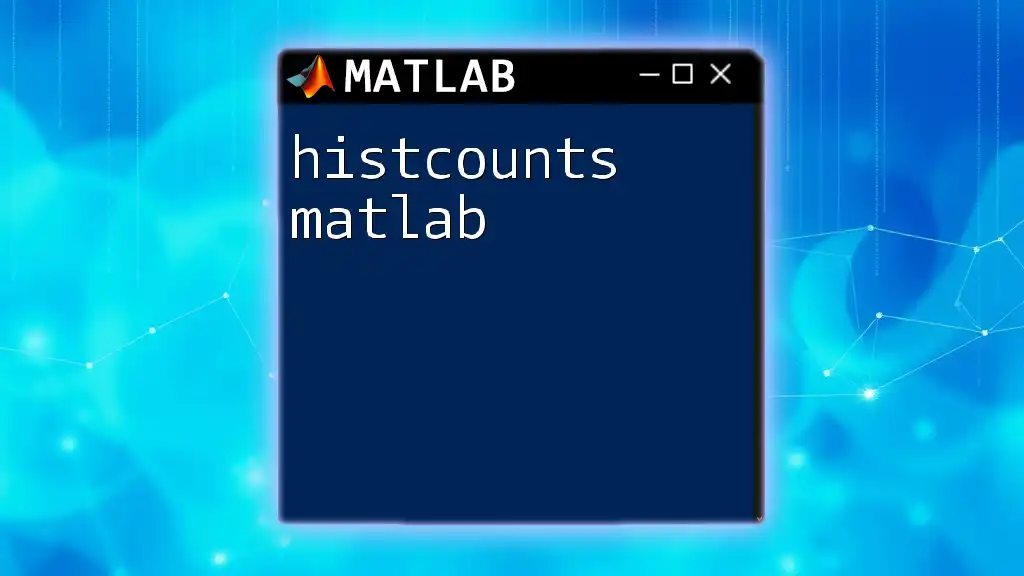
Tips and Best Practices for Using Butterworth Filters
Choosing Filter Order
Selecting the appropriate filter order is critical as it influences both performance and computational efficiency. While a higher order filter provides a steeper transition between the passband and stopband, it may also introduce complexity and computation time. Balancing these aspects according to your specific use case is important.
Avoiding Common Pitfalls
Ensure that your cutoff frequencies are properly normalized. In MATLAB, this involves dividing the desired cutoff frequency by half the sampling frequency. Additionally, understanding the implications of the filter's phase response is crucial for applications where timing is sensitive.
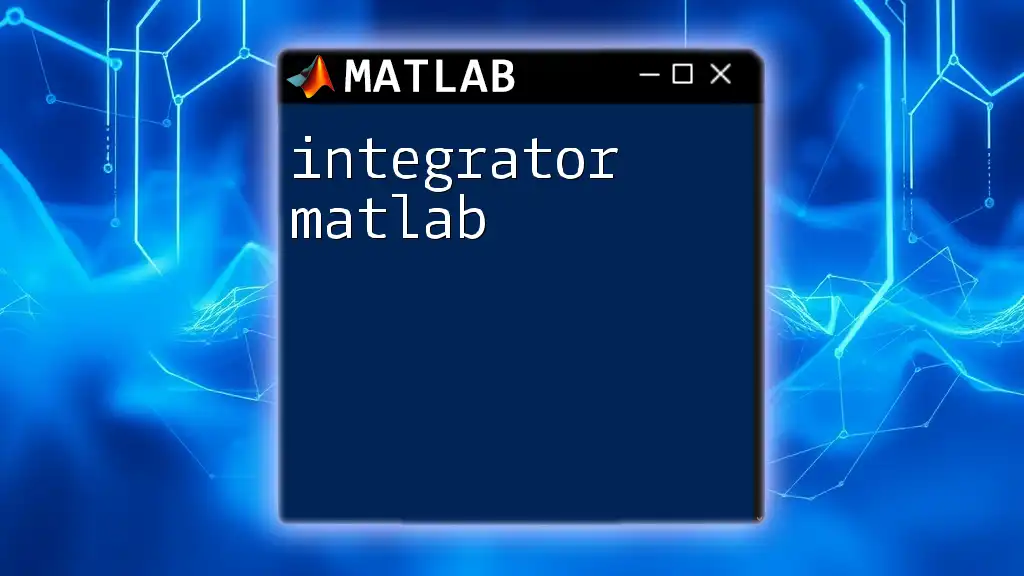
Conclusion
In summary, the Butterworth filter is an essential tool in signal processing, offering a smooth frequency response that ensures minimal distortion. MATLAB serves as a powerful platform for designing and applying these filters efficiently. By following the outlined steps and exploring the provided code snippets, you can effectively implement the Butterworth filter for your signal processing tasks. Experimenting with different filter types and MATLAB functions can further enhance your skills.
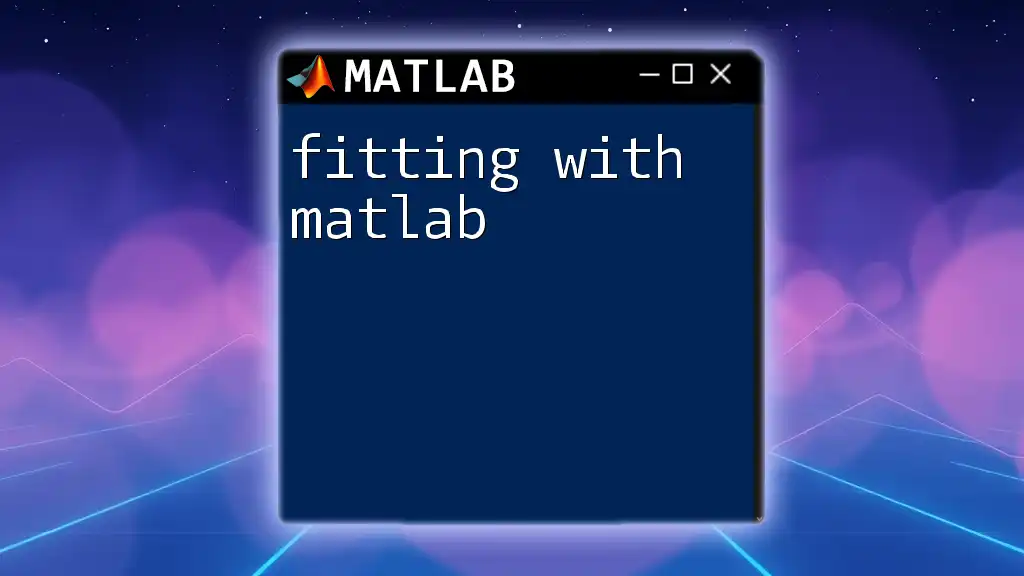
Additional Resources
For those interested in deepening their knowledge, the official MATLAB documentation offers comprehensive guides and examples for `butter()` and `freqz()`. Additionally, various online courses and tutorials are available to bolster your expertise in signal processing. Engaging in community forums can also provide valuable support and insights as you navigate your learning journey.