In MATLAB, the `feedback` function is used to compute the closed-loop transfer function of a system with feedback, allowing you to analyze the stability and performance of control systems.
sys = tf(num, den); % Define the transfer function
feedbackSys = feedback(sys, 1); % Calculate closed-loop transfer function with unity feedback
Introduction to Feedback in MATLAB
Understanding Feedback Control Systems
Feedback control systems are essential in engineering and technology, serving to improve the performance and stability of dynamic systems. The fundamental principle of feedback is the process of measuring an output and using that measurement to influence the input, thereby enhancing the system's performance. Feedback plays a crucial role in everything from simple household appliances to complex systems in aerospace.
Overview of MATLAB in Feedback Systems
MATLAB provides a robust environment for designing and analyzing feedback systems. Its specialized toolboxes, such as the Control System Toolbox, offer a wide range of functionalities for modeling, simulating, and designing control systems efficiently. The flexibility and power of MATLAB make it an invaluable tool for engineers and scientists in the realm of feedback control.
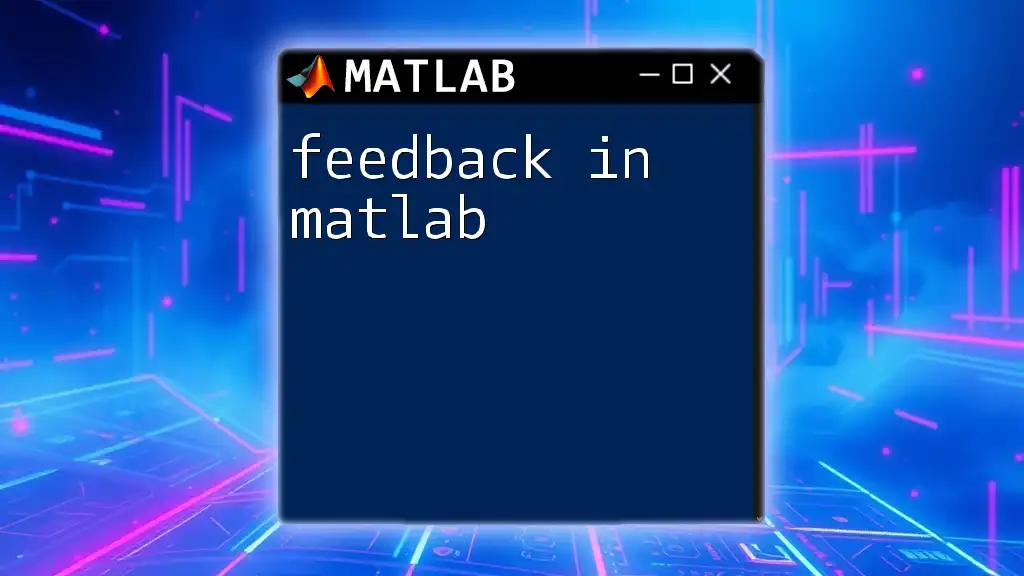
The Feedback Control Loop
What is a Feedback Control Loop?
A feedback control loop consists of several key components working together to regulate a system's output.
-
Controller: This is the brain of the operation. Controllers, such as PID (Proportional-Integral-Derivative) controllers, adjust the input based on the output's deviation from a desired setpoint.
-
Process: This refers to the system being controlled, characterized by its dynamic behavior represented often by differential equations.
-
Sensor: A sensor measures the output of the process, providing critical feedback that indicates how well the system is performing.
-
Actuator: The actuator translates the controller's commands into physical action, adjusting the input to the process in response.
Types of Feedback
Feedback can generally be categorized into two types:
-
Positive Feedback: This amplifies changes in the system. While this can lead to greater gains, it may cause instability in automation applications.
-
Negative Feedback: This counters changes, promoting stability and robustness. It is the most common form of feedback used in control systems.
Basic Concepts of Feedback Control
Stability
In control systems, stability is paramount—indicating a system's ability to return to equilibrium after a disturbance. An unstable system can lead to erratic output and potentially catastrophic failures. Therefore, understanding stability is crucial for designing effective feedback solutions.
Transient and Steady-State Responses
The transient response is how the system reacts to changes, while the steady-state response indicates the output once the system has settled. This distinction is vital for evaluating feedback performance over time.
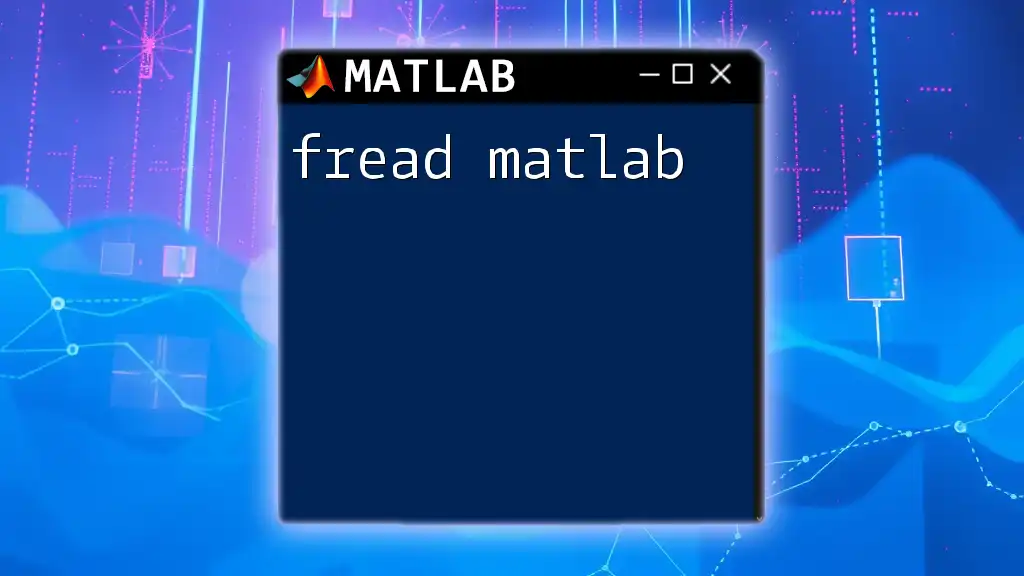
Designing Feedback Systems in MATLAB
MATLAB Functions for Feedback Systems
`feedback` Function
The `feedback` function is a powerful built-in MATLAB tool that computes the closed-loop transfer function of a system. Its syntax is straightforward:
T = feedback(G, H);
In this context:
- G represents the transfer function of the system.
- H denotes the transfer function of feedback.
Example: Creating a Closed-Loop System
G = tf(1, [1, 2, 1]); % Transfer function of the system
H = tf(1, 1); % Transfer function of feedback
T = feedback(G, H); % Closed-loop transfer function
In this example, the transfer function G is defined and represents a second-order system, while H is the feedback path, typically unity (1). The resulting T represents the closed-loop system, which can be analyzed for stability and performance.
`controlsysdesigner` Tool
MATLAB's `controlsysdesigner` is an interactive tool designed for the synthesis of control systems. This graphical interface allows users to adjust parameters dynamically and see the impact in real-time, facilitating the rapid development of effective feedback strategies.
Building a PID Controller
Understanding PID Control
A PID controller combines three control mechanisms: Proportional, Integral, and Derivative. Each plays a unique role:
- Proportional Control: Provides immediate control action proportional to the error.
- Integral Control: Accounts for past errors by integrating the error over time, eliminating steady-state error.
- Derivative Control: Predicts future errors based on current rates of change, improving system responsiveness.
Implementing a PID Controller in MATLAB
Creating a PID controller in MATLAB follows a straightforward process.
Example: Designing a PID Controller
Kp = 1; Ki = 1; Kd = 0.1; % PID parameters
C = pid(Kp, Ki, Kd); % Create PID controller
T_feedback = feedback(C*G, 1); % Closed-loop system
In this example:
- Kp, Ki, and Kd are the tuning parameters for the PID controller.
- The controller C is defined using the `pid` function, while T_feedback computes the closed-loop transfer function using the combination of the PID controller and the system transfer function G.
Analyzing Feedback Systems
Time Response Analysis
To analyze how a feedback system behaves over time, MATLAB offers the `step` and `impulse` functions. These functions allow you to visualize the system's response to various inputs.
Example
step(T_feedback); % Step response of the closed-loop system
This command provides a graphical representation of the step response, showing how the output changes when a step input is applied.
Frequency Response Analysis
Feedback systems are also analyzed in the frequency domain, particularly using Bode plots. These plots provide insight into how the system behaves at different frequencies.
Example
bode(T_feedback);
The command above generates a Bode plot, which displays gain and phase shift across a range of frequencies. This analysis is critical for understanding the stability and responsiveness of the feedback system.
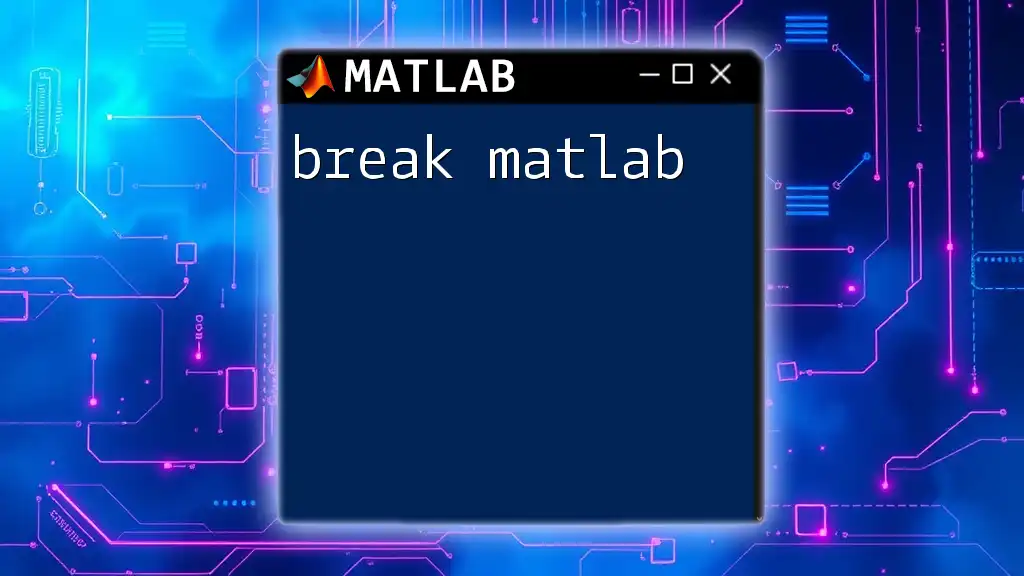
Practical Applications of Feedback in MATLAB
Real-World Examples
Feedback control is pervasive in various industries. In industrial automation, feedback mechanisms regulate machine performance, ensuring quality and output consistency. In robotics, feedback loops enable precise motion control, allowing robots to adapt to environmental changes dynamically.
Case Study
Example Case Study: Temperature Control System
Consider a temperature control system utilized in a heating application. The system continuously measures the temperature of a room and adjusts heating based on the desired set point.
-
System Components:
- Controller: A PID controller regulates temperature.
- Process: The heating element and the environment.
- Sensor: Temperature sensor monitoring current temperature.
- Actuator: The heating system itself, which adjusts power based on control output.
-
MATLAB Code Example:
G = tf(1, [10, 1]); % Example transfer function for heating system Kp = 2; Ki = 1; Kd = 0.5; % PID parameters tuned for heating system C = pid(Kp, Ki, Kd); T_temp = feedback(C*G, 1); step(T_temp); % Plot the step response for temperature control
This code models the temperature control system and provides insight into how quickly the system reaches the desired temperature.
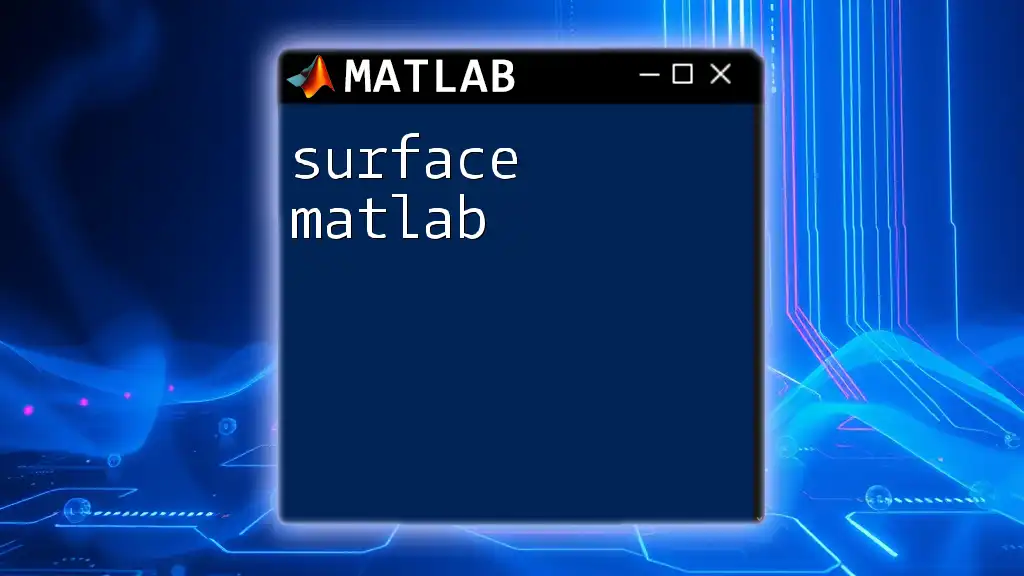
Best Practices for Using Feedback in MATLAB
Common Pitfalls to Avoid
When working with feedback systems, one common mistake is neglecting stability analysis. Always ensure that your system remains stable after implementing feedback; failure to do so can lead to oscillations or instability.
Tips for Effective Feedback Control
- Iterative Tuning: Use simulation results to iteratively adjust PID parameters for optimal performance.
- Document Your Process: Keep thorough documentation of your designs and parameter selections to facilitate future references and improve learning.
- Leverage Built-in Functions: Make the most of MATLAB's built-in functions for efficiency and accuracy in implementing your feedback designs.
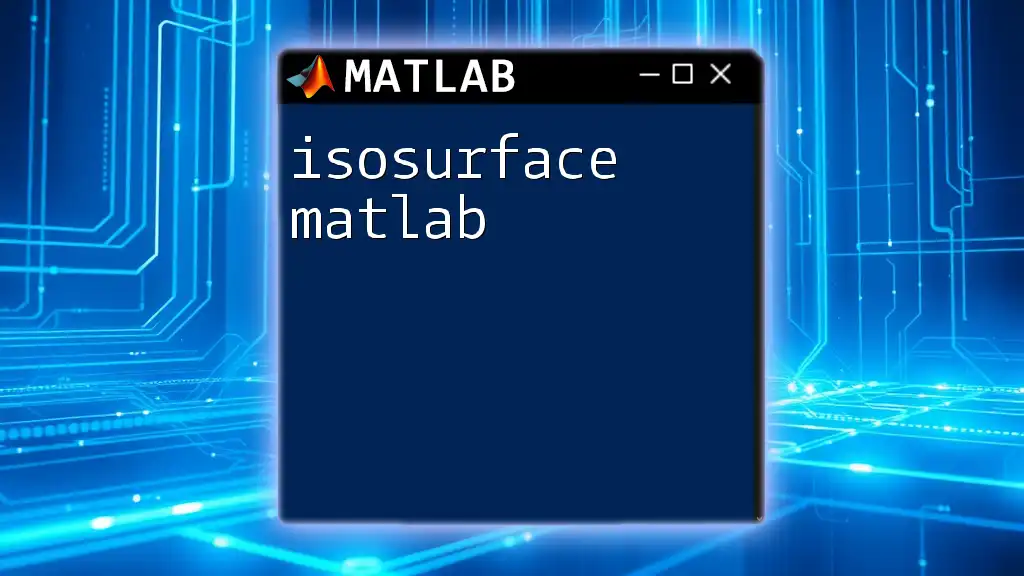
Conclusion
Mastering feedback control in MATLAB is essential for developing robust and efficient systems. By understanding foundational principles and leveraging the power of MATLAB, engineers can enhance their design processes and create more reliable systems. Explore feedback control systems today and take your MATLAB skills to the next level.