"break" in MATLAB is used to exit a loop or switch statement prematurely when a specific condition is met.
Here’s an example of using the "break" command in a loop:
for i = 1:10
if i == 5
break; % Exit the loop when i equals 5
end
disp(i); % Display the current value of i
end
Understanding the "break" Command
What is the "break" Command?
The `break` command in MATLAB is a powerful tool for controlling the flow of your code. It allows you to exit from a loop prematurely when a specific condition is met. This can be extremely useful in scenarios where you only need to perform a part of the loop's iterations or when a certain condition signifies that the required data has been found.
Where Can You Use the "break" Command?
The `break` command is primarily used in loops, such as `for` loops and `while` loops. Understanding how it interacts with these structures is crucial for effective programming in MATLAB.
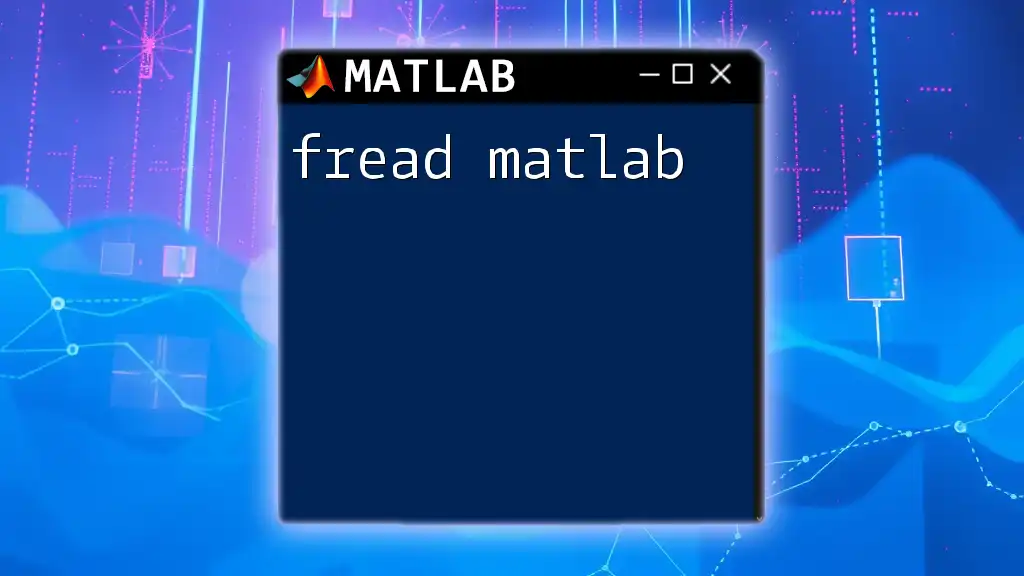
Syntax of the "break" Command
The basic syntax for the `break` command is deceptively simple:
break
You can place the `break` statement anywhere inside the loop, generally within a conditional statement. The command will terminate the loop immediately and transfer control to the statement that follows the loop.
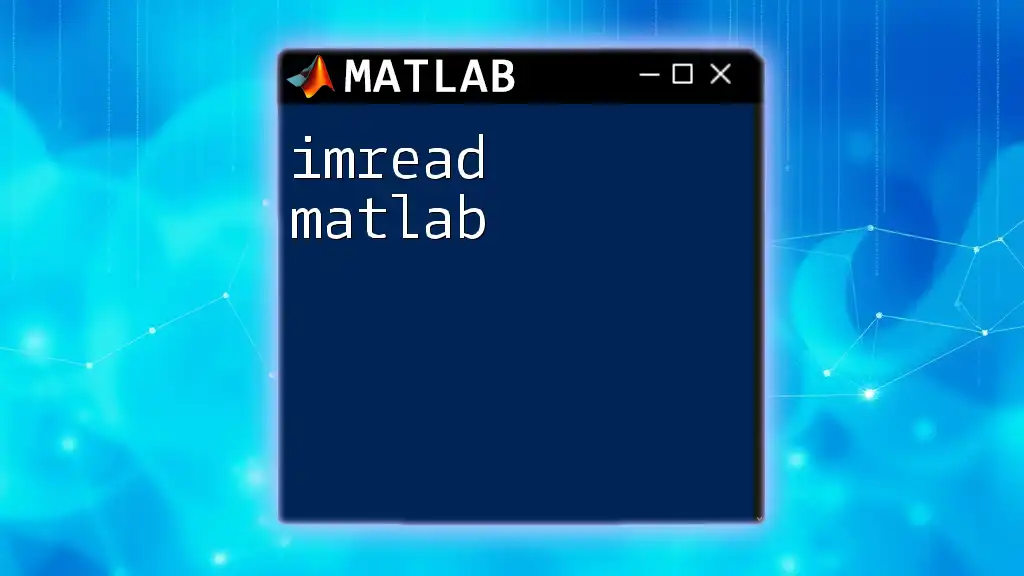
Practical Examples of Using the "break" Command
Using "break" in a For Loop
Consider the following example where we want to display numbers from 1 to 10 but stop the loop when we reach the number 5:
for i = 1:10
if i == 5
break;
end
disp(i);
end
In this code:
- The loop starts with `i` initialized at 1.
- As the loop iterates, it checks if `i` equals 5.
- Once `i` reaches 5, the `break` statement is executed, terminating the loop.
- Thus, the output will be:
1 2 3 4
This illustrates how `break matlab` allows you to halt execution based on conditions dynamically.
Using "break" in a While Loop
The `break` command is equally useful in `while` loops. For example, consider this code snippet:
j = 1;
while j < 10
if j == 6
break;
end
disp(j);
j = j + 1;
end
Here:
- The loop starts with `j` initialized at 1 and continues as long as `j` is less than 10.
- This time, if `j` equals 6, the `break` statement comes into play and terminates the loop.
- Therefore, the output will be:
1 2 3 4 5
This example highlights the versatility of `break matlab` in controlling flow through different types of loops.
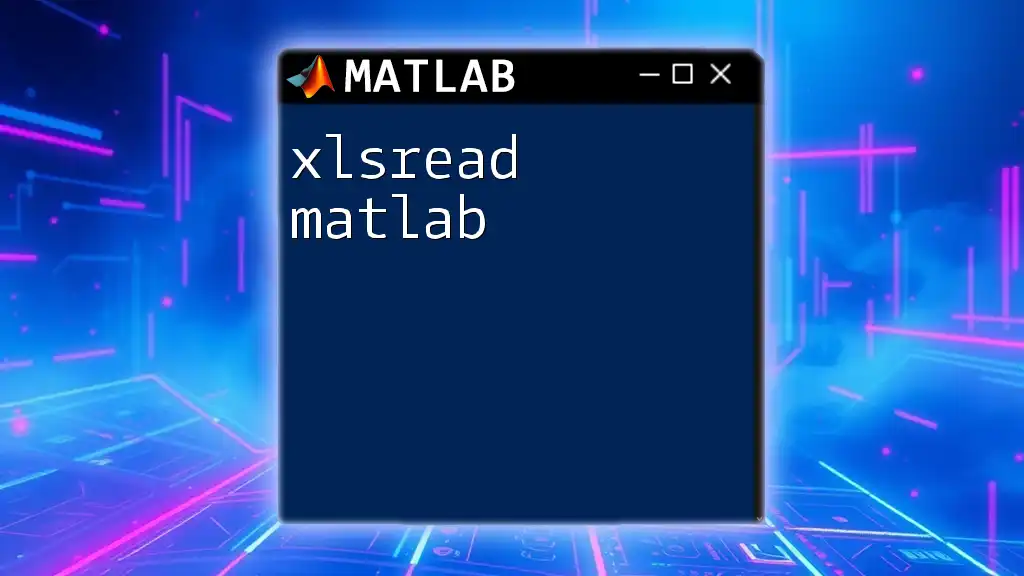
Best Practices for Using the "break" Command
When to Use "break"
To make the most of the `break` command, it's vital to understand appropriate scenarios for its use. Effective applications include:
- Exiting loops when a specific condition is met, such as finding a required element in a dataset.
- Stopping an infinite loop based on user input or validation checks.
For instance, using a `break` statement to halt execution if the user inputs invalid data can prevent unnecessary iterations.
Avoiding Common Pitfalls
While `break` is a useful command, overusing it can lead to confusing code that is hard to follow. Common pitfalls include:
- Using `break` indiscriminately, which may obscure the logic of the loop.
- Failing to comment or document when `break` is used, leading to decreased code readability.
To mitigate these issues, aim to keep your code clean and logical. Use comments to explain why a `break` statement is necessary, thus improving maintainability.
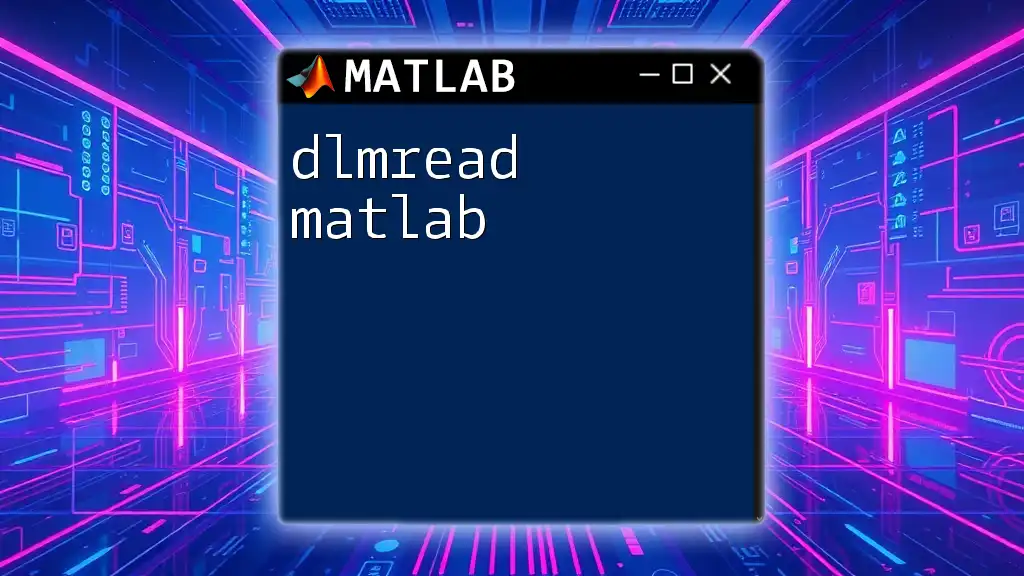
Alternatives to "break"
In some cases, you may find it beneficial to use alternative methods rather than `break`. One such approach is employing a flag variable to indicate when to exit a loop. Here’s an example of how this could replace `break`:
flag = true;
for i = 1:10
if i == 5
flag = false;
end
if flag
disp(i);
end
end
In this case, a flag is initialized to `true`. The loop continues displaying numbers until `i` equals 5, at which point the flag becomes `false`. This prevents further output without breaking the loop.
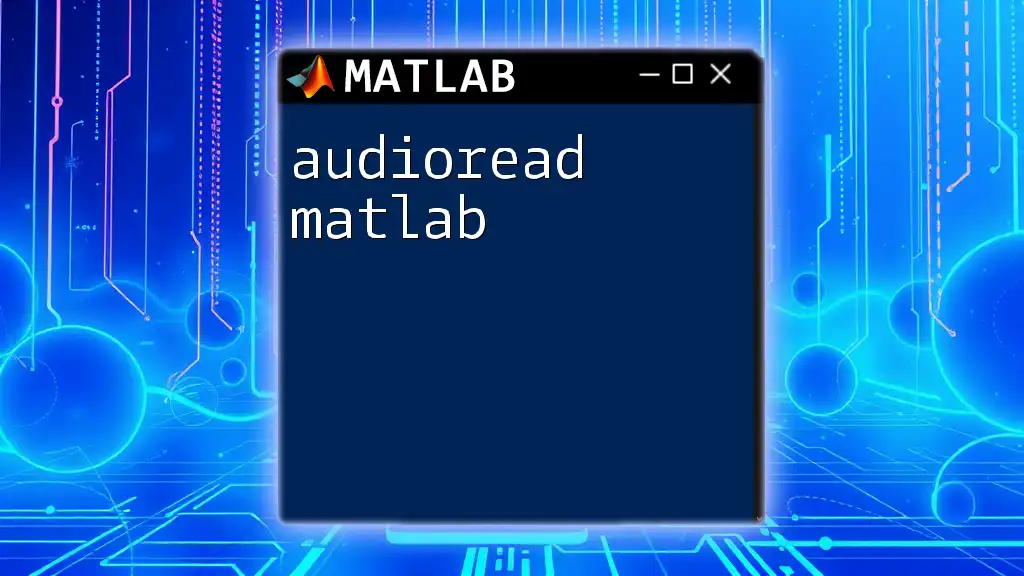
Conclusion
Understanding and utilizing the `break` command in MATLAB is essential for effective programming. By mastering when and how to use `break`, you can control the flow of your loops more precisely, enhancing the functionality and efficiency of your code. Experiment with `break` in your own projects, and remember to keep your code clean and understandable for future reference.
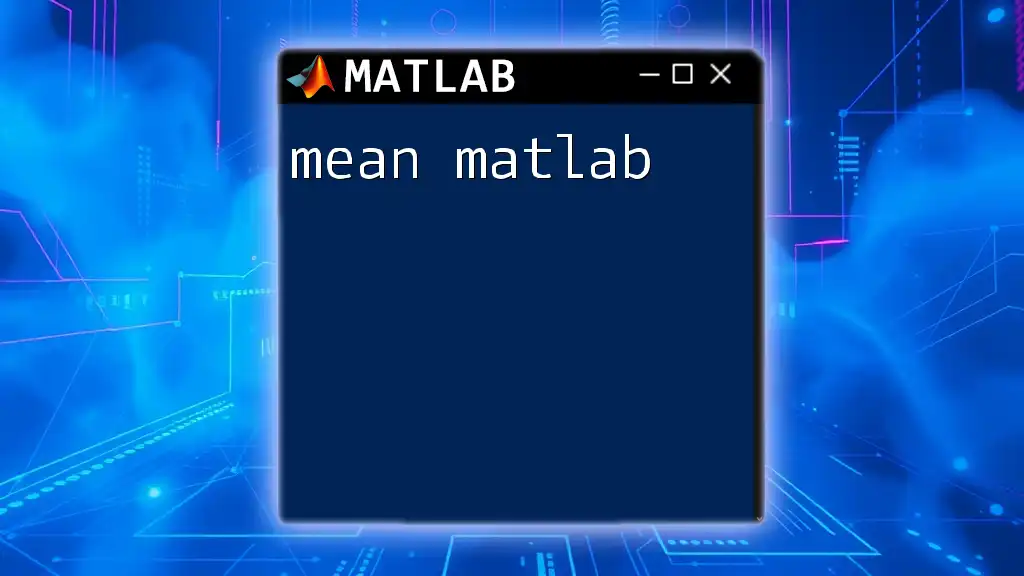
Additional Resources
To deepen your understanding of control flow in MATLAB, consider checking out the official documentation online, where you can find more examples and use cases for using the `break` command effectively. Additionally, joining forums and MATLAB user communities can provide insights and real-world applications of `break matlab`, enriching your learning experience.
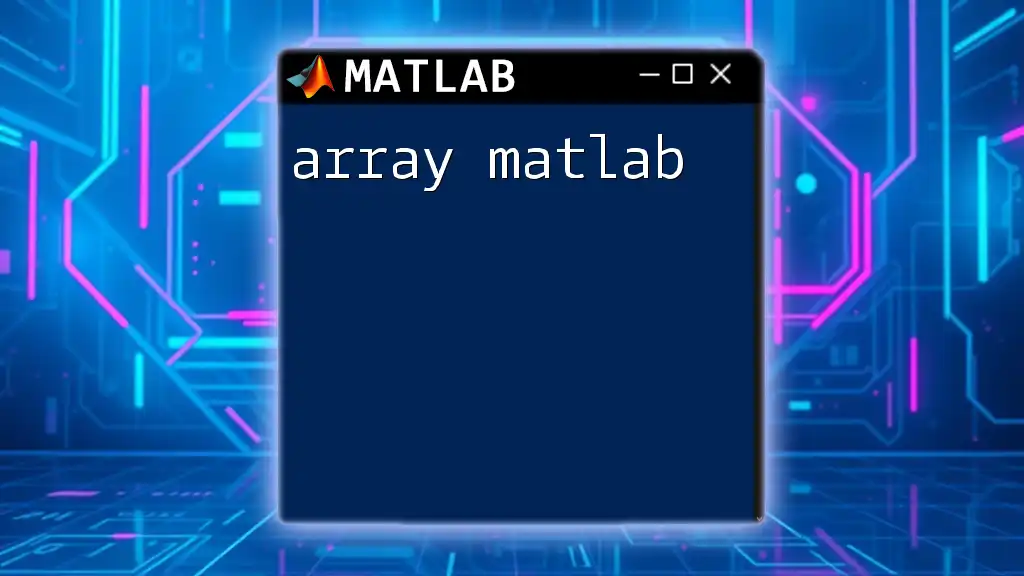
Call to Action
If you're eager to refine your MATLAB skills, join our learning community! We offer tailored tutorials, exercises, and resources to help you master MATLAB with concise and effective learning methods. Take your coding to the next level today!