To effectively use MATLAB, familiarize yourself with its basic commands and syntax, which allow you to perform complex calculations and data visualizations efficiently. Here's a simple example of how to create a basic plot:
% Generate some data
x = 0:0.1:10; % Create a vector from 0 to 10 with increments of 0.1
y = sin(x); % Calculate the sine of each value in x
% Create the plot
plot(x, y); % Plot y versus x
title('Sine Wave');% Add a title
xlabel('x'); % Label the x-axis
ylabel('sin(x)'); % Label the y-axis
grid on; % Turn on the grid
Understanding the MATLAB Environment
MATLAB Interface
The MATLAB interface is designed to facilitate various tasks, making it accessible for both beginners and experienced users. Understanding its components is essential for efficient operation.
- Command Window: This is where you can type commands and see immediate results. It acts as the interactive console, allowing for quick calculations and function testing.
- Workspace: The workspace provides a list of currently defined variables. By monitoring the workspace, you can keep track of your data while running various scripts and commands.
- Command History: This window records all previously entered commands. You can easily rerun or modify commands without retyping them.
- Current Folder: This pane helps in managing files within your MATLAB directory. You can see all scripts and functions in the current folder and organize your files efficiently.
To navigate the interface effectively, you can customize the layout via the Layout option in the Environment section, which allows you to optimize your workspace according to your needs.
Getting Help in MATLAB
Learning how to use MATLAB efficiently involves using the built-in help resources effectively.
- Built-in Documentation: Use the `help` command followed by the function name to get detailed description and usage examples. For instance, typing `help plot` provides information on how to use the plot function.
- Using the MATLAB Documentation Online: MATLAB's online documentation is robust, containing tutorials, function documentation, and FAQs. It’s an invaluable resource for anyone looking to deepen their understanding or troubleshoot issues.
- Community and Forums: Engaging with the MATLAB Central community allows you to connect with other users, share insights, and find solutions to common problems. It’s a great way to learn from real-world issues addressed by other users.
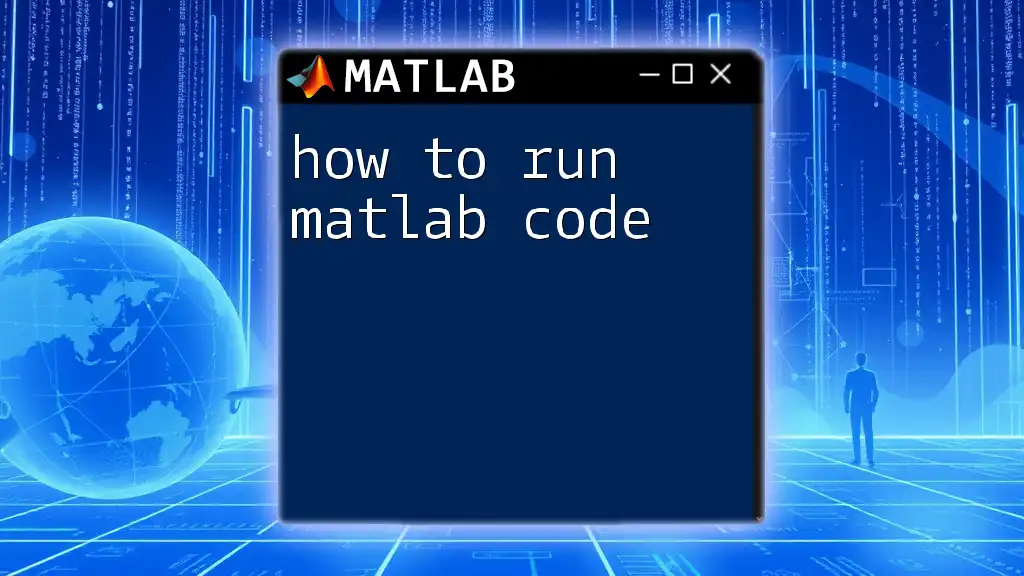
Basic MATLAB Commands
Starting with MATLAB Commands
Understanding basic commands in MATLAB is crucial for anyone interested in programming or data analysis.
- Arithmetic Operations: MATLAB excels in handling basic arithmetic. You can perform operations directly, which is intuitive for users. Here’s a simple example:
a = 5;
b = 3;
c = a + b; % Result will be 8
Variables and Data Types
Creating and managing variables is fundamental in MATLAB.
- Creating Variables: You can assign values to variables easily. However, keep in mind that variable names must start with a letter and can include letters, numbers, and underscores.
- Common Data Types: MATLAB primarily deals with several data types such as:
- Numeric: These include integers and floating-point numbers.
- Character: Strings and character arrays for text handling.
- Logical: Boolean values (true/false) for conditional operations.
- Cell Arrays: A flexible type that can contain different types of data.
Matrices and Arrays
MATLAB stands for Matrix Laboratory, highlighting its proficiency in matrix operations.
- Introduction to MATLAB's Matrix Operations: Matrices are foundational in MATLAB. You can create and manipulate them easily. Here’s how to create a simple matrix:
A = [1, 2, 3; 4, 5, 6]; % Creates a 2x3 matrix
- Basic Matrix Operations: MATLAB allows various matrix operations such as addition, subtraction, and multiplication. For example:
B = [1, 2; 3, 4];
C = A + B; % Element-wise addition (size must match)
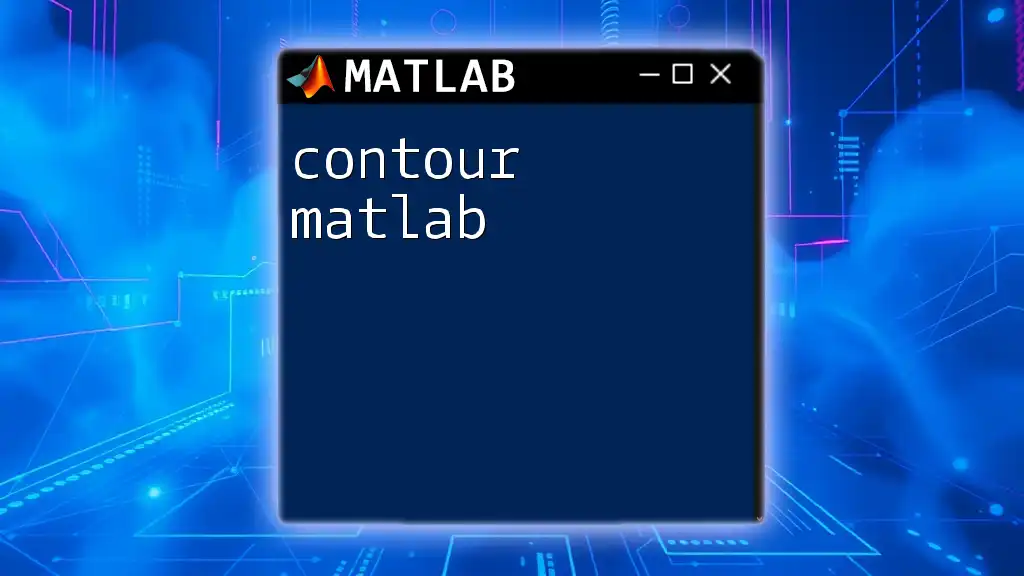
Control Structures in MATLAB
Conditional Statements
Control structures are vital for creating logical workflows.
- Using if-else Statements: The `if-else` structure allows branching logic. For instance:
x = 10;
if x > 0
disp('Positive');
else
disp('Non-positive');
end
This code checks the value of `x` and displays whether it's positive or not.
Loops in MATLAB
Loops are essential for repeating tasks efficiently.
- For Loop: The `for` loop is commonly used for iterating over a sequence. Here’s a simple example:
for i = 1:5
disp(i); % Displays numbers 1 to 5
end
- While Loop: The `while` loop continues execution as long as a condition is true. An example would be:
count = 1;
while count <= 5
disp(count);
count = count + 1;
end
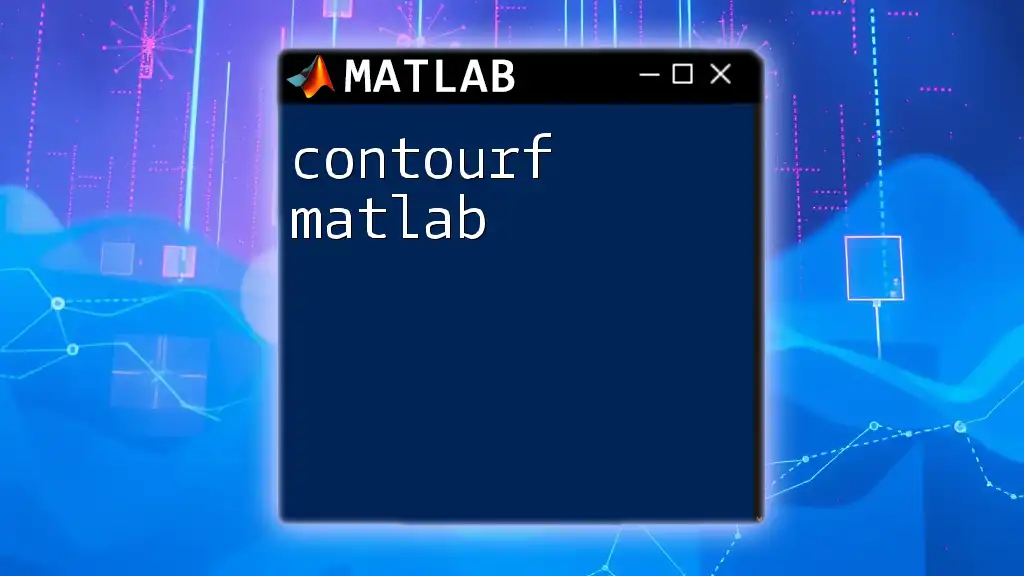
Functions and Scripts
Creating Functions
Functions in MATLAB are crucial for modular programming. They allow you to encapsulate code for reuse and can take inputs and return outputs.
- Defining a Function: To create a function, you use the `function` keyword followed by the output variable, function name, and input variables. Here’s a simple function:
function sum = addNumbers(a, b)
sum = a + b;
end
This function returns the sum of two numbers.
Writing Scripts
Scripts are collections of MATLAB commands saved in a file. A script runs in the context of the current workspace.
- Difference between Functions and Scripts: While scripts rely on existing variables, functions create their own scope. This distinction is crucial when organizing code.
- Executing a Script: To run a script, simply type the filename (without the `.m` extension) in the command window. For example, if your script is named `myscript.m`, just type `myscript`.
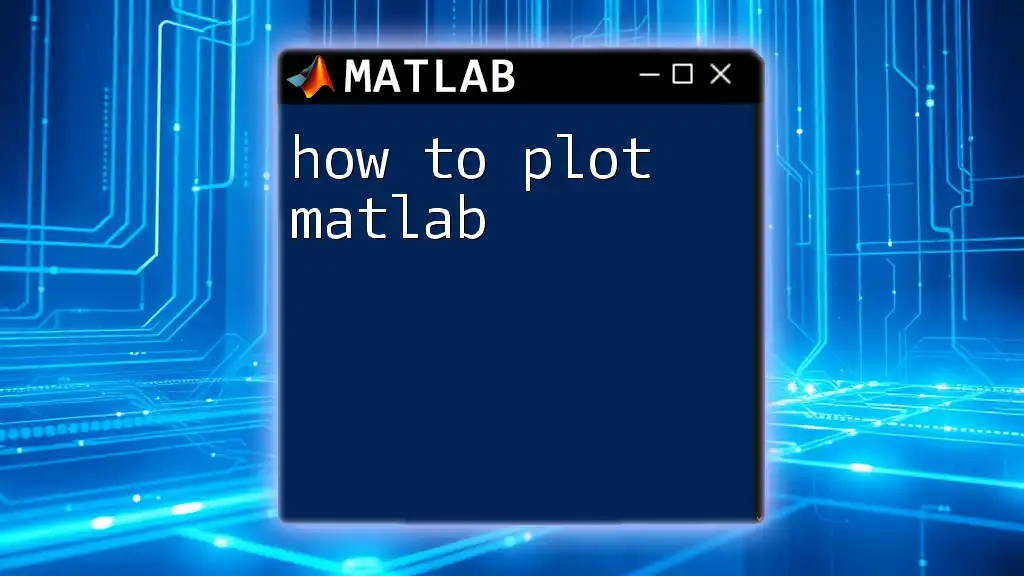
Plotting and Visualization
Basic Plotting
Visual representation of data enhances understanding.
- Creating Basic Plots: MATLAB provides straightforward plotting functionality. For instance, to plot a sine wave, you can use:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
title('Sine Wave');
xlabel('Time');
ylabel('Amplitude');
This code generates a simple 2D plot of a sine wave.
Customizing Plots
To make your plots more informative and appealing, customization is key.
- Adding Titles, Labels, and Legends: You can enhance clarity by adding titles, labels, and legends to your plots. Use the `title`, `xlabel`, and `ylabel` functions.
- Saving Plots: You can save plots to files using the `saveas` function. For instance, to save the sine wave plot:
saveas(gcf, 'sine_wave.png'); % Saves the current figure as a PNG file
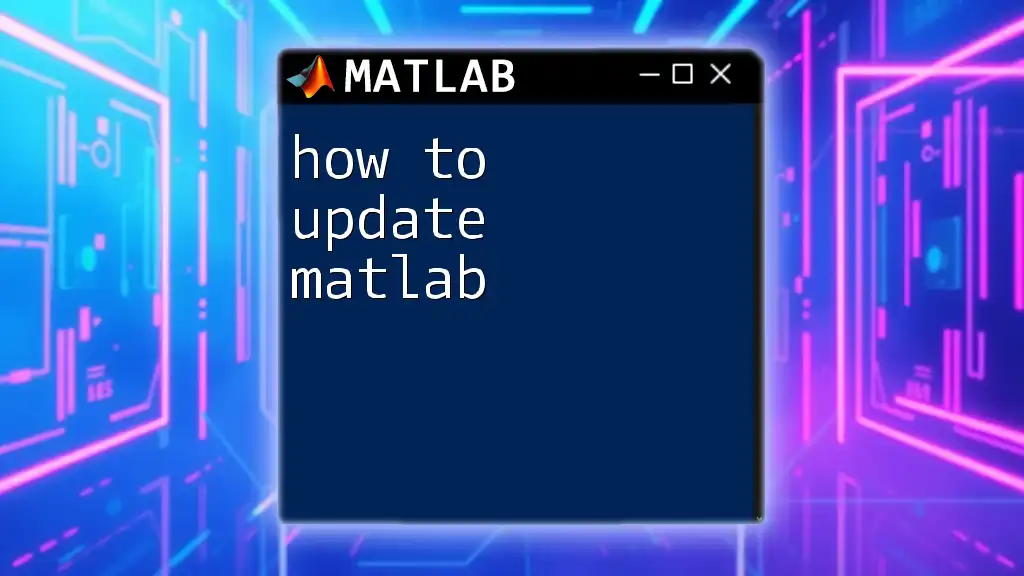
Advanced Topics
File Input and Output
Manipulating data from files is an important aspect of MATLAB programming.
- Reading Data from Files: Use `csvread` for CSV files or `readtable` for more complex data structures. For example:
data = readtable('datafile.csv'); % Loads data from a CSV file
- Writing Data to Files: Output your results with commands like `csvwrite` or `writetable`. For instance:
writetable(data, 'outputfile.csv'); % Writes data to a new CSV file
Toolboxes and Extensions
MATLAB offers a wide range of toolboxes tailored for various applications, enhancing its functionality.
- Exploring Available Toolboxes: Toolboxes include specialized functions and apps. For instance, the Signal Processing Toolbox adds powerful capabilities for handling signals.
- Example: To utilize the functionalities of a toolbox, simply install it via the Add-On Explorer and refer to its documentation for specific functions.
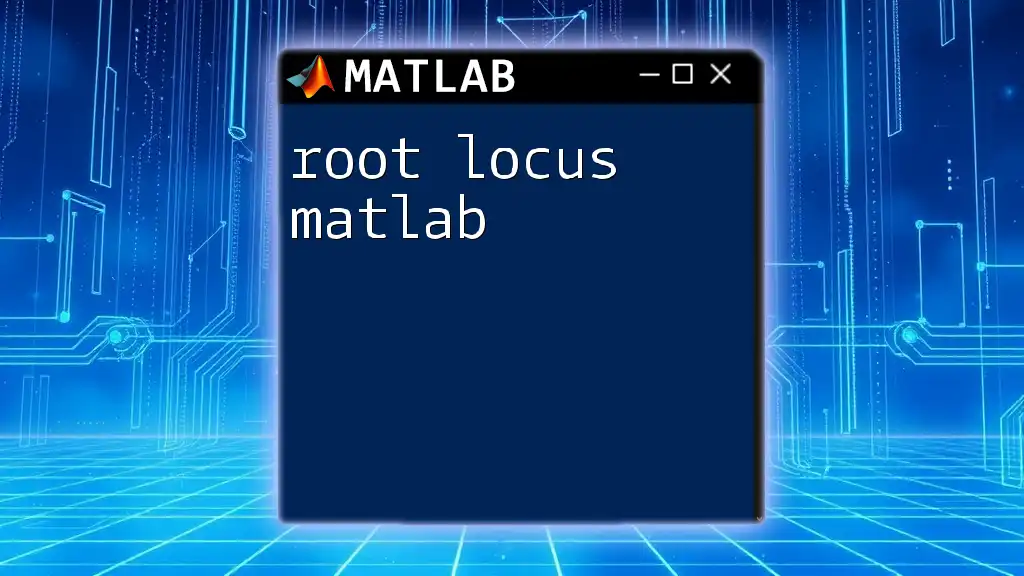
Best Practices for MATLAB Programming
Writing Efficient Code
Efficiency is paramount in programming. Focus on writing clear, concise code that adheres to best practices.
- Code Optimization Techniques: Use vectorization where possible rather than loops, as this significantly speeds up computations.
Debugging and Error Handling
Debugging is an inevitable part of programming, and MATLAB offers tools to simplify this process.
- Using the Debugger: The built-in debugger allows you to set breakpoints, inspect variables, and step through your code to find issues.
- Common Errors and How to Fix Them: Familiarize yourself with common MATLAB errors, such as dimension mismatches or undefined variables, and understand how to troubleshoot them through error messages.
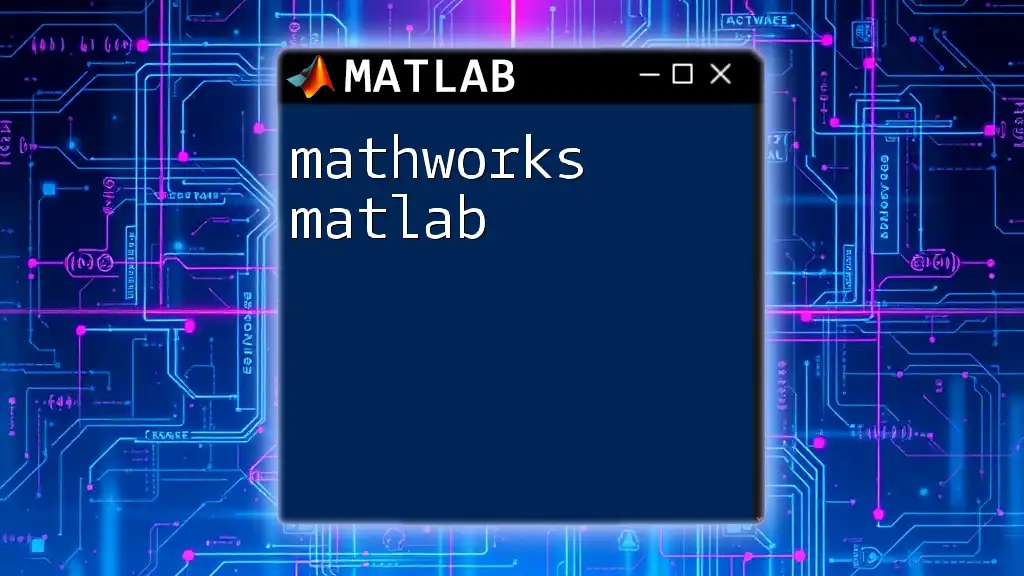
Conclusion
In this guide on how to use MATLAB, we covered a range of topics from navigating the interface to advanced programming techniques. The key to becoming proficient in MATLAB lies in practice and exploration. Start implementing these concepts, and you will find yourself mastering MATLAB in no time.
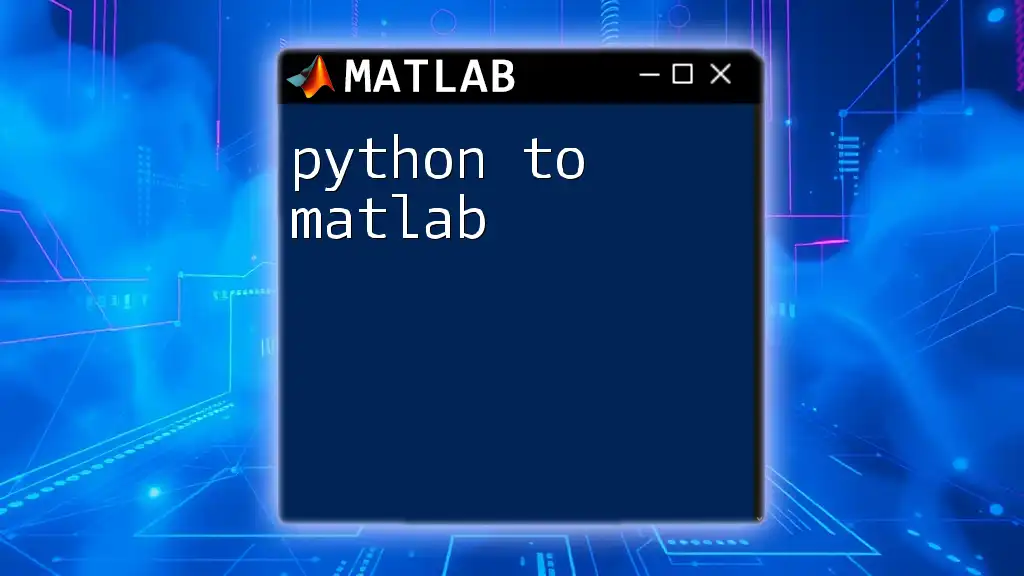
Additional Resources
- MATLAB documentation is your best friend for ongoing learning.
- Explore online courses for in-depth instruction.
- Engage with community forums to ask questions and share knowledge.
By delving into these resources and continually practicing your MATLAB skills, you'll enhance your understanding and capabilities in this powerful programming environment.