Dan Ellis's audio fingerprinting in MATLAB provides an efficient way to identify audio content using distinct features, enabling applications such as music recognition and audio retrieval.
Here's a simple code snippet to get you started on building an audio fingerprinting system in MATLAB:
% Load audio file
[audioIn, fs] = audioread('your_audio_file.mp3');
% Compute Short-Time Fourier Transform (STFT)
window_length = 512;
overlap = window_length / 2;
[S, F, T] = stft(audioIn, 'Window', hamming(window_length), 'OverlapLength', overlap);
% Generate fingerprint from the spectrogram
fingerprint = abs(S) > 1e-2; % Setting a threshold to generate binary fingerprint
Make sure to replace `'your_audio_file.mp3'` with the actual file name you want to analyze.
What is Audio Fingerprinting?
Audio fingerprinting is a powerful technique used to identify and catalog audio content. It creates a unique identifier, or "fingerprint," from an audio signal, allowing for quick recognition of the same audio file, even if it’s been compressed or altered. This process is essential in applications such as music recognition, copyright enforcement, and content identification.
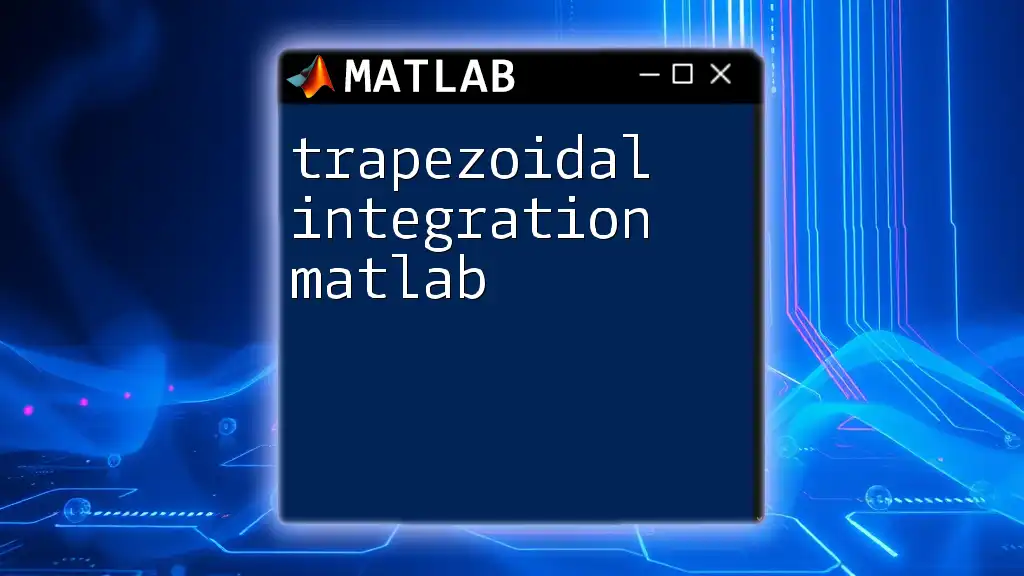
Overview of Dan Ellis' Work
Dan Ellis is a prominent figure in audio processing, particularly known for his contributions to audio fingerprinting. His research has paved the way for various algorithms and techniques that are widely used in the field today. Notably, Ellis has focused on creating robust and efficient methods for identifying audio content across different mediums and environments.
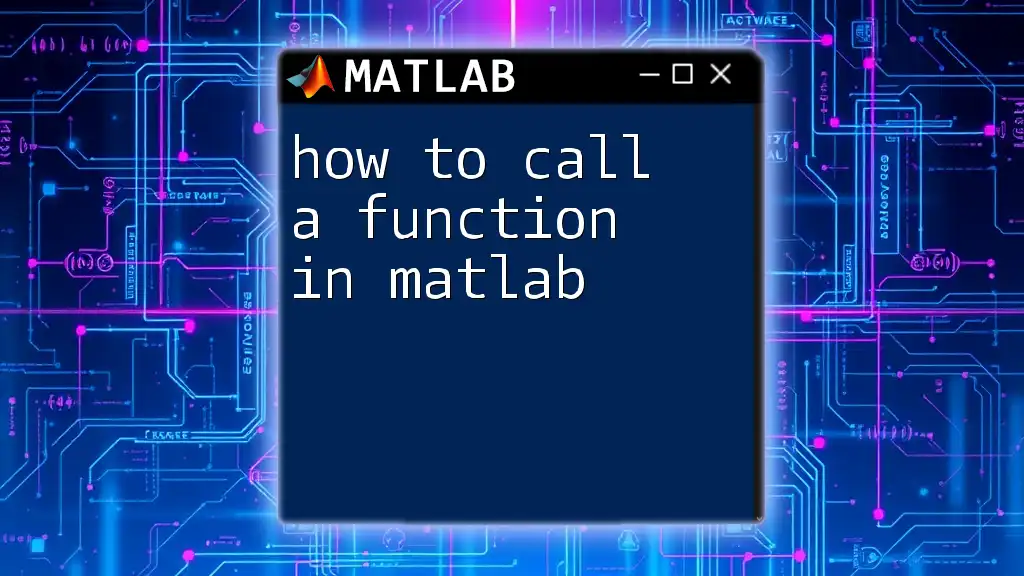
Understanding Audio Fingerprinting Techniques
Basics of Audio Signal Processing
To start with audio fingerprinting in MATLAB, it's crucial to understand how audio signals are represented. Audio data is typically captured as a time-series signal composed of amplitude values sampled over time. To prepare the data for analysis, common preprocessing steps include normalization, which adjusts the amplitude levels, filtering to remove noise, and resampling to ensure a consistent sampling rate.
Core Audio Fingerprinting Algorithms
Spectrogram Analysis
A spectrogram provides a visual representation of the spectrum of frequencies of a signal as they vary with time. It is instrumental for audio fingerprinting as it highlights the unique frequency components.
To generate a spectrogram in MATLAB, use the following code snippet:
[audioData, fs] = audioread('your_audio_file.mp3'); % Load audio file
spectrogram(audioData, 256, [], [], fs, 'yaxis'); % Generate the spectrogram
title('Spectrogram of Audio Signal');
This command splits the audio data into overlapping segments (256 samples in this case) and provides a visual representation of the audio signal.
Feature Extraction Methods
Key features used in audio fingerprinting include:
-
Mel-Frequency Cepstral Coefficients (MFCC)
MFCCs capture the timbral characteristics of audio relevant for speech and music recognition. They convert the frequency spectrum into a representation that more closely resembles how humans perceive sound.
To extract MFCC features in MATLAB, you can use this code:
coeffs = mfcc(audioData, fs); % Compute MFCCs melSpectrogram(audioData, fs); % Visualize the mel spectrogram
-
Chroma Features
Chroma features highlight the pitch content of auditory signals and are particularly useful in music analysis.
Calculate chroma features with the following command:
chroma = chromaFeatures(audioData, fs); % Extract chroma features
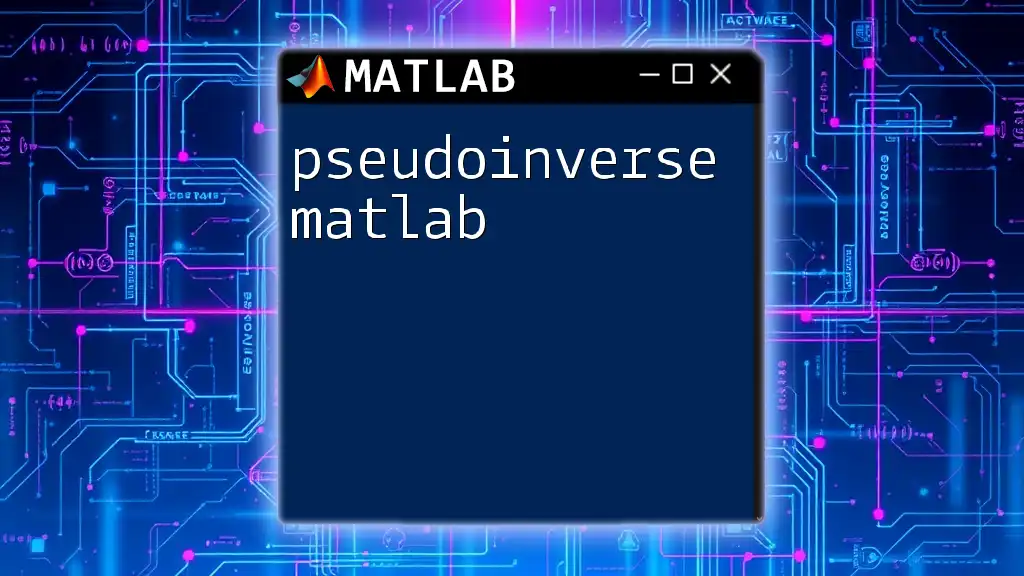
Implementing Audio Fingerprinting in MATLAB
Setting Up Your MATLAB Environment
Before you can start building your audio fingerprinting system in MATLAB, ensure you have all the necessary toolboxes installed, specifically the Signal Processing Toolbox. To install any required packages, access MATLAB's Add-On Explorer and search for the Signal Processing Toolbox.
Step-by-Step Guide to Building an Audio Fingerprinting System
Data Collection
Begin by identifying a dataset of audio files for your project. Several public datasets are available, such as the Million Song Dataset or Free Music Archive. Once you've chosen your dataset, you can load audio files into MATLAB for processing.
[audioData, fs] = audioread('path_to_audio_file.wav'); % Load audio file
Feature Extraction
Next, implement feature extraction using the earlier discussed methods. Features extracted from audio provide the foundational elements for building an audio fingerprinting model.
mfccFeatures = mfcc(audioData, fs); % Extract MFCC features
chromaFeatures = chromaFeatures(audioData, fs); % Extract Chroma features
These feature sets will serve as the building blocks for your audio fingerprint.
Fingerprint Construction
The extracted features must be converted into a unique fingerprint for matching. Techniques like hashing and quantization are typically used here to create a compact representation of the audio.
A simple approach to construct an audio fingerprint could include combining the features, followed by applying a hashing function:
fingerprint = hash(mfccFeatures + chromaFeatures); % Simple hash function example
Matching and Identifying Audio
Once you've constructed a fingerprint, the next step is to develop a matching algorithm that can identify audio files. A common method for matching audio fingerprints is using a nearest neighbor search approach.
For instance:
distance = pdist2(fingerprint, databaseFingerprints); % Calculate distance from database
This command calculates the Euclidean distance between the generated fingerprint and those stored in your database, allowing you to find the closest match effectively.
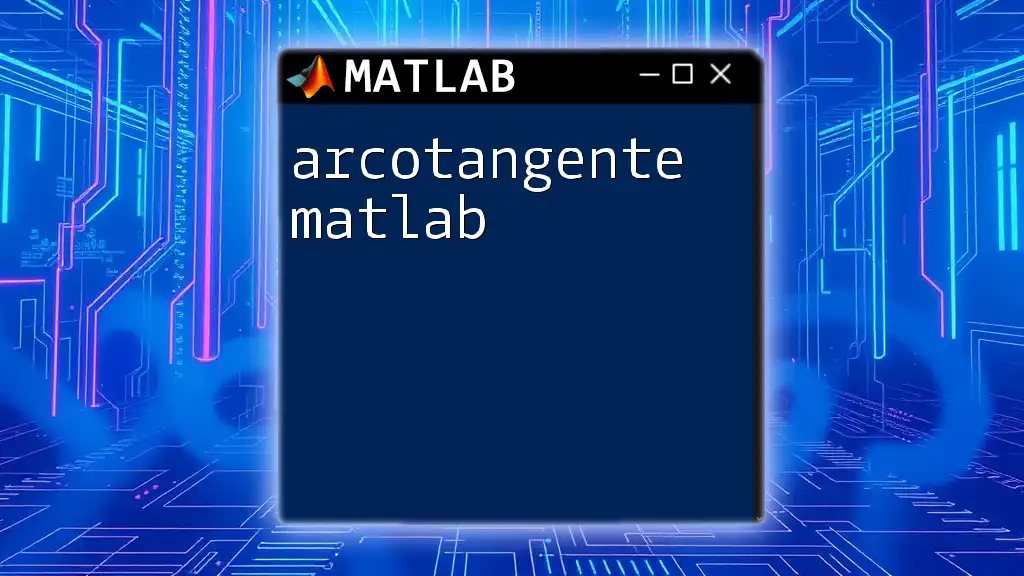
Real-World Applications and Case Studies
Successful Implementations
Audio fingerprinting has been successfully implemented by numerous companies and research institutions. For instance, applications like Shazam utilize advanced audio fingerprinting to identify songs quickly. SoundCloud leverages these techniques to assist in copyright detection for user-uploaded content.
Case Studies
Several studies have shown the effectiveness of audio fingerprinting, such as using Dan Ellis’ algorithms to identify clips in noisy environments or analyze musical tracks for unintentional copyright violations.
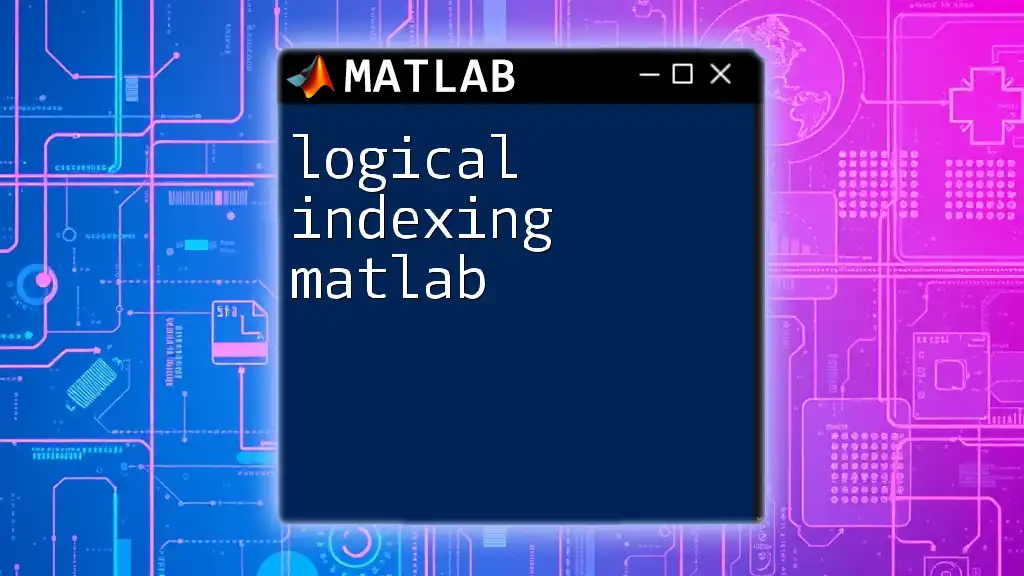
Challenges in Audio Fingerprinting
Common Issues and Solutions
Despite its effectiveness, audio fingerprinting is not without challenges. Common issues include:
-
Noise Handling: Background noise can skew the accuracy of fingerprints. Implementing noise reduction techniques during preprocessing can help mitigate this issue.
-
Diverse Audio Qualities: Variability in audio quality affects feature extraction. Standardizing audio input and using robust feature extraction methods can address this challenge.
-
Latency in Processing: Real-time audio fingerprinting requires swift algorithms. Optimizing code and reducing computational overhead is key to ensuring rapid identification.
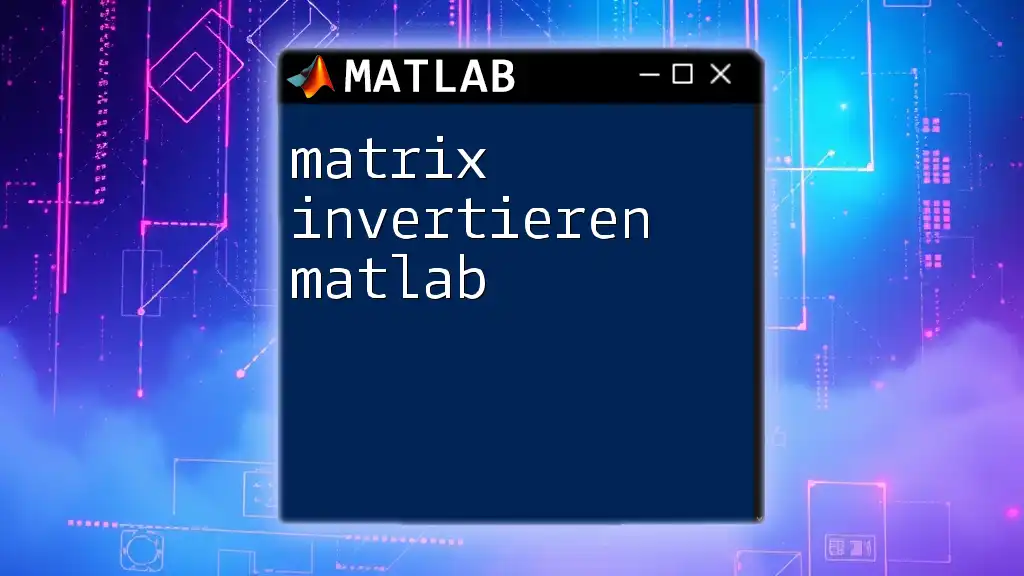
Conclusion
Building an audio fingerprinting system using MATLAB based on Dan Ellis' techniques is not only feasible but also an excellent way to explore audio processing fundamentals. The combination of feature extraction, fingerprint construction, and matching algorithms creates a solid framework for identifying audio content. As technology advances, so do the methods of audio recognition, presenting exciting opportunities for future development.
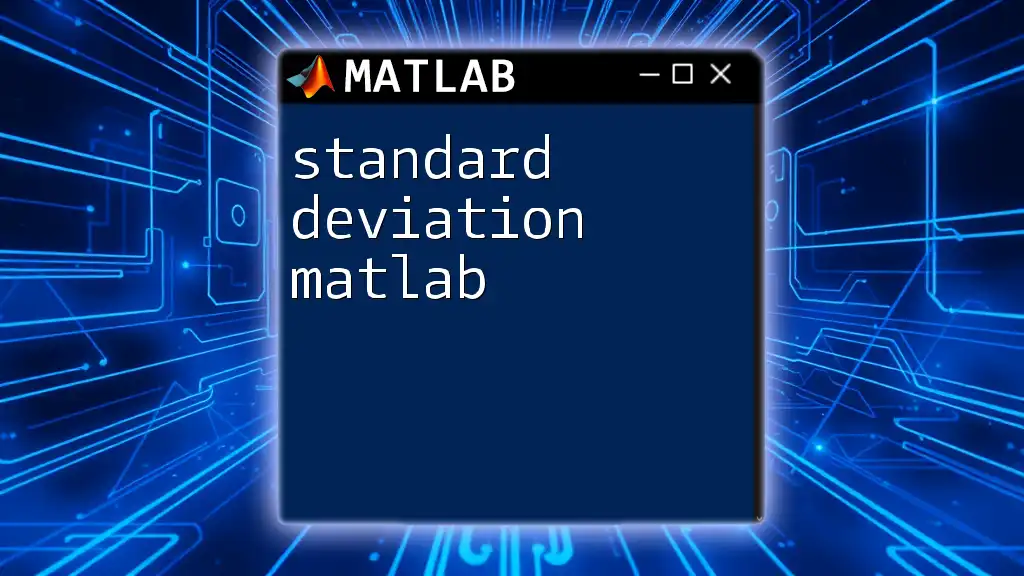
Additional Resources
For those interested in further exploring audio fingerprinting, consider diving into online resources, advanced courses, or textbooks dedicated to audio signal processing. Notably, Dan Ellis’ published papers provide deep insights into his methodologies and their practical applications. Engaging with such materials will enrich your understanding and stimulate innovative approaches to audio engineering challenges.
Now, practice implementing the techniques discussed in this article, and experiment with building your own audio fingerprinting systems in MATLAB!