In MATLAB, the command `exp` is used to calculate the exponential of a number or array, utilizing the base of the natural logarithm, \( e \).
Here’s an example of how to use it:
result = exp(1); % Calculates e^1
Understanding the Constant `e`
What Is the Constant `e`?
The constant `e` is a fundamental mathematical constant approximately equal to 2.71828. Known as Euler's number, it serves as the base for natural logarithms. `e` appears in various mathematical contexts, especially in calculus, where it holds tremendous significance in derivative and integral calculations. It is also critical in modeling continuous growth processes, such as population dynamics and finance.
How `e` Appears in MATLAB
In MATLAB, there is no specific variable called `e`. Instead, you can obtain the value of `e` using the predefined function `exp(1)`, which calculates the exponential function of e raised to the power of 1. This method ensures accurate computation of `e`.
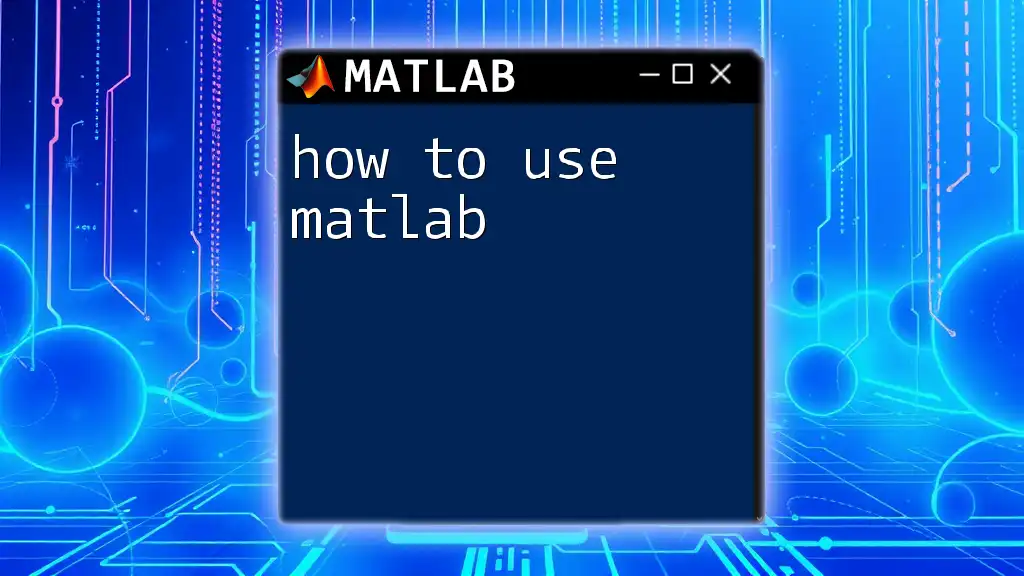
Working with `e` in MATLAB
Basic Usage of `e`
To utilize `e` in your MATLAB programs, you can store it in a variable for easy access:
e_value = exp(1); % Calculates e
disp(e_value);
Here, `e_value` will hold the value of `e`, which is 2.718281828459045.
Creating Exponential Functions
Exponential Growth and Decay
Exponential functions are essential in modeling various real-world scenarios. The basic formulas for exponential growth and decay can be expressed as follows:
- Growth Formula: \( N(t) = N_0 e^{rt} \)
- Decay Formula: \( N(t) = N_0 e^{-kt} \)
In MATLAB, you can implement these formulas easily. For example, if you want to model exponential growth, you can use the following script:
N0 = 100; % Initial value
r = 0.05; % Growth rate
t = 0:1:10; % Time from 0 to 10
N = N0 * exp(r * t); % Exponential growth function
plot(t, N);
title('Exponential Growth');
xlabel('Time');
ylabel('Population');
This code snippet creates a time vector and uses it to calculate the population over time, producing a visual representation of exponential growth.
Plotting Exponential Functions
Visualizing `e^x`
The function \( e^x \) is frequently plotted to observe its behavior, particularly in different ranges. The following code demonstrates how to graph \( e^x \):
x = -5:0.1:5; % Range of x
y = exp(x); % e^x
plot(x, y);
title('Plot of e^x');
xlabel('x');
ylabel('e^x');
grid on;
In this example, you create a range of `x` values from -5 to 5 and calculate the corresponding `e^x` values. When plotted, this graph exhibits the classic exponential growth curve.
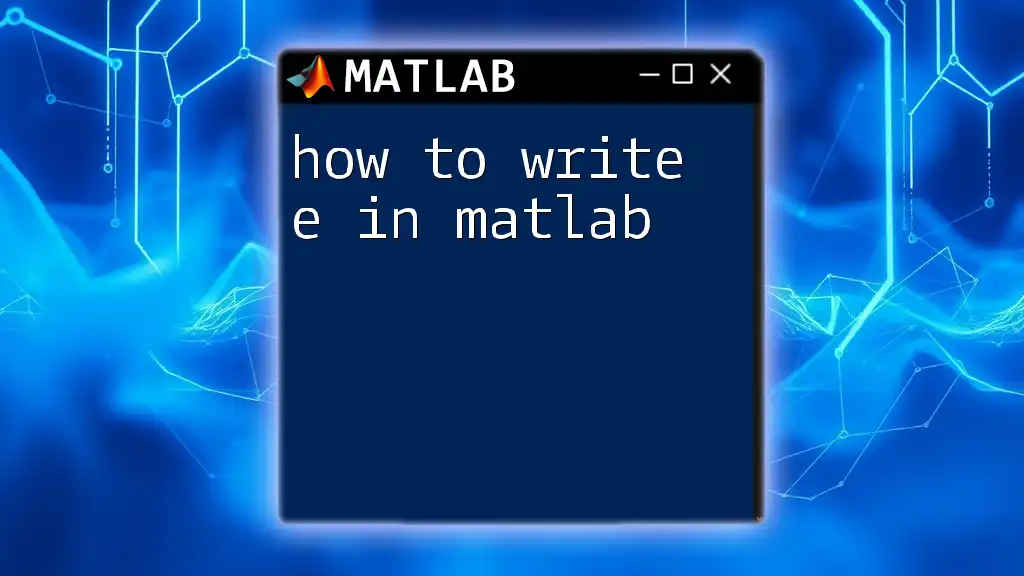
Advanced Applications of `e`
Using `e` in Complex Numbers
Euler's Formula
Euler's Formula states that \( e^{ix} = \cos(x) + i\sin(x) \). This formula connects exponential functions to trigonometric functions and is widely used in complex number analysis. You can calculate and visualize the unit circle in MATLAB using this formula:
theta = 0:0.1:2*pi; % Angle in radians
y = exp(1i * theta); % Calculating e^(ix)
plot(real(y), imag(y)); % Plot the unit circle
title('Euler\'s Formula Visualization');
xlabel('Real Part');
ylabel('Imaginary Part');
axis equal;
grid on;
This code generates points on the unit circle, demonstrating how `e` interacts with complex numbers.
Solving Differential Equations Using `e`
Differential equations are critical in modeling dynamic systems. By incorporating `e`, you can solve many of these equations effectively. Consider a simple first-order differential equation:
Equation: \( \frac{dy}{dt} = 2y \)
The general solution to this equation involves `e`, as shown in the following MATLAB code:
syms t C;
ode = diff(y, t) == 2 * y; % Example: dy/dt = 2y
ySol(t) = dsolve(ode, y(0) == C);
ySol(t) = C * exp(2 * t); % General solution
This script defines the differential equation and solves for `y(t)`, demonstrating how `e` resolves the components of the solution.
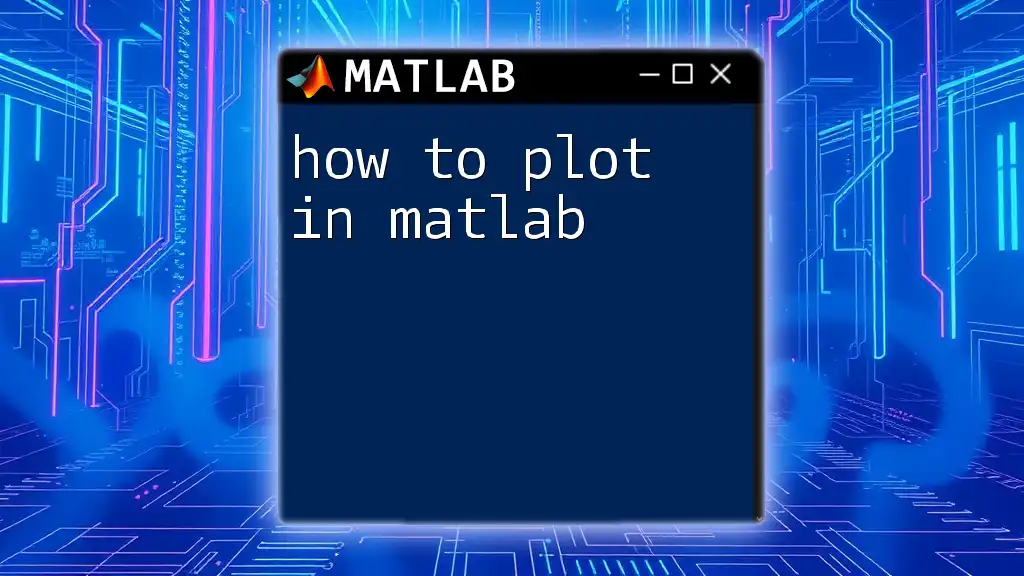
Common Mistakes When Using `e` in MATLAB
When using `e` in MATLAB, several common mistakes can occur. For instance, ensure you set initial parameters correctly when working with exponential growth and decay formulas. Additionally, misusing the syntax—such as failing to use the `exp()` function—can lead to errors in calculations.
It’s also vital to remember that confusion may arise when using `e` in the context of complex numbers. Be cautious when manipulating complex exponential forms to ensure accurate computations.
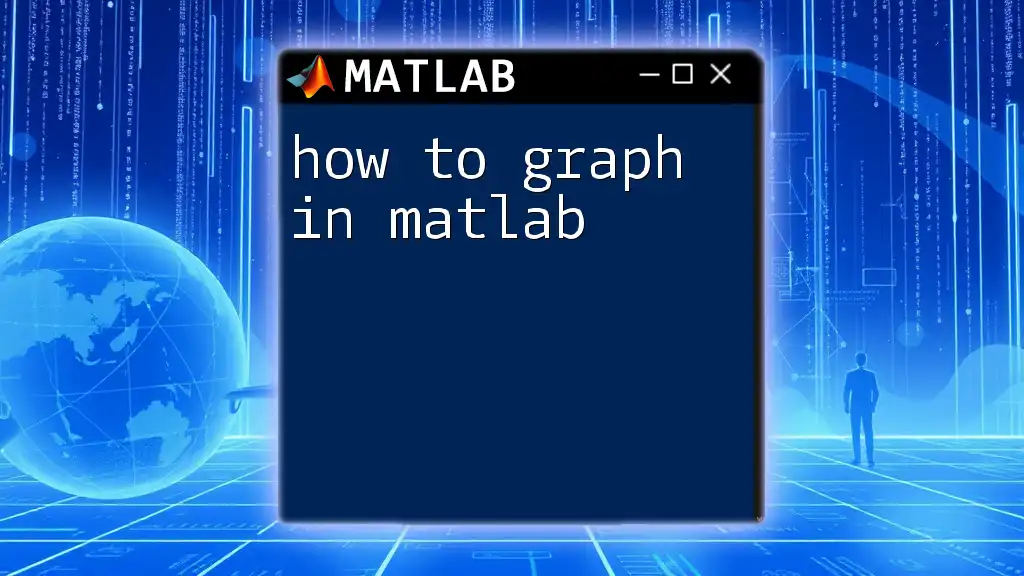
Conclusion
In this guide, you have learned how to use e in MATLAB effectively. From basic applications to advanced concepts such as Euler's formula and differential equations, the constant `e` provides a robust framework for mathematical modeling. Practice implementing these functions in your MATLAB code to enhance your understanding and mastery of the programming environment.
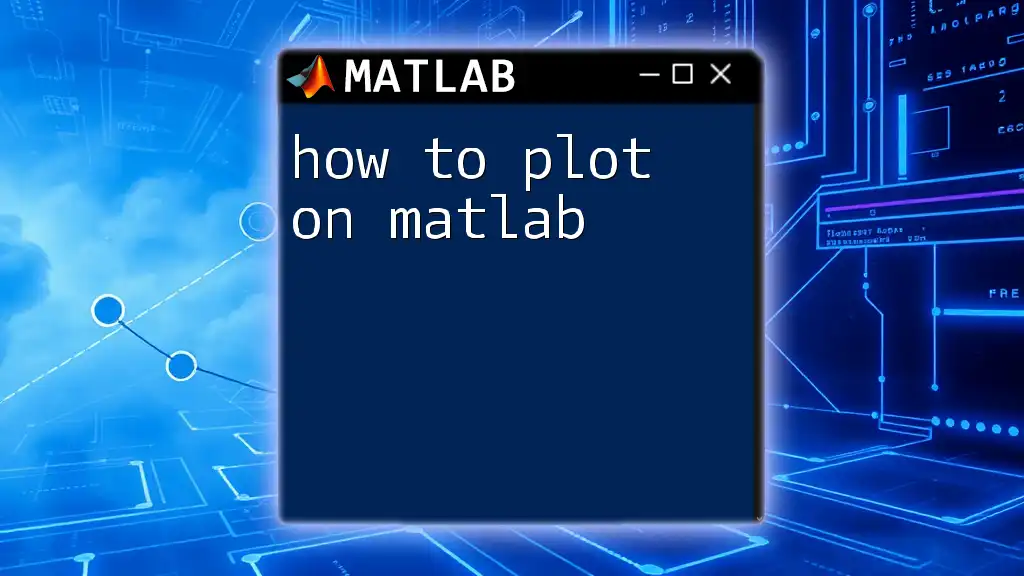
Additional Resources
For further learning, consider reviewing MATLAB’s documentation specifically on the `exp()` function and exploring online tutorials to deepen your understanding of both MATLAB usage and mathematical concepts involving `e`. Engaging with community forums can provide additional insights and support as you delve deeper into the world of MATLAB programming.