To convert a table to a cell array in MATLAB, you can use the `table2cell` function which extracts the data in a format suitable for cell arrays.
Here’s a code snippet demonstrating this conversion:
% Sample table creation
T = table([1; 2; 3], {'A'; 'B'; 'C'}, [4.5; 5.5; 6.5], 'VariableNames', {'ID', 'Letter', 'Value'});
% Convert table to cell array
C = table2cell(T);
What is a Table in MATLAB?
Definition of a Table
In MATLAB, a table is a data type specifically designed for handling datasets that contain variables of different types. This means you can have a table that includes numeric arrays, character vectors, and cell arrays, all organized in rows and columns. The table is an incredibly useful structure for data analysis because it allows for intuitive access to data as well as easy manipulation, making it a preferred format for many data analysts and scientists.
Key Features of Tables
Tables come with several advantageous features:
-
Heterogeneous Data Types: You can mix different data types within the same table. For example, one column could contain integers, while another might contain strings or categorical data.
-
Row and Column Names: Tables utilize variable names (column headers) and row names, which provide clarity and context to the data being analyzed.
-
Built-In Functions: MATLAB provides specialized functions to manipulate tables, such as sorting, filtering, and summarizing.
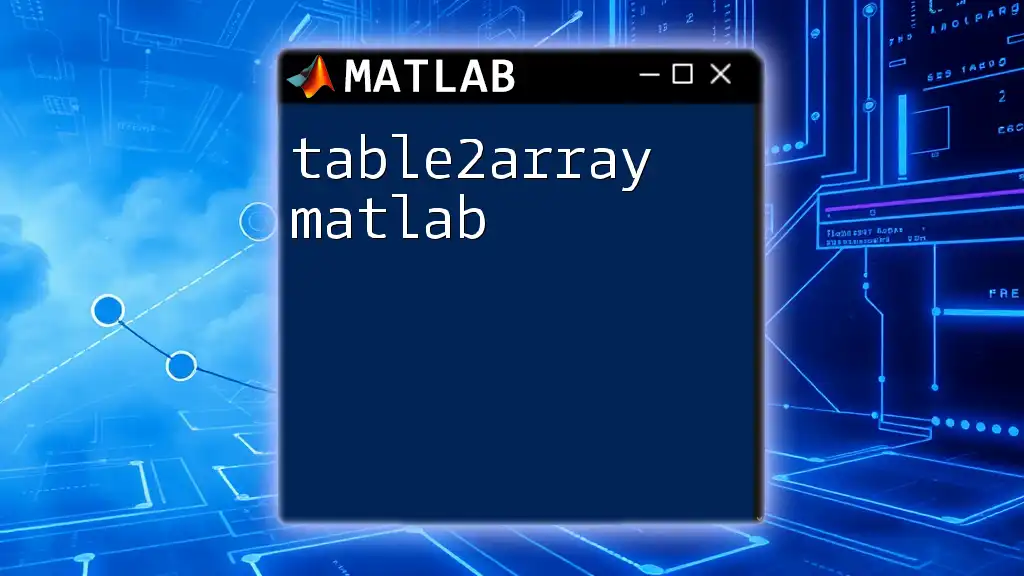
What is a Cell Array in MATLAB?
Definition of a Cell Array
A cell array is a versatile and flexible data structure in MATLAB that allows you to store arrays of different sizes and types in a single variable. Each element of a cell array can contain different types of data, including matrices, strings, or even other cell arrays.
Key Features of Cell Arrays
The cell array offers some unique advantages:
-
Diverse Data Types: Unlike regular arrays that are limited to a single data type, cell arrays can hold various types of data, making them ideal for complex datasets.
-
Variable Dimensions: Each cell can have a different size and shape, enabling more intricate data arrangements.
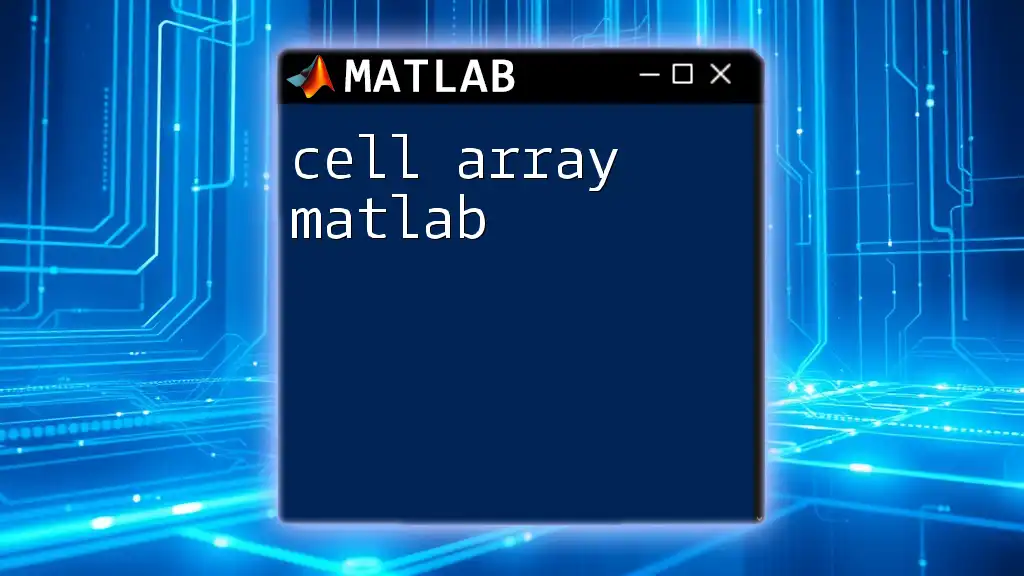
Reasons to Convert Table to Cell Array
Flexibility of Cell Arrays
There are several scenarios where it makes sense to convert a table to a cell array. For instance, if your analysis requires passing data to functions that only accept cell arrays, this conversion is essential. Moreover, when exporting data to file formats less accommodating of the table format, a cell array might be preferred.
Compatibility with Functions
Some built-in MATLAB functions exclusively require data in cell array format. This necessitates the conversion from table format to cell format, ensuring smooth integration and functionality within your MATLAB scripts.
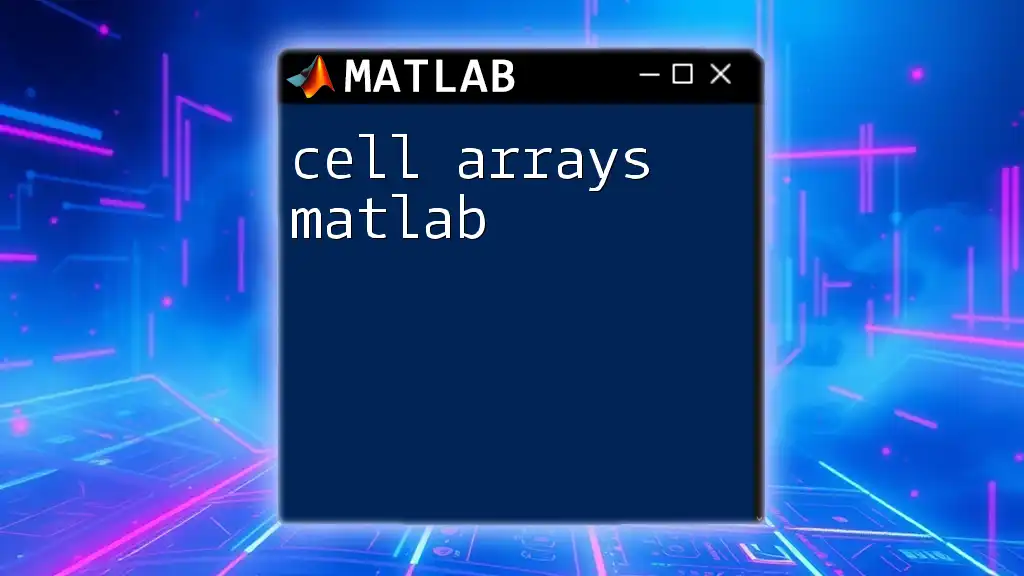
How to Convert Table to Cell Array in MATLAB
Using the `table2cell` Function
The primary function used to convert a table into a cell array in MATLAB is `table2cell`. This function extracts all the data from a table and stores it into a cell array, allowing for flexible data manipulation.
Example 1: Basic Conversion
Let’s start with a straightforward example:
% Creating a sample table
T = table([1; 2; 3], {'A'; 'B'; 'C'}, 'VariableNames', {'Numbers', 'Letters'});
% Converting table to cell array
C = table2cell(T);
disp(C);
In this example, a table `T` is created with two columns: one numeric and one string. The `table2cell` function converts this table into a cell array `C`, displaying the results.
Handling Different Data Types in Tables
When the table consists of a mix of data types, the conversion process maintains this distinction, allowing for varied data types in the resulting cell array.
Example 2: Converting Tables with Mixed Data Types
Here’s another example that demonstrates converting a table with mixed data types:
% Creating a sample table with mixed data types
T = table([1; 2; 3], {'X'; 'Y'; 'Z'}, [10.5; 12.3; 9.8], 'VariableNames', {'Index', 'Label', 'Value'});
% Converting to cell array
C = table2cell(T);
disp(C);
In this case, the table \( T \) includes an integer column, a string column, and a floating-point column. The conversion results in a cell array \( C \) that retains all types within its structure.
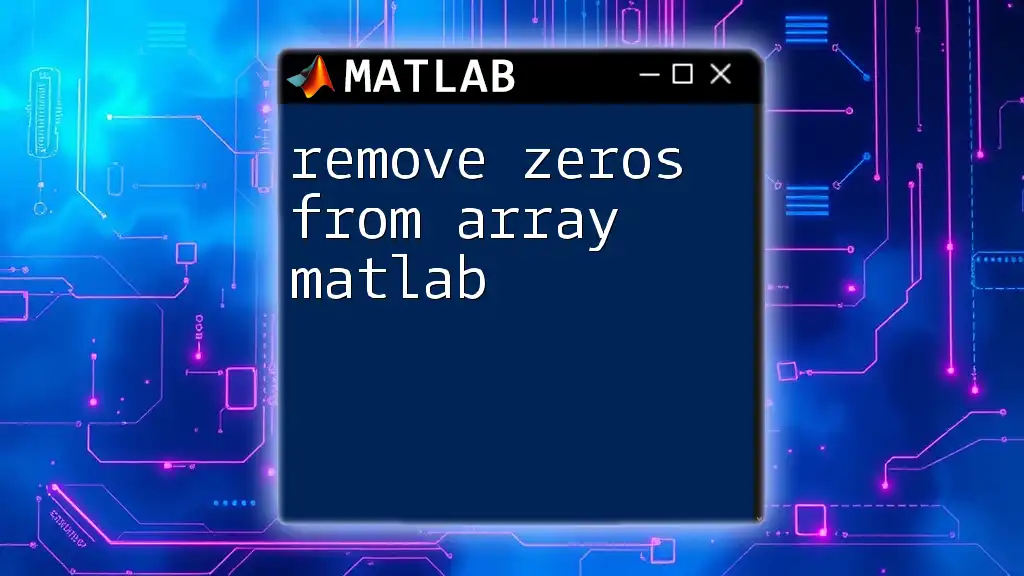
Considerations When Converting
Performance Implications
When dealing with large datasets, be mindful of the performance implications during conversion. Converting massive tables into cell arrays can be computationally expensive. In such cases, consider whether the conversion is necessary for your analysis or if you can utilize table operations instead.
Maintaining Data Integrity
To ensure data integrity during and after conversion, double-check the cell array structure. Make sure that each piece of data is displayed as expected, and verify that no data has been lost or misrepresented in the conversion process.
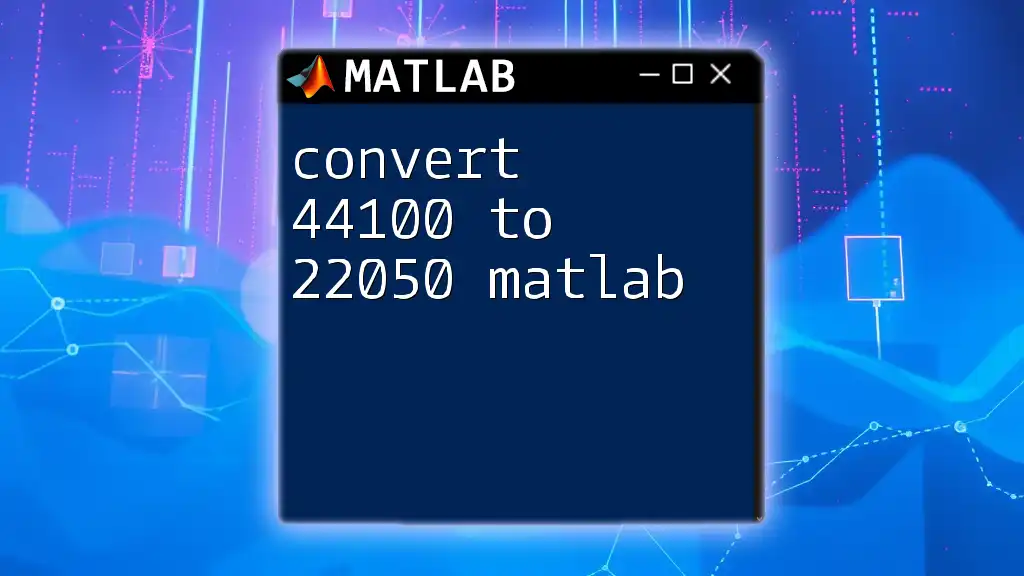
Common Error Messages and Troubleshooting
Error Handling
When working with the `table2cell` function, users may encounter issues. One common error is when the input table has unsupported data types. Ensure that all variable types in the table are compatible for conversion to a cell array.
Debugging Tips
If the output from `table2cell` does not match expectations, use these debugging techniques:
- Check the variable types in your table using `varfun`.
- Inspect the table’s structure with `summary` to ensure it is in the format intended for conversion.
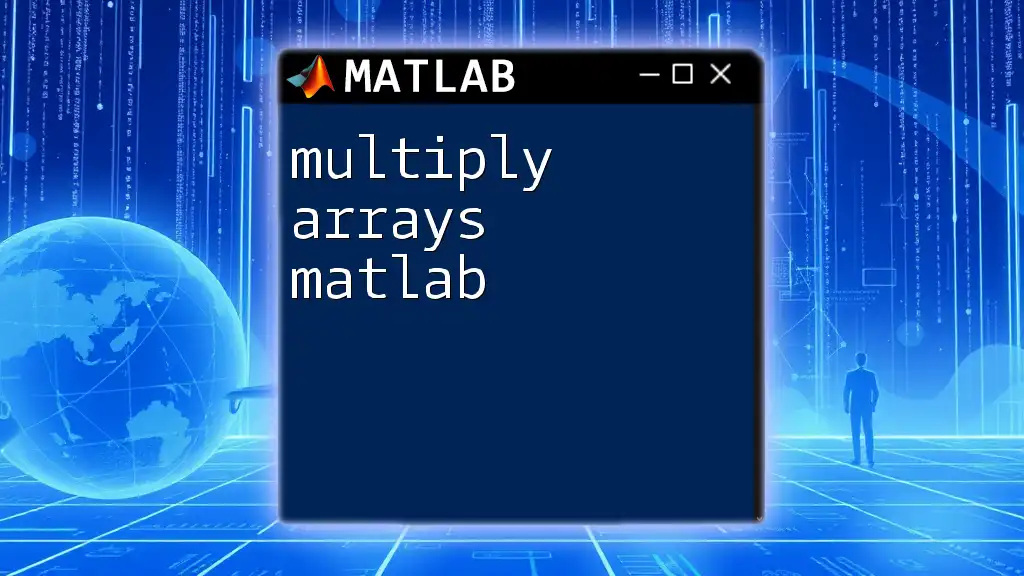
Additional Functions and Techniques
Using Implicit Conversion
Sometimes, MATLAB allows implicit conversion when passing a table directly into a function expecting a cell array. While this can be convenient, it is not always reliable. Always verify the behavior of your specific functions.
Other Relevant Functions
In addition to `table2cell`, consider using related functions like `cell2table`, which allows you to convert a cell array back into a table, strengthening the interchangeability of these two data types. Furthermore, MATLAB’s `vertcat` function can concatenate tables and cell arrays efficiently.
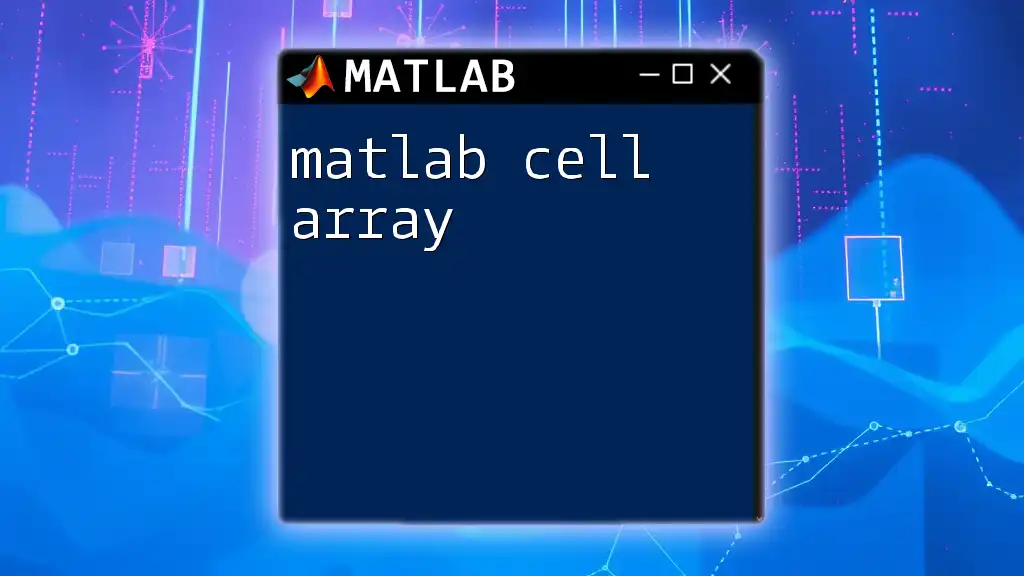
Conclusion
Understanding how to convert table to cell array in MATLAB is crucial for enhancing your data analysis capabilities. This conversion allows for greater flexibility in data manipulation and expands the set of MATLAB functions available to you. Dive deeper into MATLAB's comprehensive documentation and leverage your newfound knowledge to optimize your data handling techniques effectively.
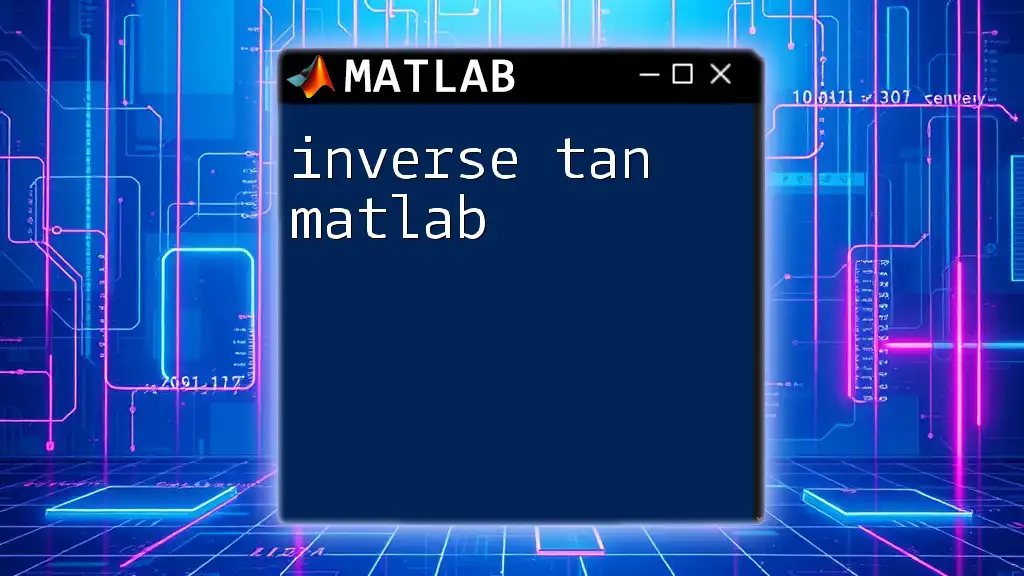
References
Be sure to refer to MATLAB's official documentation for further reading and explore additional functionalities that can complement your use of tables and cell arrays.
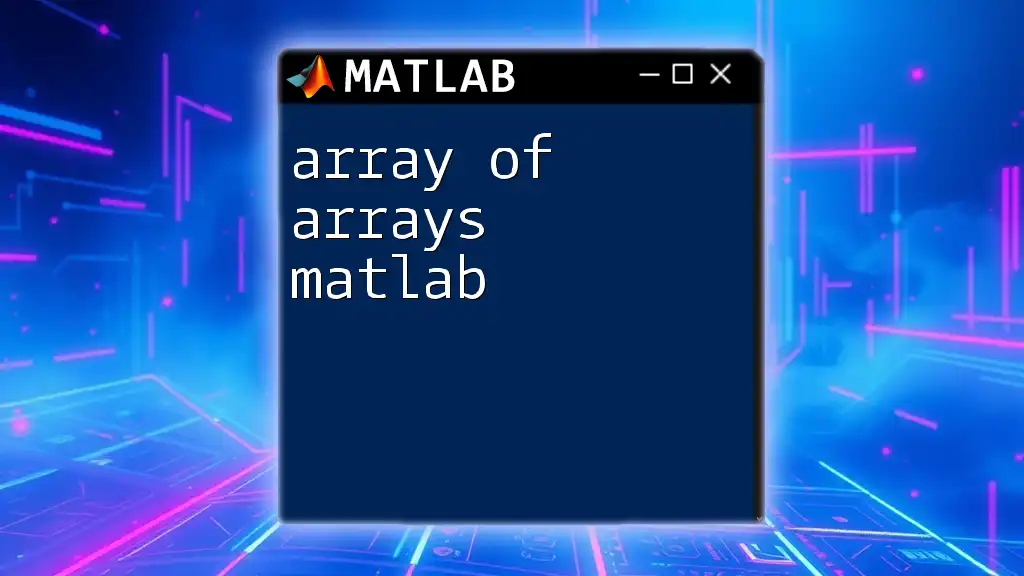
Call to Action
Share your experiences with converting tables to cell arrays in the comments below. If you have questions or need further assistance, don’t hesitate to ask! Stay tuned for upcoming workshops where we delve into mastering MATLAB commands effectively.