The Generalized Voronoi Diagram in MATLAB allows you to create partitioned areas based on a weighted distance to a set of generator points, facilitating tasks in spatial analysis and optimization.
Here's a simple code snippet for generating a Generalized Voronoi Diagram in MATLAB:
% Define generator points and weights
P = [1, 2; 3, 4; 5, 1]; % Generator points
weights = [1; 2; 0.5]; % Corresponding weights
% Plotting settings
figure; hold on;
scatter(P(:,1), P(:,2), 'filled', 'MarkerFaceColor', 'r');
% Create and plot Voronoi Diagram
[vx, vy] = voronoi(P(:,1), P(:,2));
plot(vx, vy, 'b-');
% Set axis limits
xlim([0 6]); ylim([0 5]);
title('Generalized Voronoi Diagram');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
hold off;
This code defines three generator points with specific weights, plots the Voronoi diagram, and visualizes it within a coordinate system.
What is a Voronoi Diagram?
A Voronoi diagram is a partitioning of a plane into regions based on the distance to a specific set of points, known as sites or seeds. Each region corresponds to one site and comprises all points closer to that site than to any other. The significance of Voronoi diagrams stretches across various fields such as urban planning, resource management, and geographical analysis. Their ability to visually depict spatial relationships makes them an invaluable tool for decision-making processes that require geographical reasoning.
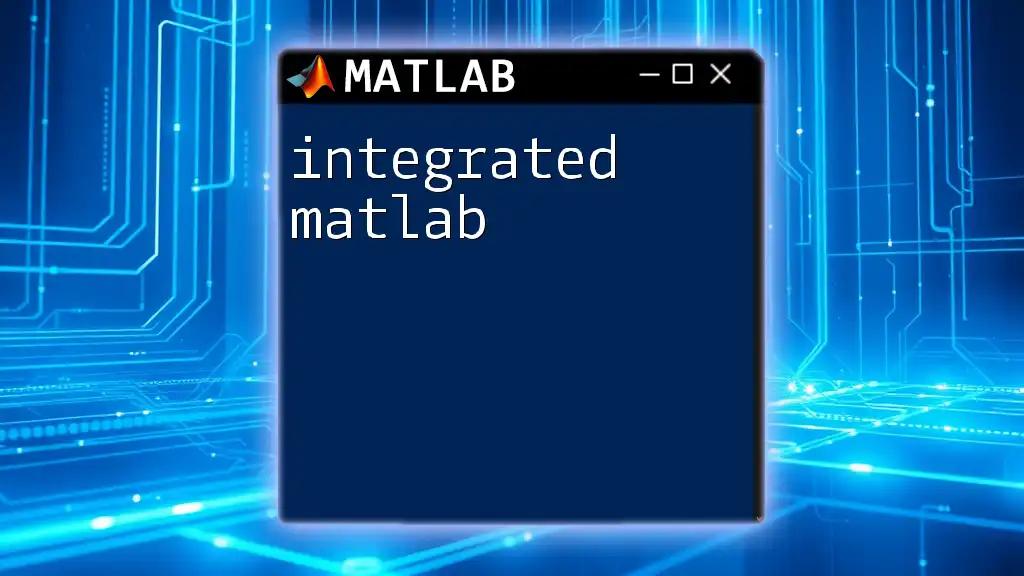
What is a Generalized Voronoi Diagram (GVD)?
A Generalized Voronoi Diagram (GVD) extends the concept of traditional Voronoi diagrams by allowing for variations in the distance metrics used to calculate the regions. Unlike standard Voronoi diagrams that typically use the Euclidean distance, GVDs can leverage other distance metrics, making them suitable for complex applications where different distances reflect real-world constraints more accurately. The key difference lies in their ability to accommodate an expanded range of site types and distance functions, making the analysis highly flexible and adaptable.
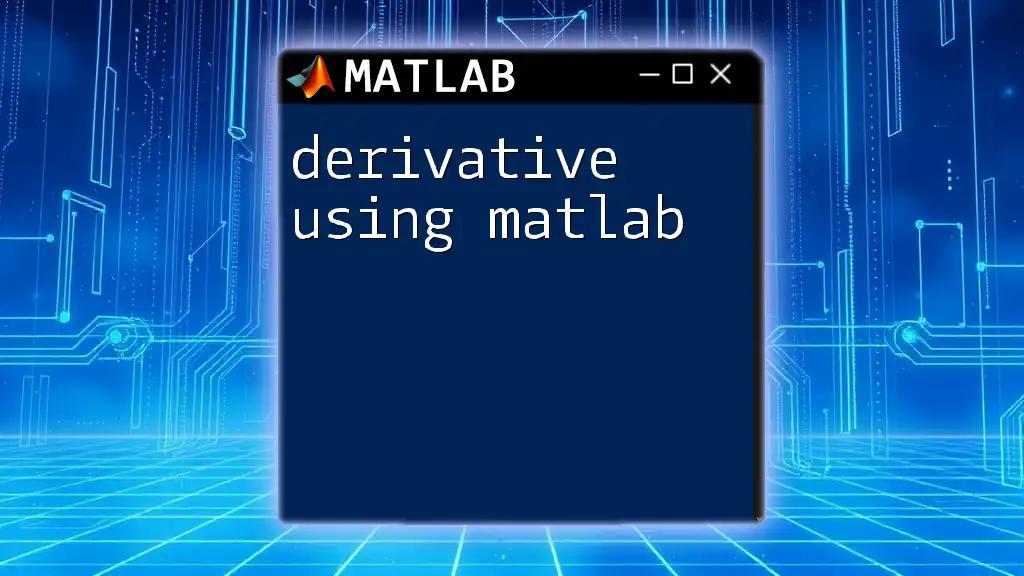
Prerequisites for Using MATLAB
Overview of MATLAB
MATLAB is a powerful computational environment widely utilized for mathematical analysis, data visualization, and algorithm development. With its rich set of built-in functions and extensive libraries, MATLAB empowers users to solve complex mathematical problems and effectively visualize the results. For tasks involving generalized Voronoi diagrams, its functionalities can greatly streamline the coding and computation process.
Necessary Toolboxes
To take full advantage of MATLAB’s capabilities, certain toolboxes may be necessary. Some key toolboxes include:
- Image Processing Toolbox: Helps in image-related computations that may arise in GVD applications.
- Parallel Computing Toolbox: Enhances performance for large datasets by utilizing multiple cores.
You can verify the installed toolboxes using the command:
ver
To install a toolbox, navigate to the MATLAB Add-On Explorer.
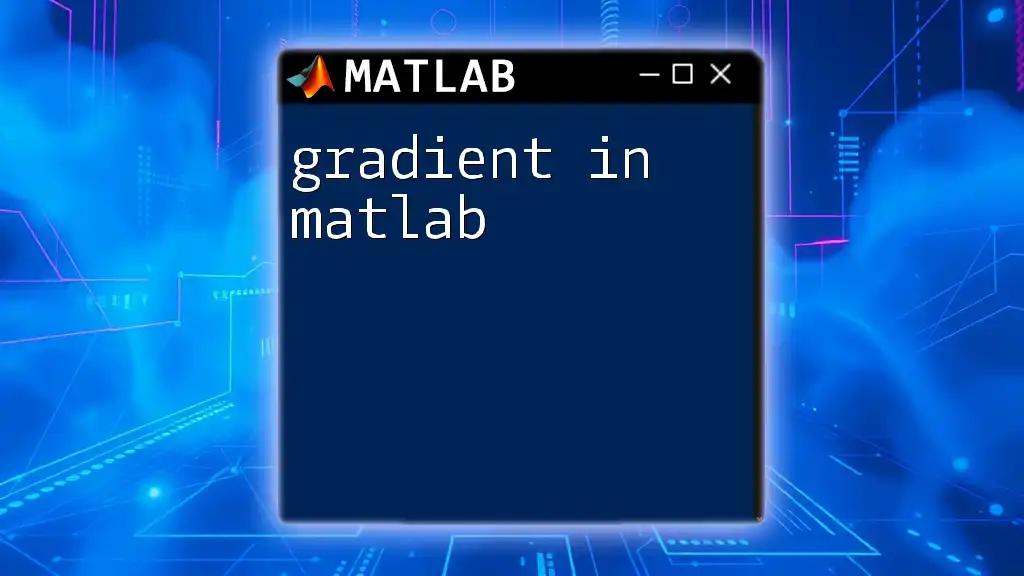
Setting Up the MATLAB Environment
Installing MATLAB
If you haven't installed MATLAB yet, you can follow these simple steps:
- Visit the official [MATLAB website](https://www.mathworks.com/downloads/) and select your version.
- Follow the on-screen instructions to download and install the software.
For optimal performance, it’s recommended to use the latest version available.
Creating a New Script for GVD
Once MATLAB is installed, you can initiate a new script for your GVD implementation. To create a new script:
- Open MATLAB and click on "New Script" in the Home tab.
- Set up your script with proper headings and comments to organize the content:
%% Generalized Voronoi Diagram Implementation
clc; clear; close all; % Clear the command window, workspace, and close figures
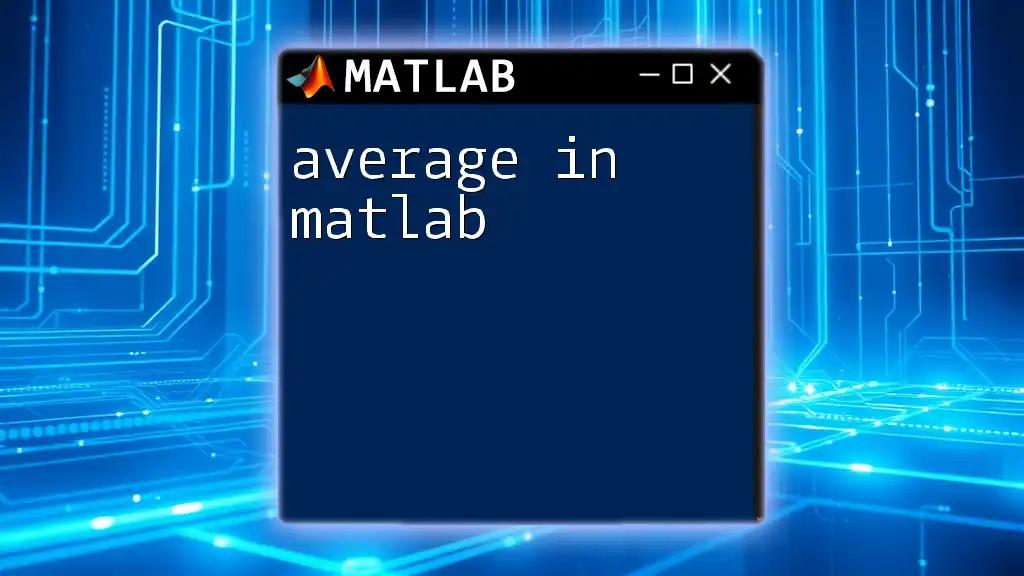
Basic Concepts of Voronoi Diagrams in MATLAB
Generating Standard Voronoi Diagrams
To familiarize yourself with Voronoi diagrams, you can generate a basic example in MATLAB. Here’s how:
points = rand(10, 2); % Generate random points
voronoi(points(:,1), points(:,2));
title('Standard Voronoi Diagram');
axis equal;
This code snippet creates a Voronoi diagram for 10 random points. The output visualizes the spatial partitions, demonstrating how each point influences its respective area.
Introduction to Generalized Voronoi Diagrams
The construction of Generalized Voronoi Diagrams begins by analyzing what sets them apart from standard ones. A fundamental aspect is the flexibility in defining the distance metric. Traditional Voronoi diagrams rely solely on the Euclidean distance, while GVDs can leverage various customizable distance functions. This enables researchers and practitioners to model more complex relationships in spatial data.
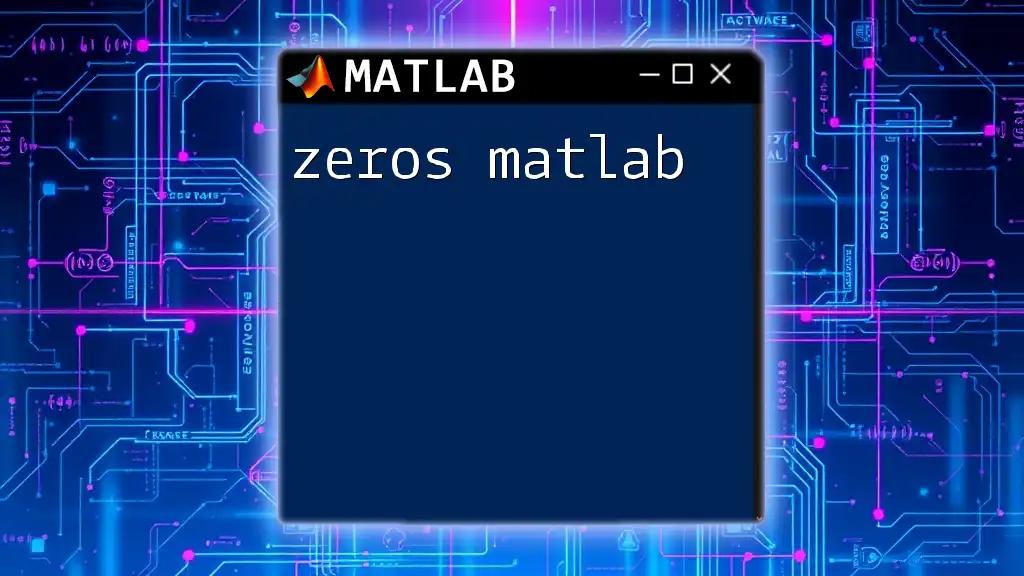
Implementing Generalized Voronoi Diagrams in MATLAB
Custom Distance Metrics
To utilize a custom distance metric in your GVD, you will first need to define a distance function. This is crucial as it allows you to tailor the GVD to fit specific application needs. For example, you can define a custom distance as follows:
function d = customDistance(p1, p2)
% Example of a custom distance metric (Manhattan distance)
d = sum(abs(p1 - p2));
end
Implementing this function enables you to modify the generalized Voronoi algorithm to calculate regions based on this new metric.
GVD Construction Algorithm
Input Data Preparation
Before constructing a GVD, it's essential to prepare your input data properly. This may include normalizing the data to ensure consistent scaling across different dimensions, which helps in achieving reliable results.
Algorithm Steps
The algorithm for generating a Generalized Voronoi Diagram typically involves:
- Choosing a set of points (sites).
- Calculating regions based on the defined distance metric.
- Visualizing the resulting GVD.
Let’s explore a conceptual algorithm and corresponding MATLAB code snippet:
function [V, C] = generalizedVoronoi(data_points, distFunc)
% Initial setup; preallocate output
V = []; % Voronoi vertices
C = {}; % Cell array to contain each region
% Implementation of GVD
% Iterate through every pair of points, compute distances
for i = 1:size(data_points, 1)
% Add distance calculations using distFunc
% Logic to define regions based on distance below:
for j = 1:size(data_points, 1)
if i ~= j
% Custom logic goes here
end
end
end
% Your code for assigning Voronoi vertices and vertices to each region would go here
end
Example: Generating a GVD
To see the GVD in practice, here’s a full MATLAB code example that constructs and visualizes a generalized Voronoi diagram:
data_points = rand(15, 2); % Example input
% Custom function calling
[V, C] = generalizedVoronoi(data_points, @customDistance); % Use predefined custom distance function
% Visualization of the GVD
figure;
plotV = voronoi(data_points); % Visualization
title('Generalized Voronoi Diagram');
axis equal;
This code creates a random set of points and displays the GVD accordingly, providing insights into how different regions are formed based on the custom metrics and input data.
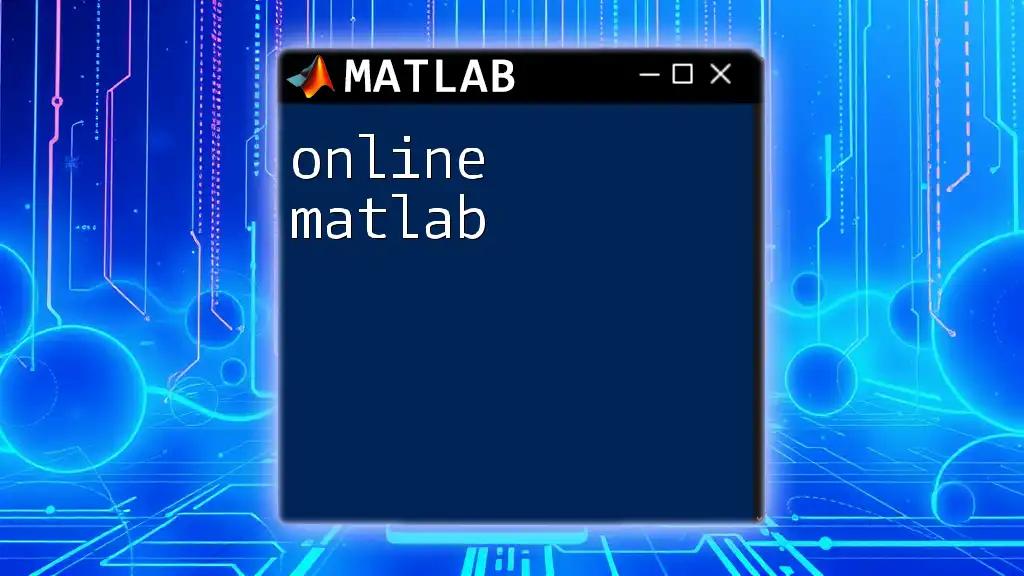
Visualization Techniques for GVDs
Using MATLAB’s Built-in Plotting Functions
MATLAB offers an array of built-in plotting functions to enhance the visualization of GVDs. For customizing your plots, consider changing aspects like colors, marker types, and adding legends for clarity. A simple modification can be made with:
plot(data_points(:,1), data_points(:,2), 'ro'); % Red dots for data points
Exporting Visuals
To share your results or include them in presentations, exporting diagrams to different file formats is essential. You can save your visualizations easily with the following command:
saveas(gcf, 'GVD.png');
Make sure to adjust the file format according to your presentation or reporting needs.
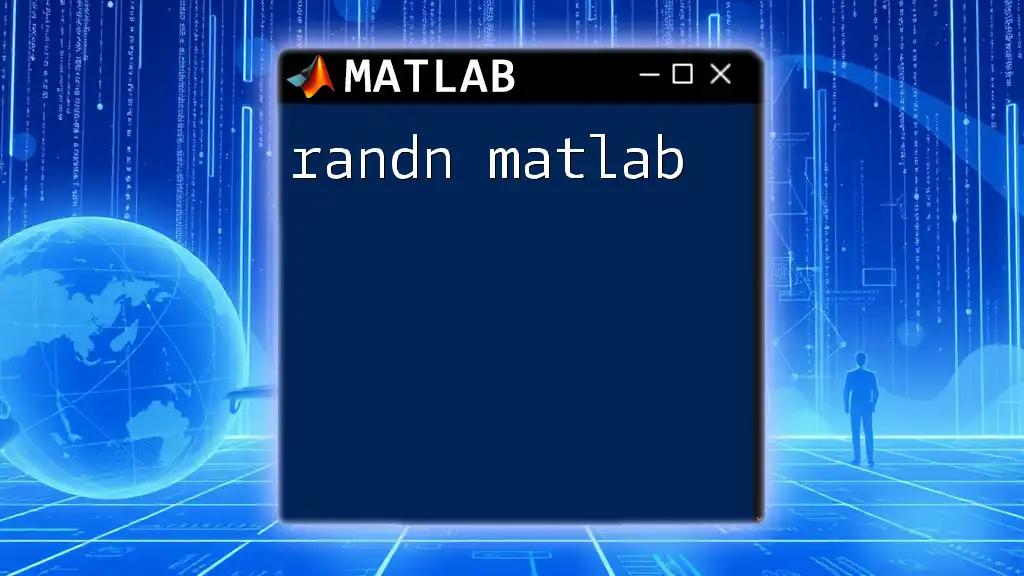
Practical Applications of Generalized Voronoi Diagrams
Case Study: Urban Planning
In urban planning, GVDs can aid in making decisions related to resource allocation, such as utility placements or emergency services. By analyzing spatial relationships through GVDs, planners can ensure equitable access to resources across different districts, ultimately optimizing city infrastructure.
Case Study: Computational Biology
In computational biology, GVDs are instrumental for modeling cellular structures and interactions. For example, they can assist in simulating cell growth and distributing nutrients in a manner that reflects the proximity influences observed in biological systems. Implementing GVD algorithms on biological data can reveal intricate patterns and enhance our understanding of these complex systems.
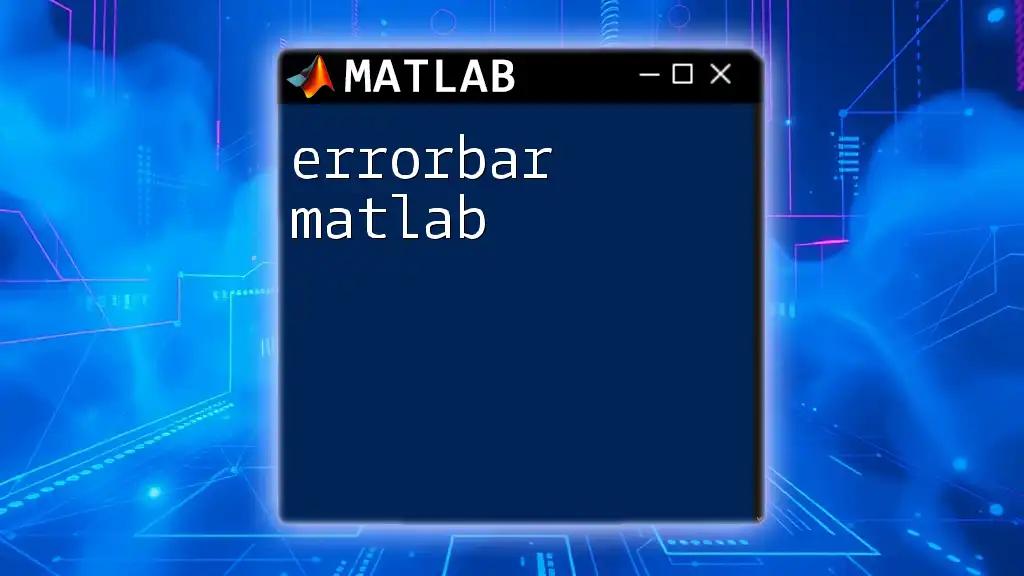
Conclusion
This guide provided a comprehensive exploration of generalized Voronoi diagrams in MATLAB. You learned not only about the definitions and differences between Voronoi types but also how to implement custom distance metrics, visualize results, and analyze real-world applications.
Encouragement for Further Exploration
As you grow more comfortable with GVDs, consider diving deeper into combining them with other algorithms or exploring more complex distance metrics. Abundant resources, including MATLAB documentation and online communities, await to support your continued growth in this fascinating field.
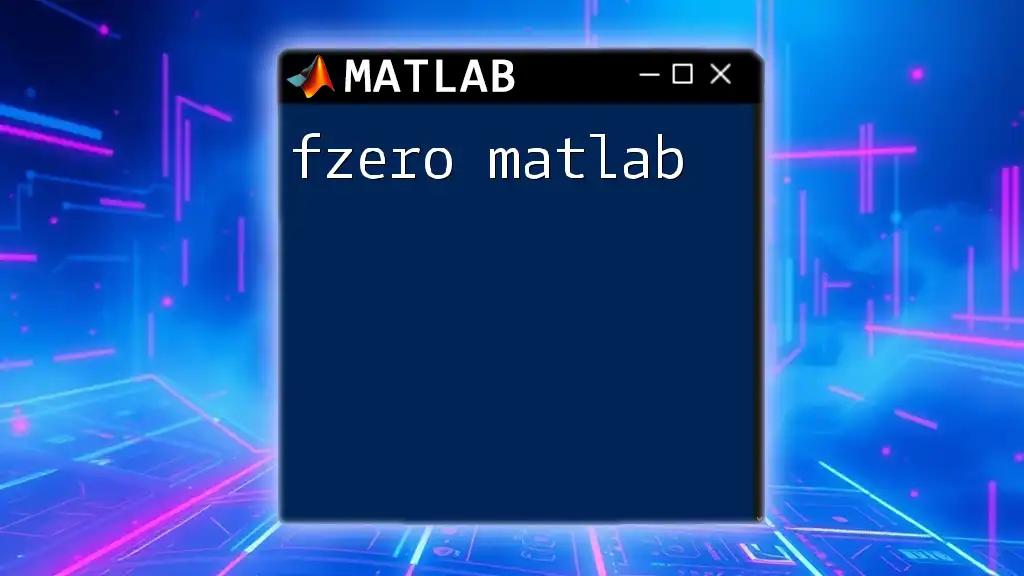
Additional Resources
References and Further Reading
For further insights and advanced applications related to GVDs, explore academic papers or articles available in libraries and online databases. The MATLAB documentation is also an excellent resource for extensive guidance on specific commands and functionalities.
Community and Help
Joining MATLAB communities can provide invaluable support and insights. Engaging in forums allows you to ask questions, share experiences, and collaborate with others in the field.
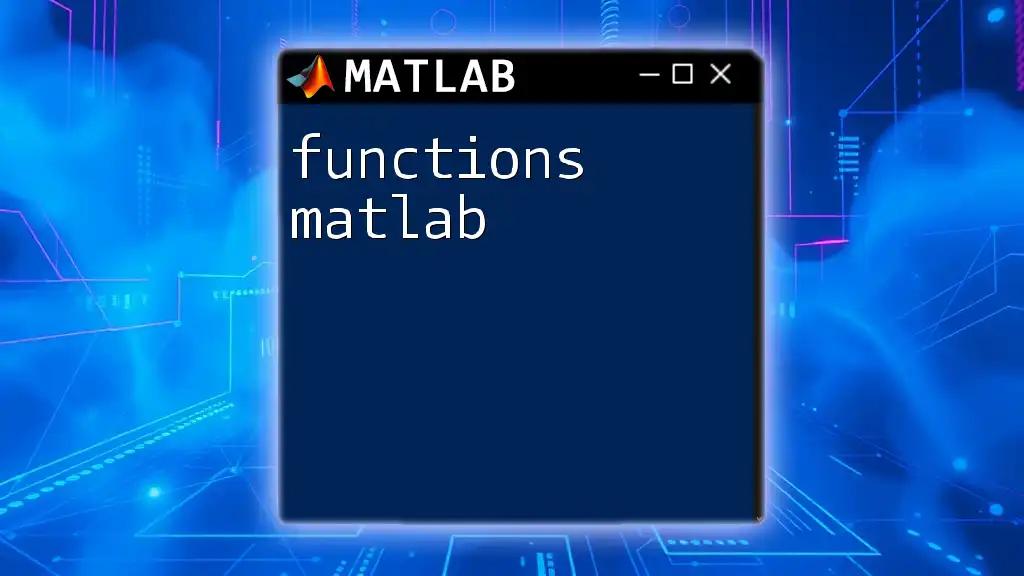
Call to Action
If you found this guide valuable, consider subscribing to my updates on future posts addressing MATLAB commands and techniques. By staying updated, you can deepen your expertise and possibly discover new ways to apply your knowledge.
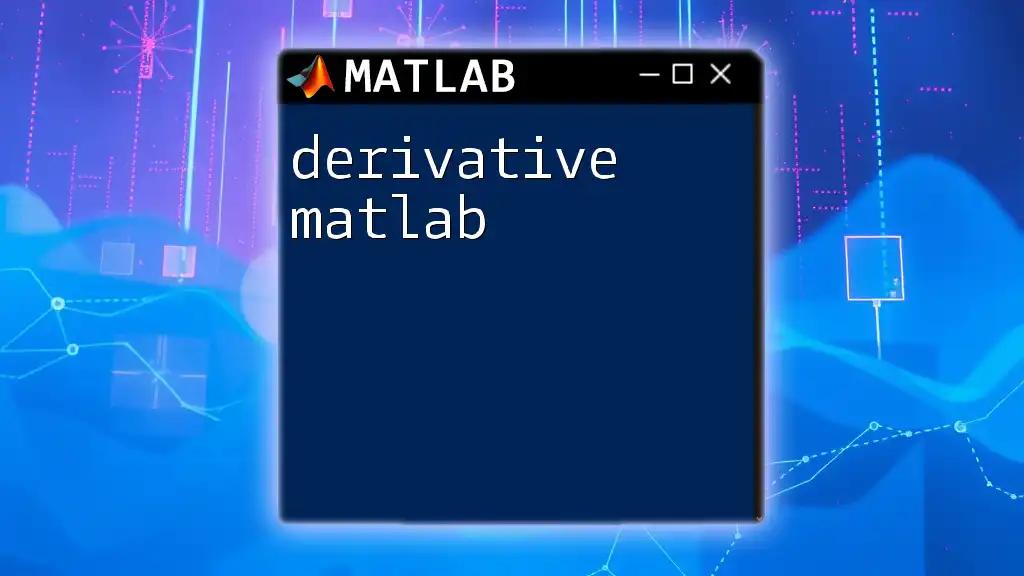
Feedback and Engagement
I invite you to leave your comments below, sharing your own experiences with generalized Voronoi diagrams in MATLAB. What challenges have you faced? What projects are you working on? Let’s engage and learn together!