The inner product in MATLAB, also known as the dot product, computes the sum of the products of the corresponding entries of two vectors, and can be easily performed using the `dot` function or the `*` operator for matrix multiplication.
% Example of computing the inner product using the dot function
A = [1, 2, 3];
B = [4, 5, 6];
innerProduct = dot(A, B);
% Alternatively, using the * operator
innerProduct2 = A * B'; % B must be transposed for this to work
What is an Inner Product?
The inner product is a fundamental concept in linear algebra, providing a way to define the angle between two vectors and the notion of length in vector spaces. Mathematically, it can be represented as follows:
For two vectors \( \vec{A} = [A_1, A_2, \ldots, A_n] \) and \( \vec{B} = [B_1, B_2, \ldots, B_n] \), the inner product is defined as:
\[ \langle \vec{A}, \vec{B} \rangle = A_1B_1 + A_2B_2 + \ldots + A_nB_n \]
The inner product possesses several important properties such as commutativity and linearity. It forms the foundation for numerous applications in fields like geometry, data science, and physics, enabling researchers to quantify the relationship between data points.
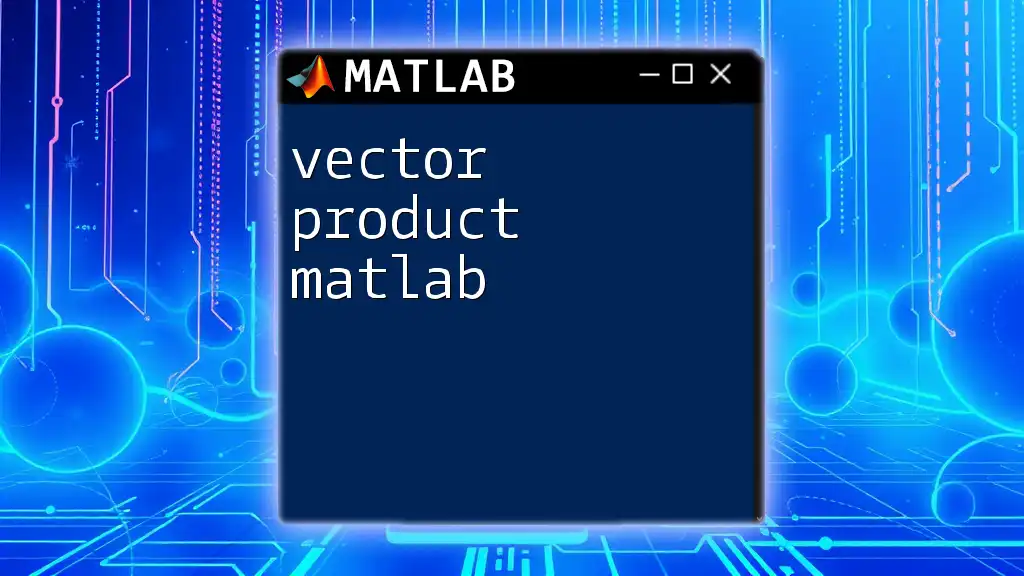
Applications of Inner Product
The inner product is not only a theoretical construct but also a practical tool. Here are some applications where the inner product plays a crucial role:
- Machine Learning: In algorithms such as Support Vector Machines (SVM), the inner product helps measure the similarity between data points, essential for classification tasks.
- Signal Processing: Inner products are used to determine the correlation between signals, allowing for effective filtering and analysis.
- Physics: Calculating work done, projections of vectors, and more require understanding the inner product of force and displacement vectors.
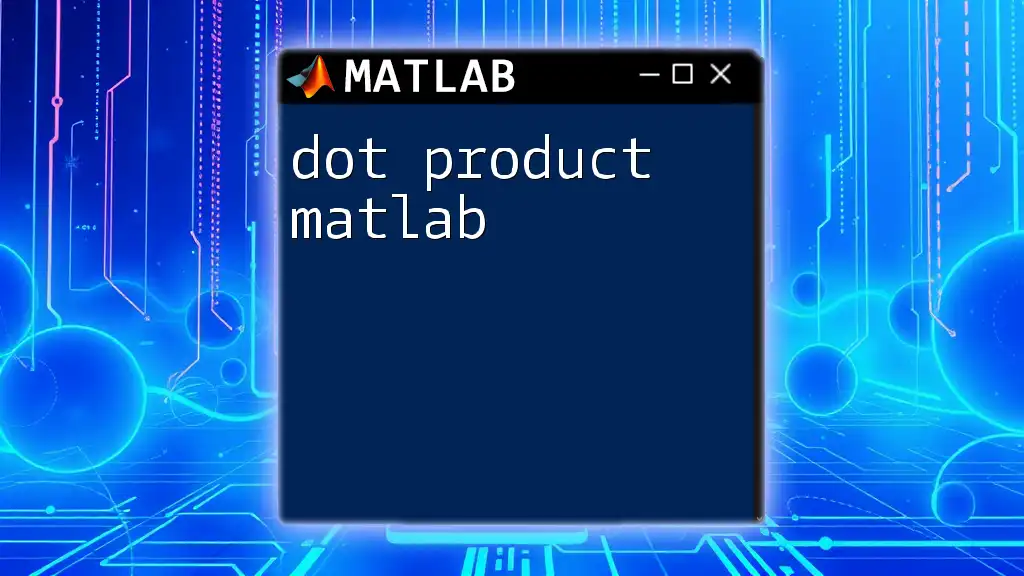
Understanding Vectors and Matrices in MATLAB
Basics of Vectors in MATLAB
In MATLAB, vectors can be easily created using syntax such as:
A = [1, 2, 3]; % Row vector
B = [4; 5; 6]; % Column vector
Vectors are ordered collections of numbers, and MATLAB distinguishes between row and column vectors based on their orientation.
Matrix Representation
A matrix is an extension of a vector that allows for two-dimensional data storage. The basic structure of a matrix in MATLAB is built by arranging numbers in rows and columns:
C = [1, 2, 3; 4, 5, 6]; % A 2x3 matrix
While vectors are a special case of matrices (1D matrices), understanding the two-dimensional structure is essential for applying inner products, especially in more advanced calculations.
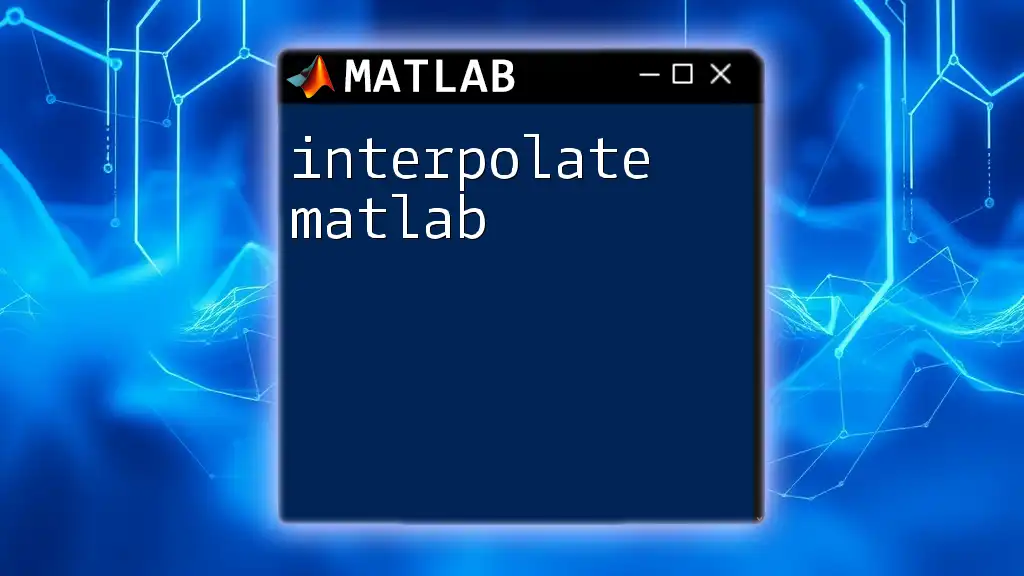
The Mathematical Foundation of Inner Product
Geometric Interpretation
In geometry, the inner product provides insight into the angle between two vectors. If the inner product is zero, it signifies that the vectors are orthogonal (perpendicular). The relationship is captured by the formula:
\[ \langle \vec{A}, \vec{B} \rangle = ||\vec{A}|| \cdot ||\vec{B}|| \cdot \cos(\theta) \]
Here, \( ||\vec{A}|| \) and \( ||\vec{B}|| \) denote the magnitudes of the vectors, while \( \theta \) is the angle between them.
Algebraic Definition
The algebraic definition of the inner product simplifies computations. The aforementioned properties help to derive critical outcomes in vector calculus.
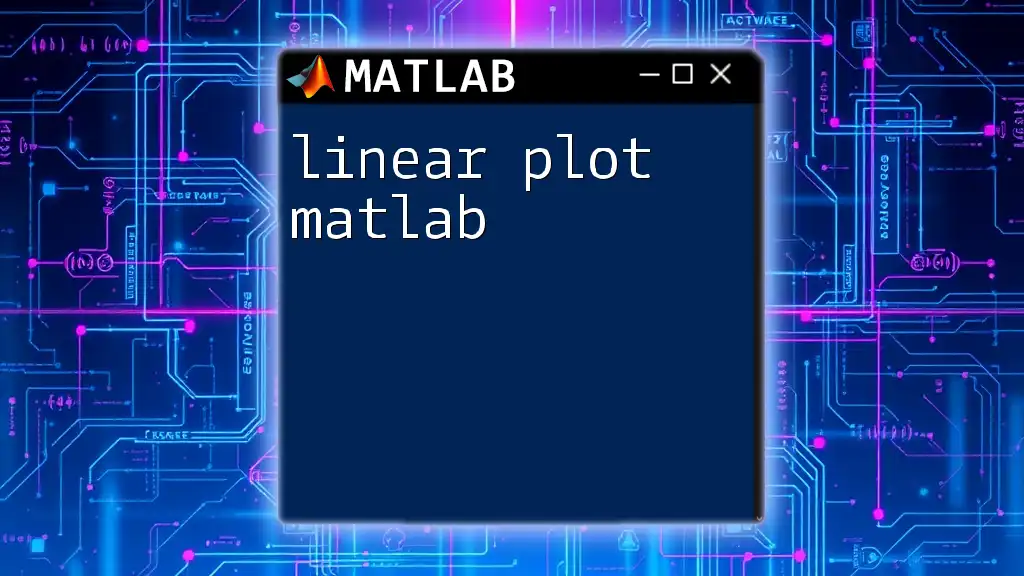
Using Inner Product in MATLAB
Command Syntax in MATLAB
In MATLAB, the inner product is easily computed using the `dot()` function. Its syntax follows the straightforward pattern:
inner_product = dot(vectorA, vectorB);
This command efficiently computes the inner product of two vectors, provided they are of the same dimension.
Example 1: Simple Inner Product Calculation
Here's how to compute the inner product of two basic vectors in MATLAB:
% Defining vectors
A = [1, 2, 3];
B = [4, 5, 6];
% Computing inner product
inner_product = dot(A, B);
disp(inner_product);
Explanation: In this example, `A` and `B` represent two vectors. When computing the inner product, MATLAB multiplies the corresponding elements and sums them up, resulting in 32.
Example 2: Inner Product of Collected Data
Inner products can also be used for matrix operations, particularly when analyzing datasets. Here's an example of how to do this in MATLAB:
% Example data
X = [2, 3, 5; 1, 4, 6];
Y = [3; 2; 1];
% Inner product calculation
result = X * Y;
disp(result);
Explanation: Here, `X` is a matrix, and `Y` is a vector. The inner product (matrix multiplication) yields a new vector, which results in the combined contributions of `Y` across each row of `X`.
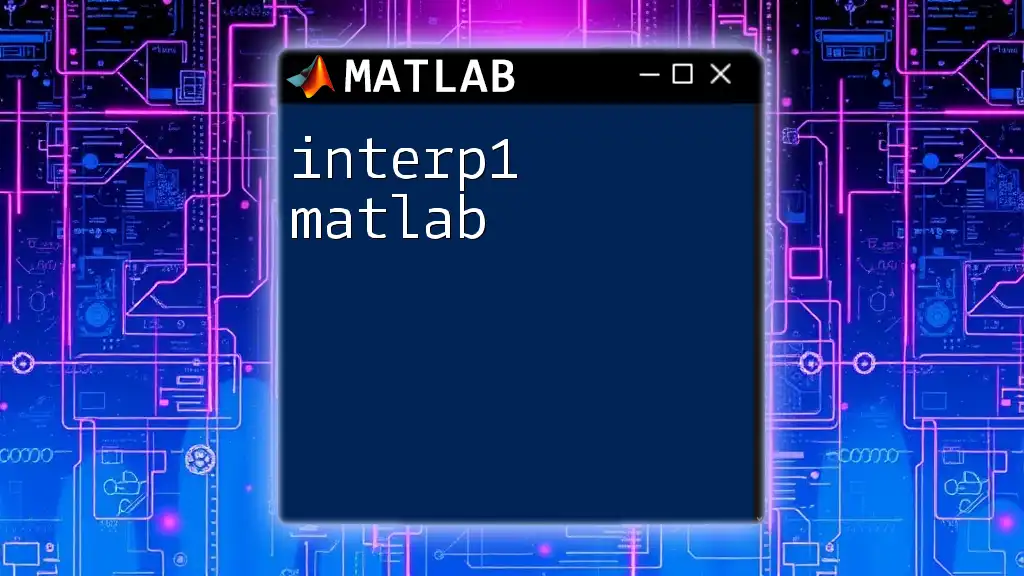
Advanced Inner Product Techniques
Handling Complex Vectors
The inner product can also apply to complex vectors. In MATLAB, complex numbers are simply represented with the `i` or `j` suffix, allowing for calculations directly in a similar syntax.
Example 3: Inner Product with Complex Numbers
Here’s how you can compute an inner product with complex vectors in MATLAB:
% Complex vectors
C = [1 + 2i, 3 + 4i];
D = [5 + 6i, 7 + 8i];
% Complex inner product
complex_inner_product = dot(C, D);
disp(complex_inner_product);
Explanation: The inner product calculates not only the products of the real parts but also combines the effects of the imaginary parts. This is essential for applications that involve signal processing and other fields with complex data.
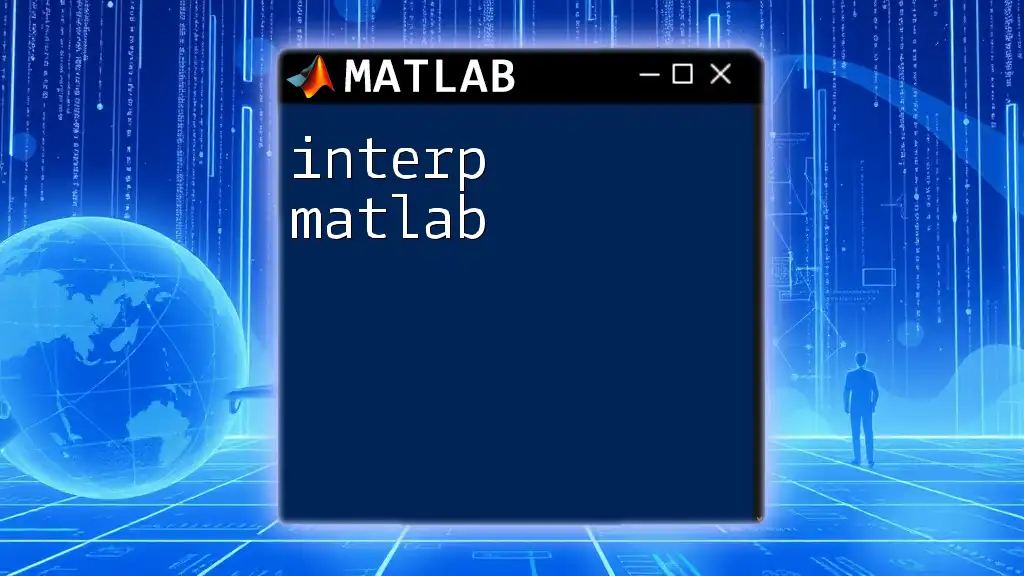
Performance Considerations
When working with large datasets, the efficiency of the inner product computations becomes crucial. To ensure performance:
- Vectorization: Utilize matrix operations over loops as MATLAB is optimized for matrix and vector operations.
- Preallocation: Allocate memory before performing operations to enhance memory management.
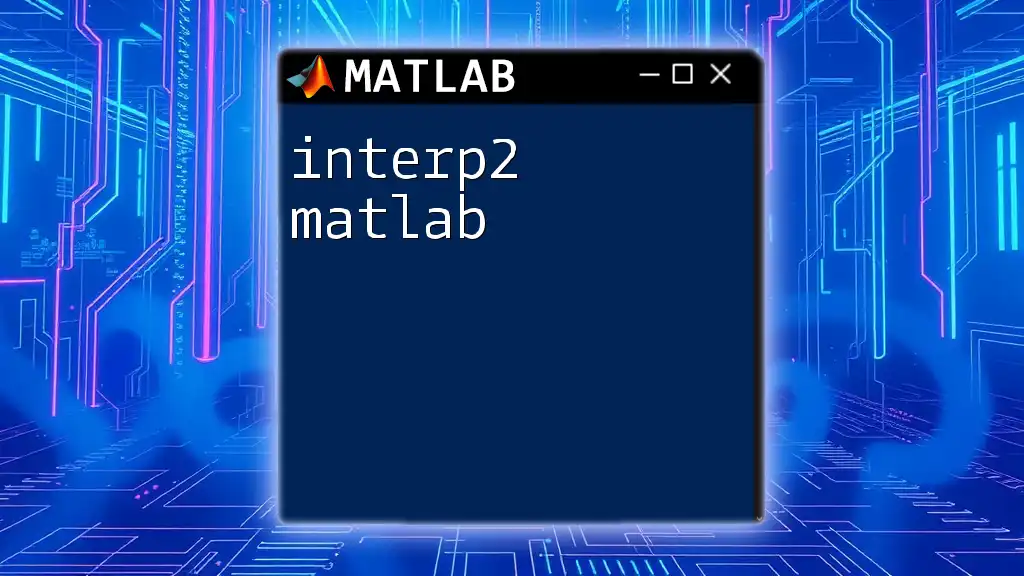
Common Errors and Troubleshooting
Common Mistakes When Computing Inner Products
One frequent error relates to dimension mismatch. The inner product can only be calculated for vectors of the same length. If vectors are incompatible, MATLAB will throw an error.
Debugging Tips
When encountering issues, use debugging commands like `size()` to check dimensions or `disp()` to output intermediate results, making it easier to pinpoint where things went awry.
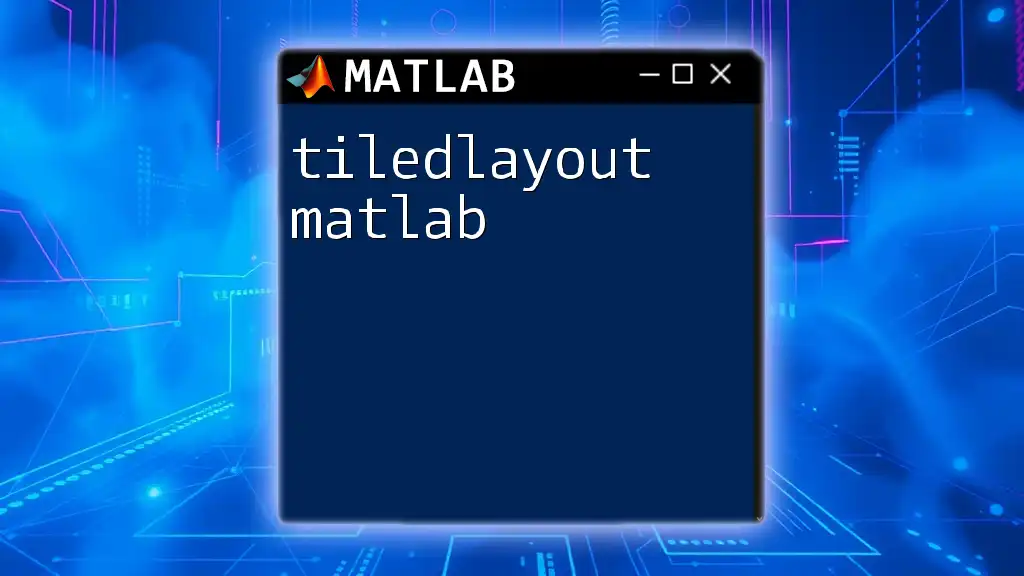
Conclusion
In conclusion, the inner product is a vital mathematical tool widely used in MATLAB for its simplicity and power. By understanding both the theoretical foundation and the practical applications, you can confidently leverage the inner product in MATLAB across various domains. Encourage continued exploration and practice to master this essential concept!
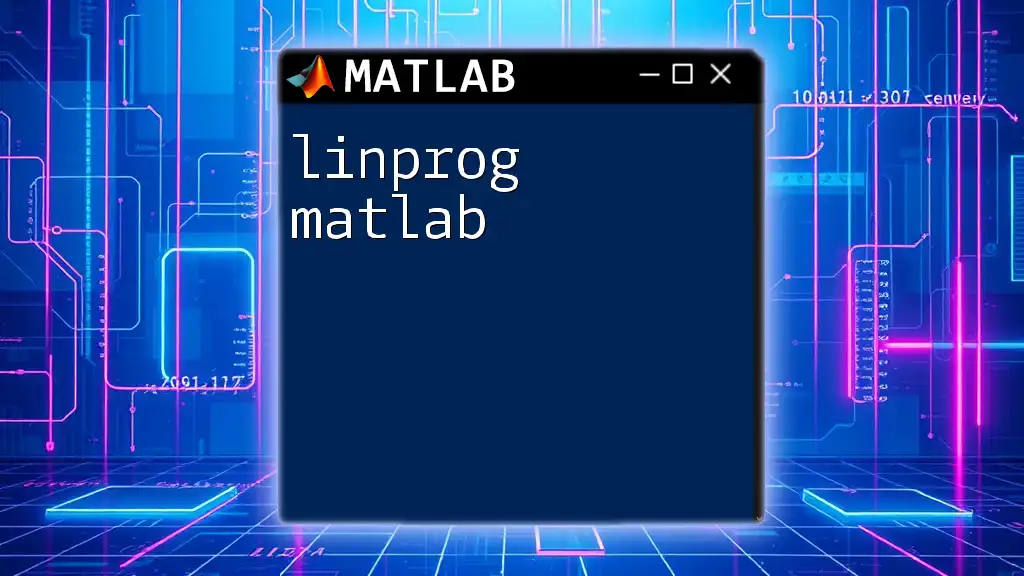
Further Resources
For those eager to dive deeper into MATLAB and linear algebra, numerous textbooks, online courses, and educational websites provide extensive insights and exercises to strengthen your knowledge and skills in these areas.