Getting started with MATLAB code involves understanding basic commands and syntax to perform computations and visualize data effectively, such as initializing a simple variable and displaying its value.
Here's a basic example:
% Initializing a variable
x = 10;
% Displaying the value of x
disp(x);
Introduction to MATLAB
What is MATLAB?
MATLAB (Matrix Laboratory) is a high-performance language primarily used for technical computing, encompassing areas such as mathematics, engineering, and data analysis. It provides a platform where you can integrate computations, visualize data, and develop algorithms effortlessly. With its extensive toolboxes and user-friendly environment, MATLAB caters to both novices and seasoned professionals.
Why Learn MATLAB?
In an era where data is crucial, knowing how to utilize MATLAB can set you apart. It’s not only widely used in educational institutions but also extensively recognized and trusted in various industries, including finance, automotive, aerospace, and biotechnology. Several factors to consider:
- User-Friendly: MATLAB’s intuitive interface simplifies the learning curve, allowing users to focus on solving problems without getting bogged down by syntax complexities.
- Extensive Libraries: With robust built-in functions and a plethora of toolboxes, MATLAB enables users to tackle advanced computations and simulations efficiently.
- Strong Community Support: An active user community and abundant resources make troubleshooting easier, allowing beginners to learn from others’ experiences.
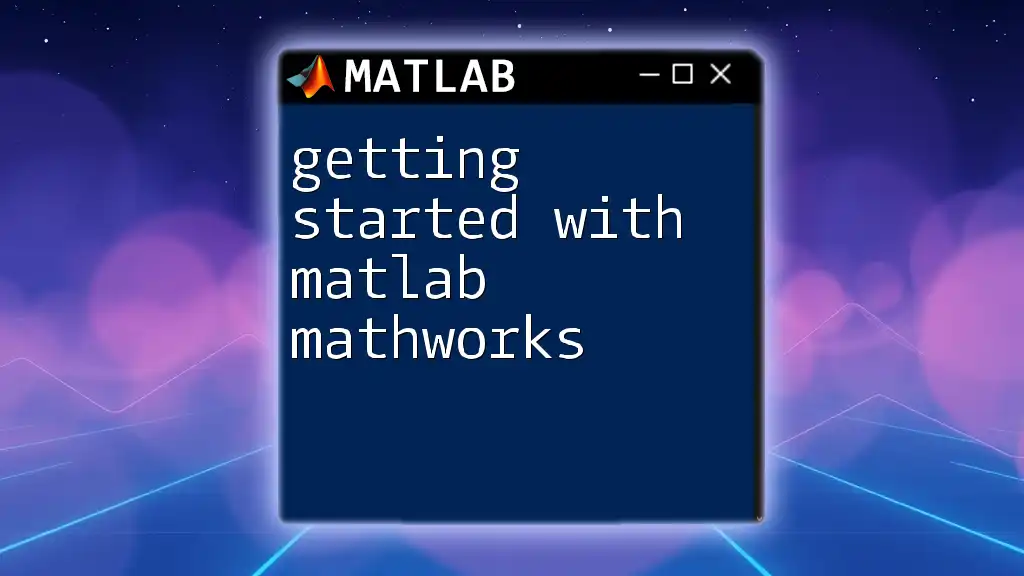
Setting Up MATLAB
Downloading and Installing MATLAB
To get started, you’ll first need to download and install MATLAB. Visit the [official MATLAB website](https://www.mathworks.com/downloads/) to find the latest version for your operating system. Follow the installation instructions provided on the site, which typically involve:
- Creating a MathWorks account.
- Downloading the installer.
- Running the installer with administrative privileges on your machine and following the prompts.
Navigating the MATLAB Interface
Upon launching MATLAB, you will encounter a set of distinct components that allow you to interact with the software. Key elements include:
- Command Window: The hub for executing commands and displaying output.
- Workspace: Displays all variables currently in memory, giving you a quick view of your data.
- Command History: A record of all commands you've executed, making it easy to revisit and reuse them.
- Editor: A script editor where you can write, save, and run scripts.
Tip: Customizing your layout by rearranging these components can significantly enhance productivity.
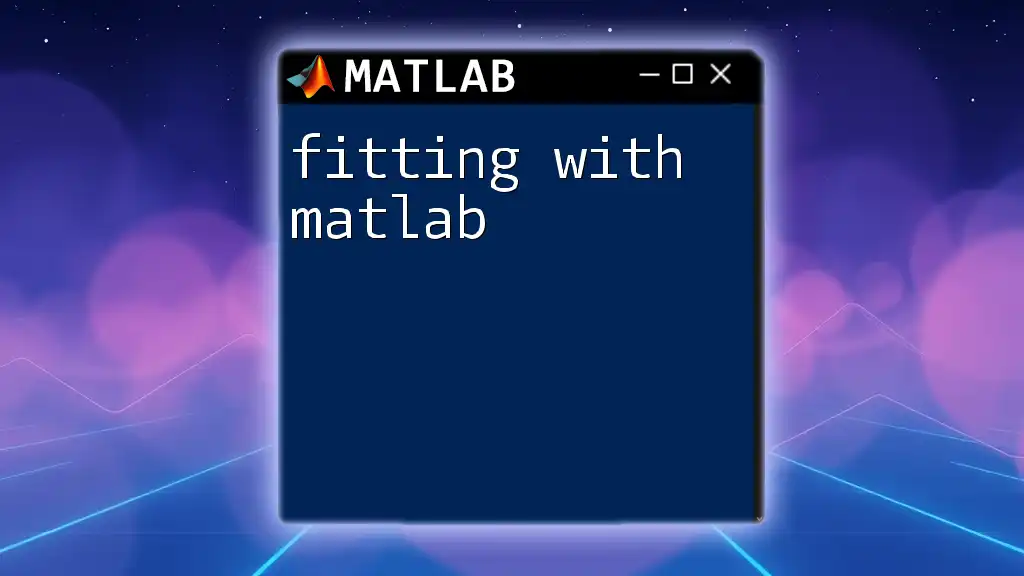
Basic Commands in MATLAB
Getting Started with the Command Window
The Command Window is your starting point for executing MATLAB code. Here's how to perform basic arithmetic operations:
% Example: Basic arithmetic
a = 10;
b = 5;
c = a + b; % Result: 15
disp(c);
Here, `disp(c)` displays the result in the Command Window, showing how easy it is to perform calculations.
Variables and Data Types
Variables in MATLAB must follow certain conventions, starting with a letter, followed by letters, numbers, or underscores. Understanding data types is crucial for effective programming.
% Example: Variable definition
x = 3.14; % x is a floating-point number
MATLAB supports various data types including scalars, arrays, and structures. Becoming familiar with these will empower you to manage data efficiently.
Arrays and Matrices
Creating Arrays and Matrices
MATLAB excels in handling arrays and matrices, which are foundational to its functionality. To create an array:
% Example: Creating an array
myArray = [1, 2, 3, 4, 5];
Basic Operations on Arrays
You can perform element-wise operations easily. For instance, to add a constant to every element:
% Example: Element-wise addition
result = myArray + 5; % Result: [6, 7, 8, 9, 10]
disp(result);
Understanding these operations lays the groundwork for more complex data manipulation.
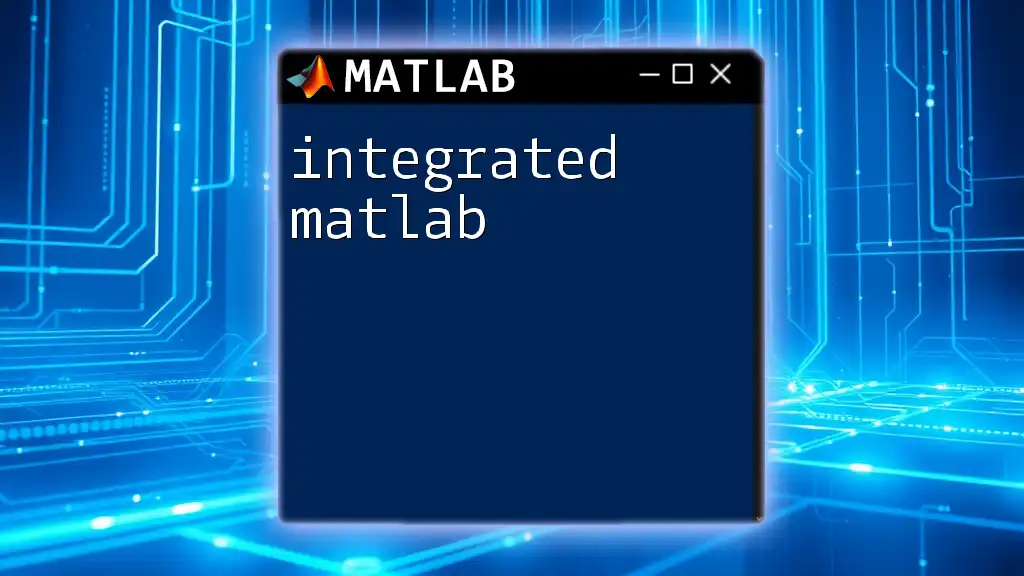
Control Flow in MATLAB
Conditional Statements
Control flow manages the logic in your code. For example, using `if`, `else`, and `elseif`:
% Example: Conditional statement
if x > 0
disp('x is positive');
else
disp('x is non-positive');
end
This small snippet illustrates how conditions can dictate the flow of execution, which is key in programming logic.
Loops in MATLAB
For Loops
Loops, particularly `for` loops, enable repetitive execution of code:
% Example: For loop
for i = 1:5
disp(['Iteration number: ', num2str(i)]);
end
This code iterates five times, showcasing how loops can make your work much more efficient.
While Loops
While loops operate under given conditions, running until a specified condition is false:
% Example: While loop
count = 1;
while count <= 5
disp(['Count is: ', num2str(count)]);
count = count + 1;
end
Both types of loops can provide powerful constructs for automating repetitive tasks.
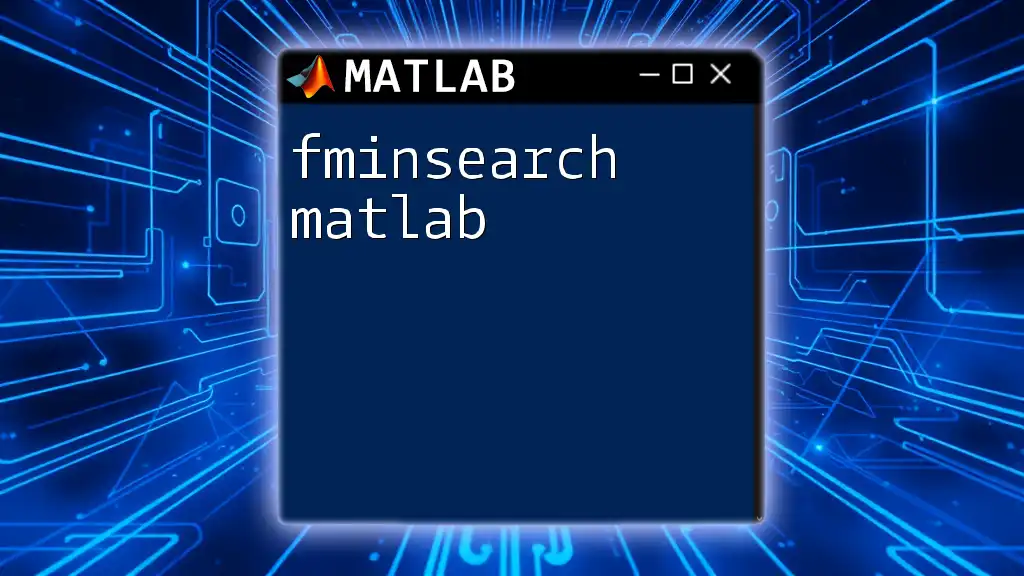
Functions in MATLAB
Creating a Function
Functions are self-contained blocks of code designed to perform specific tasks. Defining one is straightforward:
% Example: Function definition
function output = squareNumber(x)
output = x^2;
end
This function takes an input, `x`, and returns its square.
Calling a Function
Once you’ve defined a function, using it in your script is easy:
% Example: Function call
result = squareNumber(4); % Result: 16
disp(result);
Functions promote code reusability and organization, improving overall code quality.
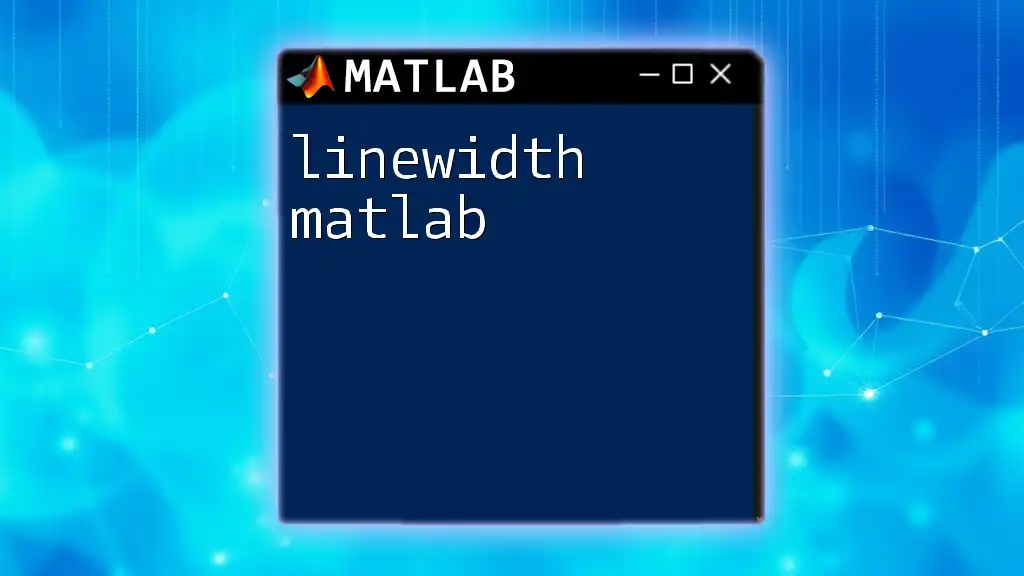
Plotting and Visualization
Introduction to Plotting
Data visualization is an essential part of data analysis. MATLAB provides robust plotting tools to help you visualize relationships in your data.
Basic Plotting Commands
Creating plots can be done with just a few lines of code:
% Example: Simple line plot
x = 0:0.1:10; % X values from 0 to 10
y = sin(x); % Y values are the sine of X
plot(x, y);
title('Sine Wave');
xlabel('x');
ylabel('sin(x)');
This snippet illustrates the basic principles of plotting in MATLAB, making it easy to analyze trends visually.
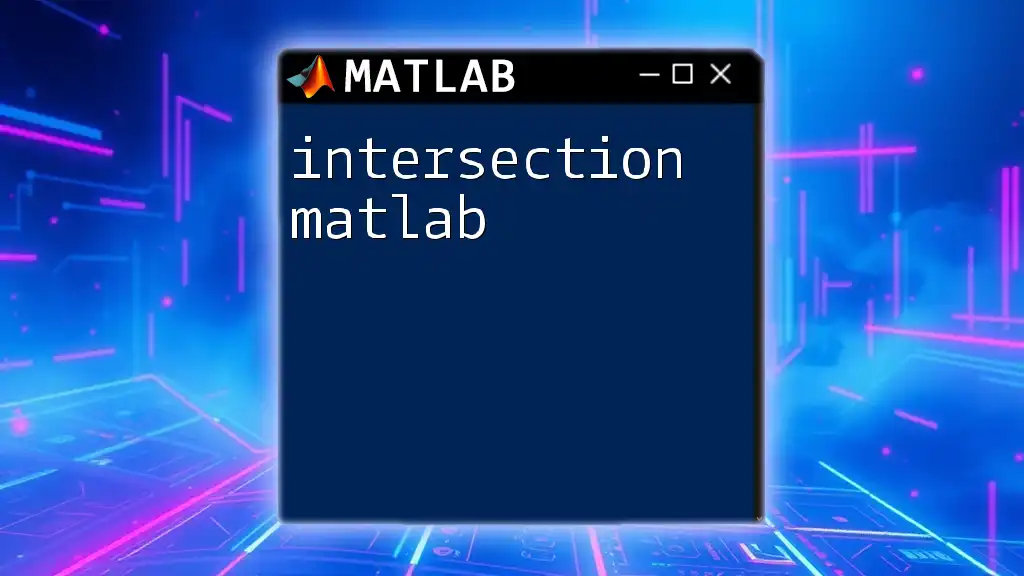
Debugging and Errors in MATLAB
Common Errors and Troubleshooting Tips
As you work with MATLAB, you may encounter various errors such as syntax errors, logic errors, or runtime errors. Debugging effectively involves:
- Reading the error messages to understand the issue.
- Using the `dbstop` command to set breakpoints in your code, allowing you to pause execution and examine variables.
Tip: Utilizing MATLAB’s built-in help and documentation can also help clarify commands and resolve confusion.
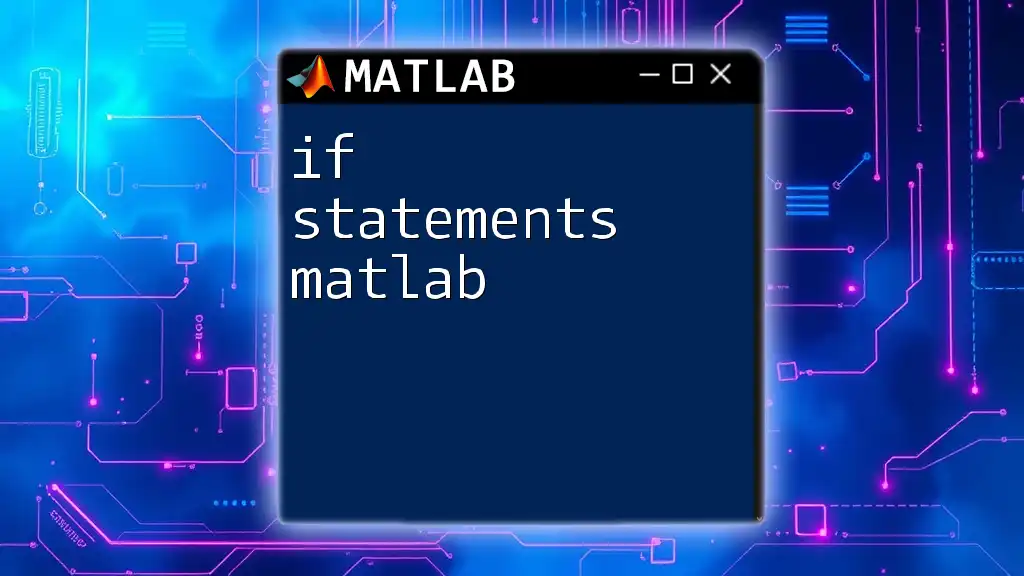
Conclusion
Next Steps in Learning MATLAB
To deepen your MATLAB knowledge, consider referring to official documentation, enrolled courses, and actively participating in forums such as MATLAB Central. Engaging with the community can provide invaluable insights and tips.
Encouragement to Experiment
Practice is vital for mastering MATLAB. Experiment with code, explore different functions, and create personal projects. The more you engage with MATLAB, the more adept you will become.
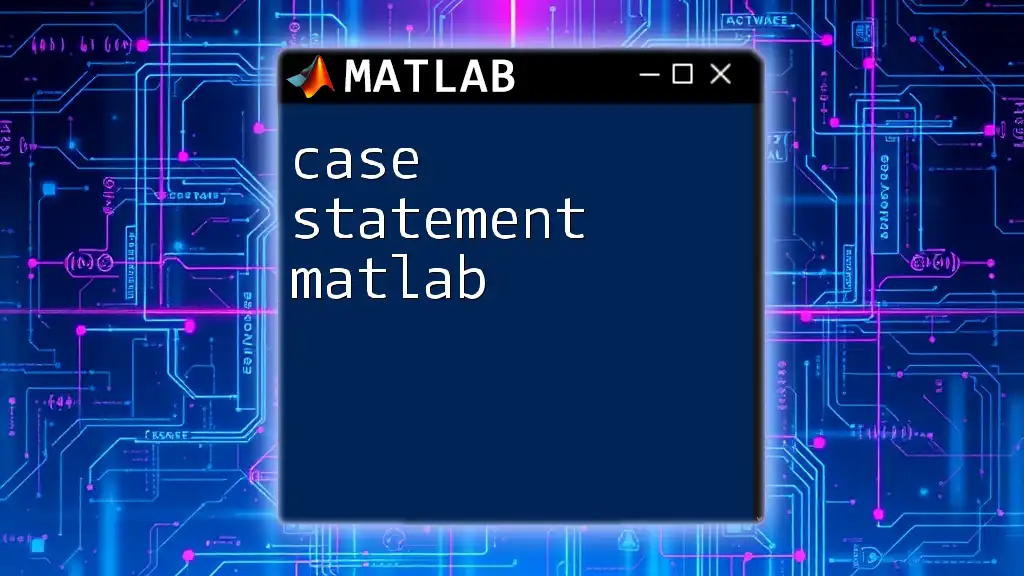
Call to Action
Join our MATLAB learning community today! Subscribe to our updates for tips, tutorials, and resources to help you on your journey to getting started with MATLAB code. Let’s build your MATLAB skills together!