Monte Carlo simulation in MATLAB is a statistical technique that uses random sampling to approximate solutions to quantitative problems, often used for estimating the probability of different outcomes in a process that cannot easily be predicted due to the intervention of random variables.
Here’s a simple code snippet demonstrating a Monte Carlo simulation to estimate the value of π:
N = 10000; % Number of random points
x = rand(N, 1); % Generate random x coordinates
y = rand(N, 1); % Generate random y coordinates
inside_circle = (x.^2 + y.^2) <= 1; % Check if points are inside the unit circle
pi_estimate = 4 * sum(inside_circle) / N; % Estimate π
disp(pi_estimate) % Display the estimated value of π
What is Monte Carlo Simulation?
Monte Carlo simulation is a computational technique that utilizes random sampling to obtain numerical results. By simulating the outcomes of uncertain variables, it provides insights into systems with inherent randomness. This method is particularly useful in fields such as finance, engineering, and physics, where understanding risk and variability is paramount.
The technique derives its name from the famous Monte Carlo Casino because of the inherent randomness in games of chance. Historically, it was developed during World War II for nuclear weapon projects but has since expanded into numerous applications.
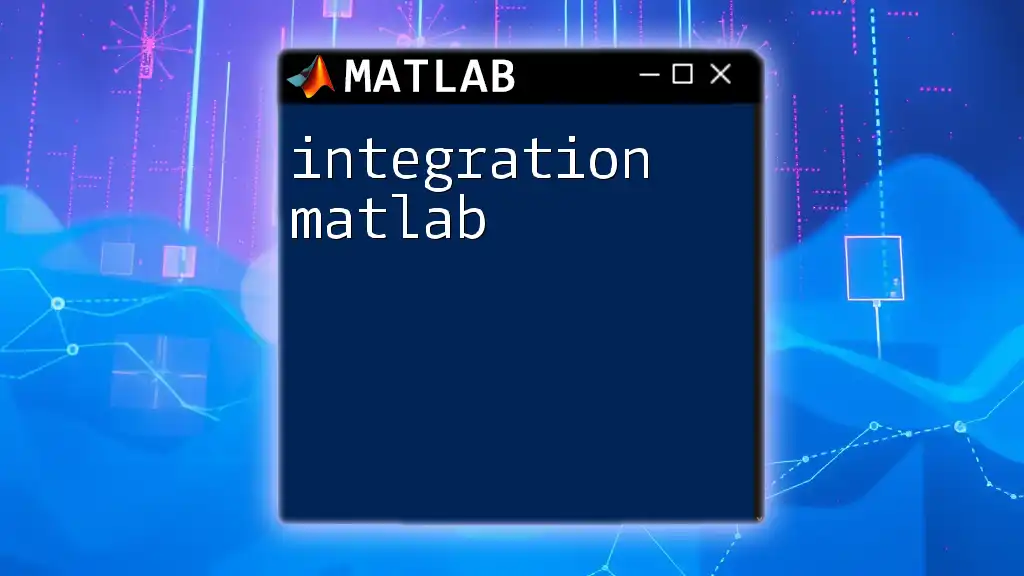
Importance of Monte Carlo Simulation in MATLAB
MATLAB is an excellent platform for performing Monte Carlo simulations due to its powerful computational capabilities and ease of use. It provides an array of built-in functions specifically designed to handle complex mathematical operations essential for simulations. Furthermore, its visualization tools enable users to interpret results efficiently, leading to better understanding and decision-making.
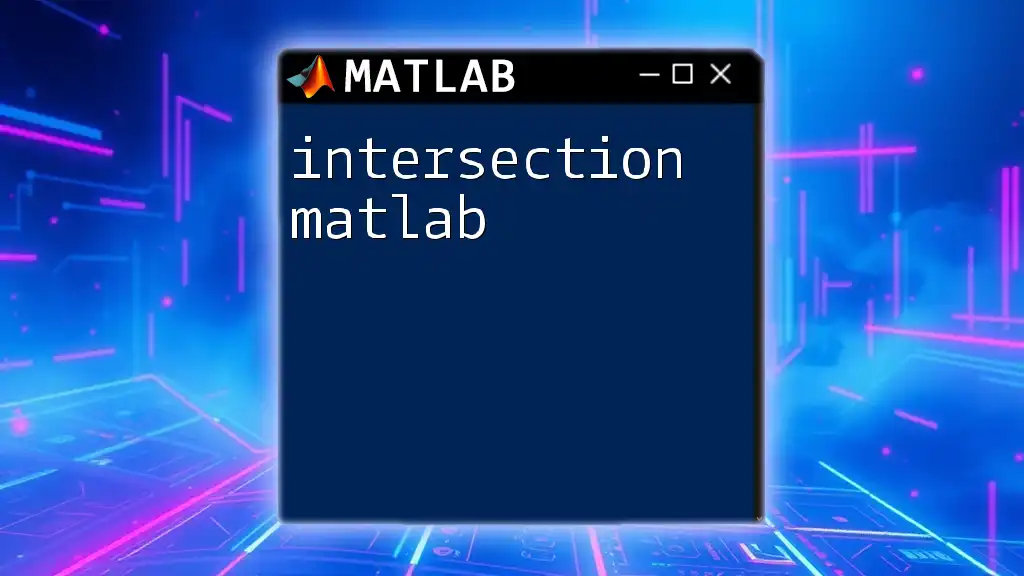
Setting Up the Environment
Installing MATLAB
To get started, the first step is to install MATLAB. Simply visit the official MathWorks website, download the installer, and follow the prompts. Ensure you select the appropriate toolboxes that meet your simulation needs, such as the Statistics and Machine Learning Toolbox, for enhanced functionality.
Basic MATLAB Commands Overview
Before diving into Monte Carlo simulations, it's helpful to familiarize yourself with essential MATLAB commands. This includes understanding variables, loops, and functions, which are fundamental for creating scripts and managing data effectively.
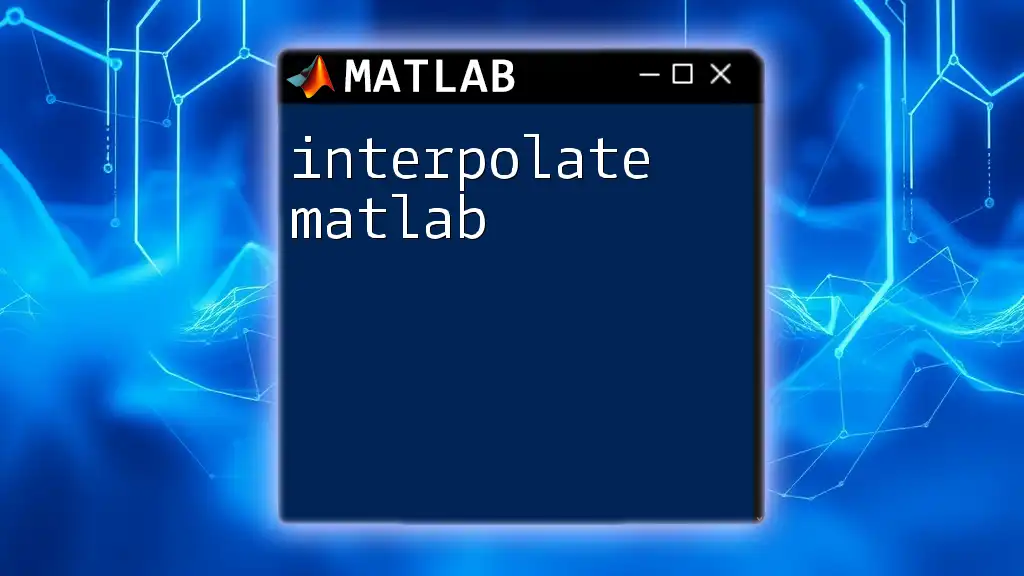
Understanding Monte Carlo Methods
How Monte Carlo Methods Work
The essence of Monte Carlo methods lies in randomness and statistical sampling. By generating random samples and analyzing the results, the approach effectively approximates complex integrals or solves differential equations. The law of large numbers states that as the sample size increases, the sample mean converges to the expected value, making Monte Carlo an effective technique for approximating statistical measures.
Common Applications of Monte Carlo Simulation
Monte Carlo simulations are versatile and applicable across various fields:
- Finance: Used for risk assessment and evaluating investment strategies, helping to determine the potential future performance of assets.
- Engineering: Assists in reliability analysis and design optimization, providing insights into system resilience.
- Physics: Employed in particle simulation and other phenomena where randomness plays a critical role.
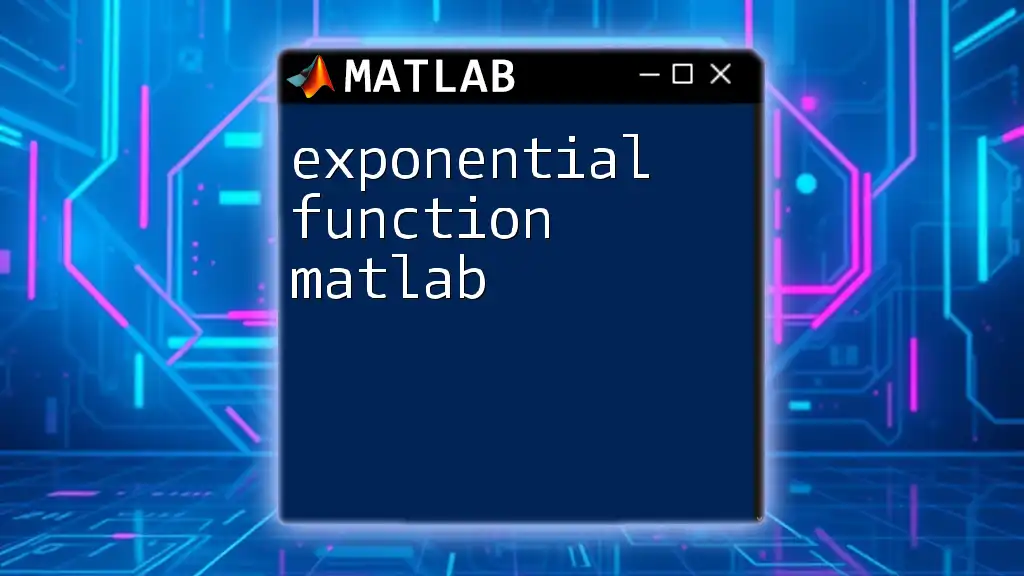
Implementing Monte Carlo Simulations in MATLAB
Step-by-Step Guide to Creating Your First Monte Carlo Simulation
Defining the Problem
As an illustration, let’s compute the value of Pi using a Monte Carlo simulation. The problem is straightforward: if we randomly place points in a unit square and count how many fall within a quarter circle, we can estimate Pi based on the ratio of points inside the circle to the total number of points.
Generating Random Samples
To generate random samples, you can use MATLAB’s random number generators. Here’s how to create random coordinates:
num_samples = 10000;
x = rand(num_samples, 1); % Generate random samples for X
y = rand(num_samples, 1); % Generate random samples for Y
This code snippet generates `num_samples` points uniformly distributed between 0 and 1 for both the x and y coordinates.
Evaluating the Results
After generating the random samples, the next step is evaluating whether each point falls within the quarter circle.
inside_circle = sum(x.^2 + y.^2 <= 1);
pi_estimate = (inside_circle / num_samples) * 4;
In this example, `inside_circle` counts how many points lie within the radius of 1 (inside the quarter circle). The final estimate of Pi is calculated by the ratio of points inside to the total number of points, scaled by 4.
Example 1: Estimating Pi using Monte Carlo
By implementing the steps outlined above, you can effectively estimate the value of Pi. After running the simulation, you’ll find an estimate that gets closer to the actual value (3.14159) as you increase `num_samples`. The significance of this example is not only in computing Pi but also in understanding how randomness can be harnessed in MATLAB to solve mathematical problems effectively.
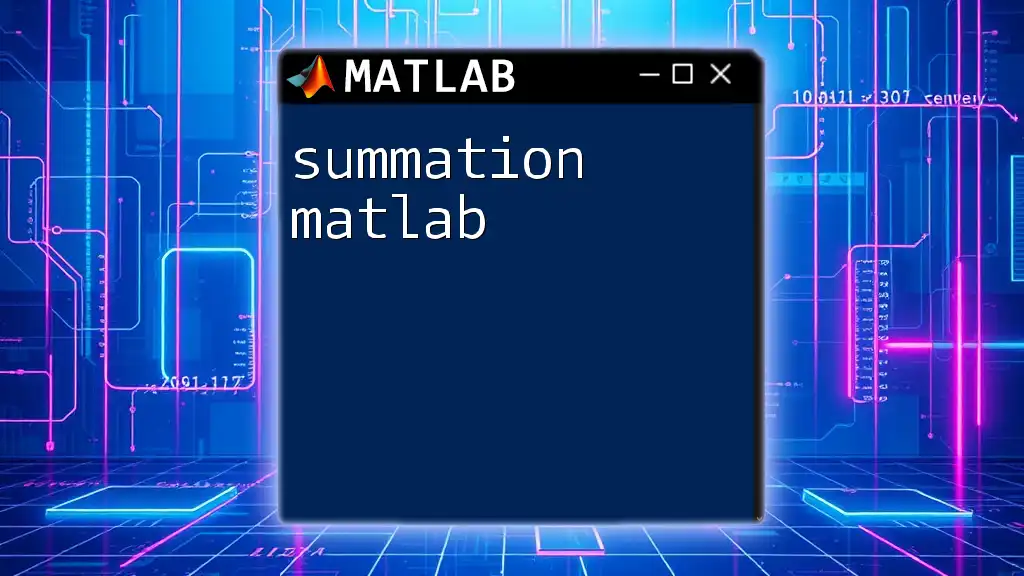
Advanced Monte Carlo Techniques
Variance Reduction Techniques
In scenarios where accuracy is crucial, variance reduction techniques can help enhance the efficiency of Monte Carlo simulations. Techniques like stratified sampling and control variates serve to minimize estimation errors. For instance, in stratified sampling, you divide the population into distinct subgroups and sample from each to achieve more accurate estimates.
Parallel Computing for Enhanced Performance
For complex simulations, MATLAB’s Parallel Computing Toolbox can significantly boost performance. By leveraging multithreading, you can perform multiple simulations at once, which is particularly useful when estimating probabilities across numerous scenarios.
parfor i = 1:num_samples
% Example code for parallel processing
% Perform simulation here for each sample
end
By using `parfor`, you can distribute the iterations across available CPU cores, reducing the total computation time compared to traditional loops.
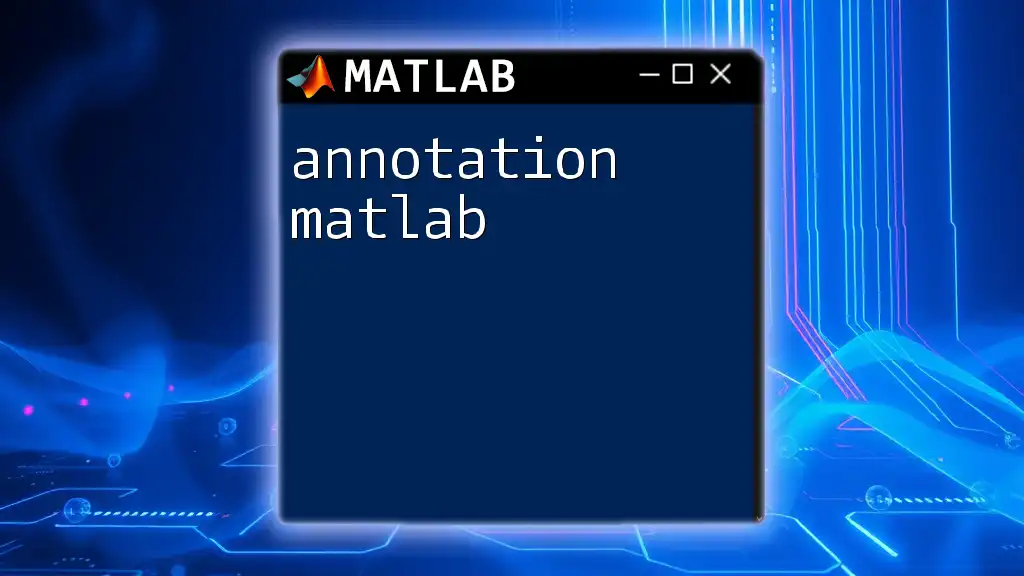
Visualization of Results
Plotting in MATLAB
Visualizing the results of Monte Carlo simulations can provide invaluable insights. MATLAB offers robust plotting functions to aid in data interpretation. For instance, you can create a scatter plot of the random points generated in the earlier examples:
scatter(x, y, 1, 'filled');
axis square;
title('Monte Carlo Simulation: Points inside Circle');
This code generates a scatter plot showcasing the distribution of points and visually indicates which lie inside the quarter circle.
Interpreting the Visual Data
When visualizing your simulation results, it’s essential to analyze the graphical outputs critically. Look for patterns, such as clustering of points that may indicate biases in randomness, and consider common pitfalls in data interpretation to avoid drawing inaccurate conclusions.
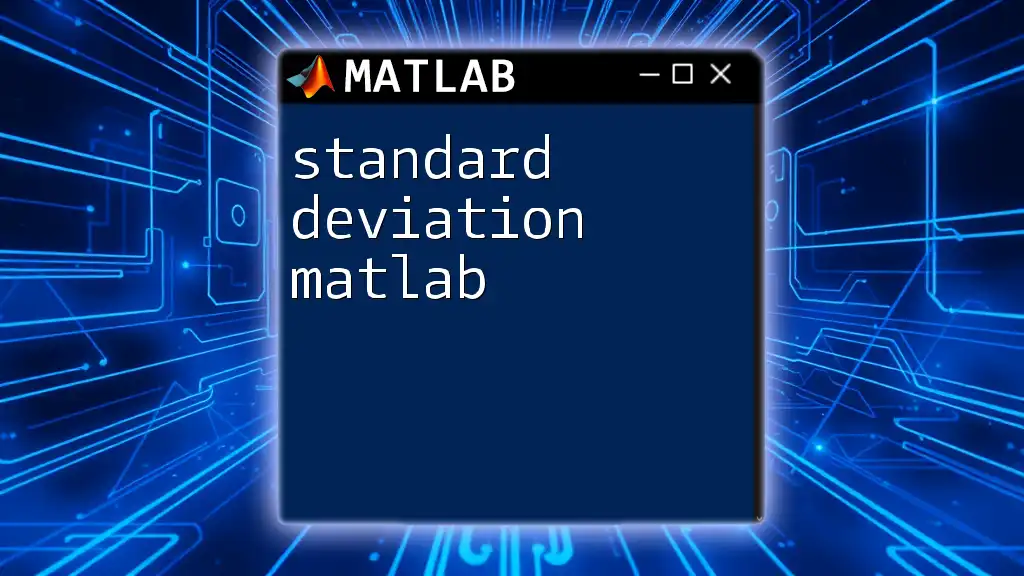
Challenges and Limitations of Monte Carlo Simulation
Common Challenges
While Monte Carlo simulations are powerful tools, they are not without challenges. One common issue is convergence, particularly when dealing with high-dimensional spaces. The number of samples required for an accurate estimate typically grows exponentially with dimensions, which can make computations infeasible.
Addressing Limitations
To enhance accuracy, it’s crucial to ensure that you have a sufficiently large sample size. Often, running multiple iterations can yield better estimates, and employing techniques like those mentioned above—such as variance reduction—can also mitigate issues related to large sample requirements.
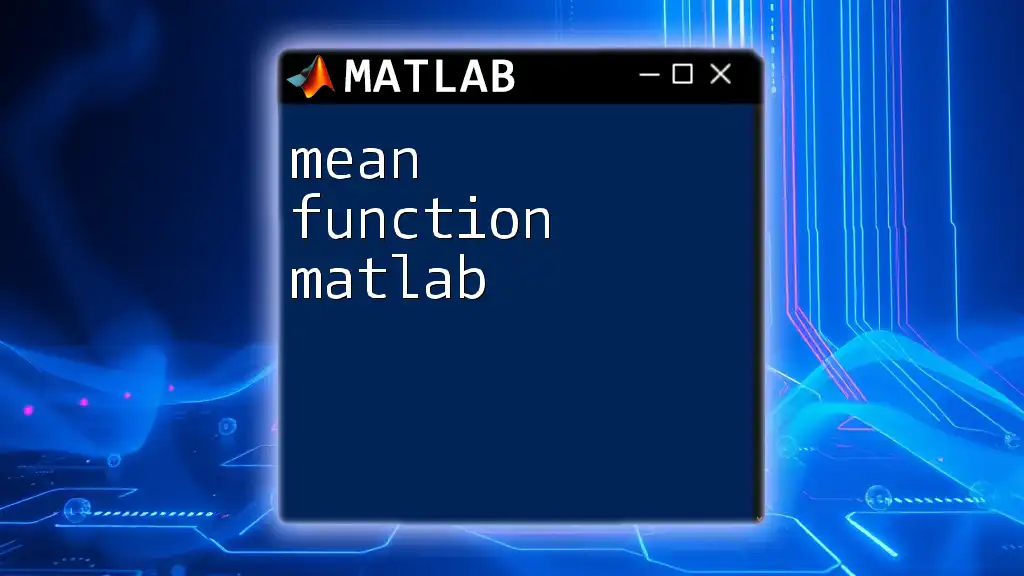
Conclusion
In summary, Monte Carlo simulation in MATLAB serves as a robust framework for tackling complex problems characterized by uncertainty and randomness. By following the outlined steps, you can harness the power of random sampling to achieve useful insights across various fields.
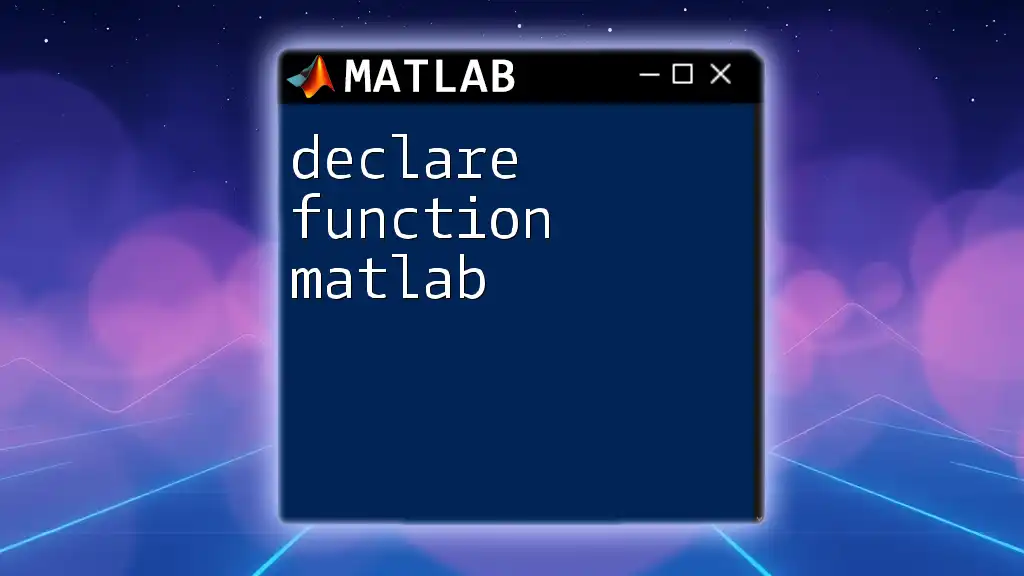
Further Resources
To further deepen your understanding of Monte Carlo simulations, consider exploring additional readings and online courses that focus on this statistical technique. MATLAB toolboxes, such as the Statistics and Machine Learning Toolbox, offer a wide range of functions that can complement your simulations, while community forums can provide support and insights from other users.
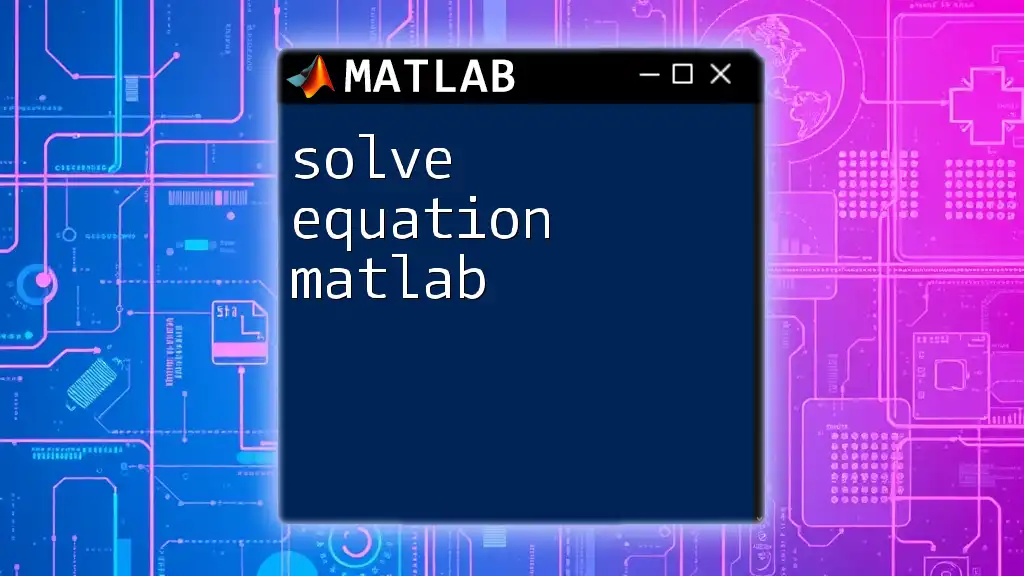
Call to Action
As you delve into Monte Carlo simulations, remember that practice is key to mastery. Consider enrolling in upcoming workshops or courses offered by our company to enhance your skills and understanding further. Embrace the power of randomness and unlock the potential of statistical methods in your projects!
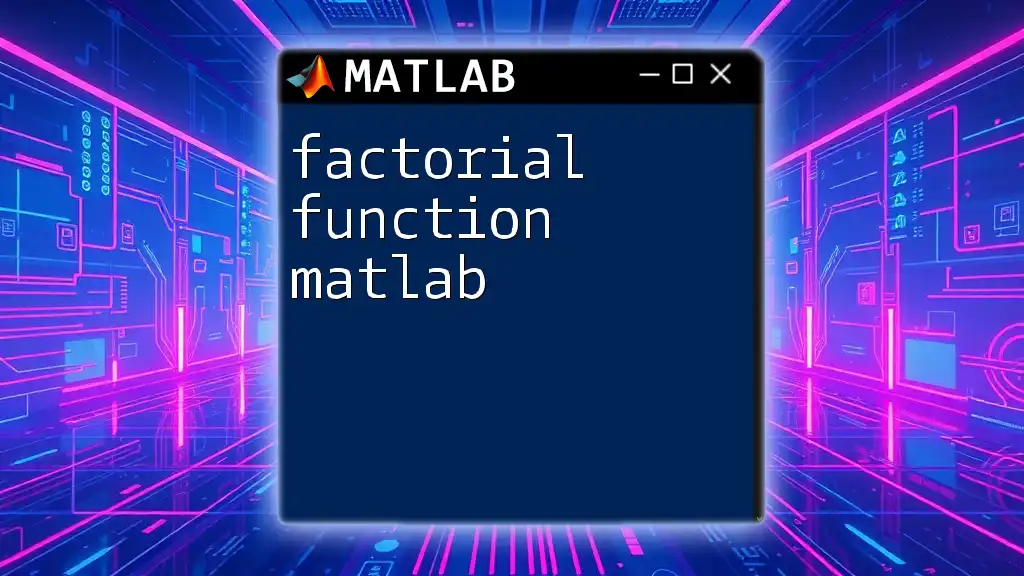
References
Here, you can include academic papers, books, and online resources tailored toward deeper engagement with the Monte Carlo simulation methodologies and their applications.
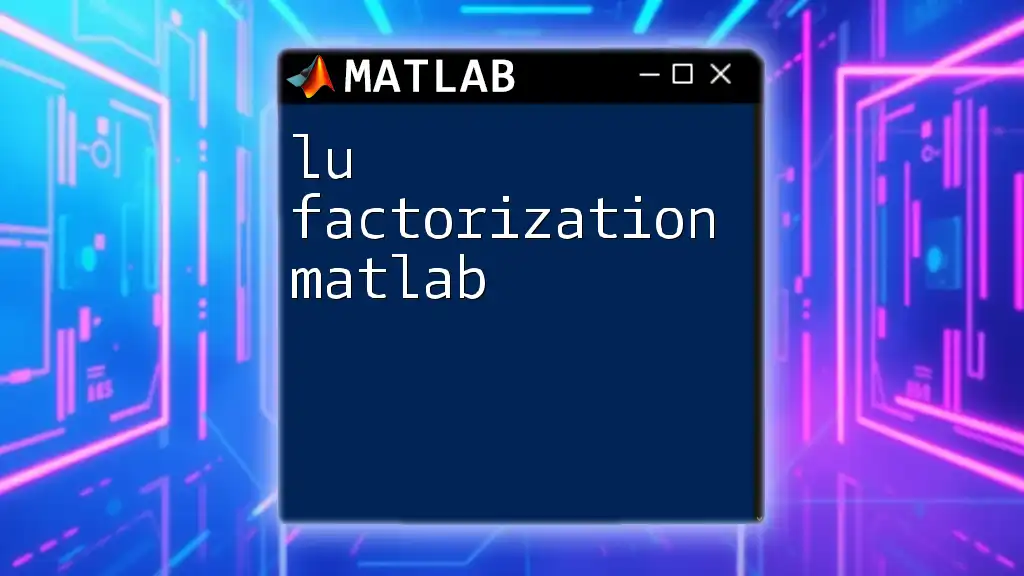
Appendix
Additional MATLAB Functions for Monte Carlo Simulations
Several MATLAB functions can enhance your Monte Carlo simulations, including:
- rand: For generating uniformly distributed random numbers.
- randn: For generating normally distributed random numbers.
- histogram: For visual representation of data distribution.
- mean and std: To calculate the mean and standard deviation of your output data.
Utilizing these functions effectively can complement your simulation efforts, allowing for richer analyses and improved results.