In MATLAB, you can plot points on a graph by using the `plot` function, which allows you to visualize data easily.
Here’s a simple example of how to plot points:
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 1, 4];
plot(x, y, 'o'); % 'o' specifies marker type as circles
xlabel('X-axis');
ylabel('Y-axis');
title('Plotting Points in MATLAB');
grid on; % Adds a grid for better visualization
Understanding Basic Plotting in MATLAB
What is Plotting?
Plotting is a crucial aspect of data visualization, allowing you to visually represent information in a way that is easy to understand. In MATLAB, plotting enables you to convey complex datasets and analytical insights effectively through graphical representation.
Key MATLAB Functions for Plotting
MATLAB offers several essential functions to create plots, including:
- `plot()`: Primarily used for creating line plots and connecting data points with lines.
- `scatter()`: Used for creating scatter plots, which display values for typically two variables for a set of data.
- `hold on` and `hold off`: Commands to overlay multiple plots on the same figure without erasing previous plots.
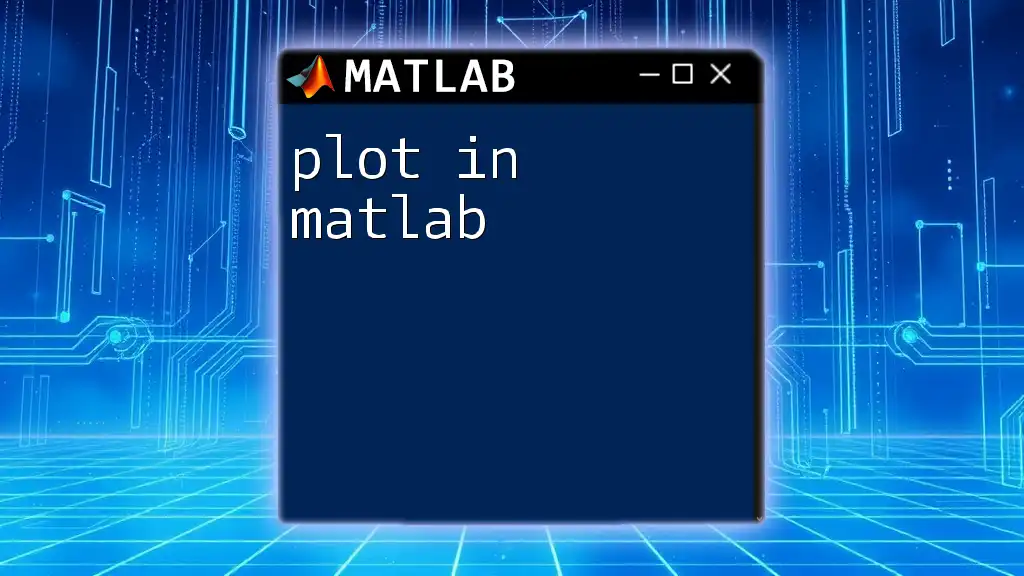
How to Plot Points in MATLAB
Using the `plot()` Function
The `plot()` function is one of the most commonly used commands in MATLAB for plotting data. Its syntax is straightforward and can be easily implemented.
x = [1, 2, 3, 4];
y = [10, 15, 20, 25];
plot(x, y);
title('Simple Line Plot');
xlabel('X-axis Label');
ylabel('Y-axis Label');
grid on;
In this example, we define two arrays, `x` and `y`, representing the coordinates of the points to be plotted. The `plot()` command connects these points with lines.
- `xlabel` and `ylabel` enhance the plot's readability by labeling the axes accordingly.
- `title` provides context to the viewer about what the plot represents.
- `grid on` adds a grid to the background, making it easier to read values.
By default, `plot()` connects the points with straight lines, making it ideal for time-series data or other sequential datasets.
Using the `scatter()` Function
While the `plot()` function is useful, `scatter()` serves to highlight individual data points without connecting them with lines.
x = [1, 2, 3, 4];
y = [10, 15, 20, 25];
scatter(x, y, 'filled');
title('Scatter Plot Example');
xlabel('X-axis Label');
ylabel('Y-axis Label');
grid on;
Here, `scatter(x, y, 'filled')` creates a scatter plot where each point is represented by a filled marker. This is particularly beneficial when looking for relationships between two variables without implying a continuous trend.
Summary of Differences:
- `plot()` connects points, making it suitable for trends.
- `scatter()` represents data points individually, ideal for correlation analysis.
Combining `plot()` and `scatter()`
Combining multiple plotting techniques allows for greater insight into data. You can use both `plot()` and `scatter()` in the same figure.
hold on; % Retains current plot
plot(x, y, '-o');
scatter(x, y, 'filled');
title('Combined Plot Example');
xlabel('X-axis Label');
ylabel('Y-axis Label');
legend('Line Plot', 'Scatter Points');
grid on;
hold off;
In this combined plot, the `hold on` command allows adding the scatter plot without erasing the previous line plot. Using different markers and colors helps differentiate between the data representations, providing clarity and depth to your analysis.
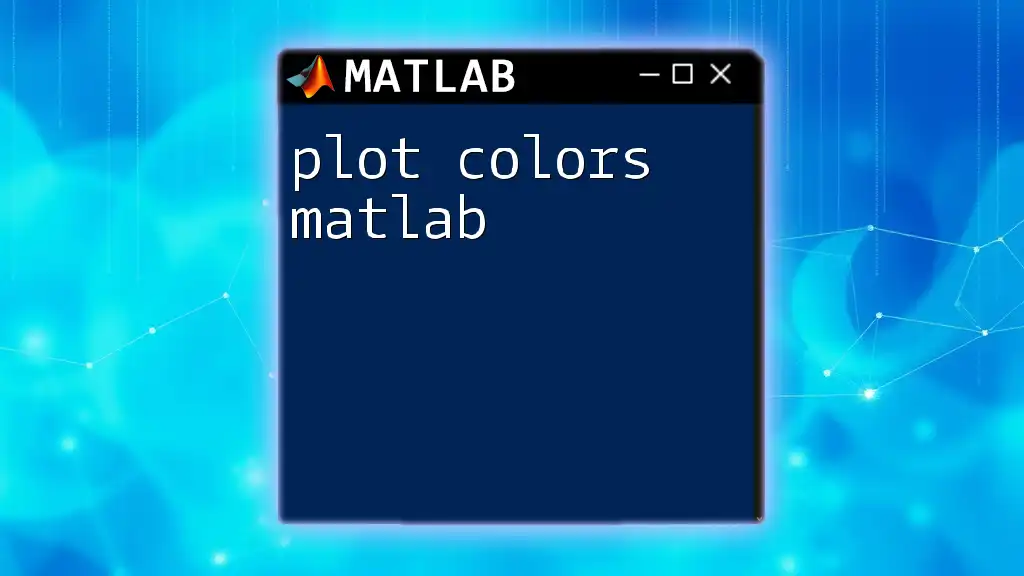
Customizing Your Plots
Adding Titles and Labels
Effective data visualization requires clear titles and labels. A well-defined title and labeled axes contribute to the viewer's understanding of the information provided.
title('Customized Plot');
xlabel('Independent Variable');
ylabel('Dependent Variable');
Modifying Axes
You can customize the display of axes with the `xlim()` and `ylim()` functions to set limits on the x-axis and y-axis, respectively.
xlim([0 5]);
ylim([0 30]);
This feature allows you to focus more closely on specific areas of interest within your data.
Changing Plot Appearance
MATLAB provides extensive options for customizing the appearance of your plots through colors, line styles, and marker types.
plot(x, y, 'r--', 'MarkerSize', 10, 'Marker', 's'); % Red dashed line with square markers
In this command, `'r--'` sets the line to red and dashed, while the marker size and style customize how points are represented visually.
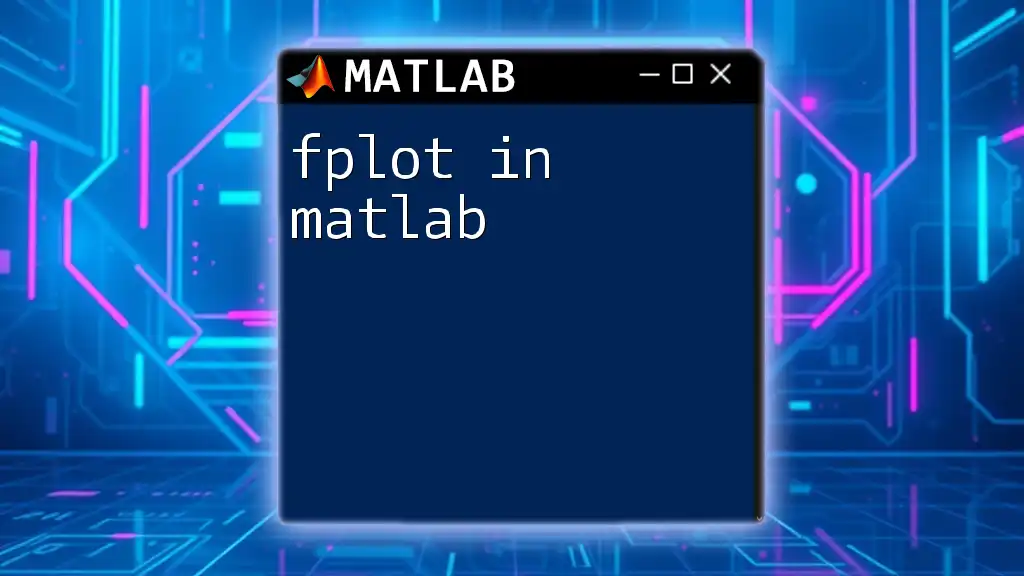
Advanced Plotting Techniques
Adding Annotations and Text
To add context or emphasize specific data points, you can insert text and annotations with the `text()` and `annotation()` functions.
text(3, 20, 'This is point (3,20)', 'FontSize', 10, 'Color', 'blue');
Annotations can clarify what specific points signify, thus enhancing understanding.
Subplots for Multiple Data Sets
If you need to visualize multiple datasets simultaneously, the `subplot()` function helps create an organized layout.
subplot(2, 1, 1);
plot(x, y);
title('First Plot');
subplot(2, 1, 2);
scatter(x, y);
title('Second Plot');
In this example, the figure is divided into two subplots, one for a line plot and another for a scatter plot. This organization allows for clear comparisons.
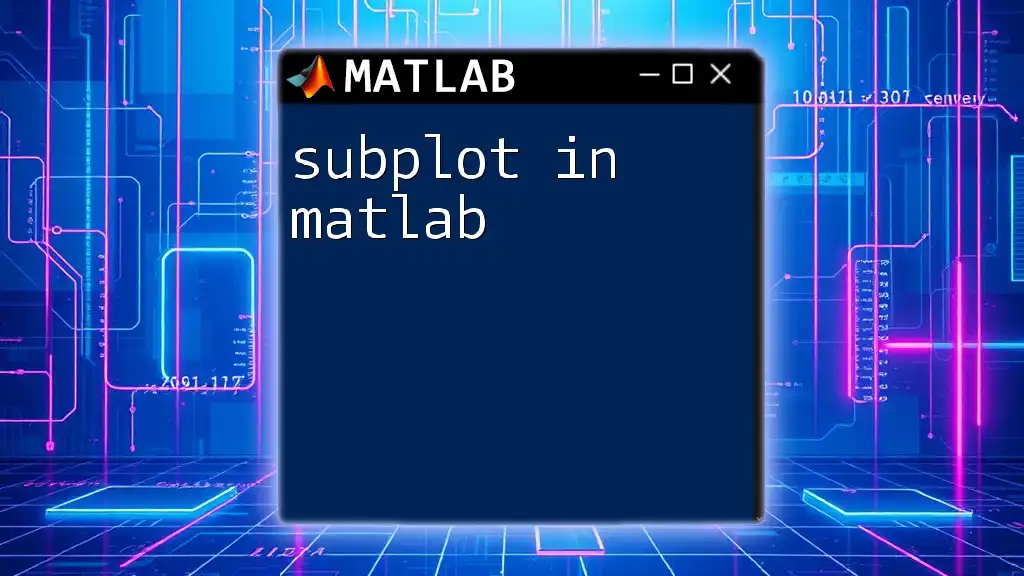
Saving and Exporting Plots
Saving Your Plots
Once you've created an insightful plot, saving it for future reference or sharing is essential. Use the `saveas()` or `exportgraphics()` functions to export your figures.
saveas(gcf, 'myPlot.png');
In this command, `gcf` refers to the current figure, and you define the filename and format you wish to save it in.
File Formats for Exporting
You can export your plots in various formats, including PNG, JPEG, and PDF. Remember to consider quality and scaling needs for your chosen format, particularly if your visuals will be part of presentations or publications.
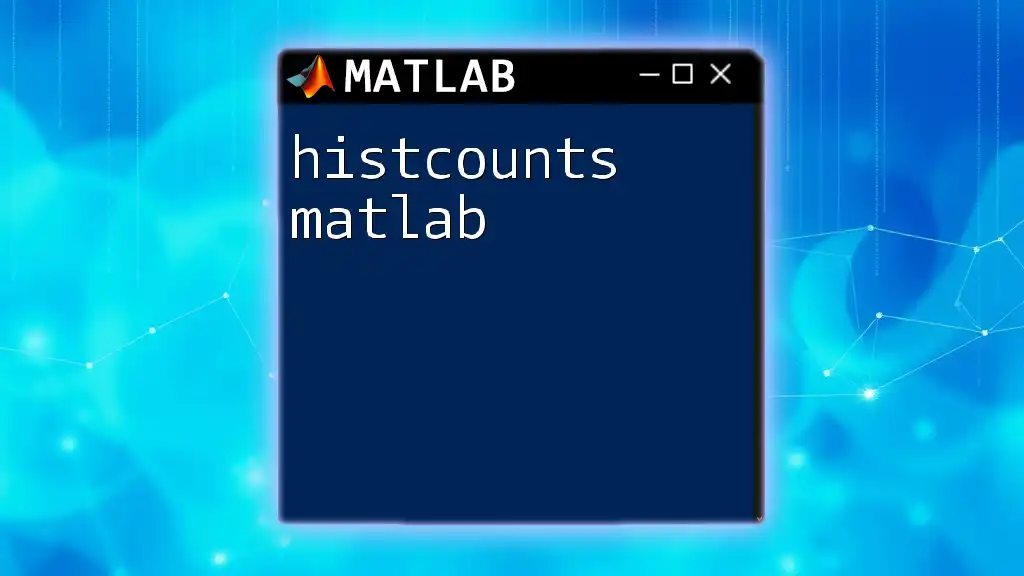
Conclusion
Plotting points in MATLAB is a valuable skill that enhances data visualization, allowing for clearer insights into your datasets. By mastering commands like `plot()` and `scatter()`, and learning to customize your plots, you're equipped to present your findings effectively.
The world of data visualization is vast and ever-evolving; therefore, it’s beneficial to continue exploring and practicing these techniques. With time and experimentation, your ability to communicate complex information through clear visuals will significantly improve.
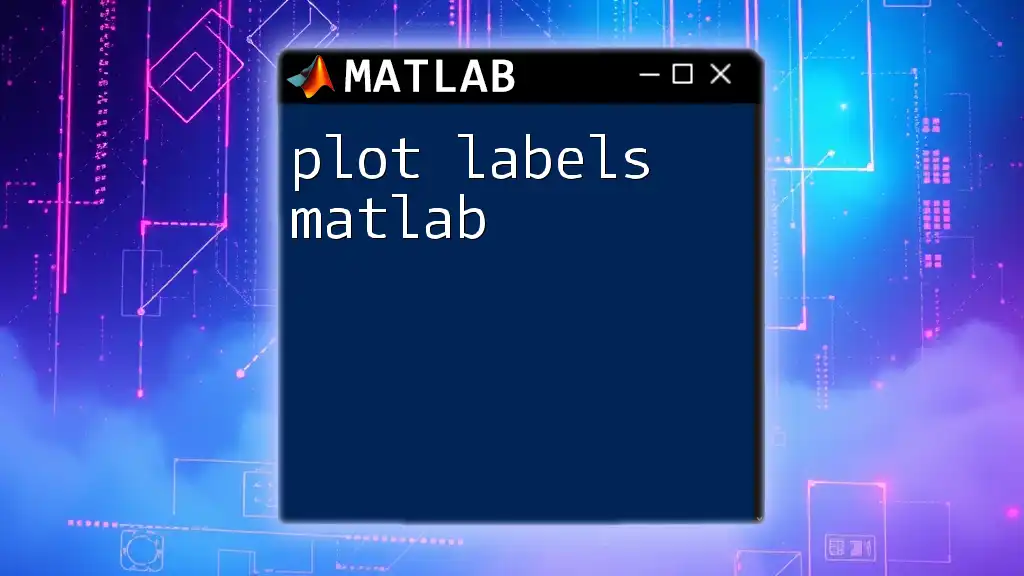
Call to Action
Sign up for our courses or workshops for deeper hands-on practice and to learn advanced techniques in MATLAB plotting. Explore our resources to help you become proficient in visualizing data using MATLAB’s powerful capabilities.