Element-wise multiplication in MATLAB is performed using the `.*` operator, which multiplies corresponding elements of two arrays of the same size. Here's a code snippet demonstrating this:
A = [1, 2, 3; 4, 5, 6];
B = [7, 8, 9; 10, 11, 12];
C = A .* B; % Element-wise multiplication
What is Element-Wise Multiplication?
Element-wise multiplication in MATLAB refers to the operation where corresponding elements from two matrices or arrays are multiplied together. This operation is crucial in various mathematical computations, particularly in data analysis, image processing, and scientific modeling. Unlike matrix multiplication, which follows specific algebraic rules, element-wise multiplication operates element by element, provided that the matrices are of compatible dimensions (i.e., they must either be the same size or one of them must be a scalar).
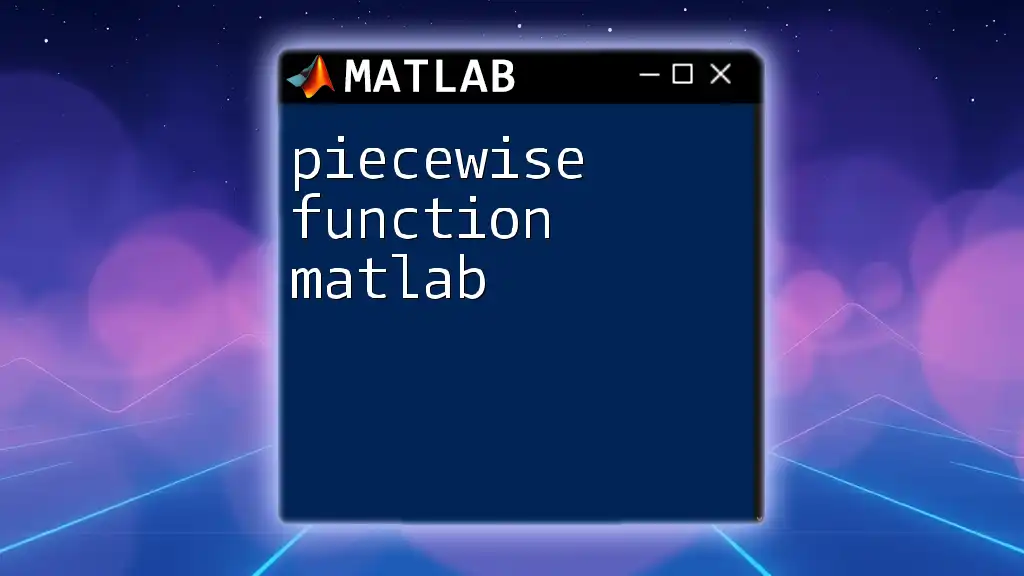
When to Use Element-Wise Multiplication
You might opt for element-wise multiplication in several scenarios, including:
- Scaling data: Adjusting datasets by applying multiplicative factors.
- Combining transformations: Applying multiple transformations to datasets in an efficient manner.
- Pixel manipulation: In image processing, scaling colors or applying filters.
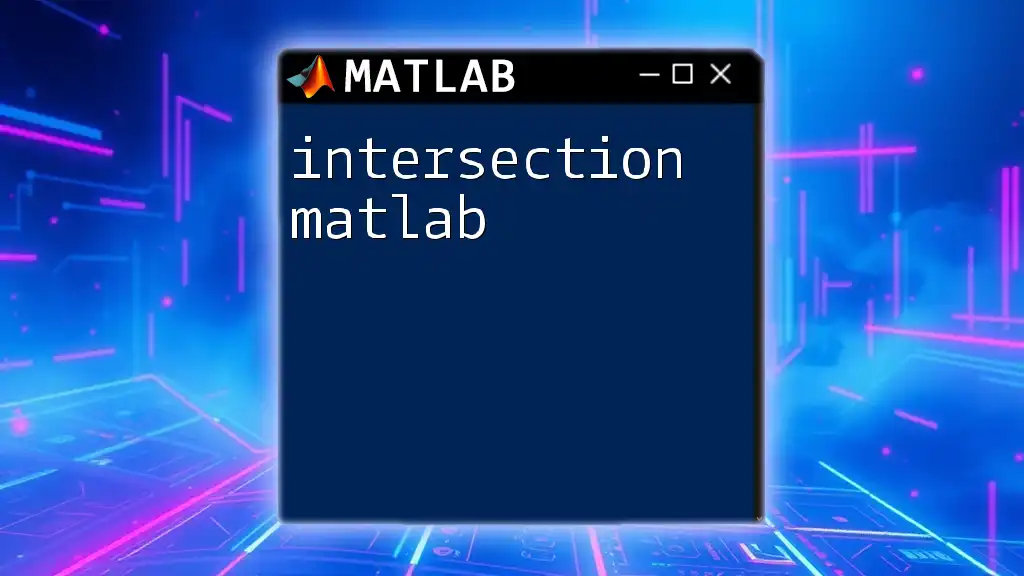
Understanding MATLAB Syntax for Element-Wise Multiplication
Basic Syntax
The syntax for performing element-wise multiplication in MATLAB is straightforward. You use the `.*` operator to denote the multiplication of two arrays. Here's the essence of this syntax:
C = A .* B;
Matrix Requirements
For MATLAB to execute element-wise multiplication, the matrices must meet size compatibility requirements. They should either be the same dimensions or one of the arrays should be a scalar. It’s also crucial to understand how MATLAB handles dimensional mismatches through broadcasting, allowing you to perform operations even when the sizes differ, under certain conditions.
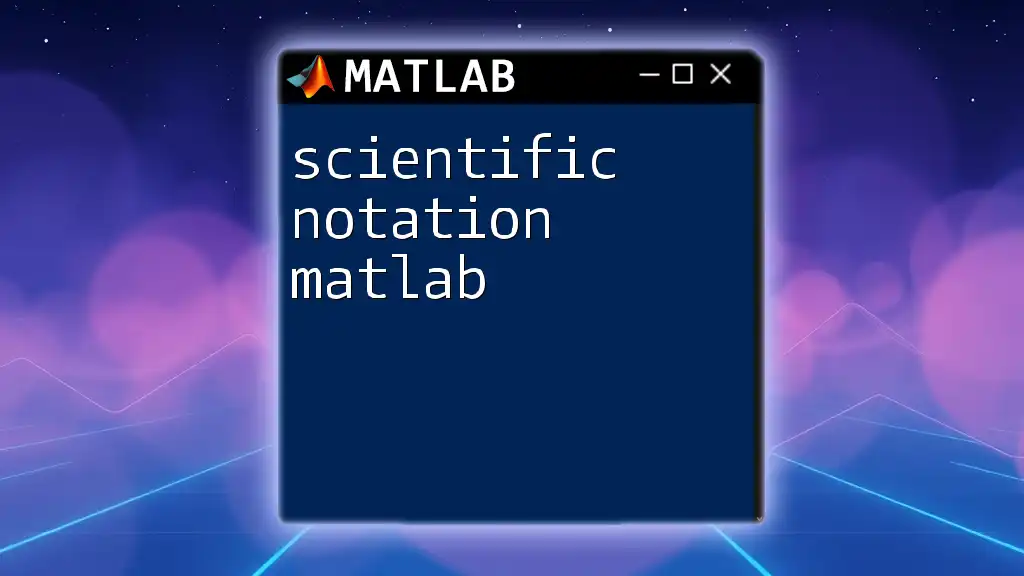
Implementing Element-Wise Multiplication
Basic Example
To illustrate the simplicity of element-wise multiplication, consider the following matrices:
A = [1, 2, 3; 4, 5, 6];
B = [7, 8, 9; 10, 11, 12];
C = A .* B; % Element-wise multiplication
disp(C);
The expected output would be:
7 16 27
40 55 72
In this example, each entry in the resulting matrix `C` represents the product of the corresponding elements in `A` and `B`, demonstrating the operational mechanics of element-wise multiplication in MATLAB.
Using Vectors and Matrices
Element-wise multiplication is also applicable when working with vectors. Observe this example:
v1 = [1, 2, 3];
v2 = [4, 5, 6];
result_vector = v1 .* v2; % Element-wise multiplication with vectors
disp(result_vector);
The output here will be:
4 10 18
It's important to note that in order to perform element-wise multiplication between vectors, they must be of the same length. MATLAB efficiently performs this operation and produces the output as indicated.
Advanced Practices in Element-Wise Multiplication
Element-Wise Operations on Multi-Dimensional Arrays
MATLAB allows element-wise multiplication on multi-dimensional arrays. This feature is particularly useful in fields requiring multidimensional data operations. For example:
A = rand(3,3,3); % 3D array
B = rand(3,3,3);
C = A .* B; % Element-wise multiplication in 3D
This code snippet multiplies each corresponding component in three-dimensional space, maintaining the same structural integrity as the original arrays.
Broadcasting Behavior
MATLAB also supports broadcasting, where smaller arrays can ‘stretch’ to match the size of larger ones. For instance:
A = [1, 2; 3, 4];
B = 2; % Scalar
C = A .* B; % Each element of A is multiplied by B
disp(C);
The output will be:
2 4
6 8
This demonstrates how MATLAB simplifies operations, eliminating the need for manual replication of matrices.
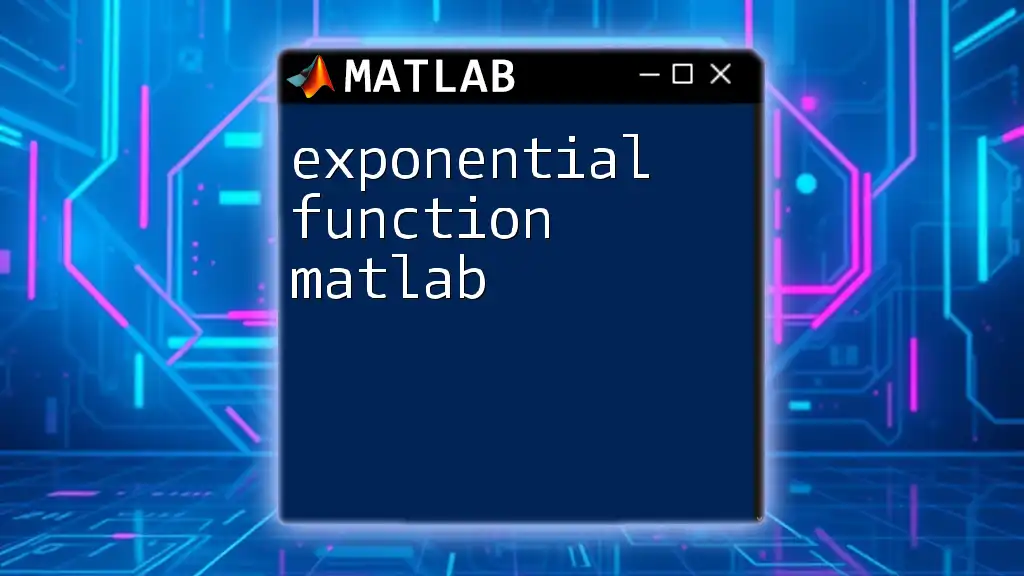
Common Errors and Debugging Techniques
One of the most frequent issues users encounter when performing element-wise multiplication in MATLAB is mismatched dimensions. When you try to multiply matrices of differing sizes that do not adhere to the rules of broadcasting, MATLAB will issue an error.
To mitigate this, always ensure that the matrices being multiplied are compatible in size. If you receive an error, consider using the `size()` function to inspect and verify the dimensions of your matrices.
Troubleshooting Element-Wise Multiplication
- Check dimensions: Use MATLAB commands to inspect the sizes of your matrices or vectors.
- Utilize broadcasting: If appropriate, leverage the broadcasting functions to match dimensions dynamically.
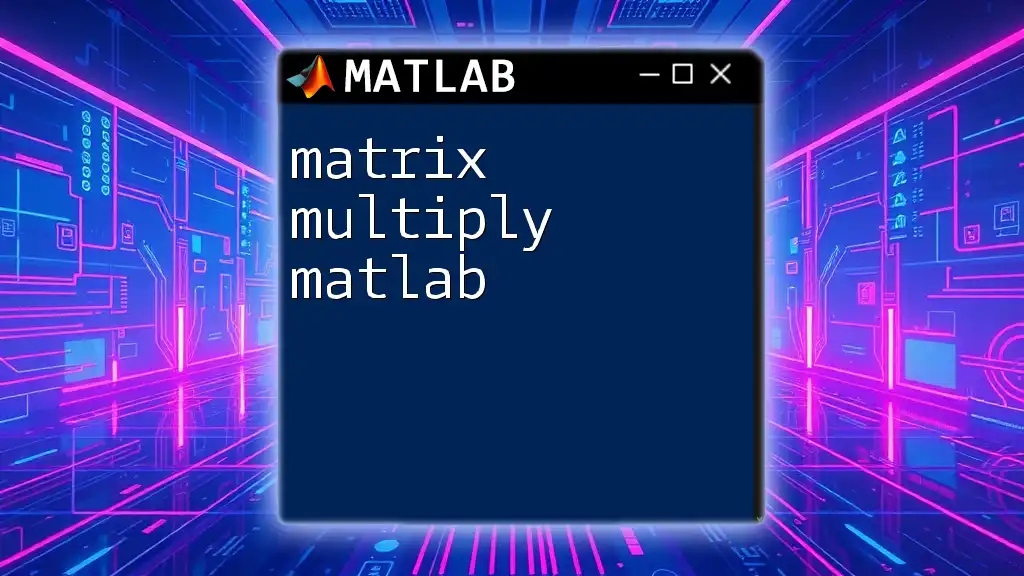
Practical Applications of Element-Wise Multiplication
Data Analysis
In data analysis, element-wise multiplication can be invaluable. Consider a scenario where you're scaling a dataset by specific weight factors. Here’s an example:
data = [10, 20, 30; 40, 50, 60];
weights = [1.1, 0.9, 1.05; 1, 0.8, 1.2];
scaled_data = data .* weights; % Scaling values
disp(scaled_data);
Image Processing
Another significant application arises in image processing, particularly with color manipulation. A simple example might involve reducing the intensity of the red color channel in an image:
img = imread('image.jpg');
img_red = img(:,:,1) .* 0.5; % Red channel scaling
imshow(img_red);
By adjusting the intensity, the overall image can be visually transformed, showcasing the power of element-wise multiplication in practical computations.
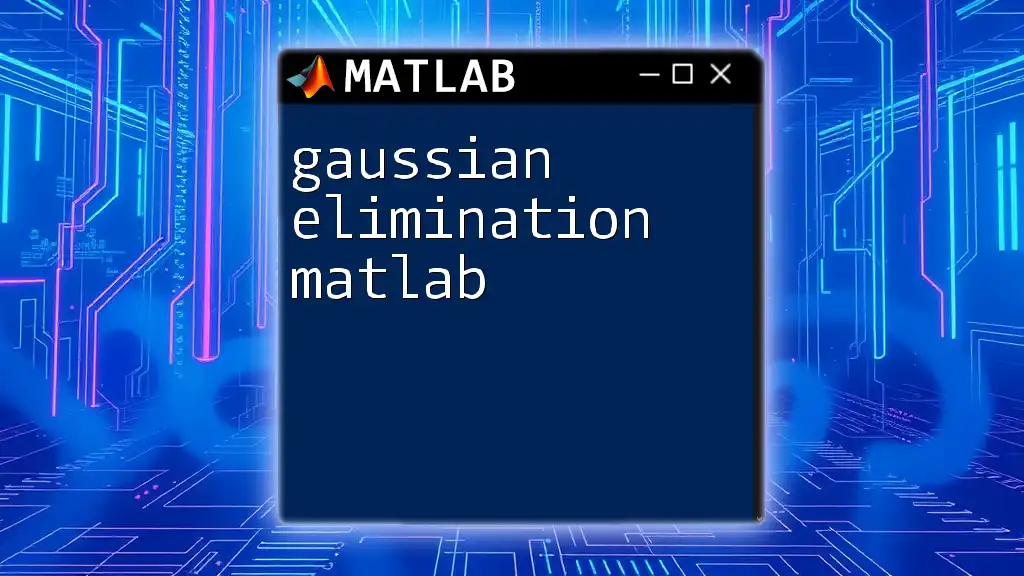
Summary of Key Points
Element-wise multiplication in MATLAB is a potent tool that simplifies computational tasks by multiplying corresponding elements of matrices and arrays. Whether working with simple vectors, multi-dimensional data, or in specific applications like data analysis and image processing, mastering this concept is imperative for anyone leveraging MATLAB for scientific computing or data-related work.
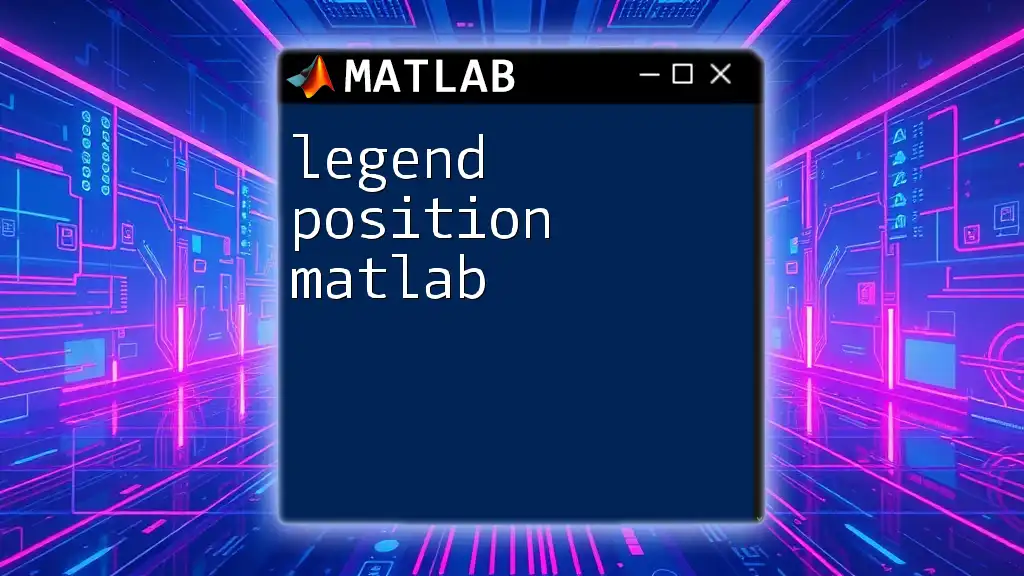
Encouragement for Further Learning
As you grow more proficient with element-wise multiplication, consider exploring related topics within MATLAB such as enhanced matrix operations, advanced mathematical functions, and further applications of broadcasting. Continuous learning will support your development as a confident MATLAB user.
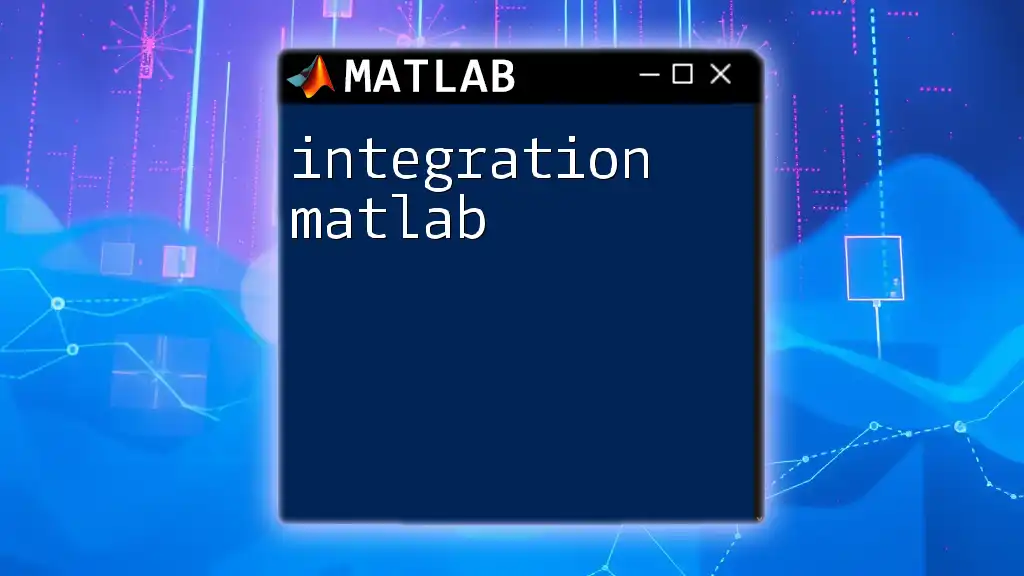
Additional Resources
To broaden your understanding, visit MATLAB’s official documentation, where you'll find extensive resources and tutorials. Engaging with MATLAB community forums can also provide valuable insights and encouragement as you delve deeper into your MATLAB journey.