The `readtable` function in MATLAB is used to import data from a file into a table, making it easy to manipulate and analyze the data in a structured format.
data = readtable('filename.csv');
Understanding Tables in MATLAB
What is a Table?
In MATLAB, a table is a type of data structure that allows for the storage of different types of data (numeric, text, etc.) within a single entity. Each column in a table can contain variables of different types, making it a versatile tool for handling mixed data. Tables come in handy when dealing with datasets where information is organized in rows and columns, much like a spreadsheet.
Why Use Tables?
Using tables in MATLAB provides several advantages:
- Structured Data Management: Tables automatically manage the column headers, making them easy to read and reference.
- Enhanced Data Analysis: Tables work seamlessly with many built-in MATLAB functions, particularly those that require structured data.
- Categorical Data Handling: Tables can contain categorical data, enabling users to perform advanced statistical analysis efficiently.
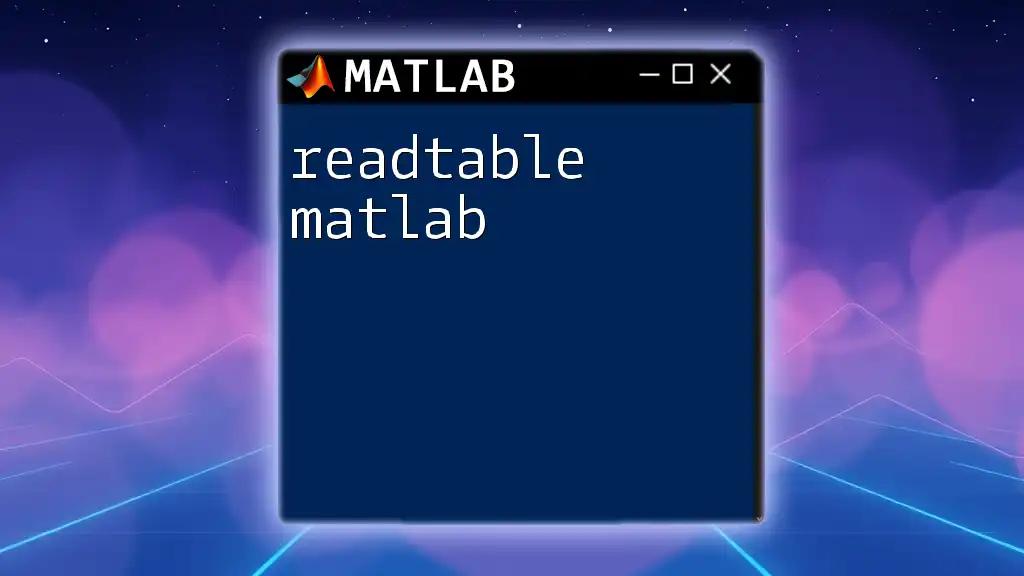
Loading Tables in MATLAB
Overview of Table Loading Techniques
There are various ways to load tables into MATLAB, with a variety of functions designed to suit different data formats. When looking to import data, one of the simplest yet most powerful functions is `readtable`.
Using `readtable` Function
The `readtable` function is primarily used to import data from text files, spreadsheets, and databases into a table. It infers datatypes automatically, making it user-friendly.
Syntax:
T = readtable(filename)
For example, if you have a CSV file named `data.csv`, you can easily read it into a table:
T = readtable('data.csv');
Explanation: This simple line of code imports the data from the specified CSV file and stores it into a table named `T`. Now, you can manipulate or analyze `T` just like any other MATLAB variable.
Other File Types Supported by `readtable`
The `readtable` function can handle various file types aside from CSV files:
-
Excel Files: You can directly read `.xls` and `.xlsx` files, seamlessly integrating data stored in spreadsheets.
-
Text Files: The function can also manage tab-delimited text files (`.txt`), providing flexibility in data importation.
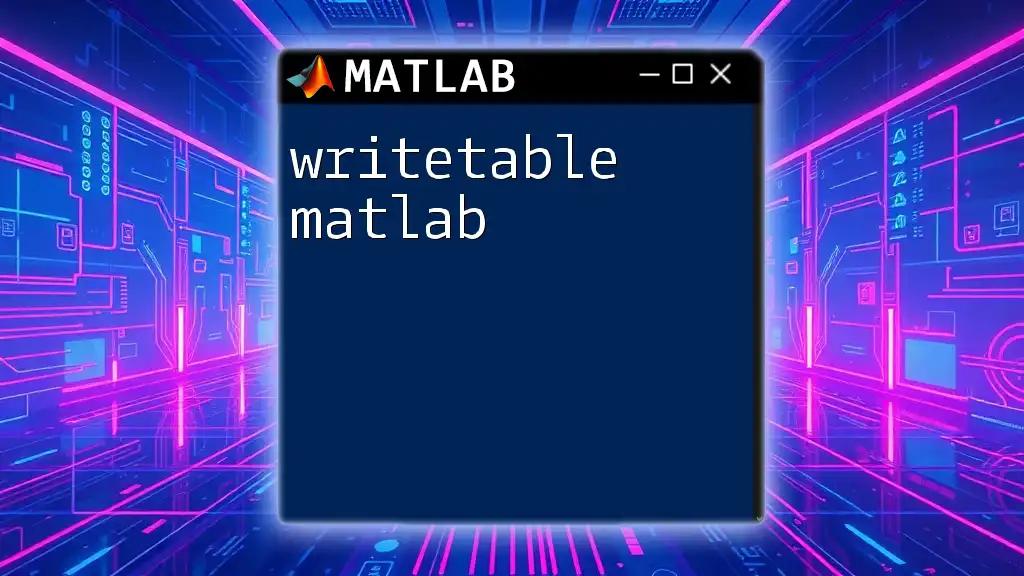
Customizing Data Import
Specifying Variable Names
When dealing with datasets, you might want to assign custom names to your variables for better clarity. Here’s how you can do it using `readtable`:
opts = detectImportOptions('data.csv');
opts.VariableNames = {'ID', 'Name', 'Score'};
T = readtable('data.csv', opts);
In this example, `opts.VariableNames` allows you to define the headers for the columns explicitly, aiding in data organization upon loading.
Handling Missing Data
Missing values are common in datasets. To manage this, you can configure the import options accordingly:
opts = detectImportOptions('data.csv');
opts.MissingValue = 'NA'; % Specify how to handle missing values
T = readtable('data.csv', opts);
This approach informs MATLAB to treat 'NA' as a missing value during the loading process, ensuring data integrity.
Selecting Rows and Columns During Import
If you're dealing with large datasets and only need specific parts, `readtable` has options for selective loading:
opts = detectImportOptions('data.csv');
opts.SelectedVariableNames = {'ID', 'Score'};
T = readtable('data.csv', opts);
This snippet focuses on only the `ID` and `Score` columns from the original dataset, streamlining your analysis.
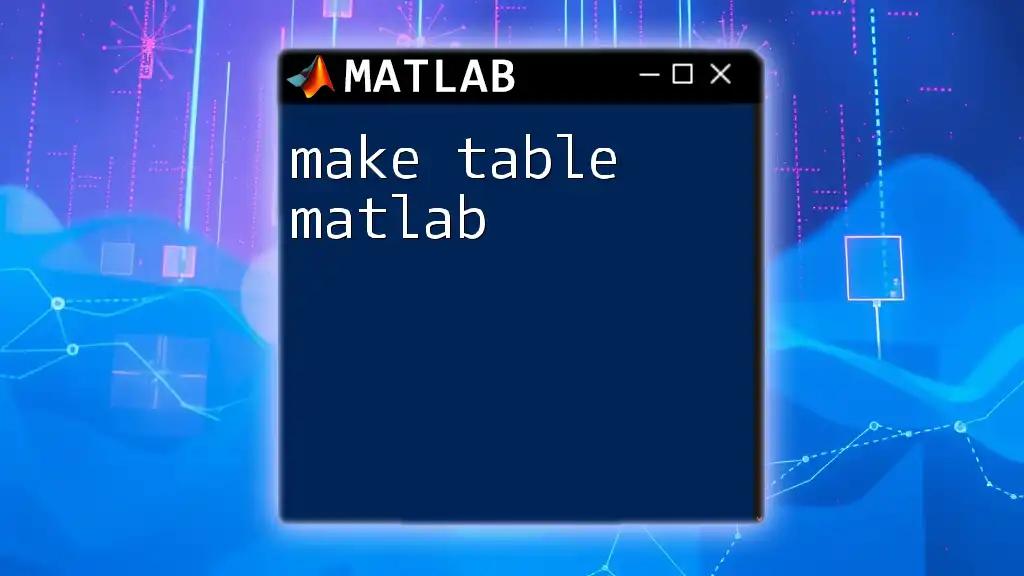
Exploring the Imported Table
Inspecting the Table Structure
Once you have loaded your data into a table, it's essential to inspect its structure to understand its contents better. You can use the following functions:
head(T)
tail(T)
summary(T)
- `head(T)` provides a glimpse of the first few rows.
- `tail(T)` displays the last few rows.
- `summary(T)` gives a concise overview of the variables, highlighting data types and any missing values.
Accessing Data in a Table
MATLAB allows you to easily navigate through your table to access specific data:
scores = T.Score; % Accessing the 'Score' column
row1 = T(1, :); % Accessing the first row
This code retrieves the entire `Score` column and the first row of the table, empowering you to manipulate and analyze specific segments of your dataset.
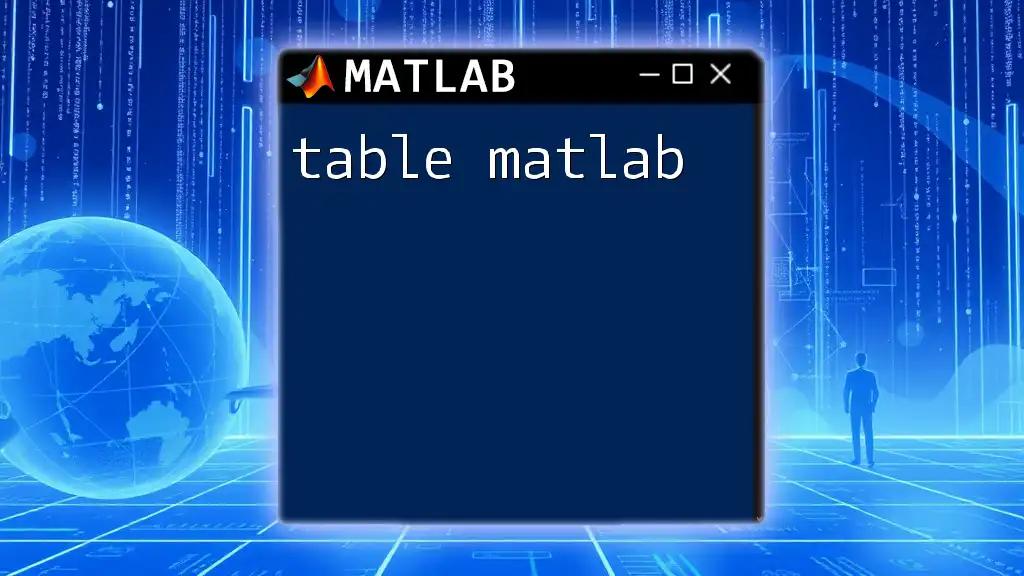
Modifying and Updating Tables
Adding New Variables
With MATLAB tables, you can enrich your dataset by adding new columns:
T.PassFail = T.Score >= 60; % Adding a logical column based on scores
Here, a new column `PassFail` is created, indicating whether each score meets the passing threshold (in this case, 60).
Removing Variables
In case you no longer need specific data, you can easily remove unnecessary columns from your table:
T.PassFail = []; % Removing the 'PassFail' column
This straightforward command clears the `PassFail` column from the table, ensuring your data remains relevant.
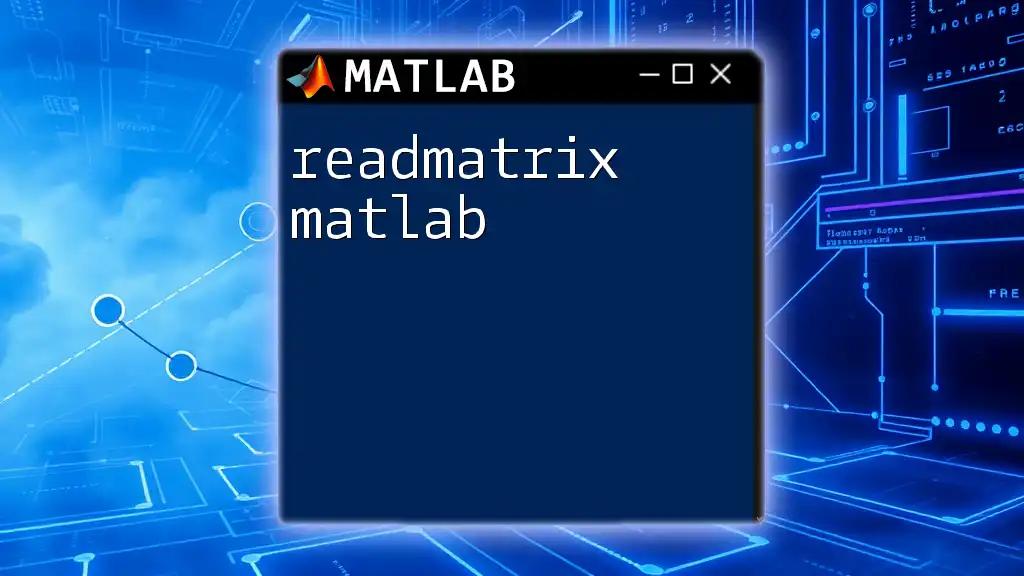
Saving Tables
Writing Tables to Files
To export your modified table back into a file format for future use, you can utilize `writetable`:
writetable(T, 'output.csv');
This command saves the table `T` to a new CSV file called `output.csv`, making the data available for sharing or further analysis.
Specifying Options for Writing
While saving tables, you can customize export options:
writetable(T, 'output.csv', 'WriteRowNames', true);
By including `WriteRowNames`, the row names of your table are saved as well, preserving data context for future reference.
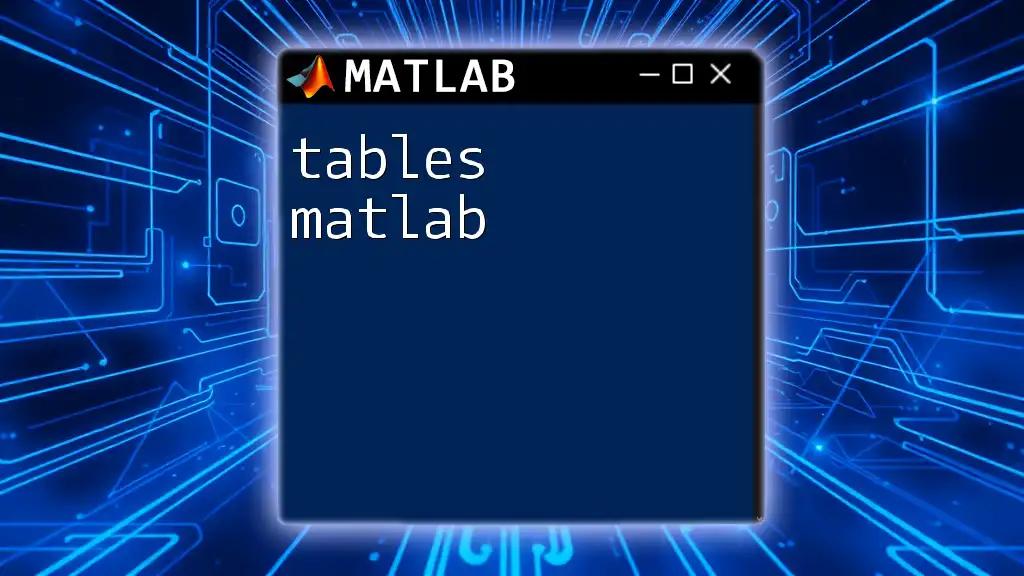
Troubleshooting Common Issues
Errors in Reading Tables
When using `readtable`, you might encounter errors or warnings relating to file formats or data types. Common issues include misaligned columns or unexpected datatypes. To avoid this, always ensure your data files are clean and conform to expected structures before importing.
Performance Considerations
When dealing with large datasets, the reading process can be time-consuming. Using options like `ImportOptions` can help streamline this process by limiting the amount of data being read or precisely defining the structure of the data being imported.
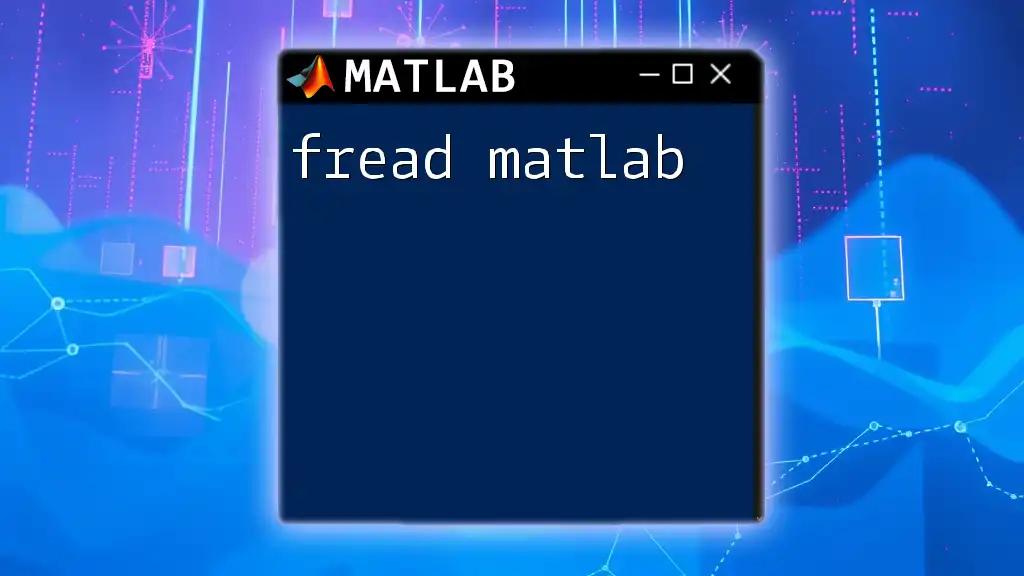
Conclusion
In this comprehensive guide, we explored the MATLAB command to read tables, delving into the functionalities of `readtable`, customizing imports, accessing and modifying table data, and finally saving your tables. Understanding how to effectively utilize tables not only enhances your data handling skills in MATLAB but also opens the door to more advanced data analysis techniques. Don’t hesitate to practice these concepts in your coding sessions to solidify your skills and confidence in using tables in MATLAB!
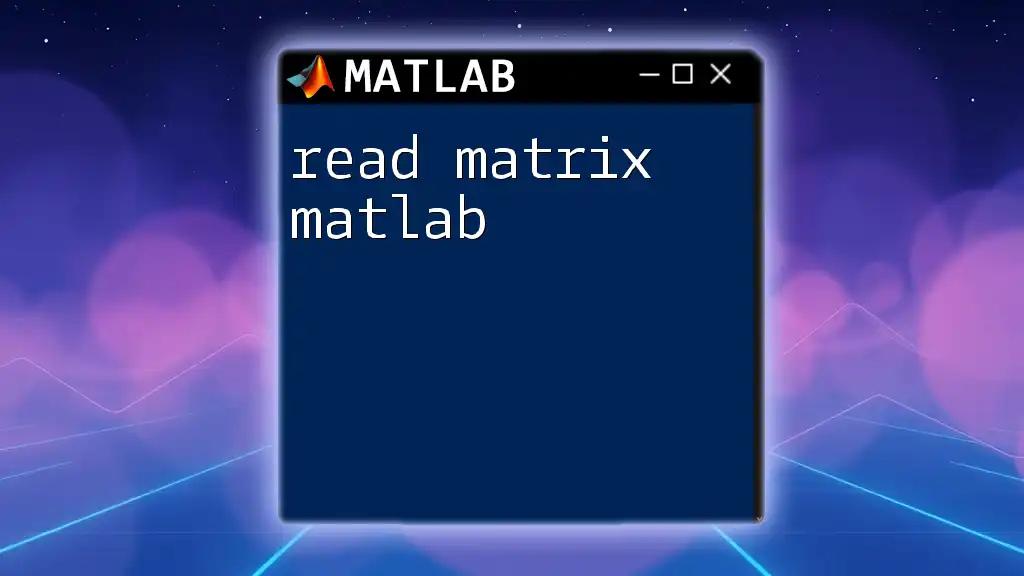
Additional Resources
For further learning and exploration of MATLAB tables, you can refer to the official MATLAB documentation and engage with tutorials that focus on practical exercises. The hands-on application will enrich your command of "read table matlab" and its associated functionalities.