In MATLAB, you can add a title to your plot using the `title` function, which enhances the clarity of your visualizations by providing context about the displayed data.
Here’s a code snippet demonstrating how to set a title:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
title('Sine Wave Plot');
Understanding MATLAB Plot Titles
Definition of a Plot Title
A plot title in MATLAB serves as a descriptive label for your graphical representation of data. It helps to clarify what information is being displayed, thus enhancing the viewer's understanding. A well-crafted title succinctly encapsulates the essence of the plot and sets the context for interpreting the data shown.
When to Use Plot Titles
Using plot titles is essential, especially in the following scenarios:
- When presenting data: A title provides immediate context to your audience about the nature of the plot.
- In research or reports: Titles facilitate comprehension for readers who might not be familiar with the data.
However, be cautious of using overly verbose or ambiguous titles that can confuse the audience. Strive for clarity without unnecessary complexity.
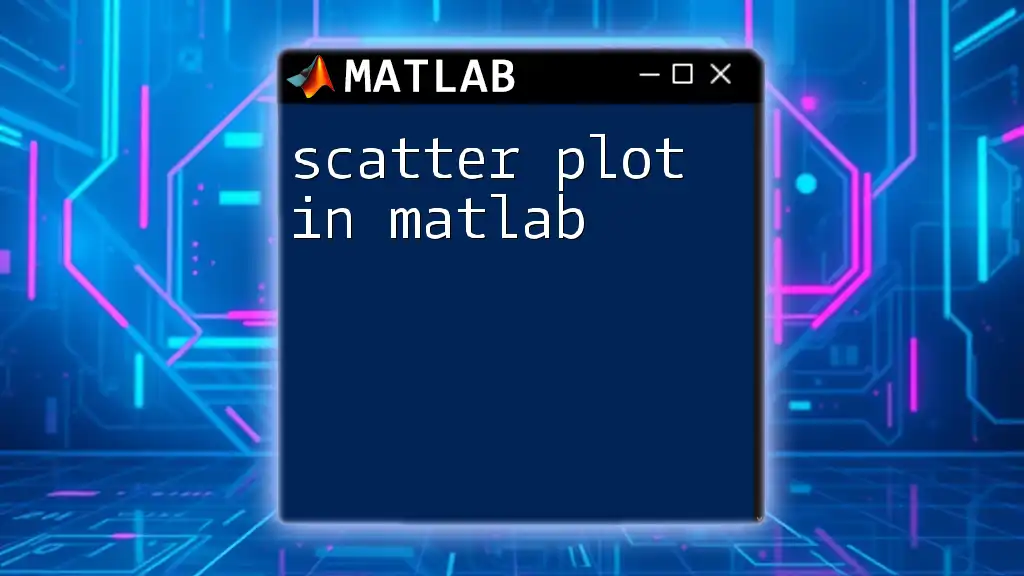
How to Add Titles in MATLAB
Basic Syntax for Adding a Title
In MATLAB, the `title` function is what you will use to add a title to your plots. Here’s the simplest way to do it:
plot(x, y);
title('My Plot Title');
When you run this command, MATLAB generates a plot based on the values in `x` and `y`, and adds “My Plot Title” at the top of the graph as the title.
Customizing Titles
Font Size and Style
You might want to adjust the appearance of your title for better visibility. The `FontSize` and `FontWeight` properties can affect how the title appears. Example:
title('My Plot Title', 'FontSize', 14, 'FontWeight', 'bold');
This command makes the title larger and bold, drawing the viewer’s attention.
Color Customization
Changing the title's color can also aid in distinguishing different data outputs. You can do this by setting the `Color` property:
title('My Plot Title', 'Color', 'red');
Here, the title will appear in red, which can help it stand out against different background colors.
Positioning the Title
Understanding Default Position
MATLAB automatically positions titles, typically centered above the plot. However, there may be scenarios where this default positioning doesn’t suit your needs.
Adjusting Title Position
If you want to move the title slightly for clarity, you can adjust its position using the `Position` property. Here’s how you can move the title upwards:
hTitle = title('My Plot Title');
hTitle.Position(2) = hTitle.Position(2) + 0.1; % move title up
This snippet shifts the title position slightly upward, allowing for better text alignment or visibility above your plot.
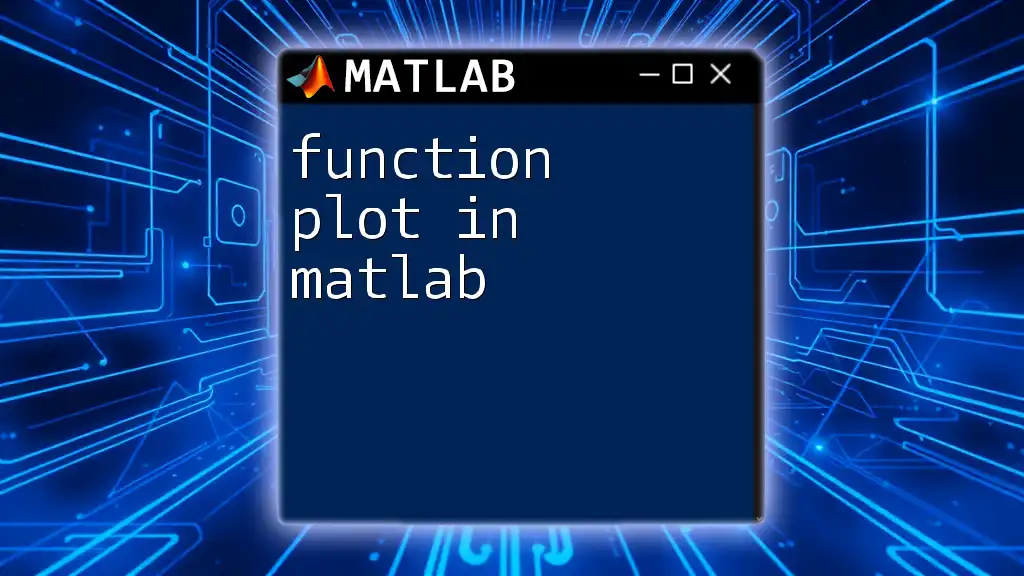
Combining Titles with Other Annotations
Adding Subtitles
In some cases, a main title may not provide all the necessary context—this is where subtitles come in. Subtitles give additional detail or classification, enhancing clarity:
subplot(2,1,1);
plot(x, y);
title('Main Plot Title');
subtitle('This is the subtitle providing more details');
Using Annotations and Text
Incorporating text annotations allows you to highlight specific data points or features on your plot. For instance:
text(0, 0, 'Sample Annotation', 'FontSize', 12);
This code will place a text annotation at the coordinates (0,0) on your plot, enhancing the informational richness of your visual.
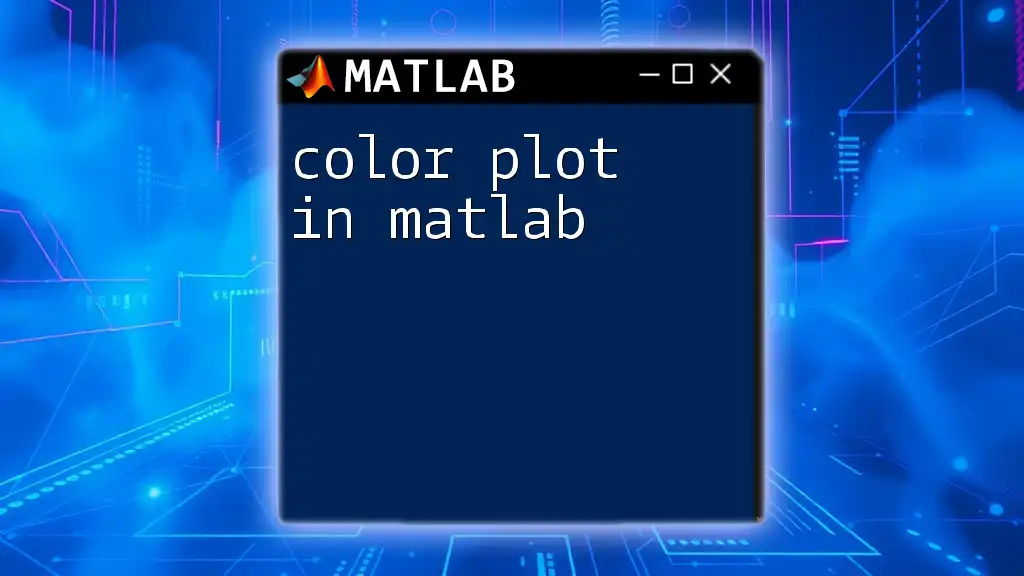
Common Mistakes and How to Avoid Them
Overly Complex Titles
Striking a balance between thoroughness and brevity in titles is vital. Lengthy and complicated titles can obscure rather than clarify. Aim for simplicity and directness—typically, a title should be informative yet concise.
Misalignment with Data
Ensure that your title accurately reflects the contents of your plot. Misleading titles can confuse viewers and undermine the integrity of your data presentation. Always review your title to confirm it aligns well with the graphical data.
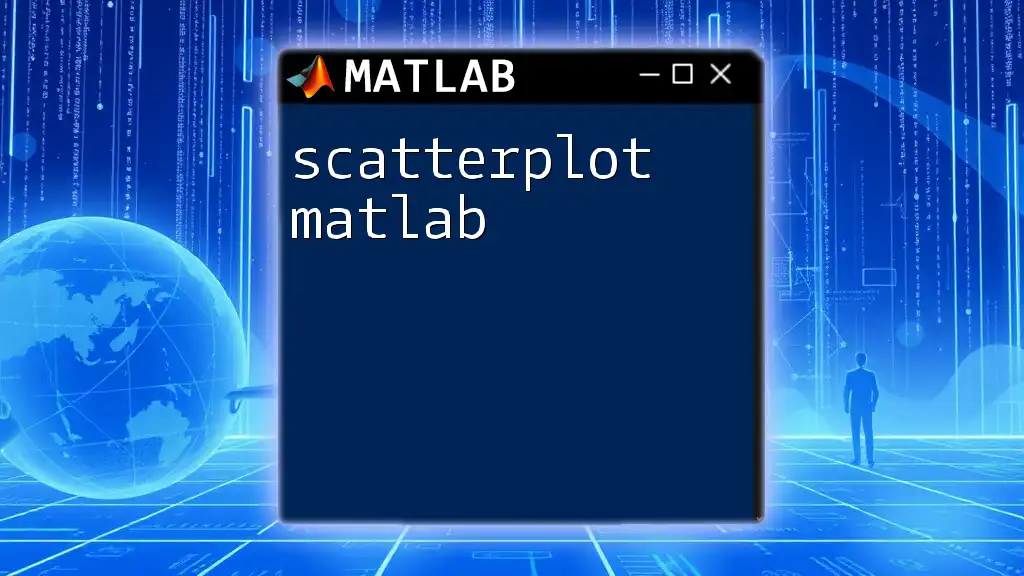
Example Scenarios
Simple Plot with a Title
Here’s a straightforward example of generating a sine wave plot with an appropriate title:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
title('Sine Wave');
This simple script effectively demonstrates how to add a title to a basic plot.
Creating a Multi-line Title
In some cases, you may need a title that contains multiple lines. You can achieve this using cell arrays:
title({'First Line of Title', 'Second Line of Title'});
This flexibility allows you to add context or clarification across multiple lines, improving readability for complex titles.
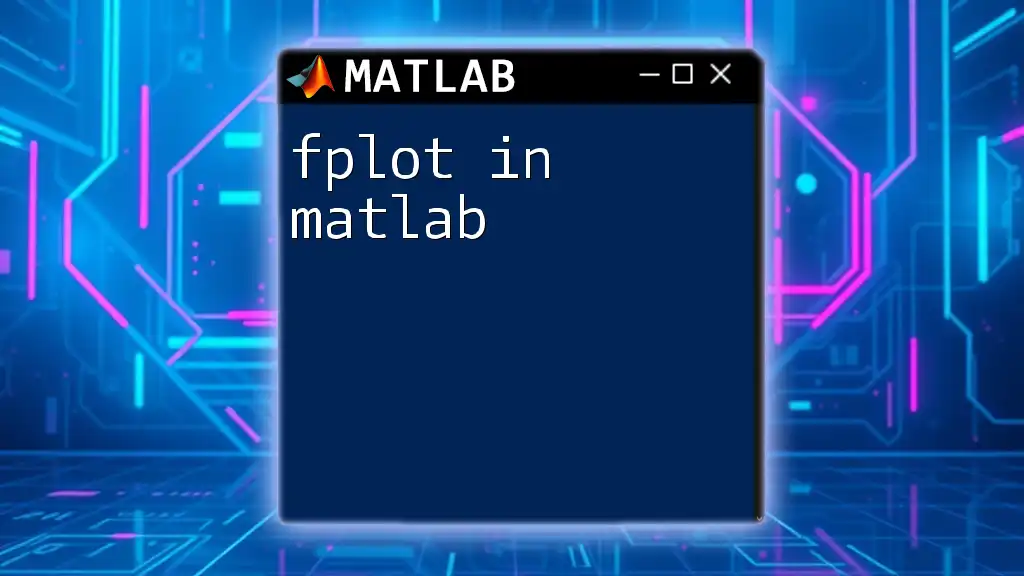
Conclusion
In summary, the title of a plot in MATLAB plays a crucial role in effective data visualization. It not only guides viewers but also enhances comprehension of the presented data. By mastering the adding and customizing of titles, you will significantly improve the quality of your presentations.
As you explore MATLAB further, do not hesitate to experiment with different styles and formats for your plot titles. This practice will refine your skills and enhance your overall data visualization literacy.
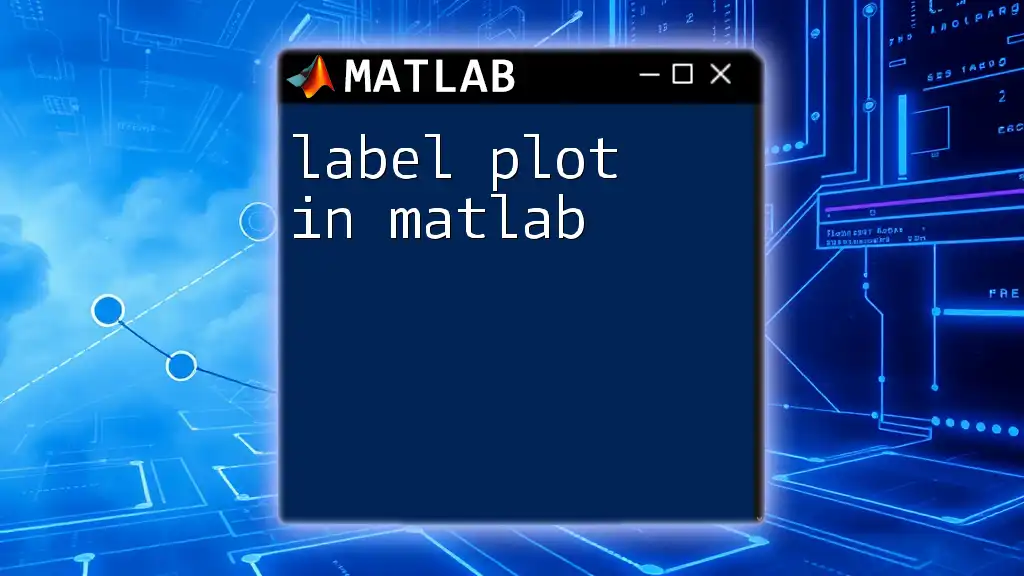
Additional Resources
For further exploration, consider reviewing the official MATLAB documentation on plotting, which provides an extensive overview of plots, their components, and styling options. Online tutorials and courses can also deepen your understanding and skill set in MATLAB plotting.
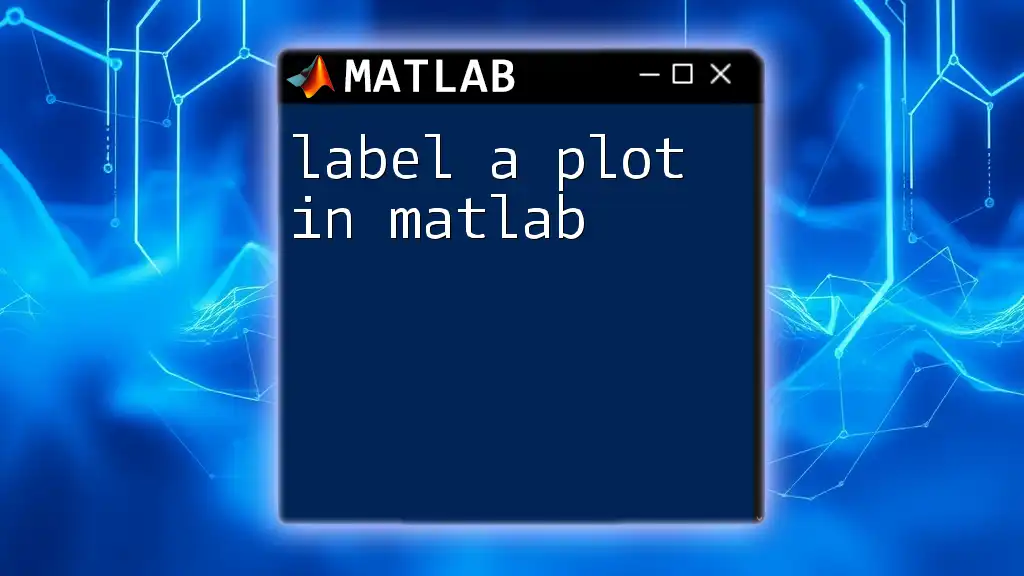
FAQs
What if my title is too long?
If your title exceeds reasonable length, consider summarizing it or split into a main title and a subtitle for clarity.
Can I include special characters in my title?
Yes, you can include special characters. If necessary, escape them according to MATLAB’s documentation, ensuring they render correctly within the title.