The `ilaplace` command in MATLAB is used to compute the inverse Laplace transform of a given symbolic expression, facilitating the analysis of systems in the time domain.
Here's a code snippet demonstrating its usage:
syms s t
F = 1/(s^2 + 1); % Define the Laplace transform
f_t = ilaplace(F, s, t); % Compute the inverse Laplace transform
disp(f_t); % Display the result
Understanding the Inverse Laplace Transform
What is the Laplace Transform?
The Laplace transform is a powerful mathematical tool that translates complex differential equations from the time domain into the frequency domain. This transformation helps simplify the process of solving linear ordinary differential equations by converting them into algebraic equations. The Laplace transform is defined as:
$$ L\{f(t)\} = F(s) = \int_0^\infty e^{-st} f(t) dt $$
In various fields such as engineering, control theory, and signal processing, the Laplace transform is widely used for analyzing linear time-invariant systems.
Overview of the Inverse Laplace Transform
The inverse Laplace transform is essential for converting frequency-domain functions back into time-domain functions. This process is crucial for understanding how a system behaves over time based on its frequency response. Mathematically, the inverse Laplace transform is defined as:
$$ f(t) = L^{-1}\{F(s)\} = \frac{1}{2\pi j} \int_{c - j\infty}^{c + j\infty} e^{st} F(s) ds $$
Understanding how to apply the `ilaplace` function in MATLAB is vital for anyone working in applied mathematics, engineering, or any field requiring the analysis of dynamic systems.
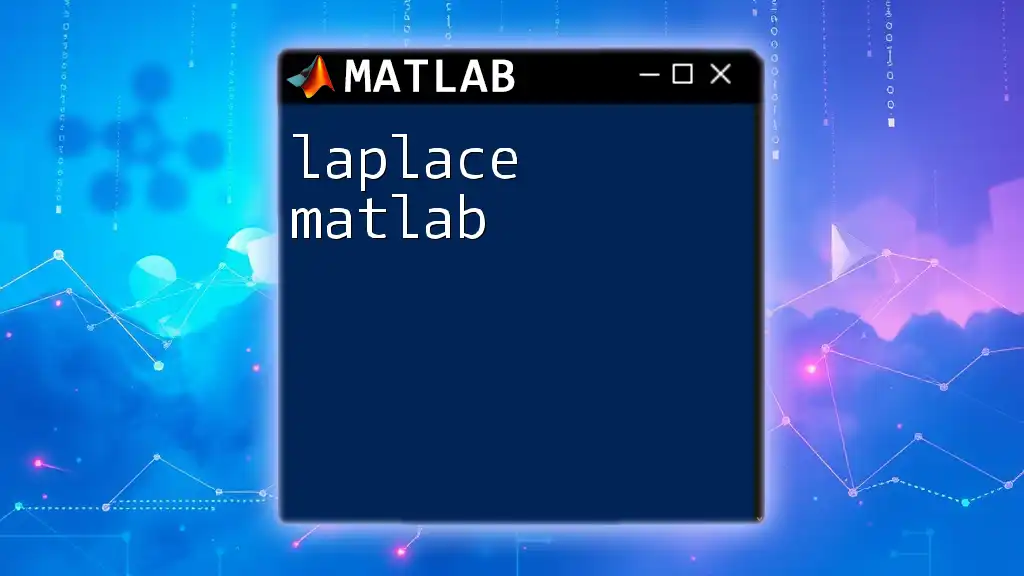
Using `ilaplace` Function in MATLAB
Basic Syntax
To use the `ilaplace` function, you define your variables alongside the Laplace transform function. The syntax for the `ilaplace` function is straightforward. Here’s how it looks:
syms t s
F = 1/s^2; % Example Laplace Transform
f_t = ilaplace(F, s, t);
In this code, `F` represents the Laplace transform of a function. The output `f_t` is the corresponding time-domain function.
Required Packages
Make sure you have the Symbolic Math Toolbox installed in MATLAB, as the `ilaplace` function requires this toolbox to work. You can check if it's available by using the command `ver`. If it's not included in the list, you will need to install it to access symbolic math features, including `ilaplace`.
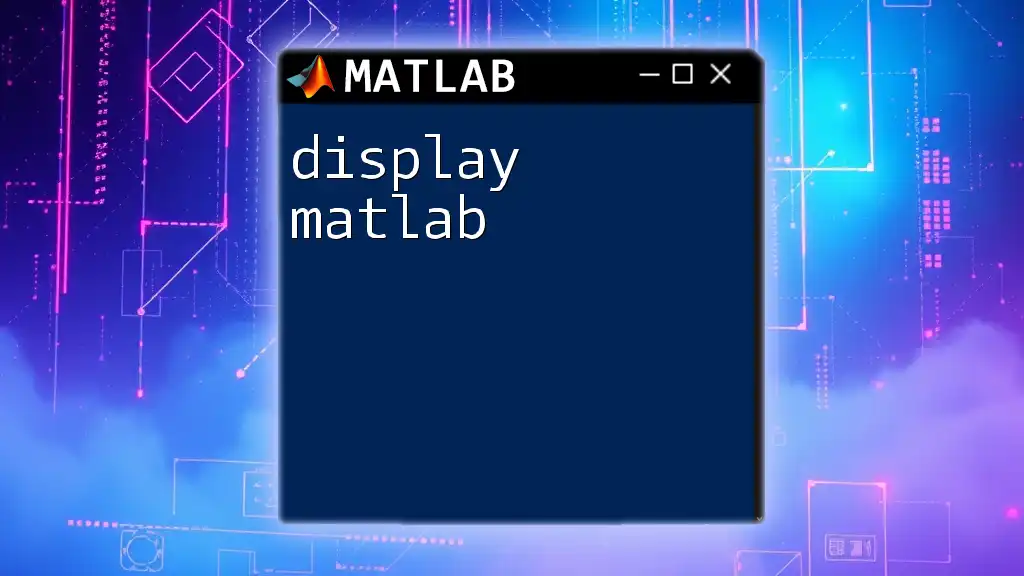
Example Scenarios
Example 1: Simple Function
Let’s start with a basic example of finding the inverse Laplace transform of a simple function, like:
syms t s
F = 2/(s^2 + 1); % Laplace Transform of sin(t)
f_t = ilaplace(F, s, t);
In this case, the result returned by `ilaplace` will be `2*sin(t)`. This illustrates how the inverse transform successfully retrieves a familiar time-domain function from its Laplace representation.
Example 2: Complex Function
When working with more complex Laplace transforms, such as polynomial terms, the process remains similar:
syms t s
F = (s^2 + 3*s + 2)/(s^3 + 3*s^2 + 3*s + 1); % Example function
f_t = ilaplace(F, s, t);
Running this code retrieves the time-domain function that corresponds to the given Laplace transform. Depending on the complexity, the output may involve using special functions or combinations of elementary functions.
Example 3: Piecewise Functions
MATLAB can also handle piecewise functions with the `ilaplace` function. For instance, if you define an exponential decay function:
syms t s
F = piecewise(t < 0, 0, t >= 0, exp(-t)); % Exponential decay
f_t = ilaplace(F, s, t);
The result correctly adapts to the definition of the piecewise function, demonstrating how `ilaplace` can manage varying function definitions over different intervals.
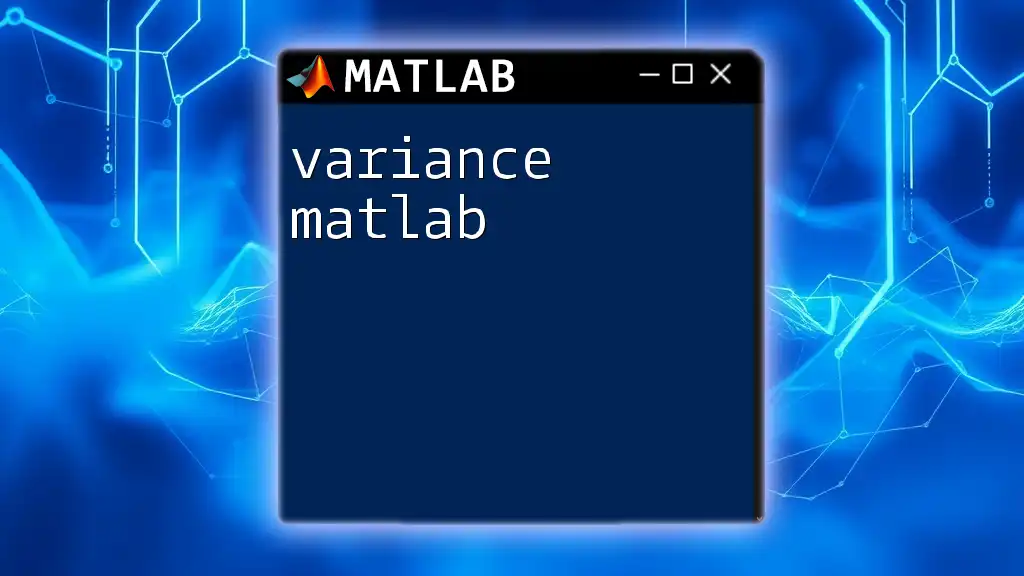
Advanced Features of `ilaplace`
Using Assumptions
When computing transforms, defining assumptions can be invaluable for simplifying results. For example:
syms t s
assume(t >= 0);
F = 1/(s^2 + 1);
f_t = ilaplace(F, s, t);
Here, the assumption specifies that time must be non-negative, which results in more precise output tailored to typical physical scenarios.
Handling Multiple Variables
You may encounter functions with multiple variables where `ilaplace` remains effective. Here's an example:
syms t s x
F = (s + x)/(s^2 + x^2);
f_t = ilaplace(F, s, t);
With the presence of `x`, it is crucial to understand how variable interactions affect the transformed output, often requiring separate evaluations or transformations depending on specific contexts.
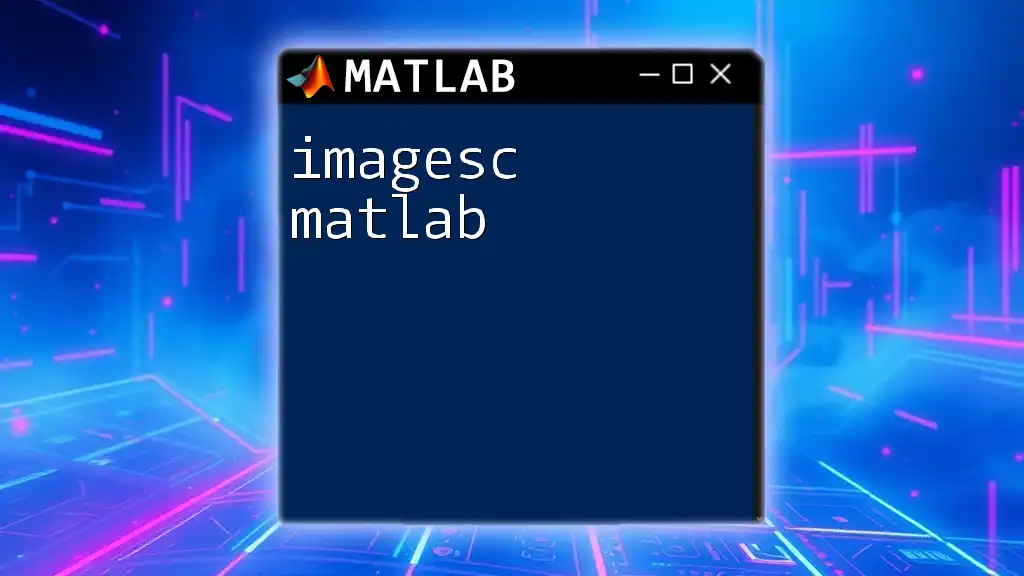
Common Errors and Troubleshooting
Common Issues with `ilaplace`
While using `ilaplace`, users may experience various common errors. One typical issue is forgetting to define the symbolic variables. Such an oversight will lead to unexpected outputs or errors indicating that variables are undefined.
Example of an Error
Here's an example of an incorrect usage that results in an error:
syms t s
F = 1/(s - 1); % Incorrect assumption
f_t = ilaplace(F, s, t);
This code will typically fail due to incorrect assumptions leading to singularities. Understanding how to anticipate and debug these issues enhances your usability of `ilaplace`.
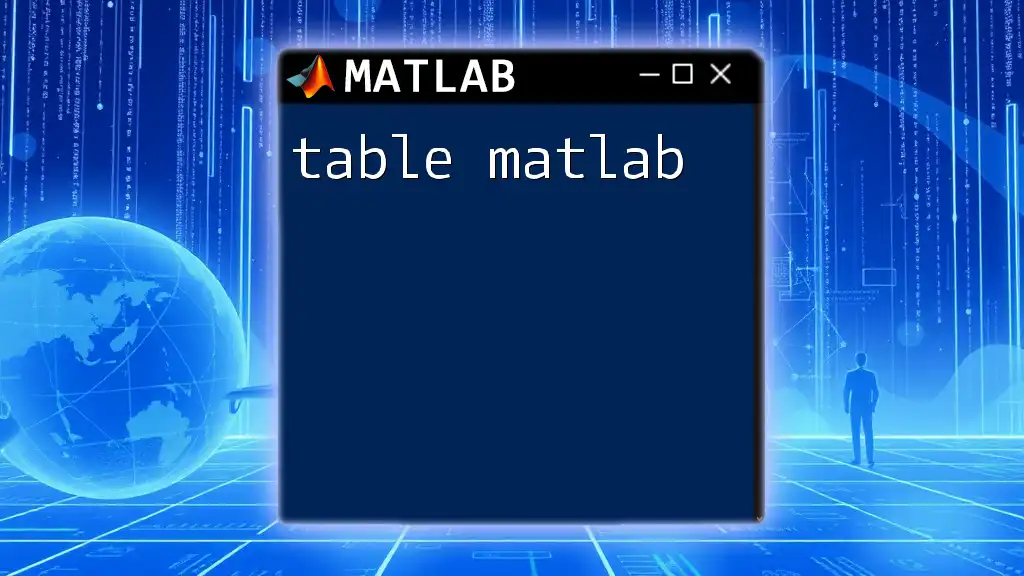
Conclusion
In summary, the `ilaplace` function in MATLAB is a powerful tool for converting Laplace transform representations back into time-domain functions. Mastering this command can significantly streamline your analysis of dynamic systems in various fields. By practicing with the examples provided and understanding the common pitfalls, you can confidently utilize the `ilaplace` function to tackle more complex computational challenges.
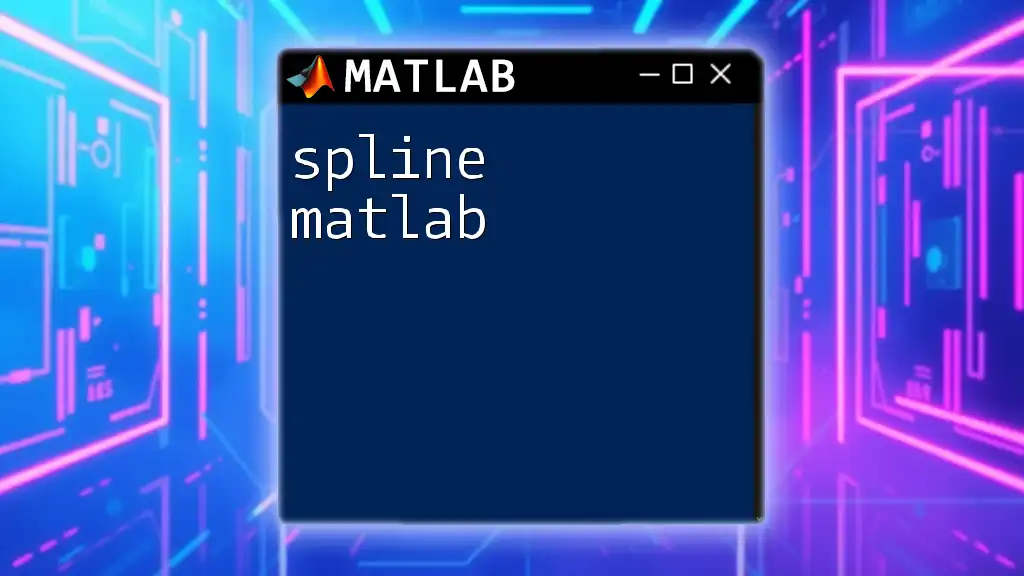
Additional Resources
For further exploration, don't hesitate to consult the official MATLAB documentation on the `ilaplace` function. There are numerous online courses and tutorials available that delve into symbolic computation and Laplace transforms, providing a deeper understanding of both theoretical and practical applications.
Engage in our workshops or online classes to enhance your MATLAB skills. Practicing the examples provided will solidify your understanding, enabling you to tackle real-world problems using `ilaplace` in MATLAB effectively.